react-msa-viewer
is a performant, extendable, highly-customizable, production-ready
React Component that renders a Multiple Sequence Alignment (MSA).
WARNING: Work in progress - use with caution
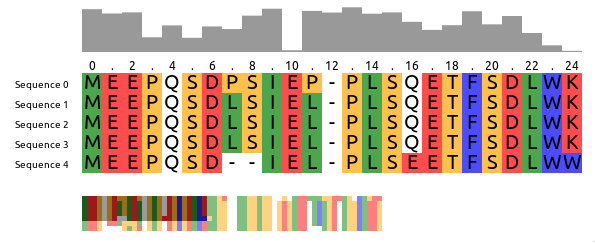
Checkout the storybook at https://msa.bio.sh
import MSAViewer from '@plotly/react-msa-viewer';
function MSA() {
const options = {
sequences: [
{
name: "seq.1",
sequence: "MEEPQSDPSIEP-PLSQETFSDLWKLLPENNVLSPLPS-QA-VDDLMLSPDDLAQWLTED"
},
{
name: "seq.2",
sequence: "MEEPQSDLSIEL-PLSQETFSDLWKLLPPNNVLSTLPS-SDSIEE-LFLSENVAGWLEDP"
},
{
name: "seq.3",
sequence: "MEEPQSDLSIEL-PLSQETFSDLWKLLPPNNVLSTLPS-SDSIEE-LFLSENVAGWLEDP"
},
],
height: 60,
colorScheme: "zappo",
};
return (
<MSAViewer {...options} />
);
}
For npm users, run:
npm i --save @plotly/react-msa-viewer
For yarn users, run:
yarn add @plotly/react-msa-viewer
<MSAViewer>
acts a Context Provider for all MSA subcomponents.
Hence, it will automatically take care of synchronization between all MSA components in its tree:
import {
Labels,
OverviewBar,
PositionBar,
SequenceOverview,
SequenceViewer,
} from '@plotly/react-msa-viewer';
function MSA() {
const options = {
sequences: [
{
name: "seq.1",
sequence: "MEEPQSDPSIEP-PLSQETFSDLWKLLPENNVLSPLPS-QA-VDDLMLSPDDLAQWLTED"
},
{
name: "seq.2",
sequence: "MEEPQSDLSIEL-PLSQETFSDLWKLLPPNNVLSTLPS-SDSIEE-LFLSENVAGWLEDP"
},
{
name: "seq.3",
sequence: "MEEPQSDLSIEL-PLSQETFSDLWKLLPPNNVLSTLPS-SDSIEE-LFLSENVAGWLEDP"
},
],
height: 60,
};
return (
<MSAViewer sequences={sequences}>
<SequenceOverview method="information-content"/>
<div style={{display: "flex"}} >
<div>
<SequenceViewer/>
<br/>
<OverviewBar/>
<PositionBar/>
</div>
<Labels/>
</div>
</MSAViewer>
);
}
Using the react-msa-viewer
with in React is highly recommended.
However, it can be used in Vanilla JS:
<html>
<meta charset="utf-8" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/react/15.4.2/react.min.js" crossorigin="anonymous"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/prop-types/15.5.2/prop-types.js"></script>
<script src="https://cdnjs.cloudflare.com/ajax/libs/react-dom/15.4.2/react-dom.min.js"></script>
<script src="https://unpkg.com/@plotly/react-msa-viewer/dist/index.js"></script>
<body>
<div id="my-msa" />
<script>
var options = {
sequences: [
{
name: "seq.1",
sequence: "MEEPQSDPSIEP-PLSQETFSDLWKLLPENNVLSPLPS-QA-VDDLMLSPDDLAQWLTED"
},
{
name: "seq.2",
sequence: "MEEPQSDLSIEL-PLSQETFSDLWKLLPPNNVLSTLPS-SDSIEE-LFLSENVAGWLEDP"
},
{
name: "seq.3",
sequence: "MEEPQSDLSIEL-PLSQETFSDLWKLLPPNNVLSTLPS-SDSIEE-LFLSENVAGWLEDP"
},
],
height: 60,
colorScheme: "zappo",
};
ReactDOM.render(
React.createElement(ReactMSAViewer.MSAViewer, options),
document.getElementById('my-msa')
);
</script>
</body>
</html>
See an example on CodePen.
Warning: these properties are still suspectible to a change at any moment.
TBD.
Displays the sequence names.
Rendering engine: canvas
or webgl
(experimental).
type: enum('canvas'|'webgl')
defaultValue: "canvas"
Font of the sequence labels, e.g. 20px Arial
type: string
Width of the component (in pixels), e.g. 100
type: number
defaultValue: 100
defaultValue: 60
Maximum number of frames per second, e.g. 1000 / 60
type: number
Custom style configuration.
type: object
Width of the component (in pixels), e.g. 100
type: number
defaultValue: 100
Creates a small overview box of the sequences for a general overview.
defaultValue: "#999999"
defaultValue: 50
defaultValue: "conservation"
Creates a PositionBar of markers for every n-th sequence column.
Rendering engine: canvas
or webgl
(experimental).
type: enum('canvas'|'webgl')
defaultValue: "canvas"
Font of the sequence labels, e.g. 20px Arial
type: string
defaultValue: "12px Arial"
Width of the component (in pixels), e.g. 100
type: number
defaultValue: 100
At which steps the position labels should appear, e.g. 2
for (1, 3, 5)
type: number
defaultValue: 2
defaultValue: 60
Maximum number of frames per second, e.g. 1000 / 60
type: number
At which number the PositionBar marker should start counting.
Typical values are: 1
(1-based indexing) and 0
(0-based indexing).
type: number
defaultValue: 1
Custom style configuration.
type: object
Width of the component (in pixels), e.g. 100
type: number
defaultValue: 100
Height of the SequenceOverview (in pixels), e.g. 50
type: number
defaultValue: 50
Height of a tile in the OverviewBar, e.g. 5
type: number
defaultValue: 5
Width of a tile in the OverviewBar, e.g. 5
type: number
defaultValue: 5
defaultValue: true
The React MSA Viewer uses an Redux store internally. You can connect your components with it too.
import React, {Component} from 'react';
import msaConnect from 'react-msa-viewer';
class MyFirstMSAPluginComponent extends Component {
render() {
return (
<div>
x: {this.props.xPos},
y: {this.props.yPos}
</div>
);
}
}
const mapStateToProps = state => {
return {
xPos: state.position.xPos,
yPos: state.position.yPos,
}
}
export default msaConnect(
mapStateToProps,
)(MyFirstMSAPluginComponent);
And then use your plugin in your App:
import {
MSAViewer,
SequenceViewer,
} from 'react-msa-viewer';
import MyFirstMSAPlugin from './MyFirstMSAPlugin'
function MyApp() {
return (
<MSAViewer sequences={sequences} height={60}>
<SequenceViewer />
<MyFirstMSA />
</MSAViewer>
);
}
Alternatively, you can also listen to events.
Get the code:
git clone https://github.com/plotly/react-msa-viewer
Install the project dev
dependencies:
yarn install
Please, see the CONTRIBUTING file.
Please note that this project is released with a Contributor Code of Conduct. By participating in this project you agree to abide by its terms. See CODE_OF_CONDUCT file.
react-msa-viewer is released under the MIT License. See the bundled LICENSE file for details.