diff --git a/.gitignore b/.gitignore
index a36426cecd1..696b8d7a80d 100644
--- a/.gitignore
+++ b/.gitignore
@@ -13,6 +13,5 @@ output/
_book/
dist/
coverage.*
-docs/api.swagger.json
Dockerfile-plugin-*
plugin-*.so
\ No newline at end of file
diff --git a/.travis.yml b/.travis.yml
index 47dc8a81216..ba7f1aa68ac 100644
--- a/.travis.yml
+++ b/.travis.yml
@@ -23,7 +23,7 @@ before_install:
- sudo apt-get install curl
install:
- - go get -u github.com/go-swagger/go-swagger github.com/bradfitz/goimports github.com/mattn/goveralls golang.org/x/tools/cmd/cover github.com/Masterminds/glide github.com/mitchellh/gox github.com/ory/go-acc
+ - go get -u github.com/go-swagger/go-swagger/cmd/swagger github.com/bradfitz/goimports github.com/mattn/goveralls golang.org/x/tools/cmd/cover github.com/Masterminds/glide github.com/mitchellh/gox github.com/ory/go-acc
- git clone https://github.com/docker-library/official-images.git ~/official-images
- glide install
- go install github.com/ory/hydra
diff --git a/client/client.go b/client/client.go
index a0911fd1148..e63ca9ad111 100644
--- a/client/client.go
+++ b/client/client.go
@@ -8,7 +8,7 @@ import (
// Client represents an OAuth 2.0 Client.
//
-// swagger:model oauthClient
+// swagger:model oAuth2Client
type Client struct {
// ID is the id for this client.
ID string `json:"id" gorethink:"id"`
diff --git a/client/doc.go b/client/doc.go
index a829d519c5f..7455776aee1 100644
--- a/client/doc.go
+++ b/client/doc.go
@@ -1,14 +1,21 @@
-// Package client implements the OAuth 2.0 Client functionality and provides http handlers, http clients and storage adapters.
+// Package client implements OAuth 2.0 client management capabilities
+//
+// OAuth 2.0 clients are used to perform OAuth 2.0 and OpenID Connect flows. Usually, OAuth 2.0 clients are granted
+// to applications that want to use OAuth 2.0 access and refresh tokens.
+//
+//
+// In ORY Hydra, OAuth 2.0 clients are used to manage ORY Hydra itself. These clients may gain highly privileged access
+// if configured that way. This endpoint should be well protected and only called by code you trust.
package client
-// swagger:parameters createOAuthClient
+// swagger:parameters createOAuth2Client
type swaggerCreateClientPayload struct {
// in: body
// required: true
Body Client
}
-// swagger:parameters updateOAuthClient
+// swagger:parameters updateOAuth2Client
type swaggerUpdateClientPayload struct {
// in: path
// required: true
@@ -20,13 +27,14 @@ type swaggerUpdateClientPayload struct {
}
// A list of clients.
-// swagger:response clientsList
+// swagger:response oAuth2ClientList
type swaggerListClientsResult struct {
// in: body
+ // type: array
Body []Client
}
-// swagger:parameters getOAuthClient deleteOAuthClient
+// swagger:parameters getOAuth2Client deleteOAuth2Client
type swaggerQueryClientPayload struct {
// The id of the OAuth 2.0 Client.
//
diff --git a/client/handler.go b/client/handler.go
index bae500842c9..7efd28d4d76 100644
--- a/client/handler.go
+++ b/client/handler.go
@@ -37,12 +37,14 @@ func (h *Handler) SetRoutes(r *httprouter.Router) {
r.DELETE(ClientsHandlerPath+"/:id", h.Delete)
}
-// swagger:route POST /clients oauth2 clients createOAuthClient
+// swagger:route POST /clients oAuth2 createOAuth2Client
//
-// Creates an OAuth 2.0 Client
+// Create an OAuth 2.0 client
+//
+// If you pass `client_secret` the secret will be used, otherwise a random secret will be generated. The secret will
+// be returned in the response and you will not be able to retrieve it later on. Write the secret down and keep
+// it somwhere safe.
//
-// Be aware that an OAuth 2.0 Client may gain highly priviledged access if configured that way. This
-// endpoint should be well protected and only called by code you trust.
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -77,7 +79,7 @@ func (h *Handler) SetRoutes(r *httprouter.Router) {
// oauth2: hydra.clients
//
// Responses:
-// 200: oauthClient
+// 200: oAuth2Client
// 401: genericError
// 403: genericError
// 500: genericError
@@ -122,12 +124,13 @@ func (h *Handler) Create(w http.ResponseWriter, r *http.Request, _ httprouter.Pa
h.H.WriteCreated(w, r, ClientsHandlerPath+"/"+c.GetID(), &c)
}
-// swagger:route PUT /clients/{id} oauth2 clients updateOAuthClient
+// swagger:route PUT /clients/{id} oAuth2 updateOAuth2Client
+//
+// Update an OAuth 2.0 Client
//
-// Updates an OAuth 2.0 Client
+// If you pass `client_secret` the secret will be updated and returned via the API. This is the only time you will
+// be able to retrieve the client secret, so write it down and keep it safe.
//
-// Be aware that an OAuth 2.0 Client may gain highly priviledged access if configured that way. This
-// endpoint should be well protected and only called by code you trust.
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -162,7 +165,7 @@ func (h *Handler) Create(w http.ResponseWriter, r *http.Request, _ httprouter.Pa
// oauth2: hydra.clients
//
// Responses:
-// 200: oauthClient
+// 200: oAuth2Client
// 401: genericError
// 403: genericError
// 500: genericError
@@ -192,8 +195,12 @@ func (h *Handler) Update(w http.ResponseWriter, r *http.Request, ps httprouter.P
return
}
+ var secret string
if len(c.Secret) > 0 && len(c.Secret) < 6 {
h.H.WriteError(w, r, errors.New("The client secret must be at least 6 characters long"))
+ return
+ } else {
+ secret = c.Secret
}
c.ID = ps.ByName("id")
@@ -202,14 +209,16 @@ func (h *Handler) Update(w http.ResponseWriter, r *http.Request, ps httprouter.P
return
}
+ c.Secret = secret
h.H.WriteCreated(w, r, ClientsHandlerPath+"/"+c.GetID(), &c)
}
-// swagger:route GET /clients oauth2 clients listOAuthClients
+// swagger:route GET /clients oAuth2 listOAuth2Clients
//
-// Lists OAuth 2.0 Clients
+// List OAuth 2.0 Clients
+//
+// This endpoint never returns passwords.
//
-// Never returns a client's secret.
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -233,7 +242,7 @@ func (h *Handler) Update(w http.ResponseWriter, r *http.Request, ps httprouter.P
// oauth2: hydra.clients
//
// Responses:
-// 200: clientsList
+// 200: oAuth2ClientList
// 401: genericError
// 403: genericError
// 500: genericError
@@ -254,19 +263,23 @@ func (h *Handler) List(w http.ResponseWriter, r *http.Request, ps httprouter.Par
return
}
- for k, cc := range c {
- cc.Secret = ""
- c[k] = cc
+ clients := make([]Client, len(c))
+ k := 0
+ for _, cc := range c {
+ clients[k] = cc
+ clients[k].Secret = ""
+ k++
}
- h.H.Write(w, r, c)
+ h.H.Write(w, r, clients)
}
-// swagger:route GET /clients/{id} oauth2 clients getOAuthClient
+// swagger:route GET /clients/{id} oAuth2 getOAuth2Client
+//
+// Retrieve an OAuth 2.0 Client.
//
-// Fetches an OAuth 2.0 Client.
+// This endpoint never returns passwords.
//
-// Never returns the client's secret.
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -301,7 +314,7 @@ func (h *Handler) List(w http.ResponseWriter, r *http.Request, ps httprouter.Par
// oauth2: hydra.clients
//
// Responses:
-// 200: oauthClient
+// 200: oAuth2Client
// 401: genericError
// 403: genericError
// 500: genericError
@@ -330,7 +343,7 @@ func (h *Handler) Get(w http.ResponseWriter, r *http.Request, ps httprouter.Para
h.H.Write(w, r, c)
}
-// swagger:route DELETE /clients/{id} oauth2 clients deleteOAuthClient
+// swagger:route DELETE /clients/{id} oAuth2 deleteOAuth2Client
//
// Deletes an OAuth 2.0 Client
//
diff --git a/client/manager_http.go b/client/manager_http.go
deleted file mode 100644
index e22d322c9b6..00000000000
--- a/client/manager_http.go
+++ /dev/null
@@ -1,74 +0,0 @@
-package client
-
-import (
- "net/http"
- "net/url"
-
- "context"
-
- "github.com/ory/fosite"
- "github.com/ory/hydra/pkg"
- "github.com/pkg/errors"
-)
-
-type HTTPManager struct {
- Client *http.Client
- Endpoint *url.URL
- Dry bool
- FakeTLSTermination bool
-}
-
-func (m *HTTPManager) GetConcreteClient(id string) (*Client, error) {
- var c Client
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
-
- if err := r.Get(&c); err != nil {
- return nil, errors.WithStack(err)
- }
-
- return &c, nil
-}
-
-func (m *HTTPManager) GetClient(_ context.Context, id string) (fosite.Client, error) {
- return m.GetConcreteClient(id)
-}
-
-func (m *HTTPManager) UpdateClient(c *Client) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, c.ID).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Update(c)
-}
-
-func (m *HTTPManager) CreateClient(c *Client) error {
- var r = pkg.NewSuperAgent(m.Endpoint.String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Create(c)
-}
-
-func (m *HTTPManager) DeleteClient(id string) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Delete()
-}
-
-func (m *HTTPManager) GetClients() (map[string]Client, error) {
- cs := make(map[string]Client)
- var r = pkg.NewSuperAgent(m.Endpoint.String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- if err := r.Get(&cs); err != nil {
- return nil, errors.WithStack(err)
- }
-
- return cs, nil
-}
diff --git a/client/manager_memory.go b/client/manager_memory.go
index aeb81de6530..378047514b6 100644
--- a/client/manager_memory.go
+++ b/client/manager_memory.go
@@ -1,9 +1,8 @@
package client
import (
- "sync"
-
"context"
+ "sync"
"github.com/imdario/mergo"
"github.com/ory/fosite"
@@ -18,6 +17,17 @@ type MemoryManager struct {
sync.RWMutex
}
+func NewMemoryManager(hasher fosite.Hasher) *MemoryManager {
+ if hasher == nil {
+ hasher = new(fosite.BCrypt)
+ }
+
+ return &MemoryManager{
+ Clients: map[string]Client{},
+ Hasher: hasher,
+ }
+}
+
func (m *MemoryManager) GetConcreteClient(id string) (*Client, error) {
m.RLock()
defer m.RUnlock()
diff --git a/client/manager_test.go b/client/manager_test.go
index 2fe850e17df..911629d3700 100644
--- a/client/manager_test.go
+++ b/client/manager_test.go
@@ -4,56 +4,21 @@ import (
"flag"
"fmt"
"log"
- "net/http/httptest"
- "net/url"
"os"
"testing"
- "github.com/julienschmidt/httprouter"
"github.com/ory/fosite"
- "github.com/ory/herodot"
. "github.com/ory/hydra/client"
- "github.com/ory/hydra/compose"
"github.com/ory/hydra/integration"
- "github.com/ory/ladon"
)
-var clientManagers = map[string]Storage{}
-
-var ts *httptest.Server
+var clientManagers = map[string]Manager{}
func init() {
clientManagers["memory"] = &MemoryManager{
Clients: map[string]Client{},
Hasher: &fosite.BCrypt{},
}
-
- localWarden, httpClient := compose.NewMockFirewall("foo", "alice", fosite.Arguments{Scope}, &ladon.DefaultPolicy{
- ID: "1",
- Subjects: []string{"alice"},
- Resources: []string{"rn:hydra:clients<.*>"},
- Actions: []string{"create", "get", "delete", "update"},
- Effect: ladon.AllowAccess,
- })
-
- s := &Handler{
- Manager: &MemoryManager{
- Clients: map[string]Client{},
- Hasher: &fosite.BCrypt{},
- },
- H: herodot.NewJSONWriter(nil),
- W: localWarden,
- }
-
- routing := httprouter.New()
- s.SetRoutes(routing)
- ts = httptest.NewServer(routing)
-
- u, _ := url.Parse(ts.URL + ClientsHandlerPath)
- clientManagers["http"] = &HTTPManager{
- Client: httpClient,
- Endpoint: u,
- }
}
func TestMain(m *testing.M) {
@@ -89,23 +54,20 @@ func connectToPG() {
clientManagers["postgres"] = s
}
-func TestClientAutoGenerateKey(t *testing.T) {
+func TestCreateGetDeleteClient(t *testing.T) {
for k, m := range clientManagers {
- t.Run(fmt.Sprintf("case=%s", k), TestHelperClientAutoGenerateKey(k, m))
+ t.Run(fmt.Sprintf("case=%s", k), TestHelperCreateGetDeleteClient(k, m))
}
}
-func TestAuthenticateClient(t *testing.T) {
- var mem = &MemoryManager{
- Clients: map[string]Client{},
- Hasher: &fosite.BCrypt{},
+func TestClientAutoGenerateKey(t *testing.T) {
+ for k, m := range clientManagers {
+ t.Run(fmt.Sprintf("case=%s", k), TestHelperClientAutoGenerateKey(k, m))
}
-
- TestHelperClientAuthenticate("", mem)(t)
}
-func TestCreateGetDeleteClient(t *testing.T) {
+func TestAuthenticateClient(t *testing.T) {
for k, m := range clientManagers {
- t.Run(fmt.Sprintf("case=%s", k), TestHelperCreateGetDeleteClient(k, m))
+ t.Run(fmt.Sprintf("case=%s", k), TestHelperClientAuthenticate(k, m))
}
}
diff --git a/client/sdk_test.go b/client/sdk_test.go
new file mode 100644
index 00000000000..08a61eaebdf
--- /dev/null
+++ b/client/sdk_test.go
@@ -0,0 +1,95 @@
+package client_test
+
+import (
+ "net/http"
+ "net/http/httptest"
+ "testing"
+
+ "github.com/julienschmidt/httprouter"
+ "github.com/ory/fosite"
+ "github.com/ory/herodot"
+ "github.com/ory/hydra/client"
+ "github.com/ory/hydra/compose"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
+ "github.com/ory/ladon"
+ "github.com/stretchr/testify/assert"
+ "github.com/stretchr/testify/require"
+)
+
+func createTestClient(prefix string) hydra.OAuth2Client {
+ return hydra.OAuth2Client{
+ Id: "1234",
+ ClientName: prefix + "name",
+ ClientSecret: prefix + "secret",
+ ClientUri: prefix + "uri",
+ Contacts: []string{prefix + "peter", prefix + "pan"},
+ GrantTypes: []string{prefix + "client_credentials", prefix + "authorize_code"},
+ LogoUri: prefix + "logo",
+ Owner: prefix + "an-owner",
+ PolicyUri: prefix + "policy-uri",
+ Scope: prefix + "foo bar baz",
+ TosUri: prefix + "tos-uri",
+ ResponseTypes: []string{prefix + "id_token", prefix + "code"},
+ RedirectUris: []string{prefix + "redirect-url", prefix + "redirect-uri"},
+ }
+}
+
+func TestClientSDK(t *testing.T) {
+ manager := client.NewMemoryManager(nil)
+
+ localWarden, httpClient := compose.NewMockFirewall("foo", "alice", fosite.Arguments{client.Scope}, &ladon.DefaultPolicy{
+ ID: "1",
+ Subjects: []string{"alice"},
+ Resources: []string{"rn:hydra:clients<.*>"},
+ Actions: []string{"create", "get", "delete", "update"},
+ Effect: ladon.AllowAccess,
+ })
+
+ handler := &client.Handler{
+ Manager: manager,
+ H: herodot.NewJSONWriter(nil),
+ W: localWarden,
+ }
+
+ router := httprouter.New()
+ handler.SetRoutes(router)
+ server := httptest.NewServer(router)
+ c := hydra.NewOAuth2ApiWithBasePath(server.URL)
+ c.Configuration.Transport = httpClient.Transport
+
+ t.Run("foo", func(t *testing.T) {
+ createClient := createTestClient("")
+
+ result, _, err := c.CreateOAuth2Client(createClient)
+ require.NoError(t, err)
+ assert.EqualValues(t, createClient, *result)
+
+ compareClient := createClient
+ compareClient.ClientSecret = ""
+ result, _, err = c.GetOAuth2Client(createClient.Id)
+ assert.EqualValues(t, compareClient, *result)
+
+ results, _, err := c.ListOAuth2Clients()
+ require.NoError(t, err)
+ assert.Len(t, results, 1)
+ assert.EqualValues(t, compareClient, results[0])
+
+ updateClient := createTestClient("foo")
+ result, _, err = c.UpdateOAuth2Client(createClient.Id, updateClient)
+ require.NoError(t, err)
+ assert.EqualValues(t, updateClient, *result)
+
+ compareClient = updateClient
+ compareClient.ClientSecret = ""
+ result, _, err = c.GetOAuth2Client(updateClient.Id)
+ require.NoError(t, err)
+ assert.EqualValues(t, compareClient, *result)
+
+ _, err = c.DeleteOAuth2Client(updateClient.Id)
+ require.NoError(t, err)
+
+ _, response, err := c.GetOAuth2Client(updateClient.Id)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNotFound, response.StatusCode)
+ })
+}
diff --git a/cmd/cli/handler.go b/cmd/cli/handler.go
index f1905af5692..edfdd5aa73c 100644
--- a/cmd/cli/handler.go
+++ b/cmd/cli/handler.go
@@ -8,7 +8,7 @@ type Handler struct {
Clients *ClientHandler
Policies *PolicyHandler
Keys *JWKHandler
- Warden *WardenHandler
+ Warden *IntrospectionHandler
Revocation *RevocationHandler
Groups *GroupHandler
Migration *MigrateHandler
@@ -19,7 +19,7 @@ func NewHandler(c *config.Config) *Handler {
Clients: newClientHandler(c),
Policies: newPolicyHandler(c),
Keys: newJWKHandler(c),
- Warden: newWardenHandler(c),
+ Warden: newIntrospectionHandler(c),
Revocation: newRevocationHandler(c),
Groups: newGroupHandler(c),
Migration: newMigrateHandler(c),
diff --git a/cmd/cli/handler_client.go b/cmd/cli/handler_client.go
index ab911d1e62f..700037148cd 100644
--- a/cmd/cli/handler_client.go
+++ b/cmd/cli/handler_client.go
@@ -6,11 +6,11 @@ import (
"os"
"strings"
- "context"
+ "net/http"
- "github.com/ory/hydra/client"
"github.com/ory/hydra/config"
"github.com/ory/hydra/pkg"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/spf13/cobra"
)
@@ -24,16 +24,15 @@ func newClientHandler(c *config.Config) *ClientHandler {
}
}
-func (h *ClientHandler) newClientManager(cmd *cobra.Command) *client.HTTPManager {
- dry, _ := cmd.Flags().GetBool("dry")
- term, _ := cmd.Flags().GetBool("fake-tls-termination")
+func (h *ClientHandler) newClientManager(cmd *cobra.Command) *hydra.OAuth2Api {
+ c := hydra.NewOAuth2ApiWithBasePath(h.Config.ClusterURL)
+ c.Configuration.Transport = h.Config.OAuth2Client(cmd).Transport
- return &client.HTTPManager{
- Dry: dry,
- Endpoint: h.Config.Resolve("/clients"),
- Client: h.Config.OAuth2Client(cmd),
- FakeTLSTermination: term,
+ if term, _ := cmd.Flags().GetBool("fake-tls-termination"); term {
+ c.Configuration.DefaultHeader["X-Forwarded-Proto"] = "https"
}
+
+ return c
}
func (h *ClientHandler) ImportClients(cmd *cobra.Command, args []string) {
@@ -47,17 +46,13 @@ func (h *ClientHandler) ImportClients(cmd *cobra.Command, args []string) {
for _, path := range args {
reader, err := os.Open(path)
pkg.Must(err, "Could not open file %s: %s", path, err)
- var c client.Client
+ var c hydra.OAuth2Client
err = json.NewDecoder(reader).Decode(&c)
pkg.Must(err, "Could not parse JSON: %s", err)
- err = m.CreateClient(&c)
- if m.Dry {
- fmt.Printf("%s\n", err)
- continue
- }
- pkg.Must(err, "Could not create client: %s", err)
- fmt.Printf("Imported client %s:%s from %s.\n", c.ID, c.Secret, path)
+ result, response, err := m.CreateOAuth2Client(c)
+ checkResponse(response, err, http.StatusCreated)
+ fmt.Printf("Imported OAuth2 client %s:%s from %s.\n", result.Id, result.ClientSecret, path)
}
}
@@ -82,25 +77,22 @@ func (h *ClientHandler) CreateClient(cmd *cobra.Command, args []string) {
fmt.Println("You should not provide secrets using command line flags. The secret might leak to bash history and similar systems.")
}
- cc := &client.Client{
- ID: id,
- Secret: secret,
+ cc := hydra.OAuth2Client{
+ Id: id,
+ ClientSecret: secret,
ResponseTypes: responseTypes,
Scope: strings.Join(allowedScopes, " "),
GrantTypes: grantTypes,
- RedirectURIs: callbacks,
- Name: name,
+ RedirectUris: callbacks,
+ ClientName: name,
Public: public,
}
- err = m.CreateClient(cc)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not create client: %s", err)
- fmt.Printf("Client ID: %s\n", cc.ID)
- fmt.Printf("Client Secret: %s\n", secret)
+ result, response, err := m.CreateOAuth2Client(cc)
+ checkResponse(response, err, http.StatusCreated)
+
+ fmt.Printf("OAuth2 client id: %s\n", result.Id)
+ fmt.Printf("OAuth2 client secret: %s\n", result.ClientSecret)
}
func (h *ClientHandler) DeleteClient(cmd *cobra.Command, args []string) {
@@ -112,15 +104,11 @@ func (h *ClientHandler) DeleteClient(cmd *cobra.Command, args []string) {
}
for _, c := range args {
- err := m.DeleteClient(c)
- if m.Dry {
- fmt.Printf("%s\n", err)
- continue
- }
- pkg.Must(err, "Could not delete client: %s", err)
+ response, err := m.DeleteOAuth2Client(c)
+ checkResponse(response, err, http.StatusNoContent)
}
- fmt.Println("Client(s) deleted.")
+ fmt.Println("OAuth2 client(s) deleted.")
}
func (h *ClientHandler) GetClient(cmd *cobra.Command, args []string) {
@@ -131,15 +119,7 @@ func (h *ClientHandler) GetClient(cmd *cobra.Command, args []string) {
return
}
- cl, err := m.GetClient(context.Background(), args[0])
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not delete client: %s", err)
-
- out, err := json.MarshalIndent(cl, "", "\t")
- pkg.Must(err, "Could not convert client to JSON: %s", err)
-
- fmt.Printf("%s\n", out)
+ cl, response, err := m.GetOAuth2Client(args[0])
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("%s\n", formatResponse(cl))
}
diff --git a/cmd/cli/handler_groups.go b/cmd/cli/handler_groups.go
index 7cf44e8c2ee..663636ba0f6 100644
--- a/cmd/cli/handler_groups.go
+++ b/cmd/cli/handler_groups.go
@@ -3,9 +3,10 @@ package cli
import (
"fmt"
+ "net/http"
+
"github.com/ory/hydra/config"
- "github.com/ory/hydra/pkg"
- "github.com/ory/hydra/warden/group"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/spf13/cobra"
)
@@ -13,16 +14,14 @@ type GroupHandler struct {
Config *config.Config
}
-func (h *GroupHandler) newGroupManager(cmd *cobra.Command) *group.HTTPManager {
- dry, _ := cmd.Flags().GetBool("dry")
- term, _ := cmd.Flags().GetBool("fake-tls-termination")
-
- return &group.HTTPManager{
- Dry: dry,
- Endpoint: h.Config.Resolve("/warden/groups"),
- Client: h.Config.OAuth2Client(cmd),
- FakeTLSTermination: term,
+func (h *GroupHandler) newGroupManager(cmd *cobra.Command) *hydra.WardenApi {
+ client := hydra.NewWardenApiWithBasePath(h.Config.ClusterURL)
+ client.Configuration.Transport = h.Config.OAuth2Client(cmd).Transport
+ if term, _ := cmd.Flags().GetBool("fake-tls-termination"); term {
+ client.Configuration.DefaultHeader["X-Forwarded-Proto"] = "https"
}
+
+ return client
}
func newGroupHandler(c *config.Config) *GroupHandler {
@@ -38,16 +37,9 @@ func (h *GroupHandler) CreateGroup(cmd *cobra.Command, args []string) {
}
m := h.newGroupManager(cmd)
- var err error
- cc := &group.Group{ID: args[0]}
- err = m.CreateGroup(cc)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
-
- pkg.Must(err, "Could not create group: %s", err)
- fmt.Printf("Group %s created.\n", cc.ID)
+ _, response, err := m.CreateGroup(hydra.Group{Id: args[0]})
+ checkResponse(response, err, http.StatusCreated)
+ fmt.Printf("Group %s created.\n", args[0])
}
func (h *GroupHandler) DeleteGroup(cmd *cobra.Command, args []string) {
@@ -55,16 +47,10 @@ func (h *GroupHandler) DeleteGroup(cmd *cobra.Command, args []string) {
fmt.Print(cmd.UsageString())
return
}
- m := h.newGroupManager(cmd)
- var err error
- err = m.DeleteGroup(args[0])
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
-
- pkg.Must(err, "Could not create group: %s", err)
+ m := h.newGroupManager(cmd)
+ response, err := m.DeleteGroup(args[0])
+ checkResponse(response, err, http.StatusNoContent)
fmt.Printf("Group %s deleted.\n", args[0])
}
@@ -73,16 +59,10 @@ func (h *GroupHandler) AddMembers(cmd *cobra.Command, args []string) {
fmt.Print(cmd.UsageString())
return
}
- m := h.newGroupManager(cmd)
- var err error
- err = m.AddGroupMembers(args[0], args[1:])
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
-
- pkg.Must(err, "Could not add members to group: %s", err)
+ m := h.newGroupManager(cmd)
+ response, err := m.AddMembersToGroup(args[0], hydra.GroupMembers{Members: args[1:]})
+ checkResponse(response, err, http.StatusNoContent)
fmt.Printf("Members %v added to group %s.\n", args[1:], args[0])
}
@@ -91,16 +71,10 @@ func (h *GroupHandler) RemoveMembers(cmd *cobra.Command, args []string) {
fmt.Print(cmd.UsageString())
return
}
- m := h.newGroupManager(cmd)
-
- var err error
- err = m.RemoveGroupMembers(args[0], args[1:])
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not remove members to group: %s", err)
+ m := h.newGroupManager(cmd)
+ response, err := m.RemoveMembersFromGroup(args[0], hydra.GroupMembers{Members: args[1:]})
+ checkResponse(response, err, http.StatusNoContent)
fmt.Printf("Members %v removed from group %s.\n", args[1:], args[0])
}
@@ -109,13 +83,9 @@ func (h *GroupHandler) FindGroups(cmd *cobra.Command, args []string) {
fmt.Print(cmd.UsageString())
return
}
- m := h.newGroupManager(cmd)
- gn, err := m.FindGroupNames(args[0])
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not find groups: %s", err)
- fmt.Printf("Subject %s belongs to groups %v.\n", args[0], gn)
+ m := h.newGroupManager(cmd)
+ groups, response, err := m.FindGroupsByMember(args[0])
+ checkResponse(response, err, http.StatusOK)
+ formatResponse(groups)
}
diff --git a/cmd/cli/handler_helper.go b/cmd/cli/handler_helper.go
new file mode 100644
index 00000000000..f8129a96e95
--- /dev/null
+++ b/cmd/cli/handler_helper.go
@@ -0,0 +1,26 @@
+package cli
+
+import (
+ "encoding/json"
+ "fmt"
+ "os"
+
+ "github.com/ory/hydra/pkg"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
+)
+
+func checkResponse(response *hydra.APIResponse, err error, expectedStatusCode int) {
+ pkg.Must(err, "Could not validate token: %s", err)
+
+ if response.StatusCode != expectedStatusCode {
+ fmt.Printf("Command failed because status code %d was expeceted but code %d was received.", expectedStatusCode, response.StatusCode)
+ os.Exit(1)
+ return
+ }
+}
+
+func formatResponse(response interface{}) string {
+ out, err := json.MarshalIndent(response, "", "\t")
+ pkg.Must(err, `Command failed because an error ("%s") occurred while prettifying output.`, err)
+ return string(out)
+}
diff --git a/cmd/cli/handler_introspection.go b/cmd/cli/handler_introspection.go
new file mode 100644
index 00000000000..a3a26954cfe
--- /dev/null
+++ b/cmd/cli/handler_introspection.go
@@ -0,0 +1,45 @@
+package cli
+
+import (
+ //"context"
+ //"encoding/json"
+ "fmt"
+
+ "github.com/ory/hydra/config"
+ //"github.com/ory/hydra/oauth2"
+
+ "net/http"
+ "strings"
+
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
+ "github.com/spf13/cobra"
+)
+
+type IntrospectionHandler struct {
+ Config *config.Config
+}
+
+func newIntrospectionHandler(c *config.Config) *IntrospectionHandler {
+ return &IntrospectionHandler{
+ Config: c,
+ }
+}
+
+func (h *IntrospectionHandler) IsAuthorized(cmd *cobra.Command, args []string) {
+ if len(args) != 1 {
+ fmt.Print(cmd.UsageString())
+ return
+ }
+
+ c := hydra.NewOAuth2ApiWithBasePath(h.Config.ClusterURL)
+ c.Configuration.Transport = h.Config.OAuth2Client(cmd).Transport
+
+ if term, _ := cmd.Flags().GetBool("fake-tls-termination"); term {
+ c.Configuration.DefaultHeader["X-Forwarded-Proto"] = "https"
+ }
+
+ scopes, _ := cmd.Flags().GetStringSlice("scopes")
+ result, response, err := c.IntrospectOAuth2Token(args[0], strings.Join(scopes, " "))
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("%s\n", formatResponse(result))
+}
diff --git a/cmd/cli/handler_jwk.go b/cmd/cli/handler_jwk.go
index af788f047e0..22b46f7c5d8 100644
--- a/cmd/cli/handler_jwk.go
+++ b/cmd/cli/handler_jwk.go
@@ -1,12 +1,12 @@
package cli
import (
- "encoding/json"
"fmt"
+ "net/http"
+
"github.com/ory/hydra/config"
- "github.com/ory/hydra/jwk"
- "github.com/ory/hydra/pkg"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/spf13/cobra"
)
@@ -14,77 +14,58 @@ type JWKHandler struct {
Config *config.Config
}
-func (h *JWKHandler) newJwkManager(cmd *cobra.Command) *jwk.HTTPManager {
- dry, _ := cmd.Flags().GetBool("dry")
- term, _ := cmd.Flags().GetBool("fake-tls-termination")
-
- return &jwk.HTTPManager{
- Dry: dry,
- Endpoint: h.Config.Resolve("/keys"),
- Client: h.Config.OAuth2Client(cmd),
- FakeTLSTermination: term,
+func (h *JWKHandler) newJwkManager(cmd *cobra.Command) *hydra.JsonWebKeyApi {
+ c := hydra.NewJsonWebKeyApiWithBasePath(h.Config.ClusterURL)
+ c.Configuration.Transport = h.Config.OAuth2Client(cmd).Transport
+ if term, _ := cmd.Flags().GetBool("fake-tls-termination"); term {
+ c.Configuration.DefaultHeader["X-Forwarded-Proto"] = "https"
}
+
+ return c
}
func newJWKHandler(c *config.Config) *JWKHandler {
- return &JWKHandler{
- Config: c,
- }
+ return &JWKHandler{Config: c}
}
func (h *JWKHandler) CreateKeys(cmd *cobra.Command, args []string) {
m := h.newJwkManager(cmd)
- if len(args) == 0 {
+ if len(args) < 1 || len(args) > 2 {
fmt.Println(cmd.UsageString())
return
}
- alg, _ := cmd.Flags().GetString("alg")
- keys, err := m.CreateKeys(args[0], alg)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
+ kid := ""
+ if len(args) == 2 {
+ kid = args[1]
}
- pkg.Must(err, "Could not generate keys: %s", err)
-
- out, err := json.MarshalIndent(keys, "", "\t")
- pkg.Must(err, "Could not marshall keys: %s", err)
- fmt.Printf("%s\n", out)
+ alg, _ := cmd.Flags().GetString("alg")
+ keys, response, err := m.CreateJsonWebKeySet(args[0], hydra.JsonWebKeySetGeneratorRequest{Alg: alg, Kid: kid})
+ checkResponse(response, err, http.StatusCreated)
+ fmt.Printf("%s\n", formatResponse(keys))
}
func (h *JWKHandler) GetKeys(cmd *cobra.Command, args []string) {
m := h.newJwkManager(cmd)
- if len(args) == 0 {
+ if len(args) != 1 {
fmt.Println(cmd.UsageString())
return
}
- keys, err := m.GetKeySet(args[0])
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not generate keys: %s", err)
-
- out, err := json.MarshalIndent(keys, "", "\t")
- pkg.Must(err, "Could not marshall keys: %s", err)
-
- fmt.Printf("%s\n", out)
+ keys, response, err := m.GetJsonWebKeySet(args[0])
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("%s\n", formatResponse(keys))
}
func (h *JWKHandler) DeleteKeys(cmd *cobra.Command, args []string) {
m := h.newJwkManager(cmd)
- if len(args) == 0 {
+ if len(args) != 1 {
fmt.Println(cmd.UsageString())
return
}
- err := m.DeleteKeySet(args[0])
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not generate keys: %s", err)
- fmt.Println("Key set deleted.")
+ response, err := m.DeleteJsonWebKeySet(args[0])
+ checkResponse(response, err, http.StatusNoContent)
+ fmt.Printf("Key set %s deleted.\n", args[0])
}
diff --git a/cmd/cli/handler_migrate.go b/cmd/cli/handler_migrate.go
index 415cf65c964..b24a28de90c 100644
--- a/cmd/cli/handler_migrate.go
+++ b/cmd/cli/handler_migrate.go
@@ -21,14 +21,10 @@ import (
type MigrateHandler struct {
c *config.Config
- M *jwk.HTTPManager
}
func newMigrateHandler(c *config.Config) *MigrateHandler {
- return &MigrateHandler{
- c: c,
- M: &jwk.HTTPManager{},
- }
+ return &MigrateHandler{c: c}
}
type schemaCreator interface {
diff --git a/cmd/cli/handler_policy.go b/cmd/cli/handler_policy.go
index 50a7537d9e5..d609f442701 100644
--- a/cmd/cli/handler_policy.go
+++ b/cmd/cli/handler_policy.go
@@ -4,9 +4,11 @@ import (
"fmt"
"os"
+ "net/http"
+
"github.com/ory/hydra/config"
"github.com/ory/hydra/pkg"
- "github.com/ory/hydra/policy"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/ory/ladon"
"github.com/spf13/cobra"
"github.com/square/go-jose/json"
@@ -16,16 +18,15 @@ type PolicyHandler struct {
Config *config.Config
}
-func (h *PolicyHandler) newJwkManager(cmd *cobra.Command) *policy.HTTPManager {
- dry, _ := cmd.Flags().GetBool("dry")
- term, _ := cmd.Flags().GetBool("fake-tls-termination")
+func (h *PolicyHandler) newPolicyManager(cmd *cobra.Command) *hydra.PolicyApi {
+ c := hydra.NewPolicyApiWithBasePath(h.Config.ClusterURL)
+ c.Configuration.Transport = h.Config.OAuth2Client(cmd).Transport
- return &policy.HTTPManager{
- Dry: dry,
- Endpoint: h.Config.Resolve("/policies"),
- Client: h.Config.OAuth2Client(cmd),
- FakeTLSTermination: term,
+ if term, _ := cmd.Flags().GetBool("fake-tls-termination"); term {
+ c.Configuration.DefaultHeader["X-Forwarded-Proto"] = "https"
}
+
+ return c
}
func newPolicyHandler(c *config.Config) *PolicyHandler {
@@ -35,18 +36,20 @@ func newPolicyHandler(c *config.Config) *PolicyHandler {
}
func (h *PolicyHandler) CreatePolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
- files, _ := cmd.Flags().GetStringSlice("files")
- if len(files) > 0 {
+ m := h.newPolicyManager(cmd)
+
+ if files, _ := cmd.Flags().GetStringSlice("files"); len(files) > 0 {
for _, path := range files {
reader, err := os.Open(path)
pkg.Must(err, "Could not open file %s: %s", path, err)
- var p ladon.DefaultPolicy
+
+ var p hydra.Policy
err = json.NewDecoder(reader).Decode(&p)
pkg.Must(err, "Could not parse JSON: %s", err)
- err = m.Create(&p)
- pkg.Must(err, "Could not create policy: %s", err)
- fmt.Printf("Imported policy %s from %s.\n", p.ID, path)
+
+ _, response, err := m.CreatePolicy(p)
+ checkResponse(response, err, http.StatusCreated)
+ fmt.Printf("Imported policy %s from %s.\n", p.Id, path)
}
return
}
@@ -69,57 +72,45 @@ func (h *PolicyHandler) CreatePolicy(cmd *cobra.Command, args []string) {
effect = ladon.AllowAccess
}
- p := &ladon.DefaultPolicy{
- ID: id,
+ result, response, err := m.CreatePolicy(hydra.Policy{
+ Id: id,
Description: description,
Subjects: subjects,
Resources: resources,
Actions: actions,
Effect: effect,
- }
- err := m.Create(p)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not create policy: %s", err)
- fmt.Printf("Created policy %s.\n", p.ID)
-
+ })
+ checkResponse(response, err, http.StatusCreated)
+ fmt.Printf("Created policy %s.\n", result.Id)
}
func (h *PolicyHandler) AddResourceToPolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
+ m := h.newPolicyManager(cmd)
if len(args) < 2 {
fmt.Print(cmd.UsageString())
return
}
- pp, err := m.Get(args[0])
- pkg.Must(err, "Could not get policy: %s", err)
+ p, response, err := m.GetPolicy(args[0])
+ checkResponse(response, err, http.StatusOK)
- p := pp.(*ladon.DefaultPolicy)
p.Resources = append(p.Resources, args[1:]...)
- err = m.Update(p)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not update policy: %s", err)
- fmt.Printf("Added resources to policy %s", p.ID)
+ _, response, err = m.UpdatePolicy(p.Id, *p)
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("Added resources to policy %s", p.Id)
}
func (h *PolicyHandler) RemoveResourceFromPolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
+ m := h.newPolicyManager(cmd)
if len(args) < 2 {
fmt.Print(cmd.UsageString())
return
}
- pp, err := m.Get(args[0])
- pkg.Must(err, "Could not get policy: %s", err)
+ p, response, err := m.GetPolicy(args[0])
+ checkResponse(response, err, http.StatusOK)
- p := pp.(*ladon.DefaultPolicy)
resources := []string{}
for _, r := range p.Resources {
var filter bool
@@ -134,52 +125,38 @@ func (h *PolicyHandler) RemoveResourceFromPolicy(cmd *cobra.Command, args []stri
}
p.Resources = resources
- err = m.Update(p)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not update policy: %s", err)
- fmt.Printf("Removed resources from policy %s", p.ID)
+ _, response, err = m.UpdatePolicy(p.Id, *p)
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("Removed resources from policy %s", p.Id)
}
func (h *PolicyHandler) AddSubjectToPolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
+ m := h.newPolicyManager(cmd)
if len(args) < 2 {
fmt.Print(cmd.UsageString())
return
}
- pp, err := m.Get(args[0])
- if m.Dry {
- fmt.Printf("%s\n", err)
- } else {
- pkg.Must(err, "Could not get policy: %s", err)
- }
+ p, response, err := m.GetPolicy(args[0])
+ checkResponse(response, err, http.StatusOK)
- p := pp.(*ladon.DefaultPolicy)
p.Subjects = append(p.Subjects, args[1:]...)
- err = m.Update(p)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not update policy: %s", err)
- fmt.Printf("Added subjects to policy %s", p.ID)
+ _, response, err = m.UpdatePolicy(p.Id, *p)
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("Added subjects to policy %s", p.Id)
}
func (h *PolicyHandler) RemoveSubjectFromPolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
+ m := h.newPolicyManager(cmd)
if len(args) < 2 {
fmt.Print(cmd.UsageString())
return
}
- pp, err := m.Get(args[0])
- pkg.Must(err, "Could not get policy: %s", err)
+ p, response, err := m.GetPolicy(args[0])
+ checkResponse(response, err, http.StatusOK)
- p := pp.(*ladon.DefaultPolicy)
subjects := []string{}
for _, r := range p.Subjects {
var filter bool
@@ -193,49 +170,40 @@ func (h *PolicyHandler) RemoveSubjectFromPolicy(cmd *cobra.Command, args []strin
}
}
p.Subjects = subjects
+ p.Subjects = append(p.Subjects, args[1:]...)
- err = m.Update(p)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not update policy: %s", err)
- fmt.Printf("Removed subjects from policy %s", p.ID)
+ _, response, err = m.UpdatePolicy(p.Id, *p)
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("Removed subjects from policy %s", p.Id)
}
func (h *PolicyHandler) AddActionToPolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
+ m := h.newPolicyManager(cmd)
if len(args) < 2 {
fmt.Print(cmd.UsageString())
return
}
- pp, err := m.Get(args[0])
- pkg.Must(err, "Could not get policy: %s", err)
+ p, response, err := m.GetPolicy(args[0])
+ checkResponse(response, err, http.StatusOK)
- p := pp.(*ladon.DefaultPolicy)
p.Actions = append(p.Actions, args[1:]...)
- err = m.Update(p)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not update policy: %s", err)
- fmt.Printf("Added actions to policy %s", p.ID)
+ _, response, err = m.UpdatePolicy(p.Id, *p)
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("Added actions to policy %s", p.Id)
}
func (h *PolicyHandler) RemoveActionFromPolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
+ m := h.newPolicyManager(cmd)
if len(args) < 2 {
fmt.Print(cmd.UsageString())
return
}
- pp, err := m.Get(args[0])
- pkg.Must(err, "Could not get policy: %s", err)
+ p, response, err := m.GetPolicy(args[0])
+ checkResponse(response, err, http.StatusOK)
- p := pp.(*ladon.DefaultPolicy)
actions := []string{}
for _, r := range p.Actions {
var filter bool
@@ -250,49 +218,34 @@ func (h *PolicyHandler) RemoveActionFromPolicy(cmd *cobra.Command, args []string
}
p.Actions = actions
- err = m.Update(p)
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not update policy: %s", err)
- fmt.Printf("Removed actions from policy %s", p.ID)
+ _, response, err = m.UpdatePolicy(p.Id, *p)
+ checkResponse(response, err, http.StatusOK)
+ fmt.Printf("Removed actions from policy %s", p.Id)
}
func (h *PolicyHandler) GetPolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
+ m := h.newPolicyManager(cmd)
if len(args) == 0 {
fmt.Print(cmd.UsageString())
return
}
- p, err := m.Get(args[0])
- if m.Dry {
- fmt.Printf("%s\n", err)
- return
- }
- pkg.Must(err, "Could not retrieve policy: %s", err)
-
- out, err := json.MarshalIndent(p, "", "\t")
- pkg.Must(err, "Could not convert policy to JSON: %s", err)
+ p, response, err := m.GetPolicy(args[0])
+ checkResponse(response, err, http.StatusOK)
- fmt.Printf("%s\n", out)
+ fmt.Printf("%s\n", formatResponse(p))
}
func (h *PolicyHandler) DeletePolicy(cmd *cobra.Command, args []string) {
- m := h.newJwkManager(cmd)
+ m := h.newPolicyManager(cmd)
if len(args) == 0 {
fmt.Print(cmd.UsageString())
return
}
for _, arg := range args {
- err := m.Delete(arg)
- if m.Dry {
- fmt.Printf("%s\n", err)
- continue
- }
- pkg.Must(err, "Could not delete policy: %s", err)
+ response, err := m.DeletePolicy(arg)
+ checkResponse(response, err, http.StatusNoContent)
fmt.Printf("Connection %s deleted.\n", arg)
}
}
diff --git a/cmd/cli/handler_recovation.go b/cmd/cli/handler_recovation.go
index 38e166332eb..d04070c42de 100644
--- a/cmd/cli/handler_recovation.go
+++ b/cmd/cli/handler_recovation.go
@@ -1,51 +1,46 @@
package cli
import (
- "context"
- "crypto/tls"
"fmt"
"net/http"
+ "crypto/tls"
+
"github.com/ory/hydra/config"
- "github.com/ory/hydra/oauth2"
- "github.com/ory/hydra/pkg"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/spf13/cobra"
- "golang.org/x/oauth2/clientcredentials"
)
type RevocationHandler struct {
Config *config.Config
- M *oauth2.HTTPRecovator
}
func newRevocationHandler(c *config.Config) *RevocationHandler {
- return &RevocationHandler{
- Config: c,
- M: &oauth2.HTTPRecovator{},
- }
+ return &RevocationHandler{Config: c}
}
func (h *RevocationHandler) RevokeToken(cmd *cobra.Command, args []string) {
- if ok, _ := cmd.Flags().GetBool("skip-tls-verify"); ok {
- // fmt.Println("Warning: Skipping TLS Certificate Verification.")
- h.M.Client = &http.Client{Transport: &http.Transport{
- TLSClientConfig: &tls.Config{InsecureSkipVerify: true},
- }}
+ if len(args) != 1 {
+ fmt.Print(cmd.UsageString())
+ return
}
- h.M.Endpoint = h.Config.Resolve("/oauth2/revoke")
- h.M.Config = &clientcredentials.Config{
- ClientID: h.Config.ClientID,
- ClientSecret: h.Config.ClientSecret,
+ handler := hydra.NewOAuth2ApiWithBasePath(h.Config.ClusterURL)
+ handler.Configuration.Username = h.Config.ClientID
+ handler.Configuration.Password = h.Config.ClientSecret
+
+ if skip, _ := cmd.Flags().GetBool("skip-tls-verify"); skip {
+ handler.Configuration.Transport = &http.Transport{
+ TLSClientConfig: &tls.Config{InsecureSkipVerify: true},
+ }
}
- if len(args) != 1 {
- fmt.Print(cmd.UsageString())
- return
+ if term, _ := cmd.Flags().GetBool("fake-tls-termination"); term {
+ handler.Configuration.DefaultHeader["X-Forwarded-Proto"] = "https"
}
token := args[0]
- err := h.M.RevokeToken(context.Background(), args[0])
- pkg.Must(err, "Could not revoke token: %s", err)
+ response, err := handler.RevokeOAuth2Token(args[0])
+ checkResponse(response, err, http.StatusOK)
fmt.Printf("Revoked token %s", token)
}
diff --git a/cmd/cli/handler_warden.go b/cmd/cli/handler_warden.go
deleted file mode 100644
index 060a083a1a7..00000000000
--- a/cmd/cli/handler_warden.go
+++ /dev/null
@@ -1,43 +0,0 @@
-package cli
-
-import (
- "context"
- "encoding/json"
- "fmt"
-
- "github.com/ory/hydra/config"
- "github.com/ory/hydra/oauth2"
- "github.com/ory/hydra/pkg"
- "github.com/spf13/cobra"
-)
-
-type WardenHandler struct {
- Config *config.Config
-}
-
-func newWardenHandler(c *config.Config) *WardenHandler {
- return &WardenHandler{
- Config: c,
- }
-}
-
-func (h *WardenHandler) IsAuthorized(cmd *cobra.Command, args []string) {
- m := &oauth2.HTTPIntrospector{
- Endpoint: h.Config.Resolve("/oauth2/introspect"),
- Client: h.Config.OAuth2Client(cmd),
- }
-
- if len(args) != 1 {
- fmt.Print(cmd.UsageString())
- return
- }
-
- scopes, _ := cmd.Flags().GetStringSlice("scopes")
- res, err := m.IntrospectToken(context.Background(), args[0], scopes...)
- pkg.Must(err, "Could not validate token: %s", err)
-
- out, err := json.MarshalIndent(res, "", "\t")
- pkg.Must(err, "Could not prettify token: %s", err)
-
- fmt.Printf("%s\n", out)
-}
diff --git a/cmd/clients.go b/cmd/clients.go
index c2586fffe6c..e999f004035 100644
--- a/cmd/clients.go
+++ b/cmd/clients.go
@@ -27,7 +27,7 @@ var clientsCmd = &cobra.Command{
func init() {
RootCmd.AddCommand(clientsCmd)
- clientsCmd.PersistentFlags().Bool("dry", false, "do not execute the command but show the corresponding curl command instead")
+ //clientsCmd.PersistentFlags().Bool("dry", false, "do not execute the command but show the corresponding curl command instead")
clientsCmd.PersistentFlags().Bool("fake-tls-termination", false, `fake tls termination by adding "X-Forwarded-Proto: https"" to http headers`)
// Here you will define your flags and configuration settings.
diff --git a/cmd/keys.go b/cmd/keys.go
index c1e6389b1e2..b4f444051dd 100644
--- a/cmd/keys.go
+++ b/cmd/keys.go
@@ -26,7 +26,7 @@ var keysCmd = &cobra.Command{
func init() {
RootCmd.AddCommand(keysCmd)
- keysCmd.PersistentFlags().Bool("dry", false, "do not execute the command but show the corresponding curl command instead")
+ //keysCmd.PersistentFlags().Bool("dry", false, "do not execute the command but show the corresponding curl command instead")
keysCmd.PersistentFlags().Bool("fake-tls-termination", false, `fake tls termination by adding "X-Forwarded-Proto: https"" to http headers`)
// Here you will define your flags and configuration settings.
diff --git a/cmd/keys_create.go b/cmd/keys_create.go
index 2337038e631..c0759ddd9c7 100644
--- a/cmd/keys_create.go
+++ b/cmd/keys_create.go
@@ -20,7 +20,7 @@ import (
// createCmd represents the create command
var keysCreateCmd = &cobra.Command{
- Use: "create ",
+ Use: "create ",
Short: "Create a new JSON Web Key Set",
Run: cmdHandler.Keys.CreateKeys,
}
diff --git a/cmd/root_test.go b/cmd/root_test.go
index 4ccd130f94d..5b18248138e 100644
--- a/cmd/root_test.go
+++ b/cmd/root_test.go
@@ -40,7 +40,7 @@ func TestExecute(t *testing.T) {
return err != nil
},
},
- {args: []string{"connect", "--id", "admin", "--secret", "pw", "--url", "https://127.0.0.1:4444/"}},
+ {args: []string{"connect", "--id", "admin", "--secret", "pw", "--url", "https://127.0.0.1:4444"}},
{args: []string{"clients", "create", "--id", "foobarbaz"}},
{args: []string{"clients", "get", "foobarbaz"}},
{args: []string{"clients", "create", "--id", "public-foo", "--is-public"}},
diff --git a/cmd/token.go b/cmd/token.go
index 0a7efd9346c..f307815edb6 100644
--- a/cmd/token.go
+++ b/cmd/token.go
@@ -12,6 +12,6 @@ var tokenCmd = &cobra.Command{
func init() {
RootCmd.AddCommand(tokenCmd)
- tokenCmd.PersistentFlags().Bool("dry", false, "do not execute the command but show the corresponding curl command instead")
+ //tokenCmd.PersistentFlags().Bool("dry", false, "do not execute the command but show the corresponding curl command instead")
tokenCmd.PersistentFlags().Bool("fake-tls-termination", false, `fake tls termination by adding "X-Forwarded-Proto: https"" to http headers`)
}
diff --git a/config/config.go b/config/config.go
index 12ad83817bf..5d80e799e80 100644
--- a/config/config.go
+++ b/config/config.go
@@ -136,6 +136,10 @@ func (c *Config) DoesRequestSatisfyTermination(r *http.Request) error {
return errors.New("TLS termination is not enabled")
}
+ if r.URL.Path == "/health" {
+ return nil
+ }
+
ranges := strings.Split(c.AllowTLSTermination, ",")
if err := matchesRange(r, ranges); err != nil {
return err
diff --git a/config/config_test.go b/config/config_test.go
index 5003d2aaace..f8bf37b2473 100644
--- a/config/config_test.go
+++ b/config/config_test.go
@@ -2,6 +2,7 @@ package config
import (
"net/http"
+ "net/url"
"testing"
"time"
@@ -19,26 +20,35 @@ func TestConfig(t *testing.T) {
func TestDoesRequestSatisfyTermination(t *testing.T) {
c := &Config{AllowTLSTermination: ""}
- assert.NotNil(t, c.DoesRequestSatisfyTermination(new(http.Request)))
+ assert.Error(t, c.DoesRequestSatisfyTermination(&http.Request{Header: http.Header{}, URL: new(url.URL)}))
c = &Config{AllowTLSTermination: "127.0.0.1/24"}
- r := &http.Request{Header: http.Header{}}
- assert.NotNil(t, c.DoesRequestSatisfyTermination(r))
+ r := &http.Request{Header: http.Header{}, URL: new(url.URL)}
+ assert.Error(t, c.DoesRequestSatisfyTermination(r))
- r = &http.Request{Header: http.Header{"X-Forwarded-Proto": []string{"http"}}}
- assert.NotNil(t, c.DoesRequestSatisfyTermination(r))
+ r = &http.Request{Header: http.Header{"X-Forwarded-Proto": []string{"http"}}, URL: new(url.URL)}
+ assert.Error(t, c.DoesRequestSatisfyTermination(r))
r = &http.Request{
RemoteAddr: "227.0.0.1:123",
Header: http.Header{"X-Forwarded-Proto": []string{"https"}},
+ URL: new(url.URL),
}
- assert.NotNil(t, c.DoesRequestSatisfyTermination(r))
+ assert.Error(t, c.DoesRequestSatisfyTermination(r))
r = &http.Request{
RemoteAddr: "127.0.0.1:123",
Header: http.Header{"X-Forwarded-Proto": []string{"https"}},
+ URL: new(url.URL),
}
- assert.Nil(t, c.DoesRequestSatisfyTermination(r))
+ assert.NoError(t, c.DoesRequestSatisfyTermination(r))
+
+ r = &http.Request{
+ RemoteAddr: "127.0.0.1:123",
+ Header: http.Header{"X-Forwarded-Proto": []string{"https"}},
+ URL: &url.URL{Path: "/health"},
+ }
+ assert.NoError(t, c.DoesRequestSatisfyTermination(r))
}
func TestSystemSecret(t *testing.T) {
diff --git a/doc.go b/doc.go
index 37e0b856f81..67cbb052a51 100644
--- a/doc.go
+++ b/doc.go
@@ -1,4 +1,4 @@
-// Package main Hydra OAuth2 & OpenID Connect Server (1.0.0-aplha1)
+// Package main Hydra OAuth2 & OpenID Connect Server
//
// Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/
//
@@ -11,13 +11,19 @@
// The official repository is located at https://github.com/ory/hydra
//
//
-// ### ATTENTION - IMPORTANT NOTE
-//
+// ### Important REST API Documentation Notes
//
// The swagger generator used to create this documentation does currently not support example responses. To see
// request and response payloads click on **"Show JSON schema"**:
// 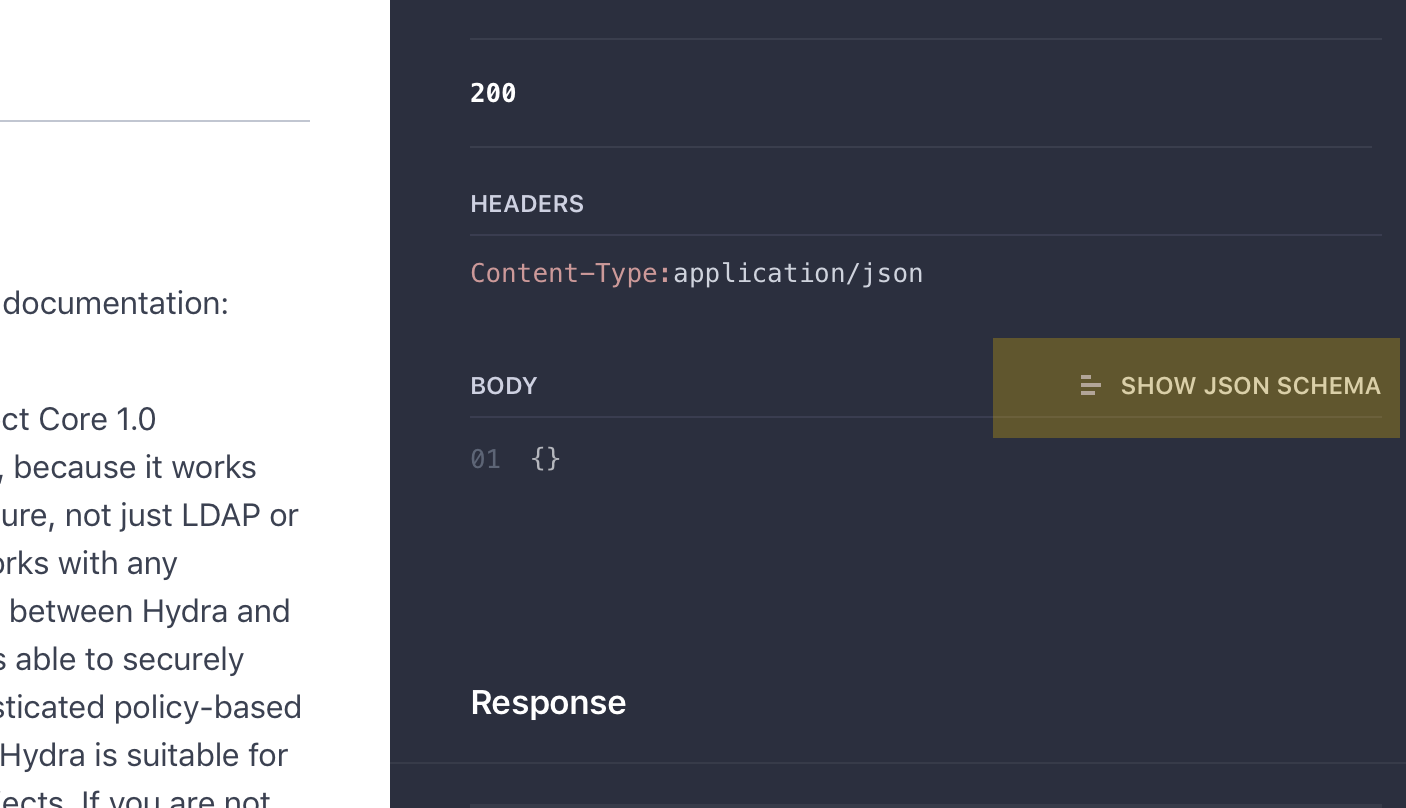
//
+//
+// The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please
+// refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example:
+//
+// - 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml
+// - 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+//
// Schemes: http, https
// Host:
// BasePath: /
diff --git a/docs/api.swagger.json b/docs/api.swagger.json
new file mode 100644
index 00000000000..b28d5bbc381
--- /dev/null
+++ b/docs/api.swagger.json
@@ -0,0 +1,3161 @@
+{
+ "consumes": [
+ "application/json",
+ "application/x-www-form-urlencoded"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "swagger": "2.0",
+ "info": {
+ "description": "Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/\n\n\nHydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure.\nHydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to.\nHydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works.\n\n\nThe official repository is located at https://github.com/ory/hydra\n\n\n### Important REST API Documentation Notes\n\nThe swagger generator used to create this documentation does currently not support example responses. To see\nrequest and response payloads click on **\"Show JSON schema\"**:\n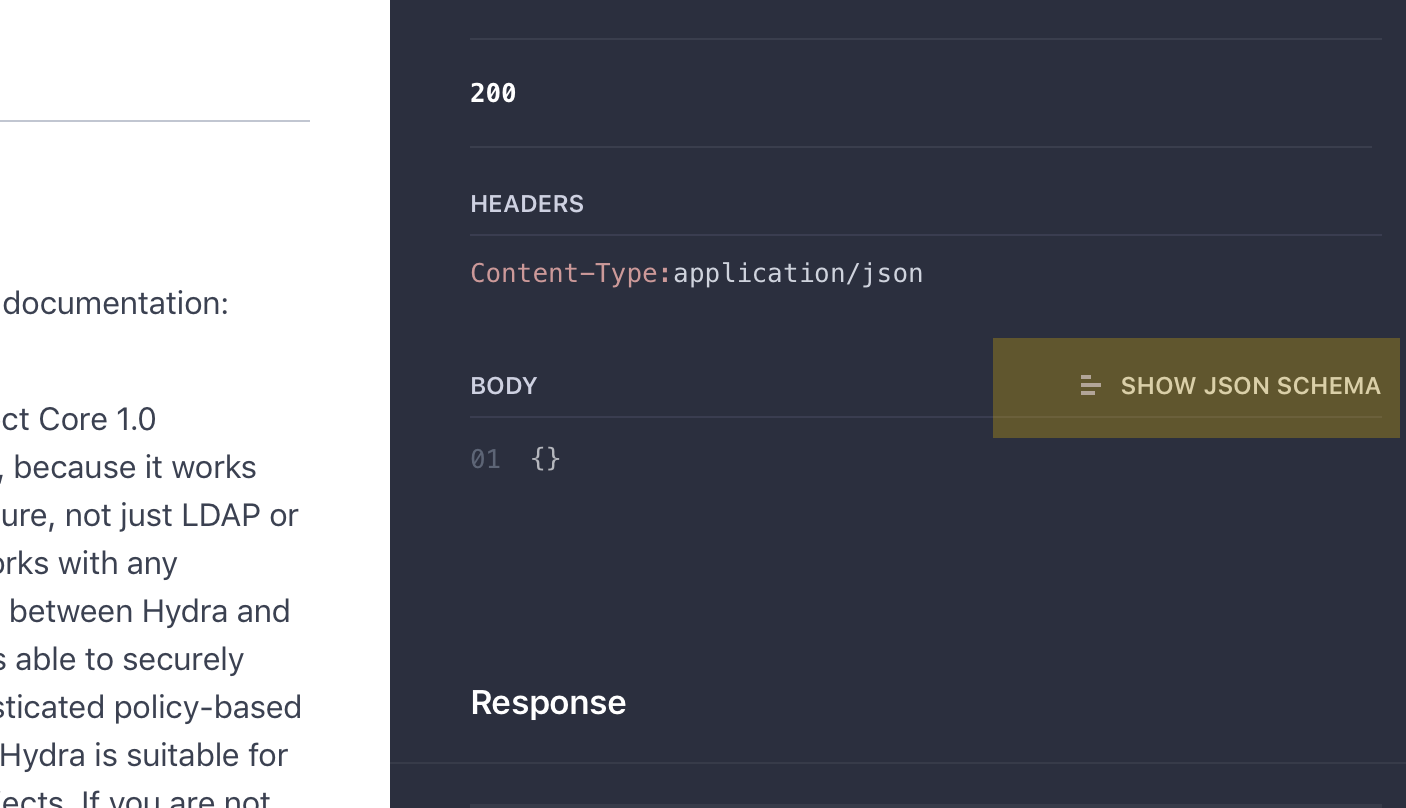\n\n\nThe API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please\nrefer to https://github.com/ory/hydra/blob/\u003ctag-id\u003e/docs/api.swagger.yaml - for example:\n\n0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml\n0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml",
+ "title": "Hydra OAuth2 \u0026 OpenID Connect Server",
+ "contact": {
+ "name": "ORY",
+ "url": "https://www.ory.am",
+ "email": "hi@ory.am"
+ },
+ "license": {
+ "name": "Apache 2.0",
+ "url": "https://github.com/ory/hydra/blob/master/LICENSE"
+ },
+ "version": "Latest"
+ },
+ "basePath": "/",
+ "paths": {
+ "/.well-known/jwks.json": {
+ "get": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:keys:hydra.openid.id-token:public\"],\n\"actions\": [\"GET\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Get list of well known JSON Web Keys",
+ "operationId": "wellKnown",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.keys.get"
+ ]
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "jsonWebKeySet",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKeySet"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/.well-known/openid-configuration": {
+ "get": {
+ "description": "The well known endpoint an be used to retrieve information for OpenID Connect clients. We encourage you to not roll\nyour own OpenID Connect client but to use an OpenID Connect client library instead. You can learn more on this\nflow at https://openid.net/specs/openid-connect-discovery-1_0.html",
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Server well known configuration",
+ "operationId": "getWellKnown",
+ "responses": {
+ "200": {
+ "description": "wellKnown",
+ "schema": {
+ "$ref": "#/definitions/wellKnown"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/clients": {
+ "get": {
+ "description": "This endpoint never returns passwords.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:clients\"],\n\"actions\": [\"get\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "List OAuth 2.0 Clients",
+ "operationId": "listOAuth2Clients",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.clients"
+ ]
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/oAuth2ClientList"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "post": {
+ "description": "If you pass `client_secret` the secret will be used, otherwise a random secret will be generated. The secret will\nbe returned in the response and you will not be able to retrieve it later on. Write the secret down and keep\nit somwhere safe.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:clients\"],\n\"actions\": [\"create\"],\n\"effect\": \"allow\"\n}\n```\n\nAdditionally, the context key \"owner\" is set to the owner of the client, allowing policies such as:\n\n```\n{\n\"resources\": [\"rn:hydra:clients\"],\n\"actions\": [\"create\"],\n\"effect\": \"allow\",\n\"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } }\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Create an OAuth 2.0 client",
+ "operationId": "createOAuth2Client",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.clients"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "name": "Body",
+ "in": "body",
+ "required": true,
+ "schema": {
+ "$ref": "#/definitions/oAuth2Client"
+ }
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "oAuth2Client",
+ "schema": {
+ "$ref": "#/definitions/oAuth2Client"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/clients/{id}": {
+ "get": {
+ "description": "This endpoint never returns passwords.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:clients:\u003csome-id\u003e\"],\n\"actions\": [\"get\"],\n\"effect\": \"allow\"\n}\n```\n\nAdditionally, the context key \"owner\" is set to the owner of the client, allowing policies such as:\n\n```\n{\n\"resources\": [\"rn:hydra:clients:\u003csome-id\u003e\"],\n\"actions\": [\"get\"],\n\"effect\": \"allow\",\n\"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } }\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Retrieve an OAuth 2.0 Client.",
+ "operationId": "getOAuth2Client",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.clients"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "uniqueItems": true,
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the OAuth 2.0 Client.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "oAuth2Client",
+ "schema": {
+ "$ref": "#/definitions/oAuth2Client"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "put": {
+ "description": "If you pass `client_secret` the secret will be updated and returned via the API. This is the only time you will\nbe able to retrieve the client secret, so write it down and keep it safe.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:clients\"],\n\"actions\": [\"update\"],\n\"effect\": \"allow\"\n}\n```\n\nAdditionally, the context key \"owner\" is set to the owner of the client, allowing policies such as:\n\n```\n{\n\"resources\": [\"rn:hydra:clients\"],\n\"actions\": [\"update\"],\n\"effect\": \"allow\",\n\"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } }\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Update an OAuth 2.0 Client",
+ "operationId": "updateOAuth2Client",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.clients"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "name": "id",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "required": true,
+ "schema": {
+ "$ref": "#/definitions/oAuth2Client"
+ }
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "oAuth2Client",
+ "schema": {
+ "$ref": "#/definitions/oAuth2Client"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "delete": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:clients:\u003csome-id\u003e\"],\n\"actions\": [\"delete\"],\n\"effect\": \"allow\"\n}\n```\n\nAdditionally, the context key \"owner\" is set to the owner of the client, allowing policies such as:\n\n```\n{\n\"resources\": [\"rn:hydra:clients:\u003csome-id\u003e\"],\n\"actions\": [\"delete\"],\n\"effect\": \"allow\",\n\"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } }\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Deletes an OAuth 2.0 Client",
+ "operationId": "deleteOAuth2Client",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.clients"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "uniqueItems": true,
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the OAuth 2.0 Client.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/health/metrics": {
+ "get": {
+ "description": "This endpoint returns an instance's metrics, such as average response time, status code distribution, hits per\nsecond and so on. The return values are currently not documented as this endpoint is still experimental.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:health:stats\"],\n\"actions\": [\"get\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "health"
+ ],
+ "summary": "Show instance metrics (experimental)",
+ "operationId": "getInstanceMetrics",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.health"
+ ]
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/health/status": {
+ "get": {
+ "description": "This endpoint returns `{ \"status\": \"ok\" }`. This status let's you know that the HTTP server is up and running. This\nstatus does currently not include checks whether the database connection is up and running. This endpoint does not\nrequire the `X-Forwarded-Proto` header when TLS termination is set.\n\n\nBe aware that if you are running multiple nodes of ORY Hydra, the health status will never refer to the cluster state,\nonly to a single instance.",
+ "tags": [
+ "health"
+ ],
+ "summary": "Check health status of this instance",
+ "operationId": "getInstanceStatus",
+ "responses": {
+ "200": {
+ "$ref": "#/responses/healthStatus"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/keys/{set}": {
+ "get": {
+ "description": "This endpoint can be used to retrieve JWK Sets stored in ORY Hydra.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:keys:\u003cset\u003e:\u003ckid\u003e\"],\n\"actions\": [\"get\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "jsonWebKey"
+ ],
+ "summary": "Retrieve a JSON Web Key Set",
+ "operationId": "getJsonWebKeySet",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.keys.get"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "Set",
+ "description": "The set",
+ "name": "set",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "jsonWebKeySet",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKeySet"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "put": {
+ "description": "Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:keys:\u003cset\u003e\"],\n\"actions\": [\"update\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "jsonWebKey"
+ ],
+ "summary": "Update a JSON Web Key Set",
+ "operationId": "updateJsonWebKeySet",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.keys.update"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "Set",
+ "description": "The set",
+ "name": "set",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKeySet"
+ }
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "jsonWebKeySet",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKeySet"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "post": {
+ "description": "This endpoint is capable of generating JSON Web Key Sets for you. There a different strategies available, such as\nsymmetric cryptographic keys (HS256) and asymetric cryptographic keys (RS256, ECDSA).\n\n\nIf the specified JSON Web Key Set does not exist, it will be created.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:keys:\u003cset\u003e:\u003ckid\u003e\"],\n\"actions\": [\"create\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "jsonWebKey"
+ ],
+ "summary": "Generate a new JSON Web Key",
+ "operationId": "createJsonWebKeySet",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.keys.create"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "Set",
+ "description": "The set",
+ "name": "set",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKeySetGeneratorRequest"
+ }
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "jsonWebKeySet",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKeySet"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "delete": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:keys:\u003cset\u003e\"],\n\"actions\": [\"delete\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "jsonWebKey"
+ ],
+ "summary": "Delete a JSON Web Key",
+ "operationId": "deleteJsonWebKeySet",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.keys.delete"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "Set",
+ "description": "The set",
+ "name": "set",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/keys/{set}/{kid}": {
+ "get": {
+ "description": "This endpoint can be used to retrieve JWKs stored in ORY Hydra.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:keys:\u003cset\u003e:\u003ckid\u003e\"],\n\"actions\": [\"get\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "jsonWebKey"
+ ],
+ "summary": "Retrieve a JSON Web Key",
+ "operationId": "getJsonWebKey",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.keys.get"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "KID",
+ "description": "The kid of the desired key",
+ "name": "kid",
+ "in": "path",
+ "required": true
+ },
+ {
+ "type": "string",
+ "x-go-name": "Set",
+ "description": "The set",
+ "name": "set",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "jsonWebKeySet",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKeySet"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "put": {
+ "description": "Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:keys:\u003cset\u003e:\u003ckid\u003e\"],\n\"actions\": [\"update\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "jsonWebKey"
+ ],
+ "summary": "Update a JSON Web Key",
+ "operationId": "updateJsonWebKey",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.keys.update"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "KID",
+ "description": "The kid of the desired key",
+ "name": "kid",
+ "in": "path",
+ "required": true
+ },
+ {
+ "type": "string",
+ "x-go-name": "Set",
+ "description": "The set",
+ "name": "set",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKey"
+ }
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "jsonWebKey",
+ "schema": {
+ "$ref": "#/definitions/jsonWebKey"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "delete": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:keys:\u003cset\u003e:\u003ckid\u003e\"],\n\"actions\": [\"delete\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "jsonWebKey"
+ ],
+ "summary": "Delete a JSON Web Key",
+ "operationId": "deleteJsonWebKey",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.keys.delete"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "KID",
+ "description": "The kid of the desired key",
+ "name": "kid",
+ "in": "path",
+ "required": true
+ },
+ {
+ "type": "string",
+ "x-go-name": "Set",
+ "description": "The set",
+ "name": "set",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/oauth2/auth": {
+ "get": {
+ "description": "This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows.\nOAuth2 is a very popular protocol and a library for your programming language will exists.\n\nTo learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749",
+ "consumes": [
+ "application/x-www-form-urlencoded"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "The OAuth 2.0 authorize endpoint",
+ "operationId": "oauthAuth",
+ "responses": {
+ "302": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/oauth2/consent/requests/{id}": {
+ "get": {
+ "description": "Call this endpoint to receive information on consent requests.\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:oauth2:consent:requests:\u003crequest-id\u003e\"],\n\"actions\": [\"get\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Receive consent request information",
+ "operationId": "getOAuth2ConsentRequest",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.consent"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "uniqueItems": true,
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the OAuth 2.0 Consent Request.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/oAuth2ConsentRequest"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/oauth2/consent/requests/{id}/accept": {
+ "patch": {
+ "description": "Call this endpoint to accept a consent request. This usually happens when a user agrees to give access rights to\nan application.\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:oauth2:consent:requests:\u003crequest-id\u003e\"],\n\"actions\": [\"accept\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Accept a consent request",
+ "operationId": "acceptOAuth2ConsentRequest",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.consent"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "name": "id",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "required": true,
+ "schema": {
+ "$ref": "#/definitions/consentRequestAcceptance"
+ }
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/oauth2/consent/requests/{id}/reject": {
+ "patch": {
+ "description": "Call this endpoint to reject a consent request. This usually happens when a user denies access rights to an\napplication.\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:oauth2:consent:requests:\u003crequest-id\u003e\"],\n\"actions\": [\"reject\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Reject a consent request",
+ "operationId": "rejectOAuth2ConsentRequest",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.consent"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "name": "id",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "required": true,
+ "schema": {
+ "$ref": "#/definitions/consentRequestRejection"
+ }
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/oauth2/introspect": {
+ "post": {
+ "description": "The introspection endpoint allows to check if a token (both refresh and access) is active or not. An active token\nis neither expired nor revoked. If a token is active, additional information on the token will be included. You can\nset additional data for a token by setting `accessTokenExtra` during the consent flow.",
+ "consumes": [
+ "application/x-www-form-urlencoded"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Introspect OAuth2 tokens",
+ "operationId": "introspectOAuth2Token",
+ "security": [
+ {
+ "basic": []
+ },
+ {
+ "oauth2": []
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "Token",
+ "description": "The string value of the token. For access tokens, this\nis the \"access_token\" value returned from the token endpoint\ndefined in OAuth 2.0 [RFC6749], Section 5.1.\nThis endpoint DOES NOT accept refresh tokens for validation.",
+ "name": "token",
+ "in": "formData",
+ "required": true
+ },
+ {
+ "type": "string",
+ "x-go-name": "Scope",
+ "description": "An optional, space separated list of required scopes. If the access token was not granted one of the\nscopes, the result of active will be false.",
+ "name": "scope",
+ "in": "formData"
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/introspectOAuth2TokenResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/oauth2/revoke": {
+ "post": {
+ "description": "Revoking a token (both access and refresh) means that the tokens will be invalid. A revoked access token can no\nlonger be used to make access requests, and a revoked refresh token can no longer be used to refresh an access token.\nRevoking a refresh token also invalidates the access token that was created with it.",
+ "consumes": [
+ "application/x-www-form-urlencoded"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "Revoke OAuth2 tokens",
+ "operationId": "revokeOAuth2Token",
+ "security": [
+ {
+ "basic": []
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "Token",
+ "name": "token",
+ "in": "formData",
+ "required": true
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/oauth2/token": {
+ "post": {
+ "description": "This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows.\nOAuth2 is a very popular protocol and a library for your programming language will exists.\n\nTo learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749",
+ "consumes": [
+ "application/x-www-form-urlencoded"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "oAuth2"
+ ],
+ "summary": "The OAuth 2.0 token endpoint",
+ "operationId": "oauthToken",
+ "security": [
+ {
+ "basic": []
+ },
+ {
+ "oauth2": []
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/oauthTokenResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/policies": {
+ "get": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:policies\"],\n\"actions\": [\"list\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "policy"
+ ],
+ "summary": "List Access Control Policies",
+ "operationId": "listPolicies",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.policies"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "Offset",
+ "description": "The offset from where to start looking.",
+ "name": "offset",
+ "in": "query"
+ },
+ {
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "Limit",
+ "description": "The maximum amount of policies returned.",
+ "name": "limit",
+ "in": "query"
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/policyList"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "post": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:policies\"],\n\"actions\": [\"create\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "policy"
+ ],
+ "summary": "Create an Access Control Policy",
+ "operationId": "createPolicy",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.policies"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/policy"
+ }
+ }
+ ],
+ "responses": {
+ "201": {
+ "description": "policy",
+ "schema": {
+ "$ref": "#/definitions/policy"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/policies/{id}": {
+ "get": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:policies:\u003cid\u003e\"],\n\"actions\": [\"get\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "policy"
+ ],
+ "summary": "Get an Access Control Policy",
+ "operationId": "getPolicy",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.policies"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the policy.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "policy",
+ "schema": {
+ "$ref": "#/definitions/policy"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "put": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:policies\"],\n\"actions\": [\"update\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "policy"
+ ],
+ "summary": "Update an Access Control Polic",
+ "operationId": "updatePolicy",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.policies"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the policy.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/policy"
+ }
+ }
+ ],
+ "responses": {
+ "200": {
+ "description": "policy",
+ "schema": {
+ "$ref": "#/definitions/policy"
+ }
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "delete": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:policies:\u003cid\u003e\"],\n\"actions\": [\"delete\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "policy"
+ ],
+ "summary": "Delete an Access Control Policy",
+ "operationId": "deletePolicy",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.policies"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the policy.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/warden/allowed": {
+ "post": {
+ "description": "Checks if a subject (typically a user or a service) is allowed to perform an action on a resource. This endpoint requires a subject,\na resource name, an action name and a context. If the subject is not allowed to perform the action on the resource,\nthis endpoint returns a 200 response with `{ \"allowed\": false}`, otherwise `{ \"allowed\": true }` is returned.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:warden:allowed\"],\n\"actions\": [\"decide\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "warden"
+ ],
+ "summary": "Check if an access request is valid (without providing an access token)",
+ "operationId": "doesWardenAllowAccessRequest",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.warden"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/wardenAccessRequest"
+ }
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/wardenAccessRequestResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/warden/groups": {
+ "get": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:warden:groups\"],\n\"actions\": [\"list\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "warden"
+ ],
+ "summary": "Find groups by member",
+ "operationId": "findGroupsByMember",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.groups"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "Member",
+ "description": "The id of the member to look up.",
+ "name": "member",
+ "in": "query",
+ "required": true
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/findGroupsByMemberResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "post": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:warden:groups\"],\n\"actions\": [\"create\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "warden"
+ ],
+ "summary": "Create a group",
+ "operationId": "createGroup",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.groups"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/group"
+ }
+ }
+ ],
+ "responses": {
+ "201": {
+ "$ref": "#/responses/groupResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/warden/groups/{id}": {
+ "get": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:warden:groups:\u003cid\u003e\"],\n\"actions\": [\"create\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "warden"
+ ],
+ "summary": "Get a group by id",
+ "operationId": "getGroup",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.groups"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the group to look up.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "201": {
+ "$ref": "#/responses/groupResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "delete": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:warden:groups:\u003cid\u003e\"],\n\"actions\": [\"delete\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "warden"
+ ],
+ "summary": "Delete a group by id",
+ "operationId": "deleteGroup",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.groups"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the group to look up.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/warden/groups/{id}/members": {
+ "post": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:warden:groups:\u003cid\u003e\"],\n\"actions\": [\"members.add\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "warden"
+ ],
+ "summary": "Add members to a group",
+ "operationId": "addMembersToGroup",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.groups"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the group to modify.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/groupMembers"
+ }
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ },
+ "delete": {
+ "description": "The subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:warden:groups:\u003cid\u003e\"],\n\"actions\": [\"members.remove\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "warden"
+ ],
+ "summary": "Remove members from a group",
+ "operationId": "removeMembersFromGroup",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.groups"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "type": "string",
+ "x-go-name": "ID",
+ "description": "The id of the group to modify.",
+ "name": "id",
+ "in": "path",
+ "required": true
+ },
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/groupMembers"
+ }
+ }
+ ],
+ "responses": {
+ "204": {
+ "$ref": "#/responses/emptyResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ },
+ "/warden/token/allowed": {
+ "post": {
+ "description": "Checks if a token is valid and if the token subject is allowed to perform an action on a resource.\nThis endpoint requires a token, a scope, a resource name, an action name and a context.\n\n\nIf a token is expired/invalid, has not been granted the requested scope or the subject is not allowed to\nperform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`.\n\n\nExtra data set through the `accessTokenExtra` field in the consent flow will be included in the response.\n\n\nThe subject making the request needs to be assigned to a policy containing:\n\n```\n{\n\"resources\": [\"rn:hydra:warden:token:allowed\"],\n\"actions\": [\"decide\"],\n\"effect\": \"allow\"\n}\n```",
+ "consumes": [
+ "application/json"
+ ],
+ "produces": [
+ "application/json"
+ ],
+ "schemes": [
+ "http",
+ "https"
+ ],
+ "tags": [
+ "warden"
+ ],
+ "summary": "Check if an access request is valid (providing an access token)",
+ "operationId": "doesWardenAllowTokenAccessRequest",
+ "security": [
+ {
+ "oauth2": [
+ "hydra.warden"
+ ]
+ }
+ ],
+ "parameters": [
+ {
+ "name": "Body",
+ "in": "body",
+ "schema": {
+ "$ref": "#/definitions/wardenTokenAccessRequest"
+ }
+ }
+ ],
+ "responses": {
+ "200": {
+ "$ref": "#/responses/wardenTokenAccessRequestResponse"
+ },
+ "401": {
+ "$ref": "#/responses/genericError"
+ },
+ "403": {
+ "$ref": "#/responses/genericError"
+ },
+ "500": {
+ "$ref": "#/responses/genericError"
+ }
+ }
+ }
+ }
+ },
+ "definitions": {
+ "ConsentRequestManager": {
+ "type": "object",
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "Context": {
+ "description": "Context contains an access token's session data",
+ "type": "object",
+ "properties": {
+ "aud": {
+ "description": "Audience is who the token was issued for. This is an OAuth2 app usually.",
+ "type": "string",
+ "x-go-name": "Audience"
+ },
+ "exp": {
+ "description": "ExpiresAt is the expiry timestamp.",
+ "x-go-name": "ExpiresAt"
+ },
+ "ext": {
+ "description": "Extra represents arbitrary session data.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "Extra"
+ },
+ "iat": {
+ "description": "IssuedAt is the token creation time stamp.",
+ "x-go-name": "IssuedAt"
+ },
+ "iss": {
+ "description": "Issuer is the id of the issuer, typically an hydra instance.",
+ "type": "string",
+ "x-go-name": "Issuer"
+ },
+ "scopes": {
+ "description": "GrantedScopes is a list of scopes that the subject authorized when asked for consent.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "GrantedScopes"
+ },
+ "sub": {
+ "description": "Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app.\nThis is usually a uuid but you can choose a urn or some other id too.",
+ "type": "string",
+ "x-go-name": "Subject"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/firewall"
+ },
+ "Firewall": {
+ "type": "object",
+ "title": "Firewall offers various validation strategies for access tokens.",
+ "x-go-package": "github.com/ory/hydra/firewall"
+ },
+ "Handler": {
+ "type": "object",
+ "properties": {
+ "Generators": {
+ "type": "object",
+ "additionalProperties": {
+ "$ref": "#/definitions/KeyGenerator"
+ }
+ },
+ "H": {
+ "$ref": "#/definitions/Writer"
+ },
+ "Manager": {
+ "$ref": "#/definitions/Manager"
+ },
+ "W": {
+ "$ref": "#/definitions/Firewall"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "KeyGenerator": {
+ "type": "object",
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "Manager": {
+ "type": "object",
+ "x-go-package": "github.com/ory/hydra/warden/group"
+ },
+ "RawMessage": {
+ "description": "It implements Marshaler and Unmarshaler and can\nbe used to delay JSON decoding or precompute a JSON encoding.",
+ "type": "array",
+ "title": "RawMessage is a raw encoded JSON value.",
+ "items": {
+ "type": "integer",
+ "format": "uint8"
+ },
+ "x-go-package": "encoding/json"
+ },
+ "Writer": {
+ "description": "Writer is a helper to write arbitrary data to a ResponseWriter",
+ "type": "object",
+ "x-go-package": "github.com/ory/hydra/vendor/github.com/ory/herodot"
+ },
+ "consentRequestAcceptance": {
+ "type": "object",
+ "title": "AcceptConsentRequestPayload represents data that will be used to accept a consent request.",
+ "properties": {
+ "accessTokenExtra": {
+ "description": "AccessTokenExtra represents arbitrary data that will be added to the access token and that will be returned\non introspection and warden requests.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "AccessTokenExtra"
+ },
+ "grantScopes": {
+ "description": "A list of scopes that the user agreed to grant. It should be a subset of requestedScopes from the consent request.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "GrantScopes"
+ },
+ "idTokenExtra": {
+ "description": "IDTokenExtra represents arbitrary data that will be added to the ID token. The ID token will only be issued\nif the user agrees to it and if the client requested an ID token.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "IDTokenExtra"
+ },
+ "subject": {
+ "description": "Subject represents a unique identifier of the user (or service, or legal entity, ...) that accepted the\nOAuth2 request.",
+ "type": "string",
+ "x-go-name": "Subject"
+ }
+ },
+ "x-go-name": "AcceptConsentRequestPayload",
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "consentRequestRejection": {
+ "type": "object",
+ "title": "RejectConsentRequestPayload represents data that will be used to reject a consent request.",
+ "properties": {
+ "reason": {
+ "description": "Reason represents the reason why the user rejected the consent request.",
+ "type": "string",
+ "x-go-name": "Reason"
+ }
+ },
+ "x-go-name": "RejectConsentRequestPayload",
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "group": {
+ "description": "Group represents a warden group",
+ "type": "object",
+ "properties": {
+ "id": {
+ "description": "ID is the groups id.",
+ "type": "string",
+ "x-go-name": "ID"
+ },
+ "members": {
+ "description": "Members is who belongs to the group.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "Members"
+ }
+ },
+ "x-go-name": "Group",
+ "x-go-package": "github.com/ory/hydra/warden/group"
+ },
+ "groupMembers": {
+ "type": "object",
+ "properties": {
+ "members": {
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "Members"
+ }
+ },
+ "x-go-name": "membersRequest",
+ "x-go-package": "github.com/ory/hydra/warden/group"
+ },
+ "joseWebKeySetRequest": {
+ "type": "object",
+ "properties": {
+ "keys": {
+ "type": "array",
+ "items": {
+ "$ref": "#/definitions/RawMessage"
+ },
+ "x-go-name": "Keys"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "jsonWebKey": {
+ "type": "object",
+ "properties": {
+ "alg": {
+ "description": "The \"alg\" (algorithm) parameter identifies the algorithm intended for\nuse with the key. The values used should either be registered in the\nIANA \"JSON Web Signature and Encryption Algorithms\" registry\nestablished by [JWA] or be a value that contains a Collision-\nResistant Name.",
+ "type": "string",
+ "x-go-name": "Alg"
+ },
+ "crv": {
+ "type": "string",
+ "x-go-name": "Crv"
+ },
+ "d": {
+ "type": "string",
+ "x-go-name": "D"
+ },
+ "dp": {
+ "type": "string",
+ "x-go-name": "Dp"
+ },
+ "dq": {
+ "type": "string",
+ "x-go-name": "Dq"
+ },
+ "e": {
+ "type": "string",
+ "x-go-name": "E"
+ },
+ "k": {
+ "type": "string",
+ "x-go-name": "K"
+ },
+ "kid": {
+ "description": "The \"kid\" (key ID) parameter is used to match a specific key. This\nis used, for instance, to choose among a set of keys within a JWK Set\nduring key rollover. The structure of the \"kid\" value is\nunspecified. When \"kid\" values are used within a JWK Set, different\nkeys within the JWK Set SHOULD use distinct \"kid\" values. (One\nexample in which different keys might use the same \"kid\" value is if\nthey have different \"kty\" (key type) values but are considered to be\nequivalent alternatives by the application using them.) The \"kid\"\nvalue is a case-sensitive string.",
+ "type": "string",
+ "x-go-name": "Kid"
+ },
+ "kty": {
+ "description": "The \"kty\" (key type) parameter identifies the cryptographic algorithm\nfamily used with the key, such as \"RSA\" or \"EC\". \"kty\" values should\neither be registered in the IANA \"JSON Web Key Types\" registry\nestablished by [JWA] or be a value that contains a Collision-\nResistant Name. The \"kty\" value is a case-sensitive string.",
+ "type": "string",
+ "x-go-name": "Kty"
+ },
+ "n": {
+ "type": "string",
+ "x-go-name": "N"
+ },
+ "p": {
+ "type": "string",
+ "x-go-name": "P"
+ },
+ "q": {
+ "type": "string",
+ "x-go-name": "Q"
+ },
+ "qi": {
+ "type": "string",
+ "x-go-name": "Qi"
+ },
+ "use": {
+ "description": "The \"use\" (public key use) parameter identifies the intended use of\nthe public key. The \"use\" parameter is employed to indicate whether\na public key is used for encrypting data or verifying the signature\non data. Values are commonly \"sig\" (signature) or \"enc\" (encryption).",
+ "type": "string",
+ "x-go-name": "Use"
+ },
+ "x": {
+ "type": "string",
+ "x-go-name": "X"
+ },
+ "x5c": {
+ "description": "The \"x5c\" (X.509 certificate chain) parameter contains a chain of one\nor more PKIX certificates [RFC5280]. The certificate chain is\nrepresented as a JSON array of certificate value strings. Each\nstring in the array is a base64-encoded (Section 4 of [RFC4648] --\nnot base64url-encoded) DER [ITU.X690.1994] PKIX certificate value.\nThe PKIX certificate containing the key value MUST be the first\ncertificate.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "X5c"
+ },
+ "y": {
+ "type": "string",
+ "x-go-name": "Y"
+ }
+ },
+ "x-go-name": "swaggerJSONWebKey",
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "jsonWebKeySet": {
+ "type": "object",
+ "properties": {
+ "keys": {
+ "description": "The value of the \"keys\" parameter is an array of JWK values. By\ndefault, the order of the JWK values within the array does not imply\nan order of preference among them, although applications of JWK Sets\ncan choose to assign a meaning to the order for their purposes, if\ndesired.",
+ "type": "array",
+ "items": {
+ "$ref": "#/definitions/jsonWebKey"
+ },
+ "x-go-name": "Keys"
+ }
+ },
+ "x-go-name": "swaggerJSONWebKeySet",
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "jsonWebKeySetGeneratorRequest": {
+ "type": "object",
+ "required": [
+ "alg",
+ "kid"
+ ],
+ "properties": {
+ "alg": {
+ "description": "The algorithm to be used for creating the key. Supports \"RS256\", \"ES521\" and \"HS256\"",
+ "type": "string",
+ "x-go-name": "Algorithm"
+ },
+ "kid": {
+ "description": "The kid of the key to be created",
+ "type": "string",
+ "x-go-name": "KeyID"
+ }
+ },
+ "x-go-name": "createRequest",
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "oAuth2Client": {
+ "type": "object",
+ "title": "Client represents an OAuth 2.0 Client.",
+ "properties": {
+ "client_name": {
+ "description": "Name is the human-readable string name of the client to be presented to the\nend-user during authorization.",
+ "type": "string",
+ "x-go-name": "Name"
+ },
+ "client_secret": {
+ "description": "Secret is the client's secret. The secret will be included in the create request as cleartext, and then\nnever again. The secret is stored using BCrypt so it is impossible to recover it. Tell your users\nthat they need to write the secret down as it will not be made available again.",
+ "type": "string",
+ "x-go-name": "Secret"
+ },
+ "client_uri": {
+ "description": "ClientURI is an URL string of a web page providing information about the client.\nIf present, the server SHOULD display this URL to the end-user in\na clickable fashion.",
+ "type": "string",
+ "x-go-name": "ClientURI"
+ },
+ "contacts": {
+ "description": "Contacts is a array of strings representing ways to contact people responsible\nfor this client, typically email addresses.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "Contacts"
+ },
+ "grant_types": {
+ "description": "GrantTypes is an array of grant types the client is allowed to use.",
+ "type": "array",
+ "pattern": "client_credentials|authorize_code|implicit|refresh_token",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "GrantTypes"
+ },
+ "id": {
+ "description": "ID is the id for this client.",
+ "type": "string",
+ "x-go-name": "ID"
+ },
+ "logo_uri": {
+ "description": "LogoURI is an URL string that references a logo for the client.",
+ "type": "string",
+ "x-go-name": "LogoURI"
+ },
+ "owner": {
+ "description": "Owner is a string identifying the owner of the OAuth 2.0 Client.",
+ "type": "string",
+ "x-go-name": "Owner"
+ },
+ "policy_uri": {
+ "description": "PolicyURI is a URL string that points to a human-readable privacy policy document\nthat describes how the deployment organization collects, uses,\nretains, and discloses personal data.",
+ "type": "string",
+ "x-go-name": "PolicyURI"
+ },
+ "public": {
+ "description": "Public is a boolean that identifies this client as public, meaning that it\ndoes not have a secret. It will disable the client_credentials grant type for this client if set.",
+ "type": "boolean",
+ "x-go-name": "Public"
+ },
+ "redirect_uris": {
+ "description": "RedirectURIs is an array of allowed redirect urls for the client, for example: http://mydomain/oauth/callback .",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "RedirectURIs"
+ },
+ "response_types": {
+ "description": "ResponseTypes is an array of the OAuth 2.0 response type strings that the client can\nuse at the authorization endpoint.",
+ "type": "array",
+ "pattern": "id_token|code|token",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "ResponseTypes"
+ },
+ "scope": {
+ "description": "Scope is a string containing a space-separated list of scope values (as\ndescribed in Section 3.3 of OAuth 2.0 [RFC6749]) that the client\ncan use when requesting access tokens.",
+ "type": "string",
+ "pattern": "([a-zA-Z0-9\\.\\*]+\\s)+",
+ "x-go-name": "Scope"
+ },
+ "tos_uri": {
+ "description": "TermsOfServiceURI is a URL string that points to a human-readable terms of service\ndocument for the client that describes a contractual relationship\nbetween the end-user and the client that the end-user accepts when\nauthorizing the client.",
+ "type": "string",
+ "x-go-name": "TermsOfServiceURI"
+ }
+ },
+ "x-go-name": "Client",
+ "x-go-package": "github.com/ory/hydra/client"
+ },
+ "oAuth2TokenIntrospection": {
+ "type": "object",
+ "properties": {
+ "active": {
+ "description": "Active is a boolean indicator of whether or not the presented token\nis currently active. The specifics of a token's \"active\" state\nwill vary depending on the implementation of the authorization\nserver and the information it keeps about its tokens, but a \"true\"\nvalue return for the \"active\" property will generally indicate\nthat a given token has been issued by this authorization server,\nhas not been revoked by the resource owner, and is within its\ngiven time window of validity (e.g., after its issuance time and\nbefore its expiration time).",
+ "type": "boolean",
+ "x-go-name": "Active"
+ },
+ "aud": {
+ "description": "Audience is a service-specific string identifier or list of string\nidentifiers representing the intended audience for this token.",
+ "type": "string",
+ "x-go-name": "Audience"
+ },
+ "client_id": {
+ "description": "ClientID is aclient identifier for the OAuth 2.0 client that\nrequested this token.",
+ "type": "string",
+ "x-go-name": "ClientID"
+ },
+ "exp": {
+ "description": "Expires at is an integer timestamp, measured in the number of seconds\nsince January 1 1970 UTC, indicating when this token will expire.",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "ExpiresAt"
+ },
+ "ext": {
+ "description": "Extra is arbitrary data set by the session.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "Extra"
+ },
+ "iat": {
+ "description": "Issued at is an integer timestamp, measured in the number of seconds\nsince January 1 1970 UTC, indicating when this token was\noriginally issued.",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "IssuedAt"
+ },
+ "iss": {
+ "description": "Issuer is a string representing the issuer of this token",
+ "type": "string",
+ "x-go-name": "Issuer"
+ },
+ "nbf": {
+ "description": "NotBefore is an integer timestamp, measured in the number of seconds\nsince January 1 1970 UTC, indicating when this token is not to be\nused before.",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "NotBefore"
+ },
+ "scope": {
+ "description": "Scope is a JSON string containing a space-separated list of\nscopes associated with this token.",
+ "type": "string",
+ "x-go-name": "Scope"
+ },
+ "sub": {
+ "description": "Subject of the token, as defined in JWT [RFC7519].\nUsually a machine-readable identifier of the resource owner who\nauthorized this token.",
+ "type": "string",
+ "x-go-name": "Subject"
+ },
+ "username": {
+ "description": "Username is a human-readable identifier for the resource owner who\nauthorized this token.",
+ "type": "string",
+ "x-go-name": "Username"
+ }
+ },
+ "x-go-name": "swaggerOAuthIntrospectionResponsePayload",
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "oAuth2consentRequest": {
+ "type": "object",
+ "title": "ConsentRequest represents a consent request.",
+ "properties": {
+ "audience": {
+ "description": "Audience is the client id that initiated the OAuth2 request.",
+ "type": "string",
+ "x-go-name": "Audience"
+ },
+ "expiresAt": {
+ "description": "ExpiresAt is the time where the access request will expire.",
+ "x-go-name": "ExpiresAt"
+ },
+ "id": {
+ "description": "ID is the id of this consent request.",
+ "type": "string",
+ "x-go-name": "ID"
+ },
+ "redirectUrl": {
+ "description": "Redirect URL is the URL where the user agent should be redirected to after the consent has been\naccepted or rejected.",
+ "type": "string",
+ "x-go-name": "RedirectURL"
+ },
+ "requestedScopes": {
+ "description": "RequestedScopes represents a list of scopes that have been requested by the OAuth2 request initiator.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "RequestedScopes"
+ }
+ },
+ "x-go-name": "ConsentRequest",
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "policy": {
+ "type": "object",
+ "properties": {
+ "actions": {
+ "description": "Actions impacted by the policy.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "Actions"
+ },
+ "conditions": {
+ "description": "Conditions under which the policy is active.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object",
+ "properties": {
+ "options": {
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "Options"
+ },
+ "type": {
+ "type": "string",
+ "x-go-name": "Type"
+ }
+ }
+ },
+ "x-go-name": "Conditions"
+ },
+ "description": {
+ "description": "Description of the policy.",
+ "type": "string",
+ "x-go-name": "Description"
+ },
+ "effect": {
+ "description": "Effect of the policy",
+ "type": "string",
+ "x-go-name": "Effect"
+ },
+ "id": {
+ "description": "ID of the policy.",
+ "type": "string",
+ "x-go-name": "ID"
+ },
+ "resources": {
+ "description": "Resources impacted by the policy.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "Resources"
+ },
+ "subjects": {
+ "description": "Subjects impacted by the policy.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "Subjects"
+ }
+ },
+ "x-go-name": "swaggerPolicy",
+ "x-go-package": "github.com/ory/hydra/policy"
+ },
+ "swaggerAcceptConsentRequest": {
+ "type": "object",
+ "required": [
+ "id",
+ "Body"
+ ],
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/consentRequestAcceptance"
+ },
+ "id": {
+ "description": "in: path",
+ "type": "string",
+ "x-go-name": "ID"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "swaggerCreatePolicyParameters": {
+ "type": "object",
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/policy"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/policy"
+ },
+ "swaggerDoesWardenAllowAccessRequestParameters": {
+ "type": "object",
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/wardenAccessRequest"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/warden"
+ },
+ "swaggerDoesWardenAllowTokenAccessRequestParameters": {
+ "type": "object",
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/wardenTokenAccessRequest"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/warden"
+ },
+ "swaggerGetPolicyParameters": {
+ "type": "object",
+ "properties": {
+ "id": {
+ "description": "The id of the policy.\nin: path",
+ "type": "string",
+ "x-go-name": "ID"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/policy"
+ },
+ "swaggerJsonWebKeyQuery": {
+ "type": "object",
+ "required": [
+ "kid",
+ "set"
+ ],
+ "properties": {
+ "kid": {
+ "description": "The kid of the desired key\nin: path",
+ "type": "string",
+ "x-go-name": "KID"
+ },
+ "set": {
+ "description": "The set\nin: path",
+ "type": "string",
+ "x-go-name": "Set"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "swaggerJwkCreateSet": {
+ "type": "object",
+ "required": [
+ "set"
+ ],
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/jsonWebKeySetGeneratorRequest"
+ },
+ "set": {
+ "description": "The set\nin: path",
+ "type": "string",
+ "x-go-name": "Set"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "swaggerJwkSetQuery": {
+ "type": "object",
+ "required": [
+ "set"
+ ],
+ "properties": {
+ "set": {
+ "description": "The set\nin: path",
+ "type": "string",
+ "x-go-name": "Set"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "swaggerJwkUpdateSet": {
+ "type": "object",
+ "required": [
+ "set"
+ ],
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/jsonWebKeySet"
+ },
+ "set": {
+ "description": "The set\nin: path",
+ "type": "string",
+ "x-go-name": "Set"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "swaggerJwkUpdateSetKey": {
+ "type": "object",
+ "required": [
+ "kid",
+ "set"
+ ],
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/jsonWebKey"
+ },
+ "kid": {
+ "description": "The kid of the desired key\nin: path",
+ "type": "string",
+ "x-go-name": "KID"
+ },
+ "set": {
+ "description": "The set\nin: path",
+ "type": "string",
+ "x-go-name": "Set"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/jwk"
+ },
+ "swaggerListPolicyParameters": {
+ "type": "object",
+ "properties": {
+ "limit": {
+ "description": "The maximum amount of policies returned.\nin: query",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "Limit"
+ },
+ "offset": {
+ "description": "The offset from where to start looking.\nin: query",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "Offset"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/policy"
+ },
+ "swaggerListPolicyResponse": {
+ "description": "A policy",
+ "type": "object",
+ "properties": {
+ "Body": {
+ "description": "in: body\ntype: array",
+ "type": "array",
+ "items": {
+ "$ref": "#/definitions/policy"
+ }
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/policy"
+ },
+ "swaggerOAuthConsentRequest": {
+ "description": "The consent request response",
+ "type": "object",
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/oAuth2consentRequest"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "swaggerOAuthConsentRequestPayload": {
+ "type": "object",
+ "required": [
+ "id"
+ ],
+ "properties": {
+ "id": {
+ "description": "The id of the OAuth 2.0 Consent Request.",
+ "type": "string",
+ "uniqueItems": true,
+ "x-go-name": "ID"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "swaggerOAuthIntrospectionRequest": {
+ "type": "object",
+ "required": [
+ "token"
+ ],
+ "properties": {
+ "scope": {
+ "description": "An optional, space separated list of required scopes. If the access token was not granted one of the\nscopes, the result of active will be false.\n\nin: formData",
+ "type": "string",
+ "x-go-name": "Scope"
+ },
+ "token": {
+ "description": "The string value of the token. For access tokens, this\nis the \"access_token\" value returned from the token endpoint\ndefined in OAuth 2.0 [RFC6749], Section 5.1.\nThis endpoint DOES NOT accept refresh tokens for validation.",
+ "type": "string",
+ "x-go-name": "Token"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "swaggerOAuthIntrospectionResponse": {
+ "description": "The token introspection response",
+ "type": "object",
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/oAuth2TokenIntrospection"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "swaggerOAuthTokenResponse": {
+ "description": "The token response",
+ "type": "object",
+ "properties": {
+ "Body": {
+ "description": "in: body",
+ "type": "object",
+ "properties": {
+ "access_token": {
+ "description": "The access token issued by the authorization server.",
+ "type": "string",
+ "x-go-name": "AccessToken"
+ },
+ "expires_in": {
+ "description": "The lifetime in seconds of the access token. For\nexample, the value \"3600\" denotes that the access token will\nexpire in one hour from the time the response was generated.",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "ExpiresIn"
+ },
+ "id_token": {
+ "description": "To retrieve a refresh token request the id_token scope.",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "IDToken"
+ },
+ "refresh_token": {
+ "description": "The refresh token, which can be used to obtain new\naccess tokens. To retrieve it add the scope \"offline\" to your access token request.",
+ "type": "string",
+ "x-go-name": "RefreshToken"
+ },
+ "scope": {
+ "description": "The scope of the access token",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "Scope"
+ },
+ "token_type": {
+ "description": "The type of the token issued",
+ "type": "string",
+ "x-go-name": "TokenType"
+ }
+ }
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "swaggerRejectConsentRequest": {
+ "type": "object",
+ "required": [
+ "id",
+ "Body"
+ ],
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/consentRequestRejection"
+ },
+ "id": {
+ "description": "in: path",
+ "type": "string",
+ "x-go-name": "ID"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "swaggerRevokeOAuth2TokenParameters": {
+ "type": "object",
+ "required": [
+ "token"
+ ],
+ "properties": {
+ "token": {
+ "description": "in: formData",
+ "type": "string",
+ "x-go-name": "Token"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ },
+ "swaggerUpdatePolicyParameters": {
+ "type": "object",
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/policy"
+ },
+ "id": {
+ "description": "The id of the policy.\nin: path",
+ "type": "string",
+ "x-go-name": "ID"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/policy"
+ },
+ "swaggerWardenAccessRequestResponseParameters": {
+ "type": "object",
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/wardenAccessRequestResponse"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/warden"
+ },
+ "swaggerWardenTokenAccessRequestResponse": {
+ "type": "object",
+ "properties": {
+ "Body": {
+ "$ref": "#/definitions/wardenTokenAccessRequestResponsePayload"
+ }
+ },
+ "x-go-package": "github.com/ory/hydra/warden"
+ },
+ "tokenAllowedRequest": {
+ "type": "object",
+ "properties": {
+ "action": {
+ "description": "Action is the action that is requested on the resource.",
+ "type": "string",
+ "x-go-name": "Action"
+ },
+ "context": {
+ "description": "Context is the request's environmental context.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "Context"
+ },
+ "resource": {
+ "description": "Resource is the resource that access is requested to.",
+ "type": "string",
+ "x-go-name": "Resource"
+ }
+ },
+ "x-go-name": "TokenAccessRequest",
+ "x-go-package": "github.com/ory/hydra/firewall"
+ },
+ "wardenAccessRequest": {
+ "type": "object",
+ "title": "AccessRequest is the warden's request object.",
+ "properties": {
+ "action": {
+ "description": "Action is the action that is requested on the resource.",
+ "type": "string",
+ "x-go-name": "Action"
+ },
+ "context": {
+ "description": "Context is the request's environmental context.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "Context"
+ },
+ "resource": {
+ "description": "Resource is the resource that access is requested to.",
+ "type": "string",
+ "x-go-name": "Resource"
+ },
+ "subject": {
+ "description": "Subejct is the subject that is requesting access.",
+ "type": "string",
+ "x-go-name": "Subject"
+ }
+ },
+ "x-go-name": "AccessRequest",
+ "x-go-package": "github.com/ory/hydra/firewall"
+ },
+ "wardenAccessRequestResponse": {
+ "type": "object",
+ "properties": {
+ "allowed": {
+ "description": "Allowed is true if the request is allowed and false otherwise.",
+ "type": "boolean",
+ "x-go-name": "Allowed"
+ }
+ },
+ "x-go-name": "swaggerWardenAccessRequestResponse",
+ "x-go-package": "github.com/ory/hydra/warden"
+ },
+ "wardenTokenAccessRequest": {
+ "type": "object",
+ "properties": {
+ "action": {
+ "description": "Action is the action that is requested on the resource.",
+ "type": "string",
+ "x-go-name": "Action"
+ },
+ "context": {
+ "description": "Context is the request's environmental context.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "Context"
+ },
+ "resource": {
+ "description": "Resource is the resource that access is requested to.",
+ "type": "string",
+ "x-go-name": "Resource"
+ },
+ "scopes": {
+ "description": "Scopes is an array of scopes that are requried.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "Scopes"
+ },
+ "token": {
+ "description": "Token is the token to introspect.",
+ "type": "string",
+ "x-go-name": "Token"
+ }
+ },
+ "x-go-name": "swaggerWardenTokenAccessRequest",
+ "x-go-package": "github.com/ory/hydra/warden"
+ },
+ "wardenTokenAccessRequestResponsePayload": {
+ "type": "object",
+ "properties": {
+ "allowed": {
+ "description": "Allowed is true if the request is allowed and false otherwise.",
+ "type": "boolean",
+ "x-go-name": "Allowed"
+ },
+ "aud": {
+ "description": "Audience is who the token was issued for. This is an OAuth2 app usually.",
+ "type": "string",
+ "x-go-name": "Audience"
+ },
+ "exp": {
+ "description": "ExpiresAt is the expiry timestamp.",
+ "type": "string",
+ "x-go-name": "ExpiresAt"
+ },
+ "ext": {
+ "description": "Extra represents arbitrary session data.",
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ },
+ "x-go-name": "Extra"
+ },
+ "iat": {
+ "description": "IssuedAt is the token creation time stamp.",
+ "type": "string",
+ "x-go-name": "IssuedAt"
+ },
+ "iss": {
+ "description": "Issuer is the id of the issuer, typically an hydra instance.",
+ "type": "string",
+ "x-go-name": "Issuer"
+ },
+ "scopes": {
+ "description": "GrantedScopes is a list of scopes that the subject authorized when asked for consent.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "GrantedScopes"
+ },
+ "sub": {
+ "description": "Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app.\nThis is usually a uuid but you can choose a urn or some other id too.",
+ "type": "string",
+ "x-go-name": "Subject"
+ }
+ },
+ "x-go-name": "swaggerWardenTokenAccessRequestResponsePayload",
+ "x-go-package": "github.com/ory/hydra/warden"
+ },
+ "wellKnown": {
+ "type": "object",
+ "required": [
+ "issuer",
+ "authorization_endpoint",
+ "token_endpoint",
+ "jwks_uri",
+ "subject_types_supported",
+ "id_token_signing_alg_values_supported",
+ "response_types_supported"
+ ],
+ "properties": {
+ "authorization_endpoint": {
+ "description": "URL of the OP's OAuth 2.0 Authorization Endpoint",
+ "type": "string",
+ "x-go-name": "AuthURL"
+ },
+ "id_token_signing_alg_values_supported": {
+ "description": "JSON array containing a list of the JWS signing algorithms (alg values) supported by the OP for the ID Token\nto encode the Claims in a JWT [JWT]. The algorithm RS256 MUST be included. The value none MAY be supported,\nbut MUST NOT be used unless the Response Type used returns no ID Token from the Authorization Endpoint\n(such as when using the Authorization Code Flow).",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "SigningAlgs"
+ },
+ "issuer": {
+ "description": "URL using the https scheme with no query or fragment component that the OP asserts as its Issuer Identifier.\nIf Issuer discovery is supported , this value MUST be identical to the issuer value returned\nby WebFinger. This also MUST be identical to the iss Claim value in ID Tokens issued from this Issuer.",
+ "type": "string",
+ "x-go-name": "Issuer"
+ },
+ "jwks_uri": {
+ "description": "URL of the OP's JSON Web Key Set [JWK] document. This contains the signing key(s) the RP uses to validate\nsignatures from the OP. The JWK Set MAY also contain the Server's encryption key(s), which are used by RPs\nto encrypt requests to the Server. When both signing and encryption keys are made available, a use (Key Use)\nparameter value is REQUIRED for all keys in the referenced JWK Set to indicate each key's intended usage.\nAlthough some algorithms allow the same key to be used for both signatures and encryption, doing so is\nNOT RECOMMENDED, as it is less secure. The JWK x5c parameter MAY be used to provide X.509 representations of\nkeys provided. When used, the bare key values MUST still be present and MUST match those in the certificate.",
+ "type": "string",
+ "x-go-name": "JWKsURI"
+ },
+ "response_types_supported": {
+ "description": "JSON array containing a list of the OAuth 2.0 response_type values that this OP supports. Dynamic OpenID\nProviders MUST support the code, id_token, and the token id_token Response Type values.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "ResponseTypes"
+ },
+ "subject_types_supported": {
+ "description": "JSON array containing a list of the Subject Identifier types that this OP supports. Valid types include\npairwise and public.",
+ "type": "array",
+ "items": {
+ "type": "string"
+ },
+ "x-go-name": "SubjectTypes"
+ },
+ "token_endpoint": {
+ "description": "URL of the OP's OAuth 2.0 Token Endpoint",
+ "type": "string",
+ "x-go-name": "TokenURL"
+ }
+ },
+ "x-go-name": "WellKnown",
+ "x-go-package": "github.com/ory/hydra/oauth2"
+ }
+ },
+ "responses": {
+ "emptyResponse": {
+ "description": "An empty response"
+ },
+ "findGroupsByMemberResponse": {
+ "description": "A list of groups the member is belonging to",
+ "schema": {
+ "type": "array",
+ "items": {
+ "$ref": "#/definitions/group"
+ }
+ }
+ },
+ "genericError": {
+ "description": "The standard error format",
+ "schema": {
+ "type": "object",
+ "properties": {
+ "code": {
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "Code"
+ },
+ "details": {
+ "type": "array",
+ "items": {
+ "type": "object",
+ "additionalProperties": {
+ "type": "object"
+ }
+ },
+ "x-go-name": "Details"
+ },
+ "message": {
+ "type": "string",
+ "x-go-name": "Message"
+ },
+ "reason": {
+ "type": "string",
+ "x-go-name": "Reason"
+ },
+ "request": {
+ "type": "string",
+ "x-go-name": "Request"
+ },
+ "status": {
+ "type": "string",
+ "x-go-name": "Status"
+ }
+ }
+ }
+ },
+ "groupResponse": {
+ "description": "A group",
+ "schema": {
+ "$ref": "#/definitions/group"
+ }
+ },
+ "healthStatus": {
+ "description": "A list of clients.",
+ "schema": {
+ "type": "object",
+ "properties": {
+ "status": {
+ "description": "Status always contains \"ok\"",
+ "type": "string",
+ "x-go-name": "Status"
+ }
+ }
+ }
+ },
+ "introspectOAuth2TokenResponse": {
+ "description": "The token introspection response",
+ "schema": {
+ "$ref": "#/definitions/oAuth2TokenIntrospection"
+ }
+ },
+ "oAuth2ClientList": {
+ "description": "A list of clients.",
+ "schema": {
+ "type": "array",
+ "items": {
+ "$ref": "#/definitions/oAuth2Client"
+ }
+ }
+ },
+ "oAuth2ConsentRequest": {
+ "description": "The consent request response",
+ "schema": {
+ "$ref": "#/definitions/oAuth2consentRequest"
+ }
+ },
+ "oauthTokenResponse": {
+ "description": "The token response",
+ "schema": {
+ "type": "object",
+ "properties": {
+ "access_token": {
+ "description": "The access token issued by the authorization server.",
+ "type": "string",
+ "x-go-name": "AccessToken"
+ },
+ "expires_in": {
+ "description": "The lifetime in seconds of the access token. For\nexample, the value \"3600\" denotes that the access token will\nexpire in one hour from the time the response was generated.",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "ExpiresIn"
+ },
+ "id_token": {
+ "description": "To retrieve a refresh token request the id_token scope.",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "IDToken"
+ },
+ "refresh_token": {
+ "description": "The refresh token, which can be used to obtain new\naccess tokens. To retrieve it add the scope \"offline\" to your access token request.",
+ "type": "string",
+ "x-go-name": "RefreshToken"
+ },
+ "scope": {
+ "description": "The scope of the access token",
+ "type": "integer",
+ "format": "int64",
+ "x-go-name": "Scope"
+ },
+ "token_type": {
+ "description": "The type of the token issued",
+ "type": "string",
+ "x-go-name": "TokenType"
+ }
+ }
+ }
+ },
+ "policyList": {
+ "description": "A policy",
+ "schema": {
+ "type": "array",
+ "items": {
+ "$ref": "#/definitions/policy"
+ }
+ }
+ },
+ "wardenAccessRequestResponse": {
+ "schema": {
+ "$ref": "#/definitions/wardenAccessRequestResponse"
+ }
+ },
+ "wardenTokenAccessRequestResponse": {
+ "schema": {
+ "$ref": "#/definitions/wardenTokenAccessRequestResponsePayload"
+ }
+ }
+ },
+ "securityDefinitions": {
+ "basic": {
+ "type": "basic"
+ },
+ "oauth2": {
+ "type": "oauth2",
+ "flow": "accessCode",
+ "authorizationUrl": "https://your-hydra-instance.com/oauth2/auth",
+ "tokenUrl": "https://your-hydra-instance.com/oauth2/token",
+ "scopes": {
+ "hydra.clients": "A scope required to manage OAuth 2.0 Clients",
+ "hydra.consent": "A scope required to fetch and modify consent requests",
+ "hydra.groups": "A scope required to manage warden groups",
+ "hydra.health": "A scope required to get health information",
+ "hydra.keys.create": "A scope required to create JSON Web Keys",
+ "hydra.keys.delete": "A scope required to delete JSON Web Keys",
+ "hydra.keys.get": "A scope required to fetch JSON Web Keys",
+ "hydra.keys.update": "A scope required to get JSON Web Keys",
+ "hydra.policies": "A scope required to manage access control policies",
+ "hydra.warden": "A scope required to make access control inquiries",
+ "offline": "A scope required when requesting refresh tokens",
+ "openid": "Request an OpenID Connect ID Token"
+ }
+ }
+ },
+ "x-forwarded-proto": "string",
+ "x-request-id": "string"
+}
\ No newline at end of file
diff --git a/firewall/warden.go b/firewall/warden.go
index c8bbb67c9d4..d0ae9055867 100644
--- a/firewall/warden.go
+++ b/firewall/warden.go
@@ -34,7 +34,7 @@ type Context struct {
// AccessRequest is the warden's request object.
