-
-
Notifications
You must be signed in to change notification settings - Fork 1.8k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Stacked bar chart with both positive and negative values doesn't trigger tooltip when touching negative values #978
Comments
Hi. |
Sorry for late response, main.dart
chart_widget.dart
Bar Chart Groups containing positive values have tooltip as normally, but groups with only negative data doesn't show tooltip. |
I didn't use any additional dependencies to make this demo. 'sum' is method from collection package which can be imported ( |
Oh, you're right. Apologize. |
I've just fixed this problem in the |
Thank you! I was using older version of package which didn't had this problem but I'll wait next version to upgrade :) |
It has just been fixed in the 0.55.0 version. Please check it out! |
I wasn't able to check it out earlier, but it's working perfectly now. Thank you! |
I have stacked chart bar which can have both positive and negative values within BarChartRodStackItem and result chart looks like:
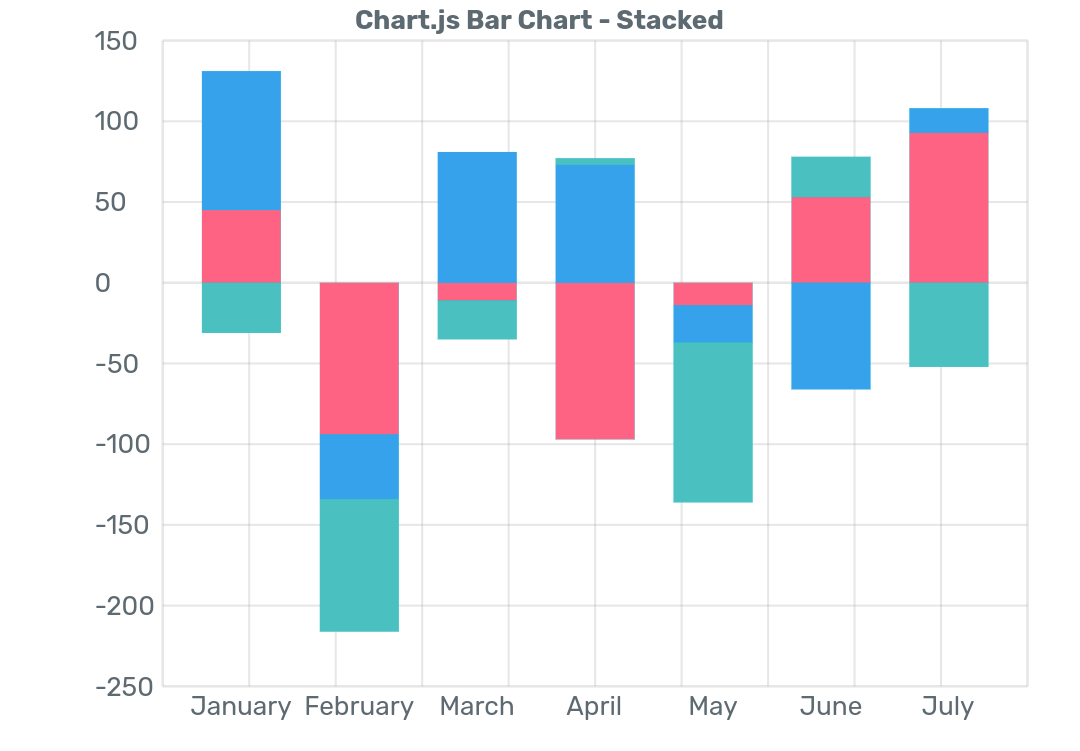
I also have tooltip which shows some detailed info:
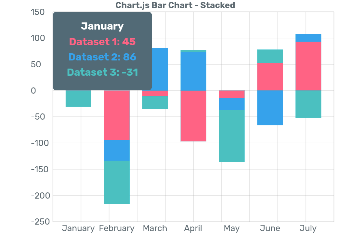
The issue I encounter is that clicking on positive values shows me tooltip, but not when I click on negative value.
With quick debugging I found out that problem lies in calculating bar Y values inside BarChartPainter class (which always calculates bar Y from 0) and replacing this piece of code (lines 520-529):
with
resolves my issue but I'm not sure if this change affects anything else.
I'm using 0.50.1 version of library.
The text was updated successfully, but these errors were encountered: