-
Notifications
You must be signed in to change notification settings - Fork 133
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Animated assign subscriber #17
Merged
Merged
Changes from 7 commits
Commits
Show all changes
8 commits
Select commit
Hold shift + click to select a range
c995de9
animated assign subscriber
icanzilb c4282e1
Update Sources/AnimatedAssignSubscriber.swift
icanzilb cd9aae1
Update Sources/AnimatedAssignSubscriber.swift
icanzilb 234d7fc
Update Sources/AnimatedAssignSubscriber.swift
icanzilb aef8160
Update Sources/AnimatedAssignSubscriber.swift
icanzilb fbbf372
Update Sources/AnimatedAssignSubscriber.swift
icanzilb 80438de
addresses Shai's feedback
icanzilb 4dcde51
addressing https://github.com/CombineCommunity/CombineCocoa/pull/17#p…
icanzilb File filter
Filter by extension
Conversations
Failed to load comments.
Loading
Jump to
Jump to file
Failed to load files.
Loading
Diff view
Diff view
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,96 @@ | ||
// | ||
// AnimatedAssignSubscriber.swift | ||
// | ||
// Created by Marin Todorov on 5/3/20. | ||
// | ||
|
||
import Foundation | ||
import Combine | ||
|
||
#if canImport(UIKit) | ||
import UIKit | ||
|
||
/// A list of animations that can be used with `Publisher.assign(to:on:animation:)` | ||
public enum AssignTransition { | ||
public enum Direction { | ||
case top, bottom, left, right | ||
} | ||
|
||
/// Flip from either bottom, top, left, or right. | ||
case flip(direction: Direction, duration: TimeInterval) | ||
|
||
/// Cross fade with previous value. | ||
case fade(duration: TimeInterval) | ||
|
||
/// A custom animation. Do not include your own code to update the target of the assign subscriber. | ||
case animation(duration: TimeInterval, options: UIView.AnimationOptions, animations: () -> Void, completion: ((Bool) -> Void)?) | ||
} | ||
|
||
extension Publisher where Self.Failure == Never { | ||
/// Behaves identically to `Publisher.assign(to:on:)` except that it allows the user to | ||
/// "wrap" emitting output in an animation transition. | ||
/// | ||
/// For example if you assign values to a `UILabel` on screen you | ||
/// can make it flip over when each new value is set: | ||
/// | ||
/// ``` | ||
/// myPublisher | ||
/// .assign(to: \.text, | ||
/// on: myLabel, | ||
/// animation: .flip(direction: .bottom, duration: 0.33)) | ||
/// ``` | ||
/// | ||
/// You may also provide a custom animation block, as follows: | ||
/// | ||
/// ``` | ||
/// myPublisher | ||
/// .assign(to: \.text, on: myLabel, animation: .animation(duration: 0.33, options: .curveEaseIn, animations: { _ in | ||
/// myLabel.center.x += 10.0 | ||
/// }, completion: nil)) | ||
/// ``` | ||
public func assign<Root: UIView>(to keyPath: ReferenceWritableKeyPath<Root, Self.Output>, on object: Root, animation: AssignTransition) -> AnyCancellable { | ||
var transition: UIView.AnimationOptions | ||
var duration: TimeInterval | ||
|
||
switch animation { | ||
case .fade(let interval): | ||
duration = interval | ||
transition = .transitionCrossDissolve | ||
case let .flip(dir, interval): | ||
duration = interval | ||
switch dir { | ||
case .bottom: transition = .transitionFlipFromBottom | ||
case .top: transition = .transitionFlipFromTop | ||
case .left: transition = .transitionFlipFromLeft | ||
case .right: transition = .transitionFlipFromRight | ||
} | ||
case let .animation(interval, options, animations, completion): | ||
// Use a custom animation. | ||
return handleEvents(receiveOutput: { value in | ||
UIView.animate(withDuration: interval, | ||
delay: 0, | ||
options: options, | ||
animations: { | ||
object[keyPath: keyPath] = value | ||
animations() | ||
}, | ||
completion: completion) | ||
}) | ||
.assign(to: keyPath, on: object) | ||
There was a problem hiding this comment. Choose a reason for hiding this commentThe reason will be displayed to describe this comment to others. Learn more. Another question that popped to my head, Maybe it's worth swapping the assign with something like |
||
} | ||
|
||
// Use one of the built-in transitions like flip or crossfade. | ||
return self | ||
.handleEvents(receiveOutput: { value in | ||
UIView.transition(with: object, | ||
duration: duration, | ||
options: transition, | ||
animations: { | ||
object[keyPath: keyPath] = value | ||
}, | ||
completion: nil) | ||
}) | ||
.assign(to: keyPath, on: object) | ||
} | ||
} | ||
#endif |
Add this suggestion to a batch that can be applied as a single commit.
This suggestion is invalid because no changes were made to the code.
Suggestions cannot be applied while the pull request is closed.
Suggestions cannot be applied while viewing a subset of changes.
Only one suggestion per line can be applied in a batch.
Add this suggestion to a batch that can be applied as a single commit.
Applying suggestions on deleted lines is not supported.
You must change the existing code in this line in order to create a valid suggestion.
Outdated suggestions cannot be applied.
This suggestion has been applied or marked resolved.
Suggestions cannot be applied from pending reviews.
Suggestions cannot be applied on multi-line comments.
Suggestions cannot be applied while the pull request is queued to merge.
Suggestion cannot be applied right now. Please check back later.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Not sure why this looks misaligned... tabs and spaces together?
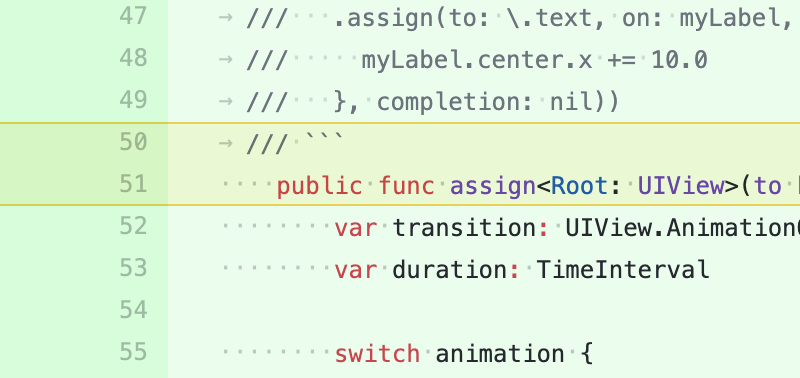