//
-// swagger:model allowedRequest
+// swagger:model wardenAccessRequest
type AccessRequest struct {
// Resource is the resource that access is requested to.
Resource string `json:"resource"`
diff --git a/glide.lock b/glide.lock
index 1403273701f..6c35ad0e63e 100644
--- a/glide.lock
+++ b/glide.lock
@@ -1,5 +1,5 @@
-hash: 52c78c986cdee750ca57036d3dca3507e23f03b2c85def17f33479d38a9ff08b
-updated: 2017-09-16T13:19:28.6519803+02:00
+hash: c4be9b74aa40e60d7722f40bf12dd531809a39647f5b1958345598024b9e8509
+updated: 2017-09-28T14:15:00.3622344+02:00
imports:
- name: github.com/asaskevich/govalidator
version: 4918b99a7cb949bb295f3c7bbaf24b577d806e35
@@ -53,6 +53,8 @@ imports:
version: 4da3e2cfbabc9f751898f250b49f2439785783a1
- name: github.com/fsouza/go-dockerclient
version: 98edf3edfae6a6500fecc69d2bcccf1302544004
+- name: github.com/go-resty/resty
+ version: 9ac9c42358f7c3c69ac9f8610e8790d7c338e85d
- name: github.com/go-sql-driver/mysql
version: a0583e0143b1624142adab07e0e97fe106d99561
- name: github.com/golang/protobuf
@@ -158,12 +160,12 @@ imports:
version: 2d840d861c322bdf5346ba7917af1c2285e653d3
- name: github.com/segmentio/backo-go
version: 204274ad699c0983a70203a566887f17a717fef4
+- name: github.com/sirupsen/logrus
+ version: 89742aefa4b206dcf400792f3bd35b542998eb3b
- name: github.com/Sirupsen/logrus
version: 89742aefa4b206dcf400792f3bd35b542998eb3b
repo: https://github.com/sirupsen/logrus.git
vcs: git
-- name: github.com/sirupsen/logrus
- version: 89742aefa4b206dcf400792f3bd35b542998eb3b
- name: github.com/spf13/afero
version: ee1bd8ee15a1306d1f9201acc41ef39cd9f99a1b
subpackages:
@@ -204,6 +206,7 @@ imports:
subpackages:
- context
- context/ctxhttp
+ - publicsuffix
- name: golang.org/x/oauth2
version: 13449ad91cb26cb47661c1b080790392170385fd
subpackages:
diff --git a/glide.yaml b/glide.yaml
index 4e8d2b37519..3f41a9413a5 100644
--- a/glide.yaml
+++ b/glide.yaml
@@ -19,6 +19,8 @@ import:
- package: github.com/jmoiron/sqlx
- package: github.com/julienschmidt/httprouter
version: 1.1.0
+- package: github.com/go-resty/resty
+ version: 1.0.0
- package: github.com/lib/pq
- package: github.com/meatballhat/negroni-logrus
- package: github.com/moul/http2curl
diff --git a/health/doc.go b/health/doc.go
index a7e15716476..b1dcbfe9640 100644
--- a/health/doc.go
+++ b/health/doc.go
@@ -1,8 +1,11 @@
package health
// A list of clients.
-// swagger:response clientsList
+// swagger:response healthStatus
type swaggerListClientsResult struct {
// in: body
- Body struct{}
+ Body struct {
+ // Status always contains "ok"
+ Status string `json:"status"`
+ }
}
diff --git a/health/handler.go b/health/handler.go
index df0b3d6f238..bc6dce9eee4 100644
--- a/health/handler.go
+++ b/health/handler.go
@@ -16,24 +16,36 @@ type Handler struct {
}
func (h *Handler) SetRoutes(r *httprouter.Router) {
- r.GET("/health", h.Health)
- r.GET("/health/stats", h.Statistics)
+ r.GET("/health/status", h.Health)
+ r.GET("/health/metrics", h.Statistics)
}
-// swagger:route GET /health health
+// swagger:route GET /health/status health getInstanceStatus
//
-// Check health status of instance
+// Check health status of this instance
+//
+// This endpoint returns `{ "status": "ok" }`. This status let's you know that the HTTP server is up and running. This
+// status does currently not include checks whether the database connection is up and running. This endpoint does not
+// require the `X-Forwarded-Proto` header when TLS termination is set.
+//
+//
+// Be aware that if you are running multiple nodes of ORY Hydra, the health status will never refer to the cluster state,
+// only to a single instance.
//
// Responses:
-// 204: emptyResponse
+// 200: healthStatus
// 500: genericError
func (h *Handler) Health(rw http.ResponseWriter, r *http.Request, _ httprouter.Params) {
- rw.Write([]byte("ok"))
+ rw.Write([]byte(`{"status": "ok"}`))
}
-// swagger:route GET /health/stats health getStatistics
+// swagger:route GET /health/metrics health getInstanceMetrics
+//
+// Show instance metrics (experimental)
+//
+// This endpoint returns an instance's metrics, such as average response time, status code distribution, hits per
+// second and so on. The return values are currently not documented as this endpoint is still experimental.
//
-// Show instance statistics
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -57,7 +69,7 @@ func (h *Handler) Health(rw http.ResponseWriter, r *http.Request, _ httprouter.P
// oauth2: hydra.health
//
// Responses:
-// 200: clientsList
+// 200: emptyResponse
// 401: genericError
// 403: genericError
// 500: genericError
diff --git a/jwk/doc.go b/jwk/doc.go
index 7ba992c7301..5e2e7880b09 100644
--- a/jwk/doc.go
+++ b/jwk/doc.go
@@ -1,7 +1,13 @@
+// Package jwk implements JSON Web Key management capabilities
+//
+// A JSON Web Key (JWK) is a JavaScript Object Notation (JSON) data
+// structure that represents a cryptographic key. A JWK Set is a JSON data structure that
+// represents a set of JWKs. A JSON Web Key is identified by its set and key id. ORY Hydra uses this functionality
+// to store cryptographic keys used for TLS and JSON Web Tokens (such as OpenID Connect ID tokens).
package jwk
-// swagger:parameters getJwkSetKey deleteJwkKey
-type swaggerJwkSetKeyQuery struct {
+// swagger:parameters getJsonWebKey deleteJsonWebKey
+type swaggerJsonWebKeyQuery struct {
// The kid of the desired key
// in: path
// required: true
@@ -13,7 +19,7 @@ type swaggerJwkSetKeyQuery struct {
Set string `json:"set"`
}
-// swagger:parameters updateJwkSet
+// swagger:parameters updateJsonWebKeySet
type swaggerJwkUpdateSet struct {
// The set
// in: path
@@ -24,8 +30,8 @@ type swaggerJwkUpdateSet struct {
Body swaggerJSONWebKeySet
}
-// swagger:parameters updateJwkKey
-type swaggerJwkUpdateKey struct {
+// swagger:parameters updateJsonWebKey
+type swaggerJwkUpdateSetKey struct {
// The kid of the desired key
// in: path
// required: true
@@ -37,11 +43,11 @@ type swaggerJwkUpdateKey struct {
Set string `json:"set"`
// in: body
- Body swaggerJSONWebKeySet
+ Body swaggerJSONWebKey
}
-// swagger:parameters createJwkKey
-type swaggerJwkCreateKey struct {
+// swagger:parameters createJsonWebKeySet
+type swaggerJwkCreateSet struct {
// The set
// in: path
// required: true
@@ -51,7 +57,7 @@ type swaggerJwkCreateKey struct {
Body createRequest
}
-// swagger:parameters getJwkSet deleteJwkSet
+// swagger:parameters getJsonWebKeySet deleteJsonWebKeySet
type swaggerJwkSetQuery struct {
// The set
// in: path
@@ -59,7 +65,7 @@ type swaggerJwkSetQuery struct {
Set string `json:"set"`
}
-// swagger:model jwkSet
+// swagger:model jsonWebKeySet
type swaggerJSONWebKeySet struct {
// The value of the "keys" parameter is an array of JWK values. By
// default, the order of the JWK values within the array does not imply
@@ -69,7 +75,7 @@ type swaggerJSONWebKeySet struct {
Keys []swaggerJSONWebKey `json:"keys"`
}
-// swagger:model jwk
+// swagger:model jsonWebKey
type swaggerJSONWebKey struct {
// The "use" (public key use) parameter identifies the intended use of
// the public key. The "use" parameter is employed to indicate whether
@@ -113,16 +119,16 @@ type swaggerJSONWebKey struct {
// certificate.
X5c []string `json:"x5c,omitempty"`
- K []byte `json:"k,omitempty"`
- X []byte `json:"x,omitempty"`
- Y []byte `json:"y,omitempty"`
- N []byte `json:"n,omitempty"`
- E []byte `json:"e,omitempty"`
-
- D []byte `json:"d,omitempty"`
- P []byte `json:"p,omitempty"`
- Q []byte `json:"q,omitempty"`
- Dp []byte `json:"dp,omitempty"`
- Dq []byte `json:"dq,omitempty"`
- Qi []byte `json:"qi,omitempty"`
+ K string `json:"k,omitempty"`
+ X string `json:"x,omitempty"`
+ Y string `json:"y,omitempty"`
+ N string `json:"n,omitempty"`
+ E string `json:"e,omitempty"`
+
+ D string `json:"d,omitempty"`
+ P string `json:"p,omitempty"`
+ Q string `json:"q,omitempty"`
+ Dp string `json:"dp,omitempty"`
+ Dq string `json:"dq,omitempty"`
+ Qi string `json:"qi,omitempty"`
}
diff --git a/jwk/generator_hs256.go b/jwk/generator_hs256.go
index b00b622da54..ff9615c09c7 100644
--- a/jwk/generator_hs256.go
+++ b/jwk/generator_hs256.go
@@ -29,6 +29,7 @@ func (g *HS256Generator) Generate(id string) (*jose.JsonWebKeySet, error) {
return &jose.JsonWebKeySet{
Keys: []jose.JsonWebKey{
{
+ Algorithm: "HS256",
Key: []byte(string(key)),
KeyID: id,
Certificates: []*x509.Certificate{},
diff --git a/jwk/generator_rs256.go b/jwk/generator_rs256.go
index 2bbf0a8ea38..b6d9a4b4e6b 100644
--- a/jwk/generator_rs256.go
+++ b/jwk/generator_rs256.go
@@ -31,11 +31,13 @@ func (g *RS256Generator) Generate(id string) (*jose.JsonWebKeySet, error) {
return &jose.JsonWebKeySet{
Keys: []jose.JsonWebKey{
{
+ Algorithm: "RS256",
Key: key,
KeyID: ider("private", id),
Certificates: []*x509.Certificate{},
},
{
+ Algorithm: "RS256",
Key: &key.PublicKey,
KeyID: ider("public", id),
Certificates: []*x509.Certificate{},
diff --git a/jwk/handler.go b/jwk/handler.go
index 0770a1573d1..65a91543d56 100644
--- a/jwk/handler.go
+++ b/jwk/handler.go
@@ -51,6 +51,7 @@ func (h *Handler) SetRoutes(r *httprouter.Router) {
r.DELETE("/keys/:set", h.DeleteKeySet)
}
+// swagger:model jsonWebKeySetGeneratorRequest
type createRequest struct {
// The algorithm to be used for creating the key. Supports "RS256", "ES521" and "HS256"
// required: true
@@ -67,11 +68,9 @@ type joseWebKeySetRequest struct {
Keys []json.RawMessage `json:"keys"`
}
-// swagger:route GET /.well-known/jwks.json jwks oauth2 openid-connect WellKnown
+// swagger:route GET /.well-known/jwks.json oAuth2 wellKnown
//
-// Public JWKs
-//
-// Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own.
+// Get list of well known JSON Web Keys
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -95,7 +94,7 @@ type joseWebKeySetRequest struct {
// oauth2: hydra.keys.get
//
// Responses:
-// 200: jwkSet
+// 200: jsonWebKeySet
// 401: genericError
// 403: genericError
// 500: genericError
@@ -126,9 +125,12 @@ func (h *Handler) WellKnown(w http.ResponseWriter, r *http.Request, ps httproute
h.H.Write(w, r, keys)
}
-// swagger:route GET /keys/{set}/{kid} jwks getJwkSetKey
+// swagger:route GET /keys/{set}/{kid} jsonWebKey getJsonWebKey
+//
+// Retrieve a JSON Web Key
+//
+// This endpoint can be used to retrieve JWKs stored in ORY Hydra.
//
-// Retrieves a JSON Web Key Set matching the set and the kid
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -152,7 +154,7 @@ func (h *Handler) WellKnown(w http.ResponseWriter, r *http.Request, ps httproute
// oauth2: hydra.keys.get
//
// Responses:
-// 200: jwkSet
+// 200: jsonWebKeySet
// 401: genericError
// 403: genericError
// 500: genericError
@@ -186,9 +188,12 @@ func (h *Handler) GetKey(w http.ResponseWriter, r *http.Request, ps httprouter.P
h.H.Write(w, r, keys)
}
-// swagger:route GET /keys/{set} jwks getJwkSet
+// swagger:route GET /keys/{set} jsonWebKey getJsonWebKeySet
+//
+// Retrieve a JSON Web Key Set
+//
+// This endpoint can be used to retrieve JWK Sets stored in ORY Hydra.
//
-// Retrieves a JSON Web Key Set matching the set
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -212,7 +217,7 @@ func (h *Handler) GetKey(w http.ResponseWriter, r *http.Request, ps httprouter.P
// oauth2: hydra.keys.get
//
// Responses:
-// 200: jwkSet
+// 200: jsonWebKeySet
// 401: genericError
// 403: genericError
// 500: genericError
@@ -239,10 +244,17 @@ func (h *Handler) GetKeySet(w http.ResponseWriter, r *http.Request, ps httproute
h.H.Write(w, r, keys)
}
-// swagger:route POST /keys/{set} jwks createJwkKey
+// swagger:route POST /keys/{set} jsonWebKey createJsonWebKeySet
//
// Generate a new JSON Web Key
//
+// This endpoint is capable of generating JSON Web Key Sets for you. There a different strategies available, such as
+// symmetric cryptographic keys (HS256) and asymetric cryptographic keys (RS256, ECDSA).
+//
+//
+// If the specified JSON Web Key Set does not exist, it will be created.
+//
+//
// The subject making the request needs to be assigned to a policy containing:
//
// ```
@@ -265,7 +277,7 @@ func (h *Handler) GetKeySet(w http.ResponseWriter, r *http.Request, ps httproute
// oauth2: hydra.keys.create
//
// Responses:
-// 200: jwkSet
+// 200: jsonWebKeySet
// 401: genericError
// 403: genericError
// 500: genericError
@@ -306,12 +318,13 @@ func (h *Handler) Create(w http.ResponseWriter, r *http.Request, ps httprouter.P
h.H.WriteCreated(w, r, fmt.Sprintf("%s://%s/keys/%s", r.URL.Scheme, r.URL.Host, set), keys)
}
-// swagger:route PUT /keys/{set} jwks updateJwkSet
+// swagger:route PUT /keys/{set} jsonWebKey updateJsonWebKeySet
//
-// Updates a JSON Web Key Set
+// Update a JSON Web Key Set
//
// Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own.
//
+//
// The subject making the request needs to be assigned to a policy containing:
//
// ```
@@ -334,7 +347,7 @@ func (h *Handler) Create(w http.ResponseWriter, r *http.Request, ps httprouter.P
// oauth2: hydra.keys.update
//
// Responses:
-// 200: jwkSet
+// 200: jsonWebKeySet
// 401: genericError
// 403: genericError
// 500: genericError
@@ -373,12 +386,13 @@ func (h *Handler) UpdateKeySet(w http.ResponseWriter, r *http.Request, ps httpro
h.H.Write(w, r, keySet)
}
-// swagger:route PUT /keys/{set}/{kid} jwks updateJwkKey
+// swagger:route PUT /keys/{set}/{kid} jsonWebKey updateJsonWebKey
//
-// Updates a JSON Web Key
+// Update a JSON Web Key
//
// Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own.
//
+//
// The subject making the request needs to be assigned to a policy containing:
//
// ```
@@ -401,7 +415,7 @@ func (h *Handler) UpdateKeySet(w http.ResponseWriter, r *http.Request, ps httpro
// oauth2: hydra.keys.update
//
// Responses:
-// 200: jwkSet
+// 200: jsonWebKey
// 401: genericError
// 403: genericError
// 500: genericError
@@ -431,7 +445,7 @@ func (h *Handler) UpdateKey(w http.ResponseWriter, r *http.Request, ps httproute
h.H.Write(w, r, key)
}
-// swagger:route DELETE /keys/{set} jwks deleteJwkSet
+// swagger:route DELETE /keys/{set} jsonWebKey deleteJsonWebKeySet
//
// Delete a JSON Web Key
//
@@ -481,7 +495,7 @@ func (h *Handler) DeleteKeySet(w http.ResponseWriter, r *http.Request, ps httpro
w.WriteHeader(http.StatusNoContent)
}
-// swagger:route DELETE /keys/{set}/{kid} jwks deleteJwkKey
+// swagger:route DELETE /keys/{set}/{kid} jsonWebKey deleteJsonWebKey
//
// Delete a JSON Web Key
//
diff --git a/jwk/manager_http.go b/jwk/manager_http.go
deleted file mode 100644
index f572baba13c..00000000000
--- a/jwk/manager_http.go
+++ /dev/null
@@ -1,95 +0,0 @@
-package jwk
-
-import (
- "net/http"
- "net/url"
-
- "github.com/ory/hydra/pkg"
- "github.com/square/go-jose"
-)
-
-type HTTPManager struct {
- Client *http.Client
- Endpoint *url.URL
- Dry bool
- FakeTLSTermination bool
-}
-
-func (m *HTTPManager) CreateKeys(set, algorithm string) (*jose.JsonWebKeySet, error) {
- var c = struct {
- Algorithm string `json:"alg"`
- Keys []jose.JsonWebKey `json:"keys"`
- }{
- Algorithm: algorithm,
- }
-
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, set).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- if err := r.Create(&c); err != nil {
- return nil, err
- }
-
- return &jose.JsonWebKeySet{
- Keys: c.Keys,
- }, nil
-}
-
-func (m *HTTPManager) AddKey(set string, key *jose.JsonWebKey) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, set, key.KeyID).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Update(key)
-}
-
-func (m *HTTPManager) AddKeySet(set string, keys *jose.JsonWebKeySet) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, set).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Update(keys)
-}
-
-func (m *HTTPManager) GetKey(set, kid string) (*jose.JsonWebKeySet, error) {
- var c jose.JsonWebKeySet
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, set, kid).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- if err := r.Get(&c); err != nil {
- return nil, err
- }
-
- return &c, nil
-}
-
-func (m *HTTPManager) GetKeySet(set string) (*jose.JsonWebKeySet, error) {
- var c jose.JsonWebKeySet
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, set).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- if err := r.Get(&c); err != nil {
- return nil, err
- }
-
- return &c, nil
-}
-
-func (m *HTTPManager) DeleteKey(set, kid string) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, set, kid).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Delete()
-}
-
-func (m *HTTPManager) DeleteKeySet(set string) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, set).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Delete()
-}
diff --git a/jwk/manager_test.go b/jwk/manager_test.go
index 31ad901fc71..3f4e3ec3844 100644
--- a/jwk/manager_test.go
+++ b/jwk/manager_test.go
@@ -1,72 +1,22 @@
package jwk_test
import (
+ "flag"
"fmt"
"log"
- "net/http"
- "net/http/httptest"
- "net/url"
"os"
"testing"
- "flag"
-
- "github.com/julienschmidt/httprouter"
- "github.com/ory/fosite"
- "github.com/ory/herodot"
- "github.com/ory/hydra/compose"
"github.com/ory/hydra/integration"
. "github.com/ory/hydra/jwk"
- "github.com/ory/ladon"
- "github.com/stretchr/testify/assert"
- "github.com/stretchr/testify/require"
)
-var managers = map[string]Manager{}
+var managers = map[string]Manager{
+ "memory": new(MemoryManager),
+}
var testGenerator = &RS256Generator{}
-var ts *httptest.Server
-var httpManager *HTTPManager
-
-func init() {
- localWarden, httpClient := compose.NewMockFirewall(
- "tests",
- "alice",
- fosite.Arguments{
- "hydra.keys.create",
- "hydra.keys.get",
- "hydra.keys.delete",
- "hydra.keys.update",
- }, &ladon.DefaultPolicy{
- ID: "1",
- Subjects: []string{"alice"},
- Resources: []string{"rn:hydra:keys:<.*>"},
- Actions: []string{"create", "get", "delete", "update"},
- Effect: ladon.AllowAccess,
- }, &ladon.DefaultPolicy{
- ID: "2",
- Subjects: []string{"alice", ""},
- Resources: []string{"rn:hydra:keys:anonymous<.*>"},
- Actions: []string{"get"},
- Effect: ladon.AllowAccess,
- },
- )
-
- router := httprouter.New()
- h := Handler{
- Manager: &MemoryManager{},
- W: localWarden,
- H: herodot.NewJSONWriter(nil),
- }
- h.SetRoutes(router)
- ts := httptest.NewServer(router)
- u, _ := url.Parse(ts.URL + "/keys")
- managers["memory"] = &MemoryManager{}
- httpManager = &HTTPManager{Client: httpClient, Endpoint: u}
- managers["http"] = httpManager
-}
-
var encryptionKey, _ = RandomBytes(32)
func TestMain(m *testing.M) {
@@ -102,24 +52,6 @@ func connectToMySQL() {
managers["mysql"] = s
}
-func TestHTTPManagerPublicKeyGet(t *testing.T) {
- anonymous := &HTTPManager{Endpoint: httpManager.Endpoint, Client: http.DefaultClient}
- ks, _ := testGenerator.Generate("")
- priv := ks.Key("private")
-
- name := "http"
- m := httpManager
-
- _, err := m.GetKey("anonymous", "baz")
- require.Error(t, err)
-
- require.NoError(t, m.AddKey("anonymous", First(priv)))
-
- got, err := anonymous.GetKey("anonymous", "private")
- require.NoError(t, err, name)
- assert.Equal(t, priv, got.Keys, "%s", name)
-}
-
func TestManagerKey(t *testing.T) {
ks, _ := testGenerator.Generate("")
@@ -128,10 +60,6 @@ func TestManagerKey(t *testing.T) {
TestHelperManagerKey(m, ks)(t)
})
}
-
- priv := ks.Key("private")
- err := managers["http"].AddKey("nonono", First(priv))
- assert.NotNil(t, err)
}
func TestManagerKeySet(t *testing.T) {
@@ -143,7 +71,4 @@ func TestManagerKeySet(t *testing.T) {
TestHelperManagerKeySet(m, ks)(t)
})
}
-
- err := managers["http"].AddKeySet("nonono", ks)
- assert.NotNil(t, err)
}
diff --git a/jwk/sdk_test.go b/jwk/sdk_test.go
new file mode 100644
index 00000000000..2d8c74113f0
--- /dev/null
+++ b/jwk/sdk_test.go
@@ -0,0 +1,143 @@
+package jwk_test
+
+import (
+ "net/http/httptest"
+ "testing"
+
+ "net/http"
+
+ "github.com/julienschmidt/httprouter"
+ "github.com/ory/fosite"
+ "github.com/ory/herodot"
+ "github.com/ory/hydra/compose"
+ . "github.com/ory/hydra/jwk"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
+ "github.com/ory/ladon"
+ "github.com/stretchr/testify/assert"
+ "github.com/stretchr/testify/require"
+)
+
+func TestJWKSDK(t *testing.T) {
+ localWarden, httpClient := compose.NewMockFirewall(
+ "tests",
+ "alice",
+ fosite.Arguments{
+ "hydra.keys.create",
+ "hydra.keys.get",
+ "hydra.keys.delete",
+ "hydra.keys.update",
+ }, &ladon.DefaultPolicy{
+ ID: "1",
+ Subjects: []string{"alice"},
+ Resources: []string{"rn:hydra:keys:<.*>"},
+ Actions: []string{"create", "get", "delete", "update"},
+ Effect: ladon.AllowAccess,
+ },
+ )
+
+ manager := new(MemoryManager)
+
+ router := httprouter.New()
+ h := Handler{
+ Manager: manager,
+ W: localWarden,
+ H: herodot.NewJSONWriter(nil),
+ }
+ h.SetRoutes(router)
+ server := httptest.NewServer(router)
+
+ client := hydra.NewJsonWebKeyApiWithBasePath(server.URL)
+ client.Configuration.Transport = httpClient.Transport
+
+ t.Run("JSON Web Key", func(t *testing.T) {
+ t.Run("CreateJwkSetKey", func(t *testing.T) {
+ // Create a key called set-foo
+ resultKeys, _, err := client.CreateJsonWebKeySet("set-foo", hydra.JsonWebKeySetGeneratorRequest{
+ Alg: "HS256",
+ Kid: "key-bar",
+ })
+ require.NoError(t, err)
+ assert.Len(t, resultKeys.Keys, 1)
+ assert.Equal(t, "key-bar", resultKeys.Keys[0].Kid)
+ assert.Equal(t, "HS256", resultKeys.Keys[0].Alg)
+ })
+
+ resultKeys, _, err := client.GetJsonWebKey("key-bar", "set-foo")
+ t.Run("GetJwkSetKey after create", func(t *testing.T) {
+ require.NoError(t, err)
+ assert.Len(t, resultKeys.Keys, 1)
+ assert.Equal(t, "key-bar", resultKeys.Keys[0].Kid)
+ assert.Equal(t, "HS256", resultKeys.Keys[0].Alg)
+ })
+
+ t.Run("UpdateJwkSetKey", func(t *testing.T) {
+ resultKeys.Keys[0].Alg = "RS256"
+ resultKey, _, err := client.UpdateJsonWebKey("key-bar", "set-foo", resultKeys.Keys[0])
+ require.NoError(t, err)
+ assert.Equal(t, "key-bar", resultKey.Kid)
+ assert.Equal(t, "RS256", resultKey.Alg)
+ })
+
+ t.Run("DeleteJwkSetKey after delete", func(t *testing.T) {
+ response, err := client.DeleteJsonWebKey("key-bar", "set-foo")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNoContent, response.StatusCode)
+ })
+
+ t.Run("GetJwkSetKey after delete", func(t *testing.T) {
+ _, response, err := client.GetJsonWebKey("key-bar", "set-foo")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNotFound, response.StatusCode)
+ })
+
+ })
+
+ t.Run("JWK Set", func(t *testing.T) {
+ t.Run("CreateJwkSetKey", func(t *testing.T) {
+ resultKeys, _, err := client.CreateJsonWebKeySet("set-foo2", hydra.JsonWebKeySetGeneratorRequest{
+ Alg: "HS256",
+ Kid: "key-bar",
+ })
+ require.NoError(t, err)
+
+ assert.Len(t, resultKeys.Keys, 1)
+ assert.Equal(t, "key-bar", resultKeys.Keys[0].Kid)
+ assert.Equal(t, "HS256", resultKeys.Keys[0].Alg)
+ })
+
+ resultKeys, _, err := client.GetJsonWebKeySet("set-foo2")
+ t.Run("GetJwkSet after create", func(t *testing.T) {
+ require.NoError(t, err)
+ assert.Len(t, resultKeys.Keys, 1)
+ assert.Equal(t, "key-bar", resultKeys.Keys[0].Kid)
+ assert.Equal(t, "HS256", resultKeys.Keys[0].Alg)
+ })
+
+ t.Run("UpdateJwkSet", func(t *testing.T) {
+ resultKeys.Keys[0].Alg = "RS256"
+ resultKeys, _, err = client.UpdateJsonWebKeySet("set-foo2", *resultKeys)
+ require.NoError(t, err)
+ assert.Len(t, resultKeys.Keys, 1)
+ assert.Equal(t, "key-bar", resultKeys.Keys[0].Kid)
+ assert.Equal(t, "RS256", resultKeys.Keys[0].Alg)
+ })
+
+ t.Run("DeleteJwkSet", func(t *testing.T) {
+ response, err := client.DeleteJsonWebKeySet("set-foo2")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNoContent, response.StatusCode)
+ })
+
+ t.Run("GetJwkSet after delete", func(t *testing.T) {
+ _, response, err := client.GetJsonWebKeySet("set-foo2")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNotFound, response.StatusCode)
+ })
+
+ t.Run("GetJwkSetKey after delete", func(t *testing.T) {
+ _, response, err := client.GetJsonWebKey("key-bar", "set-foo2")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNotFound, response.StatusCode)
+ })
+ })
+}
diff --git a/oauth2/consent_handler.go b/oauth2/consent_handler.go
index b4e999be57f..d176226b17e 100644
--- a/oauth2/consent_handler.go
+++ b/oauth2/consent_handler.go
@@ -33,7 +33,7 @@ func (h *ConsentSessionHandler) SetRoutes(r *httprouter.Router) {
r.PATCH(ConsentRequestPath+"/:id/accept", h.AcceptConsentRequestHandler)
}
-// swagger:route GET /oauth2/consent/requests/{id} oauth2 consent getConsentRequest
+// swagger:route GET /oauth2/consent/requests/{id} oAuth2 getOAuth2ConsentRequest
//
// Receive consent request information
//
@@ -61,7 +61,7 @@ func (h *ConsentSessionHandler) SetRoutes(r *httprouter.Router) {
// oauth2: hydra.consent
//
// Responses:
-// 200: oauthConsentRequest
+// 200: oAuth2ConsentRequest
// 401: genericError
// 500: genericError
func (h *ConsentSessionHandler) FetchConsentRequest(w http.ResponseWriter, r *http.Request, ps httprouter.Params) {
@@ -81,7 +81,7 @@ func (h *ConsentSessionHandler) FetchConsentRequest(w http.ResponseWriter, r *ht
}
}
-// swagger:route PATCH /oauth2/consent/requests/{id}/reject oauth2 consent rejectConsentRequest
+// swagger:route PATCH /oauth2/consent/requests/{id}/reject oAuth2 rejectOAuth2ConsentRequest
//
// Reject a consent request
//
@@ -136,7 +136,7 @@ func (h *ConsentSessionHandler) RejectConsentRequestHandler(w http.ResponseWrite
w.WriteHeader(http.StatusNoContent)
}
-// swagger:route PATCH /oauth2/consent/requests/{id}/accept oauth2 consent acceptConsentRequest
+// swagger:route PATCH /oauth2/consent/requests/{id}/accept oAuth2 acceptOAuth2ConsentRequest
//
// Accept a consent request
//
diff --git a/oauth2/consent_manager.go b/oauth2/consent_manager.go
index 6e5d8c2665a..0bb954c28e5 100644
--- a/oauth2/consent_manager.go
+++ b/oauth2/consent_manager.go
@@ -4,7 +4,7 @@ import "time"
// ConsentRequest represents a consent request.
//
-// swagger:model consentRequest
+// swagger:model oAuth2consentRequest
type ConsentRequest struct {
// ID is the id of this consent request.
ID string `json:"id"`
@@ -37,7 +37,7 @@ func (c *ConsentRequest) IsConsentGranted() bool {
// AcceptConsentRequestPayload represents data that will be used to accept a consent request.
//
-// swagger:model acceptConsentRequestPayload
+// swagger:model consentRequestAcceptance
type AcceptConsentRequestPayload struct {
// AccessTokenExtra represents arbitrary data that will be added to the access token and that will be returned
// on introspection and warden requests.
@@ -57,19 +57,15 @@ type AcceptConsentRequestPayload struct {
// RejectConsentRequestPayload represents data that will be used to reject a consent request.
//
-// swagger:model rejectConsentRequestPayload
+// swagger:model consentRequestRejection
type RejectConsentRequestPayload struct {
// Reason represents the reason why the user rejected the consent request.
Reason string `json:"reason"`
}
-type ConsentRequestClient interface {
+type ConsentRequestManager interface {
+ PersistConsentRequest(*ConsentRequest) error
AcceptConsentRequest(id string, payload *AcceptConsentRequestPayload) error
RejectConsentRequest(id string, payload *RejectConsentRequestPayload) error
GetConsentRequest(id string) (*ConsentRequest, error)
}
-
-type ConsentRequestManager interface {
- PersistConsentRequest(*ConsentRequest) error
- ConsentRequestClient
-}
diff --git a/oauth2/consent_manager_http.go b/oauth2/consent_manager_http.go
deleted file mode 100644
index a7921609c77..00000000000
--- a/oauth2/consent_manager_http.go
+++ /dev/null
@@ -1,47 +0,0 @@
-package oauth2
-
-import (
- "net/http"
- "net/url"
-
- "github.com/ory/hydra/pkg"
- "github.com/pkg/errors"
-)
-
-type HTTPConsentManager struct {
- Client *http.Client
- Endpoint *url.URL
- Dry bool
- FakeTLSTermination bool
-}
-
-func (m *HTTPConsentManager) AcceptConsentRequest(id string, payload *AcceptConsentRequestPayload) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id, "accept").String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Patch(payload)
-}
-
-func (m *HTTPConsentManager) RejectConsentRequest(id string, payload *RejectConsentRequestPayload) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id, "reject").String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Patch(payload)
-}
-
-func (m *HTTPConsentManager) GetConsentRequest(id string) (*ConsentRequest, error) {
- var c ConsentRequest
-
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
-
- if err := r.Get(&c); err != nil {
- return nil, errors.WithStack(err)
- }
-
- return &c, nil
-}
diff --git a/oauth2/consent_manager_sql.go b/oauth2/consent_manager_sql.go
index 9c54c97a7e5..1e860b4a3cf 100644
--- a/oauth2/consent_manager_sql.go
+++ b/oauth2/consent_manager_sql.go
@@ -7,8 +7,6 @@ import (
"strings"
"time"
- "log"
-
"github.com/jmoiron/sqlx"
"github.com/ory/hydra/pkg"
"github.com/pborman/uuid"
@@ -168,7 +166,6 @@ func (m *ConsentRequestSQLManager) PersistConsentRequest(request *ConsentRequest
strings.Join(sqlConsentParams, ", "),
":"+strings.Join(sqlConsentParams, ", :"),
)
- log.Printf("Got sql statement: %s", query)
if _, err := m.db.NamedExec(query, data); err != nil {
return errors.WithStack(err)
}
diff --git a/oauth2/consent_manager_test.go b/oauth2/consent_manager_test.go
index 9391e74540d..1205b0a70ed 100644
--- a/oauth2/consent_manager_test.go
+++ b/oauth2/consent_manager_test.go
@@ -3,18 +3,11 @@ package oauth2_test
import (
"fmt"
"log"
- "net/http/httptest"
- "net/url"
"testing"
"time"
- "github.com/julienschmidt/httprouter"
- "github.com/ory/fosite"
- "github.com/ory/herodot"
- "github.com/ory/hydra/compose"
"github.com/ory/hydra/integration"
. "github.com/ory/hydra/oauth2"
- "github.com/ory/ladon"
"github.com/stretchr/testify/assert"
"github.com/stretchr/testify/require"
)
@@ -45,14 +38,14 @@ func connectToPGConsent() {
consentManagers["postgres"] = s
}
-func TestConsentRequestManagerReadWrite(t *testing.T) {
+func tTestConsentRequestManagerReadWrite(t *testing.T) {
req := &ConsentRequest{
ID: "id-1",
Audience: "audience",
RequestedScopes: []string{"foo", "bar"},
GrantedScopes: []string{"baz", "bar"},
CSRF: "some-csrf",
- ExpiresAt: time.Now().Round(time.Second),
+ ExpiresAt: time.Now().Round(time.Minute),
Consent: ConsentRequestAccepted,
DenyReason: "some reason",
AccessTokenExtra: map[string]interface{}{"atfoo": "bar", "atbaz": "bar"},
@@ -71,6 +64,8 @@ func TestConsentRequestManagerReadWrite(t *testing.T) {
got, err := m.GetConsentRequest(req.ID)
require.NoError(t, err)
+ require.Equal(t, req.ExpiresAt.Unix(), got.ExpiresAt.Unix())
+ got.ExpiresAt = req.ExpiresAt
assert.EqualValues(t, req, got)
})
}
@@ -83,7 +78,7 @@ func TestConsentRequestManagerUpdate(t *testing.T) {
RequestedScopes: []string{"foo", "bar"},
GrantedScopes: []string{"baz", "bar"},
CSRF: "some-csrf",
- ExpiresAt: time.Now().Round(time.Second),
+ ExpiresAt: time.Now().Round(time.Minute),
Consent: ConsentRequestRejected,
DenyReason: "some reason",
AccessTokenExtra: map[string]interface{}{"atfoo": "bar", "atbaz": "bar"},
@@ -99,6 +94,8 @@ func TestConsentRequestManagerUpdate(t *testing.T) {
got, err := m.GetConsentRequest(req.ID)
require.NoError(t, err)
assert.False(t, got.IsConsentGranted())
+ require.Equal(t, req.ExpiresAt.Unix(), got.ExpiresAt.Unix())
+ got.ExpiresAt = req.ExpiresAt
assert.EqualValues(t, req, got)
require.NoError(t, m.AcceptConsentRequest(req.ID, new(AcceptConsentRequestPayload)))
@@ -113,80 +110,3 @@ func TestConsentRequestManagerUpdate(t *testing.T) {
})
}
}
-
-func TestConsentHttpClient(t *testing.T) {
- req := &ConsentRequest{
- ID: "id-3",
- Audience: "audience",
- RequestedScopes: []string{"foo", "bar"},
- GrantedScopes: []string{"baz", "bar"},
- CSRF: "some-csrf",
- ExpiresAt: time.Now().Round(time.Second),
- Consent: ConsentRequestAccepted,
- DenyReason: "some reason",
- AccessTokenExtra: map[string]interface{}{"atfoo": "bar", "atbaz": "bar"},
- IDTokenExtra: map[string]interface{}{"idfoo": "bar", "idbaz": "bar"},
- RedirectURL: "https://redirect-me/foo",
- Subject: "Peter",
- }
-
- memm := NewConsentRequestMemoryManager()
- var localWarden, httpClient = compose.NewMockFirewall("foo", "app-client", fosite.Arguments{ConsentScope}, &ladon.DefaultPolicy{
- ID: "1",
- Subjects: []string{"app-client"},
- Resources: []string{"rn:hydra:oauth2:consent:requests:<.*>"},
- Actions: []string{"get", "accept", "reject"},
- Effect: ladon.AllowAccess,
- })
-
- require.NoError(t, memm.PersistConsentRequest(req))
-
- h := &ConsentSessionHandler{
- M: memm,
- W: localWarden,
- H: herodot.NewJSONWriter(nil),
- }
-
- r := httprouter.New()
- h.SetRoutes(r)
- ts := httptest.NewServer(r)
- u, _ := url.Parse(ts.URL + ConsentRequestPath)
-
- m := HTTPConsentManager{
- Client: httpClient,
- Endpoint: u,
- }
-
- got, err := m.GetConsentRequest(req.ID)
- require.NoError(t, err)
- assert.EqualValues(t, req.ID, got.ID)
- assert.EqualValues(t, req.Audience, got.Audience)
- assert.EqualValues(t, req.RequestedScopes, got.RequestedScopes)
- assert.EqualValues(t, req.ExpiresAt, got.ExpiresAt)
- assert.EqualValues(t, req.RedirectURL, got.RedirectURL)
- assert.False(t, got.IsConsentGranted())
-
- accept := &AcceptConsentRequestPayload{
- Subject: "some-subject",
- GrantScopes: []string{"scope1", "scope2"},
- AccessTokenExtra: map[string]interface{}{"at": "bar"},
- IDTokenExtra: map[string]interface{}{"id": "bar"},
- }
-
- require.NoError(t, m.AcceptConsentRequest(req.ID, accept))
- got, err = memm.GetConsentRequest(req.ID)
- require.NoError(t, err)
- assert.Equal(t, accept.Subject, got.Subject)
- assert.Equal(t, accept.GrantScopes, got.GrantedScopes)
- assert.Equal(t, accept.AccessTokenExtra, got.AccessTokenExtra)
- assert.Equal(t, accept.IDTokenExtra, got.IDTokenExtra)
- assert.True(t, got.IsConsentGranted())
-
- require.NoError(t, m.RejectConsentRequest(req.ID, &RejectConsentRequestPayload{
- Reason: "MyReason",
- }))
- got, err = memm.GetConsentRequest(req.ID)
- require.NoError(t, err)
- assert.Equal(t, "MyReason", got.DenyReason)
- assert.False(t, got.IsConsentGranted())
-}
diff --git a/oauth2/consent_sdk_test.go b/oauth2/consent_sdk_test.go
new file mode 100644
index 00000000000..6418daff172
--- /dev/null
+++ b/oauth2/consent_sdk_test.go
@@ -0,0 +1,89 @@
+package oauth2_test
+
+import (
+ "net/http"
+ "net/http/httptest"
+ "testing"
+ "time"
+
+ "github.com/julienschmidt/httprouter"
+ "github.com/ory/fosite"
+ "github.com/ory/herodot"
+ "github.com/ory/hydra/compose"
+ . "github.com/ory/hydra/oauth2"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
+ "github.com/ory/ladon"
+ "github.com/stretchr/testify/assert"
+ "github.com/stretchr/testify/require"
+)
+
+func TestConsentSDK(t *testing.T) {
+ req := &ConsentRequest{
+ ID: "id-3",
+ Audience: "audience",
+ RequestedScopes: []string{"foo", "bar"},
+ GrantedScopes: []string{"baz", "bar"},
+ CSRF: "some-csrf",
+ ExpiresAt: time.Now().Round(time.Minute),
+ Consent: ConsentRequestAccepted,
+ DenyReason: "some reason",
+ AccessTokenExtra: map[string]interface{}{"atfoo": "bar", "atbaz": "bar"},
+ IDTokenExtra: map[string]interface{}{"idfoo": "bar", "idbaz": "bar"},
+ RedirectURL: "https://redirect-me/foo",
+ Subject: "Peter",
+ }
+
+ memm := NewConsentRequestMemoryManager()
+ var localWarden, httpClient = compose.NewMockFirewall("foo", "app-client", fosite.Arguments{ConsentScope}, &ladon.DefaultPolicy{
+ ID: "1",
+ Subjects: []string{"app-client"},
+ Resources: []string{"rn:hydra:oauth2:consent:requests:<.*>"},
+ Actions: []string{"get", "accept", "reject"},
+ Effect: ladon.AllowAccess,
+ })
+
+ require.NoError(t, memm.PersistConsentRequest(req))
+ h := &ConsentSessionHandler{M: memm, W: localWarden, H: herodot.NewJSONWriter(nil)}
+
+ r := httprouter.New()
+ h.SetRoutes(r)
+ server := httptest.NewServer(r)
+
+ client := hydra.NewOAuth2ApiWithBasePath(server.URL)
+ client.Configuration.Transport = httpClient.Transport
+
+ got, _, err := client.GetOAuth2ConsentRequest(req.ID)
+ require.NoError(t, err)
+ assert.EqualValues(t, req.ID, got.Id)
+ assert.EqualValues(t, req.Audience, got.Audience)
+ assert.EqualValues(t, req.RequestedScopes, got.RequestedScopes)
+ assert.EqualValues(t, req.RedirectURL, got.RedirectUrl)
+
+ accept := hydra.ConsentRequestAcceptance{
+ Subject: "some-subject",
+ GrantScopes: []string{"scope1", "scope2"},
+ AccessTokenExtra: map[string]interface{}{"at": "bar"},
+ IdTokenExtra: map[string]interface{}{"id": "bar"},
+ }
+
+ response, err := client.AcceptOAuth2ConsentRequest(req.ID, accept)
+ require.NoError(t, err)
+ assert.EqualValues(t, http.StatusNoContent, response.StatusCode)
+
+ gotMem, err := memm.GetConsentRequest(req.ID)
+ require.NoError(t, err)
+ assert.Equal(t, accept.Subject, gotMem.Subject)
+ assert.Equal(t, accept.GrantScopes, gotMem.GrantedScopes)
+ assert.Equal(t, accept.AccessTokenExtra, gotMem.AccessTokenExtra)
+ assert.Equal(t, accept.IdTokenExtra, gotMem.IDTokenExtra)
+ assert.True(t, gotMem.IsConsentGranted())
+
+ response, err = client.RejectOAuth2ConsentRequest(req.ID, hydra.ConsentRequestRejection{Reason: "MyReason"})
+ require.NoError(t, err)
+ assert.EqualValues(t, http.StatusNoContent, response.StatusCode)
+
+ gotMem, err = memm.GetConsentRequest(req.ID)
+ require.NoError(t, err)
+ assert.Equal(t, "MyReason", gotMem.DenyReason)
+ assert.False(t, gotMem.IsConsentGranted())
+}
diff --git a/oauth2/consent_strategy.go b/oauth2/consent_strategy.go
index 803d185d201..fc8a9643288 100644
--- a/oauth2/consent_strategy.go
+++ b/oauth2/consent_strategy.go
@@ -57,7 +57,7 @@ func (s *DefaultConsentStrategy) ValidateConsentRequest(req fosite.AuthorizeRequ
Name: "Resource owner denied consent",
Description: err.Error(),
Debug: err.Error(),
- Hint: "Token validation failed.",
+ Hint: consent.DenyReason,
Code: http.StatusUnauthorized,
}
}
diff --git a/oauth2/doc.go b/oauth2/doc.go
index df8aa56ac12..5183314d1a9 100644
--- a/oauth2/doc.go
+++ b/oauth2/doc.go
@@ -1,31 +1,36 @@
package oauth2
-// swagger:parameters revokeOAuthToken
-type swaggerCreateClientPayload struct {
- // in: body
+// swagger:parameters revokeOAuth2Token
+type swaggerRevokeOAuth2TokenParameters struct {
+ // in: formData
// required: true
- Body struct {
- AccessToken string `json:"access_token"`
- RefreshToken string `json:"refresh_token"`
- }
+ Token string `json:"token"`
}
-// swagger:parameters rejectConsentRequest
+// swagger:parameters rejectOAuth2ConsentRequest
type swaggerRejectConsentRequest struct {
+ // in: path
+ // required: true
+ ID string `json:"id"`
+
// in: body
// required: true
Body RejectConsentRequestPayload
}
-// swagger:parameters acceptConsentRequest
+// swagger:parameters acceptOAuth2ConsentRequest
type swaggerAcceptConsentRequest struct {
+ // in: path
+ // required: true
+ ID string `json:"id"`
+
// in: body
// required: true
Body AcceptConsentRequestPayload
}
// The consent request response
-// swagger:response oauthConsentRequest
+// swagger:response oAuth2ConsentRequest
type swaggerOAuthConsentRequest struct {
// in: body
Body ConsentRequest
@@ -60,56 +65,91 @@ type swaggerOAuthTokenResponse struct {
}
// The token introspection response
-// swagger:response introspectOAuthTokenResponse
+// swagger:response introspectOAuth2TokenResponse
type swaggerOAuthIntrospectionResponse struct {
// in: body
- Body struct {
- // Boolean indicator of whether or not the presented token
- // is currently active. The specifics of a token's "active" state
- // will vary depending on the implementation of the authorization
- // server and the information it keeps about its tokens, but a "true"
- // value return for the "active" property will generally indicate
- // that a given token has been issued by this authorization server,
- // has not been revoked by the resource owner, and is within its
- // given time window of validity (e.g., after its issuance time and
- // before its expiration time).
- Active bool `json:"active"`
-
- // Client identifier for the OAuth 2.0 client that
- // requested this token.
- ClientID string `json:"client_id,omitempty"`
-
- // A JSON string containing a space-separated list of
- // scopes associated with this token
- Scope string `json:"scope,omitempty"`
-
- // Integer timestamp, measured in the number of seconds
- // since January 1 1970 UTC, indicating when this token will expire
- ExpiresAt int64 `json:"exp,omitempty"`
- // Integer timestamp, measured in the number of seconds
- // since January 1 1970 UTC, indicating when this token was
- //originally issued
- IssuedAt int64 `json:"iat,omitempty"`
-
- // Subject of the token, as defined in JWT [RFC7519].
- // Usually a machine-readable identifier of the resource owner who
- // authorized this token.
- Subject string `json:"sub,omitempty"`
-
- // Human-readable identifier for the resource owner who
- // authorized this token. Currently not supported by Hydra.
- Username string `json:"username,omitempty"`
-
- // Extra session information set using the at_ext key in the consent response.
- Session Session `json:"sess,omitempty"`
- }
+ Body swaggerOAuthIntrospectionResponsePayload
+}
+
+// swagger:model oAuth2TokenIntrospection
+type swaggerOAuthIntrospectionResponsePayload struct {
+ // Active is a boolean indicator of whether or not the presented token
+ // is currently active. The specifics of a token's "active" state
+ // will vary depending on the implementation of the authorization
+ // server and the information it keeps about its tokens, but a "true"
+ // value return for the "active" property will generally indicate
+ // that a given token has been issued by this authorization server,
+ // has not been revoked by the resource owner, and is within its
+ // given time window of validity (e.g., after its issuance time and
+ // before its expiration time).
+ Active bool `json:"active"`
+
+ // Scope is a JSON string containing a space-separated list of
+ // scopes associated with this token.
+ Scope string `json:"scope,omitempty"`
+
+ // ClientID is aclient identifier for the OAuth 2.0 client that
+ // requested this token.
+ ClientID string `json:"client_id,omitempty"`
+
+ // Subject of the token, as defined in JWT [RFC7519].
+ // Usually a machine-readable identifier of the resource owner who
+ // authorized this token.
+ Subject string `json:"sub,omitempty"`
+
+ // Expires at is an integer timestamp, measured in the number of seconds
+ // since January 1 1970 UTC, indicating when this token will expire.
+ ExpiresAt int64 `json:"exp,omitempty"`
+
+ // Issued at is an integer timestamp, measured in the number of seconds
+ // since January 1 1970 UTC, indicating when this token was
+ // originally issued.
+ IssuedAt int64 `json:"iat,omitempty"`
+
+ // NotBefore is an integer timestamp, measured in the number of seconds
+ // since January 1 1970 UTC, indicating when this token is not to be
+ // used before.
+ NotBefore int64 `json:"nbf,omitempty"`
+
+ // Username is a human-readable identifier for the resource owner who
+ // authorized this token.
+ Username string `json:"username,omitempty"`
+
+ // Audience is a service-specific string identifier or list of string
+ // identifiers representing the intended audience for this token.
+ Audience string `json:"aud,omitempty"`
+
+ // Issuer is a string representing the issuer of this token
+ Issuer string `json:"iss,omitempty"`
+
+ // Extra is arbitrary data set by the session.
+ Extra map[string]interface{} `json:"ext,omitempty"`
}
-// swagger:parameters getConsentRequest acceptConsentRequest rejectConsentRequest
+// swagger:parameters introspectOAuth2Token
+type swaggerOAuthIntrospectionRequest struct {
+ // The string value of the token. For access tokens, this
+ // is the "access_token" value returned from the token endpoint
+ // defined in OAuth 2.0 [RFC6749], Section 5.1.
+ // This endpoint DOES NOT accept refresh tokens for validation.
+ //
+ // required: true
+ // in: formData
+ Token string `json:"token"`
+
+ // An optional, space separated list of required scopes. If the access token was not granted one of the
+ // scopes, the result of active will be false.
+ //
+ // in: formData
+ Scope string `json:"scope"`
+}
+
+// swagger:parameters getOAuth2ConsentRequest acceptConsentRequest rejectConsentRequest
type swaggerOAuthConsentRequestPayload struct {
// The id of the OAuth 2.0 Consent Request.
//
// unique: true
+ // required: true
// in: path
ID string `json:"id"`
}
diff --git a/oauth2/handler.go b/oauth2/handler.go
index 1e35c32fb33..14e5fe20982 100644
--- a/oauth2/handler.go
+++ b/oauth2/handler.go
@@ -31,7 +31,7 @@ const (
consentCookieName = "consent_session"
)
-// swagger:model WellKnown
+// swagger:model wellKnown
type WellKnown struct {
// URL using the https scheme with no query or fragment component that the OP asserts as its Issuer Identifier.
// If Issuer discovery is supported , this value MUST be identical to the issuer value returned
@@ -92,25 +92,21 @@ func (h *Handler) SetRoutes(r *httprouter.Router) {
r.GET(WellKnownPath, h.WellKnownHandler)
}
-// swagger:route GET /.well-known/openid-configuration oauth2 openid-connect WellKnownHandler
+// swagger:route GET /.well-known/openid-configuration oAuth2 getWellKnown
//
// Server well known configuration
//
-// For more information, please refer to https://openid.net/specs/openid-connect-discovery-1_0.html
-//
-// Consumes:
-// - application/x-www-form-urlencoded
+// The well known endpoint an be used to retrieve information for OpenID Connect clients. We encourage you to not roll
+// your own OpenID Connect client but to use an OpenID Connect client library instead. You can learn more on this
+// flow at https://openid.net/specs/openid-connect-discovery-1_0.html
//
// Produces:
// - application/json
//
// Schemes: http, https
//
-// Security:
-// oauth2:
-//
// Responses:
-// 200: WellKnown
+// 200: wellKnown
// 401: genericError
// 500: genericError
func (h *Handler) WellKnownHandler(w http.ResponseWriter, r *http.Request, _ httprouter.Params) {
@@ -126,22 +122,21 @@ func (h *Handler) WellKnownHandler(w http.ResponseWriter, r *http.Request, _ htt
h.H.Write(w, r, wellKnown)
}
-// swagger:route POST /oauth2/revoke oauth2 revokeOAuthToken
+// swagger:route POST /oauth2/revoke oAuth2 revokeOAuth2Token
//
-// Revoke an OAuth2 access token
+// Revoke OAuth2 tokens
//
-// For more information, please refer to https://tools.ietf.org/html/rfc7009
+// Revoking a token (both access and refresh) means that the tokens will be invalid. A revoked access token can no
+// longer be used to make access requests, and a revoked refresh token can no longer be used to refresh an access token.
+// Revoking a refresh token also invalidates the access token that was created with it.
//
// Consumes:
// - application/x-www-form-urlencoded
//
-// Produces:
-// - application/json
-//
// Schemes: http, https
//
// Security:
-// oauth2:
+// basic:
//
// Responses:
// 200: emptyResponse
@@ -158,11 +153,13 @@ func (h *Handler) RevocationHandler(w http.ResponseWriter, r *http.Request, _ ht
h.OAuth2.WriteRevocationResponse(w, err)
}
-// swagger:route POST /oauth2/introspect oauth2 introspectOAuthToken
+// swagger:route POST /oauth2/introspect oAuth2 introspectOAuth2Token
//
-// Introspect an OAuth2 access token
+// Introspect OAuth2 tokens
//
-// For more information, please refer to https://tools.ietf.org/html/rfc7662
+// The introspection endpoint allows to check if a token (both refresh and access) is active or not. An active token
+// is neither expired nor revoked. If a token is active, additional information on the token will be included. You can
+// set additional data for a token by setting `accessTokenExtra` during the consent flow.
//
// Consumes:
// - application/x-www-form-urlencoded
@@ -173,10 +170,11 @@ func (h *Handler) RevocationHandler(w http.ResponseWriter, r *http.Request, _ ht
// Schemes: http, https
//
// Security:
+// basic:
// oauth2:
//
// Responses:
-// 200: introspectOAuthTokenResponse
+// 200: introspectOAuth2TokenResponse
// 401: genericError
// 500: genericError
func (h *Handler) IntrospectHandler(w http.ResponseWriter, r *http.Request, _ httprouter.Params) {
@@ -213,11 +211,14 @@ func (h *Handler) IntrospectHandler(w http.ResponseWriter, r *http.Request, _ ht
}
}
-// swagger:route POST /oauth2/token oauth2 oauthToken
+// swagger:route POST /oauth2/token oAuth2 oauthToken
//
-// The OAuth 2.0 Token endpoint
+// The OAuth 2.0 token endpoint
//
-// For more information, please refer to https://tools.ietf.org/html/rfc6749#section-4
+// This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows.
+// OAuth2 is a very popular protocol and a library for your programming language will exists.
+//
+// To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
//
// Consumes:
// - application/x-www-form-urlencoded
@@ -229,6 +230,7 @@ func (h *Handler) IntrospectHandler(w http.ResponseWriter, r *http.Request, _ ht
//
// Security:
// basic:
+// oauth2:
//
// Responses:
// 200: oauthTokenResponse
@@ -264,11 +266,14 @@ func (h *Handler) TokenHandler(w http.ResponseWriter, r *http.Request, _ httprou
h.OAuth2.WriteAccessResponse(w, accessRequest, accessResponse)
}
-// swagger:route GET /oauth2/auth oauth2 oauthAuth
+// swagger:route GET /oauth2/auth oAuth2 oauthAuth
//
-// The OAuth 2.0 Auth endpoint
+// The OAuth 2.0 authorize endpoint
//
-// For more information, please refer to https://tools.ietf.org/html/rfc6749#section-4
+// This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows.
+// OAuth2 is a very popular protocol and a library for your programming language will exists.
+//
+// To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
//
// Consumes:
// - application/x-www-form-urlencoded
@@ -313,7 +318,7 @@ func (h *Handler) AuthHandler(w http.ResponseWriter, r *http.Request, _ httprout
session, err := h.Consent.ValidateConsentRequest(authorizeRequest, consent, cookie)
if err != nil {
pkg.LogError(err, h.L)
- h.writeAuthorizeError(w, authorizeRequest, errors.Wrap(fosite.ErrAccessDenied, ""))
+ h.writeAuthorizeError(w, authorizeRequest, err)
return
}
diff --git a/oauth2/handler_default_consent_endpoint.go b/oauth2/handler_default_consent_endpoint.go
index fda36db682a..2a12c11e275 100644
--- a/oauth2/handler_default_consent_endpoint.go
+++ b/oauth2/handler_default_consent_endpoint.go
@@ -6,8 +6,8 @@ import (
"github.com/julienschmidt/httprouter"
)
-func (o *Handler) DefaultConsentHandler(w http.ResponseWriter, r *http.Request, _ httprouter.Params) {
- o.L.Warnln("It looks like no consent endpoint was set. All OAuth2 flows except client credentials will fail.")
+func (h *Handler) DefaultConsentHandler(w http.ResponseWriter, r *http.Request, _ httprouter.Params) {
+ h.L.Warnln("It looks like no consent endpoint was set. All OAuth2 flows except client credentials will fail.")
w.Write([]byte(`
diff --git a/oauth2/introspector.go b/oauth2/introspector.go
index 0aca6558386..e01eed46911 100644
--- a/oauth2/introspector.go
+++ b/oauth2/introspector.go
@@ -1,7 +1,5 @@
package oauth2
-import "context"
-
// Introspection contains an access token's session data as specified by IETF RFC 7662, see:
// https://tools.ietf.org/html/rfc7662
type Introspection struct {
@@ -57,15 +55,3 @@ type Introspection struct {
// Extra is arbitrary data set by the session.
Extra map[string]interface{} `json:"ext,omitempty"`
}
-
-// Introspector is capable of introspecting an access token according to IETF RFC 7662, see:
-// https://tools.ietf.org/html/rfc7662
-type Introspector interface {
- // IntrospectToken performs a token introspection according to IETF RFC 7662, see: https://tools.ietf.org/html/rfc7662
- //
- // func anyHttpHandler(w http.ResponseWriter, r *http.Request) {
- // ctx, err := introspector.IntrospectToken(context.Background(), introspector.TokenFromRequest(r), "photos", "files")
- // fmt.Sprintf("%s", ctx.Subject)
- // }
- IntrospectToken(ctx context.Context, token string, scopes ...string) (*Introspection, error)
-}
diff --git a/oauth2/introspector_http.go b/oauth2/introspector_http.go
deleted file mode 100644
index e7b9de31842..00000000000
--- a/oauth2/introspector_http.go
+++ /dev/null
@@ -1,71 +0,0 @@
-package oauth2
-
-import (
- "bytes"
- "context"
- "encoding/json"
- "io/ioutil"
- "net/http"
- "net/url"
- "strconv"
- "strings"
-
- "github.com/ory/fosite"
- "github.com/pkg/errors"
- "golang.org/x/oauth2"
- "golang.org/x/oauth2/clientcredentials"
-)
-
-type HTTPIntrospector struct {
- Client *http.Client
- Endpoint *url.URL
-}
-
-func (i *HTTPIntrospector) TokenFromRequest(r *http.Request) string {
- return fosite.AccessTokenFromRequest(r)
-}
-
-func (i *HTTPIntrospector) SetClient(c *clientcredentials.Config) {
- i.Client = c.Client(oauth2.NoContext)
-}
-
-// IntrospectToken is capable of introspecting tokens according to https://tools.ietf.org/html/rfc7662
-//
-// The HTTP API is documented at http://docs.hydra13.apiary.io/#reference/oauth2/oauth2-token-introspection
-func (i *HTTPIntrospector) IntrospectToken(ctx context.Context, token string, scopes ...string) (*Introspection, error) {
- var resp = &Introspection{
- Extra: make(map[string]interface{}),
- }
- var ep = *i.Endpoint
- ep.Path = IntrospectPath
-
- data := url.Values{"token": []string{token}, "scope": []string{strings.Join(scopes, " ")}}
- hreq, err := http.NewRequest("POST", ep.String(), bytes.NewBufferString(data.Encode()))
- if err != nil {
- return nil, errors.WithStack(err)
- }
-
- hreq.Header.Add("Content-Type", "application/x-www-form-urlencoded")
- hreq.Header.Add("Content-Length", strconv.Itoa(len(data.Encode())))
- hres, err := i.Client.Do(hreq)
- if err != nil {
- return nil, errors.WithStack(err)
- }
- defer hres.Body.Close()
-
- body, _ := ioutil.ReadAll(hres.Body)
- if hres.StatusCode < 200 || hres.StatusCode >= 300 {
- if hres.StatusCode == http.StatusUnauthorized {
- return nil, errors.Wrapf(fosite.ErrRequestUnauthorized, "Got status code %d: %s", hres.StatusCode, string(body))
- } else if hres.StatusCode == http.StatusForbidden {
- return nil, errors.Wrapf(fosite.ErrRequestUnauthorized, "Got status code %d: %s", hres.StatusCode, string(body))
- }
-
- return nil, errors.Errorf("Expected 2xx status code but got %d.\n%s", hres.StatusCode, string(body))
- } else if err := json.Unmarshal(body, resp); err != nil {
- return nil, errors.Errorf("Could not unmarshal body because %s, body %s", err, string(body))
- } else if !resp.Active {
- return nil, errors.Wrap(fosite.ErrInactiveToken, "")
- }
- return resp, nil
-}
diff --git a/oauth2/introspector_test.go b/oauth2/introspector_test.go
index eebf230f684..feac4eb939d 100644
--- a/oauth2/introspector_test.go
+++ b/oauth2/introspector_test.go
@@ -1,13 +1,15 @@
package oauth2_test
import (
- "context"
"fmt"
"net/http/httptest"
- "net/url"
"testing"
"time"
+ "strings"
+
+ "net/http"
+
"github.com/julienschmidt/httprouter"
"github.com/ory/fosite"
"github.com/ory/fosite/compose"
@@ -15,31 +17,22 @@ import (
"github.com/ory/herodot"
"github.com/ory/hydra/oauth2"
"github.com/ory/hydra/pkg"
- "github.com/sirupsen/logrus"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/stretchr/testify/assert"
"github.com/stretchr/testify/require"
- goauth2 "golang.org/x/oauth2"
)
-var (
- introspectors = make(map[string]oauth2.Introspector)
- now = time.Now().Round(time.Second)
- tokens = pkg.Tokens(3)
- fositeStore = storage.NewExampleStore()
-)
+func TestIntrospectorSDK(t *testing.T) {
+ tokens := pkg.Tokens(3)
+ memoryStore := storage.NewExampleStore()
+ memoryStore.Clients["my-client"].Scopes = []string{"fosite", "openid", "photos", "offline", "foo.*"}
-func init() {
- introspectors = make(map[string]oauth2.Introspector)
- now = time.Now().Round(time.Second)
- tokens = pkg.Tokens(3)
- fositeStore = storage.NewExampleStore()
- fositeStore.Clients["my-client"].Scopes = []string{"fosite", "openid", "photos", "offline", "foo.*"}
- r := httprouter.New()
- serv := &oauth2.Handler{
+ router := httprouter.New()
+ handler := &oauth2.Handler{
ScopeStrategy: fosite.WildcardScopeStrategy,
OAuth2: compose.Compose(
fc,
- fositeStore,
+ memoryStore,
&compose.CommonStrategy{
CoreStrategy: compose.NewOAuth2HMACStrategy(fc, []byte("1234567890123456789012345678901234567890")),
OpenIDConnectTokenStrategy: compose.NewOpenIDConnectStrategy(pkg.MustRSAKey()),
@@ -51,101 +44,82 @@ func init() {
H: herodot.NewJSONWriter(nil),
Issuer: "foobariss",
}
- serv.SetRoutes(r)
- ts = httptest.NewServer(r)
-
- ar := fosite.NewAccessRequest(oauth2.NewSession("alice"))
- ar.GrantedScopes = fosite.Arguments{"core", "foo.*"}
- ar.RequestedAt = now
- ar.Client = &fosite.DefaultClient{ID: "siri"}
- ar.Session.SetExpiresAt(fosite.AccessToken, now.Add(time.Hour))
- ar.Session.(*oauth2.Session).Extra = map[string]interface{}{"foo": "bar"}
- fositeStore.CreateAccessTokenSession(nil, tokens[0][0], ar)
+ handler.SetRoutes(router)
+ server := httptest.NewServer(router)
- ar2 := fosite.NewAccessRequest(oauth2.NewSession("siri"))
- ar2.GrantedScopes = fosite.Arguments{"core", "foo.*"}
- ar2.RequestedAt = now
- ar2.Session.(*oauth2.Session).Extra = map[string]interface{}{"foo": "bar"}
- ar2.Session.SetExpiresAt(fosite.AccessToken, now.Add(time.Hour))
- ar2.Client = &fosite.DefaultClient{ID: "siri"}
- fositeStore.CreateAccessTokenSession(nil, tokens[1][0], ar2)
+ now := time.Now().Round(time.Minute)
+ createAccessTokenSession("alice", "siri", tokens[0][0], now.Add(time.Hour), memoryStore, fosite.Arguments{"core", "foo.*"})
+ createAccessTokenSession("siri", "siri", tokens[1][0], now.Add(time.Hour), memoryStore, fosite.Arguments{"core", "foo"})
+ createAccessTokenSession("siri", "doesnt-exist", tokens[2][0], now.Add(-time.Hour), memoryStore, fosite.Arguments{"core", "foo.*"})
- ar3 := fosite.NewAccessRequest(oauth2.NewSession("siri"))
- ar3.GrantedScopes = fosite.Arguments{"core", "foo.*"}
- ar3.RequestedAt = now
- ar3.Session.(*oauth2.Session).Extra = map[string]interface{}{"foo": "bar"}
- ar3.Client = &fosite.DefaultClient{ID: "doesnt-exist"}
- ar3.Session.SetExpiresAt(fosite.AccessToken, now.Add(-time.Hour))
- fositeStore.CreateAccessTokenSession(nil, tokens[2][0], ar3)
+ client := hydra.NewOAuth2ApiWithBasePath(server.URL)
+ client.Configuration.Username = "my-client"
+ client.Configuration.Password = "foobar"
- conf := &goauth2.Config{
- Scopes: []string{},
- Endpoint: goauth2.Endpoint{},
- }
-
- ep, err := url.Parse(ts.URL)
- if err != nil {
- logrus.Fatalf("%s", err)
- }
- introspectors["http"] = &oauth2.HTTPIntrospector{
- Endpoint: ep,
- Client: conf.Client(goauth2.NoContext, &goauth2.Token{
- AccessToken: tokens[1][1],
- Expiry: now.Add(time.Hour),
- TokenType: "bearer",
- }),
- }
-}
-
-func TestIntrospect(t *testing.T) {
- for k, w := range introspectors {
- for _, c := range []struct {
- token string
- expectErr bool
- scopes []string
- assert func(*oauth2.Introspection)
+ t.Run("TestIntrospect", func(t *testing.T) {
+ for k, c := range []struct {
+ token string
+ description string
+ expectErr bool
+ scopes []string
+ assert func(*testing.T, *hydra.OAuth2TokenIntrospection)
}{
{
- token: "invalid",
- expectErr: true,
+ description: "should fail because invalid token was supplied",
+ token: "invalid",
+ expectErr: true,
+ },
+ {
+ description: "should fail because token is expired",
+ token: tokens[2][1],
+ expectErr: true,
},
{
- token: tokens[2][1],
- expectErr: true,
+ description: "should pass",
+ token: tokens[1][1],
+ expectErr: false,
},
{
- token: tokens[1][1],
- expectErr: true,
+ description: "should fail because scope `foo.bar` was requested but only `foo` is granted",
+ token: tokens[1][1],
+ expectErr: true,
+ scopes: []string{"foo.bar"},
},
{
- token: tokens[0][1],
- expectErr: false,
+ description: "should pass",
+ token: tokens[0][1],
+ expectErr: false,
},
{
- token: tokens[0][1],
- expectErr: false,
- scopes: []string{"foo.bar"},
- assert: func(c *oauth2.Introspection) {
- assert.Equal(t, "alice", c.Subject)
- //assert.Equal(t, "tests", c.Issuer)
- assert.Equal(t, now.Add(time.Hour).Unix(), c.ExpiresAt, "expires at")
- assert.Equal(t, now.Unix(), c.IssuedAt, "issued at")
- assert.Equal(t, "foobariss", c.Issuer, "issuer")
- assert.Equal(t, map[string]interface{}{"foo": "bar"}, c.Extra)
+ description: "should pass",
+ token: tokens[0][1],
+ expectErr: false,
+ scopes: []string{"foo.bar"},
+ assert: func(t *testing.T, c *hydra.OAuth2TokenIntrospection) {
+ assert.Equal(t, "alice", c.Sub)
+ assert.Equal(t, now.Add(time.Hour).Unix(), c.Exp, "expires at")
+ assert.Equal(t, now.Unix(), c.Iat, "issued at")
+ assert.Equal(t, "foobariss", c.Iss, "issuer")
+ assert.Equal(t, map[string]interface{}{"foo": "bar"}, c.Ext)
},
},
} {
- t.Run(fmt.Sprintf("case=%s", k), func(t *testing.T) {
- ctx, err := w.IntrospectToken(context.Background(), c.token, c.scopes...)
+ t.Run(fmt.Sprintf("case=%d", k), func(t *testing.T) {
+ ctx, response, err := client.IntrospectOAuth2Token(c.token, strings.Join(c.scopes, " "))
+ require.NoError(t, err)
+ require.EqualValues(t, http.StatusOK, response.StatusCode)
+ t.Logf("Got %s", response.Payload)
+
if c.expectErr {
- require.Error(t, err)
+ assert.False(t, ctx.Active)
} else {
- require.NoError(t, err)
+ assert.True(t, ctx.Active)
}
- if err == nil && c.assert != nil {
- c.assert(ctx)
+
+ if !c.expectErr && c.assert != nil {
+ c.assert(t, ctx)
}
})
}
- }
+ })
}
diff --git a/oauth2/oauth2_auth_code_test.go b/oauth2/oauth2_auth_code_test.go
index b9a899cde37..acf42d67765 100644
--- a/oauth2/oauth2_auth_code_test.go
+++ b/oauth2/oauth2_auth_code_test.go
@@ -10,7 +10,7 @@ import (
"time"
"github.com/julienschmidt/httprouter"
- . "github.com/ory/hydra/oauth2"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/stretchr/testify/assert"
"github.com/stretchr/testify/require"
"golang.org/x/oauth2"
@@ -21,19 +21,22 @@ func TestAuthCode(t *testing.T) {
var validConsent bool
router.GET("/consent", func(w http.ResponseWriter, r *http.Request, _ httprouter.Params) {
- cr, err := consentClient.GetConsentRequest(r.URL.Query().Get("consent"))
+ cr, response, err := consentClient.GetOAuth2ConsentRequest(r.URL.Query().Get("consent"))
assert.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
assert.EqualValues(t, []string{"hydra.*", "offline"}, cr.RequestedScopes)
- assert.Equal(t, r.URL.Query().Get("consent"), cr.ID)
- assert.True(t, strings.Contains(cr.RedirectURL, "oauth2/auth?client_id=app-client"))
+ assert.Equal(t, r.URL.Query().Get("consent"), cr.Id)
+ assert.True(t, strings.Contains(cr.RedirectUrl, "oauth2/auth?client_id=app-client"))
- require.NoError(t, consentClient.AcceptConsentRequest(r.URL.Query().Get("consent"), &AcceptConsentRequestPayload{
+ response, err = consentClient.AcceptOAuth2ConsentRequest(r.URL.Query().Get("consent"), hydra.ConsentRequestAcceptance{
Subject: "foo",
GrantScopes: []string{"hydra.*", "offline"},
- }))
+ })
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNoContent, response.StatusCode)
- http.Redirect(w, r, cr.RedirectURL, http.StatusFound)
+ http.Redirect(w, r, cr.RedirectUrl, http.StatusFound)
validConsent = true
})
diff --git a/oauth2/oauth2_test.go b/oauth2/oauth2_test.go
index d5fa4f96c52..3418caaadb3 100644
--- a/oauth2/oauth2_test.go
+++ b/oauth2/oauth2_test.go
@@ -14,6 +14,7 @@ import (
hcompose "github.com/ory/hydra/compose"
. "github.com/ory/hydra/oauth2"
"github.com/ory/hydra/pkg"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/ory/ladon"
"github.com/sirupsen/logrus"
"golang.org/x/oauth2"
@@ -83,7 +84,7 @@ var localWarden, httpClient = hcompose.NewMockFirewall("foo", "app-client", fosi
var consentHandler *ConsentSessionHandler
var consentManager = NewConsentRequestMemoryManager()
-var consentClient *HTTPConsentManager
+var consentClient *hydra.OAuth2Api
func init() {
consentHandler = &ConsentSessionHandler{
@@ -99,8 +100,8 @@ func init() {
consentHandler.SetRoutes(router)
h, _ := hasher.Hash([]byte("secret"))
- u, _ := url.Parse(ts.URL + ConsentRequestPath)
- consentClient = &HTTPConsentManager{Client: httpClient, Endpoint: u}
+ consentClient = hydra.NewOAuth2ApiWithBasePath(ts.URL)
+ consentClient.Configuration.Transport = httpClient.Transport
c, _ := url.Parse(ts.URL + "/consent")
handler.ConsentURL = *c
diff --git a/oauth2/revocator.go b/oauth2/revocator.go
deleted file mode 100644
index 886fc449c84..00000000000
--- a/oauth2/revocator.go
+++ /dev/null
@@ -1,7 +0,0 @@
-package oauth2
-
-import "context"
-
-type Revocator interface {
- RevokeToken(ctx context.Context, token string) error
-}
diff --git a/oauth2/revocator_http.go b/oauth2/revocator_http.go
deleted file mode 100644
index af4a167eb4b..00000000000
--- a/oauth2/revocator_http.go
+++ /dev/null
@@ -1,49 +0,0 @@
-package oauth2
-
-import (
- "bytes"
- "context"
- "io/ioutil"
- "net/http"
- "net/url"
- "strconv"
-
- "github.com/pkg/errors"
- "golang.org/x/oauth2/clientcredentials"
-)
-
-type HTTPRecovator struct {
- Config *clientcredentials.Config
- Dry bool
- Endpoint *url.URL
- Client *http.Client
-}
-
-func (r *HTTPRecovator) RevokeToken(ctx context.Context, token string) error {
- var ep = *r.Endpoint
- ep.Path = RevocationPath
-
- data := url.Values{"token": []string{token}}
- hreq, err := http.NewRequest("POST", ep.String(), bytes.NewBufferString(data.Encode()))
- if err != nil {
- return errors.WithStack(err)
- }
-
- hreq.Header.Add("Content-Type", "application/x-www-form-urlencoded")
- hreq.Header.Add("Content-Length", strconv.Itoa(len(data.Encode())))
- hreq.SetBasicAuth(r.Config.ClientID, r.Config.ClientSecret)
- if r.Client == nil {
- r.Client = http.DefaultClient
- }
- hres, err := r.Client.Do(hreq)
- if err != nil {
- return errors.WithStack(err)
- }
- defer hres.Body.Close()
-
- body, _ := ioutil.ReadAll(hres.Body)
- if hres.StatusCode < 200 || hres.StatusCode >= 300 {
- return errors.Errorf("Expected 2xx status code but got %d.\n%s", hres.StatusCode, body)
- }
- return nil
-}
diff --git a/oauth2/revocator_test.go b/oauth2/revocator_test.go
index a34b8d23a09..6addb61ae99 100644
--- a/oauth2/revocator_test.go
+++ b/oauth2/revocator_test.go
@@ -1,13 +1,12 @@
package oauth2_test
import (
+ "fmt"
"net/http/httptest"
- "net/url"
"testing"
"time"
- "context"
- "fmt"
+ "net/http"
"github.com/julienschmidt/httprouter"
"github.com/ory/fosite"
@@ -16,26 +15,35 @@ import (
"github.com/ory/herodot"
"github.com/ory/hydra/oauth2"
"github.com/ory/hydra/pkg"
- "github.com/sirupsen/logrus"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
"github.com/stretchr/testify/assert"
"github.com/stretchr/testify/require"
- "golang.org/x/oauth2/clientcredentials"
)
-var (
- revocators = make(map[string]oauth2.Revocator)
- nowRecovator = time.Now().Round(time.Second)
- tokensRecovator = pkg.Tokens(3)
- fositeStoreRecovator = storage.NewExampleStore()
-)
+func createAccessTokenSession(subject, client string, token string, expiresAt time.Time, fs *storage.MemoryStore, scopes fosite.Arguments) {
+ ar := fosite.NewAccessRequest(oauth2.NewSession(subject))
+ ar.GrantedScopes = fosite.Arguments{"core"}
+ if scopes != nil {
+ ar.GrantedScopes = scopes
+ }
+ ar.RequestedAt = time.Now().Round(time.Minute)
+ ar.Client = &fosite.DefaultClient{ID: client}
+ ar.Session.SetExpiresAt(fosite.AccessToken, expiresAt)
+ ar.Session.(*oauth2.Session).Extra = map[string]interface{}{"foo": "bar"}
+ fs.CreateAccessTokenSession(nil, token, ar)
+}
-func init() {
+func TestRevoke(t *testing.T) {
+ var (
+ tokens = pkg.Tokens(3)
+ store = storage.NewExampleStore()
+ now = time.Now().Round(time.Second)
+ )
- r := httprouter.New()
- serv := &oauth2.Handler{
+ handler := &oauth2.Handler{
OAuth2: compose.Compose(
fc,
- fositeStoreRecovator,
+ store,
&compose.CommonStrategy{
CoreStrategy: compose.NewOAuth2HMACStrategy(fc, []byte("1234567890123456789012345678901234567890")),
OpenIDConnectTokenStrategy: compose.NewOpenIDConnectStrategy(pkg.MustRSAKey()),
@@ -46,95 +54,56 @@ func init() {
),
H: herodot.NewJSONWriter(nil),
}
- serv.SetRoutes(r)
- ts = httptest.NewServer(r)
- ar := fosite.NewAccessRequest(oauth2.NewSession("alice"))
- ar.GrantedScopes = fosite.Arguments{"core"}
- ar.RequestedAt = nowRecovator
- ar.Client = &fosite.DefaultClient{ID: "siri"}
- ar.Session.SetExpiresAt(fosite.AccessToken, nowRecovator.Add(time.Hour))
- ar.Session.(*oauth2.Session).Extra = map[string]interface{}{"foo": "bar"}
- fositeStoreRecovator.CreateAccessTokenSession(nil, tokensRecovator[0][0], ar)
+ router := httprouter.New()
+ handler.SetRoutes(router)
+ server := httptest.NewServer(router)
- ar2 := fosite.NewAccessRequest(oauth2.NewSession("siri"))
- ar2.GrantedScopes = fosite.Arguments{"core"}
- ar2.RequestedAt = nowRecovator
- ar2.Session.(*oauth2.Session).Extra = map[string]interface{}{"foo": "bar"}
- ar2.Session.SetExpiresAt(fosite.AccessToken, nowRecovator.Add(time.Hour))
- ar2.Client = &fosite.DefaultClient{ID: "siri"}
- fositeStoreRecovator.CreateAccessTokenSession(nil, tokensRecovator[1][0], ar2)
+ createAccessTokenSession("alice", "siri", tokens[0][0], now.Add(time.Hour), store, nil)
+ createAccessTokenSession("siri", "siri", tokens[1][0], now.Add(time.Hour), store, nil)
+ createAccessTokenSession("siri", "doesnt-exist", tokens[2][0], now.Add(-time.Hour), store, nil)
- ar3 := fosite.NewAccessRequest(oauth2.NewSession("siri"))
- ar3.GrantedScopes = fosite.Arguments{"core"}
- ar3.RequestedAt = nowRecovator
- ar3.Session.(*oauth2.Session).Extra = map[string]interface{}{"foo": "bar"}
- ar3.Client = &fosite.DefaultClient{ID: "doesnt-exist"}
- ar3.Session.SetExpiresAt(fosite.AccessToken, nowRecovator.Add(-time.Hour))
- fositeStoreRecovator.CreateAccessTokenSession(nil, tokensRecovator[2][0], ar3)
+ client := hydra.NewOAuth2ApiWithBasePath(server.URL)
+ client.Configuration.Username = "my-client"
+ client.Configuration.Password = "foobar"
- ep, err := url.Parse(ts.URL)
- if err != nil {
- logrus.Fatalf("%s", err)
- }
- revocators["http"] = &oauth2.HTTPRecovator{
- Endpoint: ep,
- Config: &clientcredentials.Config{
- ClientID: "my-client",
- ClientSecret: "foobar",
+ for k, c := range []struct {
+ token string
+ assert func(*testing.T)
+ }{
+ {
+ token: "invalid",
},
- }
-}
-
-func TestRevoke(t *testing.T) {
- for k, w := range revocators {
- for _, c := range []struct {
- token string
- expectErr bool
- assert func(*testing.T)
- }{
- {
- token: "invalid",
- expectErr: false,
- },
- {
- token: tokensRecovator[0][1],
- expectErr: false,
- assert: func(t *testing.T) {
- assert.Len(t, fositeStoreRecovator.AccessTokens, 2)
- },
+ {
+ token: tokens[0][1],
+ assert: func(t *testing.T) {
+ assert.Len(t, store.AccessTokens, 2)
},
- {
- token: tokensRecovator[0][1],
- expectErr: false,
- },
- {
- token: tokensRecovator[2][1],
- expectErr: false,
- assert: func(t *testing.T) {
- assert.Len(t, fositeStoreRecovator.AccessTokens, 1)
- },
+ },
+ {
+ token: tokens[0][1],
+ },
+ {
+ token: tokens[2][1],
+ assert: func(t *testing.T) {
+ assert.Len(t, store.AccessTokens, 1)
},
- {
- token: tokensRecovator[1][1],
- expectErr: false,
- assert: func(t *testing.T) {
- assert.Len(t, fositeStoreRecovator.AccessTokens, 0)
- },
+ },
+ {
+ token: tokens[1][1],
+ assert: func(t *testing.T) {
+ assert.Len(t, store.AccessTokens, 0)
},
- } {
- t.Run(fmt.Sprintf("case=%s", k), func(t *testing.T) {
- err := w.RevokeToken(context.Background(), c.token)
- if c.expectErr {
- require.Error(t, err)
- } else {
- require.NoError(t, err)
- }
+ },
+ } {
+ t.Run(fmt.Sprintf("case=%d", k), func(t *testing.T) {
+ response, err := client.RevokeOAuth2Token(c.token)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
- if c.assert != nil {
- c.assert(t)
- }
- })
- }
+ if c.assert != nil {
+ c.assert(t)
+ }
+ })
}
}
diff --git a/policy/doc.go b/policy/doc.go
index e6f10ba9c59..4688e30acfb 100644
--- a/policy/doc.go
+++ b/policy/doc.go
@@ -1,17 +1,29 @@
// Package policy offers management capabilities for access control policies.
-// To read up on policies, go to:
//
-// - https://github.com/ory/ladon
+// Access Control Policies (ACP) are a concept similar to Role Based Access Control and Access Control Lists. ACPs
+// however are more flexible and capable of handling complex and abstract access control scenarios. A ACP answers "**Who**
+// is **able** to do **what** on **something** given a **context**."
//
-// - https://ory-am.gitbooks.io/hydra/content/policy.html
//
-// Contains source files:
+// ACPs have five attributes:
//
-// - handler.go: A HTTP handler capable of managing policies.
+// - Subject *(who)*: An arbitrary unique subject name, for example "ken" or "printer-service.mydomain.com".
+// - Effect *(able)*: The effect which can be either "allow" or "deny".
+// - Action *(what)*: An arbitrary action name, for example "delete", "create" or "scoped:action:something".
+// - Resource *(something)*: An arbitrary unique resource name, for example "something", "resources.articles.1234" or some uniform resource name like "urn:isbn:3827370191".
+// - Condition *(context)*: An optional condition that evaluates the context (e.g. IP Address, request datetime, resource owner name, department, ...). Different strategies are available to evaluate conditions:
+// - https://github.com/ory/ladon#cidr-condition
+// - https://github.com/ory/ladon#string-equal-condition
+// - https://github.com/ory/ladon#string-match-condition
+// - https://github.com/ory/ladon#subject-condition
+// - https://github.com/ory/ladon#string-pairs-equal-condition
//
-// - warden_http.go: A Go API using HTTP to validate managing policies.
//
-// - warden_test.go: Functional tests all of the above.
+// You can find more information on ACPs here:
+//
+// - https://github.com/ory/ladon#usage for more information on policy usage.
+//
+// - https://github.com/ory/ladon#concepts
package policy
// swagger:parameters listPolicies
@@ -29,14 +41,14 @@ type swaggerListPolicyParameters struct {
type swaggerGetPolicyParameters struct {
// The id of the policy.
// in: path
- ID int `json:"id"`
+ ID string `json:"id"`
}
// swagger:parameters updatePolicy
type swaggerUpdatePolicyParameters struct {
// The id of the policy.
// in: path
- ID int `json:"id"`
+ ID string `json:"id"`
// in: body
Body swaggerPolicy
@@ -49,34 +61,35 @@ type swaggerCreatePolicyParameters struct {
}
// A policy
-// swagger:response listPolicyResponse
+// swagger:response policyList
type swaggerListPolicyResponse struct {
// in: body
- Body swaggerPolicy
+ // type: array
+ Body []swaggerPolicy
}
// swagger:model policy
type swaggerPolicy struct {
// ID of the policy.
- ID string `json:"id" gorethink:"id"`
+ ID string `json:"id"`
// Description of the policy.
- Description string `json:"description" gorethink:"description"`
+ Description string `json:"description"`
// Subjects impacted by the policy.
- Subjects []string `json:"subjects" gorethink:"subjects"`
+ Subjects []string `json:"subjects"`
// Effect of the policy
- Effect string `json:"effect" gorethink:"effect"`
+ Effect string `json:"effect"`
// Resources impacted by the policy.
- Resources []string `json:"resources" gorethink:"resources"`
+ Resources []string `json:"resources"`
// Actions impacted by the policy.
- Actions []string `json:"actions" gorethink:"actions"`
+ Actions []string `json:"actions"`
// Conditions under which the policy is active.
Conditions map[string]struct {
- Type string `json:"type"`
- Options interface{} `json:"options"`
- }
+ Type string `json:"type"`
+ Options map[string]interface{} `json:"options"`
+ } `json:"conditions"`
}
diff --git a/policy/handler.go b/policy/handler.go
index 33171650926..d1a83a754e2 100644
--- a/policy/handler.go
+++ b/policy/handler.go
@@ -10,6 +10,7 @@ import (
"github.com/julienschmidt/httprouter"
"github.com/ory/herodot"
"github.com/ory/hydra/firewall"
+ "github.com/ory/hydra/pkg"
"github.com/ory/ladon"
"github.com/pborman/uuid"
"github.com/pkg/errors"
@@ -36,11 +37,9 @@ func (h *Handler) SetRoutes(r *httprouter.Router) {
r.DELETE(endpoint+"/:id", h.Delete)
}
-// swagger:route GET /policies policies listPolicies
+// swagger:route GET /policies policy listPolicies
//
-// List access control policies
-//
-// Visit https://github.com/ory/ladon#usage for more information on policy usage.
+// List Access Control Policies
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -64,7 +63,7 @@ func (h *Handler) SetRoutes(r *httprouter.Router) {
// oauth2: hydra.policies
//
// Responses:
-// 200: listPolicyResponse
+// 200: policyList
// 401: genericError
// 403: genericError
// 500: genericError
@@ -108,11 +107,9 @@ func (h *Handler) List(w http.ResponseWriter, r *http.Request, _ httprouter.Para
h.H.Write(w, r, policies)
}
-// swagger:route POST /policies policies createPolicy
-//
-// Create an access control policy
+// swagger:route POST /policies policy createPolicy
//
-// Visit https://github.com/ory/ladon#usage for more information on policy usage.
+// Create an Access Control Policy
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -170,11 +167,9 @@ func (h *Handler) Create(w http.ResponseWriter, r *http.Request, _ httprouter.Pa
h.H.WriteCreated(w, r, "/policies/"+p.ID, &p)
}
-// swagger:route GET /policies/{id} policies getPolicy
-//
-// Get an access control policy
+// swagger:route GET /policies/{id} policy getPolicy
//
-// Visit https://github.com/ory/ladon#usage for more information on policy usage.
+// Get an Access Control Policy
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -215,17 +210,19 @@ func (h *Handler) Get(w http.ResponseWriter, r *http.Request, ps httprouter.Para
policy, err := h.Manager.Get(ps.ByName("id"))
if err != nil {
+ if err.Error() == "Not found" {
+ h.H.WriteError(w, r, errors.WithStack(pkg.ErrNotFound))
+ return
+ }
h.H.WriteError(w, r, errors.WithStack(err))
return
}
h.H.Write(w, r, policy)
}
-// swagger:route DELETE /policies/{id} policies deletePolicy
+// swagger:route DELETE /policies/{id} policy deletePolicy
//
-// Delete an access control policy
-//
-// Visit https://github.com/ory/ladon#usage for more information on policy usage.
+// Delete an Access Control Policy
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -261,23 +258,21 @@ func (h *Handler) Delete(w http.ResponseWriter, r *http.Request, ps httprouter.P
Resource: fmt.Sprintf(policiesResource, id),
Action: "get",
}, scope); err != nil {
- h.H.WriteError(w, r, err)
+ h.H.WriteError(w, r, errors.WithStack(err))
return
}
if err := h.Manager.Delete(id); err != nil {
- h.H.WriteError(w, r, errors.New("Could not delete client"))
+ h.H.WriteError(w, r, errors.WithStack(err))
return
}
w.WriteHeader(http.StatusNoContent)
}
-// swagger:route PUT /policies/{id} policies updatePolicy
-//
-// Update an access control policy
+// swagger:route PUT /policies/{id} policy updatePolicy
//
-// Visit https://github.com/ory/ladon#usage for more information on policy usage.
+// Update an Access Control Polic
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -314,7 +309,7 @@ func (h *Handler) Update(w http.ResponseWriter, r *http.Request, ps httprouter.P
Resource: fmt.Sprintf(policiesResource, id),
Action: "update",
}, scope); err != nil {
- h.H.WriteError(w, r, err)
+ h.H.WriteError(w, r, errors.WithStack(err))
return
}
diff --git a/policy/manager.go b/policy/manager.go
deleted file mode 100644
index 160a87ef9d7..00000000000
--- a/policy/manager.go
+++ /dev/null
@@ -1,23 +0,0 @@
-package policy
-
-import (
- "github.com/ory/ladon"
-)
-
-// Manager is responsible for managing and persisting policies.
-type Manager interface {
- // Create persists the policy.
- Create(policy ladon.Policy) error
-
- // Get retrieves a policy.
- Get(id string) (ladon.Policy, error)
-
- // Delete removes a policy.
- Delete(id string) error
-
- // List policies.
- List(limit, offset int64) (ladon.Policies, error)
-
- // Update a policy.
- Update(policy ladon.Policy) error
-}
diff --git a/policy/manager_http.go b/policy/manager_http.go
deleted file mode 100644
index 6fde267c983..00000000000
--- a/policy/manager_http.go
+++ /dev/null
@@ -1,108 +0,0 @@
-package policy
-
-import (
- "encoding/json"
- "fmt"
- "net/http"
- "net/url"
-
- "github.com/ory/hydra/pkg"
- "github.com/ory/ladon"
- "github.com/pkg/errors"
-)
-
-type jsonPolicy struct {
- ID string `json:"id" gorethink:"id"`
- Description string `json:"description" gorethink:"description"`
- Subjects []string `json:"subjects" gorethink:"subjects"`
- Effect string `json:"effect" gorethink:"effect"`
- Resources []string `json:"resources" gorethink:"resources"`
- Actions []string `json:"actions" gorethink:"actions"`
- Conditions json.RawMessage `json:"conditions" gorethink:"conditions"`
-}
-
-type HTTPManager struct {
- Endpoint *url.URL
- Dry bool
- FakeTLSTermination bool
- Client *http.Client
-}
-
-// Create persists the policy.
-func (m *HTTPManager) Create(policy ladon.Policy) error {
- var r = pkg.NewSuperAgent(m.Endpoint.String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Create(policy)
-}
-
-// Update the policy.
-func (m *HTTPManager) Update(policy ladon.Policy) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, policy.GetID()).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Update(policy)
-}
-
-// Get retrieves a policy.
-func (m *HTTPManager) List(limit, offset int64) (ladon.Policies, error) {
- var policies []*ladon.DefaultPolicy
- var r = pkg.NewSuperAgent(m.Endpoint.String() + fmt.Sprintf("?limit=%d&offset=%d", limit, offset))
- r.Client = m.Client
- r.FakeTLSTermination = m.FakeTLSTermination
- r.Dry = m.Dry
- if err := r.Get(&policies); err != nil {
- return nil, errors.WithStack(err)
- }
-
- ret := make(ladon.Policies, len(policies))
- for k, p := range policies {
- ret[k] = ladon.Policy(p)
- }
- return ret, nil
-}
-
-// Get retrieves a policy.
-func (m *HTTPManager) Get(id string) (ladon.Policy, error) {
- var policy = ladon.DefaultPolicy{
- Conditions: ladon.Conditions{},
- }
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id).String())
- r.Client = m.Client
- r.FakeTLSTermination = m.FakeTLSTermination
- r.Dry = m.Dry
- if err := r.Get(&policy); err != nil {
- return nil, errors.WithStack(err)
- }
-
- return &policy, nil
-}
-
-// Delete removes a policy.
-func (m *HTTPManager) Delete(id string) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Delete()
-}
-
-// Finds all policies associated with the subject.
-func (m *HTTPManager) FindPoliciesForSubject(subject string) (ladon.Policies, error) {
- var policies []*ladon.DefaultPolicy
- var r = pkg.NewSuperAgent(m.Endpoint.String() + "?subject=" + subject)
- r.Client = m.Client
- r.FakeTLSTermination = m.FakeTLSTermination
- r.Dry = m.Dry
- if err := r.Get(&policies); err != nil {
- return nil, errors.WithStack(err)
- }
-
- ret := make(ladon.Policies, len(policies))
- for k, p := range policies {
- ret[k] = ladon.Policy(p)
- }
- return ret, nil
-}
diff --git a/policy/manager_test.go b/policy/manager_test.go
deleted file mode 100644
index 4b4e1e9d719..00000000000
--- a/policy/manager_test.go
+++ /dev/null
@@ -1,94 +0,0 @@
-package policy
-
-import (
- "net/http/httptest"
- "net/url"
- "testing"
-
- "github.com/julienschmidt/httprouter"
- "github.com/ory/fosite"
- "github.com/ory/herodot"
- "github.com/ory/hydra/compose"
- "github.com/ory/ladon"
- "github.com/ory/ladon/manager/memory"
- "github.com/pborman/uuid"
- "github.com/stretchr/testify/assert"
- "github.com/stretchr/testify/require"
-)
-
-var managers = map[string]Manager{}
-
-func init() {
- localWarden, httpClient := compose.NewMockFirewall("hydra", "alice", fosite.Arguments{scope},
- &ladon.DefaultPolicy{
- ID: "1",
- Subjects: []string{"alice"},
- Resources: []string{"rn:hydra:policies<.*>"},
- Actions: []string{"create", "get", "delete", "list", "update"},
- Effect: ladon.AllowAccess,
- },
- )
-
- h := &Handler{
- Manager: &memory.MemoryManager{
- Policies: map[string]ladon.Policy{},
- },
- W: localWarden,
- H: herodot.NewJSONWriter(nil),
- }
-
- r := httprouter.New()
- h.SetRoutes(r)
- ts := httptest.NewServer(r)
-
- u, _ := url.Parse(ts.URL + endpoint)
- managers["http"] = &HTTPManager{
- Endpoint: u,
- Client: httpClient,
- }
-}
-
-func TestManagers(t *testing.T) {
- p := &ladon.DefaultPolicy{
- ID: uuid.New(),
- Description: "description",
- Subjects: []string{""},
- Effect: ladon.AllowAccess,
- Resources: []string{""},
- Actions: []string{"view"},
- Conditions: ladon.Conditions{
- "ip": &ladon.CIDRCondition{
- CIDR: "1234",
- },
- "owner": &ladon.EqualsSubjectCondition{},
- },
- }
-
- for k, m := range managers {
- t.Run("manager="+k, func(t *testing.T) {
- _, err := m.Get(p.ID)
- require.Error(t, err)
- require.NoError(t, m.Create(p))
-
- res, err := m.Get(p.ID)
- require.NoError(t, err)
- assert.Equal(t, p, res)
-
- p.Subjects = []string{"stan"}
- require.NoError(t, m.Update(p))
-
- pols, err := m.List(10, 0)
- require.NoError(t, err)
- assert.Len(t, pols, 1)
-
- res, err = m.Get(p.ID)
- require.NoError(t, err)
- assert.Equal(t, p, res)
-
- require.NoError(t, m.Delete(p.ID))
-
- _, err = m.Get(p.ID)
- assert.Error(t, err)
- })
- }
-}
diff --git a/policy/sdk_test.go b/policy/sdk_test.go
new file mode 100644
index 00000000000..25c216b1c0f
--- /dev/null
+++ b/policy/sdk_test.go
@@ -0,0 +1,118 @@
+package policy
+
+import (
+ "net/http/httptest"
+ "testing"
+
+ "encoding/json"
+ "net/http"
+
+ "github.com/julienschmidt/httprouter"
+ "github.com/ory/fosite"
+ "github.com/ory/herodot"
+ "github.com/ory/hydra/compose"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
+ "github.com/ory/ladon"
+ "github.com/ory/ladon/manager/memory"
+ "github.com/pborman/uuid"
+ "github.com/stretchr/testify/assert"
+ "github.com/stretchr/testify/require"
+)
+
+func mockPolicy(t *testing.T) hydra.Policy {
+ originalPolicy := &ladon.DefaultPolicy{
+ ID: uuid.New(),
+ Description: "description",
+ Subjects: []string{""},
+ Effect: ladon.AllowAccess,
+ Resources: []string{""},
+ Actions: []string{"view"},
+ Conditions: ladon.Conditions{
+ "ip": &ladon.CIDRCondition{
+ CIDR: "1234",
+ },
+ "owner": &ladon.EqualsSubjectCondition{},
+ },
+ }
+ out, err := json.Marshal(originalPolicy)
+ require.NoError(t, err)
+
+ var apiPolicy hydra.Policy
+ require.NoError(t, json.Unmarshal(out, &apiPolicy))
+ out, err = json.Marshal(&apiPolicy)
+ require.NoError(t, err)
+
+ var checkPolicy ladon.DefaultPolicy
+ require.NoError(t, json.Unmarshal(out, &checkPolicy))
+ require.EqualValues(t, checkPolicy.Conditions["ip"], originalPolicy.Conditions["ip"])
+ require.EqualValues(t, checkPolicy.Conditions["owner"], originalPolicy.Conditions["owner"])
+
+ return apiPolicy
+}
+
+func TestPolicySDK(t *testing.T) {
+ localWarden, httpClient := compose.NewMockFirewall("hydra", "alice", fosite.Arguments{scope},
+ &ladon.DefaultPolicy{
+ ID: "1",
+ Subjects: []string{"alice"},
+ Resources: []string{"rn:hydra:policies<.*>"},
+ Actions: []string{"create", "get", "delete", "list", "update"},
+ Effect: ladon.AllowAccess,
+ },
+ )
+
+ handler := &Handler{
+ Manager: &memory.MemoryManager{Policies: map[string]ladon.Policy{}},
+ W: localWarden,
+ H: herodot.NewJSONWriter(nil),
+ }
+
+ router := httprouter.New()
+ handler.SetRoutes(router)
+ server := httptest.NewServer(router)
+
+ client := hydra.NewPolicyApiWithBasePath(server.URL)
+ client.Configuration.Transport = httpClient.Transport
+
+ p := mockPolicy(t)
+
+ t.Run("TestPolicyManagement", func(t *testing.T) {
+ _, response, err := client.GetPolicy(p.Id)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNotFound, response.StatusCode)
+
+ result, response, err := client.CreatePolicy(p)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusCreated, response.StatusCode)
+ assert.EqualValues(t, p, *result)
+
+ result, response, err = client.GetPolicy(p.Id)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
+ assert.EqualValues(t, p, *result)
+
+ p.Subjects = []string{"stan"}
+ result, response, err = client.UpdatePolicy(p.Id, p)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
+ assert.EqualValues(t, p, *result)
+
+ results, response, err := client.ListPolicies(10, 0)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
+ assert.Len(t, results, 1)
+
+ result, response, err = client.GetPolicy(p.Id)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
+ assert.EqualValues(t, p, *result)
+
+ response, err = client.DeletePolicy(p.Id)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNoContent, response.StatusCode)
+
+ _, response, err = client.GetPolicy(p.Id)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNotFound, response.StatusCode)
+ })
+}
diff --git a/scripts/run-gensdk.sh b/scripts/run-gensdk.sh
new file mode 100755
index 00000000000..87a61d6f48c
--- /dev/null
+++ b/scripts/run-gensdk.sh
@@ -0,0 +1,22 @@
+#!/bin/bash
+
+set -euo pipefail
+
+cd "$( dirname "${BASH_SOURCE[0]}" )/.."
+
+scripts/run-genswag.sh
+
+rm -rf ./sdk/go/hydra/swagger
+rm -rf ./sdk/js/swagger
+
+# curl -O scripts/swagger-codegen-cli-2.2.3.jar http://central.maven.org/maven2/io/swagger/swagger-codegen-cli/2.2.3/swagger-codegen-cli-2.2.3.jar
+
+java -jar scripts/swagger-codegen-cli-2.2.3.jar generate -i ./docs/api.swagger.json -l go -o ./sdk/go/hydra/swagger
+java -jar scripts/swagger-codegen-cli-2.2.3.jar generate -i ./docs/api.swagger.json -l javascript -o ./sdk/js/swagger
+
+scripts/run-format.sh
+
+git add -A .
+
+git checkout HEAD -- sdk/go/hydra/swagger/configuration.go
+git checkout HEAD -- sdk/go/hydra/swagger/api_client.go
\ No newline at end of file
diff --git a/scripts/run-genswag.sh b/scripts/run-genswag.sh
index aca69582313..d47f7cfcb41 100755
--- a/scripts/run-genswag.sh
+++ b/scripts/run-genswag.sh
@@ -1,4 +1,4 @@
-#!/usr/bin/env bash
+#!/bin/bash
set -euo pipefail
diff --git a/scripts/swagger-codegen-cli-2.2.3.jar b/scripts/swagger-codegen-cli-2.2.3.jar
new file mode 100644
index 00000000000..ef3cedbd378
Binary files /dev/null and b/scripts/swagger-codegen-cli-2.2.3.jar differ
diff --git a/sdk/client.go b/sdk/client.go
deleted file mode 100644
index 4c797020661..00000000000
--- a/sdk/client.go
+++ /dev/null
@@ -1,183 +0,0 @@
-package sdk
-
-import (
- "crypto/tls"
- "net/http"
- "net/url"
- "os"
-
- "context"
-
- "github.com/ory/hydra/client"
- "github.com/ory/hydra/jwk"
- hoauth2 "github.com/ory/hydra/oauth2"
- "github.com/ory/hydra/pkg"
- "github.com/ory/hydra/policy"
- "github.com/ory/hydra/warden"
- "github.com/ory/hydra/warden/group"
- "golang.org/x/oauth2"
- "golang.org/x/oauth2/clientcredentials"
-)
-
-type option func(*Client) error
-
-// default options for hydra client
-var defaultOptions = []option{
- ClusterURL(os.Getenv("HYDRA_CLUSTER_URL")),
- ClientID(os.Getenv("HYDRA_CLIENT_ID")),
- ClientSecret(os.Getenv("HYDRA_CLIENT_SECRET")),
- Scopes("hydra"),
-}
-
-// Client offers easy use of all HTTP clients.
-type Client struct {
- // Clients offers OAuth2 Client management capabilities.
- Clients *client.HTTPManager
-
- // JSONWebKeys offers JSON Web Key management capabilities.
- JSONWebKeys *jwk.HTTPManager
-
- // Policies offers Access Policy management capabilities.
- Policies *policy.HTTPManager
-
- // Warden offers Access Token and Access Request validation strategies (for first-party resource servers).
- Warden *warden.HTTPWarden
-
- // Introspection offers Access Token and Access Request introspection strategies (according to RFC 7662).
- Introspection *hoauth2.HTTPIntrospector
-
- // Revocation offers OAuth2 Token Revocation.
- Revocator *hoauth2.HTTPRecovator
-
- // Groups offers warden group management capabilities.
- Groups *group.HTTPManager
-
- http *http.Client
- clusterURL *url.URL
- clientID string
- clientSecret string
- skipTLSVerify bool
- scopes []string
- credentials clientcredentials.Config
-}
-
-// Connect instantiates a new client to communicate with Hydra.
-//
-// import "github.com/ory-am/hydra/sdk"
-//
-// var hydra, err = sdk.Connect(
-// sdk.ClientID("client-id"),
-// sdk.ClientSecret("client-secret"),
-// sdk.ClusterURL("https://localhost:4444"),
-// )
-func Connect(opts ...option) (*Client, error) {
- c := &Client{}
-
- var err error
- // apply default options
- for _, opt := range defaultOptions {
- err = opt(c)
- if err != nil {
- return nil, err
- }
- }
-
- // override any default values with given options
- for _, opt := range opts {
- err = opt(c)
- if err != nil {
- return nil, err
- }
- }
-
- c.credentials = clientcredentials.Config{
- ClientID: c.clientID,
- ClientSecret: c.clientSecret,
- TokenURL: pkg.JoinURL(c.clusterURL, "oauth2/token").String(),
- Scopes: c.scopes,
- }
-
- c.http = http.DefaultClient
-
- if c.skipTLSVerify {
- c.http = &http.Client{
- Transport: &http.Transport{
- TLSClientConfig: &tls.Config{InsecureSkipVerify: true},
- },
- }
- }
-
- err = c.authenticate()
- if err != nil {
- return nil, err
- }
-
- // initialize service endpoints
- c.Clients = &client.HTTPManager{
- Endpoint: pkg.JoinURL(c.clusterURL, "/clients"),
- Client: c.http,
- }
-
- c.Revocator = &hoauth2.HTTPRecovator{
- Endpoint: pkg.JoinURL(c.clusterURL, hoauth2.RevocationPath),
- Config: &c.credentials,
- }
-
- c.Introspection = &hoauth2.HTTPIntrospector{
- Endpoint: pkg.JoinURL(c.clusterURL, hoauth2.IntrospectPath),
- Client: c.http,
- }
-
- c.JSONWebKeys = &jwk.HTTPManager{
- Endpoint: pkg.JoinURL(c.clusterURL, "/keys"),
- Client: c.http,
- }
-
- c.Policies = &policy.HTTPManager{
- Endpoint: pkg.JoinURL(c.clusterURL, "/policies"),
- Client: c.http,
- }
-
- c.Warden = &warden.HTTPWarden{
- Client: c.http,
- Endpoint: c.clusterURL,
- }
-
- c.Groups = &group.HTTPManager{
- Endpoint: pkg.JoinURL(c.clusterURL, "/warden/groups"),
- Client: c.http,
- }
-
- return c, nil
-}
-
-// OAuth2Config returns an oauth2 config instance which you can use to initiate various oauth2 flows.
-//
-// config := client.OAuth2Config("https://mydomain.com/oauth2_callback", "photos", "contacts.read")
-// redirectRequestTo := oauth2.AuthCodeURL()
-//
-// // in callback handler...
-// token, err := config.Exchange(oauth2.NoContext, authorizeCode)
-func (h *Client) OAuth2Config(redirectURL string, scopes ...string) *oauth2.Config {
- return &oauth2.Config{
- ClientSecret: h.clientSecret,
- ClientID: h.clientID,
- Endpoint: oauth2.Endpoint{
- TokenURL: pkg.JoinURL(h.clusterURL, "/oauth2/token").String(),
- AuthURL: pkg.JoinURL(h.clusterURL, "/oauth2/auth").String(),
- },
- Scopes: scopes,
- RedirectURL: redirectURL,
- }
-}
-
-func (h *Client) authenticate() error {
- ctx := context.WithValue(oauth2.NoContext, oauth2.HTTPClient, h.http)
- _, err := h.credentials.Token(ctx)
- if err != nil {
- return err
- }
-
- h.http = h.credentials.Client(ctx)
- return nil
-}
diff --git a/sdk/client_opts.go b/sdk/client_opts.go
deleted file mode 100644
index e96d9f64af2..00000000000
--- a/sdk/client_opts.go
+++ /dev/null
@@ -1,107 +0,0 @@
-package sdk
-
-import (
- "io/ioutil"
- "net/url"
-
- "gopkg.in/yaml.v2"
-)
-
-type hydraConfig struct {
- ClusterURL string `yaml:"cluster_url"`
- ClientID string `yaml:"client_id"`
- ClientSecret string `yaml:"client_secret"`
-}
-
-// FromYAML loads configurations from a YAML file
-func FromYAML(file string) option {
- return func(c *Client) error {
- var err error
- var config = hydraConfig{}
-
- data, err := ioutil.ReadFile(file)
- if err != nil {
- return err
- }
-
- err = yaml.Unmarshal(data, &config)
- if err != nil {
- return err
- }
-
- c.clusterURL, err = url.Parse(config.ClusterURL)
- if err != nil {
- return err
- }
-
- c.clientID = config.ClientID
- c.clientSecret = config.ClientSecret
-
- return nil
- }
-}
-
-// ClusterURL sets Hydra service URL
-//
-// var hydra, err = sdk.Connect(
-// sdk.ClientID("https://localhost:1234/"),
-// )
-func ClusterURL(urlStr string) option {
- return func(c *Client) error {
- var err error
- c.clusterURL, err = url.Parse(urlStr)
- return err
- }
-}
-
-// ClientID sets the OAuth2 Client ID.
-//
-// var hydra, err = sdk.Connect(
-// sdk.ClientID("client-id"),
-// )
-func ClientID(id string) option {
- return func(c *Client) error {
- c.clientID = id
- return nil
- }
-}
-
-// ClientSecret sets OAuth2 Client secret.
-//
-// var hydra, err = sdk.Connect(
-// sdk.ClientSecret("client-secret"),
-// )
-func ClientSecret(secret string) option {
- return func(c *Client) error {
- c.clientSecret = secret
- return nil
- }
-}
-
-// SkipTLSVerify skips TLS verification for HTTPS connections.
-//
-// var hydra, err = sdk.Connect(
-// sdk.SkipTLSVerify(),
-// )
-func SkipTLSVerify(val ...bool) option {
- return func(c *Client) error {
- if len(val) > 0 {
- c.skipTLSVerify = val[0]
- } else {
- c.skipTLSVerify = true
- }
- return nil
- }
-}
-
-// Scopes is a list of scopes that are requested in the client credentials grant.
-//
-// var hydra, err = sdk.Connect(
-// sdk.Scopes("foo", "bar"),
-// )
-func Scopes(scopes ...string) option {
- return func(c *Client) error {
- c.scopes = scopes
- return nil
- }
-}
diff --git a/sdk/client_opts_test.go b/sdk/client_opts_test.go
deleted file mode 100644
index faa2c3059e2..00000000000
--- a/sdk/client_opts_test.go
+++ /dev/null
@@ -1,99 +0,0 @@
-package sdk
-
-import (
- "io/ioutil"
- "os"
- "testing"
-
- "github.com/stretchr/testify/assert"
- "gopkg.in/yaml.v2"
-)
-
-func TestClusterURLOption(t *testing.T) {
- c := &Client{}
- expected := "https://localhost:4444"
- err := ClusterURL(expected)(c)
-
- assert.Nil(t, err)
- assert.Equal(t, expected, c.clusterURL.String())
-}
-
-func TestClientIDOption(t *testing.T) {
- c := &Client{}
- expected := "deadbeef-dead-beef-beef"
- err := ClientID(expected)(c)
-
- assert.Nil(t, err)
- assert.Equal(t, expected, c.clientID)
-}
-
-func TestClientSecretOption(t *testing.T) {
- c := &Client{}
-
- expected := "secret"
- err := ClientSecret(expected)(c)
-
- assert.Nil(t, err)
- assert.Equal(t, expected, c.clientSecret)
-}
-
-func TestSkipSSLOption(t *testing.T) {
- c := &Client{}
-
- err := SkipTLSVerify()(c)
-
- assert.Nil(t, err)
- assert.Equal(t, true, c.skipTLSVerify)
-}
-
-func TestSkipSSLOptionValue(t *testing.T) {
- c := &Client{}
-
- err := SkipTLSVerify(false)(c)
-
- assert.Nil(t, err)
- assert.Equal(t, false, c.skipTLSVerify)
-
- err = SkipTLSVerify(true)(c)
-
- assert.Nil(t, err)
- assert.Equal(t, true, c.skipTLSVerify)
-}
-
-func TestScopesOption(t *testing.T) {
- c := &Client{}
-
- expected := []string{"a", "b", "c"}
- err := Scopes(expected...)(c)
-
- assert.Nil(t, err)
- assert.Equal(t, expected, c.scopes)
-}
-
-func TestFromYAMLOption(t *testing.T) {
- c := &Client{}
-
- conf := &hydraConfig{
- ClusterURL: "https://localhost:4444",
- ClientID: "1cfe0a5e-2533-4312-9e74-128b5aab4431",
- ClientSecret: "Q6&u=iwvTPh8r)Ar",
- }
-
- tmpFile, err := ioutil.TempFile("", "hydra_sdk")
- assert.Nil(t, err)
-
- fileContent, err := yaml.Marshal(conf)
- assert.Nil(t, err)
- defer os.Remove(tmpFile.Name())
- defer tmpFile.Close()
-
- _, err = tmpFile.Write(fileContent)
- assert.Nil(t, err)
-
- err = FromYAML(tmpFile.Name())(c)
- assert.Nil(t, err)
-
- assert.Equal(t, conf.ClusterURL, c.clusterURL.String())
- assert.Equal(t, conf.ClientID, c.clientID)
- assert.Equal(t, conf.ClientSecret, c.clientSecret)
-}
diff --git a/sdk/client_test.go b/sdk/client_test.go
deleted file mode 100644
index 951f0d0aff5..00000000000
--- a/sdk/client_test.go
+++ /dev/null
@@ -1,16 +0,0 @@
-package sdk
-
-import (
- "testing"
-
- "github.com/stretchr/testify/assert"
-)
-
-func TestConnect(t *testing.T) {
- var _, err = Connect(
- ClientID("client-id"),
- ClientSecret("client-secret"),
- ClusterURL("https://localhost:4444"),
- )
- assert.NotNil(t, err)
-}
diff --git a/sdk/doc.go b/sdk/doc.go
deleted file mode 100644
index 2c1fe2eab48..00000000000
--- a/sdk/doc.go
+++ /dev/null
@@ -1,22 +0,0 @@
-// Package SDK offers convenience functions for Go code around Hydra's HTTP APIs.
-//
-// import "github.com/ory-am/hydra/sdk"
-// import "github.com/ory-am/hydra/client"
-//
-// var hydra, err = sdk.Connect(
-// sdk.ClientID("client-id"),
-// sdk.ClientSecret("client-secret"),
-// sdk.ClusterURL("https://localhost:4444"),
-// )
-//
-// // You now have access to the various API endpoints of hydra, for example the oauth2 client endpoint:
-// var newClient, err = hydra.Client.CreateClient(&client.Client{
-// ID: "deadbeef",
-// Secret: "sup3rs3cret",
-// RedirectURIs: []string{"http://yourapp/callback"},
-// // ...
-// })
-//
-// // Retrieve newly created client
-// var gotClient, err = hydra.Client.GetClient(newClient.ID)
-package sdk
diff --git a/sdk/go/hydra/sdk.go b/sdk/go/hydra/sdk.go
new file mode 100644
index 00000000000..ebbd2570f8f
--- /dev/null
+++ b/sdk/go/hydra/sdk.go
@@ -0,0 +1,91 @@
+package hydra
+
+import (
+ "github.com/ory/hydra/sdk/go/hydra/swagger"
+ "github.com/pkg/errors"
+ "context"
+ "golang.org/x/oauth2/clientcredentials"
+)
+
+// SDK contains all relevant API clients for interacting with ORY Hydra.
+type SDK struct {
+ *swagger.OAuth2Api
+ *swagger.JsonWebKeyApi
+ *swagger.WardenApi
+ *swagger.PolicyApi
+
+ Configuration *Configuration
+}
+
+// Configuration configures the SDK.
+type Configuration struct {
+ // EndpointURL should point to the url of ORY Hydra, for example: http://localhost:4444
+ EndpointURL string
+
+ // ClientID is the id of the management client. The management client should have appropriate access rights
+ // and the ability to request the client_credentials grant.
+ ClientID string
+
+ // ClientSecret is the secret of the management client.
+ ClientSecret string
+
+ // Scopes is a list of scopes the SDK should request. If no scopes are given, this defaults to `hydra.*`
+ Scopes []string
+}
+
+func removeTrailingSlash(path string) string {
+ for len(path) > 0 && path[len(path)-1] == '/' {
+ path = path[0: len(path)-1]
+ }
+ return path
+}
+
+// NewSDK instantiates a new SDK instance or returns an error.
+func NewSDK(c *Configuration) (*SDK, error) {
+ if c.EndpointURL == "" {
+ return nil, errors.New("Please specify an EndpointURL url")
+ }
+ if c.ClientSecret == "" {
+ return nil, errors.New("Please specify a client id")
+ }
+ if c.ClientID == "" {
+ return nil, errors.New("Please specify a client secret")
+ }
+ if len(c.Scopes) == 0 {
+ c.Scopes = []string{"hydra.*"}
+ }
+
+ c.EndpointURL = removeTrailingSlash(c.EndpointURL)
+
+ oAuth2Config := clientcredentials.Config{
+ ClientSecret: c.ClientSecret,
+ ClientID: c.ClientID,
+ Scopes: c.Scopes,
+ TokenURL: c.EndpointURL + "/oauth2/token",
+ }
+ oAuth2Client := oAuth2Config.Client(context.Background())
+
+ o := swagger.NewOAuth2ApiWithBasePath(c.EndpointURL)
+ o.Configuration.Transport = oAuth2Client.Transport
+ o.Configuration.Username = c.ClientID
+ o.Configuration.Password = c.ClientSecret
+
+ j := swagger.NewJsonWebKeyApiWithBasePath(c.EndpointURL)
+ j.Configuration.Transport = oAuth2Client.Transport
+
+ w := swagger.NewWardenApiWithBasePath(c.EndpointURL)
+ w.Configuration.Transport = oAuth2Client.Transport
+
+ p := swagger.NewPolicyApiWithBasePath(c.EndpointURL)
+ p.Configuration.Transport = oAuth2Client.Transport
+
+ sdk := &SDK{
+ OAuth2Api: o,
+ JsonWebKeyApi: j,
+ WardenApi: w,
+ PolicyApi: p,
+ Configuration: c,
+ }
+
+ return sdk, nil
+}
diff --git a/sdk/go/hydra/swagger/.gitignore b/sdk/go/hydra/swagger/.gitignore
new file mode 100644
index 00000000000..daf913b1b34
--- /dev/null
+++ b/sdk/go/hydra/swagger/.gitignore
@@ -0,0 +1,24 @@
+# Compiled Object files, Static and Dynamic libs (Shared Objects)
+*.o
+*.a
+*.so
+
+# Folders
+_obj
+_test
+
+# Architecture specific extensions/prefixes
+*.[568vq]
+[568vq].out
+
+*.cgo1.go
+*.cgo2.c
+_cgo_defun.c
+_cgo_gotypes.go
+_cgo_export.*
+
+_testmain.go
+
+*.exe
+*.test
+*.prof
diff --git a/sdk/go/hydra/swagger/.swagger-codegen-ignore b/sdk/go/hydra/swagger/.swagger-codegen-ignore
new file mode 100644
index 00000000000..c5fa491b4c5
--- /dev/null
+++ b/sdk/go/hydra/swagger/.swagger-codegen-ignore
@@ -0,0 +1,23 @@
+# Swagger Codegen Ignore
+# Generated by swagger-codegen https://github.com/swagger-api/swagger-codegen
+
+# Use this file to prevent files from being overwritten by the generator.
+# The patterns follow closely to .gitignore or .dockerignore.
+
+# As an example, the C# client generator defines ApiClient.cs.
+# You can make changes and tell Swagger Codgen to ignore just this file by uncommenting the following line:
+#ApiClient.cs
+
+# You can match any string of characters against a directory, file or extension with a single asterisk (*):
+#foo/*/qux
+# The above matches foo/bar/qux and foo/baz/qux, but not foo/bar/baz/qux
+
+# You can recursively match patterns against a directory, file or extension with a double asterisk (**):
+#foo/**/qux
+# This matches foo/bar/qux, foo/baz/qux, and foo/bar/baz/qux
+
+# You can also negate patterns with an exclamation (!).
+# For example, you can ignore all files in a docs folder with the file extension .md:
+#docs/*.md
+# Then explicitly reverse the ignore rule for a single file:
+#!docs/README.md
diff --git a/sdk/go/hydra/swagger/.swagger-codegen/VERSION b/sdk/go/hydra/swagger/.swagger-codegen/VERSION
new file mode 100644
index 00000000000..6b4d1577382
--- /dev/null
+++ b/sdk/go/hydra/swagger/.swagger-codegen/VERSION
@@ -0,0 +1 @@
+2.2.3
\ No newline at end of file
diff --git a/sdk/go/hydra/swagger/.travis.yml b/sdk/go/hydra/swagger/.travis.yml
new file mode 100644
index 00000000000..f5cb2ce9a5a
--- /dev/null
+++ b/sdk/go/hydra/swagger/.travis.yml
@@ -0,0 +1,8 @@
+language: go
+
+install:
+ - go get -d -v .
+
+script:
+ - go build -v ./
+
diff --git a/sdk/go/hydra/swagger/README.md b/sdk/go/hydra/swagger/README.md
new file mode 100644
index 00000000000..ab01427e90d
--- /dev/null
+++ b/sdk/go/hydra/swagger/README.md
@@ -0,0 +1,150 @@
+# Go API client for swagger
+
+Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 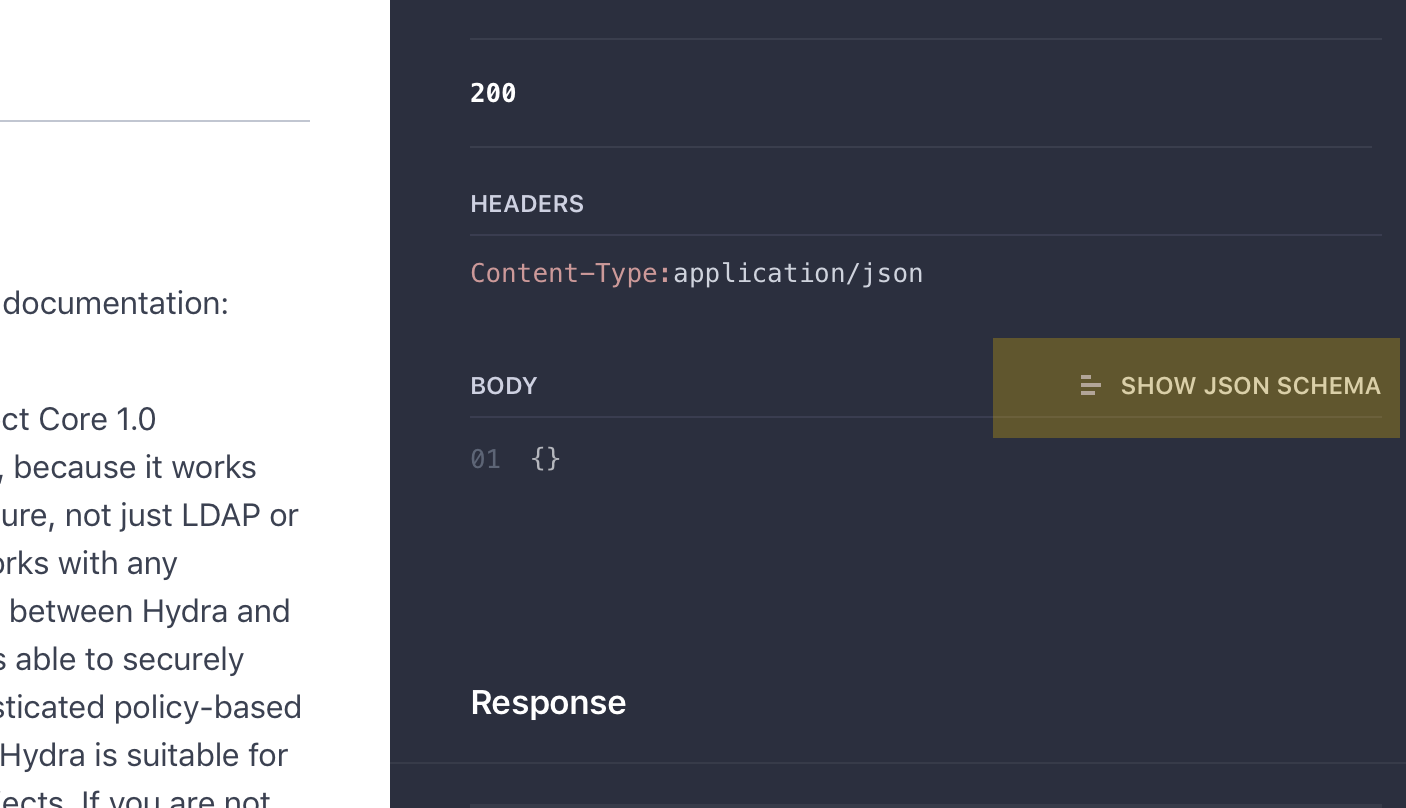 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+
+## Overview
+This API client was generated by the [swagger-codegen](https://github.com/swagger-api/swagger-codegen) project. By using the [swagger-spec](https://github.com/swagger-api/swagger-spec) from a remote server, you can easily generate an API client.
+
+- API version: Latest
+- Package version: 1.0.0
+- Build package: io.swagger.codegen.languages.GoClientCodegen
+For more information, please visit [https://www.ory.am](https://www.ory.am)
+
+## Installation
+Put the package under your project folder and add the following in import:
+```
+ "./swagger"
+```
+
+## Documentation for API Endpoints
+
+All URIs are relative to *http://localhost*
+
+Class | Method | HTTP request | Description
+------------ | ------------- | ------------- | -------------
+*HealthApi* | [**GetInstanceMetrics**](docs/HealthApi.md#getinstancemetrics) | **Get** /health/metrics | Show instance metrics (experimental)
+*HealthApi* | [**GetInstanceStatus**](docs/HealthApi.md#getinstancestatus) | **Get** /health/status | Check health status of this instance
+*JsonWebKeyApi* | [**CreateJsonWebKeySet**](docs/JsonWebKeyApi.md#createjsonwebkeyset) | **Post** /keys/{set} | Generate a new JSON Web Key
+*JsonWebKeyApi* | [**DeleteJsonWebKey**](docs/JsonWebKeyApi.md#deletejsonwebkey) | **Delete** /keys/{set}/{kid} | Delete a JSON Web Key
+*JsonWebKeyApi* | [**DeleteJsonWebKeySet**](docs/JsonWebKeyApi.md#deletejsonwebkeyset) | **Delete** /keys/{set} | Delete a JSON Web Key
+*JsonWebKeyApi* | [**GetJsonWebKey**](docs/JsonWebKeyApi.md#getjsonwebkey) | **Get** /keys/{set}/{kid} | Retrieve a JSON Web Key
+*JsonWebKeyApi* | [**GetJsonWebKeySet**](docs/JsonWebKeyApi.md#getjsonwebkeyset) | **Get** /keys/{set} | Retrieve a JSON Web Key Set
+*JsonWebKeyApi* | [**UpdateJsonWebKey**](docs/JsonWebKeyApi.md#updatejsonwebkey) | **Put** /keys/{set}/{kid} | Update a JSON Web Key
+*JsonWebKeyApi* | [**UpdateJsonWebKeySet**](docs/JsonWebKeyApi.md#updatejsonwebkeyset) | **Put** /keys/{set} | Update a JSON Web Key Set
+*OAuth2Api* | [**AcceptOAuth2ConsentRequest**](docs/OAuth2Api.md#acceptoauth2consentrequest) | **Patch** /oauth2/consent/requests/{id}/accept | Accept a consent request
+*OAuth2Api* | [**CreateOAuth2Client**](docs/OAuth2Api.md#createoauth2client) | **Post** /clients | Create an OAuth 2.0 client
+*OAuth2Api* | [**DeleteOAuth2Client**](docs/OAuth2Api.md#deleteoauth2client) | **Delete** /clients/{id} | Deletes an OAuth 2.0 Client
+*OAuth2Api* | [**GetOAuth2Client**](docs/OAuth2Api.md#getoauth2client) | **Get** /clients/{id} | Retrieve an OAuth 2.0 Client.
+*OAuth2Api* | [**GetOAuth2ConsentRequest**](docs/OAuth2Api.md#getoauth2consentrequest) | **Get** /oauth2/consent/requests/{id} | Receive consent request information
+*OAuth2Api* | [**GetWellKnown**](docs/OAuth2Api.md#getwellknown) | **Get** /.well-known/openid-configuration | Server well known configuration
+*OAuth2Api* | [**IntrospectOAuth2Token**](docs/OAuth2Api.md#introspectoauth2token) | **Post** /oauth2/introspect | Introspect OAuth2 tokens
+*OAuth2Api* | [**ListOAuth2Clients**](docs/OAuth2Api.md#listoauth2clients) | **Get** /clients | List OAuth 2.0 Clients
+*OAuth2Api* | [**OauthAuth**](docs/OAuth2Api.md#oauthauth) | **Get** /oauth2/auth | The OAuth 2.0 authorize endpoint
+*OAuth2Api* | [**OauthToken**](docs/OAuth2Api.md#oauthtoken) | **Post** /oauth2/token | The OAuth 2.0 token endpoint
+*OAuth2Api* | [**RejectOAuth2ConsentRequest**](docs/OAuth2Api.md#rejectoauth2consentrequest) | **Patch** /oauth2/consent/requests/{id}/reject | Reject a consent request
+*OAuth2Api* | [**RevokeOAuth2Token**](docs/OAuth2Api.md#revokeoauth2token) | **Post** /oauth2/revoke | Revoke OAuth2 tokens
+*OAuth2Api* | [**UpdateOAuth2Client**](docs/OAuth2Api.md#updateoauth2client) | **Put** /clients/{id} | Update an OAuth 2.0 Client
+*OAuth2Api* | [**WellKnown**](docs/OAuth2Api.md#wellknown) | **Get** /.well-known/jwks.json | Get list of well known JSON Web Keys
+*PolicyApi* | [**CreatePolicy**](docs/PolicyApi.md#createpolicy) | **Post** /policies | Create an Access Control Policy
+*PolicyApi* | [**DeletePolicy**](docs/PolicyApi.md#deletepolicy) | **Delete** /policies/{id} | Delete an Access Control Policy
+*PolicyApi* | [**GetPolicy**](docs/PolicyApi.md#getpolicy) | **Get** /policies/{id} | Get an Access Control Policy
+*PolicyApi* | [**ListPolicies**](docs/PolicyApi.md#listpolicies) | **Get** /policies | List Access Control Policies
+*PolicyApi* | [**UpdatePolicy**](docs/PolicyApi.md#updatepolicy) | **Put** /policies/{id} | Update an Access Control Polic
+*WardenApi* | [**AddMembersToGroup**](docs/WardenApi.md#addmemberstogroup) | **Post** /warden/groups/{id}/members | Add members to a group
+*WardenApi* | [**CreateGroup**](docs/WardenApi.md#creategroup) | **Post** /warden/groups | Create a group
+*WardenApi* | [**DeleteGroup**](docs/WardenApi.md#deletegroup) | **Delete** /warden/groups/{id} | Delete a group by id
+*WardenApi* | [**DoesWardenAllowAccessRequest**](docs/WardenApi.md#doeswardenallowaccessrequest) | **Post** /warden/allowed | Check if an access request is valid (without providing an access token)
+*WardenApi* | [**DoesWardenAllowTokenAccessRequest**](docs/WardenApi.md#doeswardenallowtokenaccessrequest) | **Post** /warden/token/allowed | Check if an access request is valid (providing an access token)
+*WardenApi* | [**FindGroupsByMember**](docs/WardenApi.md#findgroupsbymember) | **Get** /warden/groups | Find groups by member
+*WardenApi* | [**GetGroup**](docs/WardenApi.md#getgroup) | **Get** /warden/groups/{id} | Get a group by id
+*WardenApi* | [**RemoveMembersFromGroup**](docs/WardenApi.md#removemembersfromgroup) | **Delete** /warden/groups/{id}/members | Remove members from a group
+
+
+## Documentation For Models
+
+ - [ConsentRequestAcceptance](docs/ConsentRequestAcceptance.md)
+ - [ConsentRequestManager](docs/ConsentRequestManager.md)
+ - [ConsentRequestRejection](docs/ConsentRequestRejection.md)
+ - [Context](docs/Context.md)
+ - [Firewall](docs/Firewall.md)
+ - [Group](docs/Group.md)
+ - [GroupMembers](docs/GroupMembers.md)
+ - [Handler](docs/Handler.md)
+ - [InlineResponse200](docs/InlineResponse200.md)
+ - [InlineResponse2001](docs/InlineResponse2001.md)
+ - [InlineResponse401](docs/InlineResponse401.md)
+ - [JoseWebKeySetRequest](docs/JoseWebKeySetRequest.md)
+ - [JsonWebKey](docs/JsonWebKey.md)
+ - [JsonWebKeySet](docs/JsonWebKeySet.md)
+ - [JsonWebKeySetGeneratorRequest](docs/JsonWebKeySetGeneratorRequest.md)
+ - [KeyGenerator](docs/KeyGenerator.md)
+ - [Manager](docs/Manager.md)
+ - [OAuth2Client](docs/OAuth2Client.md)
+ - [OAuth2TokenIntrospection](docs/OAuth2TokenIntrospection.md)
+ - [OAuth2consentRequest](docs/OAuth2consentRequest.md)
+ - [Policy](docs/Policy.md)
+ - [PolicyConditions](docs/PolicyConditions.md)
+ - [RawMessage](docs/RawMessage.md)
+ - [SwaggerAcceptConsentRequest](docs/SwaggerAcceptConsentRequest.md)
+ - [SwaggerCreatePolicyParameters](docs/SwaggerCreatePolicyParameters.md)
+ - [SwaggerDoesWardenAllowAccessRequestParameters](docs/SwaggerDoesWardenAllowAccessRequestParameters.md)
+ - [SwaggerDoesWardenAllowTokenAccessRequestParameters](docs/SwaggerDoesWardenAllowTokenAccessRequestParameters.md)
+ - [SwaggerGetPolicyParameters](docs/SwaggerGetPolicyParameters.md)
+ - [SwaggerJsonWebKeyQuery](docs/SwaggerJsonWebKeyQuery.md)
+ - [SwaggerJwkCreateSet](docs/SwaggerJwkCreateSet.md)
+ - [SwaggerJwkSetQuery](docs/SwaggerJwkSetQuery.md)
+ - [SwaggerJwkUpdateSet](docs/SwaggerJwkUpdateSet.md)
+ - [SwaggerJwkUpdateSetKey](docs/SwaggerJwkUpdateSetKey.md)
+ - [SwaggerListPolicyParameters](docs/SwaggerListPolicyParameters.md)
+ - [SwaggerListPolicyResponse](docs/SwaggerListPolicyResponse.md)
+ - [SwaggerOAuthConsentRequest](docs/SwaggerOAuthConsentRequest.md)
+ - [SwaggerOAuthConsentRequestPayload](docs/SwaggerOAuthConsentRequestPayload.md)
+ - [SwaggerOAuthIntrospectionRequest](docs/SwaggerOAuthIntrospectionRequest.md)
+ - [SwaggerOAuthIntrospectionResponse](docs/SwaggerOAuthIntrospectionResponse.md)
+ - [SwaggerOAuthTokenResponse](docs/SwaggerOAuthTokenResponse.md)
+ - [SwaggerOAuthTokenResponseBody](docs/SwaggerOAuthTokenResponseBody.md)
+ - [SwaggerRejectConsentRequest](docs/SwaggerRejectConsentRequest.md)
+ - [SwaggerRevokeOAuth2TokenParameters](docs/SwaggerRevokeOAuth2TokenParameters.md)
+ - [SwaggerUpdatePolicyParameters](docs/SwaggerUpdatePolicyParameters.md)
+ - [SwaggerWardenAccessRequestResponseParameters](docs/SwaggerWardenAccessRequestResponseParameters.md)
+ - [SwaggerWardenTokenAccessRequestResponse](docs/SwaggerWardenTokenAccessRequestResponse.md)
+ - [TokenAllowedRequest](docs/TokenAllowedRequest.md)
+ - [WardenAccessRequest](docs/WardenAccessRequest.md)
+ - [WardenAccessRequestResponse](docs/WardenAccessRequestResponse.md)
+ - [WardenTokenAccessRequest](docs/WardenTokenAccessRequest.md)
+ - [WardenTokenAccessRequestResponsePayload](docs/WardenTokenAccessRequestResponsePayload.md)
+ - [WellKnown](docs/WellKnown.md)
+ - [Writer](docs/Writer.md)
+
+
+## Documentation For Authorization
+
+
+## basic
+
+- **Type**: HTTP basic authentication
+
+## oauth2
+
+- **Type**: OAuth
+- **Flow**: accessCode
+- **Authorization URL**: https://your-hydra-instance.com/oauth2/auth
+- **Scopes**:
+ - **hydra.clients**: A scope required to manage OAuth 2.0 Clients
+ - **hydra.consent**: A scope required to fetch and modify consent requests
+ - **hydra.groups**: A scope required to manage warden groups
+ - **hydra.health**: A scope required to get health information
+ - **hydra.keys.create**: A scope required to create JSON Web Keys
+ - **hydra.keys.delete**: A scope required to delete JSON Web Keys
+ - **hydra.keys.get**: A scope required to fetch JSON Web Keys
+ - **hydra.keys.update**: A scope required to get JSON Web Keys
+ - **hydra.policies**: A scope required to manage access control policies
+ - **hydra.warden**: A scope required to make access control inquiries
+ - **offline**: A scope required when requesting refresh tokens
+ - **openid**: Request an OpenID Connect ID Token
+
+
+## Author
+
+hi@ory.am
+
diff --git a/sdk/go/hydra/swagger/api_client.go b/sdk/go/hydra/swagger/api_client.go
new file mode 100644
index 00000000000..99e76c442a8
--- /dev/null
+++ b/sdk/go/hydra/swagger/api_client.go
@@ -0,0 +1,164 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server (1.0.0-aplha1)
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### ATTENTION - IMPORTANT NOTE The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 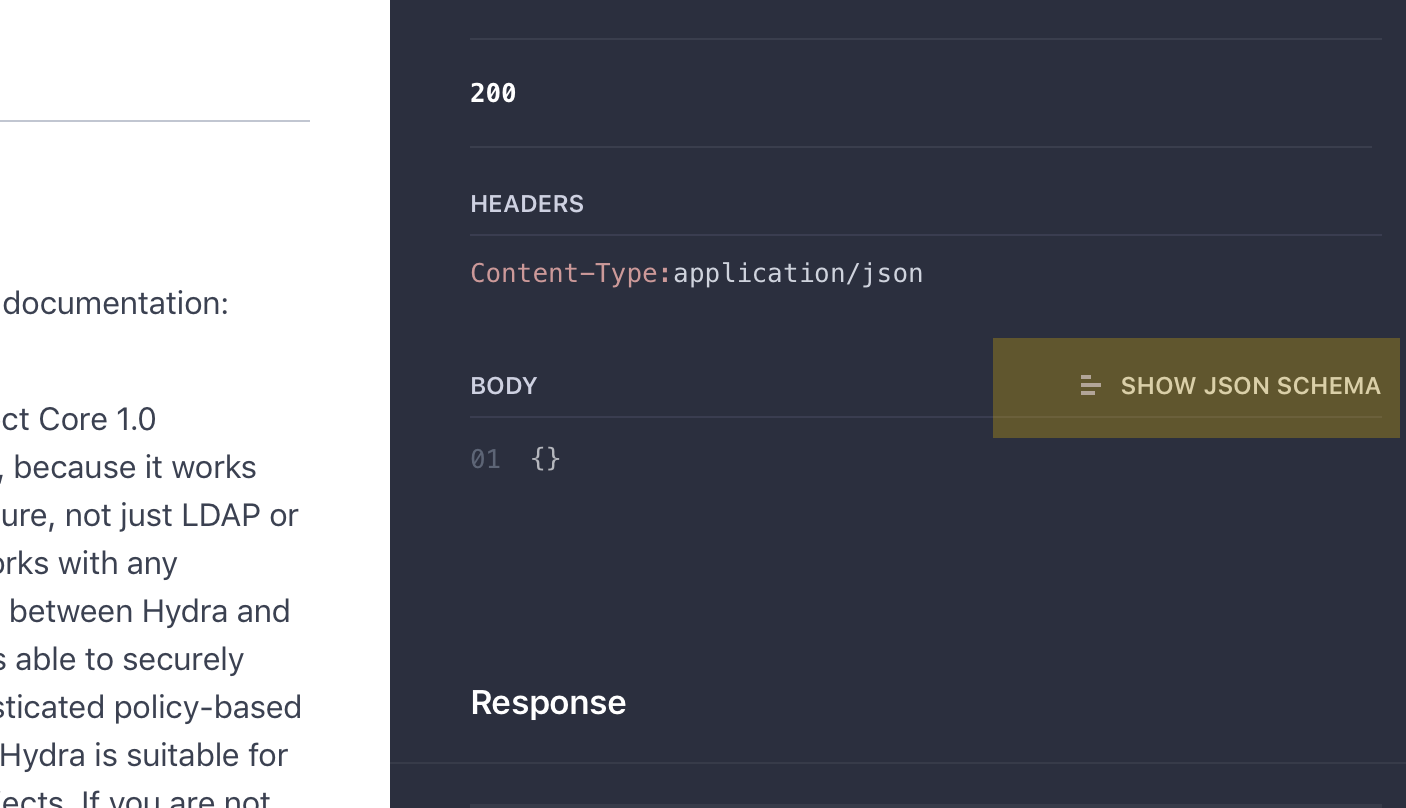
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+import (
+ "bytes"
+ "fmt"
+ "io/ioutil"
+ "net/url"
+ "path/filepath"
+ "reflect"
+ "strings"
+
+ "github.com/go-resty/resty"
+)
+
+type APIClient struct {
+ config *Configuration
+}
+
+func (c *APIClient) SelectHeaderContentType(contentTypes []string) string {
+
+ if len(contentTypes) == 0 {
+ return ""
+ }
+ if contains(contentTypes, "application/json") {
+ return "application/json"
+ }
+ return contentTypes[0] // use the first content type specified in 'consumes'
+}
+
+func (c *APIClient) SelectHeaderAccept(accepts []string) string {
+
+ if len(accepts) == 0 {
+ return ""
+ }
+ if contains(accepts, "application/json") {
+ return "application/json"
+ }
+ return strings.Join(accepts, ",")
+}
+
+func contains(haystack []string, needle string) bool {
+ for _, a := range haystack {
+ if strings.ToLower(a) == strings.ToLower(needle) {
+ return true
+ }
+ }
+ return false
+}
+
+func (c *APIClient) CallAPI(path string, method string,
+ postBody interface{},
+ headerParams map[string]string,
+ queryParams url.Values,
+ formParams map[string]string,
+ fileName string,
+ fileBytes []byte) (*resty.Response, error) {
+
+ rClient := c.prepareClient()
+ request := c.prepareRequest(rClient, postBody, headerParams, queryParams, formParams, fileName, fileBytes)
+ switch strings.ToUpper(method) {
+ case "GET":
+ response, err := request.Get(path)
+ return response, err
+ case "POST":
+ response, err := request.Post(path)
+ return response, err
+ case "PUT":
+ response, err := request.Put(path)
+ return response, err
+ case "PATCH":
+ response, err := request.Patch(path)
+ return response, err
+ case "DELETE":
+ response, err := request.Delete(path)
+ return response, err
+ }
+
+ return nil, fmt.Errorf("invalid method %v", method)
+}
+
+func (c *APIClient) ParameterToString(obj interface{}, collectionFormat string) string {
+ delimiter := ""
+ switch collectionFormat {
+ case "pipes":
+ delimiter = "|"
+ case "ssv":
+ delimiter = " "
+ case "tsv":
+ delimiter = "\t"
+ case "csv":
+ delimiter = ","
+ }
+
+ if reflect.TypeOf(obj).Kind() == reflect.Slice {
+ return strings.Trim(strings.Replace(fmt.Sprint(obj), " ", delimiter, -1), "[]")
+ }
+
+ return fmt.Sprintf("%v", obj)
+}
+
+func (c *APIClient) prepareClient() *resty.Client {
+
+ rClient := resty.New()
+
+ rClient.SetRedirectPolicy(resty.FlexibleRedirectPolicy(2))
+ rClient.SetDebug(c.config.Debug)
+ if c.config.Transport != nil {
+ rClient.SetTransport(c.config.Transport)
+ }
+
+ if c.config.Timeout != nil {
+ rClient.SetTimeout(*c.config.Timeout)
+ }
+ rClient.SetLogger(ioutil.Discard)
+ return rClient
+}
+
+func (c *APIClient) prepareRequest(
+ rClient *resty.Client,
+ postBody interface{},
+ headerParams map[string]string,
+ queryParams url.Values,
+ formParams map[string]string,
+ fileName string,
+ fileBytes []byte) *resty.Request {
+
+ request := rClient.R()
+ request.SetBody(postBody)
+
+ if c.config.UserAgent != "" {
+ request.SetHeader("User-Agent", c.config.UserAgent)
+ }
+
+ // add header parameter, if any
+ if len(headerParams) > 0 {
+ request.SetHeaders(headerParams)
+ }
+
+ // add query parameter, if any
+ if len(queryParams) > 0 {
+ request.SetMultiValueQueryParams(queryParams)
+ }
+
+ // add form parameter, if any
+ if len(formParams) > 0 {
+ request.SetFormData(formParams)
+ }
+
+ if len(fileBytes) > 0 && fileName != "" {
+ _, fileNm := filepath.Split(fileName)
+ request.SetFileReader("file", fileNm, bytes.NewReader(fileBytes))
+ }
+ return request
+}
diff --git a/sdk/go/hydra/swagger/api_response.go b/sdk/go/hydra/swagger/api_response.go
new file mode 100644
index 00000000000..447c36395c5
--- /dev/null
+++ b/sdk/go/hydra/swagger/api_response.go
@@ -0,0 +1,44 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 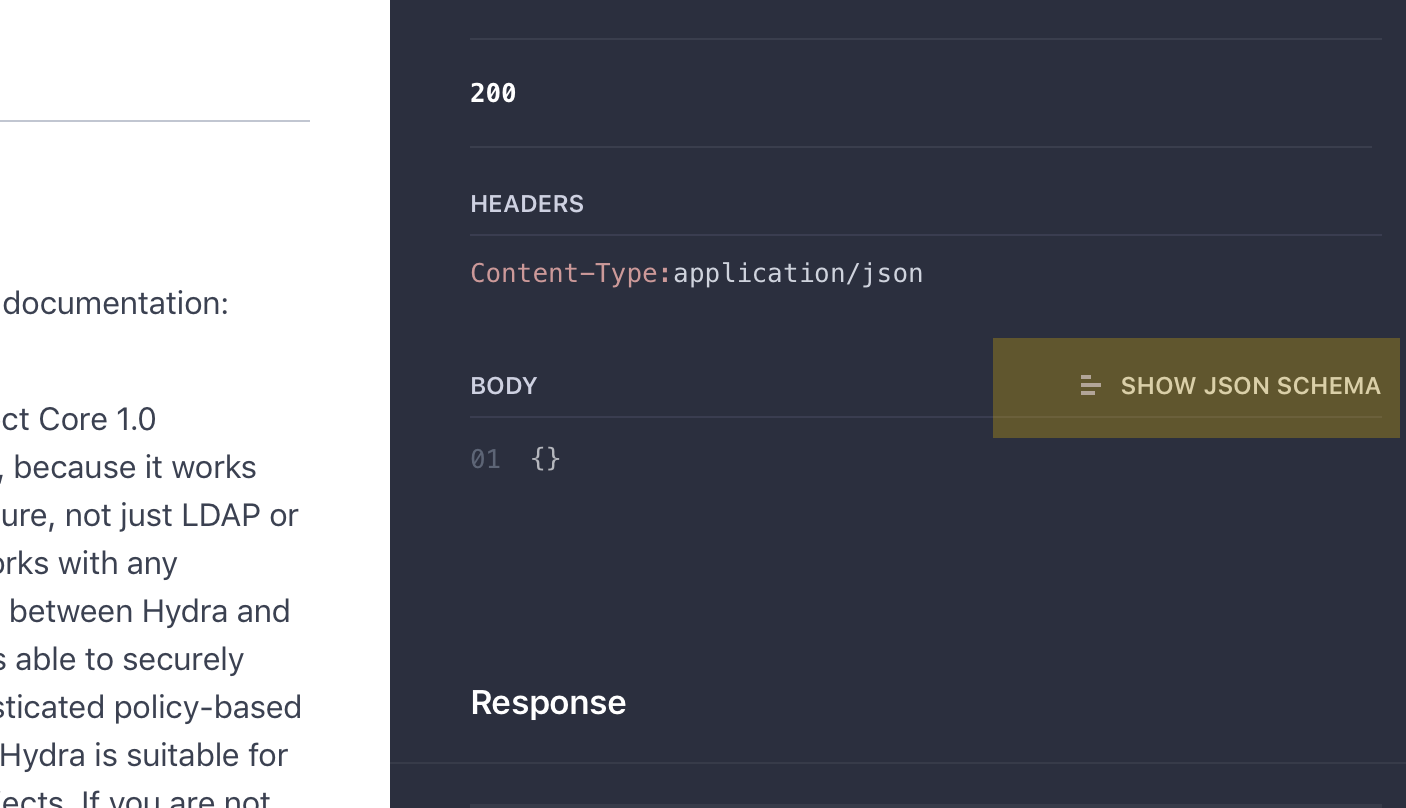 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+import (
+ "net/http"
+)
+
+type APIResponse struct {
+ *http.Response `json:"-"`
+ Message string `json:"message,omitempty"`
+ // Operation is the name of the swagger operation.
+ Operation string `json:"operation,omitempty"`
+ // RequestURL is the request URL. This value is always available, even if the
+ // embedded *http.Response is nil.
+ RequestURL string `json:"url,omitempty"`
+ // Method is the HTTP method used for the request. This value is always
+ // available, even if the embedded *http.Response is nil.
+ Method string `json:"method,omitempty"`
+ // Payload holds the contents of the response body (which may be nil or empty).
+ // This is provided here as the raw response.Body() reader will have already
+ // been drained.
+ Payload []byte `json:"-"`
+}
+
+func NewAPIResponse(r *http.Response) *APIResponse {
+
+ response := &APIResponse{Response: r}
+ return response
+}
+
+func NewAPIResponseWithError(errorMessage string) *APIResponse {
+
+ response := &APIResponse{Message: errorMessage}
+ return response
+}
diff --git a/sdk/go/hydra/swagger/configuration.go b/sdk/go/hydra/swagger/configuration.go
new file mode 100644
index 00000000000..b97fc285ea4
--- /dev/null
+++ b/sdk/go/hydra/swagger/configuration.go
@@ -0,0 +1,66 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server (1.0.0-aplha1)
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### ATTENTION - IMPORTANT NOTE The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 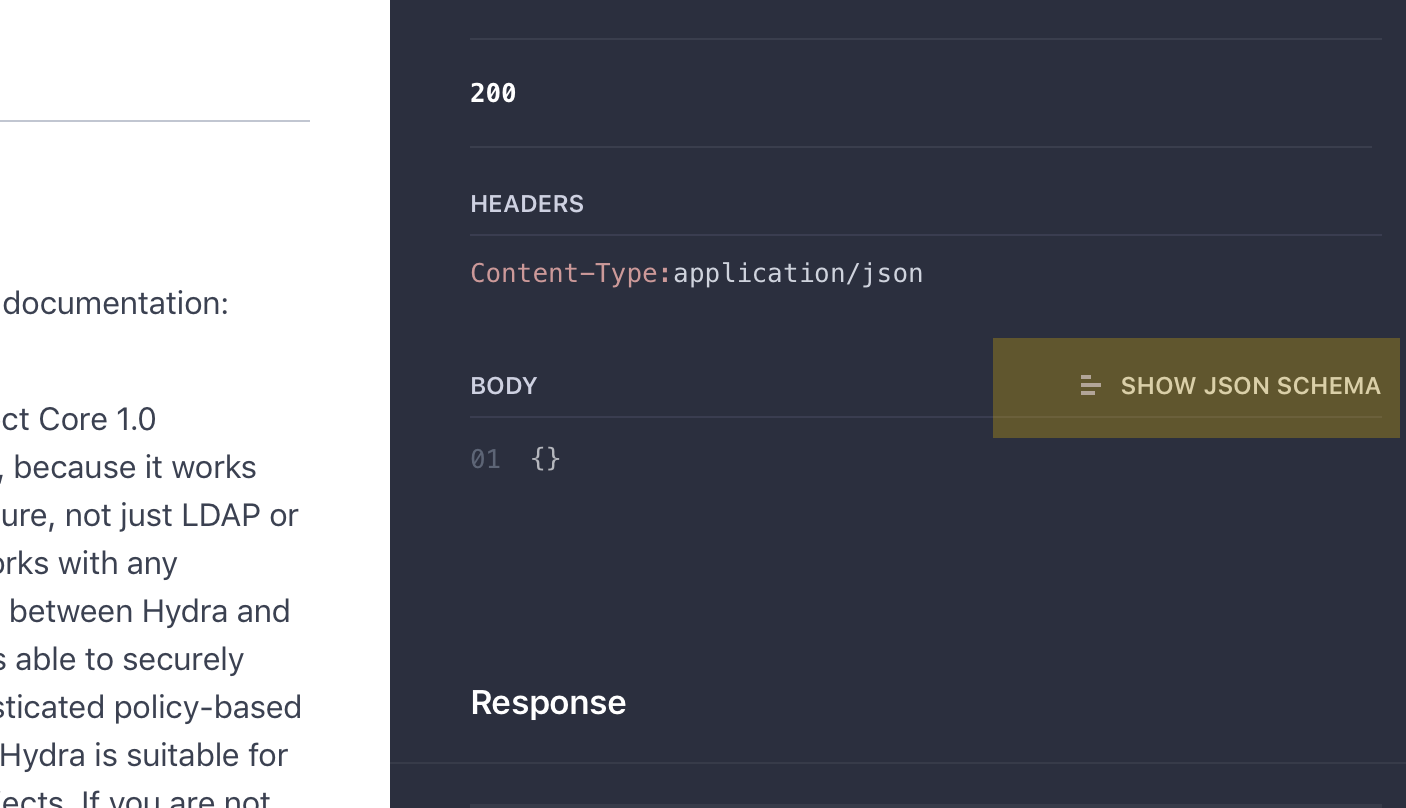
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+import (
+ "encoding/base64"
+ "net/http"
+ "time"
+)
+
+type Configuration struct {
+ Username string `json:"userName,omitempty"`
+ Password string `json:"password,omitempty"`
+ APIKeyPrefix map[string]string `json:"APIKeyPrefix,omitempty"`
+ APIKey map[string]string `json:"APIKey,omitempty"`
+ Debug bool `json:"debug,omitempty"`
+ DebugFile string `json:"debugFile,omitempty"`
+ OAuthToken string `json:"oAuthToken,omitempty"`
+ BasePath string `json:"basePath,omitempty"`
+ Host string `json:"host,omitempty"`
+ Scheme string `json:"scheme,omitempty"`
+ AccessToken string `json:"accessToken,omitempty"`
+ DefaultHeader map[string]string `json:"defaultHeader,omitempty"`
+ UserAgent string `json:"userAgent,omitempty"`
+ APIClient *APIClient
+ Transport http.RoundTripper
+ Timeout *time.Duration `json:"timeout,omitempty"`
+}
+
+func NewConfiguration() *Configuration {
+ cfg := &Configuration{
+ BasePath: "http://localhost",
+ DefaultHeader: make(map[string]string),
+ APIKey: make(map[string]string),
+ APIKeyPrefix: make(map[string]string),
+ UserAgent: "Swagger-Codegen/1.0.0/go",
+ APIClient: &APIClient{},
+ }
+
+ cfg.APIClient.config = cfg
+ return cfg
+}
+
+func (c *Configuration) GetBasicAuthEncodedString() string {
+ return base64.StdEncoding.EncodeToString([]byte(c.Username + ":" + c.Password))
+}
+
+func (c *Configuration) AddDefaultHeader(key string, value string) {
+ c.DefaultHeader[key] = value
+}
+
+func (c *Configuration) GetAPIKeyWithPrefix(APIKeyIdentifier string) string {
+ if c.APIKeyPrefix[APIKeyIdentifier] != "" {
+ return c.APIKeyPrefix[APIKeyIdentifier] + " " + c.APIKey[APIKeyIdentifier]
+ }
+
+ return c.APIKey[APIKeyIdentifier]
+}
diff --git a/sdk/go/hydra/swagger/consent_request_acceptance.go b/sdk/go/hydra/swagger/consent_request_acceptance.go
new file mode 100644
index 00000000000..0fab37e6688
--- /dev/null
+++ b/sdk/go/hydra/swagger/consent_request_acceptance.go
@@ -0,0 +1,26 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 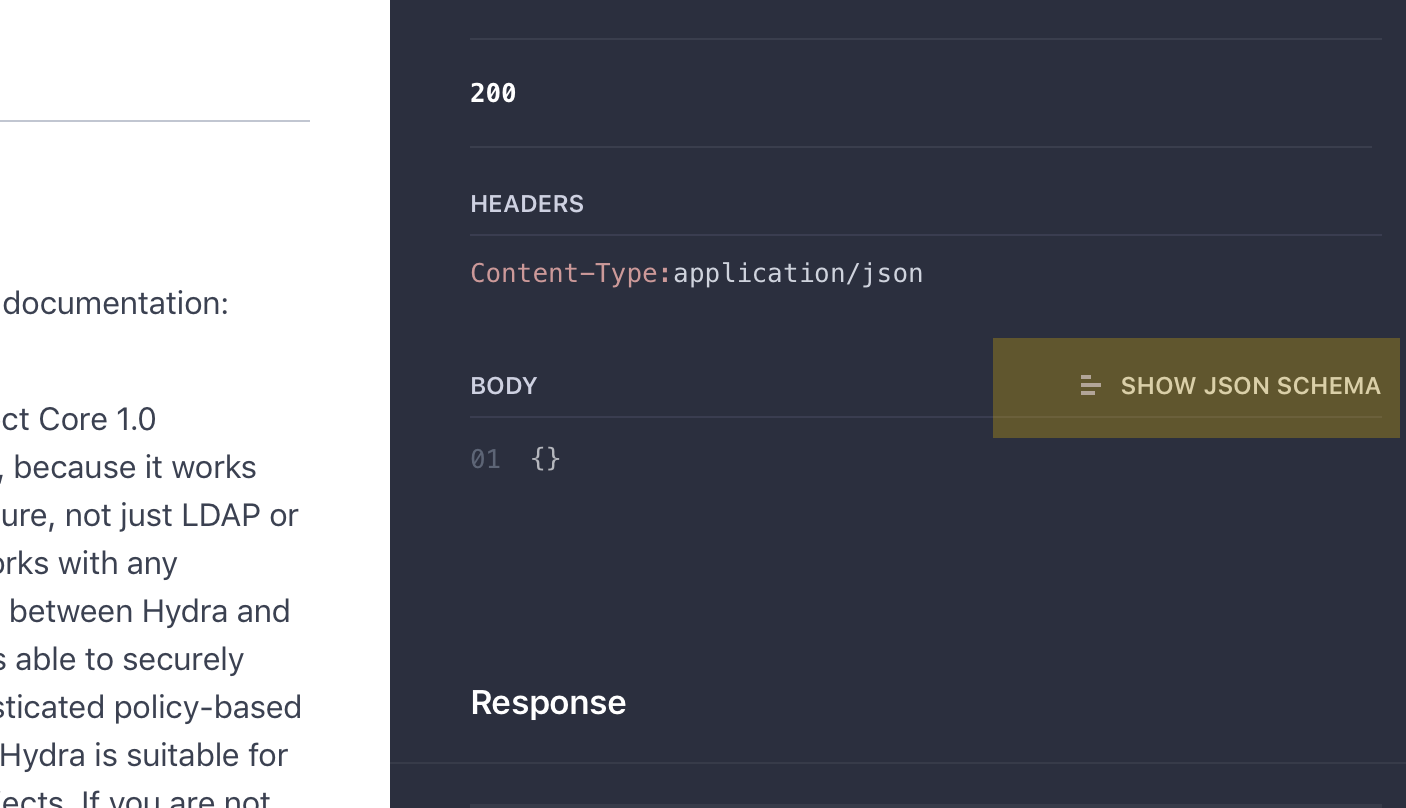 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type ConsentRequestAcceptance struct {
+
+ // AccessTokenExtra represents arbitrary data that will be added to the access token and that will be returned on introspection and warden requests.
+ AccessTokenExtra map[string]interface{} `json:"accessTokenExtra,omitempty"`
+
+ // A list of scopes that the user agreed to grant. It should be a subset of requestedScopes from the consent request.
+ GrantScopes []string `json:"grantScopes,omitempty"`
+
+ // IDTokenExtra represents arbitrary data that will be added to the ID token. The ID token will only be issued if the user agrees to it and if the client requested an ID token.
+ IdTokenExtra map[string]interface{} `json:"idTokenExtra,omitempty"`
+
+ // Subject represents a unique identifier of the user (or service, or legal entity, ...) that accepted the OAuth2 request.
+ Subject string `json:"subject,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/consent_request_manager.go b/sdk/go/hydra/swagger/consent_request_manager.go
new file mode 100644
index 00000000000..a731ed58d00
--- /dev/null
+++ b/sdk/go/hydra/swagger/consent_request_manager.go
@@ -0,0 +1,14 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 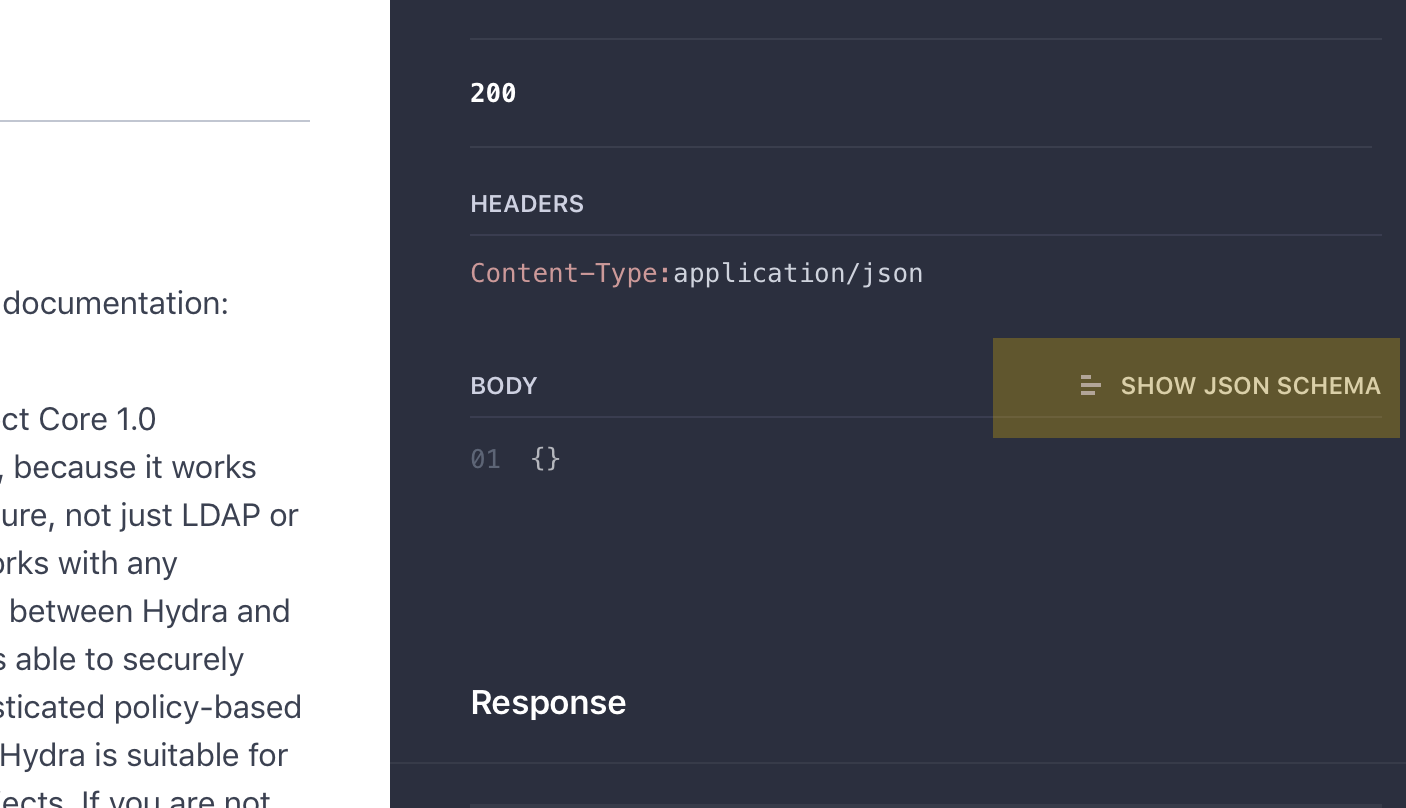 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type ConsentRequestManager struct {
+}
diff --git a/sdk/go/hydra/swagger/consent_request_rejection.go b/sdk/go/hydra/swagger/consent_request_rejection.go
new file mode 100644
index 00000000000..bb464f66b9d
--- /dev/null
+++ b/sdk/go/hydra/swagger/consent_request_rejection.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 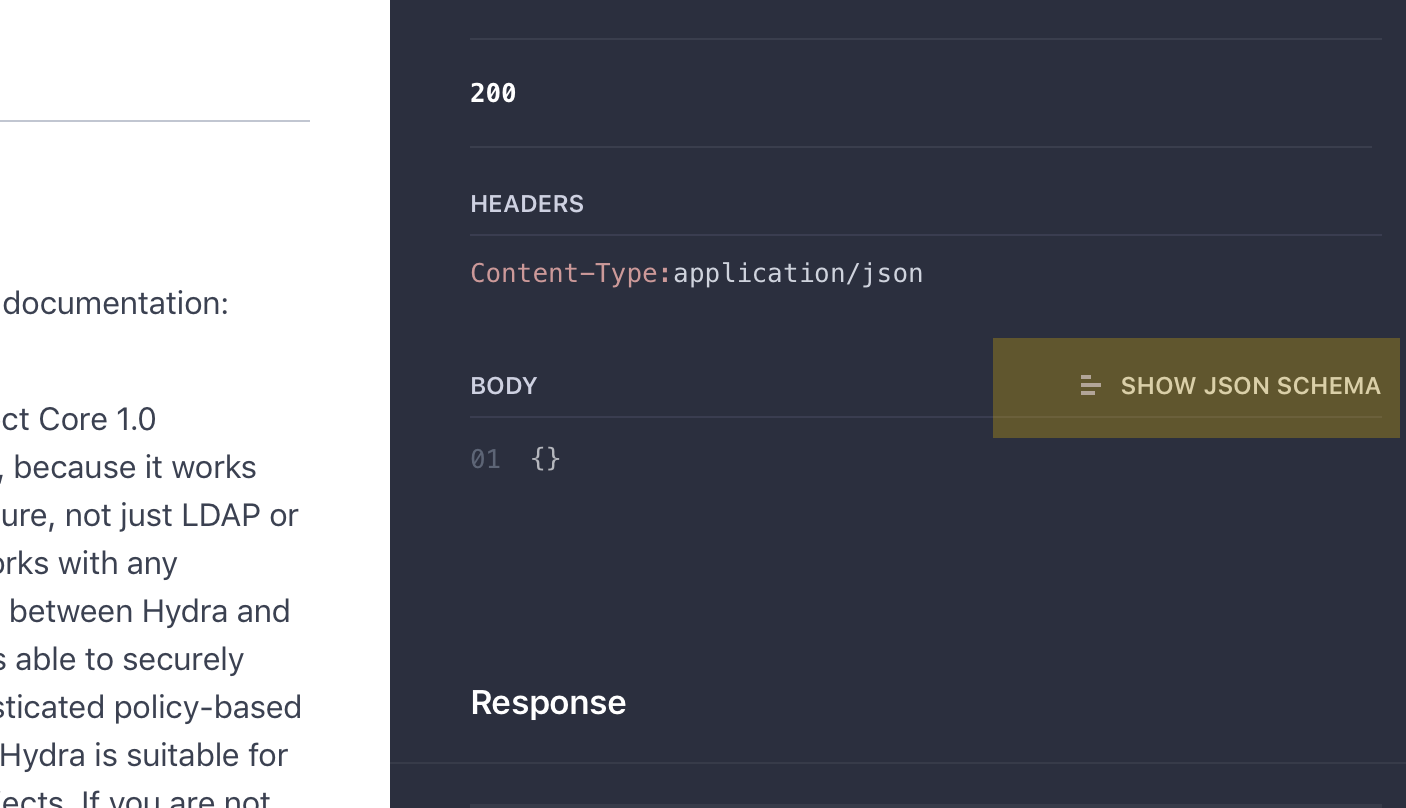 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type ConsentRequestRejection struct {
+
+ // Reason represents the reason why the user rejected the consent request.
+ Reason string `json:"reason,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/context.go b/sdk/go/hydra/swagger/context.go
new file mode 100644
index 00000000000..8d8a31b8368
--- /dev/null
+++ b/sdk/go/hydra/swagger/context.go
@@ -0,0 +1,30 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 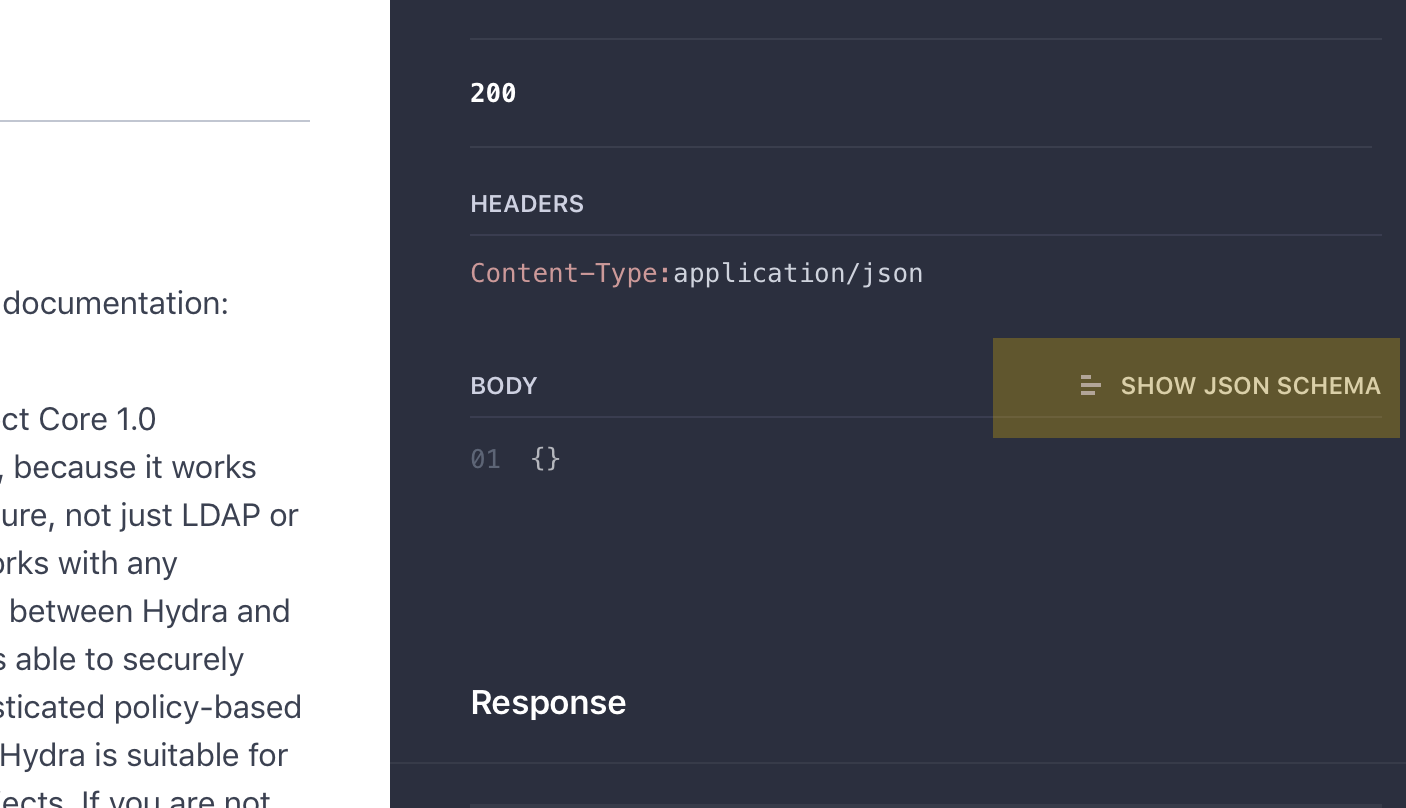 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// Context contains an access token's session data
+type Context struct {
+
+ // Audience is who the token was issued for. This is an OAuth2 app usually.
+ Aud string `json:"aud,omitempty"`
+
+ // Extra represents arbitrary session data.
+ Ext map[string]interface{} `json:"ext,omitempty"`
+
+ // Issuer is the id of the issuer, typically an hydra instance.
+ Iss string `json:"iss,omitempty"`
+
+ // GrantedScopes is a list of scopes that the subject authorized when asked for consent.
+ Scopes []string `json:"scopes,omitempty"`
+
+ // Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app. This is usually a uuid but you can choose a urn or some other id too.
+ Sub string `json:"sub,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/docs/ConsentRequestAcceptance.md b/sdk/go/hydra/swagger/docs/ConsentRequestAcceptance.md
new file mode 100644
index 00000000000..7226d7802cc
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/ConsentRequestAcceptance.md
@@ -0,0 +1,13 @@
+# ConsentRequestAcceptance
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**AccessTokenExtra** | [**map[string]interface{}**](interface{}.md) | AccessTokenExtra represents arbitrary data that will be added to the access token and that will be returned on introspection and warden requests. | [optional] [default to null]
+**GrantScopes** | **[]string** | A list of scopes that the user agreed to grant. It should be a subset of requestedScopes from the consent request. | [optional] [default to null]
+**IdTokenExtra** | [**map[string]interface{}**](interface{}.md) | IDTokenExtra represents arbitrary data that will be added to the ID token. The ID token will only be issued if the user agrees to it and if the client requested an ID token. | [optional] [default to null]
+**Subject** | **string** | Subject represents a unique identifier of the user (or service, or legal entity, ...) that accepted the OAuth2 request. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/ConsentRequestManager.md b/sdk/go/hydra/swagger/docs/ConsentRequestManager.md
new file mode 100644
index 00000000000..26f451f4d3a
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/ConsentRequestManager.md
@@ -0,0 +1,9 @@
+# ConsentRequestManager
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/ConsentRequestRejection.md b/sdk/go/hydra/swagger/docs/ConsentRequestRejection.md
new file mode 100644
index 00000000000..4b245fe779a
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/ConsentRequestRejection.md
@@ -0,0 +1,10 @@
+# ConsentRequestRejection
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Reason** | **string** | Reason represents the reason why the user rejected the consent request. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/Context.md b/sdk/go/hydra/swagger/docs/Context.md
new file mode 100644
index 00000000000..4328f833c92
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/Context.md
@@ -0,0 +1,14 @@
+# Context
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Aud** | **string** | Audience is who the token was issued for. This is an OAuth2 app usually. | [optional] [default to null]
+**Ext** | [**map[string]interface{}**](interface{}.md) | Extra represents arbitrary session data. | [optional] [default to null]
+**Iss** | **string** | Issuer is the id of the issuer, typically an hydra instance. | [optional] [default to null]
+**Scopes** | **[]string** | GrantedScopes is a list of scopes that the subject authorized when asked for consent. | [optional] [default to null]
+**Sub** | **string** | Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app. This is usually a uuid but you can choose a urn or some other id too. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/Firewall.md b/sdk/go/hydra/swagger/docs/Firewall.md
new file mode 100644
index 00000000000..134131c8cd3
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/Firewall.md
@@ -0,0 +1,9 @@
+# Firewall
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/Group.md b/sdk/go/hydra/swagger/docs/Group.md
new file mode 100644
index 00000000000..faeb0b36045
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/Group.md
@@ -0,0 +1,11 @@
+# Group
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Id** | **string** | ID is the groups id. | [optional] [default to null]
+**Members** | **[]string** | Members is who belongs to the group. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/GroupMembers.md b/sdk/go/hydra/swagger/docs/GroupMembers.md
new file mode 100644
index 00000000000..cc2e6f8a1f8
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/GroupMembers.md
@@ -0,0 +1,10 @@
+# GroupMembers
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Members** | **[]string** | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/Handler.md b/sdk/go/hydra/swagger/docs/Handler.md
new file mode 100644
index 00000000000..77f018750e1
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/Handler.md
@@ -0,0 +1,13 @@
+# Handler
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Generators** | [**map[string]KeyGenerator**](KeyGenerator.md) | | [optional] [default to null]
+**H** | [**Writer**](Writer.md) | | [optional] [default to null]
+**Manager** | [**Manager**](Manager.md) | | [optional] [default to null]
+**W** | [**Firewall**](Firewall.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/HealthApi.md b/sdk/go/hydra/swagger/docs/HealthApi.md
new file mode 100644
index 00000000000..a0575f39ec5
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/HealthApi.md
@@ -0,0 +1,62 @@
+# \HealthApi
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**GetInstanceMetrics**](HealthApi.md#GetInstanceMetrics) | **Get** /health/metrics | Show instance metrics (experimental)
+[**GetInstanceStatus**](HealthApi.md#GetInstanceStatus) | **Get** /health/status | Check health status of this instance
+
+
+# **GetInstanceMetrics**
+> GetInstanceMetrics()
+
+Show instance metrics (experimental)
+
+This endpoint returns an instance's metrics, such as average response time, status code distribution, hits per second and so on. The return values are currently not documented as this endpoint is still experimental. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:health:stats\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **GetInstanceStatus**
+> InlineResponse200 GetInstanceStatus()
+
+Check health status of this instance
+
+This endpoint returns `{ \"status\": \"ok\" }`. This status let's you know that the HTTP server is up and running. This status does currently not include checks whether the database connection is up and running. This endpoint does not require the `X-Forwarded-Proto` header when TLS termination is set. Be aware that if you are running multiple nodes of ORY Hydra, the health status will never refer to the cluster state, only to a single instance.
+
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**InlineResponse200**](inline_response_200.md)
+
+### Authorization
+
+No authorization required
+
+### HTTP request headers
+
+ - **Content-Type**: application/json, application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
diff --git a/sdk/go/hydra/swagger/docs/InlineResponse200.md b/sdk/go/hydra/swagger/docs/InlineResponse200.md
new file mode 100644
index 00000000000..848a64896a5
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/InlineResponse200.md
@@ -0,0 +1,10 @@
+# InlineResponse200
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Status** | **string** | Status always contains \"ok\" | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/InlineResponse2001.md b/sdk/go/hydra/swagger/docs/InlineResponse2001.md
new file mode 100644
index 00000000000..448259aa86a
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/InlineResponse2001.md
@@ -0,0 +1,15 @@
+# InlineResponse2001
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**AccessToken** | **string** | The access token issued by the authorization server. | [optional] [default to null]
+**ExpiresIn** | **int64** | The lifetime in seconds of the access token. For example, the value \"3600\" denotes that the access token will expire in one hour from the time the response was generated. | [optional] [default to null]
+**IdToken** | **int64** | To retrieve a refresh token request the id_token scope. | [optional] [default to null]
+**RefreshToken** | **string** | The refresh token, which can be used to obtain new access tokens. To retrieve it add the scope \"offline\" to your access token request. | [optional] [default to null]
+**Scope** | **int64** | The scope of the access token | [optional] [default to null]
+**TokenType** | **string** | The type of the token issued | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/InlineResponse401.md b/sdk/go/hydra/swagger/docs/InlineResponse401.md
new file mode 100644
index 00000000000..0ac1e5d992b
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/InlineResponse401.md
@@ -0,0 +1,15 @@
+# InlineResponse401
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Code** | **int64** | | [optional] [default to null]
+**Details** | [**[]map[string]interface{}**](map.md) | | [optional] [default to null]
+**Message** | **string** | | [optional] [default to null]
+**Reason** | **string** | | [optional] [default to null]
+**Request** | **string** | | [optional] [default to null]
+**Status** | **string** | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/JoseWebKeySetRequest.md b/sdk/go/hydra/swagger/docs/JoseWebKeySetRequest.md
new file mode 100644
index 00000000000..83846b23d85
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/JoseWebKeySetRequest.md
@@ -0,0 +1,10 @@
+# JoseWebKeySetRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Keys** | [**[]RawMessage**](RawMessage.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/JsonWebKey.md b/sdk/go/hydra/swagger/docs/JsonWebKey.md
new file mode 100644
index 00000000000..1b1e042482c
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/JsonWebKey.md
@@ -0,0 +1,26 @@
+# JsonWebKey
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Alg** | **string** | The \"alg\" (algorithm) parameter identifies the algorithm intended for use with the key. The values used should either be registered in the IANA \"JSON Web Signature and Encryption Algorithms\" registry established by [JWA] or be a value that contains a Collision- Resistant Name. | [optional] [default to null]
+**Crv** | **string** | | [optional] [default to null]
+**D** | **string** | | [optional] [default to null]
+**Dp** | **string** | | [optional] [default to null]
+**Dq** | **string** | | [optional] [default to null]
+**E** | **string** | | [optional] [default to null]
+**K** | **string** | | [optional] [default to null]
+**Kid** | **string** | The \"kid\" (key ID) parameter is used to match a specific key. This is used, for instance, to choose among a set of keys within a JWK Set during key rollover. The structure of the \"kid\" value is unspecified. When \"kid\" values are used within a JWK Set, different keys within the JWK Set SHOULD use distinct \"kid\" values. (One example in which different keys might use the same \"kid\" value is if they have different \"kty\" (key type) values but are considered to be equivalent alternatives by the application using them.) The \"kid\" value is a case-sensitive string. | [optional] [default to null]
+**Kty** | **string** | The \"kty\" (key type) parameter identifies the cryptographic algorithm family used with the key, such as \"RSA\" or \"EC\". \"kty\" values should either be registered in the IANA \"JSON Web Key Types\" registry established by [JWA] or be a value that contains a Collision- Resistant Name. The \"kty\" value is a case-sensitive string. | [optional] [default to null]
+**N** | **string** | | [optional] [default to null]
+**P** | **string** | | [optional] [default to null]
+**Q** | **string** | | [optional] [default to null]
+**Qi** | **string** | | [optional] [default to null]
+**Use** | **string** | The \"use\" (public key use) parameter identifies the intended use of the public key. The \"use\" parameter is employed to indicate whether a public key is used for encrypting data or verifying the signature on data. Values are commonly \"sig\" (signature) or \"enc\" (encryption). | [optional] [default to null]
+**X** | **string** | | [optional] [default to null]
+**X5c** | **[]string** | The \"x5c\" (X.509 certificate chain) parameter contains a chain of one or more PKIX certificates [RFC5280]. The certificate chain is represented as a JSON array of certificate value strings. Each string in the array is a base64-encoded (Section 4 of [RFC4648] -- not base64url-encoded) DER [ITU.X690.1994] PKIX certificate value. The PKIX certificate containing the key value MUST be the first certificate. | [optional] [default to null]
+**Y** | **string** | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/JsonWebKeyApi.md b/sdk/go/hydra/swagger/docs/JsonWebKeyApi.md
new file mode 100644
index 00000000000..0c5331b344d
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/JsonWebKeyApi.md
@@ -0,0 +1,224 @@
+# \JsonWebKeyApi
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**CreateJsonWebKeySet**](JsonWebKeyApi.md#CreateJsonWebKeySet) | **Post** /keys/{set} | Generate a new JSON Web Key
+[**DeleteJsonWebKey**](JsonWebKeyApi.md#DeleteJsonWebKey) | **Delete** /keys/{set}/{kid} | Delete a JSON Web Key
+[**DeleteJsonWebKeySet**](JsonWebKeyApi.md#DeleteJsonWebKeySet) | **Delete** /keys/{set} | Delete a JSON Web Key
+[**GetJsonWebKey**](JsonWebKeyApi.md#GetJsonWebKey) | **Get** /keys/{set}/{kid} | Retrieve a JSON Web Key
+[**GetJsonWebKeySet**](JsonWebKeyApi.md#GetJsonWebKeySet) | **Get** /keys/{set} | Retrieve a JSON Web Key Set
+[**UpdateJsonWebKey**](JsonWebKeyApi.md#UpdateJsonWebKey) | **Put** /keys/{set}/{kid} | Update a JSON Web Key
+[**UpdateJsonWebKeySet**](JsonWebKeyApi.md#UpdateJsonWebKeySet) | **Put** /keys/{set} | Update a JSON Web Key Set
+
+
+# **CreateJsonWebKeySet**
+> JsonWebKeySet CreateJsonWebKeySet($set, $body)
+
+Generate a new JSON Web Key
+
+This endpoint is capable of generating JSON Web Key Sets for you. There a different strategies available, such as symmetric cryptographic keys (HS256) and asymetric cryptographic keys (RS256, ECDSA). If the specified JSON Web Key Set does not exist, it will be created. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys::\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **set** | **string**| The set |
+ **body** | [**JsonWebKeySetGeneratorRequest**](JsonWebKeySetGeneratorRequest.md)| | [optional]
+
+### Return type
+
+[**JsonWebKeySet**](jsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **DeleteJsonWebKey**
+> DeleteJsonWebKey($kid, $set)
+
+Delete a JSON Web Key
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys::\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **kid** | **string**| The kid of the desired key |
+ **set** | **string**| The set |
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **DeleteJsonWebKeySet**
+> DeleteJsonWebKeySet($set)
+
+Delete a JSON Web Key
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **set** | **string**| The set |
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **GetJsonWebKey**
+> JsonWebKeySet GetJsonWebKey($kid, $set)
+
+Retrieve a JSON Web Key
+
+This endpoint can be used to retrieve JWKs stored in ORY Hydra. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys::\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **kid** | **string**| The kid of the desired key |
+ **set** | **string**| The set |
+
+### Return type
+
+[**JsonWebKeySet**](jsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **GetJsonWebKeySet**
+> JsonWebKeySet GetJsonWebKeySet($set)
+
+Retrieve a JSON Web Key Set
+
+This endpoint can be used to retrieve JWK Sets stored in ORY Hydra. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys::\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **set** | **string**| The set |
+
+### Return type
+
+[**JsonWebKeySet**](jsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **UpdateJsonWebKey**
+> JsonWebKey UpdateJsonWebKey($kid, $set, $body)
+
+Update a JSON Web Key
+
+Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys::\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **kid** | **string**| The kid of the desired key |
+ **set** | **string**| The set |
+ **body** | [**JsonWebKey**](JsonWebKey.md)| | [optional]
+
+### Return type
+
+[**JsonWebKey**](jsonWebKey.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **UpdateJsonWebKeySet**
+> JsonWebKeySet UpdateJsonWebKeySet($set, $body)
+
+Update a JSON Web Key Set
+
+Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **set** | **string**| The set |
+ **body** | [**JsonWebKeySet**](JsonWebKeySet.md)| | [optional]
+
+### Return type
+
+[**JsonWebKeySet**](jsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
diff --git a/sdk/go/hydra/swagger/docs/JsonWebKeySet.md b/sdk/go/hydra/swagger/docs/JsonWebKeySet.md
new file mode 100644
index 00000000000..b3ceaba267c
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/JsonWebKeySet.md
@@ -0,0 +1,10 @@
+# JsonWebKeySet
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Keys** | [**[]JsonWebKey**](jsonWebKey.md) | The value of the \"keys\" parameter is an array of JWK values. By default, the order of the JWK values within the array does not imply an order of preference among them, although applications of JWK Sets can choose to assign a meaning to the order for their purposes, if desired. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/JsonWebKeySetGeneratorRequest.md b/sdk/go/hydra/swagger/docs/JsonWebKeySetGeneratorRequest.md
new file mode 100644
index 00000000000..65ef3f75ae6
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/JsonWebKeySetGeneratorRequest.md
@@ -0,0 +1,11 @@
+# JsonWebKeySetGeneratorRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Alg** | **string** | The algorithm to be used for creating the key. Supports \"RS256\", \"ES521\" and \"HS256\" | [default to null]
+**Kid** | **string** | The kid of the key to be created | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/KeyGenerator.md b/sdk/go/hydra/swagger/docs/KeyGenerator.md
new file mode 100644
index 00000000000..d2d7e1c15e9
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/KeyGenerator.md
@@ -0,0 +1,9 @@
+# KeyGenerator
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/Manager.md b/sdk/go/hydra/swagger/docs/Manager.md
new file mode 100644
index 00000000000..485b366835d
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/Manager.md
@@ -0,0 +1,9 @@
+# Manager
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/OAuth2Api.md b/sdk/go/hydra/swagger/docs/OAuth2Api.md
new file mode 100644
index 00000000000..a165e338d10
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/OAuth2Api.md
@@ -0,0 +1,417 @@
+# \OAuth2Api
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**AcceptOAuth2ConsentRequest**](OAuth2Api.md#AcceptOAuth2ConsentRequest) | **Patch** /oauth2/consent/requests/{id}/accept | Accept a consent request
+[**CreateOAuth2Client**](OAuth2Api.md#CreateOAuth2Client) | **Post** /clients | Create an OAuth 2.0 client
+[**DeleteOAuth2Client**](OAuth2Api.md#DeleteOAuth2Client) | **Delete** /clients/{id} | Deletes an OAuth 2.0 Client
+[**GetOAuth2Client**](OAuth2Api.md#GetOAuth2Client) | **Get** /clients/{id} | Retrieve an OAuth 2.0 Client.
+[**GetOAuth2ConsentRequest**](OAuth2Api.md#GetOAuth2ConsentRequest) | **Get** /oauth2/consent/requests/{id} | Receive consent request information
+[**GetWellKnown**](OAuth2Api.md#GetWellKnown) | **Get** /.well-known/openid-configuration | Server well known configuration
+[**IntrospectOAuth2Token**](OAuth2Api.md#IntrospectOAuth2Token) | **Post** /oauth2/introspect | Introspect OAuth2 tokens
+[**ListOAuth2Clients**](OAuth2Api.md#ListOAuth2Clients) | **Get** /clients | List OAuth 2.0 Clients
+[**OauthAuth**](OAuth2Api.md#OauthAuth) | **Get** /oauth2/auth | The OAuth 2.0 authorize endpoint
+[**OauthToken**](OAuth2Api.md#OauthToken) | **Post** /oauth2/token | The OAuth 2.0 token endpoint
+[**RejectOAuth2ConsentRequest**](OAuth2Api.md#RejectOAuth2ConsentRequest) | **Patch** /oauth2/consent/requests/{id}/reject | Reject a consent request
+[**RevokeOAuth2Token**](OAuth2Api.md#RevokeOAuth2Token) | **Post** /oauth2/revoke | Revoke OAuth2 tokens
+[**UpdateOAuth2Client**](OAuth2Api.md#UpdateOAuth2Client) | **Put** /clients/{id} | Update an OAuth 2.0 Client
+[**WellKnown**](OAuth2Api.md#WellKnown) | **Get** /.well-known/jwks.json | Get list of well known JSON Web Keys
+
+
+# **AcceptOAuth2ConsentRequest**
+> AcceptOAuth2ConsentRequest($id, $body)
+
+Accept a consent request
+
+Call this endpoint to accept a consent request. This usually happens when a user agrees to give access rights to an application. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:\"], \"actions\": [\"accept\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| |
+ **body** | [**ConsentRequestAcceptance**](ConsentRequestAcceptance.md)| |
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **CreateOAuth2Client**
+> OAuth2Client CreateOAuth2Client($body)
+
+Create an OAuth 2.0 client
+
+If you pass `client_secret` the secret will be used, otherwise a random secret will be generated. The secret will be returned in the response and you will not be able to retrieve it later on. Write the secret down and keep it somwhere safe. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"create\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**OAuth2Client**](OAuth2Client.md)| |
+
+### Return type
+
+[**OAuth2Client**](oAuth2Client.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **DeleteOAuth2Client**
+> DeleteOAuth2Client($id)
+
+Deletes an OAuth 2.0 Client
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients:\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients:\"], \"actions\": [\"delete\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the OAuth 2.0 Client. |
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **GetOAuth2Client**
+> OAuth2Client GetOAuth2Client($id)
+
+Retrieve an OAuth 2.0 Client.
+
+This endpoint never returns passwords. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients:\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients:\"], \"actions\": [\"get\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the OAuth 2.0 Client. |
+
+### Return type
+
+[**OAuth2Client**](oAuth2Client.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **GetOAuth2ConsentRequest**
+> OAuth2consentRequest GetOAuth2ConsentRequest($id)
+
+Receive consent request information
+
+Call this endpoint to receive information on consent requests. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the OAuth 2.0 Consent Request. |
+
+### Return type
+
+[**OAuth2consentRequest**](oAuth2consentRequest.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **GetWellKnown**
+> WellKnown GetWellKnown()
+
+Server well known configuration
+
+The well known endpoint an be used to retrieve information for OpenID Connect clients. We encourage you to not roll your own OpenID Connect client but to use an OpenID Connect client library instead. You can learn more on this flow at https://openid.net/specs/openid-connect-discovery-1_0.html
+
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**WellKnown**](wellKnown.md)
+
+### Authorization
+
+No authorization required
+
+### HTTP request headers
+
+ - **Content-Type**: application/json, application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **IntrospectOAuth2Token**
+> OAuth2TokenIntrospection IntrospectOAuth2Token($token, $scope)
+
+Introspect OAuth2 tokens
+
+The introspection endpoint allows to check if a token (both refresh and access) is active or not. An active token is neither expired nor revoked. If a token is active, additional information on the token will be included. You can set additional data for a token by setting `accessTokenExtra` during the consent flow.
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **token** | **string**| The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation. |
+ **scope** | **string**| An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false. | [optional]
+
+### Return type
+
+[**OAuth2TokenIntrospection**](oAuth2TokenIntrospection.md)
+
+### Authorization
+
+[basic](../README.md#basic), [oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **ListOAuth2Clients**
+> []OAuth2Client ListOAuth2Clients()
+
+List OAuth 2.0 Clients
+
+This endpoint never returns passwords. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**[]OAuth2Client**](oAuth2Client.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **OauthAuth**
+> OauthAuth()
+
+The OAuth 2.0 authorize endpoint
+
+This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows. OAuth2 is a very popular protocol and a library for your programming language will exists. To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
+
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+No authorization required
+
+### HTTP request headers
+
+ - **Content-Type**: application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **OauthToken**
+> InlineResponse2001 OauthToken()
+
+The OAuth 2.0 token endpoint
+
+This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows. OAuth2 is a very popular protocol and a library for your programming language will exists. To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
+
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**InlineResponse2001**](inline_response_200_1.md)
+
+### Authorization
+
+[basic](../README.md#basic), [oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **RejectOAuth2ConsentRequest**
+> RejectOAuth2ConsentRequest($id, $body)
+
+Reject a consent request
+
+Call this endpoint to reject a consent request. This usually happens when a user denies access rights to an application. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:\"], \"actions\": [\"reject\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| |
+ **body** | [**ConsentRequestRejection**](ConsentRequestRejection.md)| |
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **RevokeOAuth2Token**
+> RevokeOAuth2Token($token)
+
+Revoke OAuth2 tokens
+
+Revoking a token (both access and refresh) means that the tokens will be invalid. A revoked access token can no longer be used to make access requests, and a revoked refresh token can no longer be used to refresh an access token. Revoking a refresh token also invalidates the access token that was created with it.
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **token** | **string**| |
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[basic](../README.md#basic)
+
+### HTTP request headers
+
+ - **Content-Type**: application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **UpdateOAuth2Client**
+> OAuth2Client UpdateOAuth2Client($id, $body)
+
+Update an OAuth 2.0 Client
+
+If you pass `client_secret` the secret will be updated and returned via the API. This is the only time you will be able to retrieve the client secret, so write it down and keep it safe. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"update\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| |
+ **body** | [**OAuth2Client**](OAuth2Client.md)| |
+
+### Return type
+
+[**OAuth2Client**](oAuth2Client.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **WellKnown**
+> JsonWebKeySet WellKnown()
+
+Get list of well known JSON Web Keys
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:hydra.openid.id-token:public\"], \"actions\": [\"GET\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**JsonWebKeySet**](jsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
diff --git a/sdk/go/hydra/swagger/docs/OAuth2Client.md b/sdk/go/hydra/swagger/docs/OAuth2Client.md
new file mode 100644
index 00000000000..40cf4082f48
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/OAuth2Client.md
@@ -0,0 +1,23 @@
+# OAuth2Client
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**ClientName** | **string** | Name is the human-readable string name of the client to be presented to the end-user during authorization. | [optional] [default to null]
+**ClientSecret** | **string** | Secret is the client's secret. The secret will be included in the create request as cleartext, and then never again. The secret is stored using BCrypt so it is impossible to recover it. Tell your users that they need to write the secret down as it will not be made available again. | [optional] [default to null]
+**ClientUri** | **string** | ClientURI is an URL string of a web page providing information about the client. If present, the server SHOULD display this URL to the end-user in a clickable fashion. | [optional] [default to null]
+**Contacts** | **[]string** | Contacts is a array of strings representing ways to contact people responsible for this client, typically email addresses. | [optional] [default to null]
+**GrantTypes** | **[]string** | GrantTypes is an array of grant types the client is allowed to use. | [optional] [default to null]
+**Id** | **string** | ID is the id for this client. | [optional] [default to null]
+**LogoUri** | **string** | LogoURI is an URL string that references a logo for the client. | [optional] [default to null]
+**Owner** | **string** | Owner is a string identifying the owner of the OAuth 2.0 Client. | [optional] [default to null]
+**PolicyUri** | **string** | PolicyURI is a URL string that points to a human-readable privacy policy document that describes how the deployment organization collects, uses, retains, and discloses personal data. | [optional] [default to null]
+**Public** | **bool** | Public is a boolean that identifies this client as public, meaning that it does not have a secret. It will disable the client_credentials grant type for this client if set. | [optional] [default to null]
+**RedirectUris** | **[]string** | RedirectURIs is an array of allowed redirect urls for the client, for example: http://mydomain/oauth/callback . | [optional] [default to null]
+**ResponseTypes** | **[]string** | ResponseTypes is an array of the OAuth 2.0 response type strings that the client can use at the authorization endpoint. | [optional] [default to null]
+**Scope** | **string** | Scope is a string containing a space-separated list of scope values (as described in Section 3.3 of OAuth 2.0 [RFC6749]) that the client can use when requesting access tokens. | [optional] [default to null]
+**TosUri** | **string** | TermsOfServiceURI is a URL string that points to a human-readable terms of service document for the client that describes a contractual relationship between the end-user and the client that the end-user accepts when authorizing the client. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/OAuth2TokenIntrospection.md b/sdk/go/hydra/swagger/docs/OAuth2TokenIntrospection.md
new file mode 100644
index 00000000000..e8c90f78f23
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/OAuth2TokenIntrospection.md
@@ -0,0 +1,20 @@
+# OAuth2TokenIntrospection
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Active** | **bool** | Active is a boolean indicator of whether or not the presented token is currently active. The specifics of a token's \"active\" state will vary depending on the implementation of the authorization server and the information it keeps about its tokens, but a \"true\" value return for the \"active\" property will generally indicate that a given token has been issued by this authorization server, has not been revoked by the resource owner, and is within its given time window of validity (e.g., after its issuance time and before its expiration time). | [optional] [default to null]
+**Aud** | **string** | Audience is a service-specific string identifier or list of string identifiers representing the intended audience for this token. | [optional] [default to null]
+**ClientId** | **string** | ClientID is aclient identifier for the OAuth 2.0 client that requested this token. | [optional] [default to null]
+**Exp** | **int64** | Expires at is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token will expire. | [optional] [default to null]
+**Ext** | [**map[string]interface{}**](interface{}.md) | Extra is arbitrary data set by the session. | [optional] [default to null]
+**Iat** | **int64** | Issued at is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token was originally issued. | [optional] [default to null]
+**Iss** | **string** | Issuer is a string representing the issuer of this token | [optional] [default to null]
+**Nbf** | **int64** | NotBefore is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token is not to be used before. | [optional] [default to null]
+**Scope** | **string** | Scope is a JSON string containing a space-separated list of scopes associated with this token. | [optional] [default to null]
+**Sub** | **string** | Subject of the token, as defined in JWT [RFC7519]. Usually a machine-readable identifier of the resource owner who authorized this token. | [optional] [default to null]
+**Username** | **string** | Username is a human-readable identifier for the resource owner who authorized this token. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/OAuth2consentRequest.md b/sdk/go/hydra/swagger/docs/OAuth2consentRequest.md
new file mode 100644
index 00000000000..a123d7919be
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/OAuth2consentRequest.md
@@ -0,0 +1,13 @@
+# OAuth2consentRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Audience** | **string** | Audience is the client id that initiated the OAuth2 request. | [optional] [default to null]
+**Id** | **string** | ID is the id of this consent request. | [optional] [default to null]
+**RedirectUrl** | **string** | Redirect URL is the URL where the user agent should be redirected to after the consent has been accepted or rejected. | [optional] [default to null]
+**RequestedScopes** | **[]string** | RequestedScopes represents a list of scopes that have been requested by the OAuth2 request initiator. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/Policy.md b/sdk/go/hydra/swagger/docs/Policy.md
new file mode 100644
index 00000000000..56930955e10
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/Policy.md
@@ -0,0 +1,16 @@
+# Policy
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Actions** | **[]string** | Actions impacted by the policy. | [optional] [default to null]
+**Conditions** | [**map[string]PolicyConditions**](policy_conditions.md) | Conditions under which the policy is active. | [optional] [default to null]
+**Description** | **string** | Description of the policy. | [optional] [default to null]
+**Effect** | **string** | Effect of the policy | [optional] [default to null]
+**Id** | **string** | ID of the policy. | [optional] [default to null]
+**Resources** | **[]string** | Resources impacted by the policy. | [optional] [default to null]
+**Subjects** | **[]string** | Subjects impacted by the policy. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/PolicyApi.md b/sdk/go/hydra/swagger/docs/PolicyApi.md
new file mode 100644
index 00000000000..721fe7f5015
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/PolicyApi.md
@@ -0,0 +1,160 @@
+# \PolicyApi
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**CreatePolicy**](PolicyApi.md#CreatePolicy) | **Post** /policies | Create an Access Control Policy
+[**DeletePolicy**](PolicyApi.md#DeletePolicy) | **Delete** /policies/{id} | Delete an Access Control Policy
+[**GetPolicy**](PolicyApi.md#GetPolicy) | **Get** /policies/{id} | Get an Access Control Policy
+[**ListPolicies**](PolicyApi.md#ListPolicies) | **Get** /policies | List Access Control Policies
+[**UpdatePolicy**](PolicyApi.md#UpdatePolicy) | **Put** /policies/{id} | Update an Access Control Polic
+
+
+# **CreatePolicy**
+> Policy CreatePolicy($body)
+
+Create an Access Control Policy
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**Policy**](Policy.md)| | [optional]
+
+### Return type
+
+[**Policy**](policy.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **DeletePolicy**
+> DeletePolicy($id)
+
+Delete an Access Control Policy
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies:\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the policy. |
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **GetPolicy**
+> Policy GetPolicy($id)
+
+Get an Access Control Policy
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies:\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the policy. |
+
+### Return type
+
+[**Policy**](policy.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **ListPolicies**
+> []Policy ListPolicies($offset, $limit)
+
+List Access Control Policies
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"list\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **offset** | **int64**| The offset from where to start looking. | [optional]
+ **limit** | **int64**| The maximum amount of policies returned. | [optional]
+
+### Return type
+
+[**[]Policy**](policy.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **UpdatePolicy**
+> Policy UpdatePolicy($id, $body)
+
+Update an Access Control Polic
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the policy. |
+ **body** | [**Policy**](Policy.md)| | [optional]
+
+### Return type
+
+[**Policy**](policy.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
diff --git a/sdk/go/hydra/swagger/docs/PolicyConditions.md b/sdk/go/hydra/swagger/docs/PolicyConditions.md
new file mode 100644
index 00000000000..e38c6e6a1dd
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/PolicyConditions.md
@@ -0,0 +1,11 @@
+# PolicyConditions
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Options** | [**map[string]interface{}**](interface{}.md) | | [optional] [default to null]
+**Type_** | **string** | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/RawMessage.md b/sdk/go/hydra/swagger/docs/RawMessage.md
new file mode 100644
index 00000000000..f8710fd2edc
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/RawMessage.md
@@ -0,0 +1,9 @@
+# RawMessage
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerAcceptConsentRequest.md b/sdk/go/hydra/swagger/docs/SwaggerAcceptConsentRequest.md
new file mode 100644
index 00000000000..623403ed998
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerAcceptConsentRequest.md
@@ -0,0 +1,11 @@
+# SwaggerAcceptConsentRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**ConsentRequestAcceptance**](consentRequestAcceptance.md) | | [default to null]
+**Id** | **string** | in: path | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerCreatePolicyParameters.md b/sdk/go/hydra/swagger/docs/SwaggerCreatePolicyParameters.md
new file mode 100644
index 00000000000..c8304b89405
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerCreatePolicyParameters.md
@@ -0,0 +1,10 @@
+# SwaggerCreatePolicyParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**Policy**](policy.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerDoesWardenAllowAccessRequestParameters.md b/sdk/go/hydra/swagger/docs/SwaggerDoesWardenAllowAccessRequestParameters.md
new file mode 100644
index 00000000000..b13bd4dcdb0
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerDoesWardenAllowAccessRequestParameters.md
@@ -0,0 +1,10 @@
+# SwaggerDoesWardenAllowAccessRequestParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**WardenAccessRequest**](wardenAccessRequest.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerDoesWardenAllowTokenAccessRequestParameters.md b/sdk/go/hydra/swagger/docs/SwaggerDoesWardenAllowTokenAccessRequestParameters.md
new file mode 100644
index 00000000000..4bc9e220d7e
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerDoesWardenAllowTokenAccessRequestParameters.md
@@ -0,0 +1,10 @@
+# SwaggerDoesWardenAllowTokenAccessRequestParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**WardenTokenAccessRequest**](wardenTokenAccessRequest.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerGetPolicyParameters.md b/sdk/go/hydra/swagger/docs/SwaggerGetPolicyParameters.md
new file mode 100644
index 00000000000..875e78471fd
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerGetPolicyParameters.md
@@ -0,0 +1,10 @@
+# SwaggerGetPolicyParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Id** | **string** | The id of the policy. in: path | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerJsonWebKeyQuery.md b/sdk/go/hydra/swagger/docs/SwaggerJsonWebKeyQuery.md
new file mode 100644
index 00000000000..d45d3c3d445
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerJsonWebKeyQuery.md
@@ -0,0 +1,11 @@
+# SwaggerJsonWebKeyQuery
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Kid** | **string** | The kid of the desired key in: path | [default to null]
+**Set** | **string** | The set in: path | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerJwkCreateSet.md b/sdk/go/hydra/swagger/docs/SwaggerJwkCreateSet.md
new file mode 100644
index 00000000000..478debd4358
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerJwkCreateSet.md
@@ -0,0 +1,11 @@
+# SwaggerJwkCreateSet
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**JsonWebKeySetGeneratorRequest**](jsonWebKeySetGeneratorRequest.md) | | [optional] [default to null]
+**Set** | **string** | The set in: path | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerJwkSetQuery.md b/sdk/go/hydra/swagger/docs/SwaggerJwkSetQuery.md
new file mode 100644
index 00000000000..b01da0a8ab4
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerJwkSetQuery.md
@@ -0,0 +1,10 @@
+# SwaggerJwkSetQuery
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Set** | **string** | The set in: path | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerJwkUpdateSet.md b/sdk/go/hydra/swagger/docs/SwaggerJwkUpdateSet.md
new file mode 100644
index 00000000000..8a55ce151c1
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerJwkUpdateSet.md
@@ -0,0 +1,11 @@
+# SwaggerJwkUpdateSet
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**JsonWebKeySet**](jsonWebKeySet.md) | | [optional] [default to null]
+**Set** | **string** | The set in: path | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerJwkUpdateSetKey.md b/sdk/go/hydra/swagger/docs/SwaggerJwkUpdateSetKey.md
new file mode 100644
index 00000000000..bbe84dd72e6
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerJwkUpdateSetKey.md
@@ -0,0 +1,12 @@
+# SwaggerJwkUpdateSetKey
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**JsonWebKey**](jsonWebKey.md) | | [optional] [default to null]
+**Kid** | **string** | The kid of the desired key in: path | [default to null]
+**Set** | **string** | The set in: path | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerListPolicyParameters.md b/sdk/go/hydra/swagger/docs/SwaggerListPolicyParameters.md
new file mode 100644
index 00000000000..8fc0c88627a
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerListPolicyParameters.md
@@ -0,0 +1,11 @@
+# SwaggerListPolicyParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Limit** | **int64** | The maximum amount of policies returned. in: query | [optional] [default to null]
+**Offset** | **int64** | The offset from where to start looking. in: query | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerListPolicyResponse.md b/sdk/go/hydra/swagger/docs/SwaggerListPolicyResponse.md
new file mode 100644
index 00000000000..73c549f54df
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerListPolicyResponse.md
@@ -0,0 +1,10 @@
+# SwaggerListPolicyResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**[]Policy**](policy.md) | in: body type: array | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerOAuthConsentRequest.md b/sdk/go/hydra/swagger/docs/SwaggerOAuthConsentRequest.md
new file mode 100644
index 00000000000..07c4aada4f9
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerOAuthConsentRequest.md
@@ -0,0 +1,10 @@
+# SwaggerOAuthConsentRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**OAuth2consentRequest**](oAuth2consentRequest.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerOAuthConsentRequestPayload.md b/sdk/go/hydra/swagger/docs/SwaggerOAuthConsentRequestPayload.md
new file mode 100644
index 00000000000..f92a341f9f2
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerOAuthConsentRequestPayload.md
@@ -0,0 +1,10 @@
+# SwaggerOAuthConsentRequestPayload
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Id** | **string** | The id of the OAuth 2.0 Consent Request. | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerOAuthIntrospectionRequest.md b/sdk/go/hydra/swagger/docs/SwaggerOAuthIntrospectionRequest.md
new file mode 100644
index 00000000000..4937bac6b19
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerOAuthIntrospectionRequest.md
@@ -0,0 +1,11 @@
+# SwaggerOAuthIntrospectionRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Scope** | **string** | An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false. in: formData | [optional] [default to null]
+**Token** | **string** | The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation. | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerOAuthIntrospectionResponse.md b/sdk/go/hydra/swagger/docs/SwaggerOAuthIntrospectionResponse.md
new file mode 100644
index 00000000000..fee839bf593
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerOAuthIntrospectionResponse.md
@@ -0,0 +1,10 @@
+# SwaggerOAuthIntrospectionResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**OAuth2TokenIntrospection**](oAuth2TokenIntrospection.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerOAuthTokenResponse.md b/sdk/go/hydra/swagger/docs/SwaggerOAuthTokenResponse.md
new file mode 100644
index 00000000000..002428deb42
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerOAuthTokenResponse.md
@@ -0,0 +1,10 @@
+# SwaggerOAuthTokenResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**SwaggerOAuthTokenResponseBody**](swaggerOAuthTokenResponse_Body.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerOAuthTokenResponseBody.md b/sdk/go/hydra/swagger/docs/SwaggerOAuthTokenResponseBody.md
new file mode 100644
index 00000000000..2b41bf6a972
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerOAuthTokenResponseBody.md
@@ -0,0 +1,15 @@
+# SwaggerOAuthTokenResponseBody
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**AccessToken** | **string** | The access token issued by the authorization server. | [optional] [default to null]
+**ExpiresIn** | **int64** | The lifetime in seconds of the access token. For example, the value \"3600\" denotes that the access token will expire in one hour from the time the response was generated. | [optional] [default to null]
+**IdToken** | **int64** | To retrieve a refresh token request the id_token scope. | [optional] [default to null]
+**RefreshToken** | **string** | The refresh token, which can be used to obtain new access tokens. To retrieve it add the scope \"offline\" to your access token request. | [optional] [default to null]
+**Scope** | **int64** | The scope of the access token | [optional] [default to null]
+**TokenType** | **string** | The type of the token issued | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerRejectConsentRequest.md b/sdk/go/hydra/swagger/docs/SwaggerRejectConsentRequest.md
new file mode 100644
index 00000000000..80dc405992a
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerRejectConsentRequest.md
@@ -0,0 +1,11 @@
+# SwaggerRejectConsentRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**ConsentRequestRejection**](consentRequestRejection.md) | | [default to null]
+**Id** | **string** | in: path | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerRevokeOAuth2TokenParameters.md b/sdk/go/hydra/swagger/docs/SwaggerRevokeOAuth2TokenParameters.md
new file mode 100644
index 00000000000..20e8dc548a1
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerRevokeOAuth2TokenParameters.md
@@ -0,0 +1,10 @@
+# SwaggerRevokeOAuth2TokenParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Token** | **string** | in: formData | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerUpdatePolicyParameters.md b/sdk/go/hydra/swagger/docs/SwaggerUpdatePolicyParameters.md
new file mode 100644
index 00000000000..c78915af388
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerUpdatePolicyParameters.md
@@ -0,0 +1,11 @@
+# SwaggerUpdatePolicyParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**Policy**](policy.md) | | [optional] [default to null]
+**Id** | **string** | The id of the policy. in: path | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerWardenAccessRequestResponseParameters.md b/sdk/go/hydra/swagger/docs/SwaggerWardenAccessRequestResponseParameters.md
new file mode 100644
index 00000000000..397e6984cd8
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerWardenAccessRequestResponseParameters.md
@@ -0,0 +1,10 @@
+# SwaggerWardenAccessRequestResponseParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**WardenAccessRequestResponse**](wardenAccessRequestResponse.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/SwaggerWardenTokenAccessRequestResponse.md b/sdk/go/hydra/swagger/docs/SwaggerWardenTokenAccessRequestResponse.md
new file mode 100644
index 00000000000..65a2eac2564
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/SwaggerWardenTokenAccessRequestResponse.md
@@ -0,0 +1,10 @@
+# SwaggerWardenTokenAccessRequestResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Body** | [**WardenTokenAccessRequestResponsePayload**](wardenTokenAccessRequestResponsePayload.md) | | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/TokenAllowedRequest.md b/sdk/go/hydra/swagger/docs/TokenAllowedRequest.md
new file mode 100644
index 00000000000..58d13fb76f2
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/TokenAllowedRequest.md
@@ -0,0 +1,12 @@
+# TokenAllowedRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Action** | **string** | Action is the action that is requested on the resource. | [optional] [default to null]
+**Context** | [**map[string]interface{}**](interface{}.md) | Context is the request's environmental context. | [optional] [default to null]
+**Resource** | **string** | Resource is the resource that access is requested to. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/WardenAccessRequest.md b/sdk/go/hydra/swagger/docs/WardenAccessRequest.md
new file mode 100644
index 00000000000..502fd2b941d
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/WardenAccessRequest.md
@@ -0,0 +1,13 @@
+# WardenAccessRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Action** | **string** | Action is the action that is requested on the resource. | [optional] [default to null]
+**Context** | [**map[string]interface{}**](interface{}.md) | Context is the request's environmental context. | [optional] [default to null]
+**Resource** | **string** | Resource is the resource that access is requested to. | [optional] [default to null]
+**Subject** | **string** | Subejct is the subject that is requesting access. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/WardenAccessRequestResponse.md b/sdk/go/hydra/swagger/docs/WardenAccessRequestResponse.md
new file mode 100644
index 00000000000..73325c1d009
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/WardenAccessRequestResponse.md
@@ -0,0 +1,10 @@
+# WardenAccessRequestResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Allowed** | **bool** | Allowed is true if the request is allowed and false otherwise. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/WardenApi.md b/sdk/go/hydra/swagger/docs/WardenApi.md
new file mode 100644
index 00000000000..6e14c8b9053
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/WardenApi.md
@@ -0,0 +1,250 @@
+# \WardenApi
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**AddMembersToGroup**](WardenApi.md#AddMembersToGroup) | **Post** /warden/groups/{id}/members | Add members to a group
+[**CreateGroup**](WardenApi.md#CreateGroup) | **Post** /warden/groups | Create a group
+[**DeleteGroup**](WardenApi.md#DeleteGroup) | **Delete** /warden/groups/{id} | Delete a group by id
+[**DoesWardenAllowAccessRequest**](WardenApi.md#DoesWardenAllowAccessRequest) | **Post** /warden/allowed | Check if an access request is valid (without providing an access token)
+[**DoesWardenAllowTokenAccessRequest**](WardenApi.md#DoesWardenAllowTokenAccessRequest) | **Post** /warden/token/allowed | Check if an access request is valid (providing an access token)
+[**FindGroupsByMember**](WardenApi.md#FindGroupsByMember) | **Get** /warden/groups | Find groups by member
+[**GetGroup**](WardenApi.md#GetGroup) | **Get** /warden/groups/{id} | Get a group by id
+[**RemoveMembersFromGroup**](WardenApi.md#RemoveMembersFromGroup) | **Delete** /warden/groups/{id}/members | Remove members from a group
+
+
+# **AddMembersToGroup**
+> AddMembersToGroup($id, $body)
+
+Add members to a group
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:\"], \"actions\": [\"members.add\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the group to modify. |
+ **body** | [**GroupMembers**](GroupMembers.md)| | [optional]
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **CreateGroup**
+> Group CreateGroup($body)
+
+Create a group
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**Group**](Group.md)| | [optional]
+
+### Return type
+
+[**Group**](group.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **DeleteGroup**
+> DeleteGroup($id)
+
+Delete a group by id
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the group to look up. |
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **DoesWardenAllowAccessRequest**
+> WardenAccessRequestResponse DoesWardenAllowAccessRequest($body)
+
+Check if an access request is valid (without providing an access token)
+
+Checks if a subject (typically a user or a service) is allowed to perform an action on a resource. This endpoint requires a subject, a resource name, an action name and a context. If the subject is not allowed to perform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`, otherwise `{ \"allowed\": true }` is returned. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:allowed\"], \"actions\": [\"decide\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**WardenAccessRequest**](WardenAccessRequest.md)| | [optional]
+
+### Return type
+
+[**WardenAccessRequestResponse**](wardenAccessRequestResponse.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **DoesWardenAllowTokenAccessRequest**
+> WardenTokenAccessRequestResponsePayload DoesWardenAllowTokenAccessRequest($body)
+
+Check if an access request is valid (providing an access token)
+
+Checks if a token is valid and if the token subject is allowed to perform an action on a resource. This endpoint requires a token, a scope, a resource name, an action name and a context. If a token is expired/invalid, has not been granted the requested scope or the subject is not allowed to perform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`. Extra data set through the `accessTokenExtra` field in the consent flow will be included in the response. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:token:allowed\"], \"actions\": [\"decide\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**WardenTokenAccessRequest**](WardenTokenAccessRequest.md)| | [optional]
+
+### Return type
+
+[**WardenTokenAccessRequestResponsePayload**](wardenTokenAccessRequestResponsePayload.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **FindGroupsByMember**
+> []Group FindGroupsByMember($member)
+
+Find groups by member
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups\"], \"actions\": [\"list\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **member** | **string**| The id of the member to look up. |
+
+### Return type
+
+[**[]Group**](group.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **GetGroup**
+> Group GetGroup($id)
+
+Get a group by id
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the group to look up. |
+
+### Return type
+
+[**Group**](group.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
+# **RemoveMembersFromGroup**
+> RemoveMembersFromGroup($id, $body)
+
+Remove members from a group
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:\"], \"actions\": [\"members.remove\"], \"effect\": \"allow\" } ```
+
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **string**| The id of the group to modify. |
+ **body** | [**GroupMembers**](GroupMembers.md)| | [optional]
+
+### Return type
+
+void (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+[[Back to top]](#) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to Model list]](../README.md#documentation-for-models) [[Back to README]](../README.md)
+
diff --git a/sdk/go/hydra/swagger/docs/WardenTokenAccessRequest.md b/sdk/go/hydra/swagger/docs/WardenTokenAccessRequest.md
new file mode 100644
index 00000000000..19859c91851
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/WardenTokenAccessRequest.md
@@ -0,0 +1,14 @@
+# WardenTokenAccessRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Action** | **string** | Action is the action that is requested on the resource. | [optional] [default to null]
+**Context** | [**map[string]interface{}**](interface{}.md) | Context is the request's environmental context. | [optional] [default to null]
+**Resource** | **string** | Resource is the resource that access is requested to. | [optional] [default to null]
+**Scopes** | **[]string** | Scopes is an array of scopes that are requried. | [optional] [default to null]
+**Token** | **string** | Token is the token to introspect. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/WardenTokenAccessRequestResponsePayload.md b/sdk/go/hydra/swagger/docs/WardenTokenAccessRequestResponsePayload.md
new file mode 100644
index 00000000000..0e0d0f09a46
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/WardenTokenAccessRequestResponsePayload.md
@@ -0,0 +1,17 @@
+# WardenTokenAccessRequestResponsePayload
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**Allowed** | **bool** | Allowed is true if the request is allowed and false otherwise. | [optional] [default to null]
+**Aud** | **string** | Audience is who the token was issued for. This is an OAuth2 app usually. | [optional] [default to null]
+**Exp** | **string** | ExpiresAt is the expiry timestamp. | [optional] [default to null]
+**Ext** | [**map[string]interface{}**](interface{}.md) | Extra represents arbitrary session data. | [optional] [default to null]
+**Iat** | **string** | IssuedAt is the token creation time stamp. | [optional] [default to null]
+**Iss** | **string** | Issuer is the id of the issuer, typically an hydra instance. | [optional] [default to null]
+**Scopes** | **[]string** | GrantedScopes is a list of scopes that the subject authorized when asked for consent. | [optional] [default to null]
+**Sub** | **string** | Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app. This is usually a uuid but you can choose a urn or some other id too. | [optional] [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/WellKnown.md b/sdk/go/hydra/swagger/docs/WellKnown.md
new file mode 100644
index 00000000000..94960bb3dca
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/WellKnown.md
@@ -0,0 +1,16 @@
+# WellKnown
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**AuthorizationEndpoint** | **string** | URL of the OP's OAuth 2.0 Authorization Endpoint | [default to null]
+**IdTokenSigningAlgValuesSupported** | **[]string** | JSON array containing a list of the JWS signing algorithms (alg values) supported by the OP for the ID Token to encode the Claims in a JWT [JWT]. The algorithm RS256 MUST be included. The value none MAY be supported, but MUST NOT be used unless the Response Type used returns no ID Token from the Authorization Endpoint (such as when using the Authorization Code Flow). | [default to null]
+**Issuer** | **string** | URL using the https scheme with no query or fragment component that the OP asserts as its Issuer Identifier. If Issuer discovery is supported , this value MUST be identical to the issuer value returned by WebFinger. This also MUST be identical to the iss Claim value in ID Tokens issued from this Issuer. | [default to null]
+**JwksUri** | **string** | URL of the OP's JSON Web Key Set [JWK] document. This contains the signing key(s) the RP uses to validate signatures from the OP. The JWK Set MAY also contain the Server's encryption key(s), which are used by RPs to encrypt requests to the Server. When both signing and encryption keys are made available, a use (Key Use) parameter value is REQUIRED for all keys in the referenced JWK Set to indicate each key's intended usage. Although some algorithms allow the same key to be used for both signatures and encryption, doing so is NOT RECOMMENDED, as it is less secure. The JWK x5c parameter MAY be used to provide X.509 representations of keys provided. When used, the bare key values MUST still be present and MUST match those in the certificate. | [default to null]
+**ResponseTypesSupported** | **[]string** | JSON array containing a list of the OAuth 2.0 response_type values that this OP supports. Dynamic OpenID Providers MUST support the code, id_token, and the token id_token Response Type values. | [default to null]
+**SubjectTypesSupported** | **[]string** | JSON array containing a list of the Subject Identifier types that this OP supports. Valid types include pairwise and public. | [default to null]
+**TokenEndpoint** | **string** | URL of the OP's OAuth 2.0 Token Endpoint | [default to null]
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/docs/Writer.md b/sdk/go/hydra/swagger/docs/Writer.md
new file mode 100644
index 00000000000..530fd0f80e3
--- /dev/null
+++ b/sdk/go/hydra/swagger/docs/Writer.md
@@ -0,0 +1,9 @@
+# Writer
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+[[Back to Model list]](../README.md#documentation-for-models) [[Back to API list]](../README.md#documentation-for-api-endpoints) [[Back to README]](../README.md)
+
+
diff --git a/sdk/go/hydra/swagger/firewall.go b/sdk/go/hydra/swagger/firewall.go
new file mode 100644
index 00000000000..d99211e6860
--- /dev/null
+++ b/sdk/go/hydra/swagger/firewall.go
@@ -0,0 +1,14 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 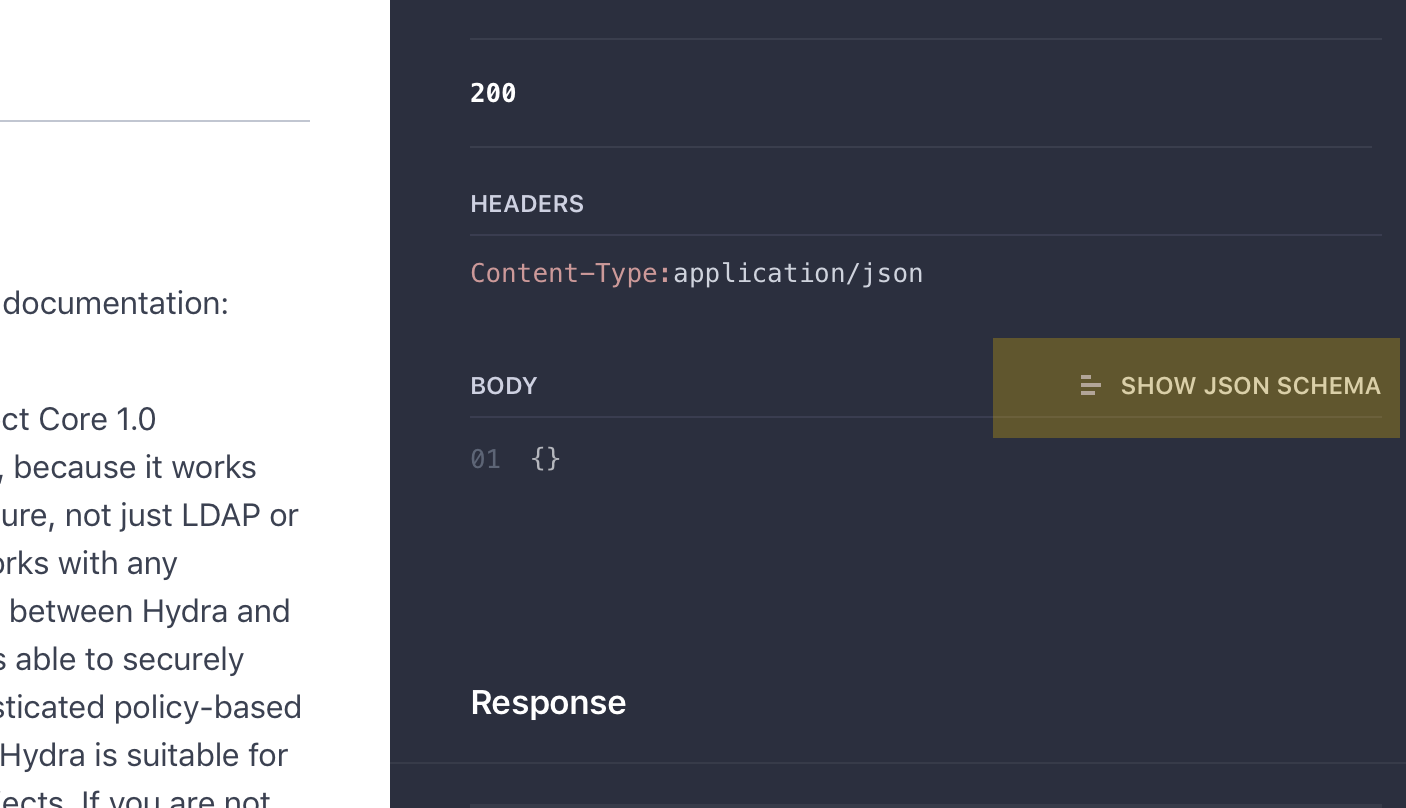 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type Firewall struct {
+}
diff --git a/sdk/go/hydra/swagger/git_push.sh b/sdk/go/hydra/swagger/git_push.sh
new file mode 100644
index 00000000000..ed374619b13
--- /dev/null
+++ b/sdk/go/hydra/swagger/git_push.sh
@@ -0,0 +1,52 @@
+#!/bin/sh
+# ref: https://help.github.com/articles/adding-an-existing-project-to-github-using-the-command-line/
+#
+# Usage example: /bin/sh ./git_push.sh wing328 swagger-petstore-perl "minor update"
+
+git_user_id=$1
+git_repo_id=$2
+release_note=$3
+
+if [ "$git_user_id" = "" ]; then
+ git_user_id="GIT_USER_ID"
+ echo "[INFO] No command line input provided. Set \$git_user_id to $git_user_id"
+fi
+
+if [ "$git_repo_id" = "" ]; then
+ git_repo_id="GIT_REPO_ID"
+ echo "[INFO] No command line input provided. Set \$git_repo_id to $git_repo_id"
+fi
+
+if [ "$release_note" = "" ]; then
+ release_note="Minor update"
+ echo "[INFO] No command line input provided. Set \$release_note to $release_note"
+fi
+
+# Initialize the local directory as a Git repository
+git init
+
+# Adds the files in the local repository and stages them for commit.
+git add .
+
+# Commits the tracked changes and prepares them to be pushed to a remote repository.
+git commit -m "$release_note"
+
+# Sets the new remote
+git_remote=`git remote`
+if [ "$git_remote" = "" ]; then # git remote not defined
+
+ if [ "$GIT_TOKEN" = "" ]; then
+ echo "[INFO] \$GIT_TOKEN (environment variable) is not set. Using the git crediential in your environment."
+ git remote add origin https://github.com/${git_user_id}/${git_repo_id}.git
+ else
+ git remote add origin https://${git_user_id}:${GIT_TOKEN}@github.com/${git_user_id}/${git_repo_id}.git
+ fi
+
+fi
+
+git pull origin master
+
+# Pushes (Forces) the changes in the local repository up to the remote repository
+echo "Git pushing to https://github.com/${git_user_id}/${git_repo_id}.git"
+git push origin master 2>&1 | grep -v 'To https'
+
diff --git a/sdk/go/hydra/swagger/group.go b/sdk/go/hydra/swagger/group.go
new file mode 100644
index 00000000000..4bacc07deb4
--- /dev/null
+++ b/sdk/go/hydra/swagger/group.go
@@ -0,0 +1,21 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 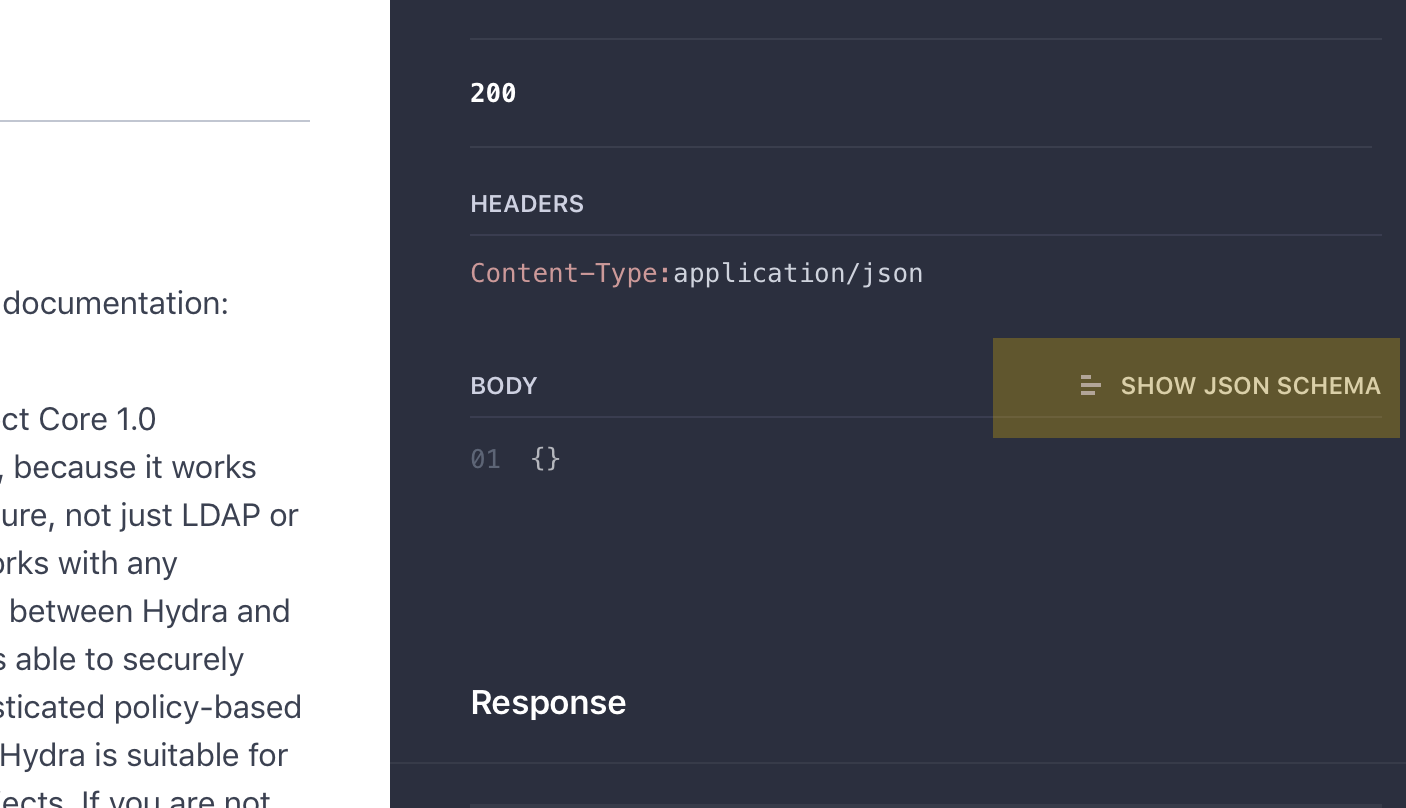 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// Group represents a warden group
+type Group struct {
+
+ // ID is the groups id.
+ Id string `json:"id,omitempty"`
+
+ // Members is who belongs to the group.
+ Members []string `json:"members,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/group_members.go b/sdk/go/hydra/swagger/group_members.go
new file mode 100644
index 00000000000..4bec5181c0b
--- /dev/null
+++ b/sdk/go/hydra/swagger/group_members.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 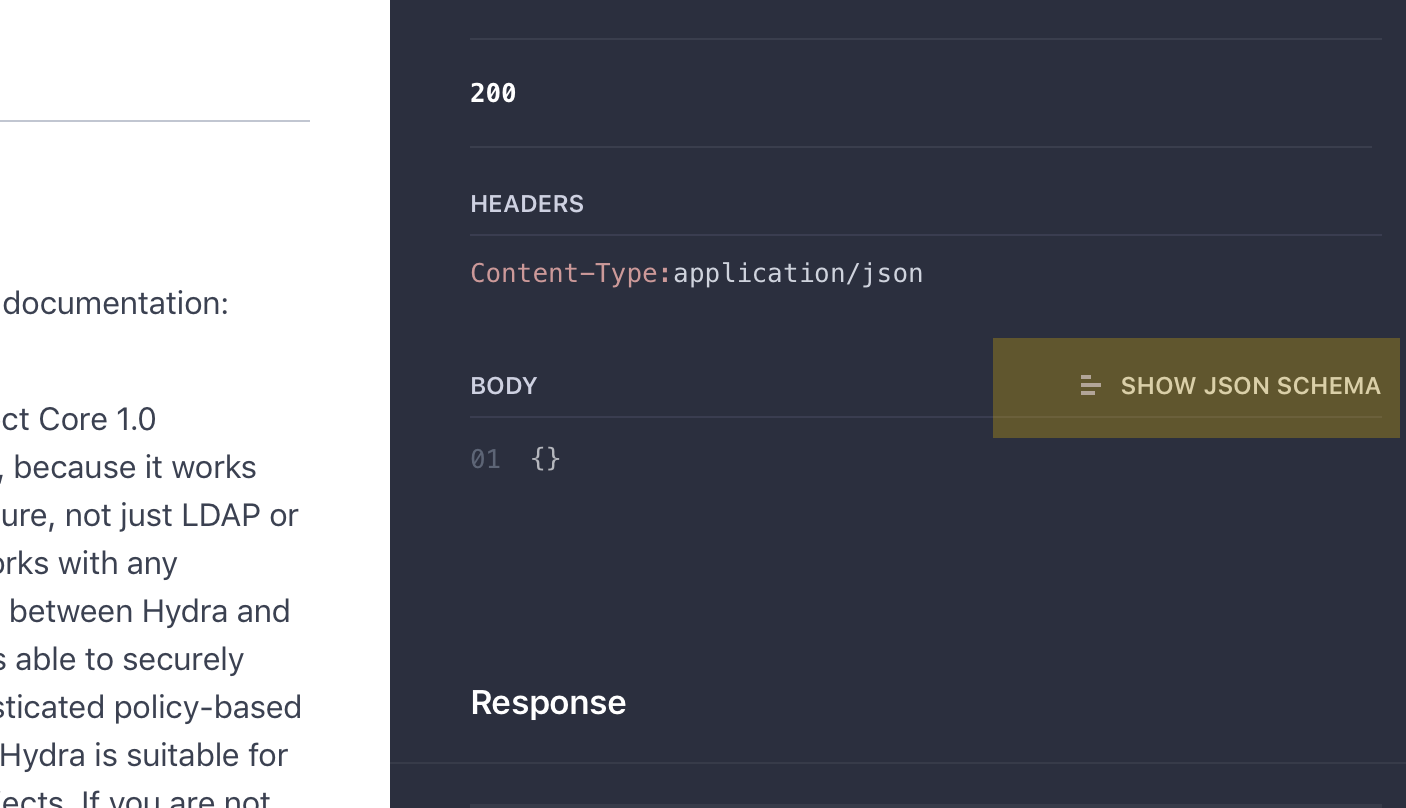 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type GroupMembers struct {
+ Members []string `json:"members,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/handler.go b/sdk/go/hydra/swagger/handler.go
new file mode 100644
index 00000000000..14c0e7786a0
--- /dev/null
+++ b/sdk/go/hydra/swagger/handler.go
@@ -0,0 +1,21 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 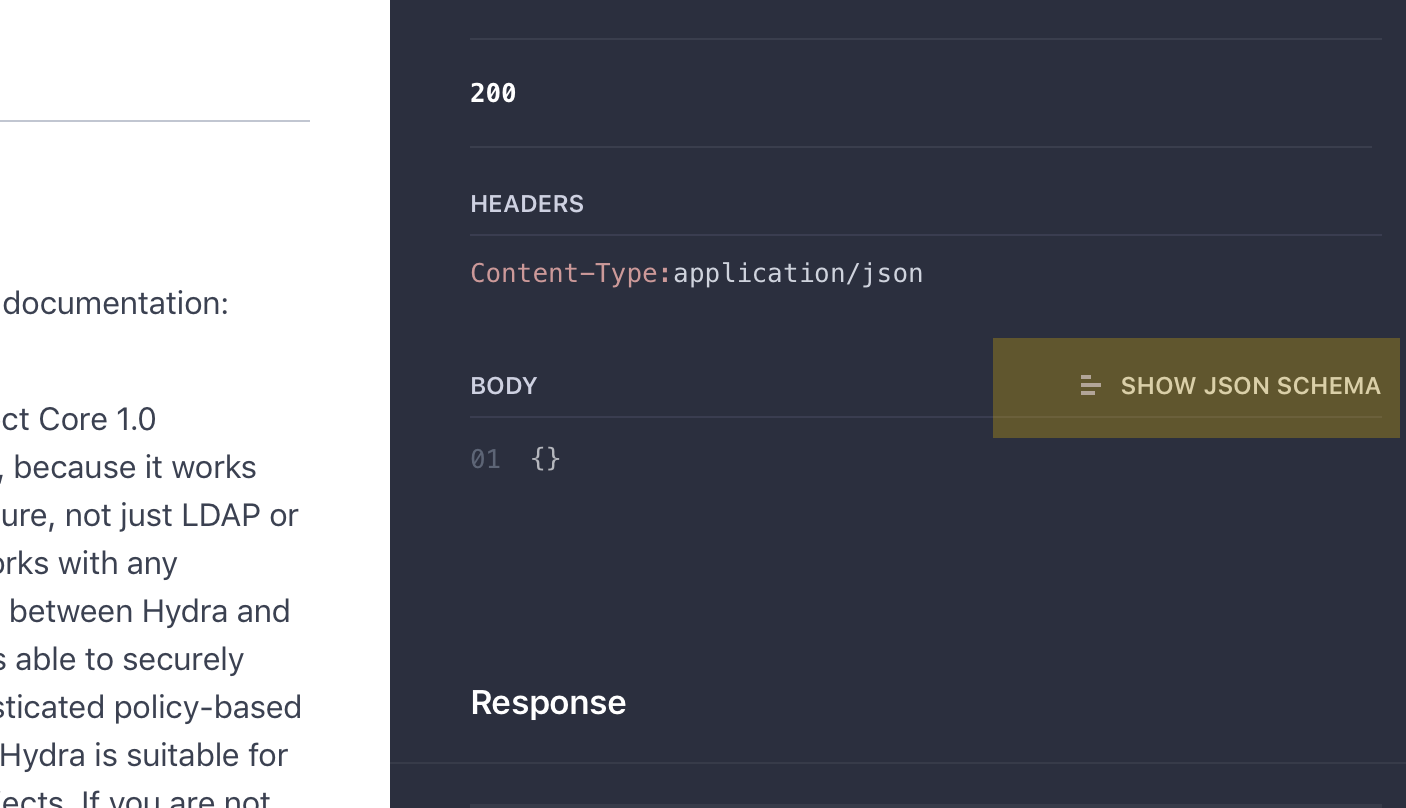 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type Handler struct {
+ Generators map[string]KeyGenerator `json:"Generators,omitempty"`
+
+ H Writer `json:"H,omitempty"`
+
+ Manager Manager `json:"Manager,omitempty"`
+
+ W Firewall `json:"W,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/health_api.go b/sdk/go/hydra/swagger/health_api.go
new file mode 100644
index 00000000000..c4347eca5a7
--- /dev/null
+++ b/sdk/go/hydra/swagger/health_api.go
@@ -0,0 +1,158 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 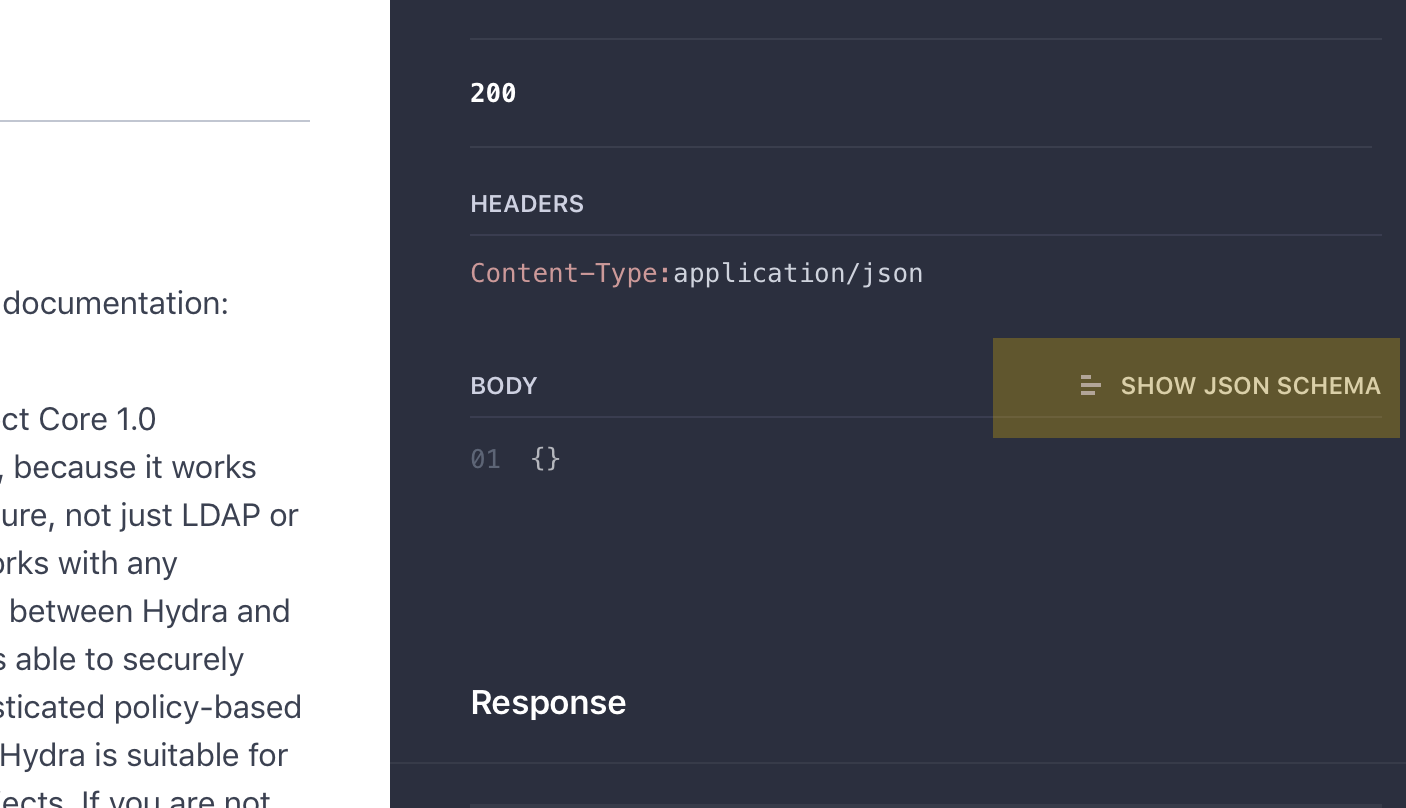 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+import (
+ "encoding/json"
+ "net/url"
+ "strings"
+)
+
+type HealthApi struct {
+ Configuration *Configuration
+}
+
+func NewHealthApi() *HealthApi {
+ configuration := NewConfiguration()
+ return &HealthApi{
+ Configuration: configuration,
+ }
+}
+
+func NewHealthApiWithBasePath(basePath string) *HealthApi {
+ configuration := NewConfiguration()
+ configuration.BasePath = basePath
+
+ return &HealthApi{
+ Configuration: configuration,
+ }
+}
+
+/**
+ * Show instance metrics (experimental)
+ * This endpoint returns an instance's metrics, such as average response time, status code distribution, hits per second and so on. The return values are currently not documented as this endpoint is still experimental. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:health:stats\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ *
+ * @return void
+ */
+func (a HealthApi) GetInstanceMetrics() (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/health/metrics"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetInstanceMetrics", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Check health status of this instance
+ * This endpoint returns `{ \"status\": \"ok\" }`. This status let's you know that the HTTP server is up and running. This status does currently not include checks whether the database connection is up and running. This endpoint does not require the `X-Forwarded-Proto` header when TLS termination is set. Be aware that if you are running multiple nodes of ORY Hydra, the health status will never refer to the cluster state, only to a single instance.
+ *
+ * @return *InlineResponse200
+ */
+func (a HealthApi) GetInstanceStatus() (*InlineResponse200, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/health/status"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json", "application/x-www-form-urlencoded"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(InlineResponse200)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetInstanceStatus", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
diff --git a/sdk/go/hydra/swagger/inline_response_200.go b/sdk/go/hydra/swagger/inline_response_200.go
new file mode 100644
index 00000000000..b80037bf5ca
--- /dev/null
+++ b/sdk/go/hydra/swagger/inline_response_200.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 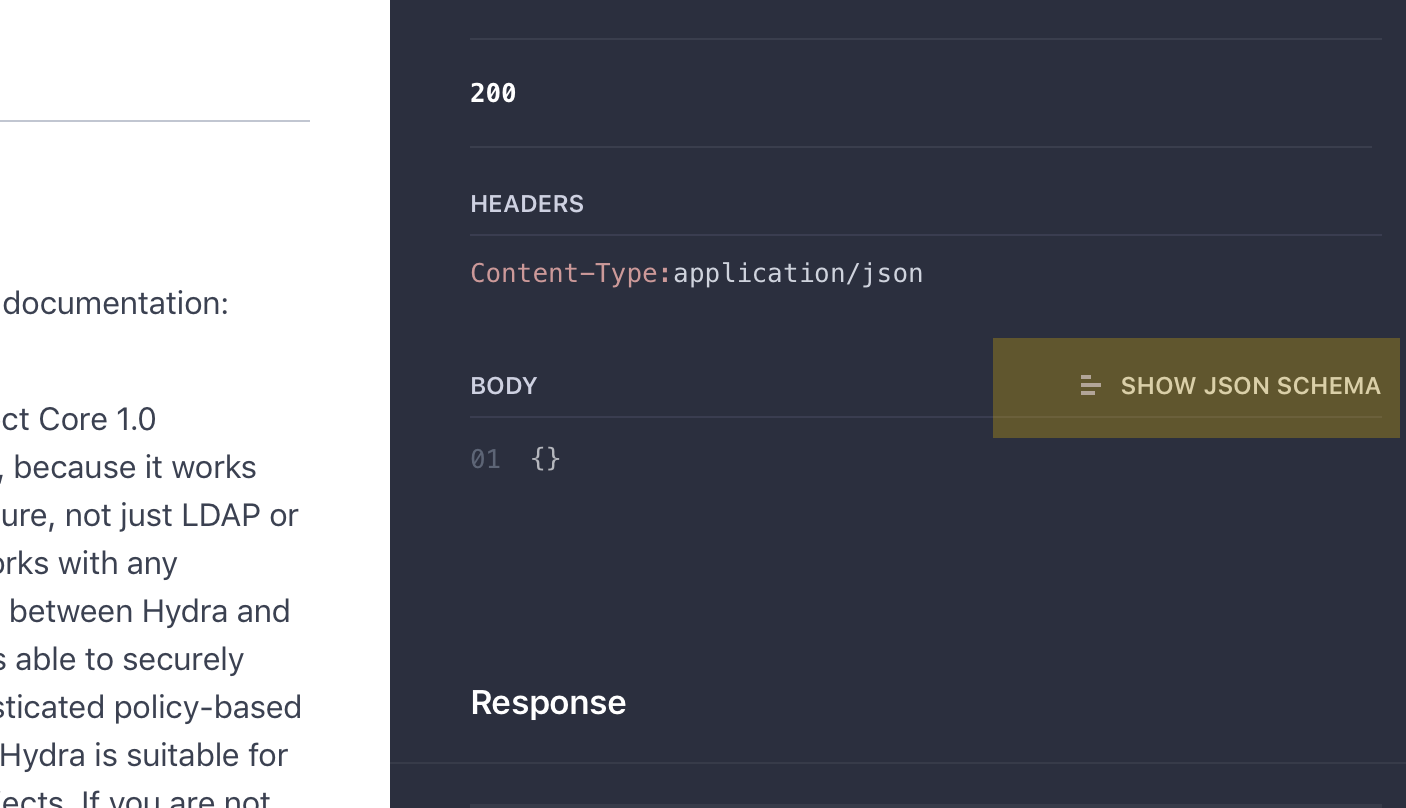 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type InlineResponse200 struct {
+
+ // Status always contains \"ok\"
+ Status string `json:"status,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/inline_response_200_1.go b/sdk/go/hydra/swagger/inline_response_200_1.go
new file mode 100644
index 00000000000..362ec27e10b
--- /dev/null
+++ b/sdk/go/hydra/swagger/inline_response_200_1.go
@@ -0,0 +1,32 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 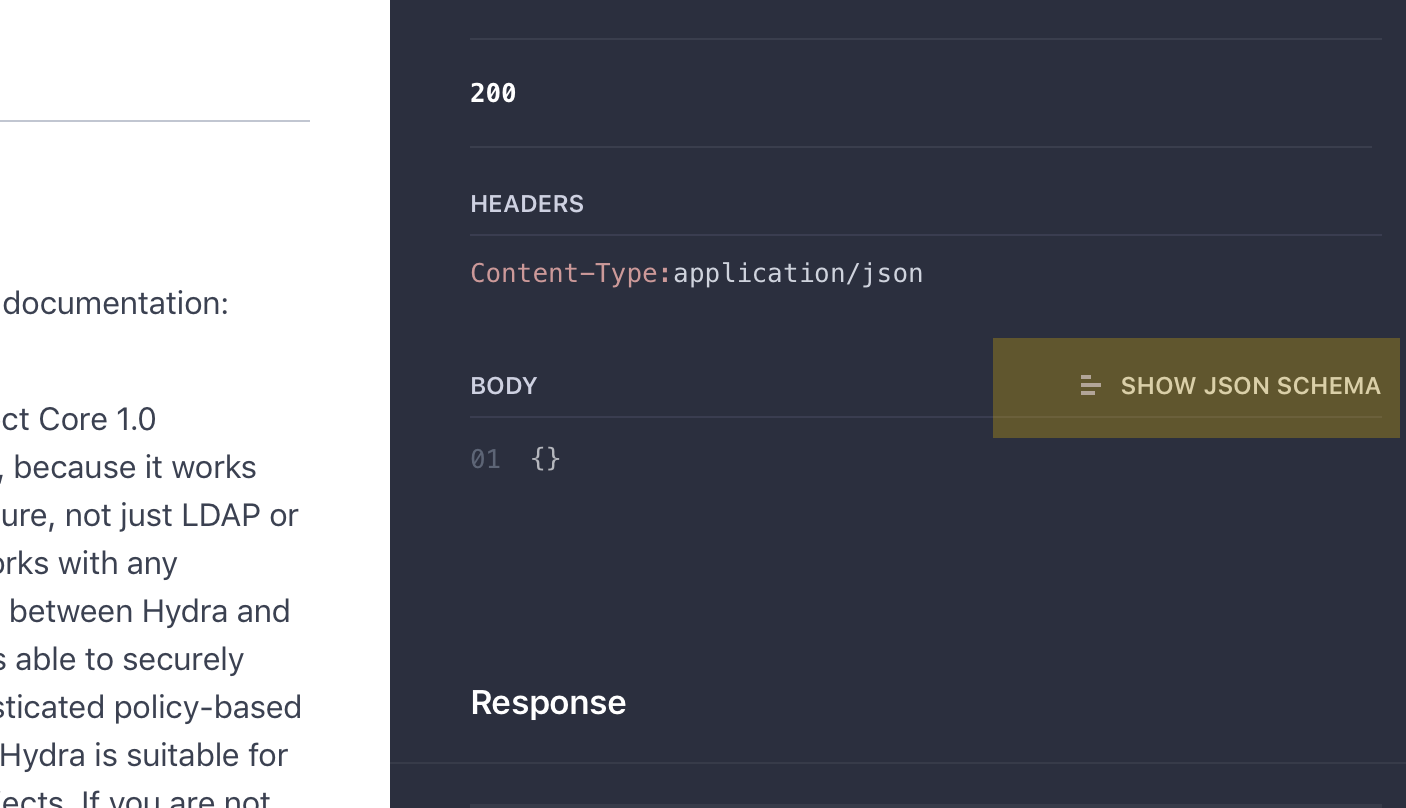 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type InlineResponse2001 struct {
+
+ // The access token issued by the authorization server.
+ AccessToken string `json:"access_token,omitempty"`
+
+ // The lifetime in seconds of the access token. For example, the value \"3600\" denotes that the access token will expire in one hour from the time the response was generated.
+ ExpiresIn int64 `json:"expires_in,omitempty"`
+
+ // To retrieve a refresh token request the id_token scope.
+ IdToken int64 `json:"id_token,omitempty"`
+
+ // The refresh token, which can be used to obtain new access tokens. To retrieve it add the scope \"offline\" to your access token request.
+ RefreshToken string `json:"refresh_token,omitempty"`
+
+ // The scope of the access token
+ Scope int64 `json:"scope,omitempty"`
+
+ // The type of the token issued
+ TokenType string `json:"token_type,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/inline_response_401.go b/sdk/go/hydra/swagger/inline_response_401.go
new file mode 100644
index 00000000000..2833c3e2e47
--- /dev/null
+++ b/sdk/go/hydra/swagger/inline_response_401.go
@@ -0,0 +1,25 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 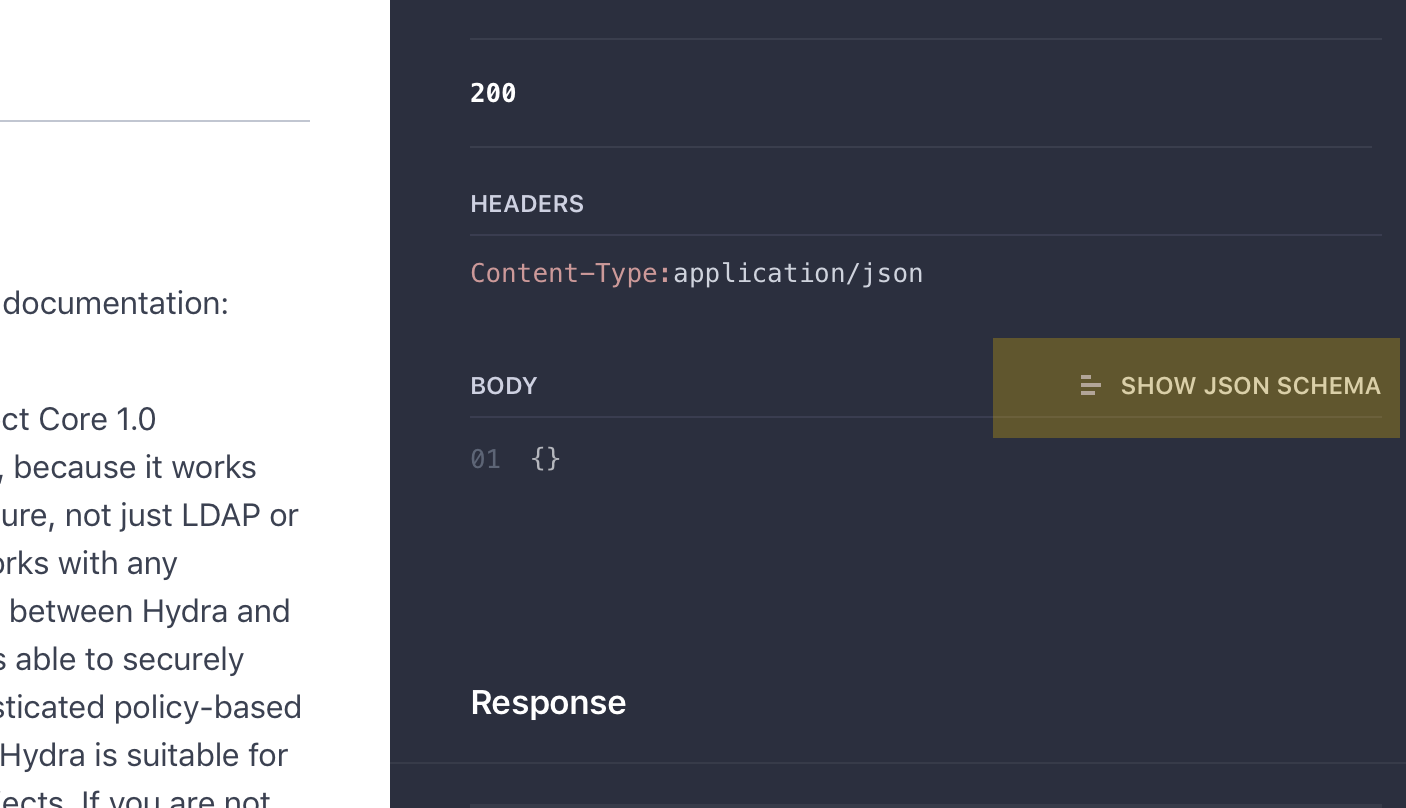 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type InlineResponse401 struct {
+ Code int64 `json:"code,omitempty"`
+
+ Details []map[string]interface{} `json:"details,omitempty"`
+
+ Message string `json:"message,omitempty"`
+
+ Reason string `json:"reason,omitempty"`
+
+ Request string `json:"request,omitempty"`
+
+ Status string `json:"status,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/jose_web_key_set_request.go b/sdk/go/hydra/swagger/jose_web_key_set_request.go
new file mode 100644
index 00000000000..e8c10758d06
--- /dev/null
+++ b/sdk/go/hydra/swagger/jose_web_key_set_request.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 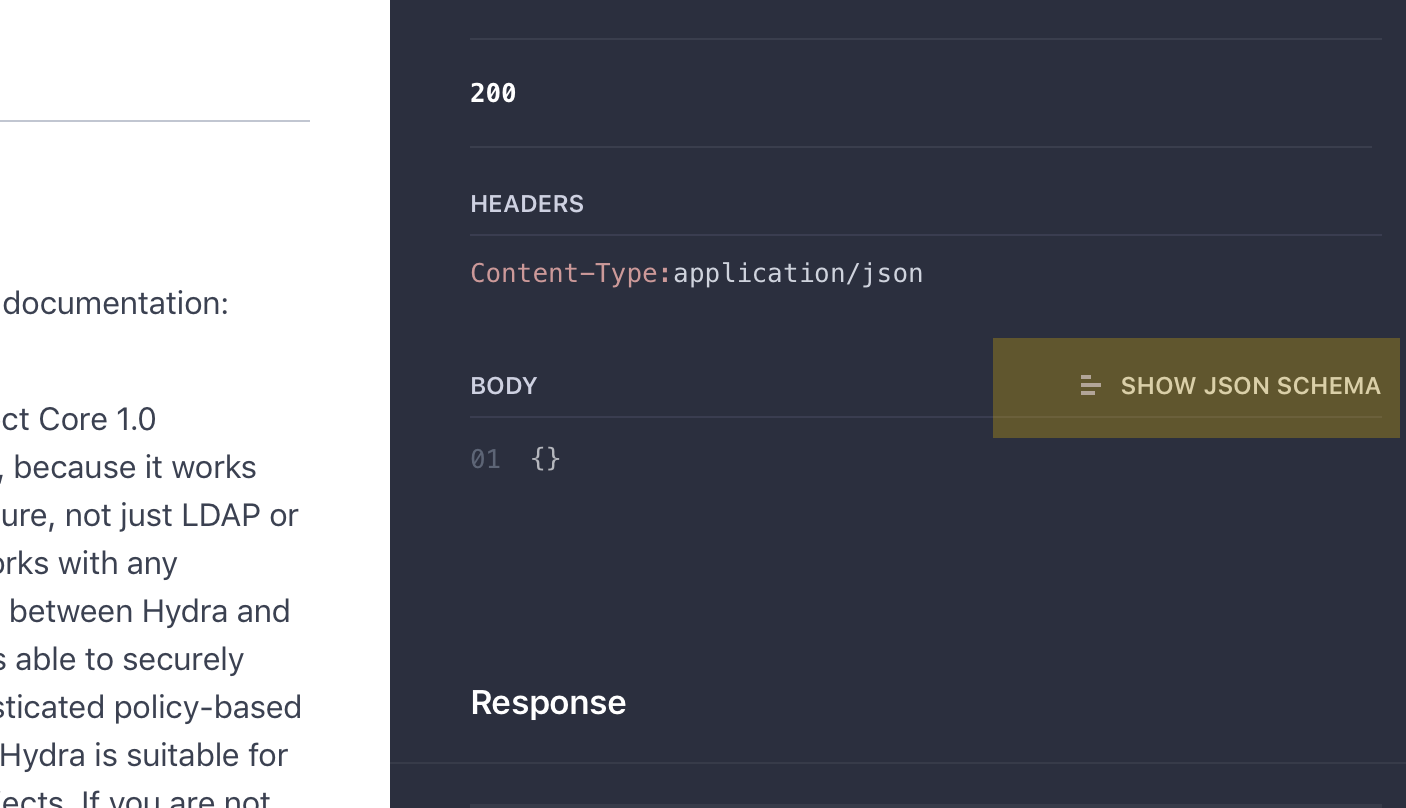 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type JoseWebKeySetRequest struct {
+ Keys []RawMessage `json:"keys,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/json_web_key.go b/sdk/go/hydra/swagger/json_web_key.go
new file mode 100644
index 00000000000..a29ca2bd00e
--- /dev/null
+++ b/sdk/go/hydra/swagger/json_web_key.go
@@ -0,0 +1,53 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 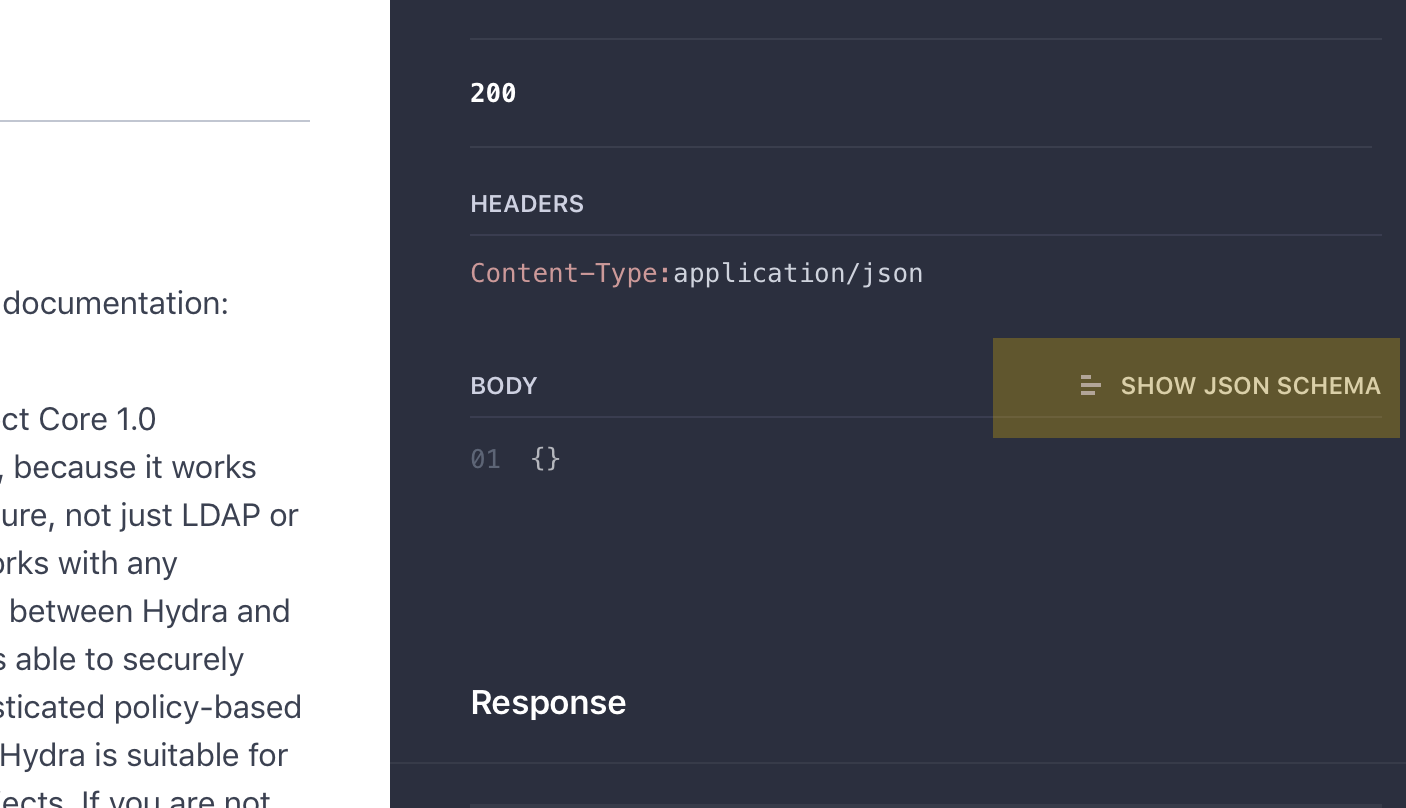 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type JsonWebKey struct {
+
+ // The \"alg\" (algorithm) parameter identifies the algorithm intended for use with the key. The values used should either be registered in the IANA \"JSON Web Signature and Encryption Algorithms\" registry established by [JWA] or be a value that contains a Collision- Resistant Name.
+ Alg string `json:"alg,omitempty"`
+
+ Crv string `json:"crv,omitempty"`
+
+ D string `json:"d,omitempty"`
+
+ Dp string `json:"dp,omitempty"`
+
+ Dq string `json:"dq,omitempty"`
+
+ E string `json:"e,omitempty"`
+
+ K string `json:"k,omitempty"`
+
+ // The \"kid\" (key ID) parameter is used to match a specific key. This is used, for instance, to choose among a set of keys within a JWK Set during key rollover. The structure of the \"kid\" value is unspecified. When \"kid\" values are used within a JWK Set, different keys within the JWK Set SHOULD use distinct \"kid\" values. (One example in which different keys might use the same \"kid\" value is if they have different \"kty\" (key type) values but are considered to be equivalent alternatives by the application using them.) The \"kid\" value is a case-sensitive string.
+ Kid string `json:"kid,omitempty"`
+
+ // The \"kty\" (key type) parameter identifies the cryptographic algorithm family used with the key, such as \"RSA\" or \"EC\". \"kty\" values should either be registered in the IANA \"JSON Web Key Types\" registry established by [JWA] or be a value that contains a Collision- Resistant Name. The \"kty\" value is a case-sensitive string.
+ Kty string `json:"kty,omitempty"`
+
+ N string `json:"n,omitempty"`
+
+ P string `json:"p,omitempty"`
+
+ Q string `json:"q,omitempty"`
+
+ Qi string `json:"qi,omitempty"`
+
+ // The \"use\" (public key use) parameter identifies the intended use of the public key. The \"use\" parameter is employed to indicate whether a public key is used for encrypting data or verifying the signature on data. Values are commonly \"sig\" (signature) or \"enc\" (encryption).
+ Use string `json:"use,omitempty"`
+
+ X string `json:"x,omitempty"`
+
+ // The \"x5c\" (X.509 certificate chain) parameter contains a chain of one or more PKIX certificates [RFC5280]. The certificate chain is represented as a JSON array of certificate value strings. Each string in the array is a base64-encoded (Section 4 of [RFC4648] -- not base64url-encoded) DER [ITU.X690.1994] PKIX certificate value. The PKIX certificate containing the key value MUST be the first certificate.
+ X5c []string `json:"x5c,omitempty"`
+
+ Y string `json:"y,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/json_web_key_api.go b/sdk/go/hydra/swagger/json_web_key_api.go
new file mode 100644
index 00000000000..bc15b383d47
--- /dev/null
+++ b/sdk/go/hydra/swagger/json_web_key_api.go
@@ -0,0 +1,511 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 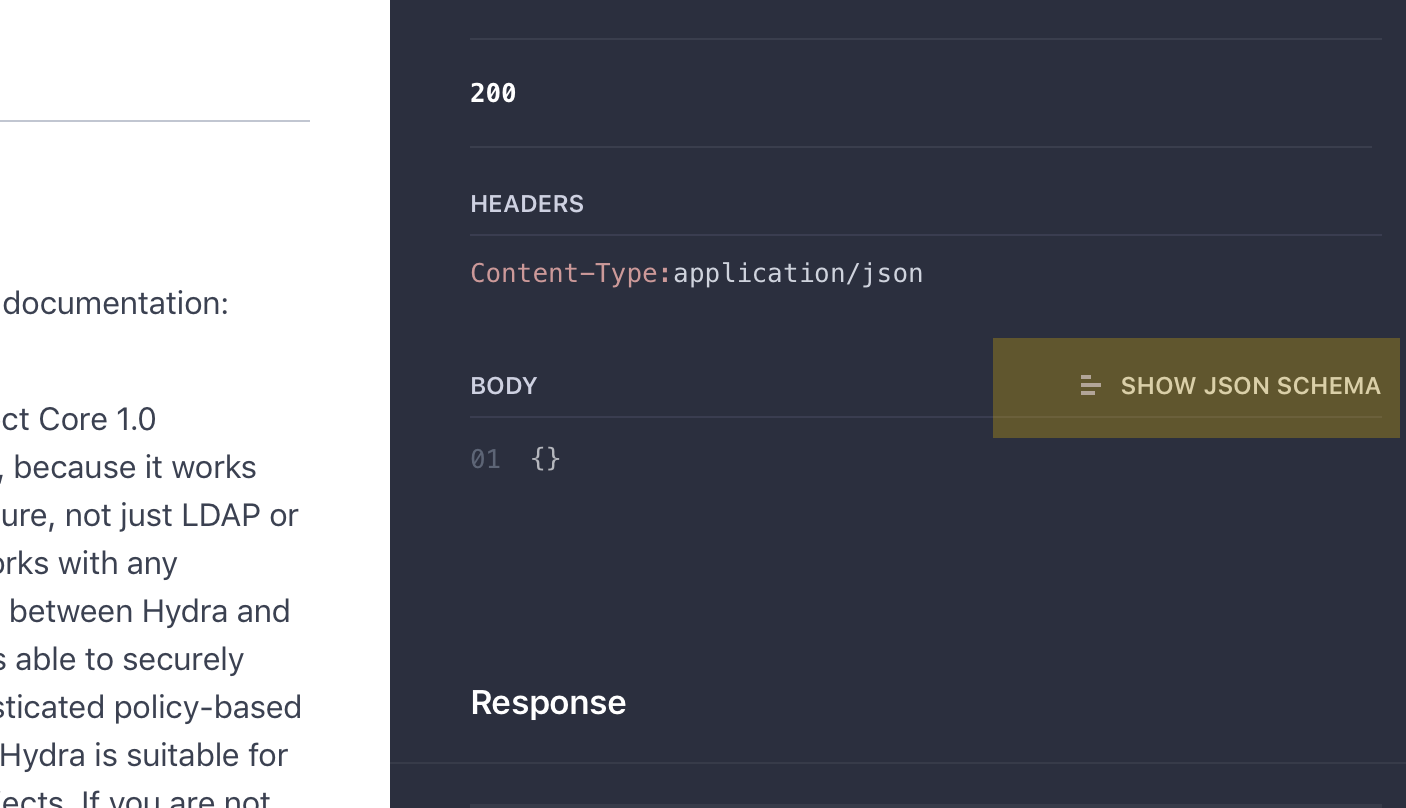 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+import (
+ "encoding/json"
+ "fmt"
+ "net/url"
+ "strings"
+)
+
+type JsonWebKeyApi struct {
+ Configuration *Configuration
+}
+
+func NewJsonWebKeyApi() *JsonWebKeyApi {
+ configuration := NewConfiguration()
+ return &JsonWebKeyApi{
+ Configuration: configuration,
+ }
+}
+
+func NewJsonWebKeyApiWithBasePath(basePath string) *JsonWebKeyApi {
+ configuration := NewConfiguration()
+ configuration.BasePath = basePath
+
+ return &JsonWebKeyApi{
+ Configuration: configuration,
+ }
+}
+
+/**
+ * Generate a new JSON Web Key
+ * This endpoint is capable of generating JSON Web Key Sets for you. There a different strategies available, such as symmetric cryptographic keys (HS256) and asymetric cryptographic keys (RS256, ECDSA). If the specified JSON Web Key Set does not exist, it will be created. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+ *
+ * @param set The set
+ * @param body
+ * @return *JsonWebKeySet
+ */
+func (a JsonWebKeyApi) CreateJsonWebKeySet(set string, body JsonWebKeySetGeneratorRequest) (*JsonWebKeySet, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/keys/{set}"
+ localVarPath = strings.Replace(localVarPath, "{"+"set"+"}", fmt.Sprintf("%v", set), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(JsonWebKeySet)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "CreateJsonWebKeySet", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Delete a JSON Web Key
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+ *
+ * @param kid The kid of the desired key
+ * @param set The set
+ * @return void
+ */
+func (a JsonWebKeyApi) DeleteJsonWebKey(kid string, set string) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Delete")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/keys/{set}/{kid}"
+ localVarPath = strings.Replace(localVarPath, "{"+"kid"+"}", fmt.Sprintf("%v", kid), -1)
+ localVarPath = strings.Replace(localVarPath, "{"+"set"+"}", fmt.Sprintf("%v", set), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "DeleteJsonWebKey", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Delete a JSON Web Key
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+ *
+ * @param set The set
+ * @return void
+ */
+func (a JsonWebKeyApi) DeleteJsonWebKeySet(set string) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Delete")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/keys/{set}"
+ localVarPath = strings.Replace(localVarPath, "{"+"set"+"}", fmt.Sprintf("%v", set), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "DeleteJsonWebKeySet", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Retrieve a JSON Web Key
+ * This endpoint can be used to retrieve JWKs stored in ORY Hydra. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ *
+ * @param kid The kid of the desired key
+ * @param set The set
+ * @return *JsonWebKeySet
+ */
+func (a JsonWebKeyApi) GetJsonWebKey(kid string, set string) (*JsonWebKeySet, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/keys/{set}/{kid}"
+ localVarPath = strings.Replace(localVarPath, "{"+"kid"+"}", fmt.Sprintf("%v", kid), -1)
+ localVarPath = strings.Replace(localVarPath, "{"+"set"+"}", fmt.Sprintf("%v", set), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(JsonWebKeySet)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetJsonWebKey", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Retrieve a JSON Web Key Set
+ * This endpoint can be used to retrieve JWK Sets stored in ORY Hydra. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ *
+ * @param set The set
+ * @return *JsonWebKeySet
+ */
+func (a JsonWebKeyApi) GetJsonWebKeySet(set string) (*JsonWebKeySet, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/keys/{set}"
+ localVarPath = strings.Replace(localVarPath, "{"+"set"+"}", fmt.Sprintf("%v", set), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(JsonWebKeySet)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetJsonWebKeySet", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Update a JSON Web Key
+ * Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+ *
+ * @param kid The kid of the desired key
+ * @param set The set
+ * @param body
+ * @return *JsonWebKey
+ */
+func (a JsonWebKeyApi) UpdateJsonWebKey(kid string, set string, body JsonWebKey) (*JsonWebKey, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Put")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/keys/{set}/{kid}"
+ localVarPath = strings.Replace(localVarPath, "{"+"kid"+"}", fmt.Sprintf("%v", kid), -1)
+ localVarPath = strings.Replace(localVarPath, "{"+"set"+"}", fmt.Sprintf("%v", set), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(JsonWebKey)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "UpdateJsonWebKey", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Update a JSON Web Key Set
+ * Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+ *
+ * @param set The set
+ * @param body
+ * @return *JsonWebKeySet
+ */
+func (a JsonWebKeyApi) UpdateJsonWebKeySet(set string, body JsonWebKeySet) (*JsonWebKeySet, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Put")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/keys/{set}"
+ localVarPath = strings.Replace(localVarPath, "{"+"set"+"}", fmt.Sprintf("%v", set), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(JsonWebKeySet)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "UpdateJsonWebKeySet", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
diff --git a/sdk/go/hydra/swagger/json_web_key_set.go b/sdk/go/hydra/swagger/json_web_key_set.go
new file mode 100644
index 00000000000..2ac53ed8be6
--- /dev/null
+++ b/sdk/go/hydra/swagger/json_web_key_set.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 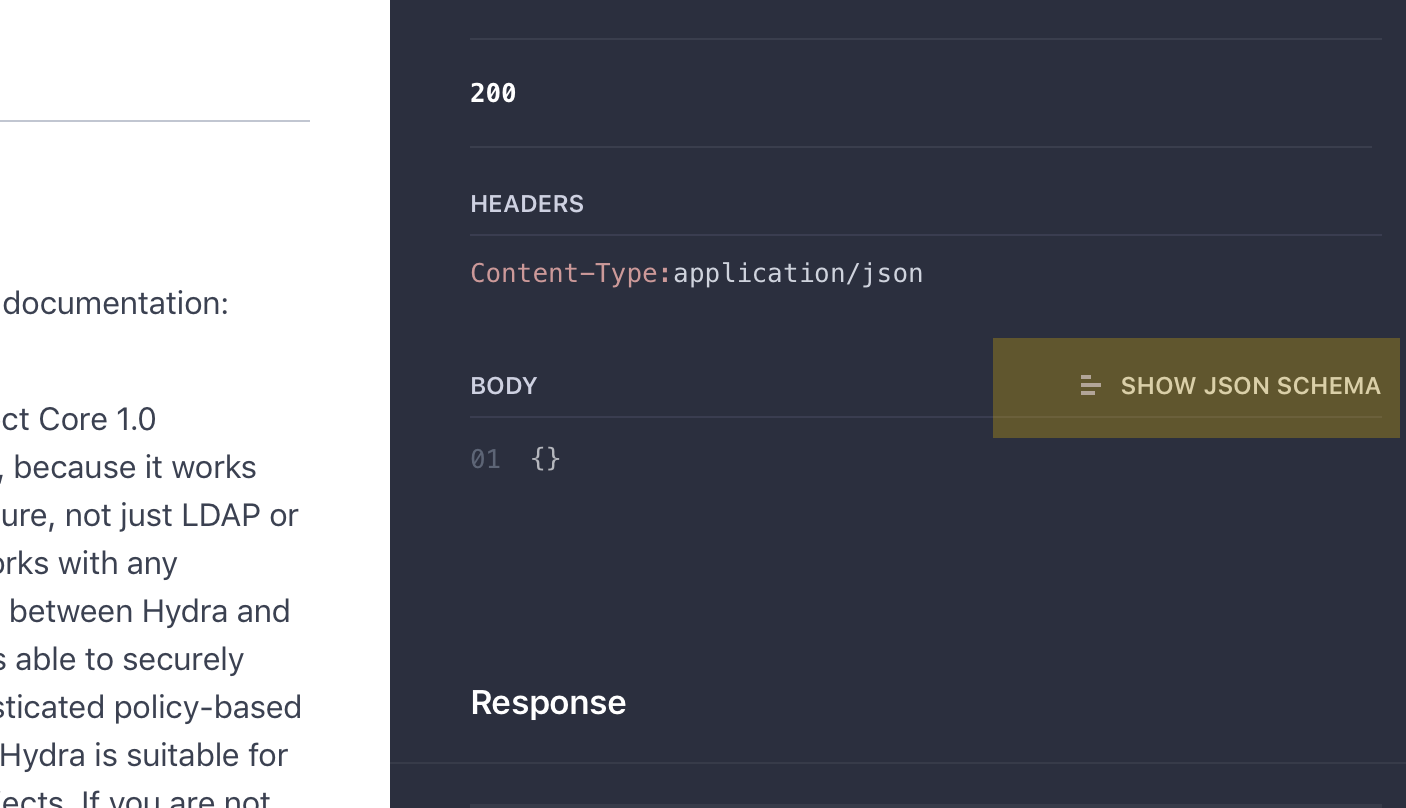 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type JsonWebKeySet struct {
+
+ // The value of the \"keys\" parameter is an array of JWK values. By default, the order of the JWK values within the array does not imply an order of preference among them, although applications of JWK Sets can choose to assign a meaning to the order for their purposes, if desired.
+ Keys []JsonWebKey `json:"keys,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/json_web_key_set_generator_request.go b/sdk/go/hydra/swagger/json_web_key_set_generator_request.go
new file mode 100644
index 00000000000..fcf1ec74d16
--- /dev/null
+++ b/sdk/go/hydra/swagger/json_web_key_set_generator_request.go
@@ -0,0 +1,20 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 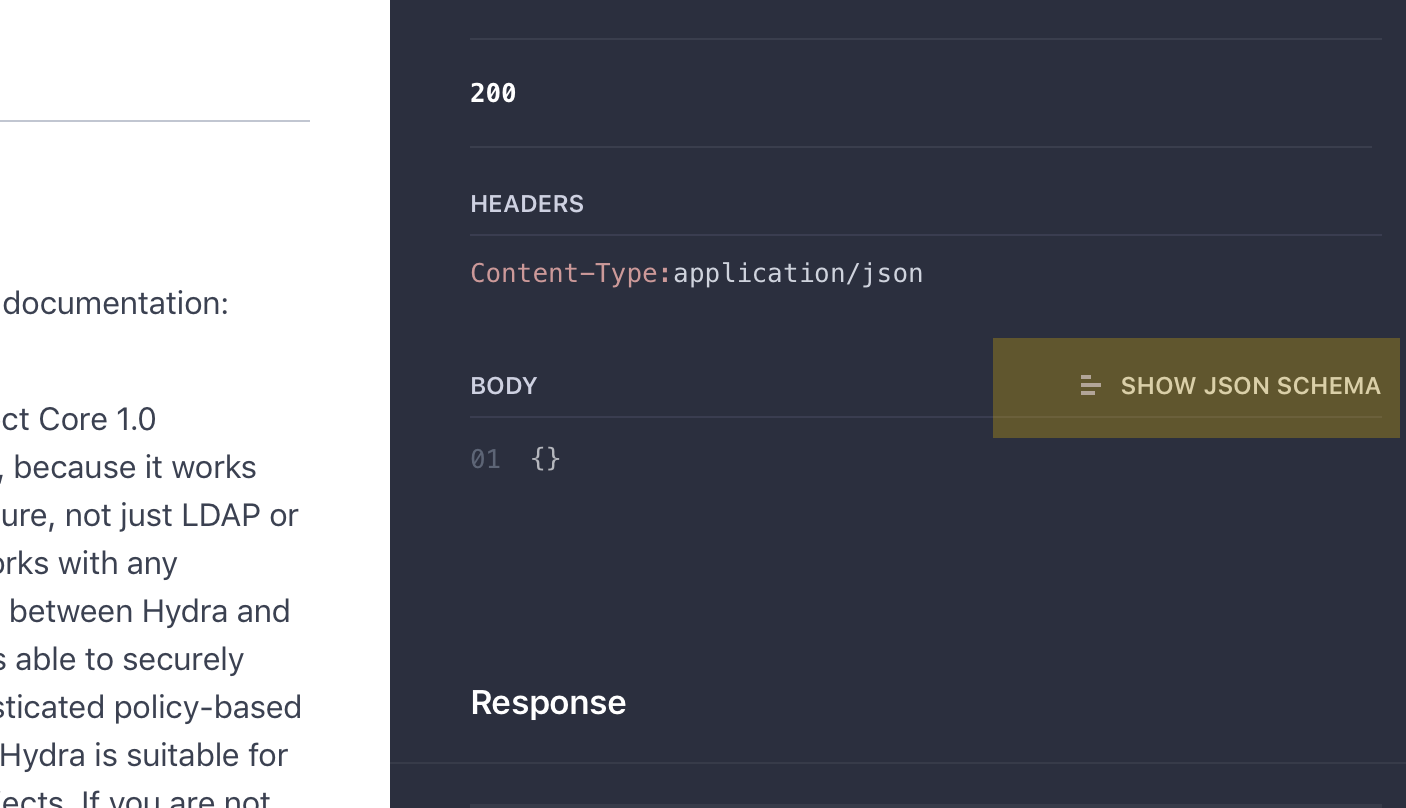 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type JsonWebKeySetGeneratorRequest struct {
+
+ // The algorithm to be used for creating the key. Supports \"RS256\", \"ES521\" and \"HS256\"
+ Alg string `json:"alg"`
+
+ // The kid of the key to be created
+ Kid string `json:"kid"`
+}
diff --git a/sdk/go/hydra/swagger/key_generator.go b/sdk/go/hydra/swagger/key_generator.go
new file mode 100644
index 00000000000..03c11fd5e7f
--- /dev/null
+++ b/sdk/go/hydra/swagger/key_generator.go
@@ -0,0 +1,14 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 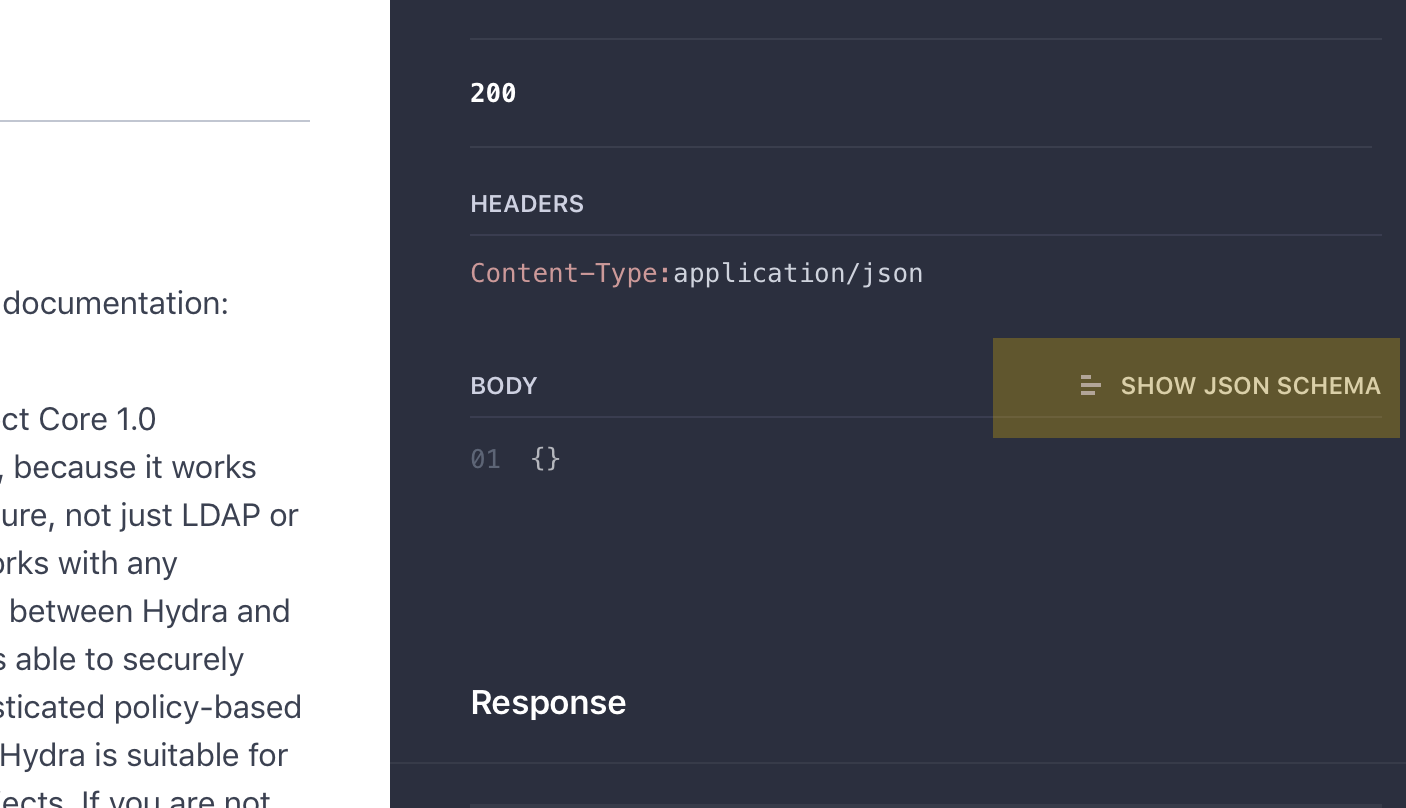 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type KeyGenerator struct {
+}
diff --git a/sdk/go/hydra/swagger/manager.go b/sdk/go/hydra/swagger/manager.go
new file mode 100644
index 00000000000..efb849112ef
--- /dev/null
+++ b/sdk/go/hydra/swagger/manager.go
@@ -0,0 +1,14 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 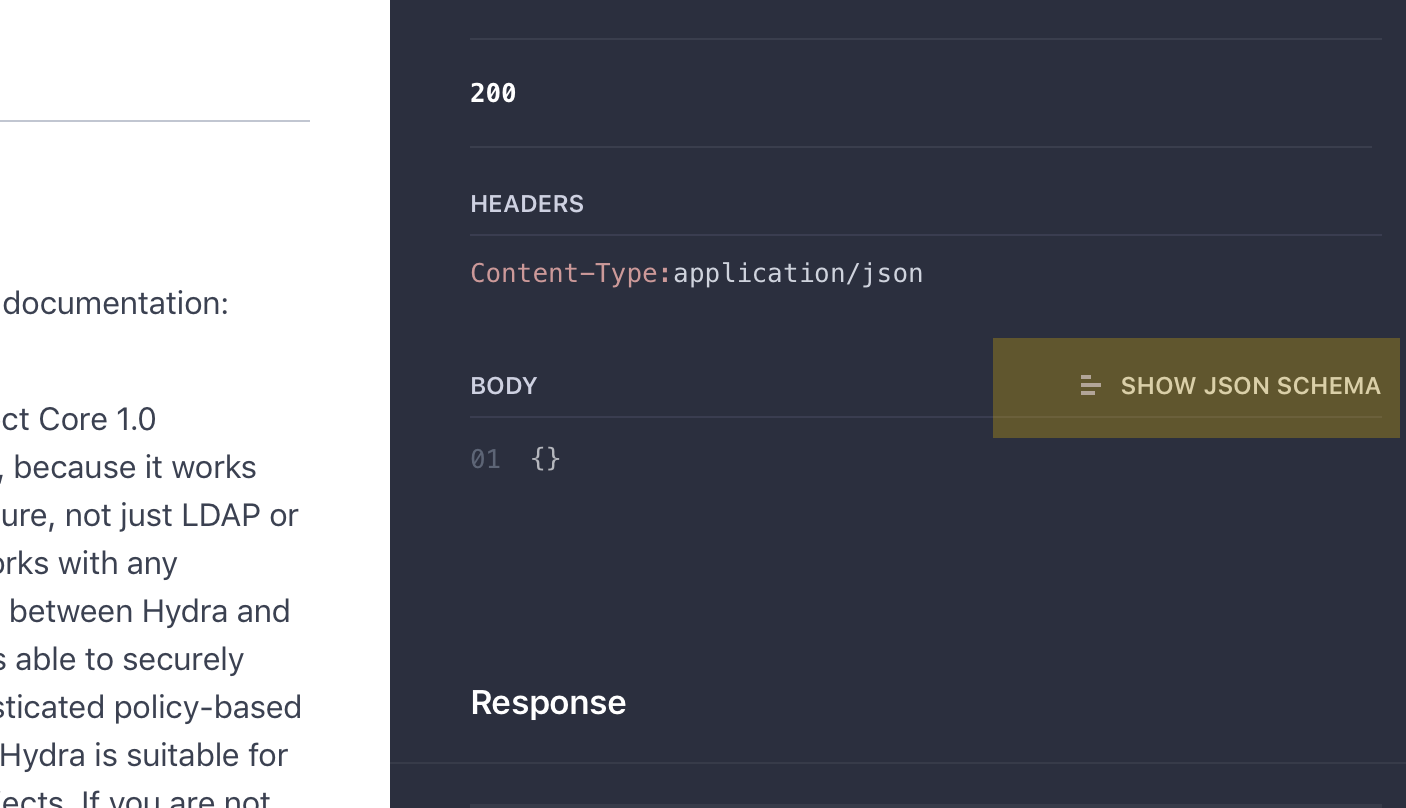 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type Manager struct {
+}
diff --git a/sdk/go/hydra/swagger/o_auth2_api.go b/sdk/go/hydra/swagger/o_auth2_api.go
new file mode 100644
index 00000000000..fe2aab0df88
--- /dev/null
+++ b/sdk/go/hydra/swagger/o_auth2_api.go
@@ -0,0 +1,954 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 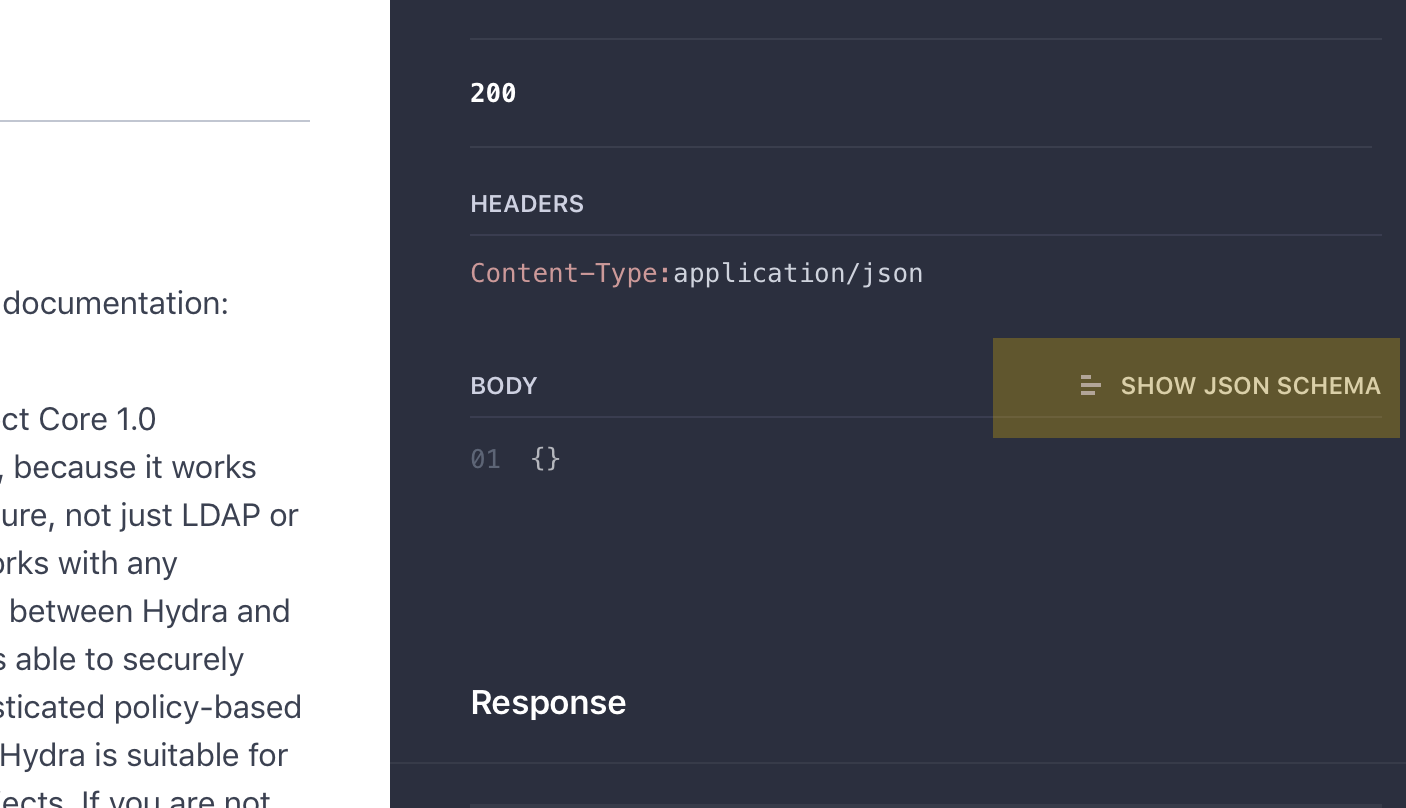 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+import (
+ "encoding/json"
+ "fmt"
+ "net/url"
+ "strings"
+)
+
+type OAuth2Api struct {
+ Configuration *Configuration
+}
+
+func NewOAuth2Api() *OAuth2Api {
+ configuration := NewConfiguration()
+ return &OAuth2Api{
+ Configuration: configuration,
+ }
+}
+
+func NewOAuth2ApiWithBasePath(basePath string) *OAuth2Api {
+ configuration := NewConfiguration()
+ configuration.BasePath = basePath
+
+ return &OAuth2Api{
+ Configuration: configuration,
+ }
+}
+
+/**
+ * Accept a consent request
+ * Call this endpoint to accept a consent request. This usually happens when a user agrees to give access rights to an application. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"accept\"], \"effect\": \"allow\" } ```
+ *
+ * @param id
+ * @param body
+ * @return void
+ */
+func (a OAuth2Api) AcceptOAuth2ConsentRequest(id string, body ConsentRequestAcceptance) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Patch")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/oauth2/consent/requests/{id}/accept"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "AcceptOAuth2ConsentRequest", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Create an OAuth 2.0 client
+ * If you pass `client_secret` the secret will be used, otherwise a random secret will be generated. The secret will be returned in the response and you will not be able to retrieve it later on. Write the secret down and keep it somwhere safe. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"create\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+ *
+ * @param body
+ * @return *OAuth2Client
+ */
+func (a OAuth2Api) CreateOAuth2Client(body OAuth2Client) (*OAuth2Client, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/clients"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(OAuth2Client)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "CreateOAuth2Client", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Deletes an OAuth 2.0 Client
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+ *
+ * @param id The id of the OAuth 2.0 Client.
+ * @return void
+ */
+func (a OAuth2Api) DeleteOAuth2Client(id string) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Delete")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/clients/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "DeleteOAuth2Client", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Retrieve an OAuth 2.0 Client.
+ * This endpoint never returns passwords. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+ *
+ * @param id The id of the OAuth 2.0 Client.
+ * @return *OAuth2Client
+ */
+func (a OAuth2Api) GetOAuth2Client(id string) (*OAuth2Client, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/clients/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(OAuth2Client)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetOAuth2Client", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Receive consent request information
+ * Call this endpoint to receive information on consent requests. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ *
+ * @param id The id of the OAuth 2.0 Consent Request.
+ * @return *OAuth2consentRequest
+ */
+func (a OAuth2Api) GetOAuth2ConsentRequest(id string) (*OAuth2consentRequest, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/oauth2/consent/requests/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(OAuth2consentRequest)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetOAuth2ConsentRequest", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Server well known configuration
+ * The well known endpoint an be used to retrieve information for OpenID Connect clients. We encourage you to not roll your own OpenID Connect client but to use an OpenID Connect client library instead. You can learn more on this flow at https://openid.net/specs/openid-connect-discovery-1_0.html
+ *
+ * @return *WellKnown
+ */
+func (a OAuth2Api) GetWellKnown() (*WellKnown, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/.well-known/openid-configuration"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json", "application/x-www-form-urlencoded"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(WellKnown)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetWellKnown", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Introspect OAuth2 tokens
+ * The introspection endpoint allows to check if a token (both refresh and access) is active or not. An active token is neither expired nor revoked. If a token is active, additional information on the token will be included. You can set additional data for a token by setting `accessTokenExtra` during the consent flow.
+ *
+ * @param token The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation.
+ * @param scope An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false.
+ * @return *OAuth2TokenIntrospection
+ */
+func (a OAuth2Api) IntrospectOAuth2Token(token string, scope string) (*OAuth2TokenIntrospection, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/oauth2/introspect"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(basic)' required
+ // http basic authentication required
+ if a.Configuration.Username != "" || a.Configuration.Password != "" {
+ localVarHeaderParams["Authorization"] = "Basic " + a.Configuration.GetBasicAuthEncodedString()
+ }
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/x-www-form-urlencoded"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarFormParams["token"] = a.Configuration.APIClient.ParameterToString(token, "")
+ localVarFormParams["scope"] = a.Configuration.APIClient.ParameterToString(scope, "")
+ var successPayload = new(OAuth2TokenIntrospection)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "IntrospectOAuth2Token", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * List OAuth 2.0 Clients
+ * This endpoint never returns passwords. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ *
+ * @return []OAuth2Client
+ */
+func (a OAuth2Api) ListOAuth2Clients() ([]OAuth2Client, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/clients"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new([]OAuth2Client)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "ListOAuth2Clients", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return *successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return *successPayload, localVarAPIResponse, err
+}
+
+/**
+ * The OAuth 2.0 authorize endpoint
+ * This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows. OAuth2 is a very popular protocol and a library for your programming language will exists. To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
+ *
+ * @return void
+ */
+func (a OAuth2Api) OauthAuth() (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/oauth2/auth"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/x-www-form-urlencoded"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "OauthAuth", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * The OAuth 2.0 token endpoint
+ * This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows. OAuth2 is a very popular protocol and a library for your programming language will exists. To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
+ *
+ * @return *InlineResponse2001
+ */
+func (a OAuth2Api) OauthToken() (*InlineResponse2001, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/oauth2/token"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(basic)' required
+ // http basic authentication required
+ if a.Configuration.Username != "" || a.Configuration.Password != "" {
+ localVarHeaderParams["Authorization"] = "Basic " + a.Configuration.GetBasicAuthEncodedString()
+ }
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/x-www-form-urlencoded"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(InlineResponse2001)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "OauthToken", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Reject a consent request
+ * Call this endpoint to reject a consent request. This usually happens when a user denies access rights to an application. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"reject\"], \"effect\": \"allow\" } ```
+ *
+ * @param id
+ * @param body
+ * @return void
+ */
+func (a OAuth2Api) RejectOAuth2ConsentRequest(id string, body ConsentRequestRejection) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Patch")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/oauth2/consent/requests/{id}/reject"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "RejectOAuth2ConsentRequest", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Revoke OAuth2 tokens
+ * Revoking a token (both access and refresh) means that the tokens will be invalid. A revoked access token can no longer be used to make access requests, and a revoked refresh token can no longer be used to refresh an access token. Revoking a refresh token also invalidates the access token that was created with it.
+ *
+ * @param token
+ * @return void
+ */
+func (a OAuth2Api) RevokeOAuth2Token(token string) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/oauth2/revoke"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(basic)' required
+ // http basic authentication required
+ if a.Configuration.Username != "" || a.Configuration.Password != "" {
+ localVarHeaderParams["Authorization"] = "Basic " + a.Configuration.GetBasicAuthEncodedString()
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/x-www-form-urlencoded"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarFormParams["token"] = a.Configuration.APIClient.ParameterToString(token, "")
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "RevokeOAuth2Token", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Update an OAuth 2.0 Client
+ * If you pass `client_secret` the secret will be updated and returned via the API. This is the only time you will be able to retrieve the client secret, so write it down and keep it safe. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"update\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+ *
+ * @param id
+ * @param body
+ * @return *OAuth2Client
+ */
+func (a OAuth2Api) UpdateOAuth2Client(id string, body OAuth2Client) (*OAuth2Client, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Put")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/clients/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(OAuth2Client)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "UpdateOAuth2Client", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Get list of well known JSON Web Keys
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:hydra.openid.id-token:public\"], \"actions\": [\"GET\"], \"effect\": \"allow\" } ```
+ *
+ * @return *JsonWebKeySet
+ */
+func (a OAuth2Api) WellKnown() (*JsonWebKeySet, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/.well-known/jwks.json"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(JsonWebKeySet)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "WellKnown", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
diff --git a/sdk/go/hydra/swagger/o_auth2_client.go b/sdk/go/hydra/swagger/o_auth2_client.go
new file mode 100644
index 00000000000..b98591ad3dc
--- /dev/null
+++ b/sdk/go/hydra/swagger/o_auth2_client.go
@@ -0,0 +1,56 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 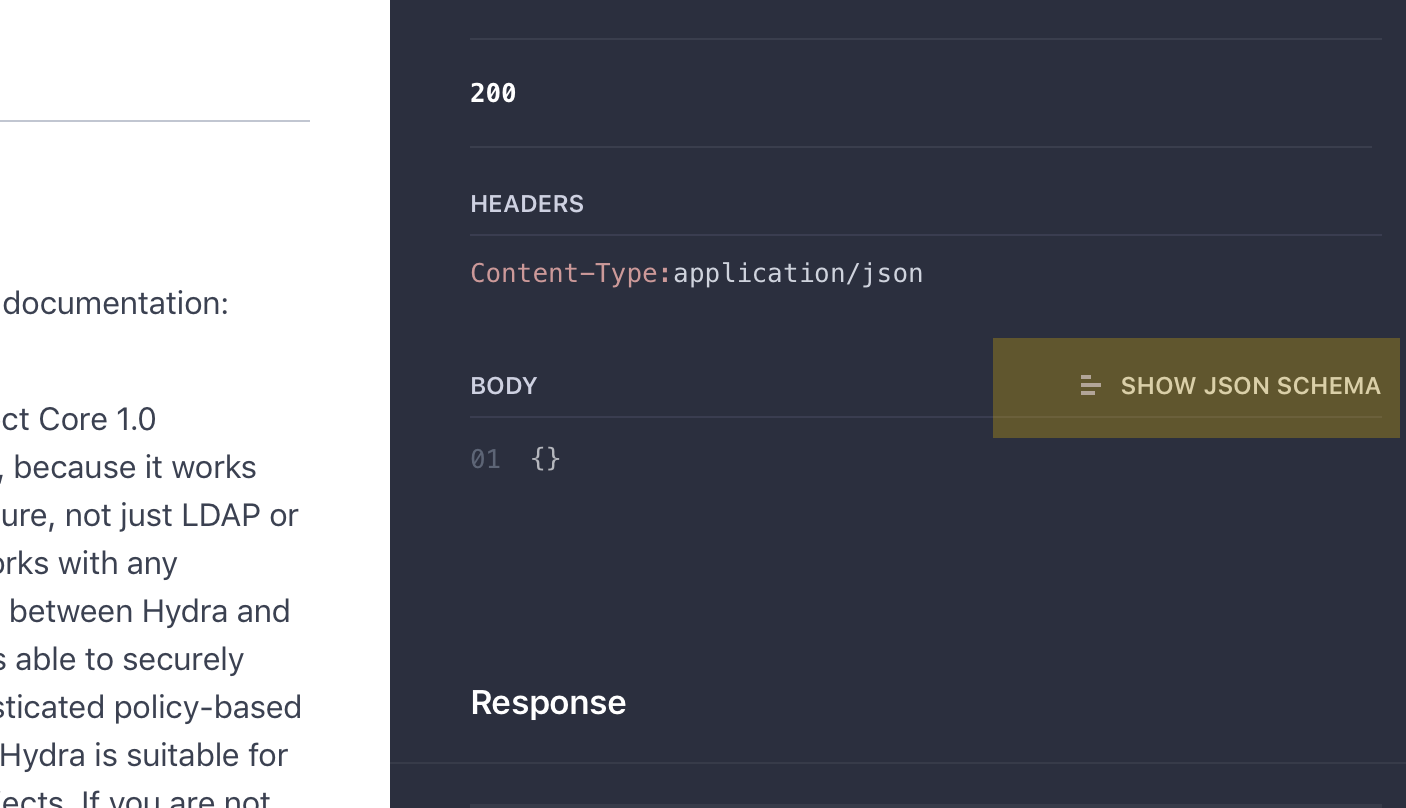 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type OAuth2Client struct {
+
+ // Name is the human-readable string name of the client to be presented to the end-user during authorization.
+ ClientName string `json:"client_name,omitempty"`
+
+ // Secret is the client's secret. The secret will be included in the create request as cleartext, and then never again. The secret is stored using BCrypt so it is impossible to recover it. Tell your users that they need to write the secret down as it will not be made available again.
+ ClientSecret string `json:"client_secret,omitempty"`
+
+ // ClientURI is an URL string of a web page providing information about the client. If present, the server SHOULD display this URL to the end-user in a clickable fashion.
+ ClientUri string `json:"client_uri,omitempty"`
+
+ // Contacts is a array of strings representing ways to contact people responsible for this client, typically email addresses.
+ Contacts []string `json:"contacts,omitempty"`
+
+ // GrantTypes is an array of grant types the client is allowed to use.
+ GrantTypes []string `json:"grant_types,omitempty"`
+
+ // ID is the id for this client.
+ Id string `json:"id,omitempty"`
+
+ // LogoURI is an URL string that references a logo for the client.
+ LogoUri string `json:"logo_uri,omitempty"`
+
+ // Owner is a string identifying the owner of the OAuth 2.0 Client.
+ Owner string `json:"owner,omitempty"`
+
+ // PolicyURI is a URL string that points to a human-readable privacy policy document that describes how the deployment organization collects, uses, retains, and discloses personal data.
+ PolicyUri string `json:"policy_uri,omitempty"`
+
+ // Public is a boolean that identifies this client as public, meaning that it does not have a secret. It will disable the client_credentials grant type for this client if set.
+ Public bool `json:"public,omitempty"`
+
+ // RedirectURIs is an array of allowed redirect urls for the client, for example: http://mydomain/oauth/callback .
+ RedirectUris []string `json:"redirect_uris,omitempty"`
+
+ // ResponseTypes is an array of the OAuth 2.0 response type strings that the client can use at the authorization endpoint.
+ ResponseTypes []string `json:"response_types,omitempty"`
+
+ // Scope is a string containing a space-separated list of scope values (as described in Section 3.3 of OAuth 2.0 [RFC6749]) that the client can use when requesting access tokens.
+ Scope string `json:"scope,omitempty"`
+
+ // TermsOfServiceURI is a URL string that points to a human-readable terms of service document for the client that describes a contractual relationship between the end-user and the client that the end-user accepts when authorizing the client.
+ TosUri string `json:"tos_uri,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/o_auth2_token_introspection.go b/sdk/go/hydra/swagger/o_auth2_token_introspection.go
new file mode 100644
index 00000000000..0eddb5fba86
--- /dev/null
+++ b/sdk/go/hydra/swagger/o_auth2_token_introspection.go
@@ -0,0 +1,47 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 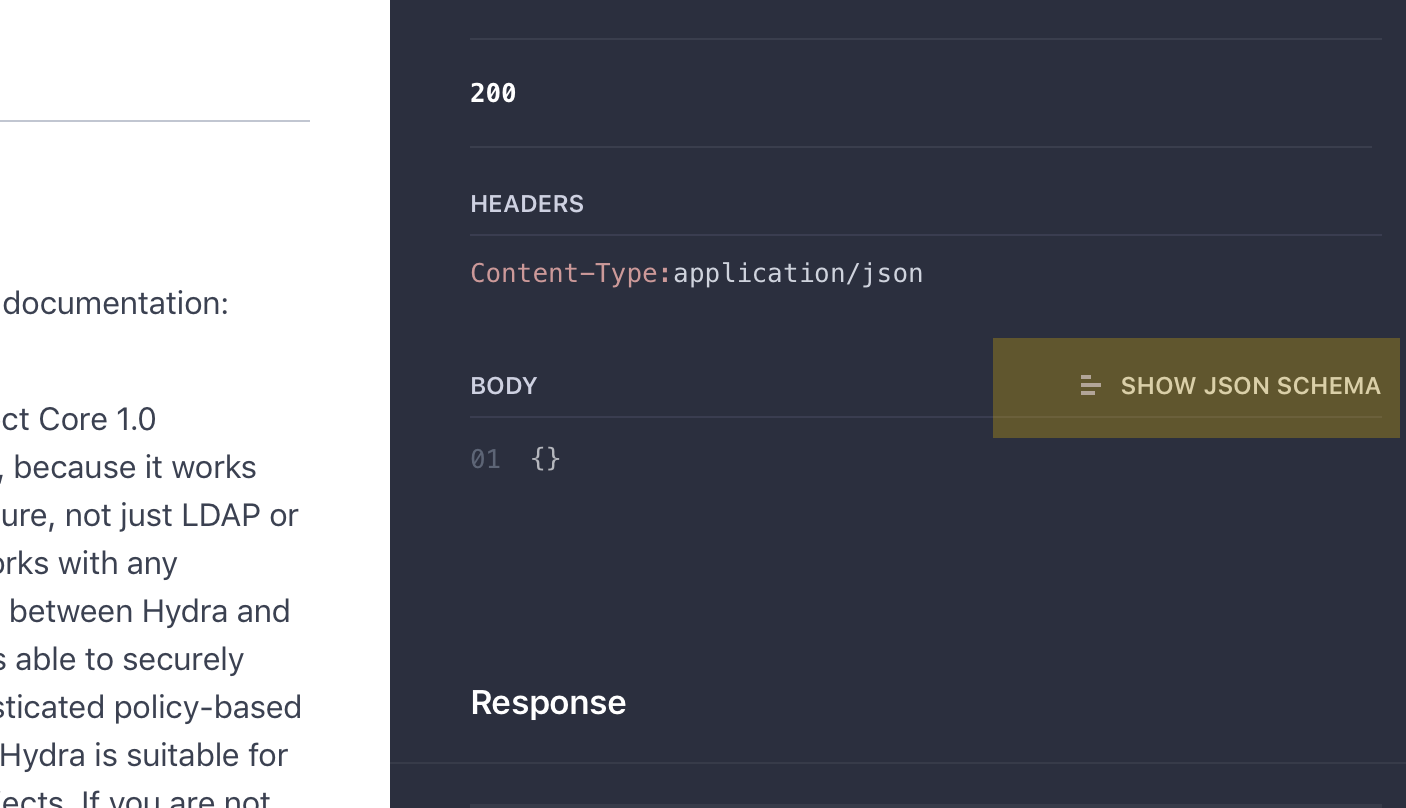 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type OAuth2TokenIntrospection struct {
+
+ // Active is a boolean indicator of whether or not the presented token is currently active. The specifics of a token's \"active\" state will vary depending on the implementation of the authorization server and the information it keeps about its tokens, but a \"true\" value return for the \"active\" property will generally indicate that a given token has been issued by this authorization server, has not been revoked by the resource owner, and is within its given time window of validity (e.g., after its issuance time and before its expiration time).
+ Active bool `json:"active,omitempty"`
+
+ // Audience is a service-specific string identifier or list of string identifiers representing the intended audience for this token.
+ Aud string `json:"aud,omitempty"`
+
+ // ClientID is aclient identifier for the OAuth 2.0 client that requested this token.
+ ClientId string `json:"client_id,omitempty"`
+
+ // Expires at is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token will expire.
+ Exp int64 `json:"exp,omitempty"`
+
+ // Extra is arbitrary data set by the session.
+ Ext map[string]interface{} `json:"ext,omitempty"`
+
+ // Issued at is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token was originally issued.
+ Iat int64 `json:"iat,omitempty"`
+
+ // Issuer is a string representing the issuer of this token
+ Iss string `json:"iss,omitempty"`
+
+ // NotBefore is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token is not to be used before.
+ Nbf int64 `json:"nbf,omitempty"`
+
+ // Scope is a JSON string containing a space-separated list of scopes associated with this token.
+ Scope string `json:"scope,omitempty"`
+
+ // Subject of the token, as defined in JWT [RFC7519]. Usually a machine-readable identifier of the resource owner who authorized this token.
+ Sub string `json:"sub,omitempty"`
+
+ // Username is a human-readable identifier for the resource owner who authorized this token.
+ Username string `json:"username,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/o_auth2consent_request.go b/sdk/go/hydra/swagger/o_auth2consent_request.go
new file mode 100644
index 00000000000..531348d3197
--- /dev/null
+++ b/sdk/go/hydra/swagger/o_auth2consent_request.go
@@ -0,0 +1,26 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 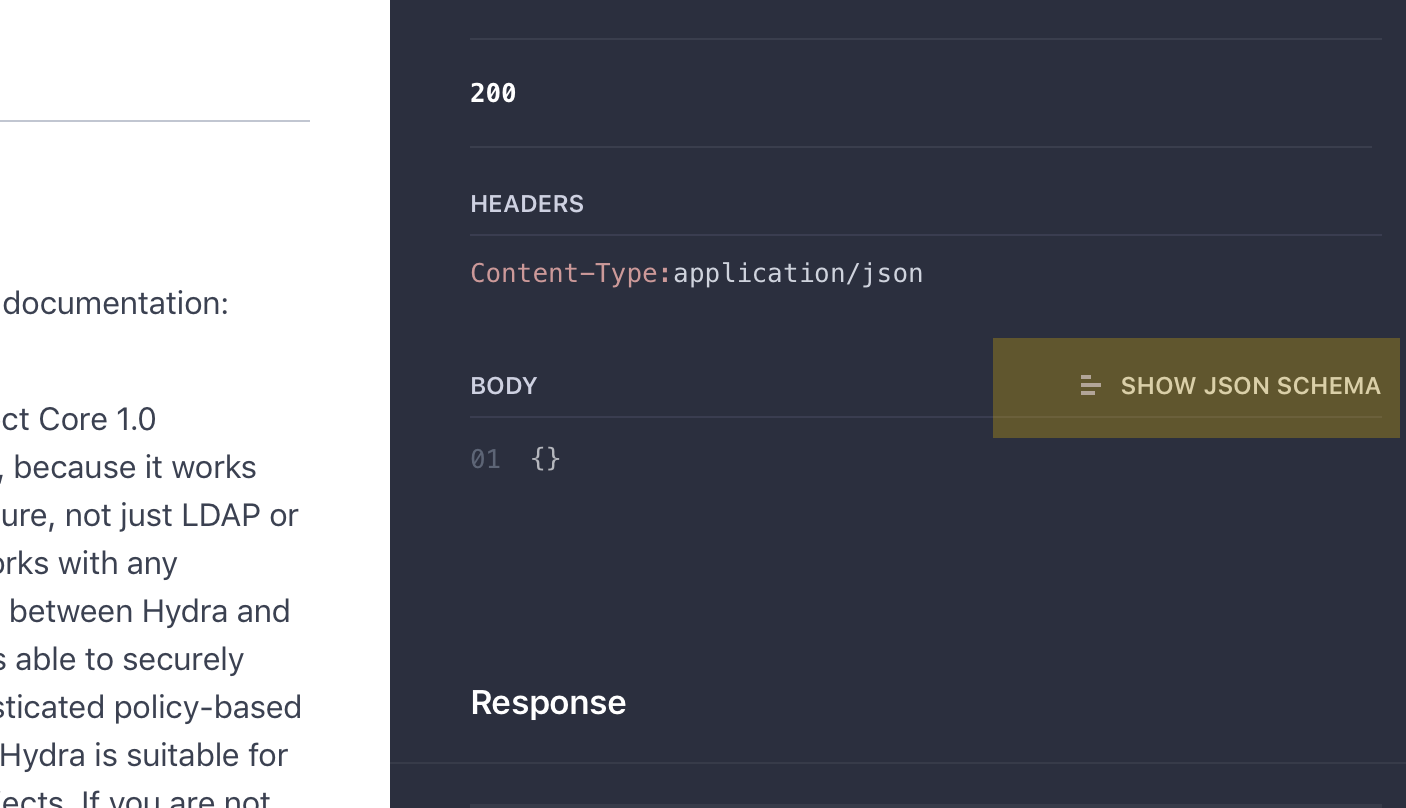 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type OAuth2consentRequest struct {
+
+ // Audience is the client id that initiated the OAuth2 request.
+ Audience string `json:"audience,omitempty"`
+
+ // ID is the id of this consent request.
+ Id string `json:"id,omitempty"`
+
+ // Redirect URL is the URL where the user agent should be redirected to after the consent has been accepted or rejected.
+ RedirectUrl string `json:"redirectUrl,omitempty"`
+
+ // RequestedScopes represents a list of scopes that have been requested by the OAuth2 request initiator.
+ RequestedScopes []string `json:"requestedScopes,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/policy.go b/sdk/go/hydra/swagger/policy.go
new file mode 100644
index 00000000000..531ae721f15
--- /dev/null
+++ b/sdk/go/hydra/swagger/policy.go
@@ -0,0 +1,35 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 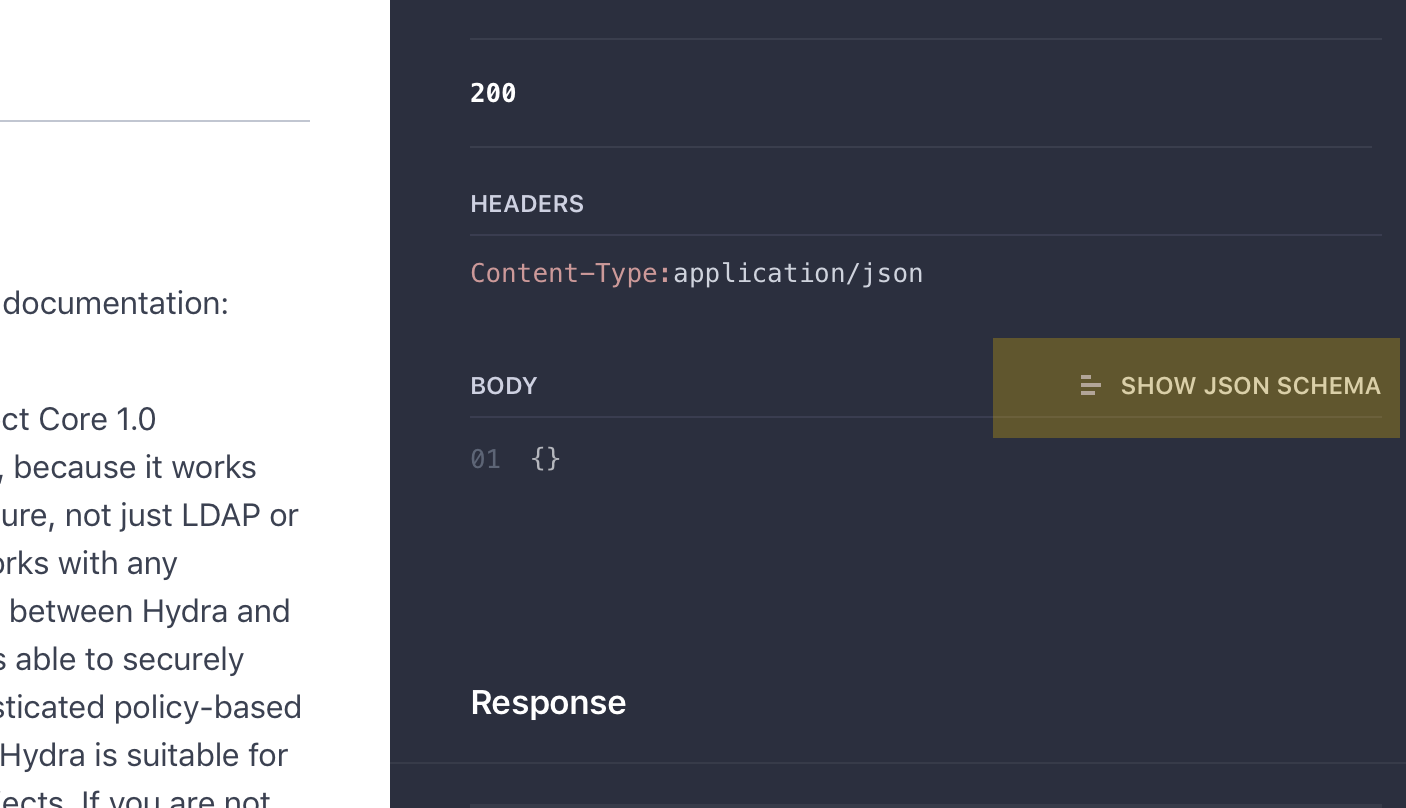 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type Policy struct {
+
+ // Actions impacted by the policy.
+ Actions []string `json:"actions,omitempty"`
+
+ // Conditions under which the policy is active.
+ Conditions map[string]PolicyConditions `json:"conditions,omitempty"`
+
+ // Description of the policy.
+ Description string `json:"description,omitempty"`
+
+ // Effect of the policy
+ Effect string `json:"effect,omitempty"`
+
+ // ID of the policy.
+ Id string `json:"id,omitempty"`
+
+ // Resources impacted by the policy.
+ Resources []string `json:"resources,omitempty"`
+
+ // Subjects impacted by the policy.
+ Subjects []string `json:"subjects,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/policy_api.go b/sdk/go/hydra/swagger/policy_api.go
new file mode 100644
index 00000000000..3f607298ee1
--- /dev/null
+++ b/sdk/go/hydra/swagger/policy_api.go
@@ -0,0 +1,372 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 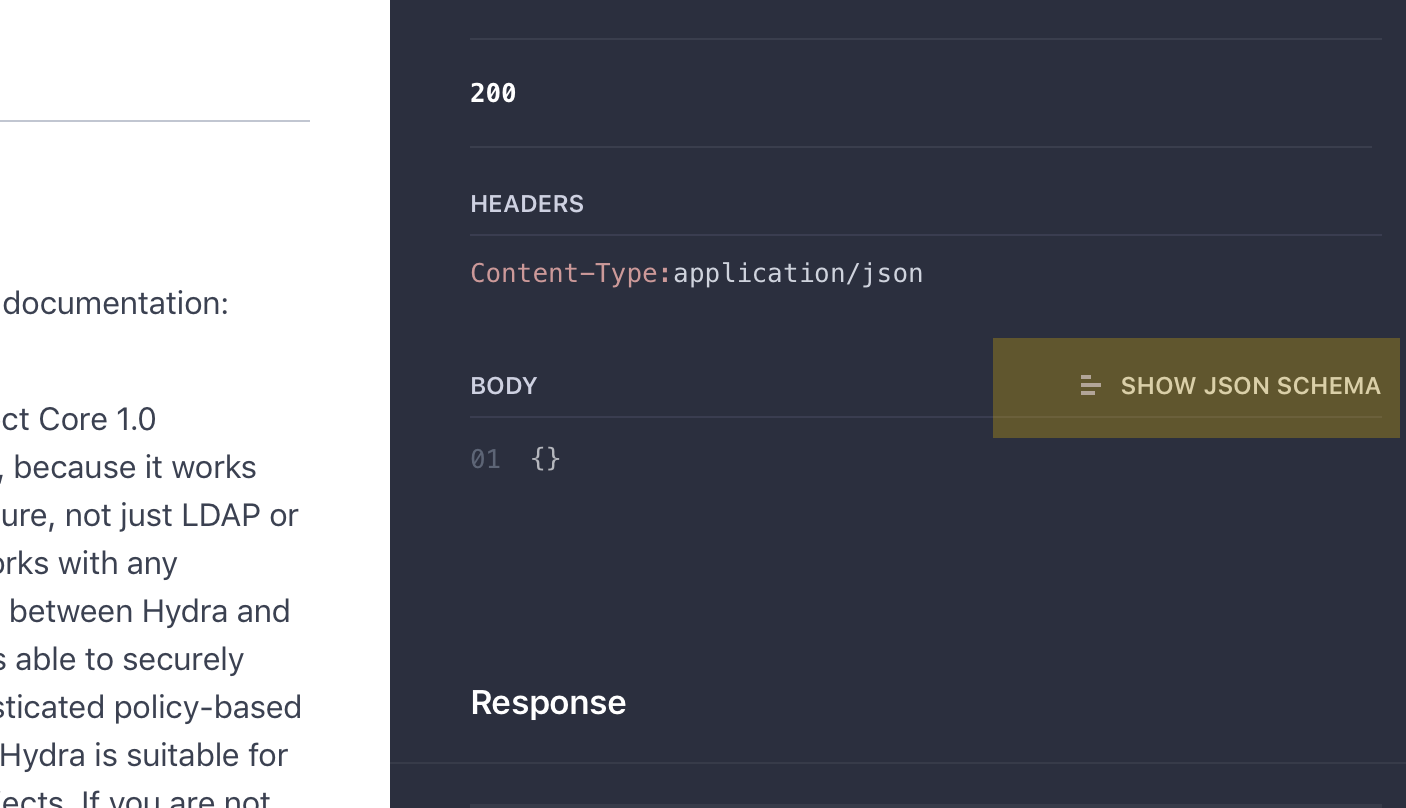 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+import (
+ "encoding/json"
+ "fmt"
+ "net/url"
+ "strings"
+)
+
+type PolicyApi struct {
+ Configuration *Configuration
+}
+
+func NewPolicyApi() *PolicyApi {
+ configuration := NewConfiguration()
+ return &PolicyApi{
+ Configuration: configuration,
+ }
+}
+
+func NewPolicyApiWithBasePath(basePath string) *PolicyApi {
+ configuration := NewConfiguration()
+ configuration.BasePath = basePath
+
+ return &PolicyApi{
+ Configuration: configuration,
+ }
+}
+
+/**
+ * Create an Access Control Policy
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+ *
+ * @param body
+ * @return *Policy
+ */
+func (a PolicyApi) CreatePolicy(body Policy) (*Policy, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/policies"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(Policy)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "CreatePolicy", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Delete an Access Control Policy
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies:<id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+ *
+ * @param id The id of the policy.
+ * @return void
+ */
+func (a PolicyApi) DeletePolicy(id string) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Delete")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/policies/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "DeletePolicy", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Get an Access Control Policy
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies:<id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ *
+ * @param id The id of the policy.
+ * @return *Policy
+ */
+func (a PolicyApi) GetPolicy(id string) (*Policy, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/policies/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(Policy)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetPolicy", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * List Access Control Policies
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"list\"], \"effect\": \"allow\" } ```
+ *
+ * @param offset The offset from where to start looking.
+ * @param limit The maximum amount of policies returned.
+ * @return []Policy
+ */
+func (a PolicyApi) ListPolicies(offset int64, limit int64) ([]Policy, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/policies"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+ localVarQueryParams.Add("offset", a.Configuration.APIClient.ParameterToString(offset, ""))
+ localVarQueryParams.Add("limit", a.Configuration.APIClient.ParameterToString(limit, ""))
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new([]Policy)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "ListPolicies", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return *successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return *successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Update an Access Control Polic
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+ *
+ * @param id The id of the policy.
+ * @param body
+ * @return *Policy
+ */
+func (a PolicyApi) UpdatePolicy(id string, body Policy) (*Policy, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Put")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/policies/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(Policy)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "UpdatePolicy", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
diff --git a/sdk/go/hydra/swagger/policy_conditions.go b/sdk/go/hydra/swagger/policy_conditions.go
new file mode 100644
index 00000000000..21fc8f4b638
--- /dev/null
+++ b/sdk/go/hydra/swagger/policy_conditions.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 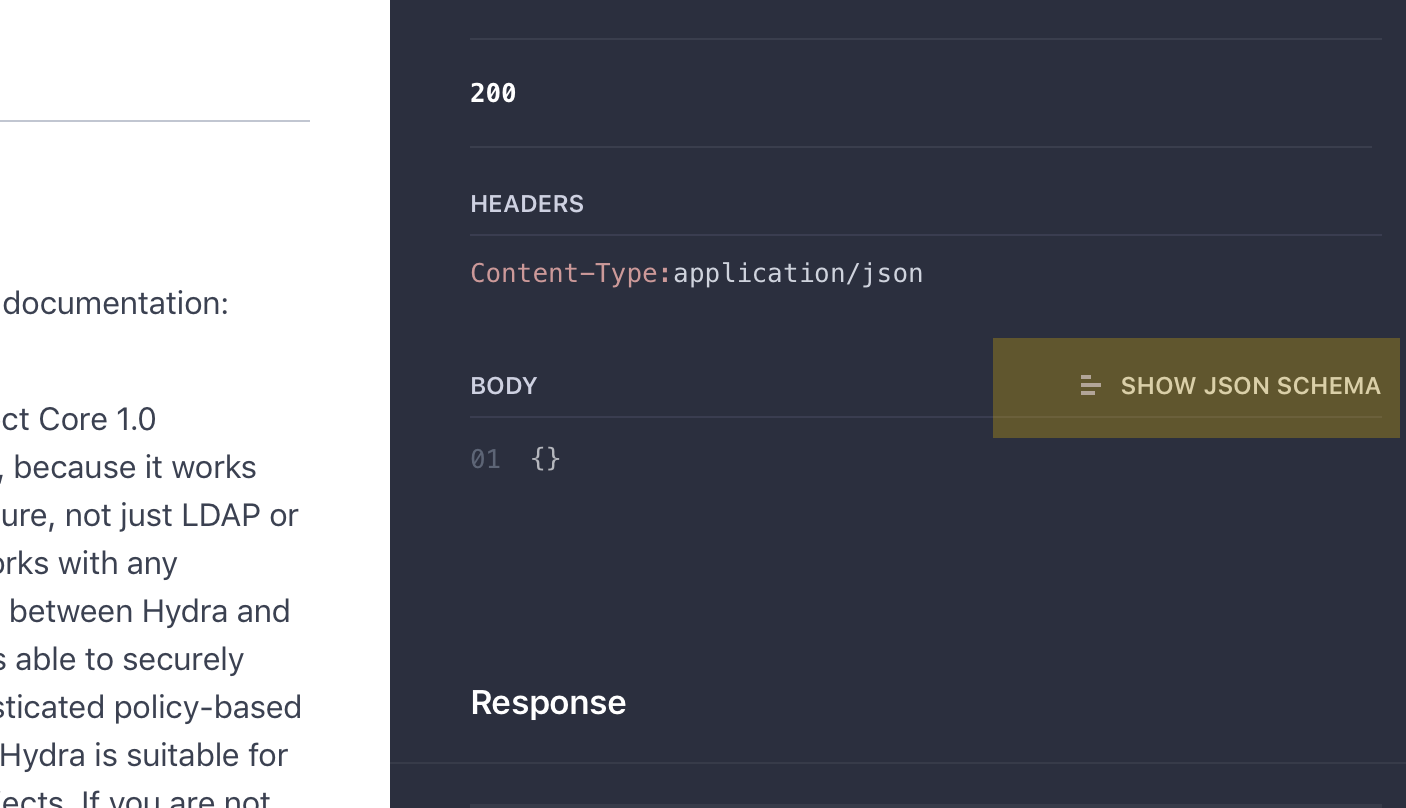 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type PolicyConditions struct {
+ Options map[string]interface{} `json:"options,omitempty"`
+
+ Type_ string `json:"type,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/raw_message.go b/sdk/go/hydra/swagger/raw_message.go
new file mode 100644
index 00000000000..eada781c39e
--- /dev/null
+++ b/sdk/go/hydra/swagger/raw_message.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 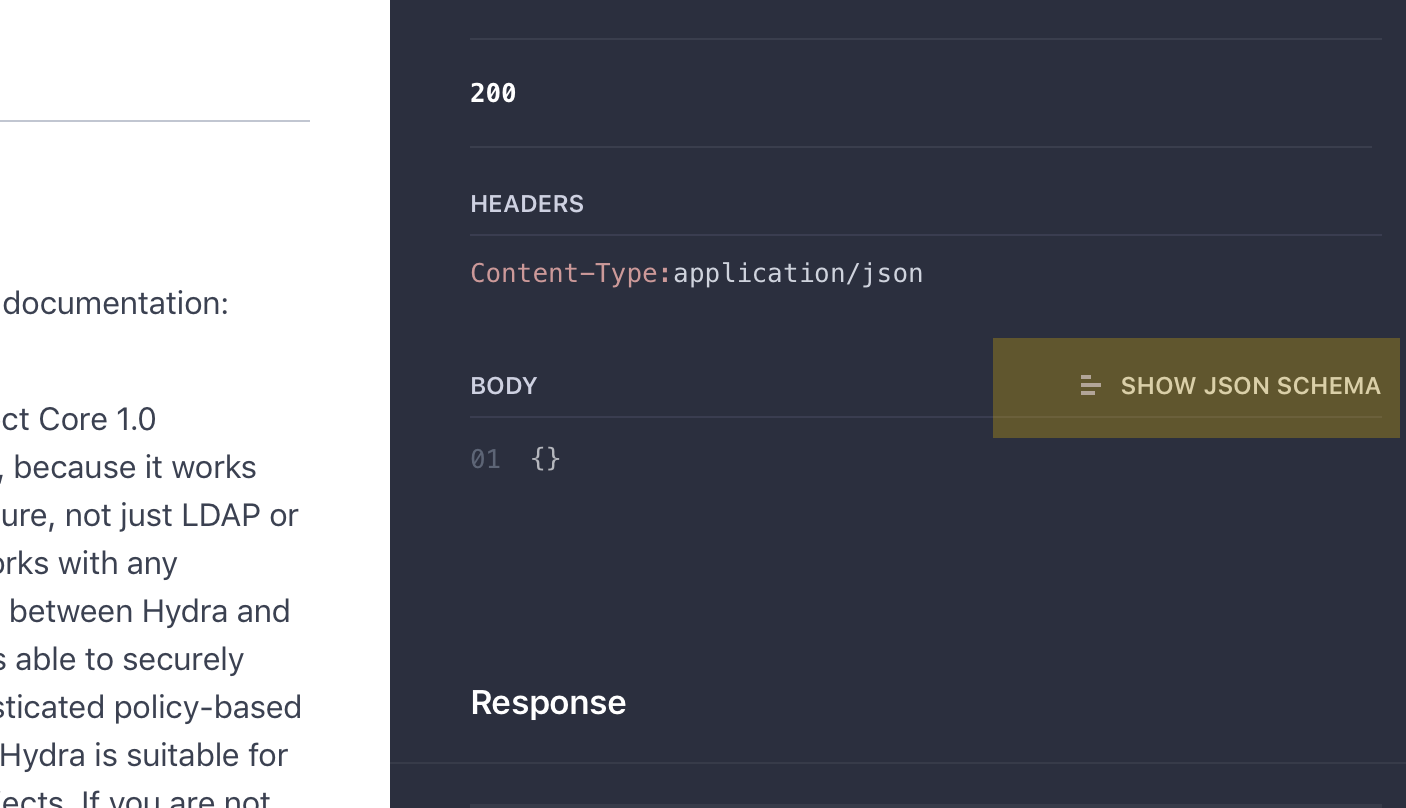 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// It implements Marshaler and Unmarshaler and can be used to delay JSON decoding or precompute a JSON encoding.
+type RawMessage struct {
+}
diff --git a/sdk/go/hydra/swagger/swagger_accept_consent_request.go b/sdk/go/hydra/swagger/swagger_accept_consent_request.go
new file mode 100644
index 00000000000..ab69c5440f5
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_accept_consent_request.go
@@ -0,0 +1,18 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 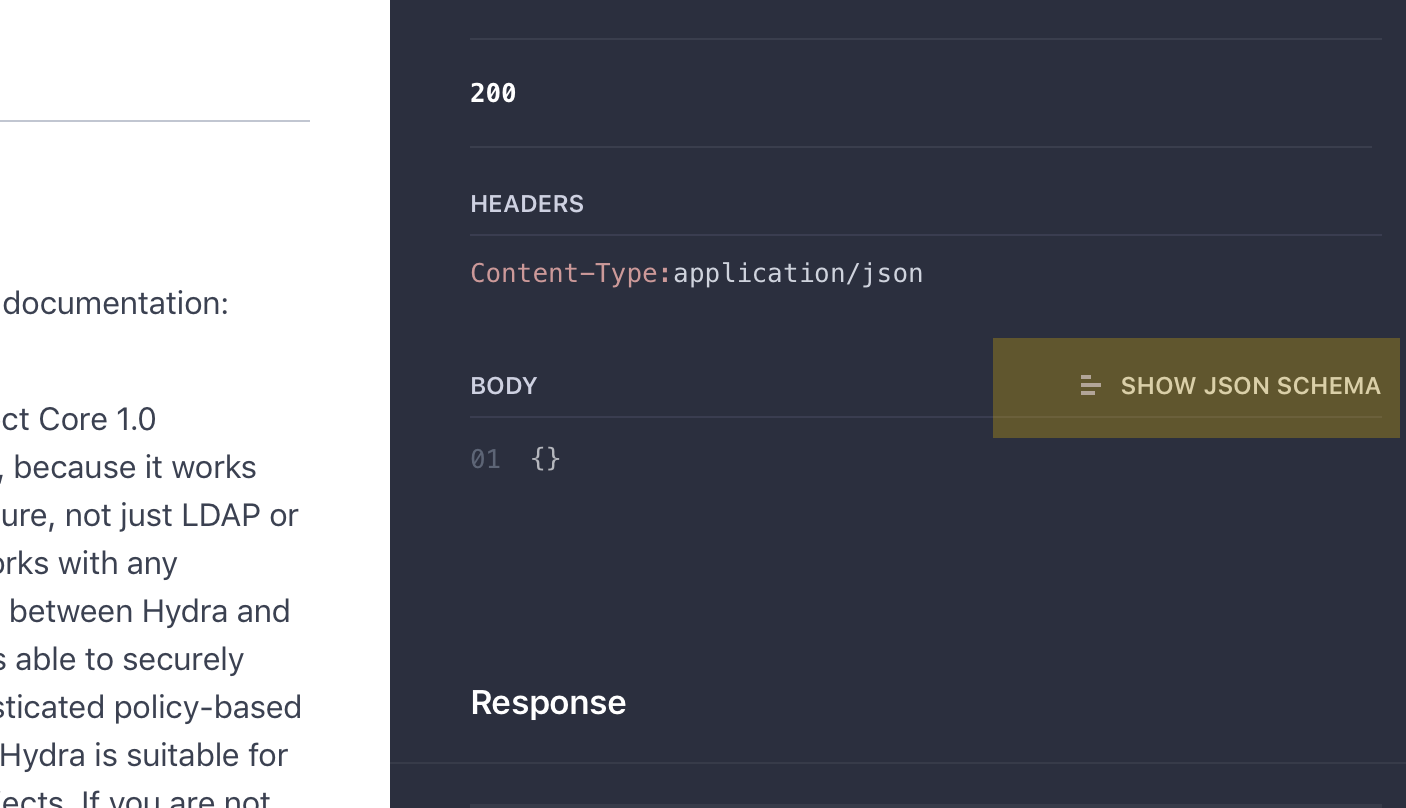 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerAcceptConsentRequest struct {
+ Body ConsentRequestAcceptance `json:"Body"`
+
+ // in: path
+ Id string `json:"id"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_create_policy_parameters.go b/sdk/go/hydra/swagger/swagger_create_policy_parameters.go
new file mode 100644
index 00000000000..0cec8bc44b9
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_create_policy_parameters.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 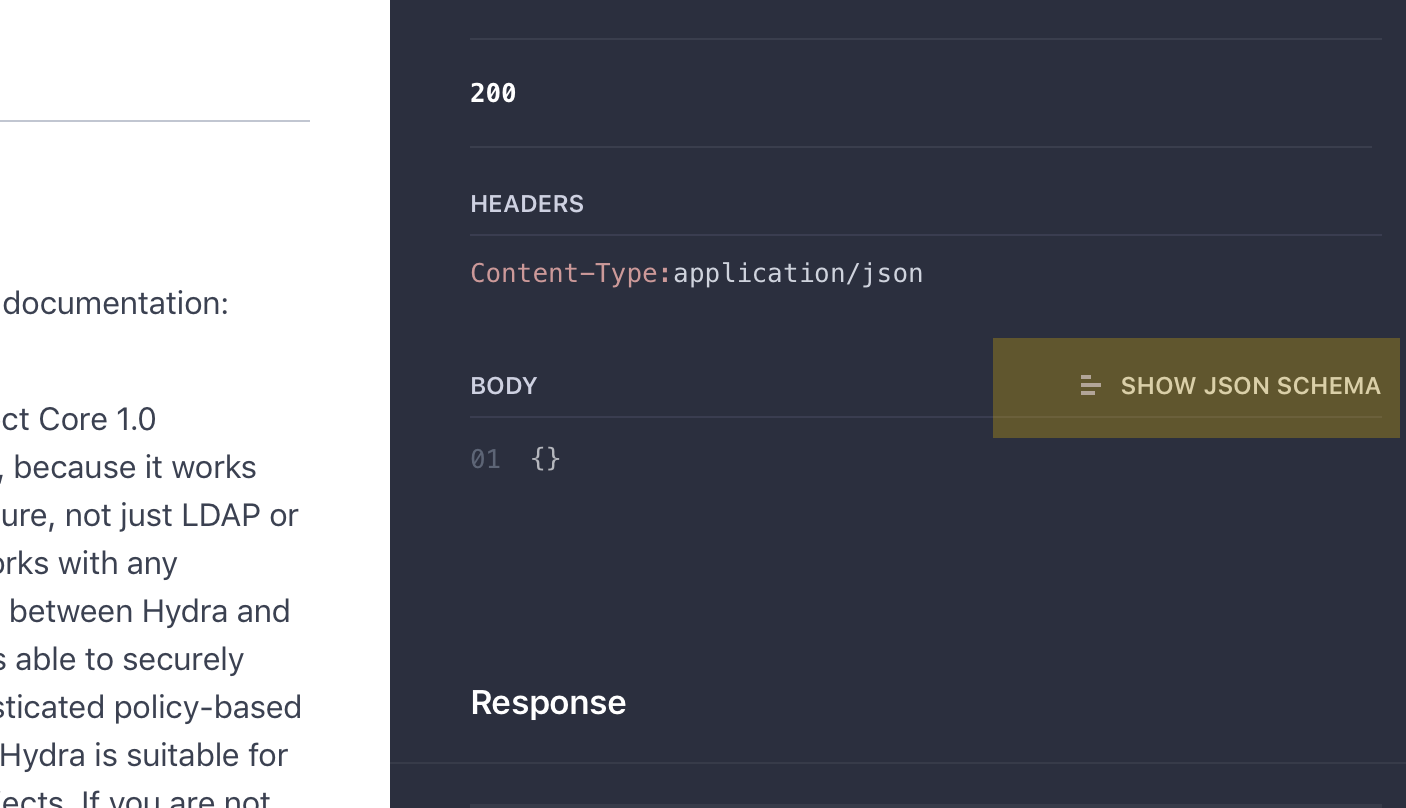 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerCreatePolicyParameters struct {
+ Body Policy `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_does_warden_allow_access_request_parameters.go b/sdk/go/hydra/swagger/swagger_does_warden_allow_access_request_parameters.go
new file mode 100644
index 00000000000..a73ff1fcb09
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_does_warden_allow_access_request_parameters.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 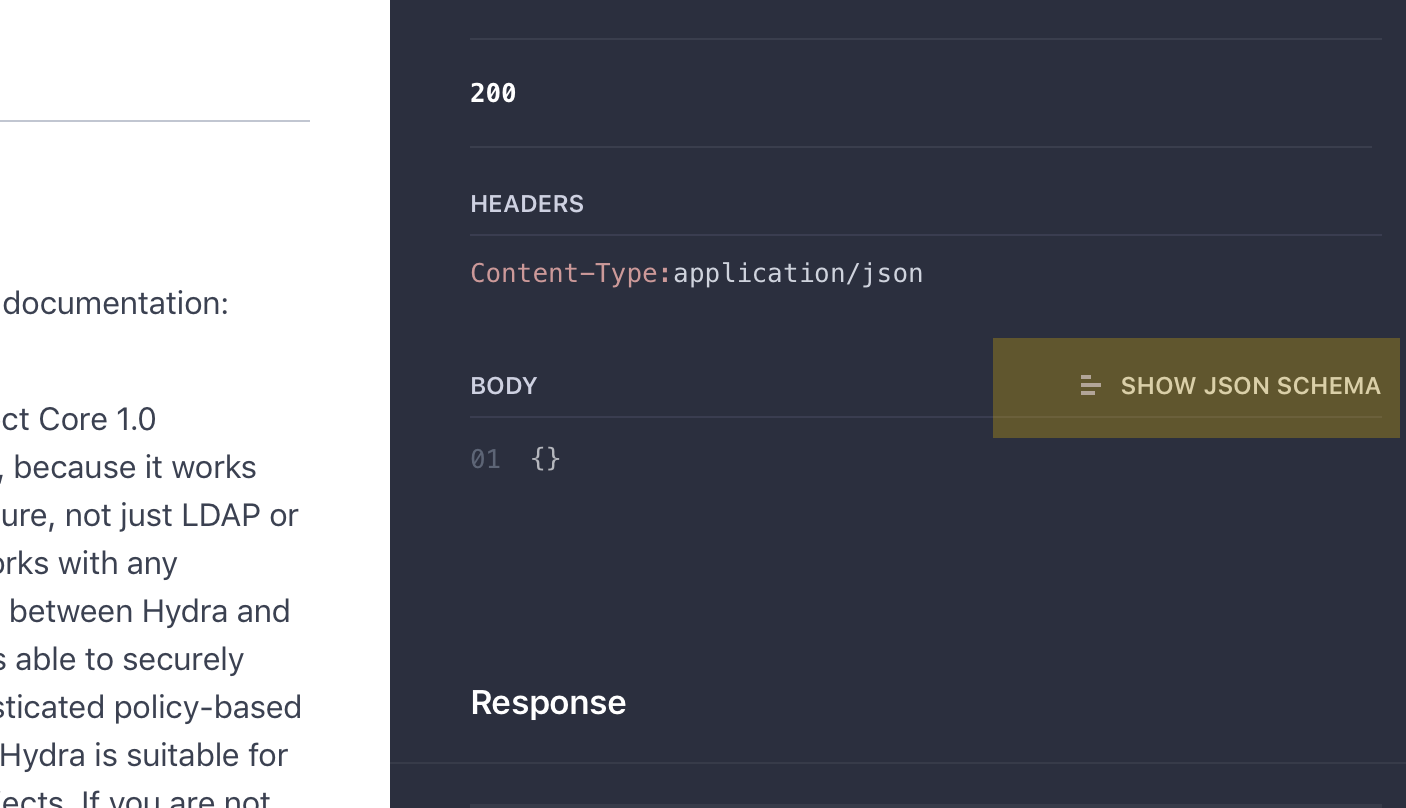 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerDoesWardenAllowAccessRequestParameters struct {
+ Body WardenAccessRequest `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_does_warden_allow_token_access_request_parameters.go b/sdk/go/hydra/swagger/swagger_does_warden_allow_token_access_request_parameters.go
new file mode 100644
index 00000000000..22acce8a1bf
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_does_warden_allow_token_access_request_parameters.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 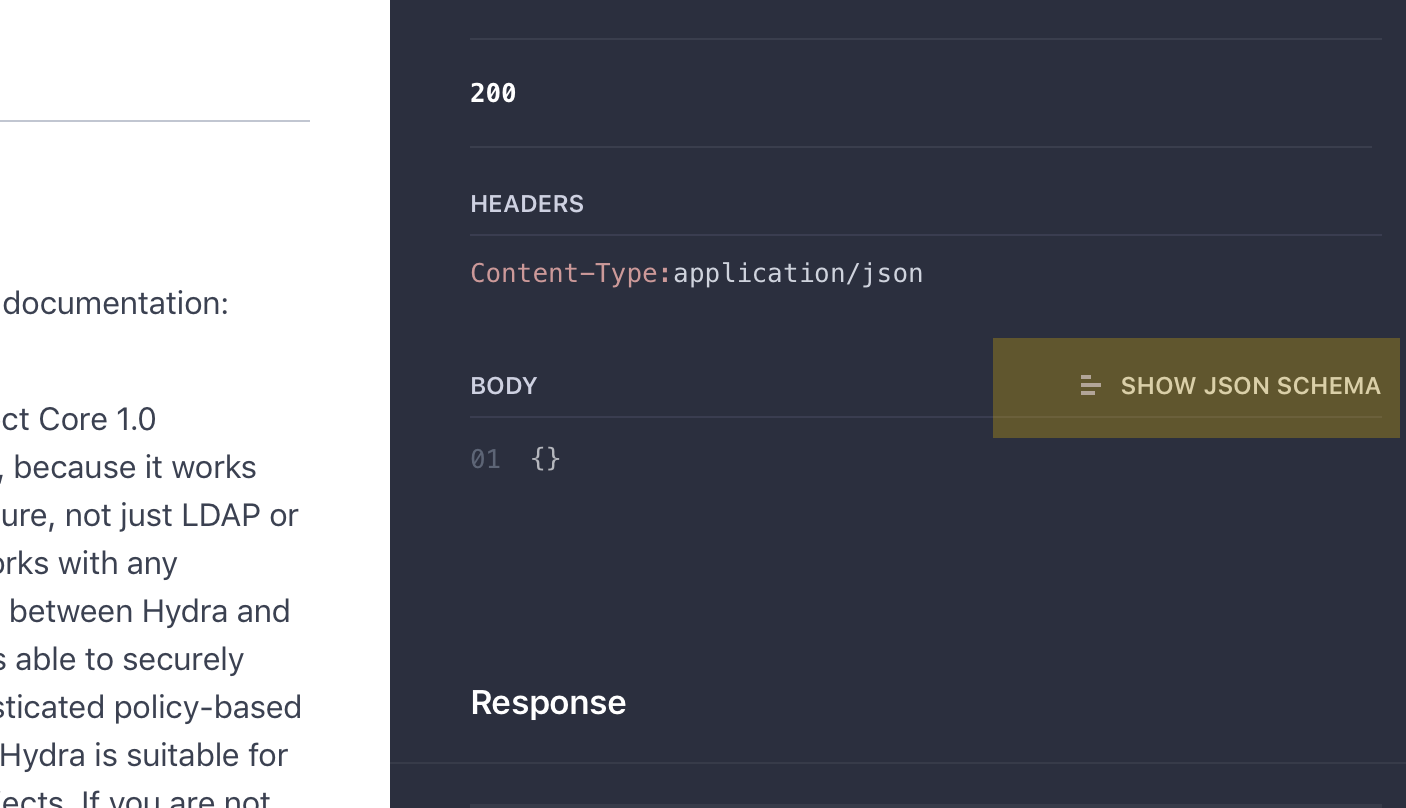 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerDoesWardenAllowTokenAccessRequestParameters struct {
+ Body WardenTokenAccessRequest `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_get_policy_parameters.go b/sdk/go/hydra/swagger/swagger_get_policy_parameters.go
new file mode 100644
index 00000000000..f6a10c24031
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_get_policy_parameters.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 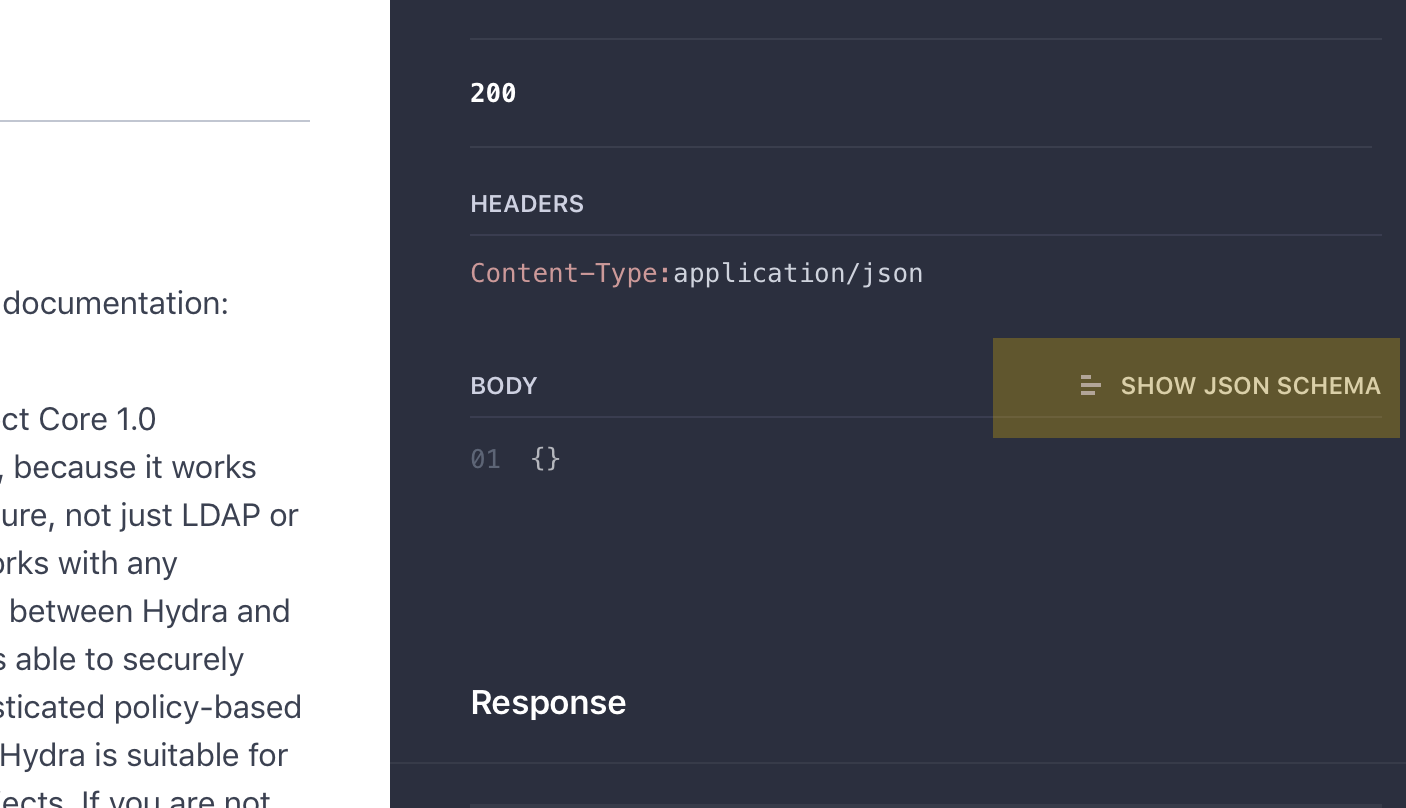 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerGetPolicyParameters struct {
+
+ // The id of the policy. in: path
+ Id string `json:"id,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_json_web_key_query.go b/sdk/go/hydra/swagger/swagger_json_web_key_query.go
new file mode 100644
index 00000000000..a557deb990d
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_json_web_key_query.go
@@ -0,0 +1,20 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 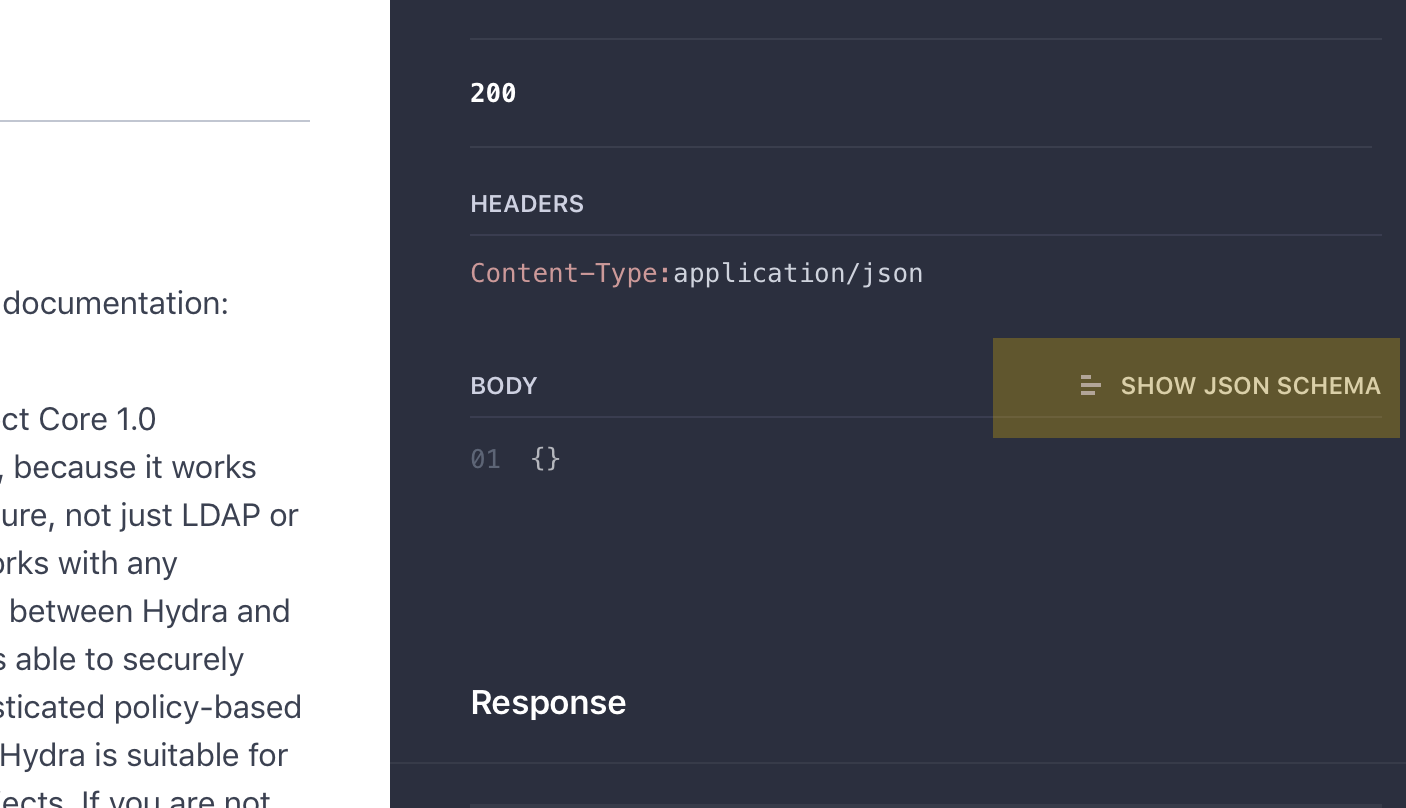 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerJsonWebKeyQuery struct {
+
+ // The kid of the desired key in: path
+ Kid string `json:"kid"`
+
+ // The set in: path
+ Set string `json:"set"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_jwk_create_set.go b/sdk/go/hydra/swagger/swagger_jwk_create_set.go
new file mode 100644
index 00000000000..70890436532
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_jwk_create_set.go
@@ -0,0 +1,18 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 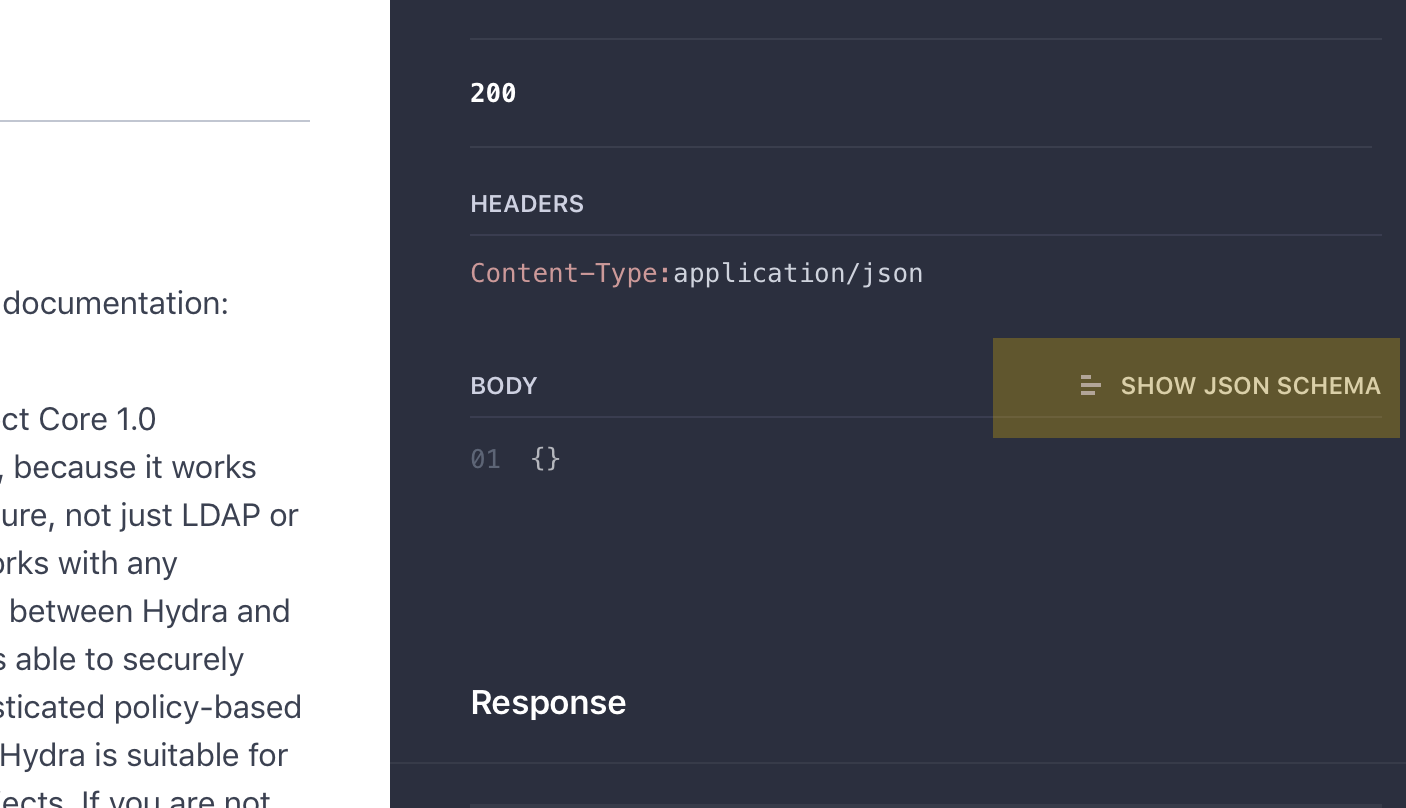 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerJwkCreateSet struct {
+ Body JsonWebKeySetGeneratorRequest `json:"Body,omitempty"`
+
+ // The set in: path
+ Set string `json:"set"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_jwk_set_query.go b/sdk/go/hydra/swagger/swagger_jwk_set_query.go
new file mode 100644
index 00000000000..b9cfa5ccf64
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_jwk_set_query.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 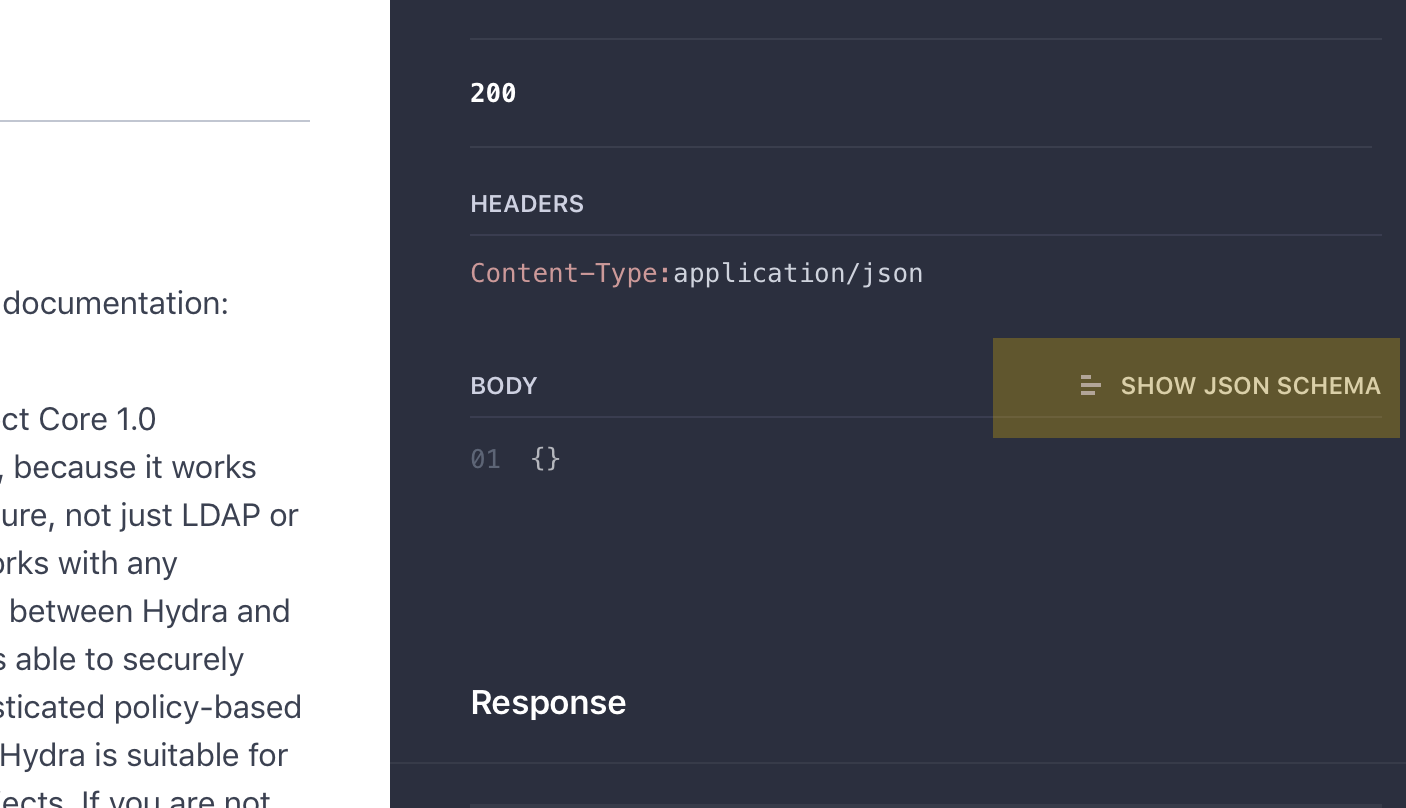 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerJwkSetQuery struct {
+
+ // The set in: path
+ Set string `json:"set"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_jwk_update_set.go b/sdk/go/hydra/swagger/swagger_jwk_update_set.go
new file mode 100644
index 00000000000..753e5c917be
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_jwk_update_set.go
@@ -0,0 +1,18 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 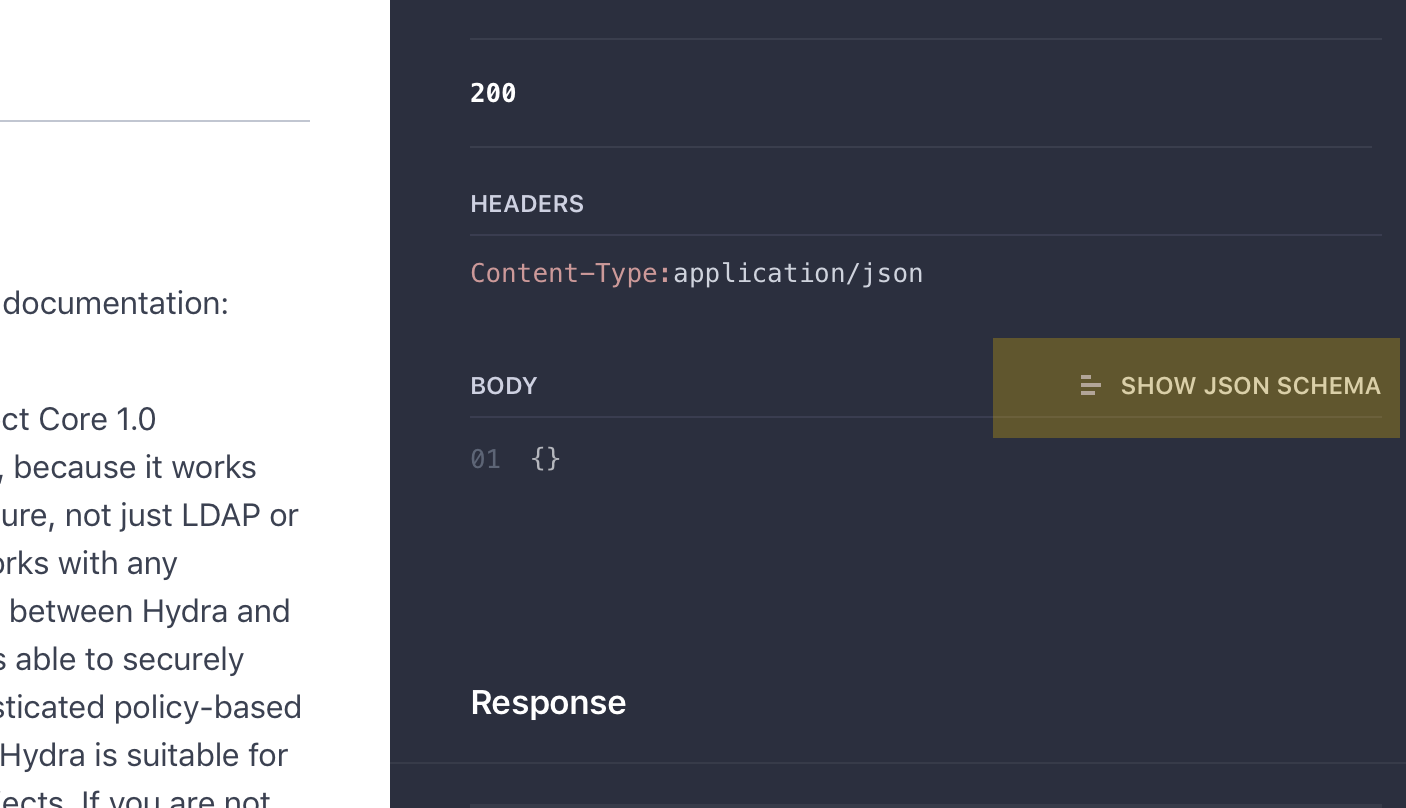 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerJwkUpdateSet struct {
+ Body JsonWebKeySet `json:"Body,omitempty"`
+
+ // The set in: path
+ Set string `json:"set"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_jwk_update_set_key.go b/sdk/go/hydra/swagger/swagger_jwk_update_set_key.go
new file mode 100644
index 00000000000..8189aa09d6b
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_jwk_update_set_key.go
@@ -0,0 +1,21 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 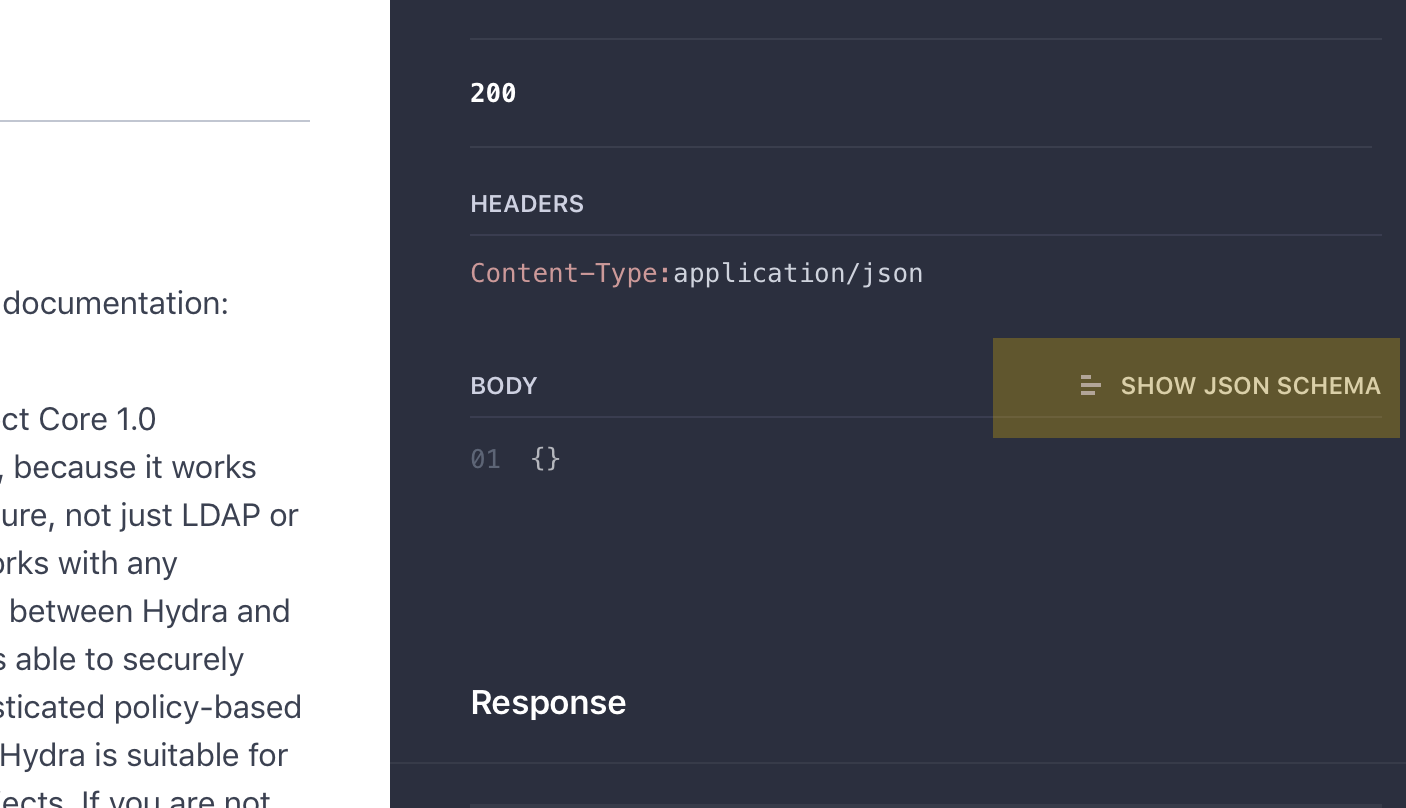 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerJwkUpdateSetKey struct {
+ Body JsonWebKey `json:"Body,omitempty"`
+
+ // The kid of the desired key in: path
+ Kid string `json:"kid"`
+
+ // The set in: path
+ Set string `json:"set"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_list_policy_parameters.go b/sdk/go/hydra/swagger/swagger_list_policy_parameters.go
new file mode 100644
index 00000000000..ef4b81de561
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_list_policy_parameters.go
@@ -0,0 +1,20 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 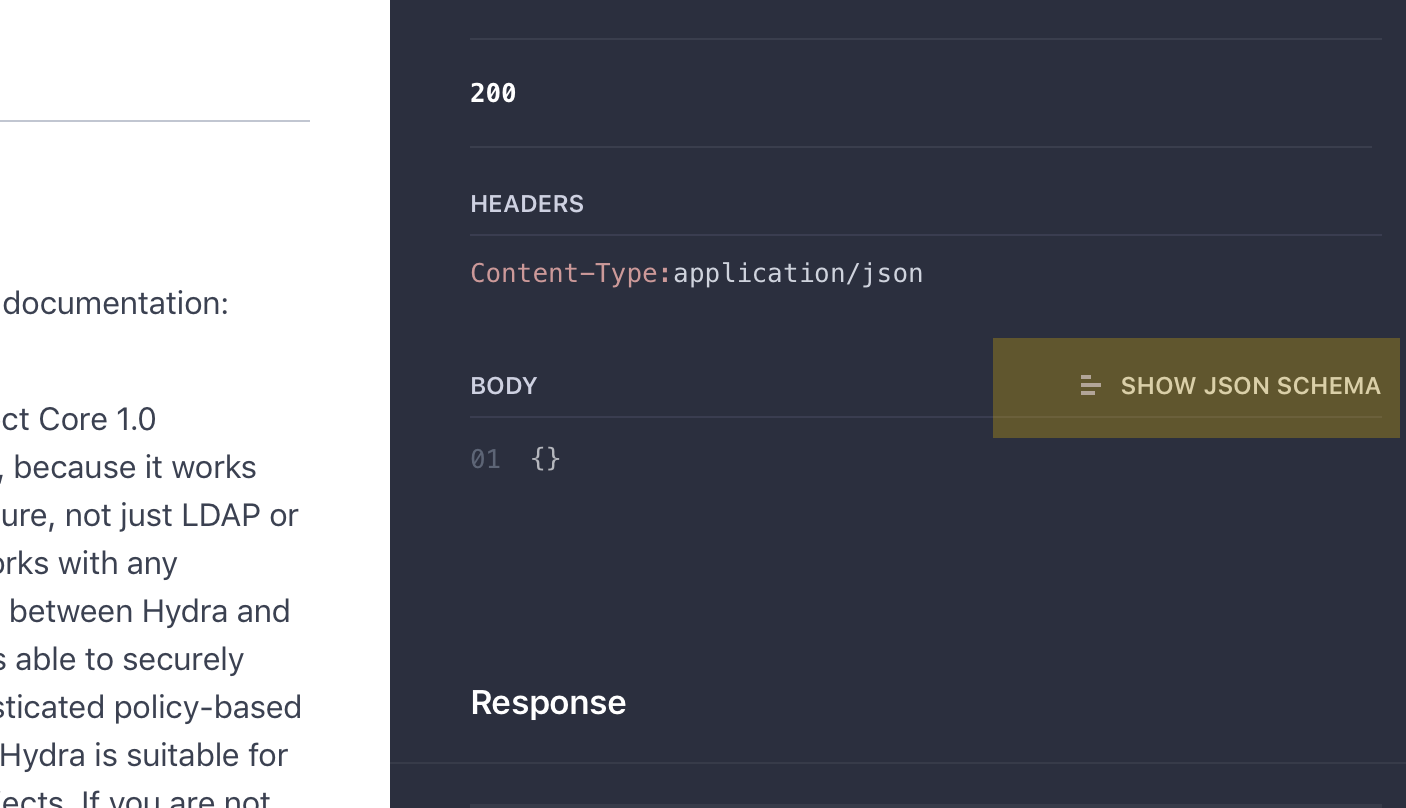 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerListPolicyParameters struct {
+
+ // The maximum amount of policies returned. in: query
+ Limit int64 `json:"limit,omitempty"`
+
+ // The offset from where to start looking. in: query
+ Offset int64 `json:"offset,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_list_policy_response.go b/sdk/go/hydra/swagger/swagger_list_policy_response.go
new file mode 100644
index 00000000000..177df45ffdb
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_list_policy_response.go
@@ -0,0 +1,18 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 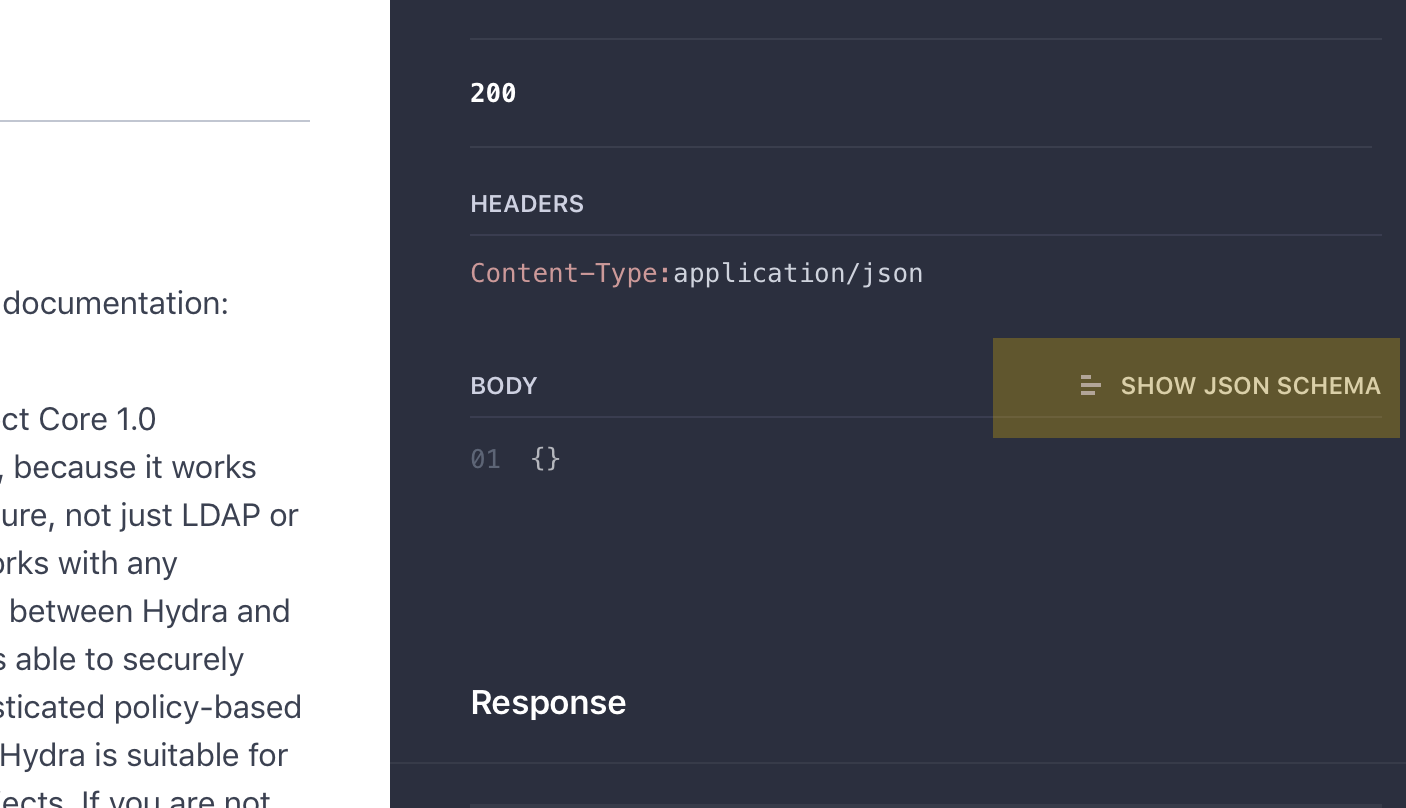 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// A policy
+type SwaggerListPolicyResponse struct {
+
+ // in: body type: array
+ Body []Policy `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_o_auth_consent_request.go b/sdk/go/hydra/swagger/swagger_o_auth_consent_request.go
new file mode 100644
index 00000000000..8cc3b5b95bf
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_o_auth_consent_request.go
@@ -0,0 +1,16 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 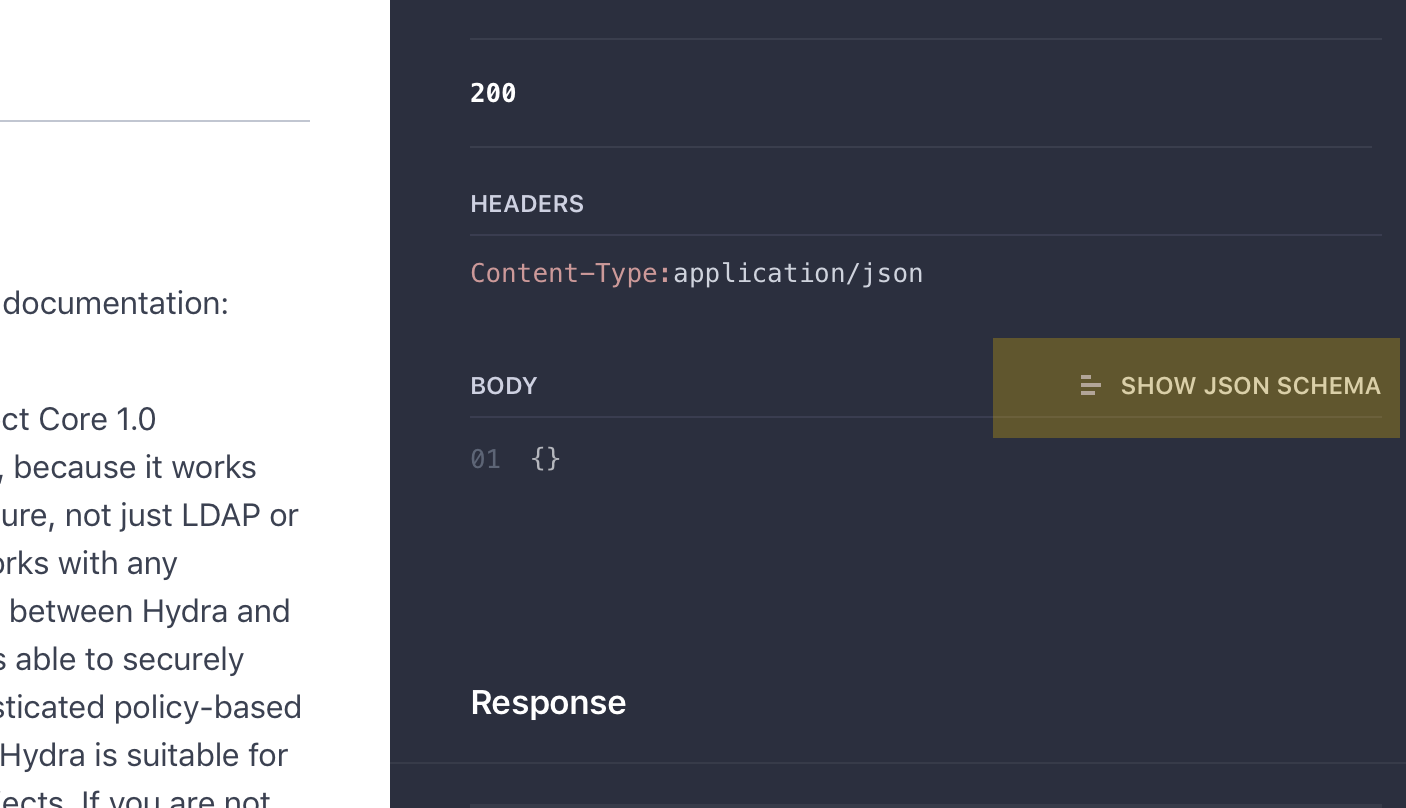 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// The consent request response
+type SwaggerOAuthConsentRequest struct {
+ Body OAuth2consentRequest `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_o_auth_consent_request_payload.go b/sdk/go/hydra/swagger/swagger_o_auth_consent_request_payload.go
new file mode 100644
index 00000000000..571e90891ee
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_o_auth_consent_request_payload.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 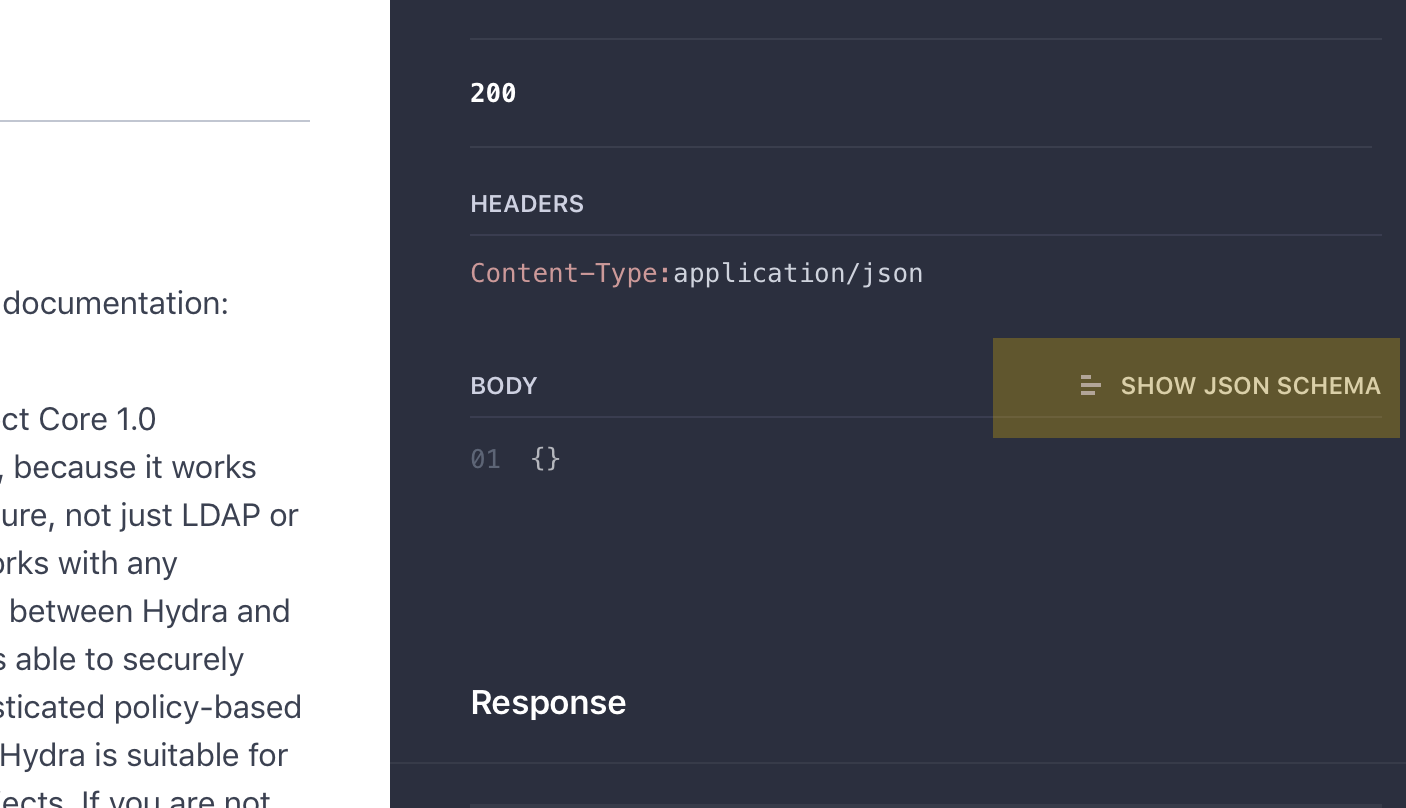 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerOAuthConsentRequestPayload struct {
+
+ // The id of the OAuth 2.0 Consent Request.
+ Id string `json:"id"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_o_auth_introspection_request.go b/sdk/go/hydra/swagger/swagger_o_auth_introspection_request.go
new file mode 100644
index 00000000000..66b97b070ea
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_o_auth_introspection_request.go
@@ -0,0 +1,20 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 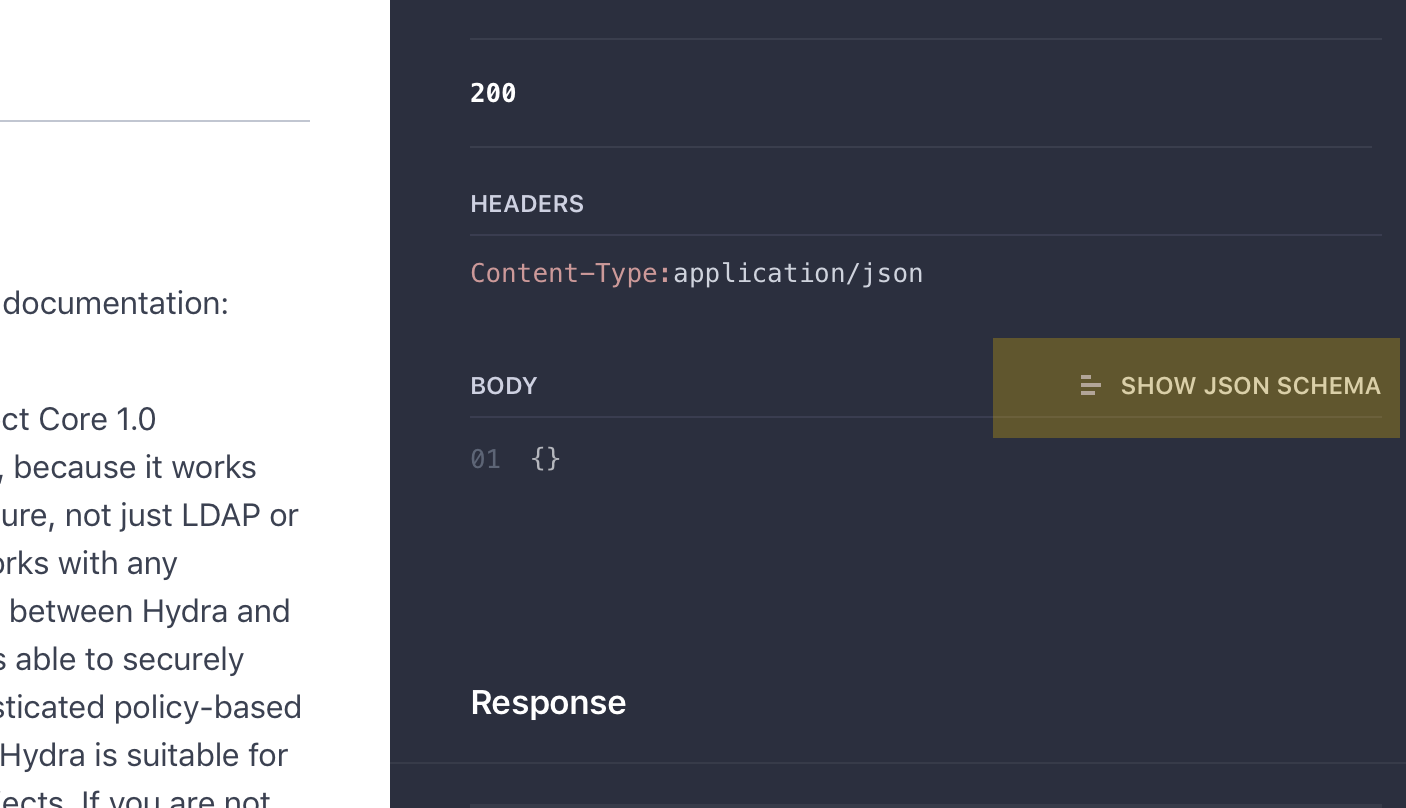 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerOAuthIntrospectionRequest struct {
+
+ // An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false. in: formData
+ Scope string `json:"scope,omitempty"`
+
+ // The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation.
+ Token string `json:"token"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_o_auth_introspection_response.go b/sdk/go/hydra/swagger/swagger_o_auth_introspection_response.go
new file mode 100644
index 00000000000..74060bf6544
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_o_auth_introspection_response.go
@@ -0,0 +1,16 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 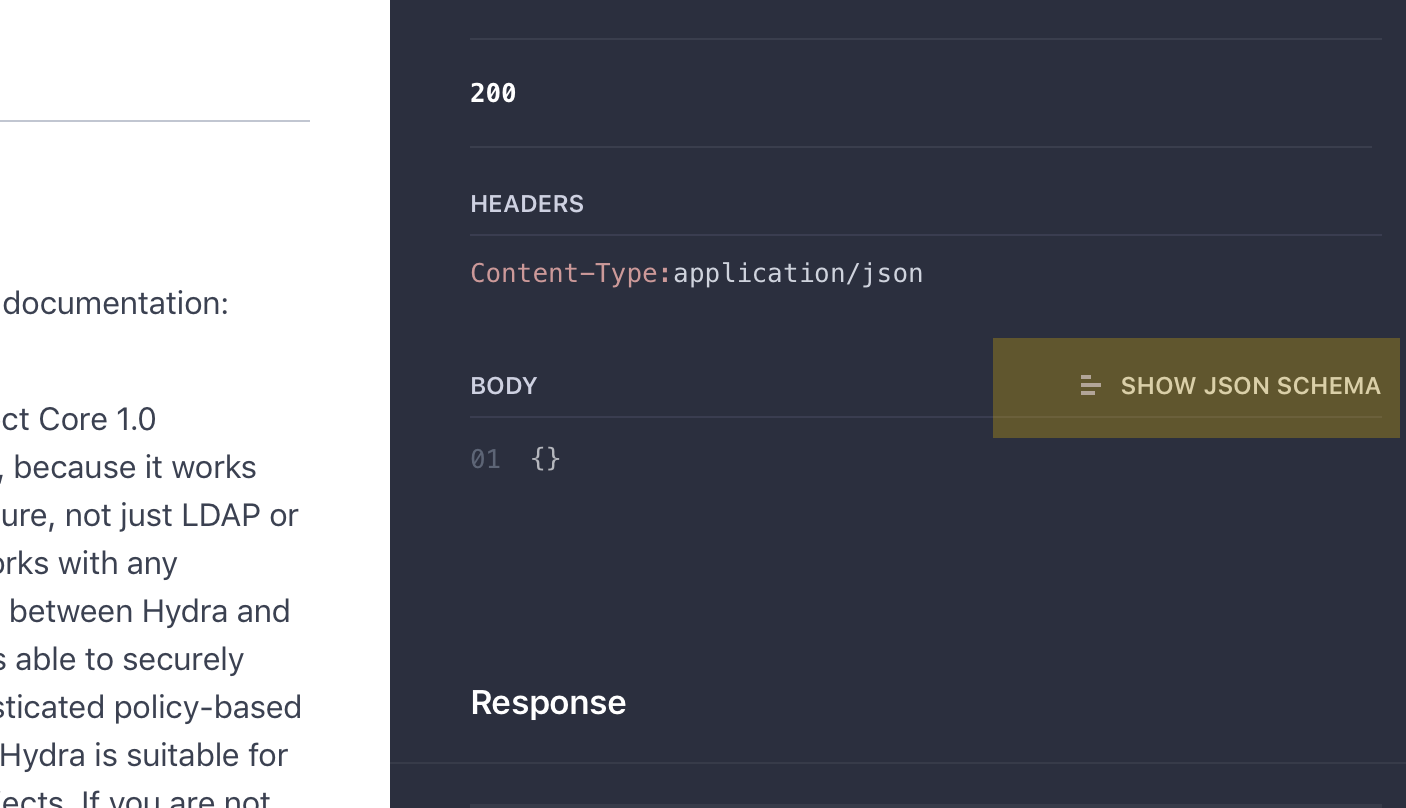 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// The token introspection response
+type SwaggerOAuthIntrospectionResponse struct {
+ Body OAuth2TokenIntrospection `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_o_auth_token_response.go b/sdk/go/hydra/swagger/swagger_o_auth_token_response.go
new file mode 100644
index 00000000000..87bb0bdf9a0
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_o_auth_token_response.go
@@ -0,0 +1,16 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 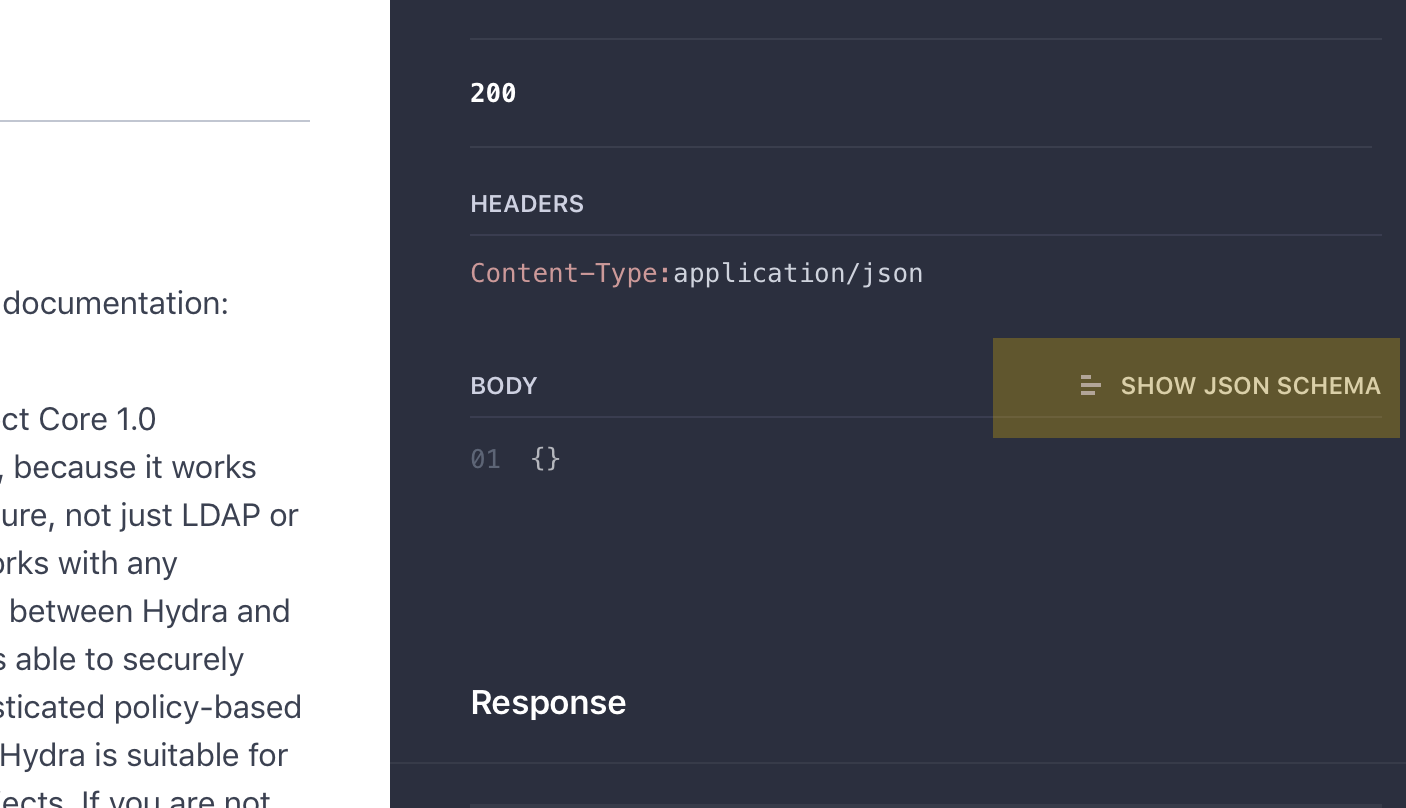 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// The token response
+type SwaggerOAuthTokenResponse struct {
+ Body SwaggerOAuthTokenResponseBody `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_o_auth_token_response_body.go b/sdk/go/hydra/swagger/swagger_o_auth_token_response_body.go
new file mode 100644
index 00000000000..d63923cbfee
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_o_auth_token_response_body.go
@@ -0,0 +1,33 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 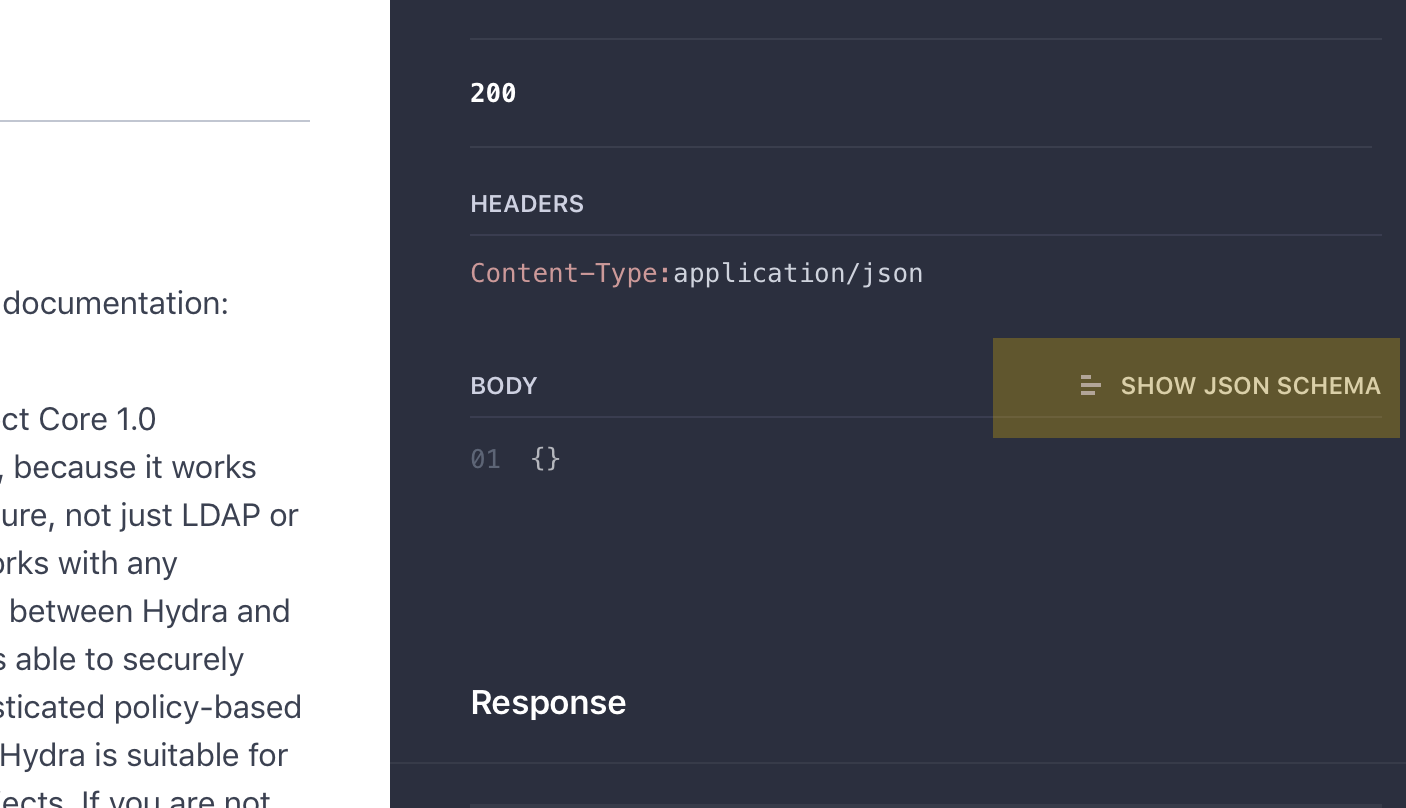 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// in: body
+type SwaggerOAuthTokenResponseBody struct {
+
+ // The access token issued by the authorization server.
+ AccessToken string `json:"access_token,omitempty"`
+
+ // The lifetime in seconds of the access token. For example, the value \"3600\" denotes that the access token will expire in one hour from the time the response was generated.
+ ExpiresIn int64 `json:"expires_in,omitempty"`
+
+ // To retrieve a refresh token request the id_token scope.
+ IdToken int64 `json:"id_token,omitempty"`
+
+ // The refresh token, which can be used to obtain new access tokens. To retrieve it add the scope \"offline\" to your access token request.
+ RefreshToken string `json:"refresh_token,omitempty"`
+
+ // The scope of the access token
+ Scope int64 `json:"scope,omitempty"`
+
+ // The type of the token issued
+ TokenType string `json:"token_type,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_reject_consent_request.go b/sdk/go/hydra/swagger/swagger_reject_consent_request.go
new file mode 100644
index 00000000000..5cd2a209198
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_reject_consent_request.go
@@ -0,0 +1,18 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 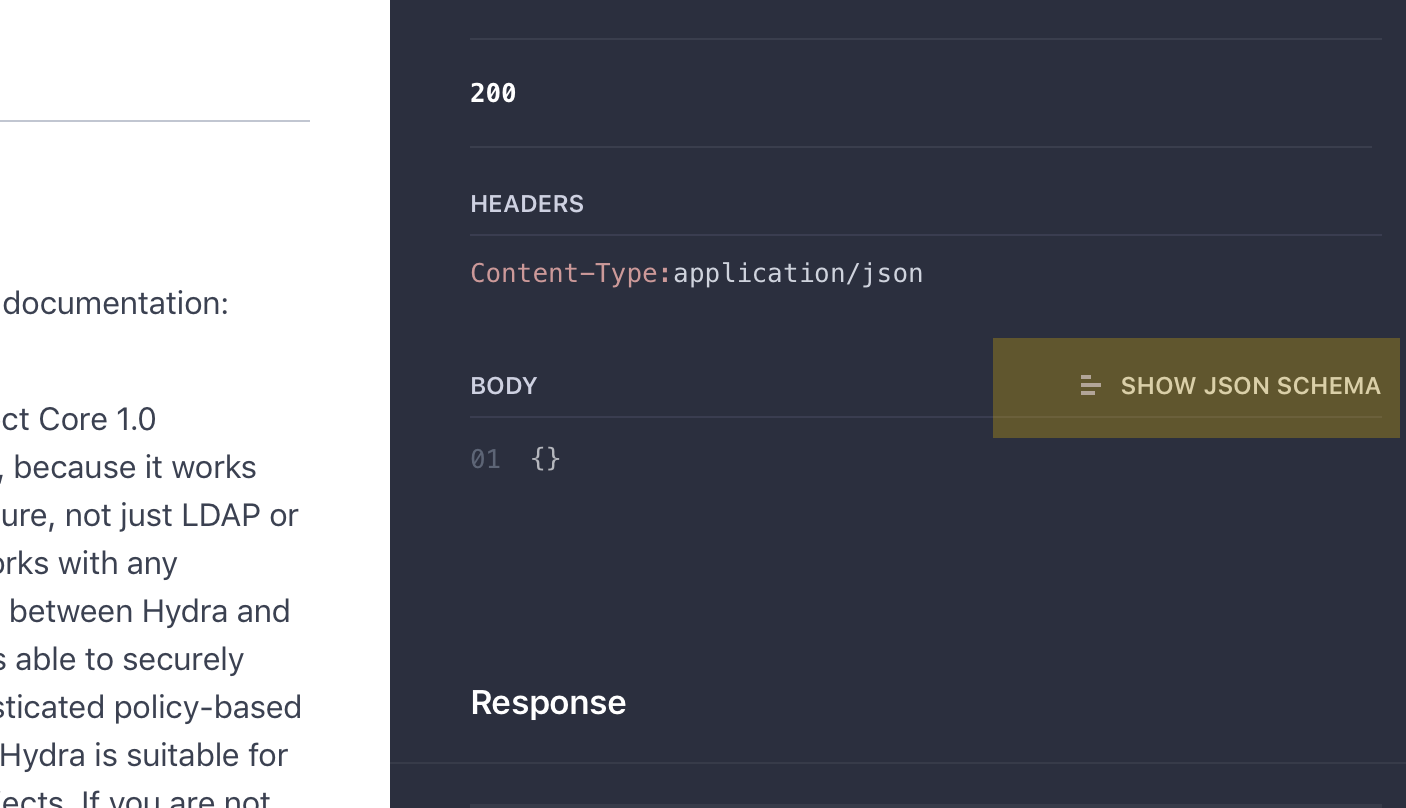 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerRejectConsentRequest struct {
+ Body ConsentRequestRejection `json:"Body"`
+
+ // in: path
+ Id string `json:"id"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_revoke_o_auth2_token_parameters.go b/sdk/go/hydra/swagger/swagger_revoke_o_auth2_token_parameters.go
new file mode 100644
index 00000000000..0185e91347b
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_revoke_o_auth2_token_parameters.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 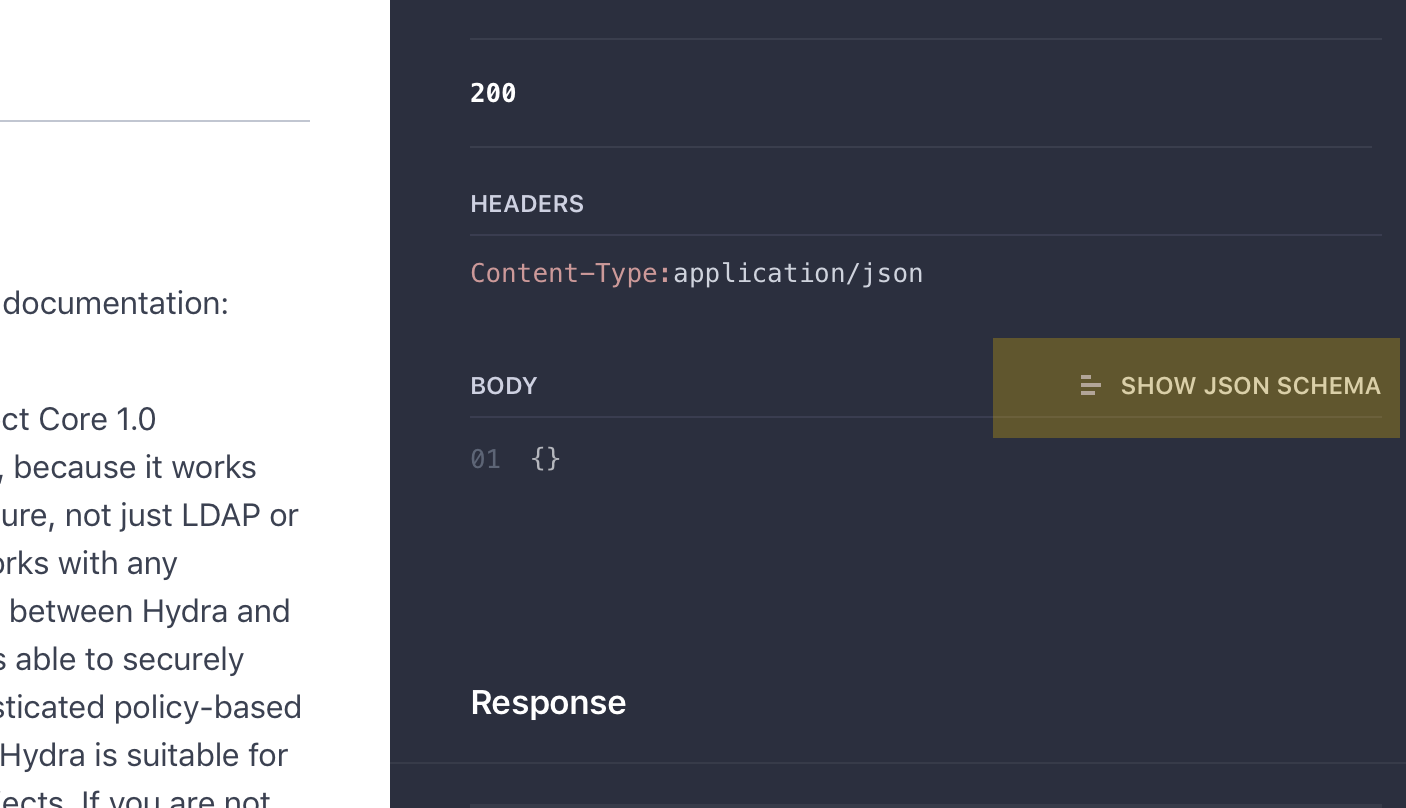 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerRevokeOAuth2TokenParameters struct {
+
+ // in: formData
+ Token string `json:"token"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_update_policy_parameters.go b/sdk/go/hydra/swagger/swagger_update_policy_parameters.go
new file mode 100644
index 00000000000..4f555615b1b
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_update_policy_parameters.go
@@ -0,0 +1,18 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 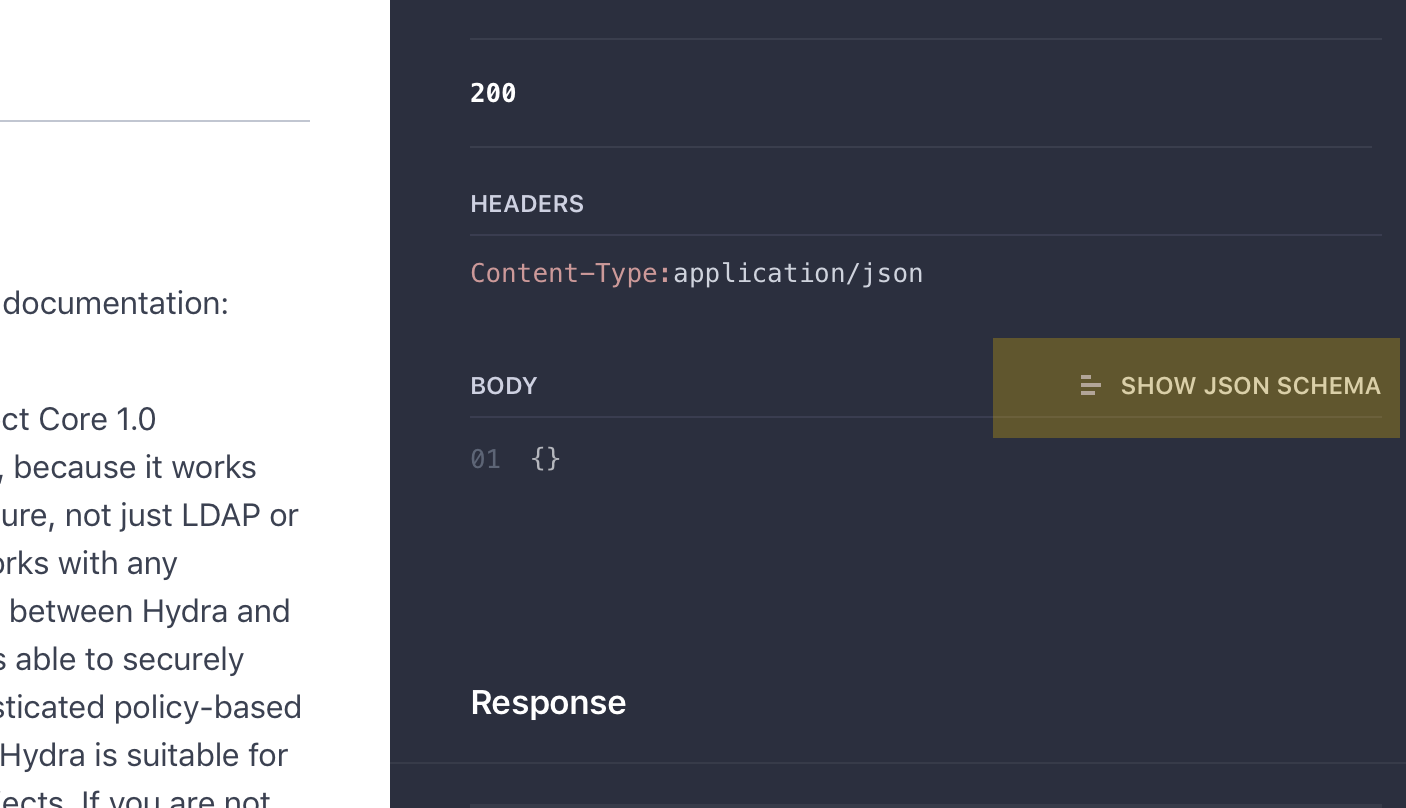 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerUpdatePolicyParameters struct {
+ Body Policy `json:"Body,omitempty"`
+
+ // The id of the policy. in: path
+ Id string `json:"id,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_warden_access_request_response_parameters.go b/sdk/go/hydra/swagger/swagger_warden_access_request_response_parameters.go
new file mode 100644
index 00000000000..0810868c686
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_warden_access_request_response_parameters.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 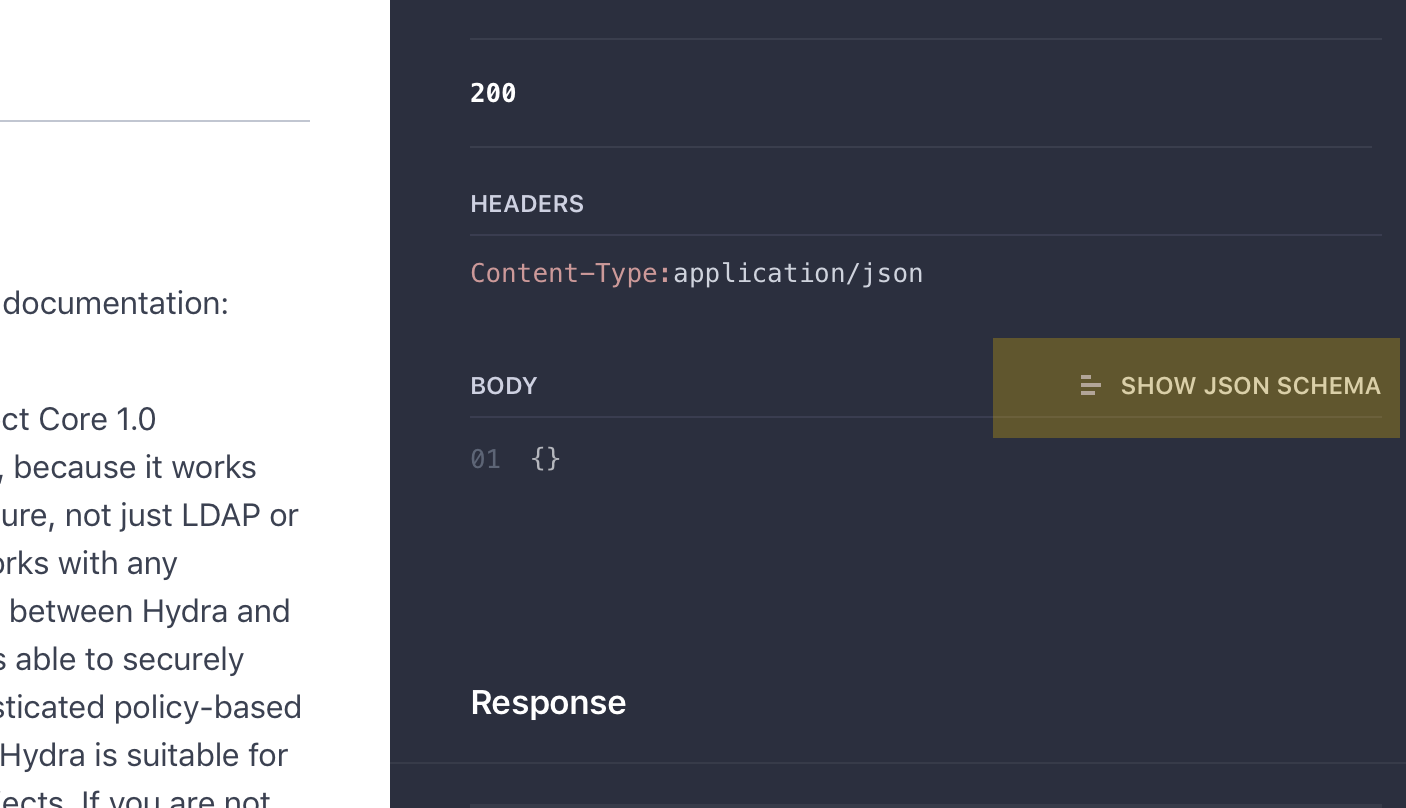 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerWardenAccessRequestResponseParameters struct {
+ Body WardenAccessRequestResponse `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/swagger_warden_token_access_request_response.go b/sdk/go/hydra/swagger/swagger_warden_token_access_request_response.go
new file mode 100644
index 00000000000..88ea6a7b2fd
--- /dev/null
+++ b/sdk/go/hydra/swagger/swagger_warden_token_access_request_response.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 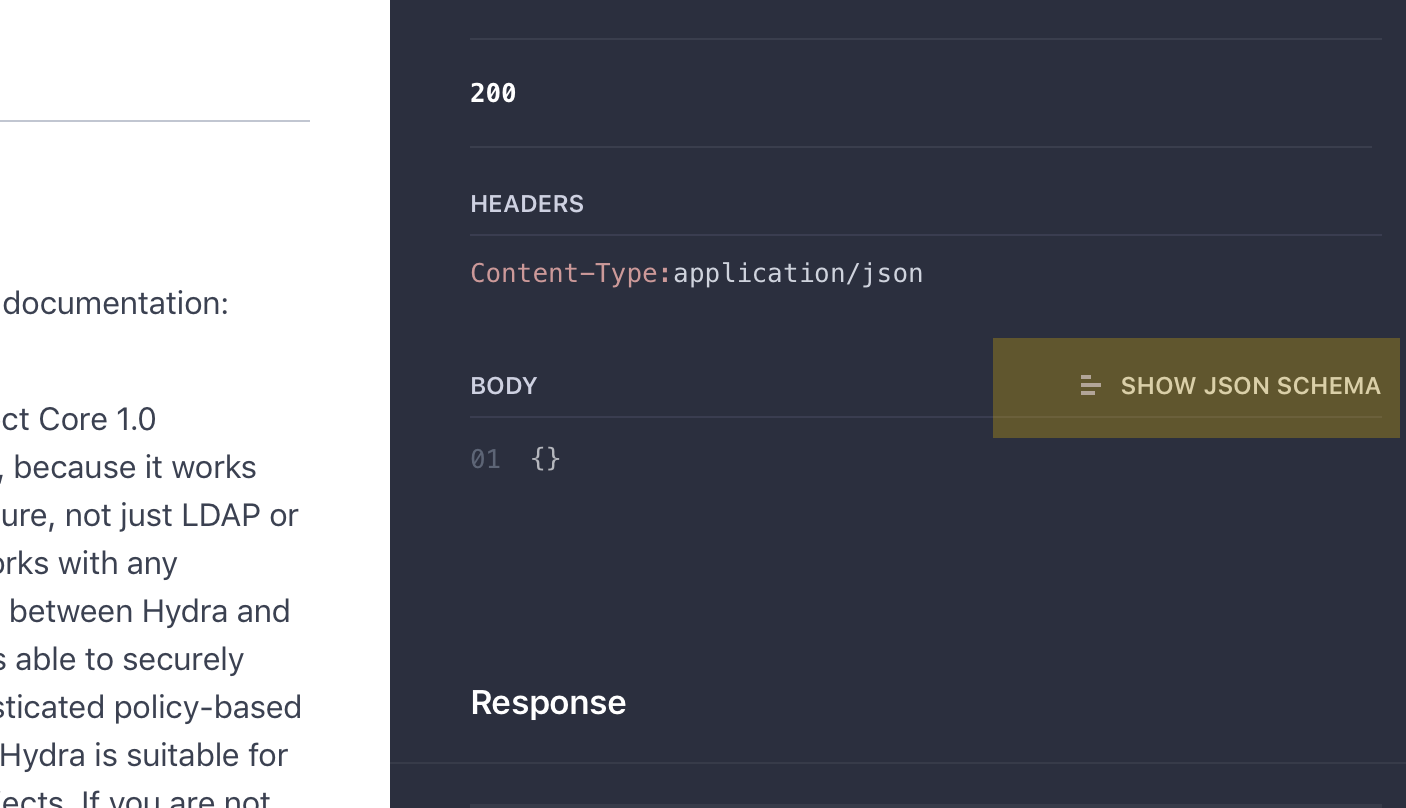 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type SwaggerWardenTokenAccessRequestResponse struct {
+ Body WardenTokenAccessRequestResponsePayload `json:"Body,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/token_allowed_request.go b/sdk/go/hydra/swagger/token_allowed_request.go
new file mode 100644
index 00000000000..c5b8e6711be
--- /dev/null
+++ b/sdk/go/hydra/swagger/token_allowed_request.go
@@ -0,0 +1,23 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 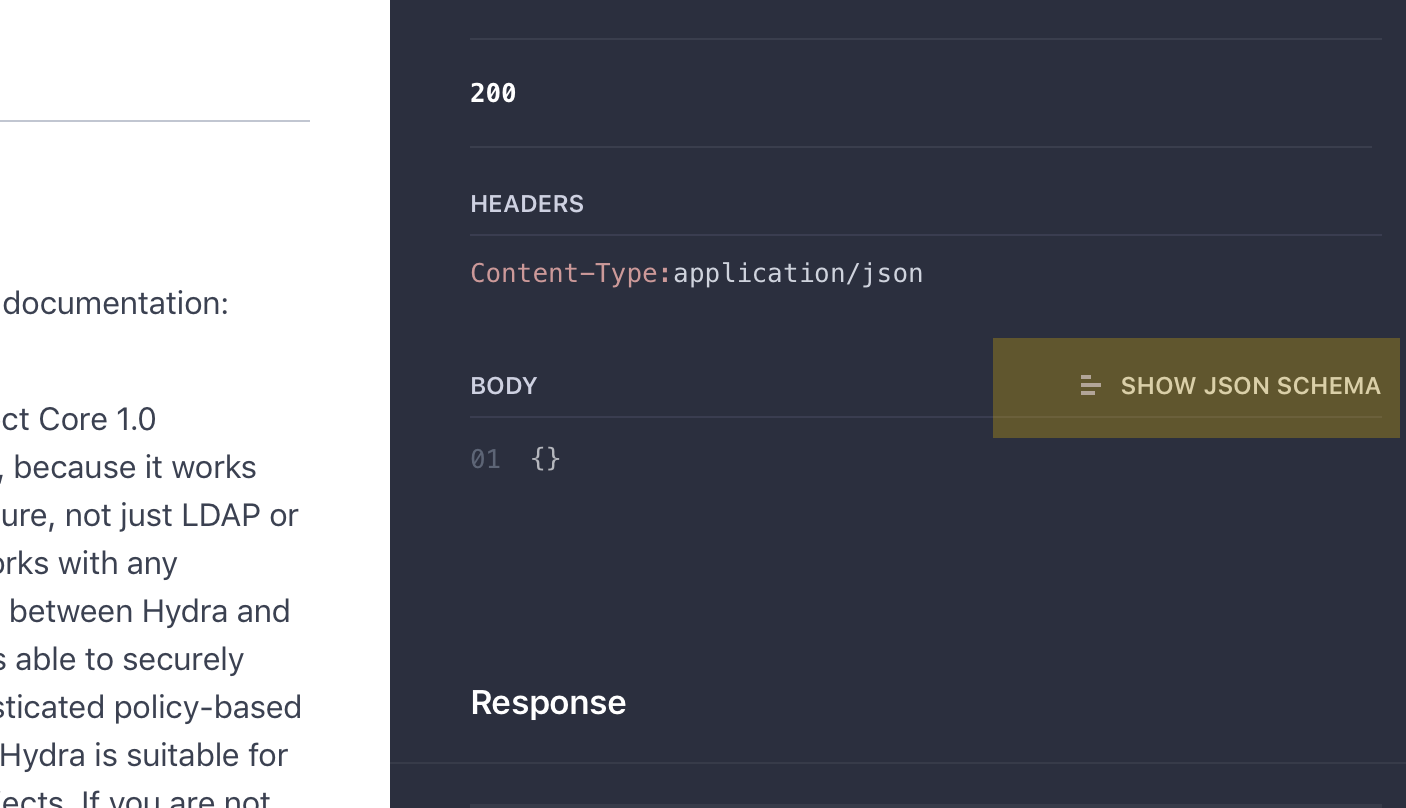 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type TokenAllowedRequest struct {
+
+ // Action is the action that is requested on the resource.
+ Action string `json:"action,omitempty"`
+
+ // Context is the request's environmental context.
+ Context map[string]interface{} `json:"context,omitempty"`
+
+ // Resource is the resource that access is requested to.
+ Resource string `json:"resource,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/warden_access_request.go b/sdk/go/hydra/swagger/warden_access_request.go
new file mode 100644
index 00000000000..9e43c65bfb9
--- /dev/null
+++ b/sdk/go/hydra/swagger/warden_access_request.go
@@ -0,0 +1,26 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 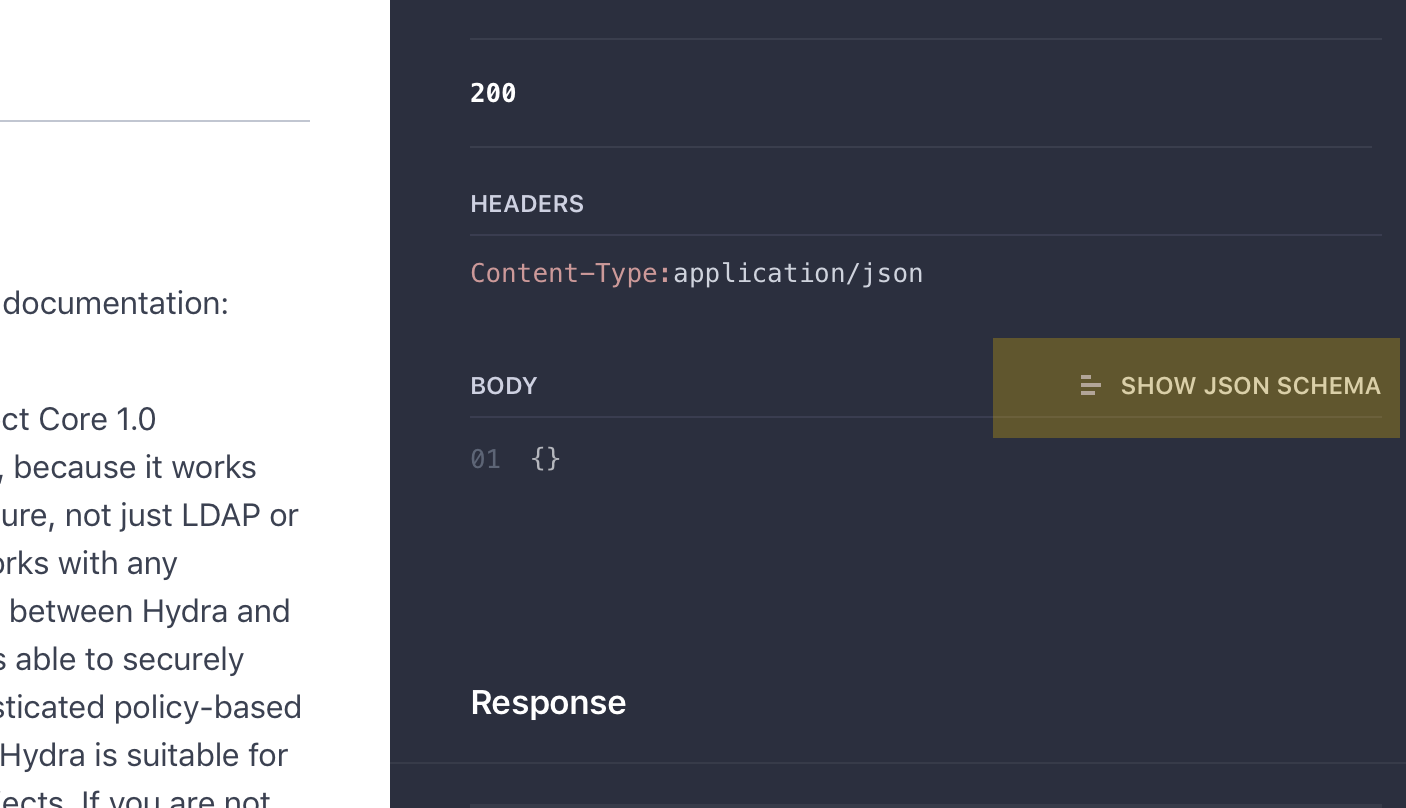 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type WardenAccessRequest struct {
+
+ // Action is the action that is requested on the resource.
+ Action string `json:"action,omitempty"`
+
+ // Context is the request's environmental context.
+ Context map[string]interface{} `json:"context,omitempty"`
+
+ // Resource is the resource that access is requested to.
+ Resource string `json:"resource,omitempty"`
+
+ // Subejct is the subject that is requesting access.
+ Subject string `json:"subject,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/warden_access_request_response.go b/sdk/go/hydra/swagger/warden_access_request_response.go
new file mode 100644
index 00000000000..f39fa6e661c
--- /dev/null
+++ b/sdk/go/hydra/swagger/warden_access_request_response.go
@@ -0,0 +1,17 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 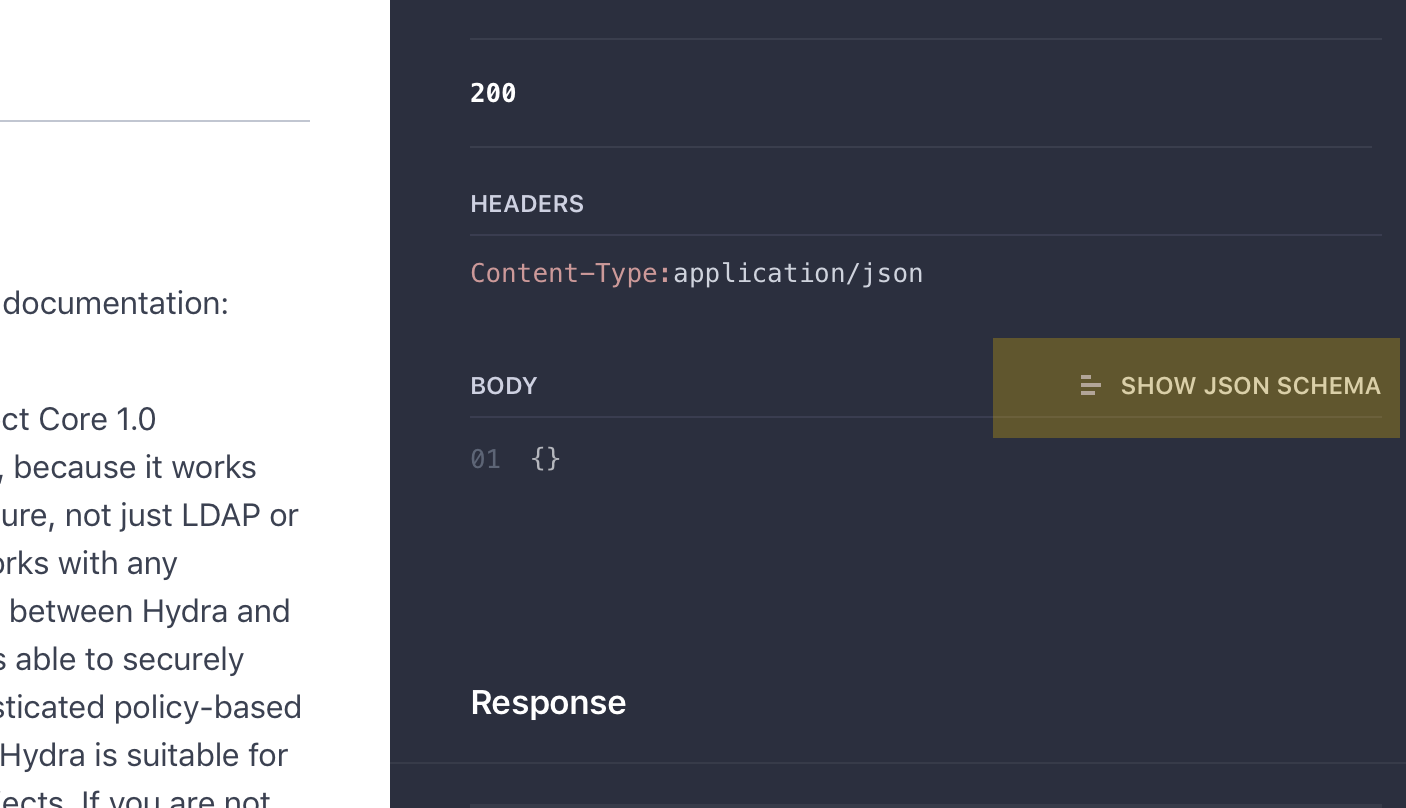 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type WardenAccessRequestResponse struct {
+
+ // Allowed is true if the request is allowed and false otherwise.
+ Allowed bool `json:"allowed,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/warden_api.go b/sdk/go/hydra/swagger/warden_api.go
new file mode 100644
index 00000000000..b8db0a1ed6f
--- /dev/null
+++ b/sdk/go/hydra/swagger/warden_api.go
@@ -0,0 +1,569 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 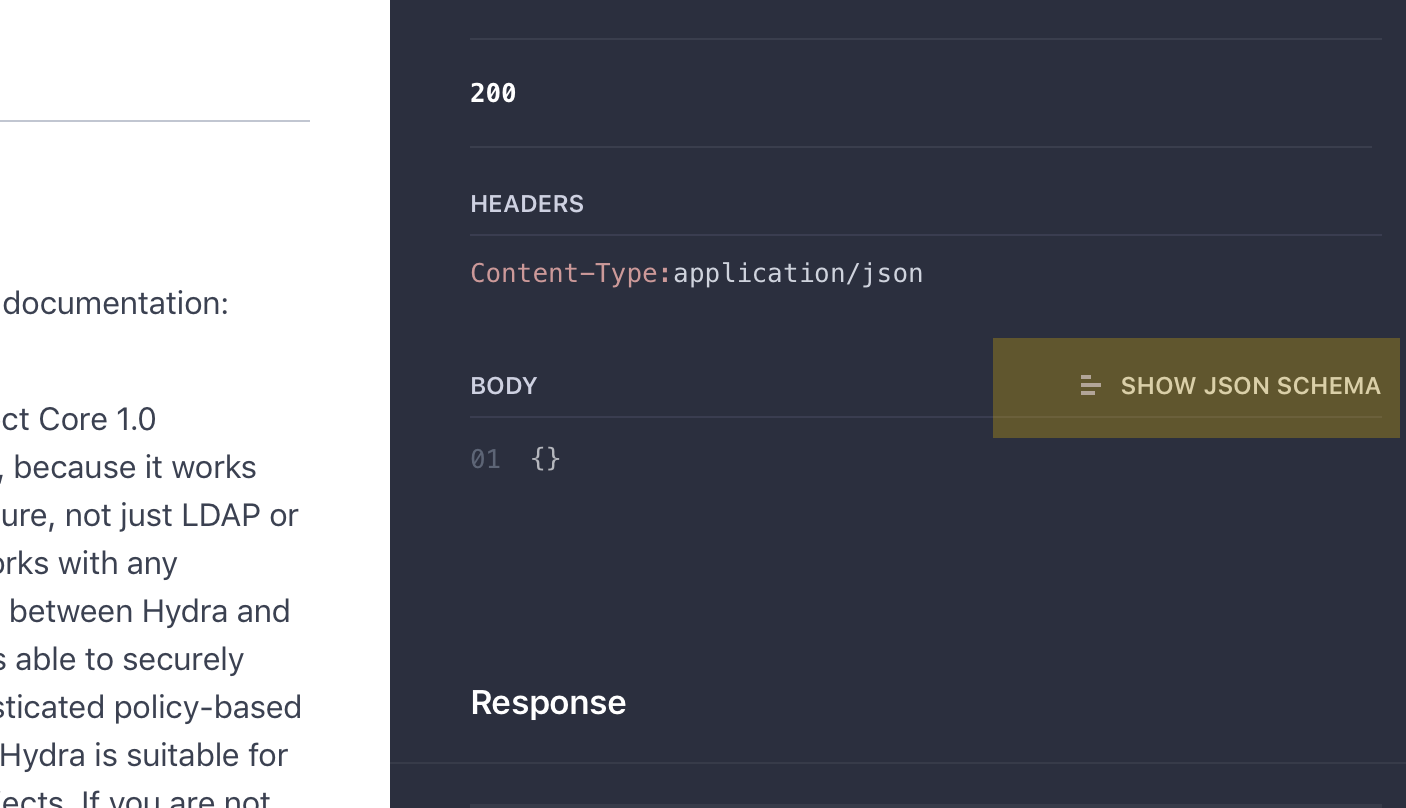 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+import (
+ "encoding/json"
+ "fmt"
+ "net/url"
+ "strings"
+)
+
+type WardenApi struct {
+ Configuration *Configuration
+}
+
+func NewWardenApi() *WardenApi {
+ configuration := NewConfiguration()
+ return &WardenApi{
+ Configuration: configuration,
+ }
+}
+
+func NewWardenApiWithBasePath(basePath string) *WardenApi {
+ configuration := NewConfiguration()
+ configuration.BasePath = basePath
+
+ return &WardenApi{
+ Configuration: configuration,
+ }
+}
+
+/**
+ * Add members to a group
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"members.add\"], \"effect\": \"allow\" } ```
+ *
+ * @param id The id of the group to modify.
+ * @param body
+ * @return void
+ */
+func (a WardenApi) AddMembersToGroup(id string, body GroupMembers) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/warden/groups/{id}/members"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "AddMembersToGroup", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Create a group
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+ *
+ * @param body
+ * @return *Group
+ */
+func (a WardenApi) CreateGroup(body Group) (*Group, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/warden/groups"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(Group)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "CreateGroup", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Delete a group by id
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+ *
+ * @param id The id of the group to look up.
+ * @return void
+ */
+func (a WardenApi) DeleteGroup(id string) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Delete")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/warden/groups/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "DeleteGroup", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
+
+/**
+ * Check if an access request is valid (without providing an access token)
+ * Checks if a subject (typically a user or a service) is allowed to perform an action on a resource. This endpoint requires a subject, a resource name, an action name and a context. If the subject is not allowed to perform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`, otherwise `{ \"allowed\": true }` is returned. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:allowed\"], \"actions\": [\"decide\"], \"effect\": \"allow\" } ```
+ *
+ * @param body
+ * @return *WardenAccessRequestResponse
+ */
+func (a WardenApi) DoesWardenAllowAccessRequest(body WardenAccessRequest) (*WardenAccessRequestResponse, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/warden/allowed"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(WardenAccessRequestResponse)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "DoesWardenAllowAccessRequest", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Check if an access request is valid (providing an access token)
+ * Checks if a token is valid and if the token subject is allowed to perform an action on a resource. This endpoint requires a token, a scope, a resource name, an action name and a context. If a token is expired/invalid, has not been granted the requested scope or the subject is not allowed to perform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`. Extra data set through the `accessTokenExtra` field in the consent flow will be included in the response. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:token:allowed\"], \"actions\": [\"decide\"], \"effect\": \"allow\" } ```
+ *
+ * @param body
+ * @return *WardenTokenAccessRequestResponsePayload
+ */
+func (a WardenApi) DoesWardenAllowTokenAccessRequest(body WardenTokenAccessRequest) (*WardenTokenAccessRequestResponsePayload, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Post")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/warden/token/allowed"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ var successPayload = new(WardenTokenAccessRequestResponsePayload)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "DoesWardenAllowTokenAccessRequest", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Find groups by member
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups\"], \"actions\": [\"list\"], \"effect\": \"allow\" } ```
+ *
+ * @param member The id of the member to look up.
+ * @return []Group
+ */
+func (a WardenApi) FindGroupsByMember(member string) ([]Group, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/warden/groups"
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+ localVarQueryParams.Add("member", a.Configuration.APIClient.ParameterToString(member, ""))
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new([]Group)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "FindGroupsByMember", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return *successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return *successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Get a group by id
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+ *
+ * @param id The id of the group to look up.
+ * @return *Group
+ */
+func (a WardenApi) GetGroup(id string) (*Group, *APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Get")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/warden/groups/{id}"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ var successPayload = new(Group)
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "GetGroup", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return successPayload, localVarAPIResponse, err
+ }
+ err = json.Unmarshal(localVarHttpResponse.Body(), &successPayload)
+ return successPayload, localVarAPIResponse, err
+}
+
+/**
+ * Remove members from a group
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"members.remove\"], \"effect\": \"allow\" } ```
+ *
+ * @param id The id of the group to modify.
+ * @param body
+ * @return void
+ */
+func (a WardenApi) RemoveMembersFromGroup(id string, body GroupMembers) (*APIResponse, error) {
+
+ var localVarHttpMethod = strings.ToUpper("Delete")
+ // create path and map variables
+ localVarPath := a.Configuration.BasePath + "/warden/groups/{id}/members"
+ localVarPath = strings.Replace(localVarPath, "{"+"id"+"}", fmt.Sprintf("%v", id), -1)
+
+ localVarHeaderParams := make(map[string]string)
+ localVarQueryParams := url.Values{}
+ localVarFormParams := make(map[string]string)
+ var localVarPostBody interface{}
+ var localVarFileName string
+ var localVarFileBytes []byte
+ // authentication '(oauth2)' required
+ // oauth required
+ if a.Configuration.AccessToken != "" {
+ localVarHeaderParams["Authorization"] = "Bearer " + a.Configuration.AccessToken
+ }
+ // add default headers if any
+ for key := range a.Configuration.DefaultHeader {
+ localVarHeaderParams[key] = a.Configuration.DefaultHeader[key]
+ }
+
+ // to determine the Content-Type header
+ localVarHttpContentTypes := []string{"application/json"}
+
+ // set Content-Type header
+ localVarHttpContentType := a.Configuration.APIClient.SelectHeaderContentType(localVarHttpContentTypes)
+ if localVarHttpContentType != "" {
+ localVarHeaderParams["Content-Type"] = localVarHttpContentType
+ }
+ // to determine the Accept header
+ localVarHttpHeaderAccepts := []string{
+ "application/json",
+ }
+
+ // set Accept header
+ localVarHttpHeaderAccept := a.Configuration.APIClient.SelectHeaderAccept(localVarHttpHeaderAccepts)
+ if localVarHttpHeaderAccept != "" {
+ localVarHeaderParams["Accept"] = localVarHttpHeaderAccept
+ }
+ // body params
+ localVarPostBody = &body
+ localVarHttpResponse, err := a.Configuration.APIClient.CallAPI(localVarPath, localVarHttpMethod, localVarPostBody, localVarHeaderParams, localVarQueryParams, localVarFormParams, localVarFileName, localVarFileBytes)
+
+ var localVarURL, _ = url.Parse(localVarPath)
+ localVarURL.RawQuery = localVarQueryParams.Encode()
+ var localVarAPIResponse = &APIResponse{Operation: "RemoveMembersFromGroup", Method: localVarHttpMethod, RequestURL: localVarURL.String()}
+ if localVarHttpResponse != nil {
+ localVarAPIResponse.Response = localVarHttpResponse.RawResponse
+ localVarAPIResponse.Payload = localVarHttpResponse.Body()
+ }
+
+ if err != nil {
+ return localVarAPIResponse, err
+ }
+ return localVarAPIResponse, err
+}
diff --git a/sdk/go/hydra/swagger/warden_token_access_request.go b/sdk/go/hydra/swagger/warden_token_access_request.go
new file mode 100644
index 00000000000..b0e081a8174
--- /dev/null
+++ b/sdk/go/hydra/swagger/warden_token_access_request.go
@@ -0,0 +1,29 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 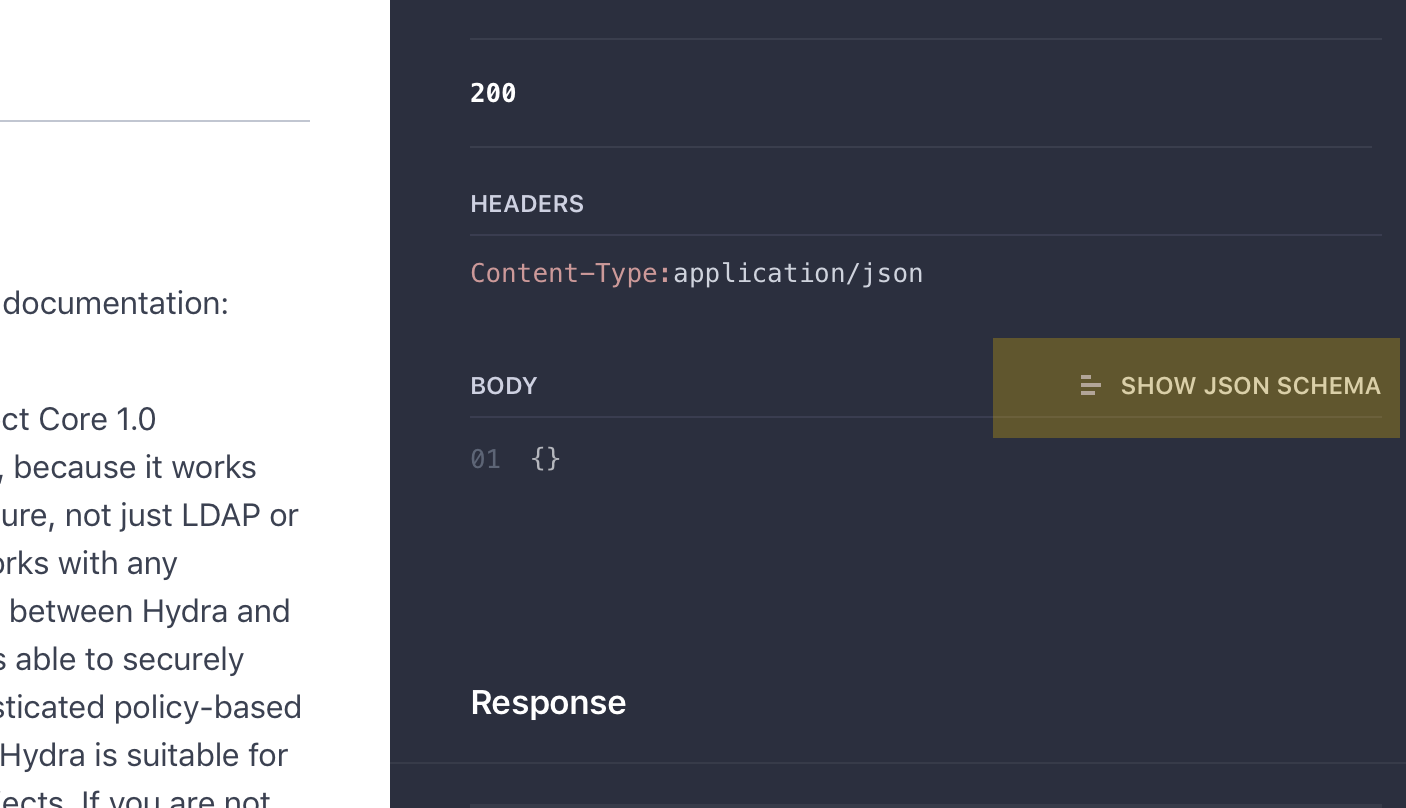 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type WardenTokenAccessRequest struct {
+
+ // Action is the action that is requested on the resource.
+ Action string `json:"action,omitempty"`
+
+ // Context is the request's environmental context.
+ Context map[string]interface{} `json:"context,omitempty"`
+
+ // Resource is the resource that access is requested to.
+ Resource string `json:"resource,omitempty"`
+
+ // Scopes is an array of scopes that are requried.
+ Scopes []string `json:"scopes,omitempty"`
+
+ // Token is the token to introspect.
+ Token string `json:"token,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/warden_token_access_request_response_payload.go b/sdk/go/hydra/swagger/warden_token_access_request_response_payload.go
new file mode 100644
index 00000000000..d34a5ab9a25
--- /dev/null
+++ b/sdk/go/hydra/swagger/warden_token_access_request_response_payload.go
@@ -0,0 +1,38 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 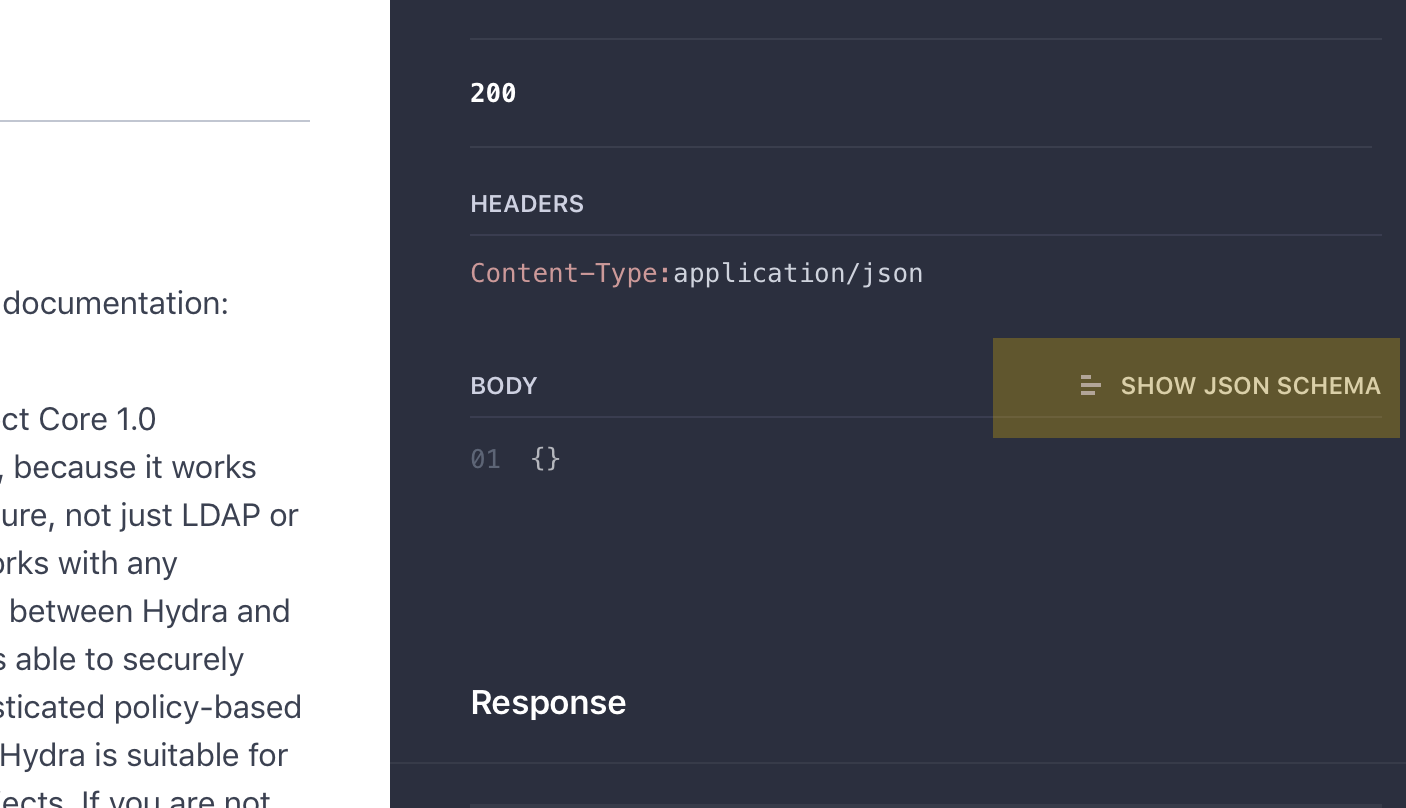 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type WardenTokenAccessRequestResponsePayload struct {
+
+ // Allowed is true if the request is allowed and false otherwise.
+ Allowed bool `json:"allowed,omitempty"`
+
+ // Audience is who the token was issued for. This is an OAuth2 app usually.
+ Aud string `json:"aud,omitempty"`
+
+ // ExpiresAt is the expiry timestamp.
+ Exp string `json:"exp,omitempty"`
+
+ // Extra represents arbitrary session data.
+ Ext map[string]interface{} `json:"ext,omitempty"`
+
+ // IssuedAt is the token creation time stamp.
+ Iat string `json:"iat,omitempty"`
+
+ // Issuer is the id of the issuer, typically an hydra instance.
+ Iss string `json:"iss,omitempty"`
+
+ // GrantedScopes is a list of scopes that the subject authorized when asked for consent.
+ Scopes []string `json:"scopes,omitempty"`
+
+ // Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app. This is usually a uuid but you can choose a urn or some other id too.
+ Sub string `json:"sub,omitempty"`
+}
diff --git a/sdk/go/hydra/swagger/well_known.go b/sdk/go/hydra/swagger/well_known.go
new file mode 100644
index 00000000000..dee740c82a8
--- /dev/null
+++ b/sdk/go/hydra/swagger/well_known.go
@@ -0,0 +1,35 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 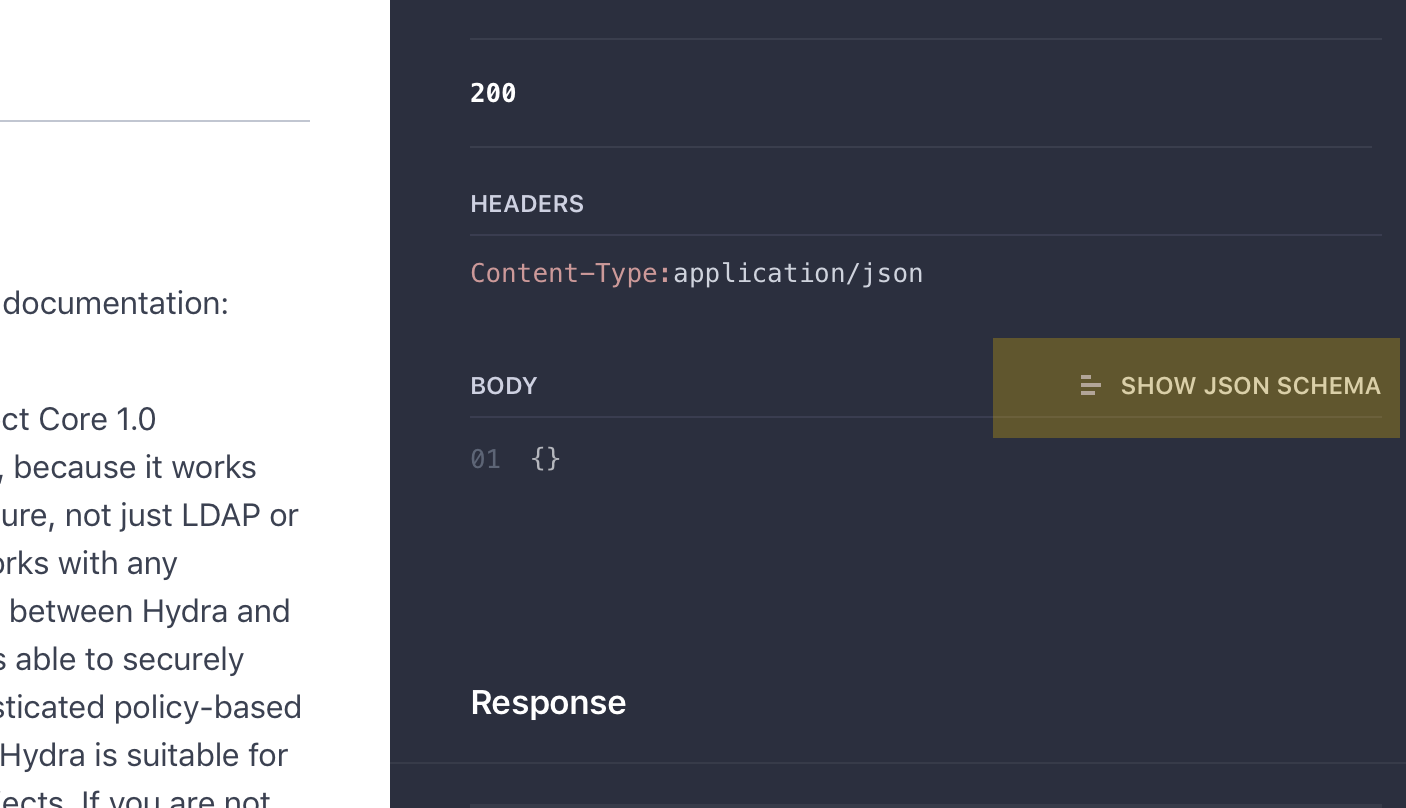 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+type WellKnown struct {
+
+ // URL of the OP's OAuth 2.0 Authorization Endpoint
+ AuthorizationEndpoint string `json:"authorization_endpoint"`
+
+ // JSON array containing a list of the JWS signing algorithms (alg values) supported by the OP for the ID Token to encode the Claims in a JWT [JWT]. The algorithm RS256 MUST be included. The value none MAY be supported, but MUST NOT be used unless the Response Type used returns no ID Token from the Authorization Endpoint (such as when using the Authorization Code Flow).
+ IdTokenSigningAlgValuesSupported []string `json:"id_token_signing_alg_values_supported"`
+
+ // URL using the https scheme with no query or fragment component that the OP asserts as its Issuer Identifier. If Issuer discovery is supported , this value MUST be identical to the issuer value returned by WebFinger. This also MUST be identical to the iss Claim value in ID Tokens issued from this Issuer.
+ Issuer string `json:"issuer"`
+
+ // URL of the OP's JSON Web Key Set [JWK] document. This contains the signing key(s) the RP uses to validate signatures from the OP. The JWK Set MAY also contain the Server's encryption key(s), which are used by RPs to encrypt requests to the Server. When both signing and encryption keys are made available, a use (Key Use) parameter value is REQUIRED for all keys in the referenced JWK Set to indicate each key's intended usage. Although some algorithms allow the same key to be used for both signatures and encryption, doing so is NOT RECOMMENDED, as it is less secure. The JWK x5c parameter MAY be used to provide X.509 representations of keys provided. When used, the bare key values MUST still be present and MUST match those in the certificate.
+ JwksUri string `json:"jwks_uri"`
+
+ // JSON array containing a list of the OAuth 2.0 response_type values that this OP supports. Dynamic OpenID Providers MUST support the code, id_token, and the token id_token Response Type values.
+ ResponseTypesSupported []string `json:"response_types_supported"`
+
+ // JSON array containing a list of the Subject Identifier types that this OP supports. Valid types include pairwise and public.
+ SubjectTypesSupported []string `json:"subject_types_supported"`
+
+ // URL of the OP's OAuth 2.0 Token Endpoint
+ TokenEndpoint string `json:"token_endpoint"`
+}
diff --git a/sdk/go/hydra/swagger/writer.go b/sdk/go/hydra/swagger/writer.go
new file mode 100644
index 00000000000..75dca3814c6
--- /dev/null
+++ b/sdk/go/hydra/swagger/writer.go
@@ -0,0 +1,15 @@
+/*
+ * Hydra OAuth2 & OpenID Connect Server
+ *
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 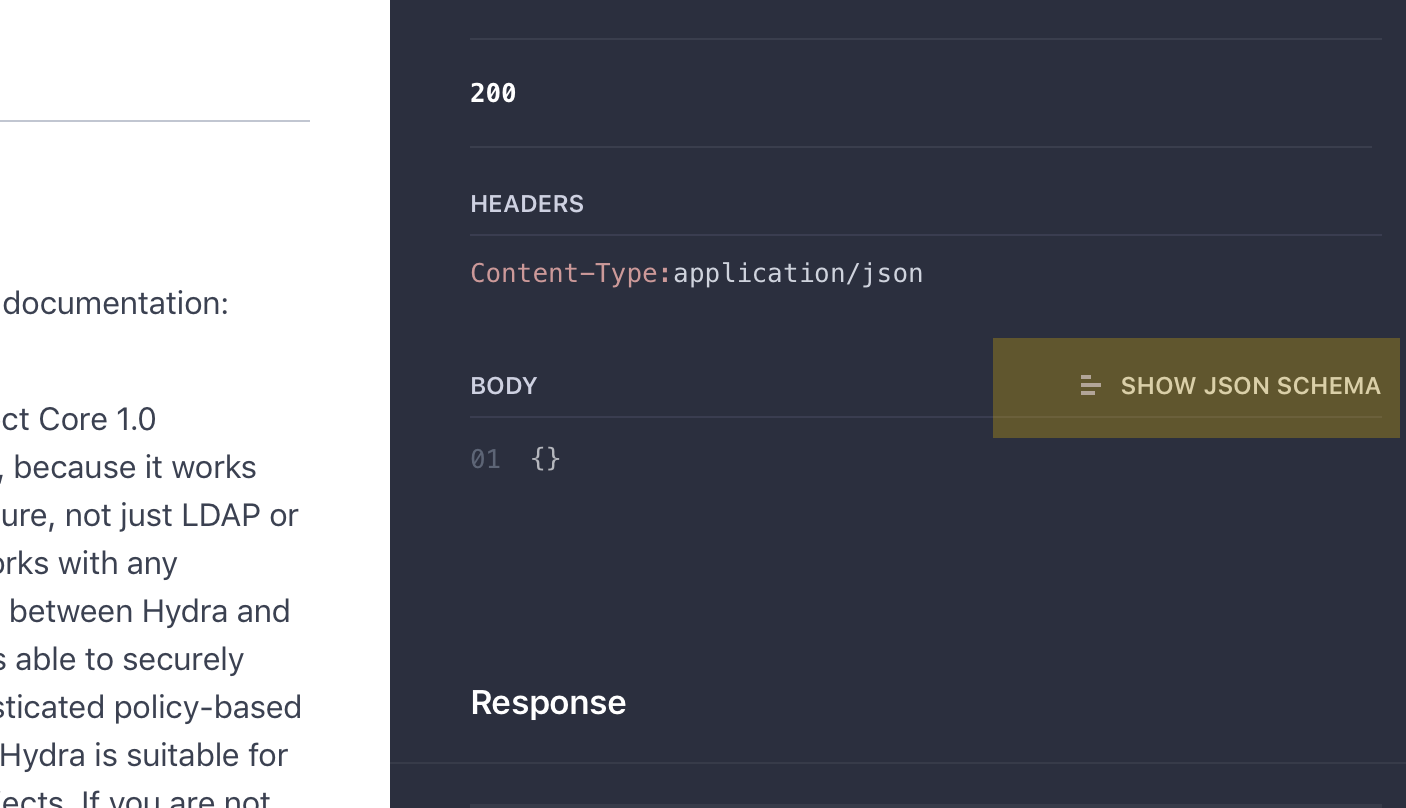 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ * Generated by: https://github.com/swagger-api/swagger-codegen.git
+ */
+
+package swagger
+
+// Writer is a helper to write arbitrary data to a ResponseWriter
+type Writer struct {
+}
diff --git a/sdk/hydra-sdk-example/main.go b/sdk/hydra-sdk-example/main.go
deleted file mode 100644
index 7f42e928e93..00000000000
--- a/sdk/hydra-sdk-example/main.go
+++ /dev/null
@@ -1,71 +0,0 @@
-package main
-
-import (
- "context"
- "fmt"
- "log"
- "net/http"
- "os"
-
- "github.com/julienschmidt/httprouter"
- "github.com/ory/hydra/firewall"
- "github.com/ory/hydra/sdk"
-)
-
-var h *sdk.Client
-
-func main() {
- var err error
-
- if h, err = sdk.Connect(
- sdk.ClientID("client-id"),
- sdk.ClientSecret("client-secret"),
- sdk.ClusterURL("https://localhost:4444"),
- ); err != nil {
- log.Fatalf("Could not connect to host: %s", err)
- }
-
- r := httprouter.New()
- r.GET("/protected", handleProtectedEndpoint)
-
- listen := fmt.Sprintf("%s:%s", os.Getenv("HOST"), os.Getenv("PORT"))
- if err := http.ListenAndServe(listen, r); err != nil {
- log.Fatalf("Could not listen on %s becase %s", listen, err)
- }
-}
-
-func handleProtectedEndpoint(w http.ResponseWriter, r *http.Request, _ httprouter.Params) {
- token := h.Warden.TokenFromRequest(r)
-
- // Access control using only access token.
- if status, err := h.Introspection.IntrospectToken(context.Background(), token, "some-scope"); err != nil {
- w.WriteHeader(http.StatusForbidden)
- w.Write([]byte(err.Error()))
- return
- } else {
- log.Printf("Token is allowed to perform action, state lookup gave: %v", status)
- }
-
- // Access control using access token and access control policies.
- if status, err := h.Warden.TokenAllowed(context.Background(), token, &firewall.TokenAccessRequest{
- Resource: "some:resource-name",
- Action: "some-action",
- }, "some-scope"); err != nil {
- w.WriteHeader(http.StatusForbidden)
- w.Write([]byte(err.Error()))
- return
- } else {
- log.Printf("Token is allowed to perform action, state lookup gave: %v", status)
- }
-
- // Access control using access control policies only.
- if err := h.Warden.IsAllowed(context.Background(), &firewall.AccessRequest{
- Resource: "some:resource-name",
- Action: "some-action",
- Subject: "some-user",
- }); err != nil {
- w.WriteHeader(http.StatusForbidden)
- w.Write([]byte(err.Error()))
- return
- }
-}
diff --git a/sdk/js/swagger/.swagger-codegen-ignore b/sdk/js/swagger/.swagger-codegen-ignore
new file mode 100644
index 00000000000..c5fa491b4c5
--- /dev/null
+++ b/sdk/js/swagger/.swagger-codegen-ignore
@@ -0,0 +1,23 @@
+# Swagger Codegen Ignore
+# Generated by swagger-codegen https://github.com/swagger-api/swagger-codegen
+
+# Use this file to prevent files from being overwritten by the generator.
+# The patterns follow closely to .gitignore or .dockerignore.
+
+# As an example, the C# client generator defines ApiClient.cs.
+# You can make changes and tell Swagger Codgen to ignore just this file by uncommenting the following line:
+#ApiClient.cs
+
+# You can match any string of characters against a directory, file or extension with a single asterisk (*):
+#foo/*/qux
+# The above matches foo/bar/qux and foo/baz/qux, but not foo/bar/baz/qux
+
+# You can recursively match patterns against a directory, file or extension with a double asterisk (**):
+#foo/**/qux
+# This matches foo/bar/qux, foo/baz/qux, and foo/bar/baz/qux
+
+# You can also negate patterns with an exclamation (!).
+# For example, you can ignore all files in a docs folder with the file extension .md:
+#docs/*.md
+# Then explicitly reverse the ignore rule for a single file:
+#!docs/README.md
diff --git a/sdk/js/swagger/.swagger-codegen/VERSION b/sdk/js/swagger/.swagger-codegen/VERSION
new file mode 100644
index 00000000000..6b4d1577382
--- /dev/null
+++ b/sdk/js/swagger/.swagger-codegen/VERSION
@@ -0,0 +1 @@
+2.2.3
\ No newline at end of file
diff --git a/sdk/js/swagger/.travis.yml b/sdk/js/swagger/.travis.yml
new file mode 100644
index 00000000000..e49f4692f7c
--- /dev/null
+++ b/sdk/js/swagger/.travis.yml
@@ -0,0 +1,7 @@
+language: node_js
+node_js:
+ - "6"
+ - "6.1"
+ - "5"
+ - "5.11"
+
diff --git a/sdk/js/swagger/README.md b/sdk/js/swagger/README.md
new file mode 100644
index 00000000000..e00b6cf767e
--- /dev/null
+++ b/sdk/js/swagger/README.md
@@ -0,0 +1,244 @@
+# hydra_o_auth2__open_id_connect_server
+
+HydraOAuth2OpenIdConnectServer - JavaScript client for hydra_o_auth2__open_id_connect_server
+Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 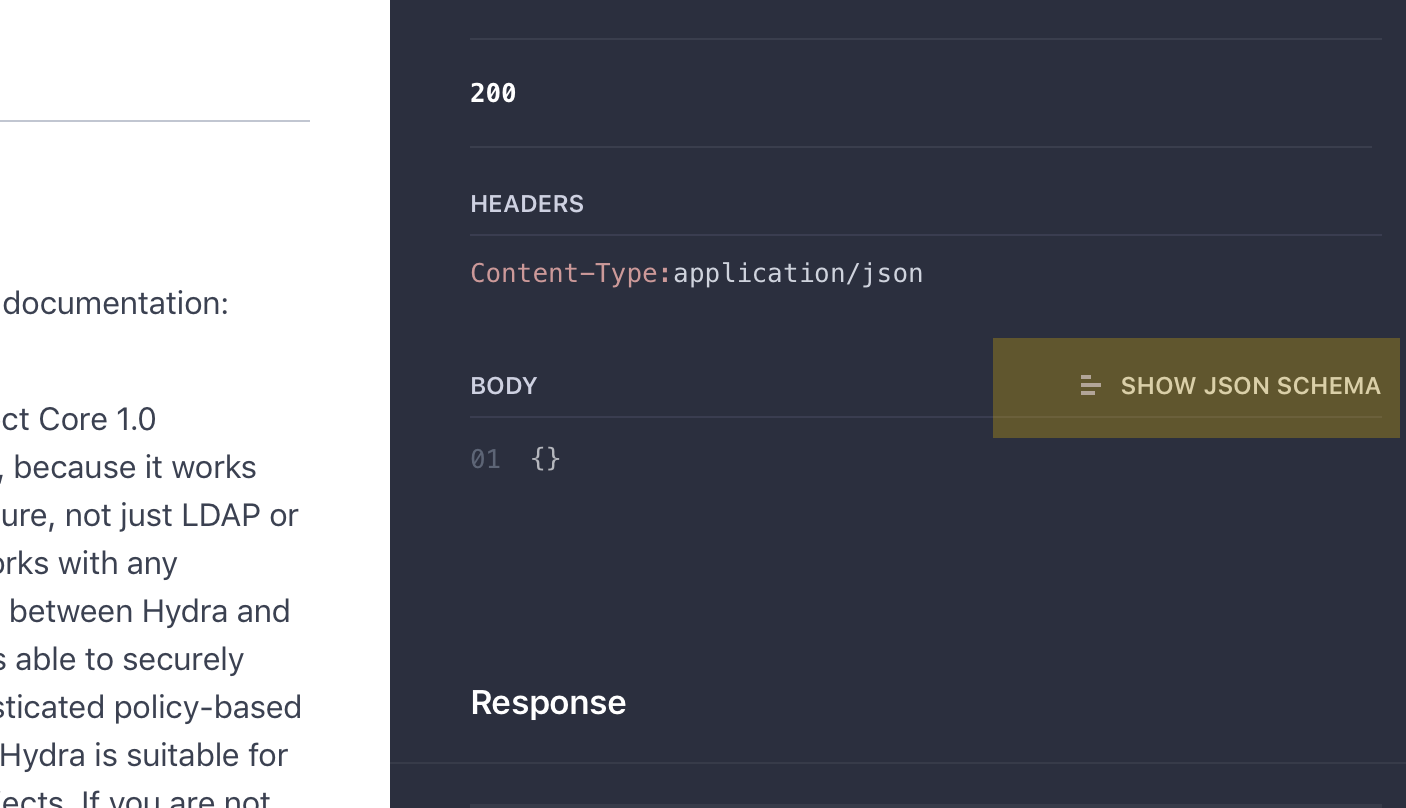 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+This SDK is automatically generated by the [Swagger Codegen](https://github.com/swagger-api/swagger-codegen) project:
+
+- API version: Latest
+- Package version: Latest
+- Build package: io.swagger.codegen.languages.JavascriptClientCodegen
+For more information, please visit [https://www.ory.am](https://www.ory.am)
+
+## Installation
+
+### For [Node.js](https://nodejs.org/)
+
+#### npm
+
+To publish the library as a [npm](https://www.npmjs.com/),
+please follow the procedure in ["Publishing npm packages"](https://docs.npmjs.com/getting-started/publishing-npm-packages).
+
+Then install it via:
+
+```shell
+npm install hydra_o_auth2__open_id_connect_server --save
+```
+
+##### Local development
+
+To use the library locally without publishing to a remote npm registry, first install the dependencies by changing
+into the directory containing `package.json` (and this README). Let's call this `JAVASCRIPT_CLIENT_DIR`. Then run:
+
+```shell
+npm install
+```
+
+Next, [link](https://docs.npmjs.com/cli/link) it globally in npm with the following, also from `JAVASCRIPT_CLIENT_DIR`:
+
+```shell
+npm link
+```
+
+Finally, switch to the directory you want to use your hydra_o_auth2__open_id_connect_server from, and run:
+
+```shell
+npm link /path/to/
+```
+
+You should now be able to `require('hydra_o_auth2__open_id_connect_server')` in javascript files from the directory you ran the last
+command above from.
+
+#### git
+#
+If the library is hosted at a git repository, e.g.
+https://github.com/GIT_USER_ID/GIT_REPO_ID
+then install it via:
+
+```shell
+ npm install GIT_USER_ID/GIT_REPO_ID --save
+```
+
+### For browser
+
+The library also works in the browser environment via npm and [browserify](http://browserify.org/). After following
+the above steps with Node.js and installing browserify with `npm install -g browserify`,
+perform the following (assuming *main.js* is your entry file, that's to say your javascript file where you actually
+use this library):
+
+```shell
+browserify main.js > bundle.js
+```
+
+Then include *bundle.js* in the HTML pages.
+
+### Webpack Configuration
+
+Using Webpack you may encounter the following error: "Module not found: Error:
+Cannot resolve module", most certainly you should disable AMD loader. Add/merge
+the following section to your webpack config:
+
+```javascript
+module: {
+ rules: [
+ {
+ parser: {
+ amd: false
+ }
+ }
+ ]
+}
+```
+
+## Getting Started
+
+Please follow the [installation](#installation) instruction and execute the following JS code:
+
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = "YOUR ACCESS TOKEN"
+
+var api = new HydraOAuth2OpenIdConnectServer.HealthApi()
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+api.getInstanceMetrics(callback);
+
+```
+
+## Documentation for API Endpoints
+
+All URIs are relative to *http://localhost*
+
+Class | Method | HTTP request | Description
+------------ | ------------- | ------------- | -------------
+*HydraOAuth2OpenIdConnectServer.HealthApi* | [**getInstanceMetrics**](docs/HealthApi.md#getInstanceMetrics) | **GET** /health/metrics | Show instance metrics (experimental)
+*HydraOAuth2OpenIdConnectServer.HealthApi* | [**getInstanceStatus**](docs/HealthApi.md#getInstanceStatus) | **GET** /health/status | Check health status of this instance
+*HydraOAuth2OpenIdConnectServer.JsonWebKeyApi* | [**createJsonWebKeySet**](docs/JsonWebKeyApi.md#createJsonWebKeySet) | **POST** /keys/{set} | Generate a new JSON Web Key
+*HydraOAuth2OpenIdConnectServer.JsonWebKeyApi* | [**deleteJsonWebKey**](docs/JsonWebKeyApi.md#deleteJsonWebKey) | **DELETE** /keys/{set}/{kid} | Delete a JSON Web Key
+*HydraOAuth2OpenIdConnectServer.JsonWebKeyApi* | [**deleteJsonWebKeySet**](docs/JsonWebKeyApi.md#deleteJsonWebKeySet) | **DELETE** /keys/{set} | Delete a JSON Web Key
+*HydraOAuth2OpenIdConnectServer.JsonWebKeyApi* | [**getJsonWebKey**](docs/JsonWebKeyApi.md#getJsonWebKey) | **GET** /keys/{set}/{kid} | Retrieve a JSON Web Key
+*HydraOAuth2OpenIdConnectServer.JsonWebKeyApi* | [**getJsonWebKeySet**](docs/JsonWebKeyApi.md#getJsonWebKeySet) | **GET** /keys/{set} | Retrieve a JSON Web Key Set
+*HydraOAuth2OpenIdConnectServer.JsonWebKeyApi* | [**updateJsonWebKey**](docs/JsonWebKeyApi.md#updateJsonWebKey) | **PUT** /keys/{set}/{kid} | Update a JSON Web Key
+*HydraOAuth2OpenIdConnectServer.JsonWebKeyApi* | [**updateJsonWebKeySet**](docs/JsonWebKeyApi.md#updateJsonWebKeySet) | **PUT** /keys/{set} | Update a JSON Web Key Set
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**acceptOAuth2ConsentRequest**](docs/OAuth2Api.md#acceptOAuth2ConsentRequest) | **PATCH** /oauth2/consent/requests/{id}/accept | Accept a consent request
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**createOAuth2Client**](docs/OAuth2Api.md#createOAuth2Client) | **POST** /clients | Create an OAuth 2.0 client
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**deleteOAuth2Client**](docs/OAuth2Api.md#deleteOAuth2Client) | **DELETE** /clients/{id} | Deletes an OAuth 2.0 Client
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**getOAuth2Client**](docs/OAuth2Api.md#getOAuth2Client) | **GET** /clients/{id} | Retrieve an OAuth 2.0 Client.
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**getOAuth2ConsentRequest**](docs/OAuth2Api.md#getOAuth2ConsentRequest) | **GET** /oauth2/consent/requests/{id} | Receive consent request information
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**getWellKnown**](docs/OAuth2Api.md#getWellKnown) | **GET** /.well-known/openid-configuration | Server well known configuration
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**introspectOAuth2Token**](docs/OAuth2Api.md#introspectOAuth2Token) | **POST** /oauth2/introspect | Introspect OAuth2 tokens
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**listOAuth2Clients**](docs/OAuth2Api.md#listOAuth2Clients) | **GET** /clients | List OAuth 2.0 Clients
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**oauthAuth**](docs/OAuth2Api.md#oauthAuth) | **GET** /oauth2/auth | The OAuth 2.0 authorize endpoint
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**oauthToken**](docs/OAuth2Api.md#oauthToken) | **POST** /oauth2/token | The OAuth 2.0 token endpoint
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**rejectOAuth2ConsentRequest**](docs/OAuth2Api.md#rejectOAuth2ConsentRequest) | **PATCH** /oauth2/consent/requests/{id}/reject | Reject a consent request
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**revokeOAuth2Token**](docs/OAuth2Api.md#revokeOAuth2Token) | **POST** /oauth2/revoke | Revoke OAuth2 tokens
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**updateOAuth2Client**](docs/OAuth2Api.md#updateOAuth2Client) | **PUT** /clients/{id} | Update an OAuth 2.0 Client
+*HydraOAuth2OpenIdConnectServer.OAuth2Api* | [**wellKnown**](docs/OAuth2Api.md#wellKnown) | **GET** /.well-known/jwks.json | Get list of well known JSON Web Keys
+*HydraOAuth2OpenIdConnectServer.PolicyApi* | [**createPolicy**](docs/PolicyApi.md#createPolicy) | **POST** /policies | Create an Access Control Policy
+*HydraOAuth2OpenIdConnectServer.PolicyApi* | [**deletePolicy**](docs/PolicyApi.md#deletePolicy) | **DELETE** /policies/{id} | Delete an Access Control Policy
+*HydraOAuth2OpenIdConnectServer.PolicyApi* | [**getPolicy**](docs/PolicyApi.md#getPolicy) | **GET** /policies/{id} | Get an Access Control Policy
+*HydraOAuth2OpenIdConnectServer.PolicyApi* | [**listPolicies**](docs/PolicyApi.md#listPolicies) | **GET** /policies | List Access Control Policies
+*HydraOAuth2OpenIdConnectServer.PolicyApi* | [**updatePolicy**](docs/PolicyApi.md#updatePolicy) | **PUT** /policies/{id} | Update an Access Control Polic
+*HydraOAuth2OpenIdConnectServer.WardenApi* | [**addMembersToGroup**](docs/WardenApi.md#addMembersToGroup) | **POST** /warden/groups/{id}/members | Add members to a group
+*HydraOAuth2OpenIdConnectServer.WardenApi* | [**createGroup**](docs/WardenApi.md#createGroup) | **POST** /warden/groups | Create a group
+*HydraOAuth2OpenIdConnectServer.WardenApi* | [**deleteGroup**](docs/WardenApi.md#deleteGroup) | **DELETE** /warden/groups/{id} | Delete a group by id
+*HydraOAuth2OpenIdConnectServer.WardenApi* | [**doesWardenAllowAccessRequest**](docs/WardenApi.md#doesWardenAllowAccessRequest) | **POST** /warden/allowed | Check if an access request is valid (without providing an access token)
+*HydraOAuth2OpenIdConnectServer.WardenApi* | [**doesWardenAllowTokenAccessRequest**](docs/WardenApi.md#doesWardenAllowTokenAccessRequest) | **POST** /warden/token/allowed | Check if an access request is valid (providing an access token)
+*HydraOAuth2OpenIdConnectServer.WardenApi* | [**findGroupsByMember**](docs/WardenApi.md#findGroupsByMember) | **GET** /warden/groups | Find groups by member
+*HydraOAuth2OpenIdConnectServer.WardenApi* | [**getGroup**](docs/WardenApi.md#getGroup) | **GET** /warden/groups/{id} | Get a group by id
+*HydraOAuth2OpenIdConnectServer.WardenApi* | [**removeMembersFromGroup**](docs/WardenApi.md#removeMembersFromGroup) | **DELETE** /warden/groups/{id}/members | Remove members from a group
+
+
+## Documentation for Models
+
+ - [HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance](docs/ConsentRequestAcceptance.md)
+ - [HydraOAuth2OpenIdConnectServer.ConsentRequestManager](docs/ConsentRequestManager.md)
+ - [HydraOAuth2OpenIdConnectServer.ConsentRequestRejection](docs/ConsentRequestRejection.md)
+ - [HydraOAuth2OpenIdConnectServer.Context](docs/Context.md)
+ - [HydraOAuth2OpenIdConnectServer.Firewall](docs/Firewall.md)
+ - [HydraOAuth2OpenIdConnectServer.Group](docs/Group.md)
+ - [HydraOAuth2OpenIdConnectServer.GroupMembers](docs/GroupMembers.md)
+ - [HydraOAuth2OpenIdConnectServer.Handler](docs/Handler.md)
+ - [HydraOAuth2OpenIdConnectServer.InlineResponse200](docs/InlineResponse200.md)
+ - [HydraOAuth2OpenIdConnectServer.InlineResponse2001](docs/InlineResponse2001.md)
+ - [HydraOAuth2OpenIdConnectServer.InlineResponse401](docs/InlineResponse401.md)
+ - [HydraOAuth2OpenIdConnectServer.JoseWebKeySetRequest](docs/JoseWebKeySetRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.JsonWebKey](docs/JsonWebKey.md)
+ - [HydraOAuth2OpenIdConnectServer.JsonWebKeySet](docs/JsonWebKeySet.md)
+ - [HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest](docs/JsonWebKeySetGeneratorRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.KeyGenerator](docs/KeyGenerator.md)
+ - [HydraOAuth2OpenIdConnectServer.Manager](docs/Manager.md)
+ - [HydraOAuth2OpenIdConnectServer.OAuth2Client](docs/OAuth2Client.md)
+ - [HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection](docs/OAuth2TokenIntrospection.md)
+ - [HydraOAuth2OpenIdConnectServer.OAuth2consentRequest](docs/OAuth2consentRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.Policy](docs/Policy.md)
+ - [HydraOAuth2OpenIdConnectServer.PolicyConditions](docs/PolicyConditions.md)
+ - [HydraOAuth2OpenIdConnectServer.RawMessage](docs/RawMessage.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerAcceptConsentRequest](docs/SwaggerAcceptConsentRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerCreatePolicyParameters](docs/SwaggerCreatePolicyParameters.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowAccessRequestParameters](docs/SwaggerDoesWardenAllowAccessRequestParameters.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowTokenAccessRequestParameters](docs/SwaggerDoesWardenAllowTokenAccessRequestParameters.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerGetPolicyParameters](docs/SwaggerGetPolicyParameters.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerJsonWebKeyQuery](docs/SwaggerJsonWebKeyQuery.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerJwkCreateSet](docs/SwaggerJwkCreateSet.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerJwkSetQuery](docs/SwaggerJwkSetQuery.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSet](docs/SwaggerJwkUpdateSet.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey](docs/SwaggerJwkUpdateSetKey.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerListPolicyParameters](docs/SwaggerListPolicyParameters.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerListPolicyResponse](docs/SwaggerListPolicyResponse.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequest](docs/SwaggerOAuthConsentRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequestPayload](docs/SwaggerOAuthConsentRequestPayload.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionRequest](docs/SwaggerOAuthIntrospectionRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionResponse](docs/SwaggerOAuthIntrospectionResponse.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponse](docs/SwaggerOAuthTokenResponse.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody](docs/SwaggerOAuthTokenResponseBody.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerRejectConsentRequest](docs/SwaggerRejectConsentRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerRevokeOAuth2TokenParameters](docs/SwaggerRevokeOAuth2TokenParameters.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerUpdatePolicyParameters](docs/SwaggerUpdatePolicyParameters.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerWardenAccessRequestResponseParameters](docs/SwaggerWardenAccessRequestResponseParameters.md)
+ - [HydraOAuth2OpenIdConnectServer.SwaggerWardenTokenAccessRequestResponse](docs/SwaggerWardenTokenAccessRequestResponse.md)
+ - [HydraOAuth2OpenIdConnectServer.TokenAllowedRequest](docs/TokenAllowedRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.WardenAccessRequest](docs/WardenAccessRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse](docs/WardenAccessRequestResponse.md)
+ - [HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest](docs/WardenTokenAccessRequest.md)
+ - [HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload](docs/WardenTokenAccessRequestResponsePayload.md)
+ - [HydraOAuth2OpenIdConnectServer.WellKnown](docs/WellKnown.md)
+ - [HydraOAuth2OpenIdConnectServer.Writer](docs/Writer.md)
+
+
+## Documentation for Authorization
+
+
+### basic
+
+- **Type**: HTTP basic authentication
+
+### oauth2
+
+- **Type**: OAuth
+- **Flow**: accessCode
+- **Authorization URL**: https://your-hydra-instance.com/oauth2/auth
+- **Scopes**:
+ - hydra.clients: A scope required to manage OAuth 2.0 Clients
+ - hydra.consent: A scope required to fetch and modify consent requests
+ - hydra.groups: A scope required to manage warden groups
+ - hydra.health: A scope required to get health information
+ - hydra.keys.create: A scope required to create JSON Web Keys
+ - hydra.keys.delete: A scope required to delete JSON Web Keys
+ - hydra.keys.get: A scope required to fetch JSON Web Keys
+ - hydra.keys.update: A scope required to get JSON Web Keys
+ - hydra.policies: A scope required to manage access control policies
+ - hydra.warden: A scope required to make access control inquiries
+ - offline: A scope required when requesting refresh tokens
+ - openid: Request an OpenID Connect ID Token
+
diff --git a/sdk/js/swagger/docs/ConsentRequestAcceptance.md b/sdk/js/swagger/docs/ConsentRequestAcceptance.md
new file mode 100644
index 00000000000..8e53bc0e108
--- /dev/null
+++ b/sdk/js/swagger/docs/ConsentRequestAcceptance.md
@@ -0,0 +1,11 @@
+# HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**accessTokenExtra** | **{String: Object}** | AccessTokenExtra represents arbitrary data that will be added to the access token and that will be returned on introspection and warden requests. | [optional]
+**grantScopes** | **[String]** | A list of scopes that the user agreed to grant. It should be a subset of requestedScopes from the consent request. | [optional]
+**idTokenExtra** | **{String: Object}** | IDTokenExtra represents arbitrary data that will be added to the ID token. The ID token will only be issued if the user agrees to it and if the client requested an ID token. | [optional]
+**subject** | **String** | Subject represents a unique identifier of the user (or service, or legal entity, ...) that accepted the OAuth2 request. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/ConsentRequestManager.md b/sdk/js/swagger/docs/ConsentRequestManager.md
new file mode 100644
index 00000000000..1f4f7ab10c5
--- /dev/null
+++ b/sdk/js/swagger/docs/ConsentRequestManager.md
@@ -0,0 +1,7 @@
+# HydraOAuth2OpenIdConnectServer.ConsentRequestManager
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+
diff --git a/sdk/js/swagger/docs/ConsentRequestRejection.md b/sdk/js/swagger/docs/ConsentRequestRejection.md
new file mode 100644
index 00000000000..f847951907e
--- /dev/null
+++ b/sdk/js/swagger/docs/ConsentRequestRejection.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.ConsentRequestRejection
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**reason** | **String** | Reason represents the reason why the user rejected the consent request. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/Context.md b/sdk/js/swagger/docs/Context.md
new file mode 100644
index 00000000000..f66aa14da0d
--- /dev/null
+++ b/sdk/js/swagger/docs/Context.md
@@ -0,0 +1,12 @@
+# HydraOAuth2OpenIdConnectServer.Context
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**aud** | **String** | Audience is who the token was issued for. This is an OAuth2 app usually. | [optional]
+**ext** | **{String: Object}** | Extra represents arbitrary session data. | [optional]
+**iss** | **String** | Issuer is the id of the issuer, typically an hydra instance. | [optional]
+**scopes** | **[String]** | GrantedScopes is a list of scopes that the subject authorized when asked for consent. | [optional]
+**sub** | **String** | Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app. This is usually a uuid but you can choose a urn or some other id too. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/Firewall.md b/sdk/js/swagger/docs/Firewall.md
new file mode 100644
index 00000000000..688ee86b506
--- /dev/null
+++ b/sdk/js/swagger/docs/Firewall.md
@@ -0,0 +1,7 @@
+# HydraOAuth2OpenIdConnectServer.Firewall
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+
diff --git a/sdk/js/swagger/docs/Group.md b/sdk/js/swagger/docs/Group.md
new file mode 100644
index 00000000000..81369468012
--- /dev/null
+++ b/sdk/js/swagger/docs/Group.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.Group
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**id** | **String** | ID is the groups id. | [optional]
+**members** | **[String]** | Members is who belongs to the group. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/GroupMembers.md b/sdk/js/swagger/docs/GroupMembers.md
new file mode 100644
index 00000000000..f34092f18e9
--- /dev/null
+++ b/sdk/js/swagger/docs/GroupMembers.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.GroupMembers
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**members** | **[String]** | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/Handler.md b/sdk/js/swagger/docs/Handler.md
new file mode 100644
index 00000000000..ca45bb05dee
--- /dev/null
+++ b/sdk/js/swagger/docs/Handler.md
@@ -0,0 +1,11 @@
+# HydraOAuth2OpenIdConnectServer.Handler
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**generators** | [**{String: KeyGenerator}**](KeyGenerator.md) | | [optional]
+**H** | [**Writer**](Writer.md) | | [optional]
+**manager** | [**Manager**](Manager.md) | | [optional]
+**W** | [**Firewall**](Firewall.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/HealthApi.md b/sdk/js/swagger/docs/HealthApi.md
new file mode 100644
index 00000000000..49b54f5af2f
--- /dev/null
+++ b/sdk/js/swagger/docs/HealthApi.md
@@ -0,0 +1,95 @@
+# HydraOAuth2OpenIdConnectServer.HealthApi
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**getInstanceMetrics**](HealthApi.md#getInstanceMetrics) | **GET** /health/metrics | Show instance metrics (experimental)
+[**getInstanceStatus**](HealthApi.md#getInstanceStatus) | **GET** /health/status | Check health status of this instance
+
+
+
+# **getInstanceMetrics**
+> getInstanceMetrics()
+
+Show instance metrics (experimental)
+
+This endpoint returns an instance's metrics, such as average response time, status code distribution, hits per second and so on. The return values are currently not documented as this endpoint is still experimental. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:health:stats\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.HealthApi();
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.getInstanceMetrics(callback);
+```
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **getInstanceStatus**
+> InlineResponse200 getInstanceStatus()
+
+Check health status of this instance
+
+This endpoint returns `{ \"status\": \"ok\" }`. This status let's you know that the HTTP server is up and running. This status does currently not include checks whether the database connection is up and running. This endpoint does not require the `X-Forwarded-Proto` header when TLS termination is set. Be aware that if you are running multiple nodes of ORY Hydra, the health status will never refer to the cluster state, only to a single instance.
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.HealthApi();
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.getInstanceStatus(callback);
+```
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**InlineResponse200**](InlineResponse200.md)
+
+### Authorization
+
+No authorization required
+
+### HTTP request headers
+
+ - **Content-Type**: application/json, application/x-www-form-urlencoded
+ - **Accept**: application/json
+
diff --git a/sdk/js/swagger/docs/InlineResponse200.md b/sdk/js/swagger/docs/InlineResponse200.md
new file mode 100644
index 00000000000..80c46a82b66
--- /dev/null
+++ b/sdk/js/swagger/docs/InlineResponse200.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.InlineResponse200
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**status** | **String** | Status always contains \"ok\" | [optional]
+
+
diff --git a/sdk/js/swagger/docs/InlineResponse2001.md b/sdk/js/swagger/docs/InlineResponse2001.md
new file mode 100644
index 00000000000..967ee180005
--- /dev/null
+++ b/sdk/js/swagger/docs/InlineResponse2001.md
@@ -0,0 +1,13 @@
+# HydraOAuth2OpenIdConnectServer.InlineResponse2001
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**accessToken** | **String** | The access token issued by the authorization server. | [optional]
+**expiresIn** | **Number** | The lifetime in seconds of the access token. For example, the value \"3600\" denotes that the access token will expire in one hour from the time the response was generated. | [optional]
+**idToken** | **Number** | To retrieve a refresh token request the id_token scope. | [optional]
+**refreshToken** | **String** | The refresh token, which can be used to obtain new access tokens. To retrieve it add the scope \"offline\" to your access token request. | [optional]
+**scope** | **Number** | The scope of the access token | [optional]
+**tokenType** | **String** | The type of the token issued | [optional]
+
+
diff --git a/sdk/js/swagger/docs/InlineResponse401.md b/sdk/js/swagger/docs/InlineResponse401.md
new file mode 100644
index 00000000000..e425d45afbf
--- /dev/null
+++ b/sdk/js/swagger/docs/InlineResponse401.md
@@ -0,0 +1,13 @@
+# HydraOAuth2OpenIdConnectServer.InlineResponse401
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**code** | **Number** | | [optional]
+**details** | **[{String: Object}]** | | [optional]
+**message** | **String** | | [optional]
+**reason** | **String** | | [optional]
+**request** | **String** | | [optional]
+**status** | **String** | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/JoseWebKeySetRequest.md b/sdk/js/swagger/docs/JoseWebKeySetRequest.md
new file mode 100644
index 00000000000..0c9e4787388
--- /dev/null
+++ b/sdk/js/swagger/docs/JoseWebKeySetRequest.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.JoseWebKeySetRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**keys** | [**[RawMessage]**](RawMessage.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/JsonWebKey.md b/sdk/js/swagger/docs/JsonWebKey.md
new file mode 100644
index 00000000000..ff9db75a2be
--- /dev/null
+++ b/sdk/js/swagger/docs/JsonWebKey.md
@@ -0,0 +1,24 @@
+# HydraOAuth2OpenIdConnectServer.JsonWebKey
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**alg** | **String** | The \"alg\" (algorithm) parameter identifies the algorithm intended for use with the key. The values used should either be registered in the IANA \"JSON Web Signature and Encryption Algorithms\" registry established by [JWA] or be a value that contains a Collision- Resistant Name. | [optional]
+**crv** | **String** | | [optional]
+**d** | **String** | | [optional]
+**dp** | **String** | | [optional]
+**dq** | **String** | | [optional]
+**e** | **String** | | [optional]
+**k** | **String** | | [optional]
+**kid** | **String** | The \"kid\" (key ID) parameter is used to match a specific key. This is used, for instance, to choose among a set of keys within a JWK Set during key rollover. The structure of the \"kid\" value is unspecified. When \"kid\" values are used within a JWK Set, different keys within the JWK Set SHOULD use distinct \"kid\" values. (One example in which different keys might use the same \"kid\" value is if they have different \"kty\" (key type) values but are considered to be equivalent alternatives by the application using them.) The \"kid\" value is a case-sensitive string. | [optional]
+**kty** | **String** | The \"kty\" (key type) parameter identifies the cryptographic algorithm family used with the key, such as \"RSA\" or \"EC\". \"kty\" values should either be registered in the IANA \"JSON Web Key Types\" registry established by [JWA] or be a value that contains a Collision- Resistant Name. The \"kty\" value is a case-sensitive string. | [optional]
+**n** | **String** | | [optional]
+**p** | **String** | | [optional]
+**q** | **String** | | [optional]
+**qi** | **String** | | [optional]
+**use** | **String** | The \"use\" (public key use) parameter identifies the intended use of the public key. The \"use\" parameter is employed to indicate whether a public key is used for encrypting data or verifying the signature on data. Values are commonly \"sig\" (signature) or \"enc\" (encryption). | [optional]
+**x** | **String** | | [optional]
+**x5c** | **[String]** | The \"x5c\" (X.509 certificate chain) parameter contains a chain of one or more PKIX certificates [RFC5280]. The certificate chain is represented as a JSON array of certificate value strings. Each string in the array is a base64-encoded (Section 4 of [RFC4648] -- not base64url-encoded) DER [ITU.X690.1994] PKIX certificate value. The PKIX certificate containing the key value MUST be the first certificate. | [optional]
+**y** | **String** | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/JsonWebKeyApi.md b/sdk/js/swagger/docs/JsonWebKeyApi.md
new file mode 100644
index 00000000000..738cb9aba3f
--- /dev/null
+++ b/sdk/js/swagger/docs/JsonWebKeyApi.md
@@ -0,0 +1,393 @@
+# HydraOAuth2OpenIdConnectServer.JsonWebKeyApi
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**createJsonWebKeySet**](JsonWebKeyApi.md#createJsonWebKeySet) | **POST** /keys/{set} | Generate a new JSON Web Key
+[**deleteJsonWebKey**](JsonWebKeyApi.md#deleteJsonWebKey) | **DELETE** /keys/{set}/{kid} | Delete a JSON Web Key
+[**deleteJsonWebKeySet**](JsonWebKeyApi.md#deleteJsonWebKeySet) | **DELETE** /keys/{set} | Delete a JSON Web Key
+[**getJsonWebKey**](JsonWebKeyApi.md#getJsonWebKey) | **GET** /keys/{set}/{kid} | Retrieve a JSON Web Key
+[**getJsonWebKeySet**](JsonWebKeyApi.md#getJsonWebKeySet) | **GET** /keys/{set} | Retrieve a JSON Web Key Set
+[**updateJsonWebKey**](JsonWebKeyApi.md#updateJsonWebKey) | **PUT** /keys/{set}/{kid} | Update a JSON Web Key
+[**updateJsonWebKeySet**](JsonWebKeyApi.md#updateJsonWebKeySet) | **PUT** /keys/{set} | Update a JSON Web Key Set
+
+
+
+# **createJsonWebKeySet**
+> JsonWebKeySet createJsonWebKeySet(set, opts)
+
+Generate a new JSON Web Key
+
+This endpoint is capable of generating JSON Web Key Sets for you. There a different strategies available, such as symmetric cryptographic keys (HS256) and asymetric cryptographic keys (RS256, ECDSA). If the specified JSON Web Key Set does not exist, it will be created. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.JsonWebKeyApi();
+
+var set = "set_example"; // String | The set
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest() // JsonWebKeySetGeneratorRequest |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.createJsonWebKeySet(set, opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **set** | **String**| The set |
+ **body** | [**JsonWebKeySetGeneratorRequest**](JsonWebKeySetGeneratorRequest.md)| | [optional]
+
+### Return type
+
+[**JsonWebKeySet**](JsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **deleteJsonWebKey**
+> deleteJsonWebKey(kid, set)
+
+Delete a JSON Web Key
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.JsonWebKeyApi();
+
+var kid = "kid_example"; // String | The kid of the desired key
+
+var set = "set_example"; // String | The set
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.deleteJsonWebKey(kid, set, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **kid** | **String**| The kid of the desired key |
+ **set** | **String**| The set |
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **deleteJsonWebKeySet**
+> deleteJsonWebKeySet(set)
+
+Delete a JSON Web Key
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.JsonWebKeyApi();
+
+var set = "set_example"; // String | The set
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.deleteJsonWebKeySet(set, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **set** | **String**| The set |
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **getJsonWebKey**
+> JsonWebKeySet getJsonWebKey(kid, set)
+
+Retrieve a JSON Web Key
+
+This endpoint can be used to retrieve JWKs stored in ORY Hydra. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.JsonWebKeyApi();
+
+var kid = "kid_example"; // String | The kid of the desired key
+
+var set = "set_example"; // String | The set
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.getJsonWebKey(kid, set, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **kid** | **String**| The kid of the desired key |
+ **set** | **String**| The set |
+
+### Return type
+
+[**JsonWebKeySet**](JsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **getJsonWebKeySet**
+> JsonWebKeySet getJsonWebKeySet(set)
+
+Retrieve a JSON Web Key Set
+
+This endpoint can be used to retrieve JWK Sets stored in ORY Hydra. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.JsonWebKeyApi();
+
+var set = "set_example"; // String | The set
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.getJsonWebKeySet(set, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **set** | **String**| The set |
+
+### Return type
+
+[**JsonWebKeySet**](JsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **updateJsonWebKey**
+> JsonWebKey updateJsonWebKey(kid, set, opts)
+
+Update a JSON Web Key
+
+Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.JsonWebKeyApi();
+
+var kid = "kid_example"; // String | The kid of the desired key
+
+var set = "set_example"; // String | The set
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.JsonWebKey() // JsonWebKey |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.updateJsonWebKey(kid, set, opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **kid** | **String**| The kid of the desired key |
+ **set** | **String**| The set |
+ **body** | [**JsonWebKey**](JsonWebKey.md)| | [optional]
+
+### Return type
+
+[**JsonWebKey**](JsonWebKey.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **updateJsonWebKeySet**
+> JsonWebKeySet updateJsonWebKeySet(set, opts)
+
+Update a JSON Web Key Set
+
+Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.JsonWebKeyApi();
+
+var set = "set_example"; // String | The set
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.JsonWebKeySet() // JsonWebKeySet |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.updateJsonWebKeySet(set, opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **set** | **String**| The set |
+ **body** | [**JsonWebKeySet**](JsonWebKeySet.md)| | [optional]
+
+### Return type
+
+[**JsonWebKeySet**](JsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
diff --git a/sdk/js/swagger/docs/JsonWebKeySet.md b/sdk/js/swagger/docs/JsonWebKeySet.md
new file mode 100644
index 00000000000..f86bb081631
--- /dev/null
+++ b/sdk/js/swagger/docs/JsonWebKeySet.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.JsonWebKeySet
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**keys** | [**[JsonWebKey]**](JsonWebKey.md) | The value of the \"keys\" parameter is an array of JWK values. By default, the order of the JWK values within the array does not imply an order of preference among them, although applications of JWK Sets can choose to assign a meaning to the order for their purposes, if desired. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/JsonWebKeySetGeneratorRequest.md b/sdk/js/swagger/docs/JsonWebKeySetGeneratorRequest.md
new file mode 100644
index 00000000000..31d45de88cf
--- /dev/null
+++ b/sdk/js/swagger/docs/JsonWebKeySetGeneratorRequest.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**alg** | **String** | The algorithm to be used for creating the key. Supports \"RS256\", \"ES521\" and \"HS256\" |
+**kid** | **String** | The kid of the key to be created |
+
+
diff --git a/sdk/js/swagger/docs/KeyGenerator.md b/sdk/js/swagger/docs/KeyGenerator.md
new file mode 100644
index 00000000000..bc8feb36003
--- /dev/null
+++ b/sdk/js/swagger/docs/KeyGenerator.md
@@ -0,0 +1,7 @@
+# HydraOAuth2OpenIdConnectServer.KeyGenerator
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+
diff --git a/sdk/js/swagger/docs/Manager.md b/sdk/js/swagger/docs/Manager.md
new file mode 100644
index 00000000000..933c5b50f7a
--- /dev/null
+++ b/sdk/js/swagger/docs/Manager.md
@@ -0,0 +1,7 @@
+# HydraOAuth2OpenIdConnectServer.Manager
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+
diff --git a/sdk/js/swagger/docs/OAuth2Api.md b/sdk/js/swagger/docs/OAuth2Api.md
new file mode 100644
index 00000000000..dc6243e2c8e
--- /dev/null
+++ b/sdk/js/swagger/docs/OAuth2Api.md
@@ -0,0 +1,720 @@
+# HydraOAuth2OpenIdConnectServer.OAuth2Api
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**acceptOAuth2ConsentRequest**](OAuth2Api.md#acceptOAuth2ConsentRequest) | **PATCH** /oauth2/consent/requests/{id}/accept | Accept a consent request
+[**createOAuth2Client**](OAuth2Api.md#createOAuth2Client) | **POST** /clients | Create an OAuth 2.0 client
+[**deleteOAuth2Client**](OAuth2Api.md#deleteOAuth2Client) | **DELETE** /clients/{id} | Deletes an OAuth 2.0 Client
+[**getOAuth2Client**](OAuth2Api.md#getOAuth2Client) | **GET** /clients/{id} | Retrieve an OAuth 2.0 Client.
+[**getOAuth2ConsentRequest**](OAuth2Api.md#getOAuth2ConsentRequest) | **GET** /oauth2/consent/requests/{id} | Receive consent request information
+[**getWellKnown**](OAuth2Api.md#getWellKnown) | **GET** /.well-known/openid-configuration | Server well known configuration
+[**introspectOAuth2Token**](OAuth2Api.md#introspectOAuth2Token) | **POST** /oauth2/introspect | Introspect OAuth2 tokens
+[**listOAuth2Clients**](OAuth2Api.md#listOAuth2Clients) | **GET** /clients | List OAuth 2.0 Clients
+[**oauthAuth**](OAuth2Api.md#oauthAuth) | **GET** /oauth2/auth | The OAuth 2.0 authorize endpoint
+[**oauthToken**](OAuth2Api.md#oauthToken) | **POST** /oauth2/token | The OAuth 2.0 token endpoint
+[**rejectOAuth2ConsentRequest**](OAuth2Api.md#rejectOAuth2ConsentRequest) | **PATCH** /oauth2/consent/requests/{id}/reject | Reject a consent request
+[**revokeOAuth2Token**](OAuth2Api.md#revokeOAuth2Token) | **POST** /oauth2/revoke | Revoke OAuth2 tokens
+[**updateOAuth2Client**](OAuth2Api.md#updateOAuth2Client) | **PUT** /clients/{id} | Update an OAuth 2.0 Client
+[**wellKnown**](OAuth2Api.md#wellKnown) | **GET** /.well-known/jwks.json | Get list of well known JSON Web Keys
+
+
+
+# **acceptOAuth2ConsentRequest**
+> acceptOAuth2ConsentRequest(id, body)
+
+Accept a consent request
+
+Call this endpoint to accept a consent request. This usually happens when a user agrees to give access rights to an application. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"accept\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var id = "id_example"; // String |
+
+var body = new HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance(); // ConsentRequestAcceptance |
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.acceptOAuth2ConsentRequest(id, body, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| |
+ **body** | [**ConsentRequestAcceptance**](ConsentRequestAcceptance.md)| |
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **createOAuth2Client**
+> OAuth2Client createOAuth2Client(body)
+
+Create an OAuth 2.0 client
+
+If you pass `client_secret` the secret will be used, otherwise a random secret will be generated. The secret will be returned in the response and you will not be able to retrieve it later on. Write the secret down and keep it somwhere safe. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"create\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var body = new HydraOAuth2OpenIdConnectServer.OAuth2Client(); // OAuth2Client |
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.createOAuth2Client(body, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**OAuth2Client**](OAuth2Client.md)| |
+
+### Return type
+
+[**OAuth2Client**](OAuth2Client.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **deleteOAuth2Client**
+> deleteOAuth2Client(id)
+
+Deletes an OAuth 2.0 Client
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var id = "id_example"; // String | The id of the OAuth 2.0 Client.
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.deleteOAuth2Client(id, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the OAuth 2.0 Client. |
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **getOAuth2Client**
+> OAuth2Client getOAuth2Client(id)
+
+Retrieve an OAuth 2.0 Client.
+
+This endpoint never returns passwords. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var id = "id_example"; // String | The id of the OAuth 2.0 Client.
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.getOAuth2Client(id, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the OAuth 2.0 Client. |
+
+### Return type
+
+[**OAuth2Client**](OAuth2Client.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **getOAuth2ConsentRequest**
+> OAuth2consentRequest getOAuth2ConsentRequest(id)
+
+Receive consent request information
+
+Call this endpoint to receive information on consent requests. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var id = "id_example"; // String | The id of the OAuth 2.0 Consent Request.
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.getOAuth2ConsentRequest(id, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the OAuth 2.0 Consent Request. |
+
+### Return type
+
+[**OAuth2consentRequest**](OAuth2consentRequest.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **getWellKnown**
+> WellKnown getWellKnown()
+
+Server well known configuration
+
+The well known endpoint an be used to retrieve information for OpenID Connect clients. We encourage you to not roll your own OpenID Connect client but to use an OpenID Connect client library instead. You can learn more on this flow at https://openid.net/specs/openid-connect-discovery-1_0.html
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.getWellKnown(callback);
+```
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**WellKnown**](WellKnown.md)
+
+### Authorization
+
+No authorization required
+
+### HTTP request headers
+
+ - **Content-Type**: application/json, application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+
+# **introspectOAuth2Token**
+> OAuth2TokenIntrospection introspectOAuth2Token(token, opts)
+
+Introspect OAuth2 tokens
+
+The introspection endpoint allows to check if a token (both refresh and access) is active or not. An active token is neither expired nor revoked. If a token is active, additional information on the token will be included. You can set additional data for a token by setting `accessTokenExtra` during the consent flow.
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure HTTP basic authorization: basic
+var basic = defaultClient.authentications['basic'];
+basic.username = 'YOUR USERNAME';
+basic.password = 'YOUR PASSWORD';
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var token = "token_example"; // String | The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation.
+
+var opts = {
+ 'scope': "scope_example" // String | An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false.
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.introspectOAuth2Token(token, opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **token** | **String**| The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation. |
+ **scope** | **String**| An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false. | [optional]
+
+### Return type
+
+[**OAuth2TokenIntrospection**](OAuth2TokenIntrospection.md)
+
+### Authorization
+
+[basic](../README.md#basic), [oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+
+# **listOAuth2Clients**
+> [OAuth2Client] listOAuth2Clients()
+
+List OAuth 2.0 Clients
+
+This endpoint never returns passwords. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.listOAuth2Clients(callback);
+```
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**[OAuth2Client]**](OAuth2Client.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **oauthAuth**
+> oauthAuth()
+
+The OAuth 2.0 authorize endpoint
+
+This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows. OAuth2 is a very popular protocol and a library for your programming language will exists. To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.oauthAuth(callback);
+```
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+No authorization required
+
+### HTTP request headers
+
+ - **Content-Type**: application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+
+# **oauthToken**
+> InlineResponse2001 oauthToken()
+
+The OAuth 2.0 token endpoint
+
+This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows. OAuth2 is a very popular protocol and a library for your programming language will exists. To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure HTTP basic authorization: basic
+var basic = defaultClient.authentications['basic'];
+basic.username = 'YOUR USERNAME';
+basic.password = 'YOUR PASSWORD';
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.oauthToken(callback);
+```
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**InlineResponse2001**](InlineResponse2001.md)
+
+### Authorization
+
+[basic](../README.md#basic), [oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+
+# **rejectOAuth2ConsentRequest**
+> rejectOAuth2ConsentRequest(id, body)
+
+Reject a consent request
+
+Call this endpoint to reject a consent request. This usually happens when a user denies access rights to an application. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"reject\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var id = "id_example"; // String |
+
+var body = new HydraOAuth2OpenIdConnectServer.ConsentRequestRejection(); // ConsentRequestRejection |
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.rejectOAuth2ConsentRequest(id, body, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| |
+ **body** | [**ConsentRequestRejection**](ConsentRequestRejection.md)| |
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **revokeOAuth2Token**
+> revokeOAuth2Token(token)
+
+Revoke OAuth2 tokens
+
+Revoking a token (both access and refresh) means that the tokens will be invalid. A revoked access token can no longer be used to make access requests, and a revoked refresh token can no longer be used to refresh an access token. Revoking a refresh token also invalidates the access token that was created with it.
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure HTTP basic authorization: basic
+var basic = defaultClient.authentications['basic'];
+basic.username = 'YOUR USERNAME';
+basic.password = 'YOUR PASSWORD';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var token = "token_example"; // String |
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.revokeOAuth2Token(token, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **token** | **String**| |
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[basic](../README.md#basic)
+
+### HTTP request headers
+
+ - **Content-Type**: application/x-www-form-urlencoded
+ - **Accept**: application/json
+
+
+# **updateOAuth2Client**
+> OAuth2Client updateOAuth2Client(id, body)
+
+Update an OAuth 2.0 Client
+
+If you pass `client_secret` the secret will be updated and returned via the API. This is the only time you will be able to retrieve the client secret, so write it down and keep it safe. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"update\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var id = "id_example"; // String |
+
+var body = new HydraOAuth2OpenIdConnectServer.OAuth2Client(); // OAuth2Client |
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.updateOAuth2Client(id, body, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| |
+ **body** | [**OAuth2Client**](OAuth2Client.md)| |
+
+### Return type
+
+[**OAuth2Client**](OAuth2Client.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **wellKnown**
+> JsonWebKeySet wellKnown()
+
+Get list of well known JSON Web Keys
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:hydra.openid.id-token:public\"], \"actions\": [\"GET\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.wellKnown(callback);
+```
+
+### Parameters
+This endpoint does not need any parameter.
+
+### Return type
+
+[**JsonWebKeySet**](JsonWebKeySet.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
diff --git a/sdk/js/swagger/docs/OAuth2Client.md b/sdk/js/swagger/docs/OAuth2Client.md
new file mode 100644
index 00000000000..150af79a60c
--- /dev/null
+++ b/sdk/js/swagger/docs/OAuth2Client.md
@@ -0,0 +1,21 @@
+# HydraOAuth2OpenIdConnectServer.OAuth2Client
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**clientName** | **String** | Name is the human-readable string name of the client to be presented to the end-user during authorization. | [optional]
+**clientSecret** | **String** | Secret is the client's secret. The secret will be included in the create request as cleartext, and then never again. The secret is stored using BCrypt so it is impossible to recover it. Tell your users that they need to write the secret down as it will not be made available again. | [optional]
+**clientUri** | **String** | ClientURI is an URL string of a web page providing information about the client. If present, the server SHOULD display this URL to the end-user in a clickable fashion. | [optional]
+**contacts** | **[String]** | Contacts is a array of strings representing ways to contact people responsible for this client, typically email addresses. | [optional]
+**grantTypes** | **[String]** | GrantTypes is an array of grant types the client is allowed to use. | [optional]
+**id** | **String** | ID is the id for this client. | [optional]
+**logoUri** | **String** | LogoURI is an URL string that references a logo for the client. | [optional]
+**owner** | **String** | Owner is a string identifying the owner of the OAuth 2.0 Client. | [optional]
+**policyUri** | **String** | PolicyURI is a URL string that points to a human-readable privacy policy document that describes how the deployment organization collects, uses, retains, and discloses personal data. | [optional]
+**_public** | **Boolean** | Public is a boolean that identifies this client as public, meaning that it does not have a secret. It will disable the client_credentials grant type for this client if set. | [optional]
+**redirectUris** | **[String]** | RedirectURIs is an array of allowed redirect urls for the client, for example: http://mydomain/oauth/callback . | [optional]
+**responseTypes** | **[String]** | ResponseTypes is an array of the OAuth 2.0 response type strings that the client can use at the authorization endpoint. | [optional]
+**scope** | **String** | Scope is a string containing a space-separated list of scope values (as described in Section 3.3 of OAuth 2.0 [RFC6749]) that the client can use when requesting access tokens. | [optional]
+**tosUri** | **String** | TermsOfServiceURI is a URL string that points to a human-readable terms of service document for the client that describes a contractual relationship between the end-user and the client that the end-user accepts when authorizing the client. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/OAuth2TokenIntrospection.md b/sdk/js/swagger/docs/OAuth2TokenIntrospection.md
new file mode 100644
index 00000000000..82619f73d62
--- /dev/null
+++ b/sdk/js/swagger/docs/OAuth2TokenIntrospection.md
@@ -0,0 +1,18 @@
+# HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**active** | **Boolean** | Active is a boolean indicator of whether or not the presented token is currently active. The specifics of a token's \"active\" state will vary depending on the implementation of the authorization server and the information it keeps about its tokens, but a \"true\" value return for the \"active\" property will generally indicate that a given token has been issued by this authorization server, has not been revoked by the resource owner, and is within its given time window of validity (e.g., after its issuance time and before its expiration time). | [optional]
+**aud** | **String** | Audience is a service-specific string identifier or list of string identifiers representing the intended audience for this token. | [optional]
+**clientId** | **String** | ClientID is aclient identifier for the OAuth 2.0 client that requested this token. | [optional]
+**exp** | **Number** | Expires at is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token will expire. | [optional]
+**ext** | **{String: Object}** | Extra is arbitrary data set by the session. | [optional]
+**iat** | **Number** | Issued at is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token was originally issued. | [optional]
+**iss** | **String** | Issuer is a string representing the issuer of this token | [optional]
+**nbf** | **Number** | NotBefore is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token is not to be used before. | [optional]
+**scope** | **String** | Scope is a JSON string containing a space-separated list of scopes associated with this token. | [optional]
+**sub** | **String** | Subject of the token, as defined in JWT [RFC7519]. Usually a machine-readable identifier of the resource owner who authorized this token. | [optional]
+**username** | **String** | Username is a human-readable identifier for the resource owner who authorized this token. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/OAuth2consentRequest.md b/sdk/js/swagger/docs/OAuth2consentRequest.md
new file mode 100644
index 00000000000..661e8465c6a
--- /dev/null
+++ b/sdk/js/swagger/docs/OAuth2consentRequest.md
@@ -0,0 +1,11 @@
+# HydraOAuth2OpenIdConnectServer.OAuth2consentRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**audience** | **String** | Audience is the client id that initiated the OAuth2 request. | [optional]
+**id** | **String** | ID is the id of this consent request. | [optional]
+**redirectUrl** | **String** | Redirect URL is the URL where the user agent should be redirected to after the consent has been accepted or rejected. | [optional]
+**requestedScopes** | **[String]** | RequestedScopes represents a list of scopes that have been requested by the OAuth2 request initiator. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/Policy.md b/sdk/js/swagger/docs/Policy.md
new file mode 100644
index 00000000000..4ff726d6419
--- /dev/null
+++ b/sdk/js/swagger/docs/Policy.md
@@ -0,0 +1,14 @@
+# HydraOAuth2OpenIdConnectServer.Policy
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**actions** | **[String]** | Actions impacted by the policy. | [optional]
+**conditions** | [**{String: PolicyConditions}**](PolicyConditions.md) | Conditions under which the policy is active. | [optional]
+**description** | **String** | Description of the policy. | [optional]
+**effect** | **String** | Effect of the policy | [optional]
+**id** | **String** | ID of the policy. | [optional]
+**resources** | **[String]** | Resources impacted by the policy. | [optional]
+**subjects** | **[String]** | Subjects impacted by the policy. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/PolicyApi.md b/sdk/js/swagger/docs/PolicyApi.md
new file mode 100644
index 00000000000..8153742fbb8
--- /dev/null
+++ b/sdk/js/swagger/docs/PolicyApi.md
@@ -0,0 +1,276 @@
+# HydraOAuth2OpenIdConnectServer.PolicyApi
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**createPolicy**](PolicyApi.md#createPolicy) | **POST** /policies | Create an Access Control Policy
+[**deletePolicy**](PolicyApi.md#deletePolicy) | **DELETE** /policies/{id} | Delete an Access Control Policy
+[**getPolicy**](PolicyApi.md#getPolicy) | **GET** /policies/{id} | Get an Access Control Policy
+[**listPolicies**](PolicyApi.md#listPolicies) | **GET** /policies | List Access Control Policies
+[**updatePolicy**](PolicyApi.md#updatePolicy) | **PUT** /policies/{id} | Update an Access Control Polic
+
+
+
+# **createPolicy**
+> Policy createPolicy(opts)
+
+Create an Access Control Policy
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.PolicyApi();
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.Policy() // Policy |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.createPolicy(opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**Policy**](Policy.md)| | [optional]
+
+### Return type
+
+[**Policy**](Policy.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **deletePolicy**
+> deletePolicy(id)
+
+Delete an Access Control Policy
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies:<id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.PolicyApi();
+
+var id = "id_example"; // String | The id of the policy.
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.deletePolicy(id, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the policy. |
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **getPolicy**
+> Policy getPolicy(id)
+
+Get an Access Control Policy
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies:<id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.PolicyApi();
+
+var id = "id_example"; // String | The id of the policy.
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.getPolicy(id, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the policy. |
+
+### Return type
+
+[**Policy**](Policy.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **listPolicies**
+> [Policy] listPolicies(opts)
+
+List Access Control Policies
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"list\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.PolicyApi();
+
+var opts = {
+ 'offset': 789, // Number | The offset from where to start looking.
+ 'limit': 789 // Number | The maximum amount of policies returned.
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.listPolicies(opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **offset** | **Number**| The offset from where to start looking. | [optional]
+ **limit** | **Number**| The maximum amount of policies returned. | [optional]
+
+### Return type
+
+[**[Policy]**](Policy.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **updatePolicy**
+> Policy updatePolicy(id, opts)
+
+Update an Access Control Polic
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.PolicyApi();
+
+var id = "id_example"; // String | The id of the policy.
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.Policy() // Policy |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.updatePolicy(id, opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the policy. |
+ **body** | [**Policy**](Policy.md)| | [optional]
+
+### Return type
+
+[**Policy**](Policy.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
diff --git a/sdk/js/swagger/docs/PolicyConditions.md b/sdk/js/swagger/docs/PolicyConditions.md
new file mode 100644
index 00000000000..51cb5ac7052
--- /dev/null
+++ b/sdk/js/swagger/docs/PolicyConditions.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.PolicyConditions
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**options** | **{String: Object}** | | [optional]
+**type** | **String** | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/RawMessage.md b/sdk/js/swagger/docs/RawMessage.md
new file mode 100644
index 00000000000..95658576fa1
--- /dev/null
+++ b/sdk/js/swagger/docs/RawMessage.md
@@ -0,0 +1,7 @@
+# HydraOAuth2OpenIdConnectServer.RawMessage
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+
diff --git a/sdk/js/swagger/docs/SwaggerAcceptConsentRequest.md b/sdk/js/swagger/docs/SwaggerAcceptConsentRequest.md
new file mode 100644
index 00000000000..2c948aeead3
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerAcceptConsentRequest.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerAcceptConsentRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**ConsentRequestAcceptance**](ConsentRequestAcceptance.md) | |
+**id** | **String** | in: path |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerCreatePolicyParameters.md b/sdk/js/swagger/docs/SwaggerCreatePolicyParameters.md
new file mode 100644
index 00000000000..edd5a659ab6
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerCreatePolicyParameters.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerCreatePolicyParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**Policy**](Policy.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerDoesWardenAllowAccessRequestParameters.md b/sdk/js/swagger/docs/SwaggerDoesWardenAllowAccessRequestParameters.md
new file mode 100644
index 00000000000..19af1a29f22
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerDoesWardenAllowAccessRequestParameters.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowAccessRequestParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**WardenAccessRequest**](WardenAccessRequest.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerDoesWardenAllowTokenAccessRequestParameters.md b/sdk/js/swagger/docs/SwaggerDoesWardenAllowTokenAccessRequestParameters.md
new file mode 100644
index 00000000000..fd6b851a802
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerDoesWardenAllowTokenAccessRequestParameters.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowTokenAccessRequestParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**WardenTokenAccessRequest**](WardenTokenAccessRequest.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerGetPolicyParameters.md b/sdk/js/swagger/docs/SwaggerGetPolicyParameters.md
new file mode 100644
index 00000000000..5bd2dfd7f09
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerGetPolicyParameters.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerGetPolicyParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**id** | **String** | The id of the policy. in: path | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerJsonWebKeyQuery.md b/sdk/js/swagger/docs/SwaggerJsonWebKeyQuery.md
new file mode 100644
index 00000000000..caef98322e1
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerJsonWebKeyQuery.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerJsonWebKeyQuery
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**kid** | **String** | The kid of the desired key in: path |
+**set** | **String** | The set in: path |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerJwkCreateSet.md b/sdk/js/swagger/docs/SwaggerJwkCreateSet.md
new file mode 100644
index 00000000000..2f951296871
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerJwkCreateSet.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerJwkCreateSet
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**JsonWebKeySetGeneratorRequest**](JsonWebKeySetGeneratorRequest.md) | | [optional]
+**set** | **String** | The set in: path |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerJwkSetQuery.md b/sdk/js/swagger/docs/SwaggerJwkSetQuery.md
new file mode 100644
index 00000000000..98595518b0a
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerJwkSetQuery.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerJwkSetQuery
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**set** | **String** | The set in: path |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerJwkUpdateSet.md b/sdk/js/swagger/docs/SwaggerJwkUpdateSet.md
new file mode 100644
index 00000000000..8b5e5968a73
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerJwkUpdateSet.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSet
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**JsonWebKeySet**](JsonWebKeySet.md) | | [optional]
+**set** | **String** | The set in: path |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerJwkUpdateSetKey.md b/sdk/js/swagger/docs/SwaggerJwkUpdateSetKey.md
new file mode 100644
index 00000000000..060f6a1e6cd
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerJwkUpdateSetKey.md
@@ -0,0 +1,10 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**JsonWebKey**](JsonWebKey.md) | | [optional]
+**kid** | **String** | The kid of the desired key in: path |
+**set** | **String** | The set in: path |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerListPolicyParameters.md b/sdk/js/swagger/docs/SwaggerListPolicyParameters.md
new file mode 100644
index 00000000000..625362f5bf6
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerListPolicyParameters.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerListPolicyParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**limit** | **Number** | The maximum amount of policies returned. in: query | [optional]
+**offset** | **Number** | The offset from where to start looking. in: query | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerListPolicyResponse.md b/sdk/js/swagger/docs/SwaggerListPolicyResponse.md
new file mode 100644
index 00000000000..f9bbd69a82f
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerListPolicyResponse.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerListPolicyResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**[Policy]**](Policy.md) | in: body type: array | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerOAuthConsentRequest.md b/sdk/js/swagger/docs/SwaggerOAuthConsentRequest.md
new file mode 100644
index 00000000000..ffbcf0e0ee5
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerOAuthConsentRequest.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**OAuth2consentRequest**](OAuth2consentRequest.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerOAuthConsentRequestPayload.md b/sdk/js/swagger/docs/SwaggerOAuthConsentRequestPayload.md
new file mode 100644
index 00000000000..22d7a8d8719
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerOAuthConsentRequestPayload.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequestPayload
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**id** | **String** | The id of the OAuth 2.0 Consent Request. |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerOAuthIntrospectionRequest.md b/sdk/js/swagger/docs/SwaggerOAuthIntrospectionRequest.md
new file mode 100644
index 00000000000..023bca1fb49
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerOAuthIntrospectionRequest.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**scope** | **String** | An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false. in: formData | [optional]
+**token** | **String** | The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation. |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerOAuthIntrospectionResponse.md b/sdk/js/swagger/docs/SwaggerOAuthIntrospectionResponse.md
new file mode 100644
index 00000000000..4010b2085df
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerOAuthIntrospectionResponse.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**OAuth2TokenIntrospection**](OAuth2TokenIntrospection.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerOAuthTokenResponse.md b/sdk/js/swagger/docs/SwaggerOAuthTokenResponse.md
new file mode 100644
index 00000000000..7d0f075c0df
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerOAuthTokenResponse.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**SwaggerOAuthTokenResponseBody**](SwaggerOAuthTokenResponseBody.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerOAuthTokenResponseBody.md b/sdk/js/swagger/docs/SwaggerOAuthTokenResponseBody.md
new file mode 100644
index 00000000000..f90caf84a9c
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerOAuthTokenResponseBody.md
@@ -0,0 +1,13 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**accessToken** | **String** | The access token issued by the authorization server. | [optional]
+**expiresIn** | **Number** | The lifetime in seconds of the access token. For example, the value \"3600\" denotes that the access token will expire in one hour from the time the response was generated. | [optional]
+**idToken** | **Number** | To retrieve a refresh token request the id_token scope. | [optional]
+**refreshToken** | **String** | The refresh token, which can be used to obtain new access tokens. To retrieve it add the scope \"offline\" to your access token request. | [optional]
+**scope** | **Number** | The scope of the access token | [optional]
+**tokenType** | **String** | The type of the token issued | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerRejectConsentRequest.md b/sdk/js/swagger/docs/SwaggerRejectConsentRequest.md
new file mode 100644
index 00000000000..1514b51a4c3
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerRejectConsentRequest.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerRejectConsentRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**ConsentRequestRejection**](ConsentRequestRejection.md) | |
+**id** | **String** | in: path |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerRevokeOAuth2TokenParameters.md b/sdk/js/swagger/docs/SwaggerRevokeOAuth2TokenParameters.md
new file mode 100644
index 00000000000..a63f0bdd5c8
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerRevokeOAuth2TokenParameters.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerRevokeOAuth2TokenParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**token** | **String** | in: formData |
+
+
diff --git a/sdk/js/swagger/docs/SwaggerUpdatePolicyParameters.md b/sdk/js/swagger/docs/SwaggerUpdatePolicyParameters.md
new file mode 100644
index 00000000000..339527105be
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerUpdatePolicyParameters.md
@@ -0,0 +1,9 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerUpdatePolicyParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**Policy**](Policy.md) | | [optional]
+**id** | **String** | The id of the policy. in: path | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerWardenAccessRequestResponseParameters.md b/sdk/js/swagger/docs/SwaggerWardenAccessRequestResponseParameters.md
new file mode 100644
index 00000000000..fb997842595
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerWardenAccessRequestResponseParameters.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerWardenAccessRequestResponseParameters
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**WardenAccessRequestResponse**](WardenAccessRequestResponse.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/SwaggerWardenTokenAccessRequestResponse.md b/sdk/js/swagger/docs/SwaggerWardenTokenAccessRequestResponse.md
new file mode 100644
index 00000000000..2da16f187d0
--- /dev/null
+++ b/sdk/js/swagger/docs/SwaggerWardenTokenAccessRequestResponse.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.SwaggerWardenTokenAccessRequestResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**body** | [**WardenTokenAccessRequestResponsePayload**](WardenTokenAccessRequestResponsePayload.md) | | [optional]
+
+
diff --git a/sdk/js/swagger/docs/TokenAllowedRequest.md b/sdk/js/swagger/docs/TokenAllowedRequest.md
new file mode 100644
index 00000000000..6c74bc77e2b
--- /dev/null
+++ b/sdk/js/swagger/docs/TokenAllowedRequest.md
@@ -0,0 +1,10 @@
+# HydraOAuth2OpenIdConnectServer.TokenAllowedRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**action** | **String** | Action is the action that is requested on the resource. | [optional]
+**context** | **{String: Object}** | Context is the request's environmental context. | [optional]
+**resource** | **String** | Resource is the resource that access is requested to. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/WardenAccessRequest.md b/sdk/js/swagger/docs/WardenAccessRequest.md
new file mode 100644
index 00000000000..ff8ffc0f101
--- /dev/null
+++ b/sdk/js/swagger/docs/WardenAccessRequest.md
@@ -0,0 +1,11 @@
+# HydraOAuth2OpenIdConnectServer.WardenAccessRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**action** | **String** | Action is the action that is requested on the resource. | [optional]
+**context** | **{String: Object}** | Context is the request's environmental context. | [optional]
+**resource** | **String** | Resource is the resource that access is requested to. | [optional]
+**subject** | **String** | Subejct is the subject that is requesting access. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/WardenAccessRequestResponse.md b/sdk/js/swagger/docs/WardenAccessRequestResponse.md
new file mode 100644
index 00000000000..236e574cd65
--- /dev/null
+++ b/sdk/js/swagger/docs/WardenAccessRequestResponse.md
@@ -0,0 +1,8 @@
+# HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**allowed** | **Boolean** | Allowed is true if the request is allowed and false otherwise. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/WardenApi.md b/sdk/js/swagger/docs/WardenApi.md
new file mode 100644
index 00000000000..ecaea18ab60
--- /dev/null
+++ b/sdk/js/swagger/docs/WardenApi.md
@@ -0,0 +1,435 @@
+# HydraOAuth2OpenIdConnectServer.WardenApi
+
+All URIs are relative to *http://localhost*
+
+Method | HTTP request | Description
+------------- | ------------- | -------------
+[**addMembersToGroup**](WardenApi.md#addMembersToGroup) | **POST** /warden/groups/{id}/members | Add members to a group
+[**createGroup**](WardenApi.md#createGroup) | **POST** /warden/groups | Create a group
+[**deleteGroup**](WardenApi.md#deleteGroup) | **DELETE** /warden/groups/{id} | Delete a group by id
+[**doesWardenAllowAccessRequest**](WardenApi.md#doesWardenAllowAccessRequest) | **POST** /warden/allowed | Check if an access request is valid (without providing an access token)
+[**doesWardenAllowTokenAccessRequest**](WardenApi.md#doesWardenAllowTokenAccessRequest) | **POST** /warden/token/allowed | Check if an access request is valid (providing an access token)
+[**findGroupsByMember**](WardenApi.md#findGroupsByMember) | **GET** /warden/groups | Find groups by member
+[**getGroup**](WardenApi.md#getGroup) | **GET** /warden/groups/{id} | Get a group by id
+[**removeMembersFromGroup**](WardenApi.md#removeMembersFromGroup) | **DELETE** /warden/groups/{id}/members | Remove members from a group
+
+
+
+# **addMembersToGroup**
+> addMembersToGroup(id, opts)
+
+Add members to a group
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"members.add\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+
+var id = "id_example"; // String | The id of the group to modify.
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.GroupMembers() // GroupMembers |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.addMembersToGroup(id, opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the group to modify. |
+ **body** | [**GroupMembers**](GroupMembers.md)| | [optional]
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **createGroup**
+> Group createGroup(opts)
+
+Create a group
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.Group() // Group |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.createGroup(opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**Group**](Group.md)| | [optional]
+
+### Return type
+
+[**Group**](Group.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **deleteGroup**
+> deleteGroup(id)
+
+Delete a group by id
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+
+var id = "id_example"; // String | The id of the group to look up.
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.deleteGroup(id, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the group to look up. |
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **doesWardenAllowAccessRequest**
+> WardenAccessRequestResponse doesWardenAllowAccessRequest(opts)
+
+Check if an access request is valid (without providing an access token)
+
+Checks if a subject (typically a user or a service) is allowed to perform an action on a resource. This endpoint requires a subject, a resource name, an action name and a context. If the subject is not allowed to perform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`, otherwise `{ \"allowed\": true }` is returned. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:allowed\"], \"actions\": [\"decide\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.WardenAccessRequest() // WardenAccessRequest |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.doesWardenAllowAccessRequest(opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**WardenAccessRequest**](WardenAccessRequest.md)| | [optional]
+
+### Return type
+
+[**WardenAccessRequestResponse**](WardenAccessRequestResponse.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **doesWardenAllowTokenAccessRequest**
+> WardenTokenAccessRequestResponsePayload doesWardenAllowTokenAccessRequest(opts)
+
+Check if an access request is valid (providing an access token)
+
+Checks if a token is valid and if the token subject is allowed to perform an action on a resource. This endpoint requires a token, a scope, a resource name, an action name and a context. If a token is expired/invalid, has not been granted the requested scope or the subject is not allowed to perform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`. Extra data set through the `accessTokenExtra` field in the consent flow will be included in the response. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:token:allowed\"], \"actions\": [\"decide\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest() // WardenTokenAccessRequest |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.doesWardenAllowTokenAccessRequest(opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **body** | [**WardenTokenAccessRequest**](WardenTokenAccessRequest.md)| | [optional]
+
+### Return type
+
+[**WardenTokenAccessRequestResponsePayload**](WardenTokenAccessRequestResponsePayload.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **findGroupsByMember**
+> [Group] findGroupsByMember(member)
+
+Find groups by member
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups\"], \"actions\": [\"list\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+
+var member = "member_example"; // String | The id of the member to look up.
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.findGroupsByMember(member, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **member** | **String**| The id of the member to look up. |
+
+### Return type
+
+[**[Group]**](Group.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **getGroup**
+> Group getGroup(id)
+
+Get a group by id
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+
+var id = "id_example"; // String | The id of the group to look up.
+
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully. Returned data: ' + data);
+ }
+};
+apiInstance.getGroup(id, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the group to look up. |
+
+### Return type
+
+[**Group**](Group.md)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
+
+# **removeMembersFromGroup**
+> removeMembersFromGroup(id, opts)
+
+Remove members from a group
+
+The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"members.remove\"], \"effect\": \"allow\" } ```
+
+### Example
+```javascript
+var HydraOAuth2OpenIdConnectServer = require('hydra_o_auth2__open_id_connect_server');
+var defaultClient = HydraOAuth2OpenIdConnectServer.ApiClient.instance;
+
+// Configure OAuth2 access token for authorization: oauth2
+var oauth2 = defaultClient.authentications['oauth2'];
+oauth2.accessToken = 'YOUR ACCESS TOKEN';
+
+var apiInstance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+
+var id = "id_example"; // String | The id of the group to modify.
+
+var opts = {
+ 'body': new HydraOAuth2OpenIdConnectServer.GroupMembers() // GroupMembers |
+};
+
+var callback = function(error, data, response) {
+ if (error) {
+ console.error(error);
+ } else {
+ console.log('API called successfully.');
+ }
+};
+apiInstance.removeMembersFromGroup(id, opts, callback);
+```
+
+### Parameters
+
+Name | Type | Description | Notes
+------------- | ------------- | ------------- | -------------
+ **id** | **String**| The id of the group to modify. |
+ **body** | [**GroupMembers**](GroupMembers.md)| | [optional]
+
+### Return type
+
+null (empty response body)
+
+### Authorization
+
+[oauth2](../README.md#oauth2)
+
+### HTTP request headers
+
+ - **Content-Type**: application/json
+ - **Accept**: application/json
+
diff --git a/sdk/js/swagger/docs/WardenTokenAccessRequest.md b/sdk/js/swagger/docs/WardenTokenAccessRequest.md
new file mode 100644
index 00000000000..a0a7f9529f1
--- /dev/null
+++ b/sdk/js/swagger/docs/WardenTokenAccessRequest.md
@@ -0,0 +1,12 @@
+# HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**action** | **String** | Action is the action that is requested on the resource. | [optional]
+**context** | **{String: Object}** | Context is the request's environmental context. | [optional]
+**resource** | **String** | Resource is the resource that access is requested to. | [optional]
+**scopes** | **[String]** | Scopes is an array of scopes that are requried. | [optional]
+**token** | **String** | Token is the token to introspect. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/WardenTokenAccessRequestResponsePayload.md b/sdk/js/swagger/docs/WardenTokenAccessRequestResponsePayload.md
new file mode 100644
index 00000000000..93a6f26f0a4
--- /dev/null
+++ b/sdk/js/swagger/docs/WardenTokenAccessRequestResponsePayload.md
@@ -0,0 +1,15 @@
+# HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**allowed** | **Boolean** | Allowed is true if the request is allowed and false otherwise. | [optional]
+**aud** | **String** | Audience is who the token was issued for. This is an OAuth2 app usually. | [optional]
+**exp** | **String** | ExpiresAt is the expiry timestamp. | [optional]
+**ext** | **{String: Object}** | Extra represents arbitrary session data. | [optional]
+**iat** | **String** | IssuedAt is the token creation time stamp. | [optional]
+**iss** | **String** | Issuer is the id of the issuer, typically an hydra instance. | [optional]
+**scopes** | **[String]** | GrantedScopes is a list of scopes that the subject authorized when asked for consent. | [optional]
+**sub** | **String** | Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app. This is usually a uuid but you can choose a urn or some other id too. | [optional]
+
+
diff --git a/sdk/js/swagger/docs/WellKnown.md b/sdk/js/swagger/docs/WellKnown.md
new file mode 100644
index 00000000000..6425b8a5487
--- /dev/null
+++ b/sdk/js/swagger/docs/WellKnown.md
@@ -0,0 +1,14 @@
+# HydraOAuth2OpenIdConnectServer.WellKnown
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+**authorizationEndpoint** | **String** | URL of the OP's OAuth 2.0 Authorization Endpoint |
+**idTokenSigningAlgValuesSupported** | **[String]** | JSON array containing a list of the JWS signing algorithms (alg values) supported by the OP for the ID Token to encode the Claims in a JWT [JWT]. The algorithm RS256 MUST be included. The value none MAY be supported, but MUST NOT be used unless the Response Type used returns no ID Token from the Authorization Endpoint (such as when using the Authorization Code Flow). |
+**issuer** | **String** | URL using the https scheme with no query or fragment component that the OP asserts as its Issuer Identifier. If Issuer discovery is supported , this value MUST be identical to the issuer value returned by WebFinger. This also MUST be identical to the iss Claim value in ID Tokens issued from this Issuer. |
+**jwksUri** | **String** | URL of the OP's JSON Web Key Set [JWK] document. This contains the signing key(s) the RP uses to validate signatures from the OP. The JWK Set MAY also contain the Server's encryption key(s), which are used by RPs to encrypt requests to the Server. When both signing and encryption keys are made available, a use (Key Use) parameter value is REQUIRED for all keys in the referenced JWK Set to indicate each key's intended usage. Although some algorithms allow the same key to be used for both signatures and encryption, doing so is NOT RECOMMENDED, as it is less secure. The JWK x5c parameter MAY be used to provide X.509 representations of keys provided. When used, the bare key values MUST still be present and MUST match those in the certificate. |
+**responseTypesSupported** | **[String]** | JSON array containing a list of the OAuth 2.0 response_type values that this OP supports. Dynamic OpenID Providers MUST support the code, id_token, and the token id_token Response Type values. |
+**subjectTypesSupported** | **[String]** | JSON array containing a list of the Subject Identifier types that this OP supports. Valid types include pairwise and public. |
+**tokenEndpoint** | **String** | URL of the OP's OAuth 2.0 Token Endpoint |
+
+
diff --git a/sdk/js/swagger/docs/Writer.md b/sdk/js/swagger/docs/Writer.md
new file mode 100644
index 00000000000..e51e085391e
--- /dev/null
+++ b/sdk/js/swagger/docs/Writer.md
@@ -0,0 +1,7 @@
+# HydraOAuth2OpenIdConnectServer.Writer
+
+## Properties
+Name | Type | Description | Notes
+------------ | ------------- | ------------- | -------------
+
+
diff --git a/sdk/js/swagger/git_push.sh b/sdk/js/swagger/git_push.sh
new file mode 100644
index 00000000000..0d041ad0ba4
--- /dev/null
+++ b/sdk/js/swagger/git_push.sh
@@ -0,0 +1,52 @@
+#!/bin/sh
+# ref: https://help.github.com/articles/adding-an-existing-project-to-github-using-the-command-line/
+#
+# Usage example: /bin/sh ./git_push.sh wing328 swagger-petstore-perl "minor update"
+
+git_user_id=$1
+git_repo_id=$2
+release_note=$3
+
+if [ "$git_user_id" = "" ]; then
+ git_user_id="GIT_USER_ID"
+ echo "[INFO] No command line input provided. Set \$git_user_id to $git_user_id"
+fi
+
+if [ "$git_repo_id" = "" ]; then
+ git_repo_id="GIT_REPO_ID"
+ echo "[INFO] No command line input provided. Set \$git_repo_id to $git_repo_id"
+fi
+
+if [ "$release_note" = "" ]; then
+ release_note="Minor update"
+ echo "[INFO] No command line input provided. Set \$release_note to $release_note"
+fi
+
+# Initialize the local directory as a Git repository
+git init
+
+# Adds the files in the local repository and stages them for commit.
+git add .
+
+# Commits the tracked changes and prepares them to be pushed to a remote repository.
+git commit -m "$release_note"
+
+# Sets the new remote
+git_remote=`git remote`
+if [ "$git_remote" = "" ]; then # git remote not defined
+
+ if [ "$GIT_TOKEN" = "" ]; then
+ echo "[INFO] \$GIT_TOKEN (environment variable) is not set. Using the Git credential in your environment."
+ git remote add origin https://github.com/${git_user_id}/${git_repo_id}.git
+ else
+ git remote add origin https://${git_user_id}:${GIT_TOKEN}@github.com/${git_user_id}/${git_repo_id}.git
+ fi
+
+fi
+
+git pull origin master
+
+# Pushes (Forces) the changes in the local repository up to the remote repository
+echo "Git pushing to https://github.com/${git_user_id}/${git_repo_id}.git"
+git push origin master 2>&1 | grep -v 'To https'
+
diff --git a/sdk/js/swagger/mocha.opts b/sdk/js/swagger/mocha.opts
new file mode 100644
index 00000000000..907011807d6
--- /dev/null
+++ b/sdk/js/swagger/mocha.opts
@@ -0,0 +1 @@
+--timeout 10000
diff --git a/sdk/js/swagger/package.json b/sdk/js/swagger/package.json
new file mode 100644
index 00000000000..f9990f030fe
--- /dev/null
+++ b/sdk/js/swagger/package.json
@@ -0,0 +1,21 @@
+{
+ "name": "hydra_o_auth2__open_id_connect_server",
+ "version": "Latest",
+ "description": "Please_refer_to_the_user_guide_for_in_depth_documentation_httpsory_gitbooks_iohydracontentHydra_offers_OAuth_2_0_and_OpenID_Connect_Core_1_0_capabilities_as_a_service__Hydra_is_different_because_it_works_with_any_existing_authentication_infrastructure_not_just_LDAP_or_SAML__By_implementing_a_consent_app__works_with_any_programming_language_you_build_a_bridge_between_Hydra_and_your_authentication_infrastructure_Hydra_is_able_to_securely_manage_JSON_Web_Keys_and_has_a_sophisticated_policy_based_access_control_you_can_use_if_you_want_to_Hydra_is_suitable_for_green___new_and_brownfield__existing_projects__If_you_are_not_familiar_with_OAuth_2_0_and_are_working_on_a_greenfield_project_we_recommend_evaluating_if_OAuth_2_0_really_serves_your_purpose__Knowledge_of_OAuth_2_0_is_imperative_in_understanding_what_Hydra_does_and_how_it_works_The_official_repository_is_located_at_httpsgithub_comoryhydra_Important_REST_API_Documentation_NotesThe_swagger_generator_used_to_create_this_documentation_does_currently_not_support_example_responses__To_seerequest_and_response_payloads_click_on_Show_JSON_schema_Enable_JSON_Schema_on_Apiary_httpsstorage_googleapis_comory_amhydrajson_schema_pngThe_API_documentation_always_refers_to_the_latest_tagged_version_of_ORY_Hydra__For_previous_API_documentations_pleaserefer_to_httpsgithub_comoryhydrablobtag_iddocsapi_swagger_yaml___for_example0_9_13_httpsgithub_comoryhydrablobv0_9_13docsapi_swagger_yaml0_8_1_httpsgithub_comoryhydrablobv0_8_1docsapi_swagger_yaml",
+ "license": "Unlicense",
+ "main": "src/index.js",
+ "scripts": {
+ "test": "./node_modules/mocha/bin/mocha --recursive"
+ },
+ "browser": {
+ "fs": false
+ },
+ "dependencies": {
+ "superagent": "3.5.2"
+ },
+ "devDependencies": {
+ "mocha": "~2.3.4",
+ "sinon": "1.17.3",
+ "expect.js": "~0.3.1"
+ }
+}
diff --git a/sdk/js/swagger/src/ApiClient.js b/sdk/js/swagger/src/ApiClient.js
new file mode 100644
index 00000000000..2dc8d5888b1
--- /dev/null
+++ b/sdk/js/swagger/src/ApiClient.js
@@ -0,0 +1,569 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 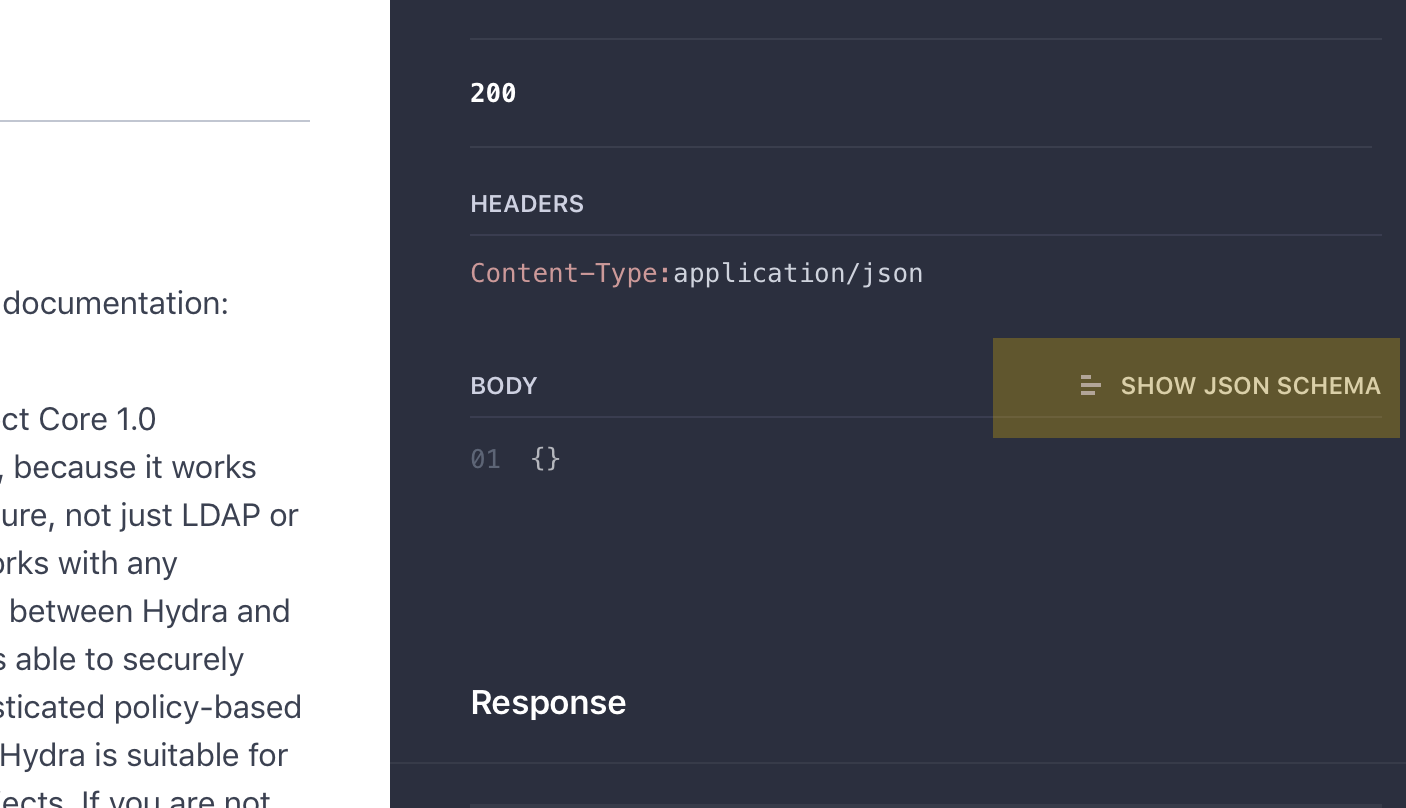 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['superagent', 'querystring'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('superagent'), require('querystring'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.ApiClient = factory(root.superagent, root.querystring);
+ }
+}(this, function(superagent, querystring) {
+ 'use strict';
+
+ /**
+ * @module ApiClient
+ * @version Latest
+ */
+
+ /**
+ * Manages low level client-server communications, parameter marshalling, etc. There should not be any need for an
+ * application to use this class directly - the *Api and model classes provide the public API for the service. The
+ * contents of this file should be regarded as internal but are documented for completeness.
+ * @alias module:ApiClient
+ * @class
+ */
+ var exports = function() {
+ /**
+ * The base URL against which to resolve every API call's (relative) path.
+ * @type {String}
+ * @default http://localhost
+ */
+ this.basePath = 'http://localhost'.replace(/\/+$/, '');
+
+ /**
+ * The authentication methods to be included for all API calls.
+ * @type {Array.}
+ */
+ this.authentications = {
+ 'basic': {type: 'basic'},
+ 'oauth2': {type: 'oauth2'}
+ };
+ /**
+ * The default HTTP headers to be included for all API calls.
+ * @type {Array.}
+ * @default {}
+ */
+ this.defaultHeaders = {};
+
+ /**
+ * The default HTTP timeout for all API calls.
+ * @type {Number}
+ * @default 60000
+ */
+ this.timeout = 60000;
+
+ /**
+ * If set to false an additional timestamp parameter is added to all API GET calls to
+ * prevent browser caching
+ * @type {Boolean}
+ * @default true
+ */
+ this.cache = true;
+
+ /**
+ * If set to true, the client will save the cookies from each server
+ * response, and return them in the next request.
+ * @default false
+ */
+ this.enableCookies = false;
+
+ /*
+ * Used to save and return cookies in a node.js (non-browser) setting,
+ * if this.enableCookies is set to true.
+ */
+ if (typeof window === 'undefined') {
+ this.agent = new superagent.agent();
+ }
+
+ };
+
+ /**
+ * Returns a string representation for an actual parameter.
+ * @param param The actual parameter.
+ * @returns {String} The string representation of param
.
+ */
+ exports.prototype.paramToString = function(param) {
+ if (param == undefined || param == null) {
+ return '';
+ }
+ if (param instanceof Date) {
+ return param.toJSON();
+ }
+ return param.toString();
+ };
+
+ /**
+ * Builds full URL by appending the given path to the base URL and replacing path parameter place-holders with parameter values.
+ * NOTE: query parameters are not handled here.
+ * @param {String} path The path to append to the base URL.
+ * @param {Object} pathParams The parameter values to append.
+ * @returns {String} The encoded path with parameter values substituted.
+ */
+ exports.prototype.buildUrl = function(path, pathParams) {
+ if (!path.match(/^\//)) {
+ path = '/' + path;
+ }
+ var url = this.basePath + path;
+ var _this = this;
+ url = url.replace(/\{([\w-]+)\}/g, function(fullMatch, key) {
+ var value;
+ if (pathParams.hasOwnProperty(key)) {
+ value = _this.paramToString(pathParams[key]);
+ } else {
+ value = fullMatch;
+ }
+ return encodeURIComponent(value);
+ });
+ return url;
+ };
+
+ /**
+ * Checks whether the given content type represents JSON.
+ * JSON content type examples:
+ *
+ * - application/json
+ * - application/json; charset=UTF8
+ * - APPLICATION/JSON
+ *
+ * @param {String} contentType The MIME content type to check.
+ * @returns {Boolean} true
if contentType
represents JSON, otherwise false
.
+ */
+ exports.prototype.isJsonMime = function(contentType) {
+ return Boolean(contentType != null && contentType.match(/^application\/json(;.*)?$/i));
+ };
+
+ /**
+ * Chooses a content type from the given array, with JSON preferred; i.e. return JSON if included, otherwise return the first.
+ * @param {Array.} contentTypes
+ * @returns {String} The chosen content type, preferring JSON.
+ */
+ exports.prototype.jsonPreferredMime = function(contentTypes) {
+ for (var i = 0; i < contentTypes.length; i++) {
+ if (this.isJsonMime(contentTypes[i])) {
+ return contentTypes[i];
+ }
+ }
+ return contentTypes[0];
+ };
+
+ /**
+ * Checks whether the given parameter value represents file-like content.
+ * @param param The parameter to check.
+ * @returns {Boolean} true
if param
represents a file.
+ */
+ exports.prototype.isFileParam = function(param) {
+ // fs.ReadStream in Node.js and Electron (but not in runtime like browserify)
+ if (typeof require === 'function') {
+ var fs;
+ try {
+ fs = require('fs');
+ } catch (err) {}
+ if (fs && fs.ReadStream && param instanceof fs.ReadStream) {
+ return true;
+ }
+ }
+ // Buffer in Node.js
+ if (typeof Buffer === 'function' && param instanceof Buffer) {
+ return true;
+ }
+ // Blob in browser
+ if (typeof Blob === 'function' && param instanceof Blob) {
+ return true;
+ }
+ // File in browser (it seems File object is also instance of Blob, but keep this for safe)
+ if (typeof File === 'function' && param instanceof File) {
+ return true;
+ }
+ return false;
+ };
+
+ /**
+ * Normalizes parameter values:
+ *
+ * - remove nils
+ * - keep files and arrays
+ * - format to string with `paramToString` for other cases
+ *
+ * @param {Object.} params The parameters as object properties.
+ * @returns {Object.} normalized parameters.
+ */
+ exports.prototype.normalizeParams = function(params) {
+ var newParams = {};
+ for (var key in params) {
+ if (params.hasOwnProperty(key) && params[key] != undefined && params[key] != null) {
+ var value = params[key];
+ if (this.isFileParam(value) || Array.isArray(value)) {
+ newParams[key] = value;
+ } else {
+ newParams[key] = this.paramToString(value);
+ }
+ }
+ }
+ return newParams;
+ };
+
+ /**
+ * Enumeration of collection format separator strategies.
+ * @enum {String}
+ * @readonly
+ */
+ exports.CollectionFormatEnum = {
+ /**
+ * Comma-separated values. Value: csv
+ * @const
+ */
+ CSV: ',',
+ /**
+ * Space-separated values. Value: ssv
+ * @const
+ */
+ SSV: ' ',
+ /**
+ * Tab-separated values. Value: tsv
+ * @const
+ */
+ TSV: '\t',
+ /**
+ * Pipe(|)-separated values. Value: pipes
+ * @const
+ */
+ PIPES: '|',
+ /**
+ * Native array. Value: multi
+ * @const
+ */
+ MULTI: 'multi'
+ };
+
+ /**
+ * Builds a string representation of an array-type actual parameter, according to the given collection format.
+ * @param {Array} param An array parameter.
+ * @param {module:ApiClient.CollectionFormatEnum} collectionFormat The array element separator strategy.
+ * @returns {String|Array} A string representation of the supplied collection, using the specified delimiter. Returns
+ * param
as is if collectionFormat
is multi
.
+ */
+ exports.prototype.buildCollectionParam = function buildCollectionParam(param, collectionFormat) {
+ if (param == null) {
+ return null;
+ }
+ switch (collectionFormat) {
+ case 'csv':
+ return param.map(this.paramToString).join(',');
+ case 'ssv':
+ return param.map(this.paramToString).join(' ');
+ case 'tsv':
+ return param.map(this.paramToString).join('\t');
+ case 'pipes':
+ return param.map(this.paramToString).join('|');
+ case 'multi':
+ // return the array directly as SuperAgent will handle it as expected
+ return param.map(this.paramToString);
+ default:
+ throw new Error('Unknown collection format: ' + collectionFormat);
+ }
+ };
+
+ /**
+ * Applies authentication headers to the request.
+ * @param {Object} request The request object created by a superagent()
call.
+ * @param {Array.} authNames An array of authentication method names.
+ */
+ exports.prototype.applyAuthToRequest = function(request, authNames) {
+ var _this = this;
+ authNames.forEach(function(authName) {
+ var auth = _this.authentications[authName];
+ switch (auth.type) {
+ case 'basic':
+ if (auth.username || auth.password) {
+ request.auth(auth.username || '', auth.password || '');
+ }
+ break;
+ case 'apiKey':
+ if (auth.apiKey) {
+ var data = {};
+ if (auth.apiKeyPrefix) {
+ data[auth.name] = auth.apiKeyPrefix + ' ' + auth.apiKey;
+ } else {
+ data[auth.name] = auth.apiKey;
+ }
+ if (auth['in'] === 'header') {
+ request.set(data);
+ } else {
+ request.query(data);
+ }
+ }
+ break;
+ case 'oauth2':
+ if (auth.accessToken) {
+ request.set({'Authorization': 'Bearer ' + auth.accessToken});
+ }
+ break;
+ default:
+ throw new Error('Unknown authentication type: ' + auth.type);
+ }
+ });
+ };
+
+ /**
+ * Deserializes an HTTP response body into a value of the specified type.
+ * @param {Object} response A SuperAgent response object.
+ * @param {(String|Array.|Object.|Function)} returnType The type to return. Pass a string for simple types
+ * or the constructor function for a complex type. Pass an array containing the type name to return an array of that type. To
+ * return an object, pass an object with one property whose name is the key type and whose value is the corresponding value type:
+ * all properties on data will be converted to this type.
+ * @returns A value of the specified type.
+ */
+ exports.prototype.deserialize = function deserialize(response, returnType) {
+ if (response == null || returnType == null || response.status == 204) {
+ return null;
+ }
+ // Rely on SuperAgent for parsing response body.
+ // See http://visionmedia.github.io/superagent/#parsing-response-bodies
+ var data = response.body;
+ if (data == null || (typeof data === 'object' && typeof data.length === 'undefined' && !Object.keys(data).length)) {
+ // SuperAgent does not always produce a body; use the unparsed response as a fallback
+ data = response.text;
+ }
+ return exports.convertToType(data, returnType);
+ };
+
+ /**
+ * Callback function to receive the result of the operation.
+ * @callback module:ApiClient~callApiCallback
+ * @param {String} error Error message, if any.
+ * @param data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Invokes the REST service using the supplied settings and parameters.
+ * @param {String} path The base URL to invoke.
+ * @param {String} httpMethod The HTTP method to use.
+ * @param {Object.} pathParams A map of path parameters and their values.
+ * @param {Object.} queryParams A map of query parameters and their values.
+ * @param {Object.} headerParams A map of header parameters and their values.
+ * @param {Object.} formParams A map of form parameters and their values.
+ * @param {Object} bodyParam The value to pass as the request body.
+ * @param {Array.} authNames An array of authentication type names.
+ * @param {Array.} contentTypes An array of request MIME types.
+ * @param {Array.} accepts An array of acceptable response MIME types.
+ * @param {(String|Array|ObjectFunction)} returnType The required type to return; can be a string for simple types or the
+ * constructor for a complex type.
+ * @param {module:ApiClient~callApiCallback} callback The callback function.
+ * @returns {Object} The SuperAgent request object.
+ */
+ exports.prototype.callApi = function callApi(path, httpMethod, pathParams,
+ queryParams, headerParams, formParams, bodyParam, authNames, contentTypes, accepts,
+ returnType, callback) {
+
+ var _this = this;
+ var url = this.buildUrl(path, pathParams);
+ var request = superagent(httpMethod, url);
+
+ // apply authentications
+ this.applyAuthToRequest(request, authNames);
+
+ // set query parameters
+ if (httpMethod.toUpperCase() === 'GET' && this.cache === false) {
+ queryParams['_'] = new Date().getTime();
+ }
+ request.query(this.normalizeParams(queryParams));
+
+ // set header parameters
+ request.set(this.defaultHeaders).set(this.normalizeParams(headerParams));
+
+ // set request timeout
+ request.timeout(this.timeout);
+
+ var contentType = this.jsonPreferredMime(contentTypes);
+ if (contentType) {
+ // Issue with superagent and multipart/form-data (https://github.com/visionmedia/superagent/issues/746)
+ if(contentType != 'multipart/form-data') {
+ request.type(contentType);
+ }
+ } else if (!request.header['Content-Type']) {
+ request.type('application/json');
+ }
+
+ if (contentType === 'application/x-www-form-urlencoded') {
+ request.send(querystring.stringify(this.normalizeParams(formParams)));
+ } else if (contentType == 'multipart/form-data') {
+ var _formParams = this.normalizeParams(formParams);
+ for (var key in _formParams) {
+ if (_formParams.hasOwnProperty(key)) {
+ if (this.isFileParam(_formParams[key])) {
+ // file field
+ request.attach(key, _formParams[key]);
+ } else {
+ request.field(key, _formParams[key]);
+ }
+ }
+ }
+ } else if (bodyParam) {
+ request.send(bodyParam);
+ }
+
+ var accept = this.jsonPreferredMime(accepts);
+ if (accept) {
+ request.accept(accept);
+ }
+
+ if (returnType === 'Blob') {
+ request.responseType('blob');
+ } else if (returnType === 'String') {
+ request.responseType('string');
+ }
+
+ // Attach previously saved cookies, if enabled
+ if (this.enableCookies){
+ if (typeof window === 'undefined') {
+ this.agent.attachCookies(request);
+ }
+ else {
+ request.withCredentials();
+ }
+ }
+
+
+ request.end(function(error, response) {
+ if (callback) {
+ var data = null;
+ if (!error) {
+ try {
+ data = _this.deserialize(response, returnType);
+ if (_this.enableCookies && typeof window === 'undefined'){
+ _this.agent.saveCookies(response);
+ }
+ } catch (err) {
+ error = err;
+ }
+ }
+ callback(error, data, response);
+ }
+ });
+
+ return request;
+ };
+
+ /**
+ * Parses an ISO-8601 string representation of a date value.
+ * @param {String} str The date value as a string.
+ * @returns {Date} The parsed date object.
+ */
+ exports.parseDate = function(str) {
+ return new Date(str.replace(/T/i, ' '));
+ };
+
+ /**
+ * Converts a value to the specified type.
+ * @param {(String|Object)} data The data to convert, as a string or object.
+ * @param {(String|Array.|Object.|Function)} type The type to return. Pass a string for simple types
+ * or the constructor function for a complex type. Pass an array containing the type name to return an array of that type. To
+ * return an object, pass an object with one property whose name is the key type and whose value is the corresponding value type:
+ * all properties on data will be converted to this type.
+ * @returns An instance of the specified type or null or undefined if data is null or undefined.
+ */
+ exports.convertToType = function(data, type) {
+ if (data === null || data === undefined)
+ return data
+
+ switch (type) {
+ case 'Boolean':
+ return Boolean(data);
+ case 'Integer':
+ return parseInt(data, 10);
+ case 'Number':
+ return parseFloat(data);
+ case 'String':
+ return String(data);
+ case 'Date':
+ return this.parseDate(String(data));
+ case 'Blob':
+ return data;
+ default:
+ if (type === Object) {
+ // generic object, return directly
+ return data;
+ } else if (typeof type === 'function') {
+ // for model type like: User
+ return type.constructFromObject(data);
+ } else if (Array.isArray(type)) {
+ // for array type like: ['String']
+ var itemType = type[0];
+ return data.map(function(item) {
+ return exports.convertToType(item, itemType);
+ });
+ } else if (typeof type === 'object') {
+ // for plain object type like: {'String': 'Integer'}
+ var keyType, valueType;
+ for (var k in type) {
+ if (type.hasOwnProperty(k)) {
+ keyType = k;
+ valueType = type[k];
+ break;
+ }
+ }
+ var result = {};
+ for (var k in data) {
+ if (data.hasOwnProperty(k)) {
+ var key = exports.convertToType(k, keyType);
+ var value = exports.convertToType(data[k], valueType);
+ result[key] = value;
+ }
+ }
+ return result;
+ } else {
+ // for unknown type, return the data directly
+ return data;
+ }
+ }
+ };
+
+ /**
+ * Constructs a new map or array model from REST data.
+ * @param data {Object|Array} The REST data.
+ * @param obj {Object|Array} The target object or array.
+ */
+ exports.constructFromObject = function(data, obj, itemType) {
+ if (Array.isArray(data)) {
+ for (var i = 0; i < data.length; i++) {
+ if (data.hasOwnProperty(i))
+ obj[i] = exports.convertToType(data[i], itemType);
+ }
+ } else {
+ for (var k in data) {
+ if (data.hasOwnProperty(k))
+ obj[k] = exports.convertToType(data[k], itemType);
+ }
+ }
+ };
+
+ /**
+ * The default API client implementation.
+ * @type {module:ApiClient}
+ */
+ exports.instance = new exports();
+
+ return exports;
+}));
diff --git a/sdk/js/swagger/src/api/HealthApi.js b/sdk/js/swagger/src/api/HealthApi.js
new file mode 100644
index 00000000000..75359691795
--- /dev/null
+++ b/sdk/js/swagger/src/api/HealthApi.js
@@ -0,0 +1,130 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 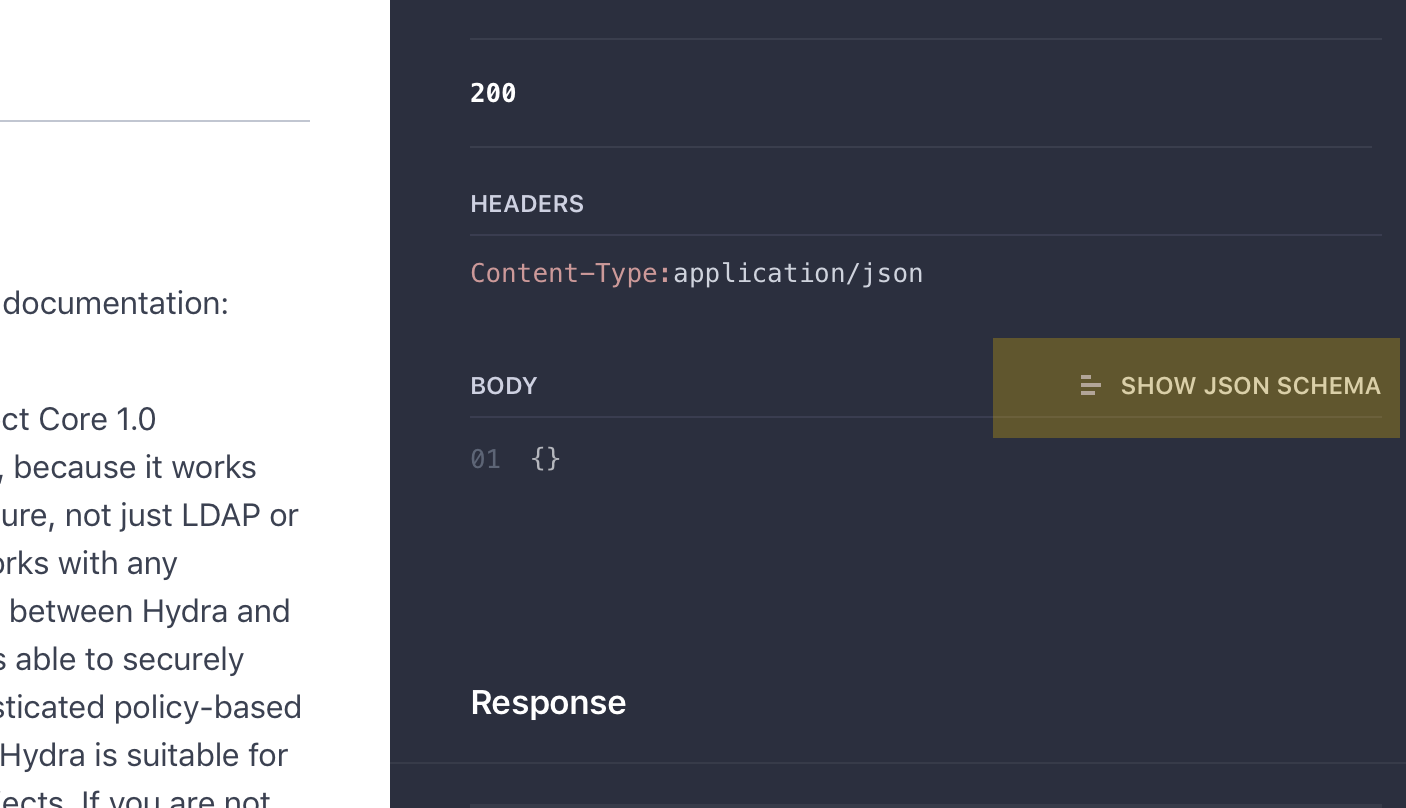 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/InlineResponse200', 'model/InlineResponse401'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('../model/InlineResponse200'), require('../model/InlineResponse401'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.HealthApi = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.InlineResponse200, root.HydraOAuth2OpenIdConnectServer.InlineResponse401);
+ }
+}(this, function(ApiClient, InlineResponse200, InlineResponse401) {
+ 'use strict';
+
+ /**
+ * Health service.
+ * @module api/HealthApi
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new HealthApi.
+ * @alias module:api/HealthApi
+ * @class
+ * @param {module:ApiClient} apiClient Optional API client implementation to use,
+ * default to {@link module:ApiClient#instance} if unspecified.
+ */
+ var exports = function(apiClient) {
+ this.apiClient = apiClient || ApiClient.instance;
+
+
+ /**
+ * Callback function to receive the result of the getInstanceMetrics operation.
+ * @callback module:api/HealthApi~getInstanceMetricsCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Show instance metrics (experimental)
+ * This endpoint returns an instance's metrics, such as average response time, status code distribution, hits per second and so on. The return values are currently not documented as this endpoint is still experimental. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:health:stats\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ * @param {module:api/HealthApi~getInstanceMetricsCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.getInstanceMetrics = function(callback) {
+ var postBody = null;
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/health/metrics', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the getInstanceStatus operation.
+ * @callback module:api/HealthApi~getInstanceStatusCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/InlineResponse200} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Check health status of this instance
+ * This endpoint returns `{ \"status\": \"ok\" }`. This status let's you know that the HTTP server is up and running. This status does currently not include checks whether the database connection is up and running. This endpoint does not require the `X-Forwarded-Proto` header when TLS termination is set. Be aware that if you are running multiple nodes of ORY Hydra, the health status will never refer to the cluster state, only to a single instance.
+ * @param {module:api/HealthApi~getInstanceStatusCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/InlineResponse200}
+ */
+ this.getInstanceStatus = function(callback) {
+ var postBody = null;
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = [];
+ var contentTypes = ['application/json', 'application/x-www-form-urlencoded'];
+ var accepts = ['application/json'];
+ var returnType = InlineResponse200;
+
+ return this.apiClient.callApi(
+ '/health/status', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+ };
+
+ return exports;
+}));
diff --git a/sdk/js/swagger/src/api/JsonWebKeyApi.js b/sdk/js/swagger/src/api/JsonWebKeyApi.js
new file mode 100644
index 00000000000..b5512052463
--- /dev/null
+++ b/sdk/js/swagger/src/api/JsonWebKeyApi.js
@@ -0,0 +1,403 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 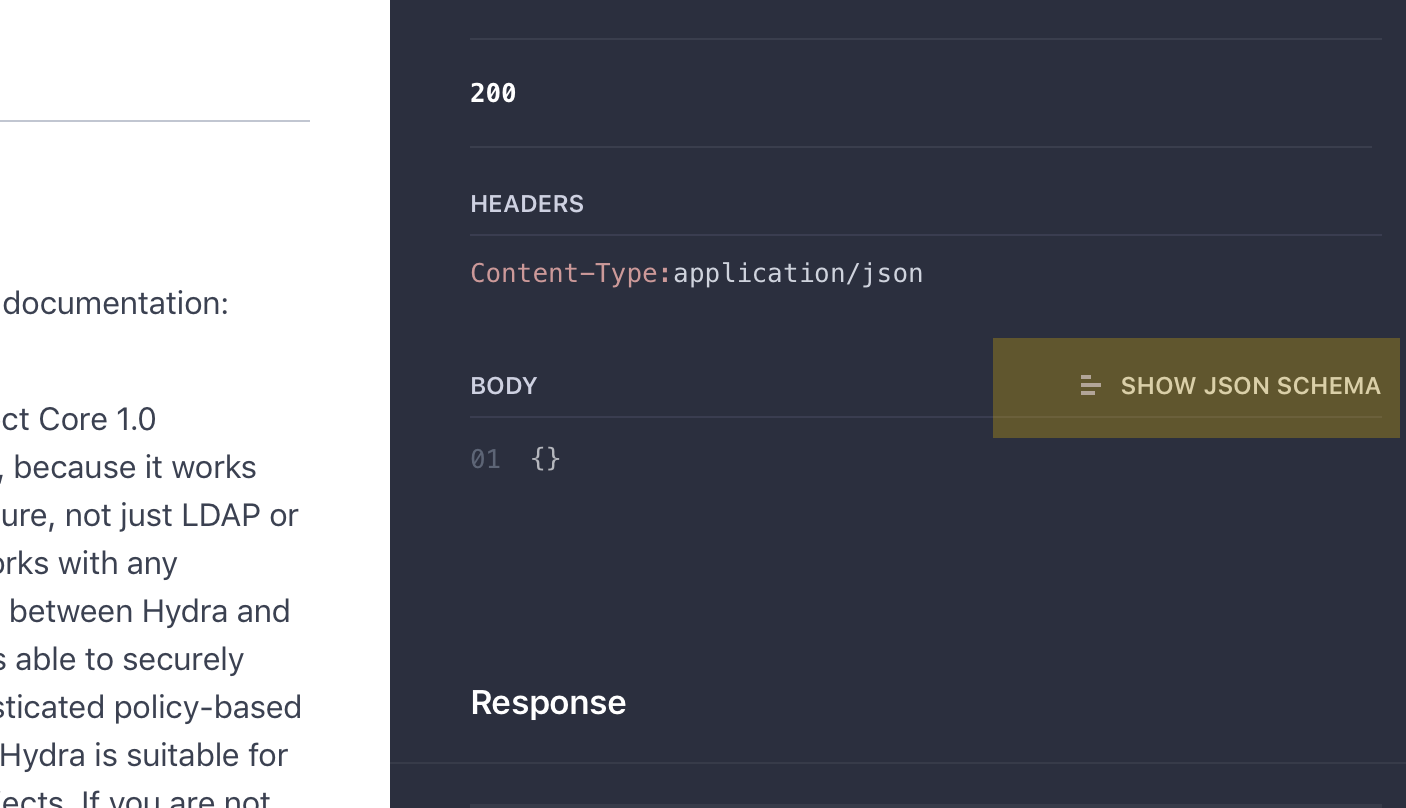 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/InlineResponse401', 'model/JsonWebKey', 'model/JsonWebKeySet', 'model/JsonWebKeySetGeneratorRequest'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('../model/InlineResponse401'), require('../model/JsonWebKey'), require('../model/JsonWebKeySet'), require('../model/JsonWebKeySetGeneratorRequest'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.JsonWebKeyApi = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.InlineResponse401, root.HydraOAuth2OpenIdConnectServer.JsonWebKey, root.HydraOAuth2OpenIdConnectServer.JsonWebKeySet, root.HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest);
+ }
+}(this, function(ApiClient, InlineResponse401, JsonWebKey, JsonWebKeySet, JsonWebKeySetGeneratorRequest) {
+ 'use strict';
+
+ /**
+ * JsonWebKey service.
+ * @module api/JsonWebKeyApi
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new JsonWebKeyApi.
+ * @alias module:api/JsonWebKeyApi
+ * @class
+ * @param {module:ApiClient} apiClient Optional API client implementation to use,
+ * default to {@link module:ApiClient#instance} if unspecified.
+ */
+ var exports = function(apiClient) {
+ this.apiClient = apiClient || ApiClient.instance;
+
+
+ /**
+ * Callback function to receive the result of the createJsonWebKeySet operation.
+ * @callback module:api/JsonWebKeyApi~createJsonWebKeySetCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/JsonWebKeySet} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Generate a new JSON Web Key
+ * This endpoint is capable of generating JSON Web Key Sets for you. There a different strategies available, such as symmetric cryptographic keys (HS256) and asymetric cryptographic keys (RS256, ECDSA). If the specified JSON Web Key Set does not exist, it will be created. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+ * @param {String} set The set
+ * @param {Object} opts Optional parameters
+ * @param {module:model/JsonWebKeySetGeneratorRequest} opts.body
+ * @param {module:api/JsonWebKeyApi~createJsonWebKeySetCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/JsonWebKeySet}
+ */
+ this.createJsonWebKeySet = function(set, opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+ // verify the required parameter 'set' is set
+ if (set === undefined || set === null) {
+ throw new Error("Missing the required parameter 'set' when calling createJsonWebKeySet");
+ }
+
+
+ var pathParams = {
+ 'set': set
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = JsonWebKeySet;
+
+ return this.apiClient.callApi(
+ '/keys/{set}', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the deleteJsonWebKey operation.
+ * @callback module:api/JsonWebKeyApi~deleteJsonWebKeyCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Delete a JSON Web Key
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+ * @param {String} kid The kid of the desired key
+ * @param {String} set The set
+ * @param {module:api/JsonWebKeyApi~deleteJsonWebKeyCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.deleteJsonWebKey = function(kid, set, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'kid' is set
+ if (kid === undefined || kid === null) {
+ throw new Error("Missing the required parameter 'kid' when calling deleteJsonWebKey");
+ }
+
+ // verify the required parameter 'set' is set
+ if (set === undefined || set === null) {
+ throw new Error("Missing the required parameter 'set' when calling deleteJsonWebKey");
+ }
+
+
+ var pathParams = {
+ 'kid': kid,
+ 'set': set
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/keys/{set}/{kid}', 'DELETE',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the deleteJsonWebKeySet operation.
+ * @callback module:api/JsonWebKeyApi~deleteJsonWebKeySetCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Delete a JSON Web Key
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+ * @param {String} set The set
+ * @param {module:api/JsonWebKeyApi~deleteJsonWebKeySetCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.deleteJsonWebKeySet = function(set, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'set' is set
+ if (set === undefined || set === null) {
+ throw new Error("Missing the required parameter 'set' when calling deleteJsonWebKeySet");
+ }
+
+
+ var pathParams = {
+ 'set': set
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/keys/{set}', 'DELETE',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the getJsonWebKey operation.
+ * @callback module:api/JsonWebKeyApi~getJsonWebKeyCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/JsonWebKeySet} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Retrieve a JSON Web Key
+ * This endpoint can be used to retrieve JWKs stored in ORY Hydra. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ * @param {String} kid The kid of the desired key
+ * @param {String} set The set
+ * @param {module:api/JsonWebKeyApi~getJsonWebKeyCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/JsonWebKeySet}
+ */
+ this.getJsonWebKey = function(kid, set, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'kid' is set
+ if (kid === undefined || kid === null) {
+ throw new Error("Missing the required parameter 'kid' when calling getJsonWebKey");
+ }
+
+ // verify the required parameter 'set' is set
+ if (set === undefined || set === null) {
+ throw new Error("Missing the required parameter 'set' when calling getJsonWebKey");
+ }
+
+
+ var pathParams = {
+ 'kid': kid,
+ 'set': set
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = JsonWebKeySet;
+
+ return this.apiClient.callApi(
+ '/keys/{set}/{kid}', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the getJsonWebKeySet operation.
+ * @callback module:api/JsonWebKeyApi~getJsonWebKeySetCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/JsonWebKeySet} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Retrieve a JSON Web Key Set
+ * This endpoint can be used to retrieve JWK Sets stored in ORY Hydra. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ * @param {String} set The set
+ * @param {module:api/JsonWebKeyApi~getJsonWebKeySetCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/JsonWebKeySet}
+ */
+ this.getJsonWebKeySet = function(set, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'set' is set
+ if (set === undefined || set === null) {
+ throw new Error("Missing the required parameter 'set' when calling getJsonWebKeySet");
+ }
+
+
+ var pathParams = {
+ 'set': set
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = JsonWebKeySet;
+
+ return this.apiClient.callApi(
+ '/keys/{set}', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the updateJsonWebKey operation.
+ * @callback module:api/JsonWebKeyApi~updateJsonWebKeyCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/JsonWebKey} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Update a JSON Web Key
+ * Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>:<kid>\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+ * @param {String} kid The kid of the desired key
+ * @param {String} set The set
+ * @param {Object} opts Optional parameters
+ * @param {module:model/JsonWebKey} opts.body
+ * @param {module:api/JsonWebKeyApi~updateJsonWebKeyCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/JsonWebKey}
+ */
+ this.updateJsonWebKey = function(kid, set, opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+ // verify the required parameter 'kid' is set
+ if (kid === undefined || kid === null) {
+ throw new Error("Missing the required parameter 'kid' when calling updateJsonWebKey");
+ }
+
+ // verify the required parameter 'set' is set
+ if (set === undefined || set === null) {
+ throw new Error("Missing the required parameter 'set' when calling updateJsonWebKey");
+ }
+
+
+ var pathParams = {
+ 'kid': kid,
+ 'set': set
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = JsonWebKey;
+
+ return this.apiClient.callApi(
+ '/keys/{set}/{kid}', 'PUT',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the updateJsonWebKeySet operation.
+ * @callback module:api/JsonWebKeyApi~updateJsonWebKeySetCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/JsonWebKeySet} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Update a JSON Web Key Set
+ * Use this method if you do not want to let Hydra generate the JWKs for you, but instead save your own. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:<set>\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+ * @param {String} set The set
+ * @param {Object} opts Optional parameters
+ * @param {module:model/JsonWebKeySet} opts.body
+ * @param {module:api/JsonWebKeyApi~updateJsonWebKeySetCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/JsonWebKeySet}
+ */
+ this.updateJsonWebKeySet = function(set, opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+ // verify the required parameter 'set' is set
+ if (set === undefined || set === null) {
+ throw new Error("Missing the required parameter 'set' when calling updateJsonWebKeySet");
+ }
+
+
+ var pathParams = {
+ 'set': set
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = JsonWebKeySet;
+
+ return this.apiClient.callApi(
+ '/keys/{set}', 'PUT',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+ };
+
+ return exports;
+}));
diff --git a/sdk/js/swagger/src/api/OAuth2Api.js b/sdk/js/swagger/src/api/OAuth2Api.js
new file mode 100644
index 00000000000..6ed40a07afb
--- /dev/null
+++ b/sdk/js/swagger/src/api/OAuth2Api.js
@@ -0,0 +1,678 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 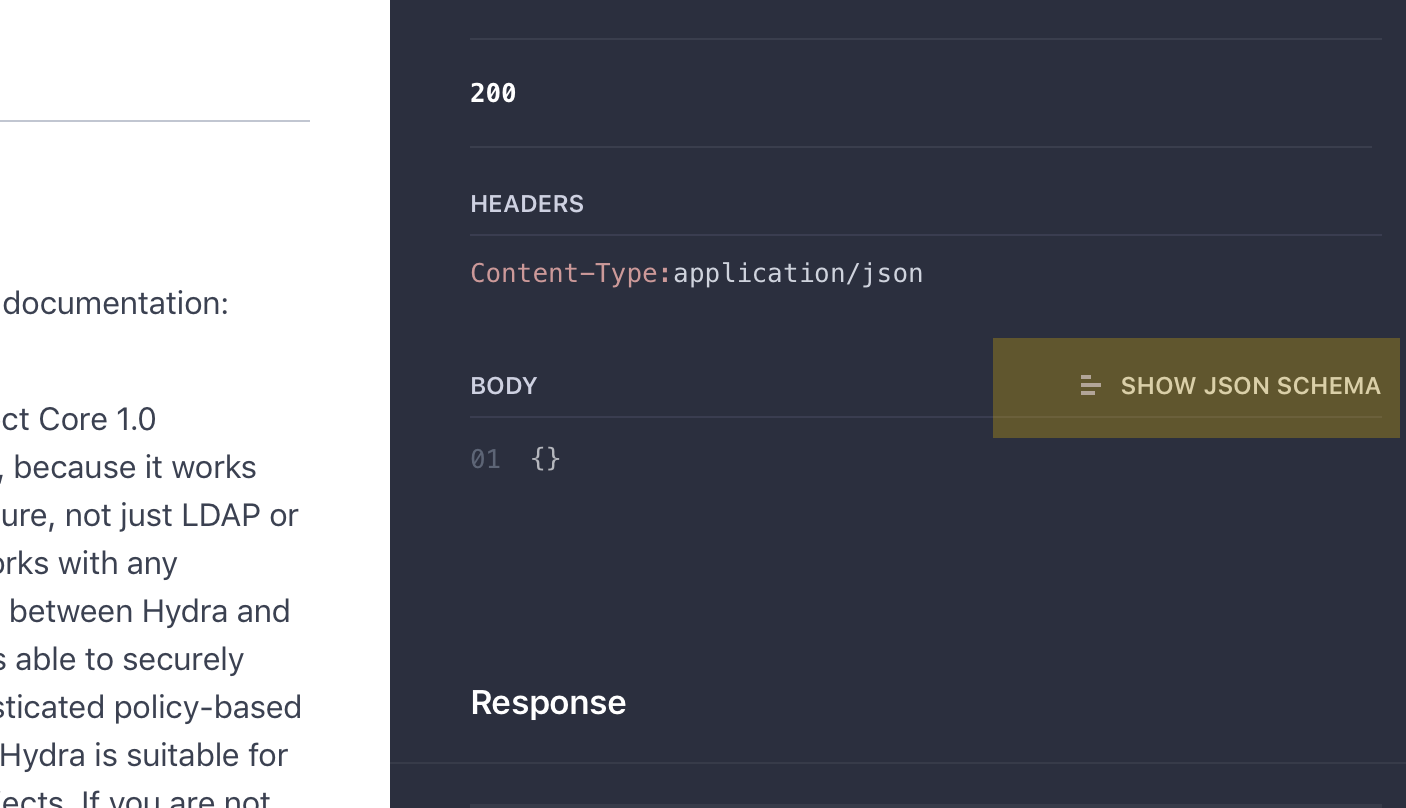 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/ConsentRequestAcceptance', 'model/ConsentRequestRejection', 'model/InlineResponse2001', 'model/InlineResponse401', 'model/JsonWebKeySet', 'model/OAuth2Client', 'model/OAuth2TokenIntrospection', 'model/OAuth2consentRequest', 'model/WellKnown'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('../model/ConsentRequestAcceptance'), require('../model/ConsentRequestRejection'), require('../model/InlineResponse2001'), require('../model/InlineResponse401'), require('../model/JsonWebKeySet'), require('../model/OAuth2Client'), require('../model/OAuth2TokenIntrospection'), require('../model/OAuth2consentRequest'), require('../model/WellKnown'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.OAuth2Api = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance, root.HydraOAuth2OpenIdConnectServer.ConsentRequestRejection, root.HydraOAuth2OpenIdConnectServer.InlineResponse2001, root.HydraOAuth2OpenIdConnectServer.InlineResponse401, root.HydraOAuth2OpenIdConnectServer.JsonWebKeySet, root.HydraOAuth2OpenIdConnectServer.OAuth2Client, root.HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection, root.HydraOAuth2OpenIdConnectServer.OAuth2consentRequest, root.HydraOAuth2OpenIdConnectServer.WellKnown);
+ }
+}(this, function(ApiClient, ConsentRequestAcceptance, ConsentRequestRejection, InlineResponse2001, InlineResponse401, JsonWebKeySet, OAuth2Client, OAuth2TokenIntrospection, OAuth2consentRequest, WellKnown) {
+ 'use strict';
+
+ /**
+ * OAuth2 service.
+ * @module api/OAuth2Api
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new OAuth2Api.
+ * @alias module:api/OAuth2Api
+ * @class
+ * @param {module:ApiClient} apiClient Optional API client implementation to use,
+ * default to {@link module:ApiClient#instance} if unspecified.
+ */
+ var exports = function(apiClient) {
+ this.apiClient = apiClient || ApiClient.instance;
+
+
+ /**
+ * Callback function to receive the result of the acceptOAuth2ConsentRequest operation.
+ * @callback module:api/OAuth2Api~acceptOAuth2ConsentRequestCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Accept a consent request
+ * Call this endpoint to accept a consent request. This usually happens when a user agrees to give access rights to an application. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"accept\"], \"effect\": \"allow\" } ```
+ * @param {String} id
+ * @param {module:model/ConsentRequestAcceptance} body
+ * @param {module:api/OAuth2Api~acceptOAuth2ConsentRequestCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.acceptOAuth2ConsentRequest = function(id, body, callback) {
+ var postBody = body;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling acceptOAuth2ConsentRequest");
+ }
+
+ // verify the required parameter 'body' is set
+ if (body === undefined || body === null) {
+ throw new Error("Missing the required parameter 'body' when calling acceptOAuth2ConsentRequest");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/oauth2/consent/requests/{id}/accept', 'PATCH',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the createOAuth2Client operation.
+ * @callback module:api/OAuth2Api~createOAuth2ClientCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/OAuth2Client} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Create an OAuth 2.0 client
+ * If you pass `client_secret` the secret will be used, otherwise a random secret will be generated. The secret will be returned in the response and you will not be able to retrieve it later on. Write the secret down and keep it somwhere safe. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"create\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+ * @param {module:model/OAuth2Client} body
+ * @param {module:api/OAuth2Api~createOAuth2ClientCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/OAuth2Client}
+ */
+ this.createOAuth2Client = function(body, callback) {
+ var postBody = body;
+
+ // verify the required parameter 'body' is set
+ if (body === undefined || body === null) {
+ throw new Error("Missing the required parameter 'body' when calling createOAuth2Client");
+ }
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = OAuth2Client;
+
+ return this.apiClient.callApi(
+ '/clients', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the deleteOAuth2Client operation.
+ * @callback module:api/OAuth2Api~deleteOAuth2ClientCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Deletes an OAuth 2.0 Client
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+ * @param {String} id The id of the OAuth 2.0 Client.
+ * @param {module:api/OAuth2Api~deleteOAuth2ClientCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.deleteOAuth2Client = function(id, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling deleteOAuth2Client");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/clients/{id}', 'DELETE',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the getOAuth2Client operation.
+ * @callback module:api/OAuth2Api~getOAuth2ClientCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/OAuth2Client} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Retrieve an OAuth 2.0 Client.
+ * This endpoint never returns passwords. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients:<some-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+ * @param {String} id The id of the OAuth 2.0 Client.
+ * @param {module:api/OAuth2Api~getOAuth2ClientCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/OAuth2Client}
+ */
+ this.getOAuth2Client = function(id, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling getOAuth2Client");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = OAuth2Client;
+
+ return this.apiClient.callApi(
+ '/clients/{id}', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the getOAuth2ConsentRequest operation.
+ * @callback module:api/OAuth2Api~getOAuth2ConsentRequestCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/OAuth2consentRequest} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Receive consent request information
+ * Call this endpoint to receive information on consent requests. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ * @param {String} id The id of the OAuth 2.0 Consent Request.
+ * @param {module:api/OAuth2Api~getOAuth2ConsentRequestCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/OAuth2consentRequest}
+ */
+ this.getOAuth2ConsentRequest = function(id, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling getOAuth2ConsentRequest");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = OAuth2consentRequest;
+
+ return this.apiClient.callApi(
+ '/oauth2/consent/requests/{id}', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the getWellKnown operation.
+ * @callback module:api/OAuth2Api~getWellKnownCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/WellKnown} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Server well known configuration
+ * The well known endpoint an be used to retrieve information for OpenID Connect clients. We encourage you to not roll your own OpenID Connect client but to use an OpenID Connect client library instead. You can learn more on this flow at https://openid.net/specs/openid-connect-discovery-1_0.html
+ * @param {module:api/OAuth2Api~getWellKnownCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/WellKnown}
+ */
+ this.getWellKnown = function(callback) {
+ var postBody = null;
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = [];
+ var contentTypes = ['application/json', 'application/x-www-form-urlencoded'];
+ var accepts = ['application/json'];
+ var returnType = WellKnown;
+
+ return this.apiClient.callApi(
+ '/.well-known/openid-configuration', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the introspectOAuth2Token operation.
+ * @callback module:api/OAuth2Api~introspectOAuth2TokenCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/OAuth2TokenIntrospection} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Introspect OAuth2 tokens
+ * The introspection endpoint allows to check if a token (both refresh and access) is active or not. An active token is neither expired nor revoked. If a token is active, additional information on the token will be included. You can set additional data for a token by setting `accessTokenExtra` during the consent flow.
+ * @param {String} token The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation.
+ * @param {Object} opts Optional parameters
+ * @param {String} opts.scope An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false.
+ * @param {module:api/OAuth2Api~introspectOAuth2TokenCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/OAuth2TokenIntrospection}
+ */
+ this.introspectOAuth2Token = function(token, opts, callback) {
+ opts = opts || {};
+ var postBody = null;
+
+ // verify the required parameter 'token' is set
+ if (token === undefined || token === null) {
+ throw new Error("Missing the required parameter 'token' when calling introspectOAuth2Token");
+ }
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ 'token': token,
+ 'scope': opts['scope']
+ };
+
+ var authNames = ['basic', 'oauth2'];
+ var contentTypes = ['application/x-www-form-urlencoded'];
+ var accepts = ['application/json'];
+ var returnType = OAuth2TokenIntrospection;
+
+ return this.apiClient.callApi(
+ '/oauth2/introspect', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the listOAuth2Clients operation.
+ * @callback module:api/OAuth2Api~listOAuth2ClientsCallback
+ * @param {String} error Error message, if any.
+ * @param {Array.} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * List OAuth 2.0 Clients
+ * This endpoint never returns passwords. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ * @param {module:api/OAuth2Api~listOAuth2ClientsCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link Array.}
+ */
+ this.listOAuth2Clients = function(callback) {
+ var postBody = null;
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = [OAuth2Client];
+
+ return this.apiClient.callApi(
+ '/clients', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the oauthAuth operation.
+ * @callback module:api/OAuth2Api~oauthAuthCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * The OAuth 2.0 authorize endpoint
+ * This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows. OAuth2 is a very popular protocol and a library for your programming language will exists. To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
+ * @param {module:api/OAuth2Api~oauthAuthCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.oauthAuth = function(callback) {
+ var postBody = null;
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = [];
+ var contentTypes = ['application/x-www-form-urlencoded'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/oauth2/auth', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the oauthToken operation.
+ * @callback module:api/OAuth2Api~oauthTokenCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/InlineResponse2001} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * The OAuth 2.0 token endpoint
+ * This endpoint is not documented here because you should never use your own implementation to perform OAuth2 flows. OAuth2 is a very popular protocol and a library for your programming language will exists. To learn more about this flow please refer to the specification: https://tools.ietf.org/html/rfc6749
+ * @param {module:api/OAuth2Api~oauthTokenCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/InlineResponse2001}
+ */
+ this.oauthToken = function(callback) {
+ var postBody = null;
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['basic', 'oauth2'];
+ var contentTypes = ['application/x-www-form-urlencoded'];
+ var accepts = ['application/json'];
+ var returnType = InlineResponse2001;
+
+ return this.apiClient.callApi(
+ '/oauth2/token', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the rejectOAuth2ConsentRequest operation.
+ * @callback module:api/OAuth2Api~rejectOAuth2ConsentRequestCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Reject a consent request
+ * Call this endpoint to reject a consent request. This usually happens when a user denies access rights to an application. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:oauth2:consent:requests:<request-id>\"], \"actions\": [\"reject\"], \"effect\": \"allow\" } ```
+ * @param {String} id
+ * @param {module:model/ConsentRequestRejection} body
+ * @param {module:api/OAuth2Api~rejectOAuth2ConsentRequestCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.rejectOAuth2ConsentRequest = function(id, body, callback) {
+ var postBody = body;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling rejectOAuth2ConsentRequest");
+ }
+
+ // verify the required parameter 'body' is set
+ if (body === undefined || body === null) {
+ throw new Error("Missing the required parameter 'body' when calling rejectOAuth2ConsentRequest");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/oauth2/consent/requests/{id}/reject', 'PATCH',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the revokeOAuth2Token operation.
+ * @callback module:api/OAuth2Api~revokeOAuth2TokenCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Revoke OAuth2 tokens
+ * Revoking a token (both access and refresh) means that the tokens will be invalid. A revoked access token can no longer be used to make access requests, and a revoked refresh token can no longer be used to refresh an access token. Revoking a refresh token also invalidates the access token that was created with it.
+ * @param {String} token
+ * @param {module:api/OAuth2Api~revokeOAuth2TokenCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.revokeOAuth2Token = function(token, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'token' is set
+ if (token === undefined || token === null) {
+ throw new Error("Missing the required parameter 'token' when calling revokeOAuth2Token");
+ }
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ 'token': token
+ };
+
+ var authNames = ['basic'];
+ var contentTypes = ['application/x-www-form-urlencoded'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/oauth2/revoke', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the updateOAuth2Client operation.
+ * @callback module:api/OAuth2Api~updateOAuth2ClientCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/OAuth2Client} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Update an OAuth 2.0 Client
+ * If you pass `client_secret` the secret will be updated and returned via the API. This is the only time you will be able to retrieve the client secret, so write it down and keep it safe. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ``` Additionally, the context key \"owner\" is set to the owner of the client, allowing policies such as: ``` { \"resources\": [\"rn:hydra:clients\"], \"actions\": [\"update\"], \"effect\": \"allow\", \"conditions\": { \"owner\": { \"type\": \"EqualsSubjectCondition\" } } } ```
+ * @param {String} id
+ * @param {module:model/OAuth2Client} body
+ * @param {module:api/OAuth2Api~updateOAuth2ClientCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/OAuth2Client}
+ */
+ this.updateOAuth2Client = function(id, body, callback) {
+ var postBody = body;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling updateOAuth2Client");
+ }
+
+ // verify the required parameter 'body' is set
+ if (body === undefined || body === null) {
+ throw new Error("Missing the required parameter 'body' when calling updateOAuth2Client");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = OAuth2Client;
+
+ return this.apiClient.callApi(
+ '/clients/{id}', 'PUT',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the wellKnown operation.
+ * @callback module:api/OAuth2Api~wellKnownCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/JsonWebKeySet} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Get list of well known JSON Web Keys
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:keys:hydra.openid.id-token:public\"], \"actions\": [\"GET\"], \"effect\": \"allow\" } ```
+ * @param {module:api/OAuth2Api~wellKnownCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/JsonWebKeySet}
+ */
+ this.wellKnown = function(callback) {
+ var postBody = null;
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = JsonWebKeySet;
+
+ return this.apiClient.callApi(
+ '/.well-known/jwks.json', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+ };
+
+ return exports;
+}));
diff --git a/sdk/js/swagger/src/api/PolicyApi.js b/sdk/js/swagger/src/api/PolicyApi.js
new file mode 100644
index 00000000000..3167c89199c
--- /dev/null
+++ b/sdk/js/swagger/src/api/PolicyApi.js
@@ -0,0 +1,280 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 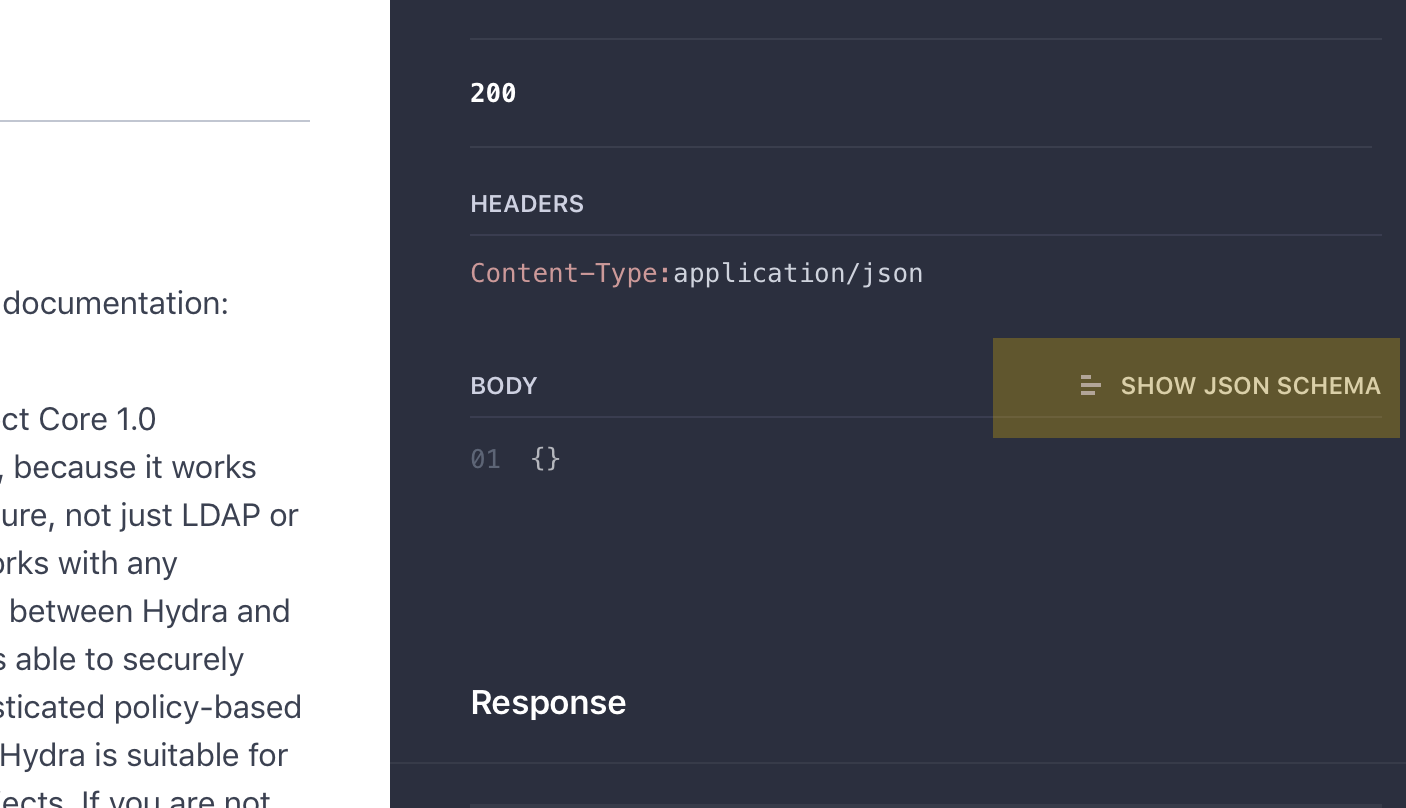 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/InlineResponse401', 'model/Policy'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('../model/InlineResponse401'), require('../model/Policy'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.PolicyApi = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.InlineResponse401, root.HydraOAuth2OpenIdConnectServer.Policy);
+ }
+}(this, function(ApiClient, InlineResponse401, Policy) {
+ 'use strict';
+
+ /**
+ * Policy service.
+ * @module api/PolicyApi
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new PolicyApi.
+ * @alias module:api/PolicyApi
+ * @class
+ * @param {module:ApiClient} apiClient Optional API client implementation to use,
+ * default to {@link module:ApiClient#instance} if unspecified.
+ */
+ var exports = function(apiClient) {
+ this.apiClient = apiClient || ApiClient.instance;
+
+
+ /**
+ * Callback function to receive the result of the createPolicy operation.
+ * @callback module:api/PolicyApi~createPolicyCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/Policy} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Create an Access Control Policy
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+ * @param {Object} opts Optional parameters
+ * @param {module:model/Policy} opts.body
+ * @param {module:api/PolicyApi~createPolicyCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/Policy}
+ */
+ this.createPolicy = function(opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = Policy;
+
+ return this.apiClient.callApi(
+ '/policies', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the deletePolicy operation.
+ * @callback module:api/PolicyApi~deletePolicyCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Delete an Access Control Policy
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies:<id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+ * @param {String} id The id of the policy.
+ * @param {module:api/PolicyApi~deletePolicyCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.deletePolicy = function(id, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling deletePolicy");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/policies/{id}', 'DELETE',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the getPolicy operation.
+ * @callback module:api/PolicyApi~getPolicyCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/Policy} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Get an Access Control Policy
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies:<id>\"], \"actions\": [\"get\"], \"effect\": \"allow\" } ```
+ * @param {String} id The id of the policy.
+ * @param {module:api/PolicyApi~getPolicyCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/Policy}
+ */
+ this.getPolicy = function(id, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling getPolicy");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = Policy;
+
+ return this.apiClient.callApi(
+ '/policies/{id}', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the listPolicies operation.
+ * @callback module:api/PolicyApi~listPoliciesCallback
+ * @param {String} error Error message, if any.
+ * @param {Array.} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * List Access Control Policies
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"list\"], \"effect\": \"allow\" } ```
+ * @param {Object} opts Optional parameters
+ * @param {Number} opts.offset The offset from where to start looking.
+ * @param {Number} opts.limit The maximum amount of policies returned.
+ * @param {module:api/PolicyApi~listPoliciesCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link Array.}
+ */
+ this.listPolicies = function(opts, callback) {
+ opts = opts || {};
+ var postBody = null;
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ 'offset': opts['offset'],
+ 'limit': opts['limit']
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = [Policy];
+
+ return this.apiClient.callApi(
+ '/policies', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the updatePolicy operation.
+ * @callback module:api/PolicyApi~updatePolicyCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/Policy} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Update an Access Control Polic
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:policies\"], \"actions\": [\"update\"], \"effect\": \"allow\" } ```
+ * @param {String} id The id of the policy.
+ * @param {Object} opts Optional parameters
+ * @param {module:model/Policy} opts.body
+ * @param {module:api/PolicyApi~updatePolicyCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/Policy}
+ */
+ this.updatePolicy = function(id, opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling updatePolicy");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = Policy;
+
+ return this.apiClient.callApi(
+ '/policies/{id}', 'PUT',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+ };
+
+ return exports;
+}));
diff --git a/sdk/js/swagger/src/api/WardenApi.js b/sdk/js/swagger/src/api/WardenApi.js
new file mode 100644
index 00000000000..9bbd33af103
--- /dev/null
+++ b/sdk/js/swagger/src/api/WardenApi.js
@@ -0,0 +1,412 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 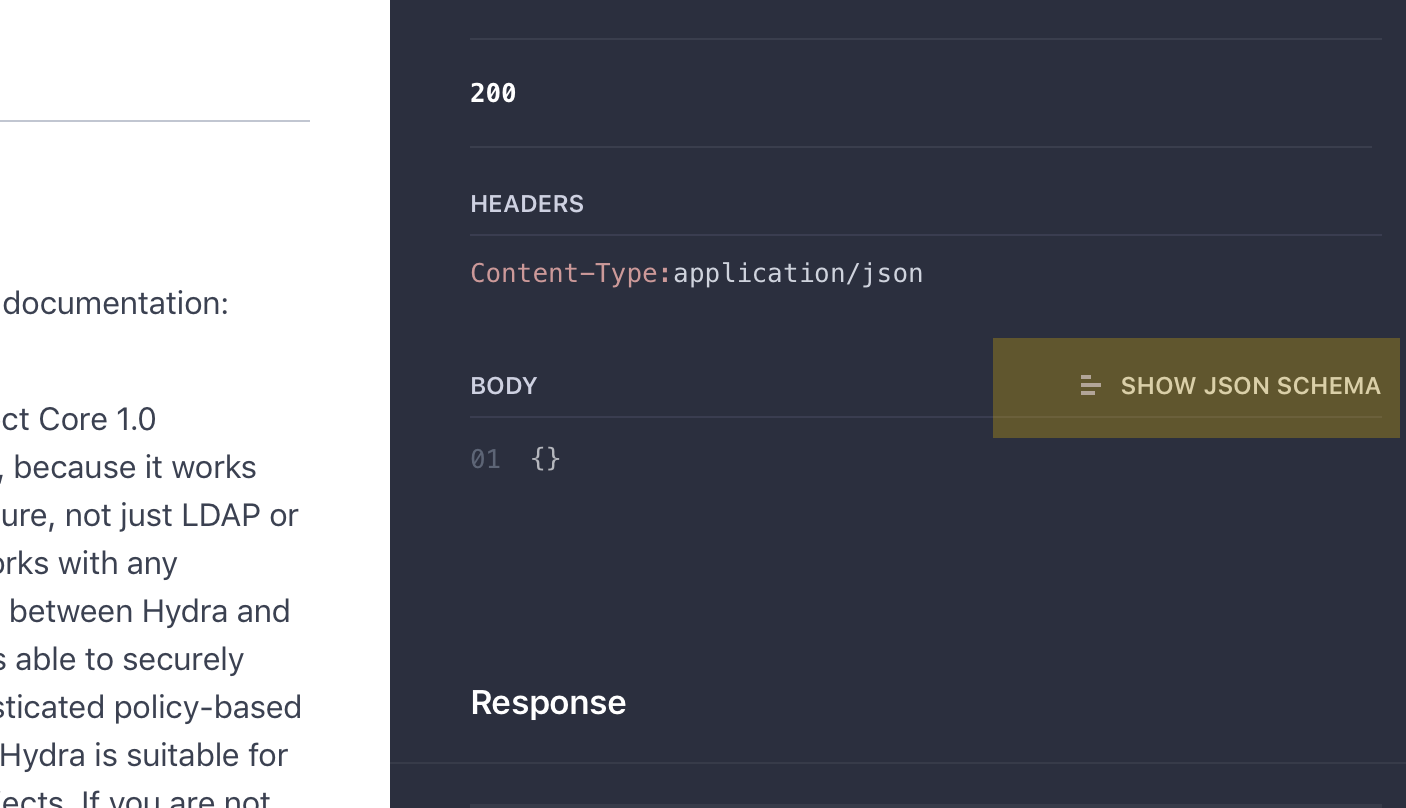 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/Group', 'model/GroupMembers', 'model/InlineResponse401', 'model/WardenAccessRequest', 'model/WardenAccessRequestResponse', 'model/WardenTokenAccessRequest', 'model/WardenTokenAccessRequestResponsePayload'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('../model/Group'), require('../model/GroupMembers'), require('../model/InlineResponse401'), require('../model/WardenAccessRequest'), require('../model/WardenAccessRequestResponse'), require('../model/WardenTokenAccessRequest'), require('../model/WardenTokenAccessRequestResponsePayload'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.WardenApi = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.Group, root.HydraOAuth2OpenIdConnectServer.GroupMembers, root.HydraOAuth2OpenIdConnectServer.InlineResponse401, root.HydraOAuth2OpenIdConnectServer.WardenAccessRequest, root.HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse, root.HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest, root.HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload);
+ }
+}(this, function(ApiClient, Group, GroupMembers, InlineResponse401, WardenAccessRequest, WardenAccessRequestResponse, WardenTokenAccessRequest, WardenTokenAccessRequestResponsePayload) {
+ 'use strict';
+
+ /**
+ * Warden service.
+ * @module api/WardenApi
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new WardenApi.
+ * @alias module:api/WardenApi
+ * @class
+ * @param {module:ApiClient} apiClient Optional API client implementation to use,
+ * default to {@link module:ApiClient#instance} if unspecified.
+ */
+ var exports = function(apiClient) {
+ this.apiClient = apiClient || ApiClient.instance;
+
+
+ /**
+ * Callback function to receive the result of the addMembersToGroup operation.
+ * @callback module:api/WardenApi~addMembersToGroupCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Add members to a group
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"members.add\"], \"effect\": \"allow\" } ```
+ * @param {String} id The id of the group to modify.
+ * @param {Object} opts Optional parameters
+ * @param {module:model/GroupMembers} opts.body
+ * @param {module:api/WardenApi~addMembersToGroupCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.addMembersToGroup = function(id, opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling addMembersToGroup");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/warden/groups/{id}/members', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the createGroup operation.
+ * @callback module:api/WardenApi~createGroupCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/Group} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Create a group
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+ * @param {Object} opts Optional parameters
+ * @param {module:model/Group} opts.body
+ * @param {module:api/WardenApi~createGroupCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/Group}
+ */
+ this.createGroup = function(opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = Group;
+
+ return this.apiClient.callApi(
+ '/warden/groups', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the deleteGroup operation.
+ * @callback module:api/WardenApi~deleteGroupCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Delete a group by id
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"delete\"], \"effect\": \"allow\" } ```
+ * @param {String} id The id of the group to look up.
+ * @param {module:api/WardenApi~deleteGroupCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.deleteGroup = function(id, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling deleteGroup");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/warden/groups/{id}', 'DELETE',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the doesWardenAllowAccessRequest operation.
+ * @callback module:api/WardenApi~doesWardenAllowAccessRequestCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/WardenAccessRequestResponse} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Check if an access request is valid (without providing an access token)
+ * Checks if a subject (typically a user or a service) is allowed to perform an action on a resource. This endpoint requires a subject, a resource name, an action name and a context. If the subject is not allowed to perform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`, otherwise `{ \"allowed\": true }` is returned. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:allowed\"], \"actions\": [\"decide\"], \"effect\": \"allow\" } ```
+ * @param {Object} opts Optional parameters
+ * @param {module:model/WardenAccessRequest} opts.body
+ * @param {module:api/WardenApi~doesWardenAllowAccessRequestCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/WardenAccessRequestResponse}
+ */
+ this.doesWardenAllowAccessRequest = function(opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = WardenAccessRequestResponse;
+
+ return this.apiClient.callApi(
+ '/warden/allowed', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the doesWardenAllowTokenAccessRequest operation.
+ * @callback module:api/WardenApi~doesWardenAllowTokenAccessRequestCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/WardenTokenAccessRequestResponsePayload} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Check if an access request is valid (providing an access token)
+ * Checks if a token is valid and if the token subject is allowed to perform an action on a resource. This endpoint requires a token, a scope, a resource name, an action name and a context. If a token is expired/invalid, has not been granted the requested scope or the subject is not allowed to perform the action on the resource, this endpoint returns a 200 response with `{ \"allowed\": false}`. Extra data set through the `accessTokenExtra` field in the consent flow will be included in the response. The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:token:allowed\"], \"actions\": [\"decide\"], \"effect\": \"allow\" } ```
+ * @param {Object} opts Optional parameters
+ * @param {module:model/WardenTokenAccessRequest} opts.body
+ * @param {module:api/WardenApi~doesWardenAllowTokenAccessRequestCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/WardenTokenAccessRequestResponsePayload}
+ */
+ this.doesWardenAllowTokenAccessRequest = function(opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = WardenTokenAccessRequestResponsePayload;
+
+ return this.apiClient.callApi(
+ '/warden/token/allowed', 'POST',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the findGroupsByMember operation.
+ * @callback module:api/WardenApi~findGroupsByMemberCallback
+ * @param {String} error Error message, if any.
+ * @param {Array.} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Find groups by member
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups\"], \"actions\": [\"list\"], \"effect\": \"allow\" } ```
+ * @param {String} member The id of the member to look up.
+ * @param {module:api/WardenApi~findGroupsByMemberCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link Array.}
+ */
+ this.findGroupsByMember = function(member, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'member' is set
+ if (member === undefined || member === null) {
+ throw new Error("Missing the required parameter 'member' when calling findGroupsByMember");
+ }
+
+
+ var pathParams = {
+ };
+ var queryParams = {
+ 'member': member
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = [Group];
+
+ return this.apiClient.callApi(
+ '/warden/groups', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the getGroup operation.
+ * @callback module:api/WardenApi~getGroupCallback
+ * @param {String} error Error message, if any.
+ * @param {module:model/Group} data The data returned by the service call.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Get a group by id
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"create\"], \"effect\": \"allow\" } ```
+ * @param {String} id The id of the group to look up.
+ * @param {module:api/WardenApi~getGroupCallback} callback The callback function, accepting three arguments: error, data, response
+ * data is of type: {@link module:model/Group}
+ */
+ this.getGroup = function(id, callback) {
+ var postBody = null;
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling getGroup");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = Group;
+
+ return this.apiClient.callApi(
+ '/warden/groups/{id}', 'GET',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+
+ /**
+ * Callback function to receive the result of the removeMembersFromGroup operation.
+ * @callback module:api/WardenApi~removeMembersFromGroupCallback
+ * @param {String} error Error message, if any.
+ * @param data This operation does not return a value.
+ * @param {String} response The complete HTTP response.
+ */
+
+ /**
+ * Remove members from a group
+ * The subject making the request needs to be assigned to a policy containing: ``` { \"resources\": [\"rn:hydra:warden:groups:<id>\"], \"actions\": [\"members.remove\"], \"effect\": \"allow\" } ```
+ * @param {String} id The id of the group to modify.
+ * @param {Object} opts Optional parameters
+ * @param {module:model/GroupMembers} opts.body
+ * @param {module:api/WardenApi~removeMembersFromGroupCallback} callback The callback function, accepting three arguments: error, data, response
+ */
+ this.removeMembersFromGroup = function(id, opts, callback) {
+ opts = opts || {};
+ var postBody = opts['body'];
+
+ // verify the required parameter 'id' is set
+ if (id === undefined || id === null) {
+ throw new Error("Missing the required parameter 'id' when calling removeMembersFromGroup");
+ }
+
+
+ var pathParams = {
+ 'id': id
+ };
+ var queryParams = {
+ };
+ var headerParams = {
+ };
+ var formParams = {
+ };
+
+ var authNames = ['oauth2'];
+ var contentTypes = ['application/json'];
+ var accepts = ['application/json'];
+ var returnType = null;
+
+ return this.apiClient.callApi(
+ '/warden/groups/{id}/members', 'DELETE',
+ pathParams, queryParams, headerParams, formParams, postBody,
+ authNames, contentTypes, accepts, returnType, callback
+ );
+ }
+ };
+
+ return exports;
+}));
diff --git a/sdk/js/swagger/src/index.js b/sdk/js/swagger/src/index.js
new file mode 100644
index 00000000000..32f05b5eac7
--- /dev/null
+++ b/sdk/js/swagger/src/index.js
@@ -0,0 +1,358 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 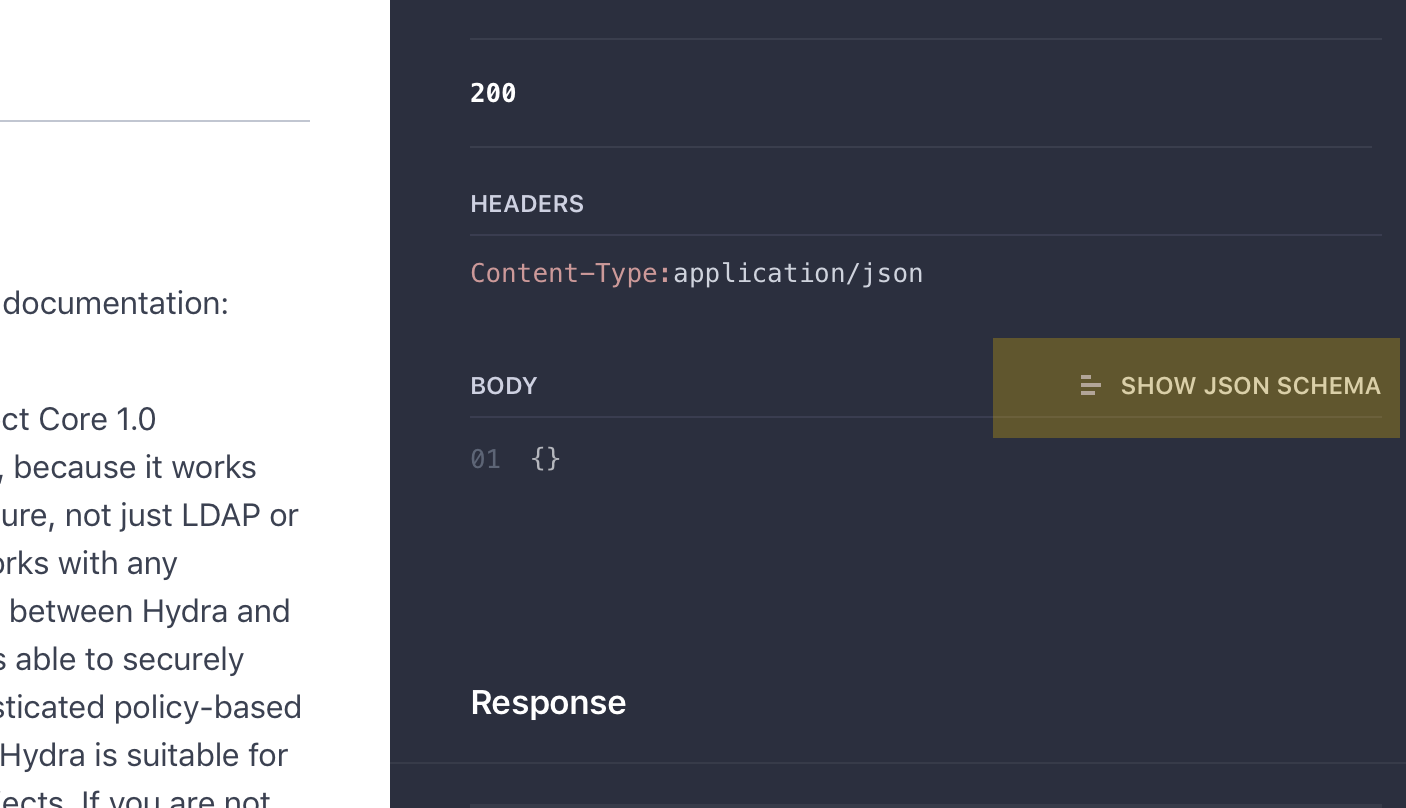 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/ConsentRequestAcceptance', 'model/ConsentRequestManager', 'model/ConsentRequestRejection', 'model/Context', 'model/Firewall', 'model/Group', 'model/GroupMembers', 'model/Handler', 'model/InlineResponse200', 'model/InlineResponse2001', 'model/InlineResponse401', 'model/JoseWebKeySetRequest', 'model/JsonWebKey', 'model/JsonWebKeySet', 'model/JsonWebKeySetGeneratorRequest', 'model/KeyGenerator', 'model/Manager', 'model/OAuth2Client', 'model/OAuth2TokenIntrospection', 'model/OAuth2consentRequest', 'model/Policy', 'model/PolicyConditions', 'model/RawMessage', 'model/SwaggerAcceptConsentRequest', 'model/SwaggerCreatePolicyParameters', 'model/SwaggerDoesWardenAllowAccessRequestParameters', 'model/SwaggerDoesWardenAllowTokenAccessRequestParameters', 'model/SwaggerGetPolicyParameters', 'model/SwaggerJsonWebKeyQuery', 'model/SwaggerJwkCreateSet', 'model/SwaggerJwkSetQuery', 'model/SwaggerJwkUpdateSet', 'model/SwaggerJwkUpdateSetKey', 'model/SwaggerListPolicyParameters', 'model/SwaggerListPolicyResponse', 'model/SwaggerOAuthConsentRequest', 'model/SwaggerOAuthConsentRequestPayload', 'model/SwaggerOAuthIntrospectionRequest', 'model/SwaggerOAuthIntrospectionResponse', 'model/SwaggerOAuthTokenResponse', 'model/SwaggerOAuthTokenResponseBody', 'model/SwaggerRejectConsentRequest', 'model/SwaggerRevokeOAuth2TokenParameters', 'model/SwaggerUpdatePolicyParameters', 'model/SwaggerWardenAccessRequestResponseParameters', 'model/SwaggerWardenTokenAccessRequestResponse', 'model/TokenAllowedRequest', 'model/WardenAccessRequest', 'model/WardenAccessRequestResponse', 'model/WardenTokenAccessRequest', 'model/WardenTokenAccessRequestResponsePayload', 'model/WellKnown', 'model/Writer', 'api/HealthApi', 'api/JsonWebKeyApi', 'api/OAuth2Api', 'api/PolicyApi', 'api/WardenApi'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('./ApiClient'), require('./model/ConsentRequestAcceptance'), require('./model/ConsentRequestManager'), require('./model/ConsentRequestRejection'), require('./model/Context'), require('./model/Firewall'), require('./model/Group'), require('./model/GroupMembers'), require('./model/Handler'), require('./model/InlineResponse200'), require('./model/InlineResponse2001'), require('./model/InlineResponse401'), require('./model/JoseWebKeySetRequest'), require('./model/JsonWebKey'), require('./model/JsonWebKeySet'), require('./model/JsonWebKeySetGeneratorRequest'), require('./model/KeyGenerator'), require('./model/Manager'), require('./model/OAuth2Client'), require('./model/OAuth2TokenIntrospection'), require('./model/OAuth2consentRequest'), require('./model/Policy'), require('./model/PolicyConditions'), require('./model/RawMessage'), require('./model/SwaggerAcceptConsentRequest'), require('./model/SwaggerCreatePolicyParameters'), require('./model/SwaggerDoesWardenAllowAccessRequestParameters'), require('./model/SwaggerDoesWardenAllowTokenAccessRequestParameters'), require('./model/SwaggerGetPolicyParameters'), require('./model/SwaggerJsonWebKeyQuery'), require('./model/SwaggerJwkCreateSet'), require('./model/SwaggerJwkSetQuery'), require('./model/SwaggerJwkUpdateSet'), require('./model/SwaggerJwkUpdateSetKey'), require('./model/SwaggerListPolicyParameters'), require('./model/SwaggerListPolicyResponse'), require('./model/SwaggerOAuthConsentRequest'), require('./model/SwaggerOAuthConsentRequestPayload'), require('./model/SwaggerOAuthIntrospectionRequest'), require('./model/SwaggerOAuthIntrospectionResponse'), require('./model/SwaggerOAuthTokenResponse'), require('./model/SwaggerOAuthTokenResponseBody'), require('./model/SwaggerRejectConsentRequest'), require('./model/SwaggerRevokeOAuth2TokenParameters'), require('./model/SwaggerUpdatePolicyParameters'), require('./model/SwaggerWardenAccessRequestResponseParameters'), require('./model/SwaggerWardenTokenAccessRequestResponse'), require('./model/TokenAllowedRequest'), require('./model/WardenAccessRequest'), require('./model/WardenAccessRequestResponse'), require('./model/WardenTokenAccessRequest'), require('./model/WardenTokenAccessRequestResponsePayload'), require('./model/WellKnown'), require('./model/Writer'), require('./api/HealthApi'), require('./api/JsonWebKeyApi'), require('./api/OAuth2Api'), require('./api/PolicyApi'), require('./api/WardenApi'));
+ }
+}(function(ApiClient, ConsentRequestAcceptance, ConsentRequestManager, ConsentRequestRejection, Context, Firewall, Group, GroupMembers, Handler, InlineResponse200, InlineResponse2001, InlineResponse401, JoseWebKeySetRequest, JsonWebKey, JsonWebKeySet, JsonWebKeySetGeneratorRequest, KeyGenerator, Manager, OAuth2Client, OAuth2TokenIntrospection, OAuth2consentRequest, Policy, PolicyConditions, RawMessage, SwaggerAcceptConsentRequest, SwaggerCreatePolicyParameters, SwaggerDoesWardenAllowAccessRequestParameters, SwaggerDoesWardenAllowTokenAccessRequestParameters, SwaggerGetPolicyParameters, SwaggerJsonWebKeyQuery, SwaggerJwkCreateSet, SwaggerJwkSetQuery, SwaggerJwkUpdateSet, SwaggerJwkUpdateSetKey, SwaggerListPolicyParameters, SwaggerListPolicyResponse, SwaggerOAuthConsentRequest, SwaggerOAuthConsentRequestPayload, SwaggerOAuthIntrospectionRequest, SwaggerOAuthIntrospectionResponse, SwaggerOAuthTokenResponse, SwaggerOAuthTokenResponseBody, SwaggerRejectConsentRequest, SwaggerRevokeOAuth2TokenParameters, SwaggerUpdatePolicyParameters, SwaggerWardenAccessRequestResponseParameters, SwaggerWardenTokenAccessRequestResponse, TokenAllowedRequest, WardenAccessRequest, WardenAccessRequestResponse, WardenTokenAccessRequest, WardenTokenAccessRequestResponsePayload, WellKnown, Writer, HealthApi, JsonWebKeyApi, OAuth2Api, PolicyApi, WardenApi) {
+ 'use strict';
+
+ /**
+ * Please_refer_to_the_user_guide_for_in_depth_documentation_httpsory_gitbooks_iohydracontentHydra_offers_OAuth_2_0_and_OpenID_Connect_Core_1_0_capabilities_as_a_service__Hydra_is_different_because_it_works_with_any_existing_authentication_infrastructure_not_just_LDAP_or_SAML__By_implementing_a_consent_app__works_with_any_programming_language_you_build_a_bridge_between_Hydra_and_your_authentication_infrastructure_Hydra_is_able_to_securely_manage_JSON_Web_Keys_and_has_a_sophisticated_policy_based_access_control_you_can_use_if_you_want_to_Hydra_is_suitable_for_green___new_and_brownfield__existing_projects__If_you_are_not_familiar_with_OAuth_2_0_and_are_working_on_a_greenfield_project_we_recommend_evaluating_if_OAuth_2_0_really_serves_your_purpose__Knowledge_of_OAuth_2_0_is_imperative_in_understanding_what_Hydra_does_and_how_it_works_The_official_repository_is_located_at_httpsgithub_comoryhydra_Important_REST_API_Documentation_NotesThe_swagger_generator_used_to_create_this_documentation_does_currently_not_support_example_responses__To_seerequest_and_response_payloads_click_on_Show_JSON_schema_Enable_JSON_Schema_on_Apiary_httpsstorage_googleapis_comory_amhydrajson_schema_pngThe_API_documentation_always_refers_to_the_latest_tagged_version_of_ORY_Hydra__For_previous_API_documentations_pleaserefer_to_httpsgithub_comoryhydrablobtag_iddocsapi_swagger_yaml___for_example0_9_13_httpsgithub_comoryhydrablobv0_9_13docsapi_swagger_yaml0_8_1_httpsgithub_comoryhydrablobv0_8_1docsapi_swagger_yaml.
+ * The index
module provides access to constructors for all the classes which comprise the public API.
+ *
+ * An AMD (recommended!) or CommonJS application will generally do something equivalent to the following:
+ *
+ * var HydraOAuth2OpenIdConnectServer = require('index'); // See note below*.
+ * var xxxSvc = new HydraOAuth2OpenIdConnectServer.XxxApi(); // Allocate the API class we're going to use.
+ * var yyyModel = new HydraOAuth2OpenIdConnectServer.Yyy(); // Construct a model instance.
+ * yyyModel.someProperty = 'someValue';
+ * ...
+ * var zzz = xxxSvc.doSomething(yyyModel); // Invoke the service.
+ * ...
+ *
+ * *NOTE: For a top-level AMD script, use require(['index'], function(){...})
+ * and put the application logic within the callback function.
+ *
+ *
+ * A non-AMD browser application (discouraged) might do something like this:
+ *
+ * var xxxSvc = new HydraOAuth2OpenIdConnectServer.XxxApi(); // Allocate the API class we're going to use.
+ * var yyy = new HydraOAuth2OpenIdConnectServer.Yyy(); // Construct a model instance.
+ * yyyModel.someProperty = 'someValue';
+ * ...
+ * var zzz = xxxSvc.doSomething(yyyModel); // Invoke the service.
+ * ...
+ *
+ *
+ * @module index
+ * @version Latest
+ */
+ var exports = {
+ /**
+ * The ApiClient constructor.
+ * @property {module:ApiClient}
+ */
+ ApiClient: ApiClient,
+ /**
+ * The ConsentRequestAcceptance model constructor.
+ * @property {module:model/ConsentRequestAcceptance}
+ */
+ ConsentRequestAcceptance: ConsentRequestAcceptance,
+ /**
+ * The ConsentRequestManager model constructor.
+ * @property {module:model/ConsentRequestManager}
+ */
+ ConsentRequestManager: ConsentRequestManager,
+ /**
+ * The ConsentRequestRejection model constructor.
+ * @property {module:model/ConsentRequestRejection}
+ */
+ ConsentRequestRejection: ConsentRequestRejection,
+ /**
+ * The Context model constructor.
+ * @property {module:model/Context}
+ */
+ Context: Context,
+ /**
+ * The Firewall model constructor.
+ * @property {module:model/Firewall}
+ */
+ Firewall: Firewall,
+ /**
+ * The Group model constructor.
+ * @property {module:model/Group}
+ */
+ Group: Group,
+ /**
+ * The GroupMembers model constructor.
+ * @property {module:model/GroupMembers}
+ */
+ GroupMembers: GroupMembers,
+ /**
+ * The Handler model constructor.
+ * @property {module:model/Handler}
+ */
+ Handler: Handler,
+ /**
+ * The InlineResponse200 model constructor.
+ * @property {module:model/InlineResponse200}
+ */
+ InlineResponse200: InlineResponse200,
+ /**
+ * The InlineResponse2001 model constructor.
+ * @property {module:model/InlineResponse2001}
+ */
+ InlineResponse2001: InlineResponse2001,
+ /**
+ * The InlineResponse401 model constructor.
+ * @property {module:model/InlineResponse401}
+ */
+ InlineResponse401: InlineResponse401,
+ /**
+ * The JoseWebKeySetRequest model constructor.
+ * @property {module:model/JoseWebKeySetRequest}
+ */
+ JoseWebKeySetRequest: JoseWebKeySetRequest,
+ /**
+ * The JsonWebKey model constructor.
+ * @property {module:model/JsonWebKey}
+ */
+ JsonWebKey: JsonWebKey,
+ /**
+ * The JsonWebKeySet model constructor.
+ * @property {module:model/JsonWebKeySet}
+ */
+ JsonWebKeySet: JsonWebKeySet,
+ /**
+ * The JsonWebKeySetGeneratorRequest model constructor.
+ * @property {module:model/JsonWebKeySetGeneratorRequest}
+ */
+ JsonWebKeySetGeneratorRequest: JsonWebKeySetGeneratorRequest,
+ /**
+ * The KeyGenerator model constructor.
+ * @property {module:model/KeyGenerator}
+ */
+ KeyGenerator: KeyGenerator,
+ /**
+ * The Manager model constructor.
+ * @property {module:model/Manager}
+ */
+ Manager: Manager,
+ /**
+ * The OAuth2Client model constructor.
+ * @property {module:model/OAuth2Client}
+ */
+ OAuth2Client: OAuth2Client,
+ /**
+ * The OAuth2TokenIntrospection model constructor.
+ * @property {module:model/OAuth2TokenIntrospection}
+ */
+ OAuth2TokenIntrospection: OAuth2TokenIntrospection,
+ /**
+ * The OAuth2consentRequest model constructor.
+ * @property {module:model/OAuth2consentRequest}
+ */
+ OAuth2consentRequest: OAuth2consentRequest,
+ /**
+ * The Policy model constructor.
+ * @property {module:model/Policy}
+ */
+ Policy: Policy,
+ /**
+ * The PolicyConditions model constructor.
+ * @property {module:model/PolicyConditions}
+ */
+ PolicyConditions: PolicyConditions,
+ /**
+ * The RawMessage model constructor.
+ * @property {module:model/RawMessage}
+ */
+ RawMessage: RawMessage,
+ /**
+ * The SwaggerAcceptConsentRequest model constructor.
+ * @property {module:model/SwaggerAcceptConsentRequest}
+ */
+ SwaggerAcceptConsentRequest: SwaggerAcceptConsentRequest,
+ /**
+ * The SwaggerCreatePolicyParameters model constructor.
+ * @property {module:model/SwaggerCreatePolicyParameters}
+ */
+ SwaggerCreatePolicyParameters: SwaggerCreatePolicyParameters,
+ /**
+ * The SwaggerDoesWardenAllowAccessRequestParameters model constructor.
+ * @property {module:model/SwaggerDoesWardenAllowAccessRequestParameters}
+ */
+ SwaggerDoesWardenAllowAccessRequestParameters: SwaggerDoesWardenAllowAccessRequestParameters,
+ /**
+ * The SwaggerDoesWardenAllowTokenAccessRequestParameters model constructor.
+ * @property {module:model/SwaggerDoesWardenAllowTokenAccessRequestParameters}
+ */
+ SwaggerDoesWardenAllowTokenAccessRequestParameters: SwaggerDoesWardenAllowTokenAccessRequestParameters,
+ /**
+ * The SwaggerGetPolicyParameters model constructor.
+ * @property {module:model/SwaggerGetPolicyParameters}
+ */
+ SwaggerGetPolicyParameters: SwaggerGetPolicyParameters,
+ /**
+ * The SwaggerJsonWebKeyQuery model constructor.
+ * @property {module:model/SwaggerJsonWebKeyQuery}
+ */
+ SwaggerJsonWebKeyQuery: SwaggerJsonWebKeyQuery,
+ /**
+ * The SwaggerJwkCreateSet model constructor.
+ * @property {module:model/SwaggerJwkCreateSet}
+ */
+ SwaggerJwkCreateSet: SwaggerJwkCreateSet,
+ /**
+ * The SwaggerJwkSetQuery model constructor.
+ * @property {module:model/SwaggerJwkSetQuery}
+ */
+ SwaggerJwkSetQuery: SwaggerJwkSetQuery,
+ /**
+ * The SwaggerJwkUpdateSet model constructor.
+ * @property {module:model/SwaggerJwkUpdateSet}
+ */
+ SwaggerJwkUpdateSet: SwaggerJwkUpdateSet,
+ /**
+ * The SwaggerJwkUpdateSetKey model constructor.
+ * @property {module:model/SwaggerJwkUpdateSetKey}
+ */
+ SwaggerJwkUpdateSetKey: SwaggerJwkUpdateSetKey,
+ /**
+ * The SwaggerListPolicyParameters model constructor.
+ * @property {module:model/SwaggerListPolicyParameters}
+ */
+ SwaggerListPolicyParameters: SwaggerListPolicyParameters,
+ /**
+ * The SwaggerListPolicyResponse model constructor.
+ * @property {module:model/SwaggerListPolicyResponse}
+ */
+ SwaggerListPolicyResponse: SwaggerListPolicyResponse,
+ /**
+ * The SwaggerOAuthConsentRequest model constructor.
+ * @property {module:model/SwaggerOAuthConsentRequest}
+ */
+ SwaggerOAuthConsentRequest: SwaggerOAuthConsentRequest,
+ /**
+ * The SwaggerOAuthConsentRequestPayload model constructor.
+ * @property {module:model/SwaggerOAuthConsentRequestPayload}
+ */
+ SwaggerOAuthConsentRequestPayload: SwaggerOAuthConsentRequestPayload,
+ /**
+ * The SwaggerOAuthIntrospectionRequest model constructor.
+ * @property {module:model/SwaggerOAuthIntrospectionRequest}
+ */
+ SwaggerOAuthIntrospectionRequest: SwaggerOAuthIntrospectionRequest,
+ /**
+ * The SwaggerOAuthIntrospectionResponse model constructor.
+ * @property {module:model/SwaggerOAuthIntrospectionResponse}
+ */
+ SwaggerOAuthIntrospectionResponse: SwaggerOAuthIntrospectionResponse,
+ /**
+ * The SwaggerOAuthTokenResponse model constructor.
+ * @property {module:model/SwaggerOAuthTokenResponse}
+ */
+ SwaggerOAuthTokenResponse: SwaggerOAuthTokenResponse,
+ /**
+ * The SwaggerOAuthTokenResponseBody model constructor.
+ * @property {module:model/SwaggerOAuthTokenResponseBody}
+ */
+ SwaggerOAuthTokenResponseBody: SwaggerOAuthTokenResponseBody,
+ /**
+ * The SwaggerRejectConsentRequest model constructor.
+ * @property {module:model/SwaggerRejectConsentRequest}
+ */
+ SwaggerRejectConsentRequest: SwaggerRejectConsentRequest,
+ /**
+ * The SwaggerRevokeOAuth2TokenParameters model constructor.
+ * @property {module:model/SwaggerRevokeOAuth2TokenParameters}
+ */
+ SwaggerRevokeOAuth2TokenParameters: SwaggerRevokeOAuth2TokenParameters,
+ /**
+ * The SwaggerUpdatePolicyParameters model constructor.
+ * @property {module:model/SwaggerUpdatePolicyParameters}
+ */
+ SwaggerUpdatePolicyParameters: SwaggerUpdatePolicyParameters,
+ /**
+ * The SwaggerWardenAccessRequestResponseParameters model constructor.
+ * @property {module:model/SwaggerWardenAccessRequestResponseParameters}
+ */
+ SwaggerWardenAccessRequestResponseParameters: SwaggerWardenAccessRequestResponseParameters,
+ /**
+ * The SwaggerWardenTokenAccessRequestResponse model constructor.
+ * @property {module:model/SwaggerWardenTokenAccessRequestResponse}
+ */
+ SwaggerWardenTokenAccessRequestResponse: SwaggerWardenTokenAccessRequestResponse,
+ /**
+ * The TokenAllowedRequest model constructor.
+ * @property {module:model/TokenAllowedRequest}
+ */
+ TokenAllowedRequest: TokenAllowedRequest,
+ /**
+ * The WardenAccessRequest model constructor.
+ * @property {module:model/WardenAccessRequest}
+ */
+ WardenAccessRequest: WardenAccessRequest,
+ /**
+ * The WardenAccessRequestResponse model constructor.
+ * @property {module:model/WardenAccessRequestResponse}
+ */
+ WardenAccessRequestResponse: WardenAccessRequestResponse,
+ /**
+ * The WardenTokenAccessRequest model constructor.
+ * @property {module:model/WardenTokenAccessRequest}
+ */
+ WardenTokenAccessRequest: WardenTokenAccessRequest,
+ /**
+ * The WardenTokenAccessRequestResponsePayload model constructor.
+ * @property {module:model/WardenTokenAccessRequestResponsePayload}
+ */
+ WardenTokenAccessRequestResponsePayload: WardenTokenAccessRequestResponsePayload,
+ /**
+ * The WellKnown model constructor.
+ * @property {module:model/WellKnown}
+ */
+ WellKnown: WellKnown,
+ /**
+ * The Writer model constructor.
+ * @property {module:model/Writer}
+ */
+ Writer: Writer,
+ /**
+ * The HealthApi service constructor.
+ * @property {module:api/HealthApi}
+ */
+ HealthApi: HealthApi,
+ /**
+ * The JsonWebKeyApi service constructor.
+ * @property {module:api/JsonWebKeyApi}
+ */
+ JsonWebKeyApi: JsonWebKeyApi,
+ /**
+ * The OAuth2Api service constructor.
+ * @property {module:api/OAuth2Api}
+ */
+ OAuth2Api: OAuth2Api,
+ /**
+ * The PolicyApi service constructor.
+ * @property {module:api/PolicyApi}
+ */
+ PolicyApi: PolicyApi,
+ /**
+ * The WardenApi service constructor.
+ * @property {module:api/WardenApi}
+ */
+ WardenApi: WardenApi
+ };
+
+ return exports;
+}));
diff --git a/sdk/js/swagger/src/model/ConsentRequestAcceptance.js b/sdk/js/swagger/src/model/ConsentRequestAcceptance.js
new file mode 100644
index 00000000000..da7c64fc962
--- /dev/null
+++ b/sdk/js/swagger/src/model/ConsentRequestAcceptance.js
@@ -0,0 +1,110 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 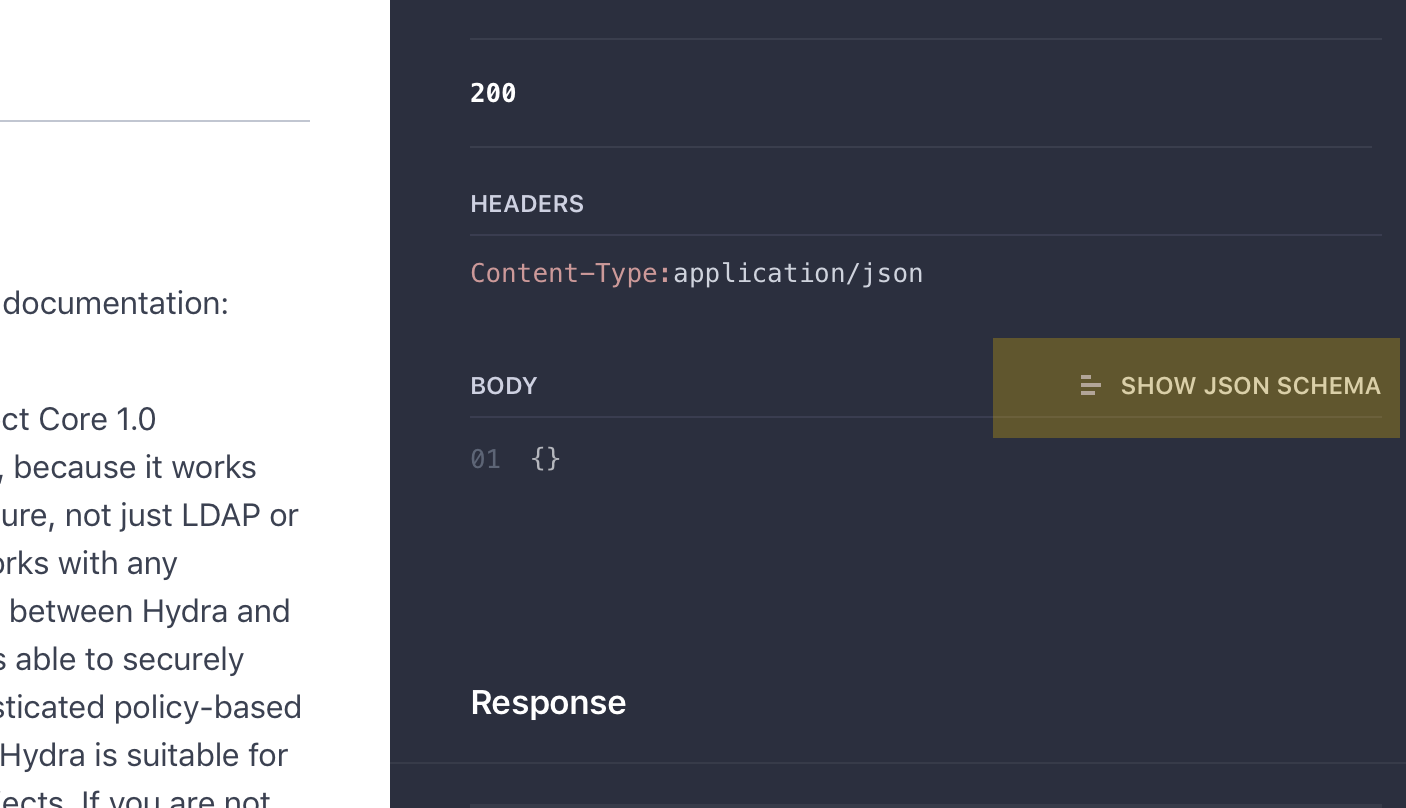 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The ConsentRequestAcceptance model module.
+ * @module model/ConsentRequestAcceptance
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new ConsentRequestAcceptance
.
+ * @alias module:model/ConsentRequestAcceptance
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a ConsentRequestAcceptance
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/ConsentRequestAcceptance} obj Optional instance to populate.
+ * @return {module:model/ConsentRequestAcceptance} The populated ConsentRequestAcceptance
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('accessTokenExtra')) {
+ obj['accessTokenExtra'] = ApiClient.convertToType(data['accessTokenExtra'], {'String': Object});
+ }
+ if (data.hasOwnProperty('grantScopes')) {
+ obj['grantScopes'] = ApiClient.convertToType(data['grantScopes'], ['String']);
+ }
+ if (data.hasOwnProperty('idTokenExtra')) {
+ obj['idTokenExtra'] = ApiClient.convertToType(data['idTokenExtra'], {'String': Object});
+ }
+ if (data.hasOwnProperty('subject')) {
+ obj['subject'] = ApiClient.convertToType(data['subject'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * AccessTokenExtra represents arbitrary data that will be added to the access token and that will be returned on introspection and warden requests.
+ * @member {Object.} accessTokenExtra
+ */
+ exports.prototype['accessTokenExtra'] = undefined;
+ /**
+ * A list of scopes that the user agreed to grant. It should be a subset of requestedScopes from the consent request.
+ * @member {Array.} grantScopes
+ */
+ exports.prototype['grantScopes'] = undefined;
+ /**
+ * IDTokenExtra represents arbitrary data that will be added to the ID token. The ID token will only be issued if the user agrees to it and if the client requested an ID token.
+ * @member {Object.} idTokenExtra
+ */
+ exports.prototype['idTokenExtra'] = undefined;
+ /**
+ * Subject represents a unique identifier of the user (or service, or legal entity, ...) that accepted the OAuth2 request.
+ * @member {String} subject
+ */
+ exports.prototype['subject'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/ConsentRequestManager.js b/sdk/js/swagger/src/model/ConsentRequestManager.js
new file mode 100644
index 00000000000..826a7f970b4
--- /dev/null
+++ b/sdk/js/swagger/src/model/ConsentRequestManager.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 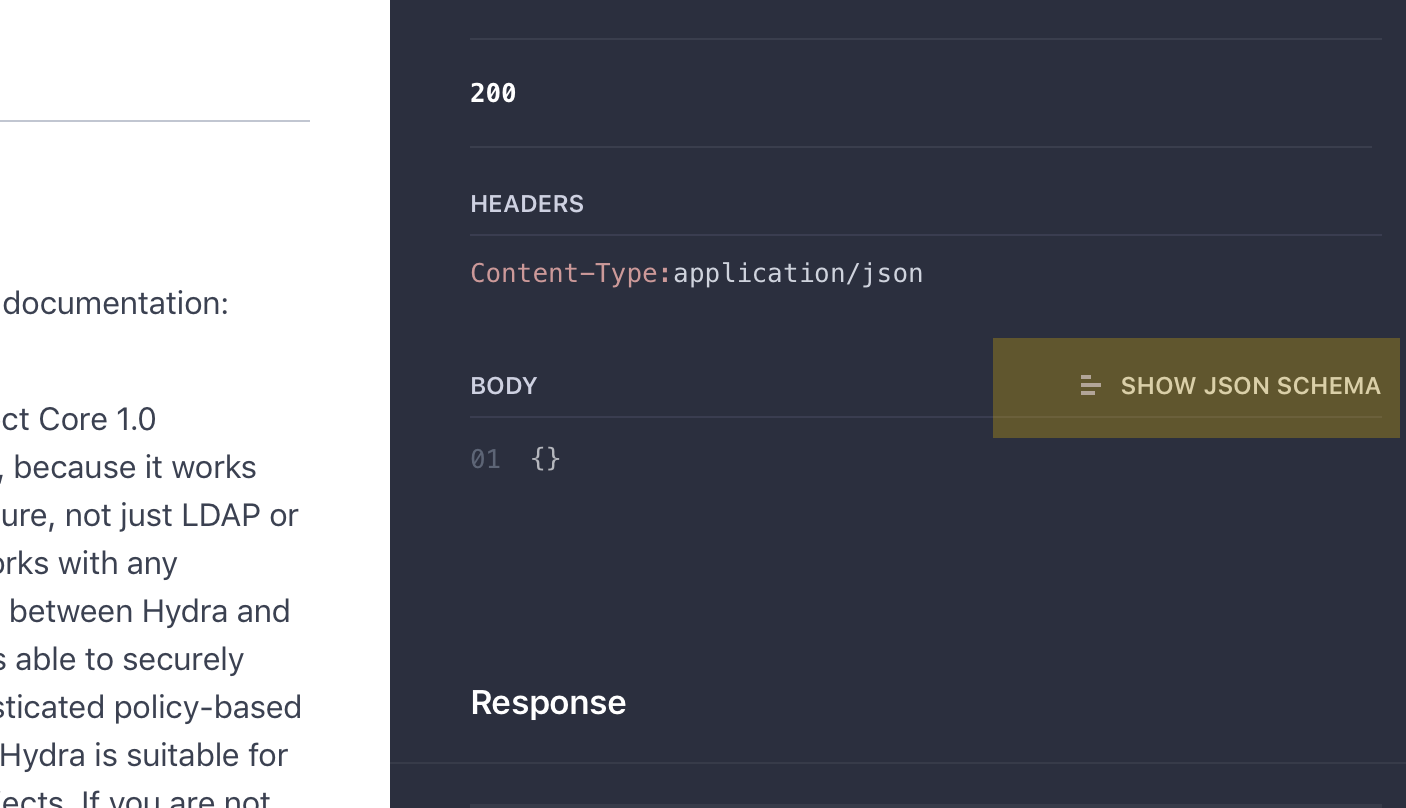 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.ConsentRequestManager = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The ConsentRequestManager model module.
+ * @module model/ConsentRequestManager
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new ConsentRequestManager
.
+ * @alias module:model/ConsentRequestManager
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+ };
+
+ /**
+ * Constructs a ConsentRequestManager
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/ConsentRequestManager} obj Optional instance to populate.
+ * @return {module:model/ConsentRequestManager} The populated ConsentRequestManager
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ }
+ return obj;
+ }
+
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/ConsentRequestRejection.js b/sdk/js/swagger/src/model/ConsentRequestRejection.js
new file mode 100644
index 00000000000..9ec23c09204
--- /dev/null
+++ b/sdk/js/swagger/src/model/ConsentRequestRejection.js
@@ -0,0 +1,83 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 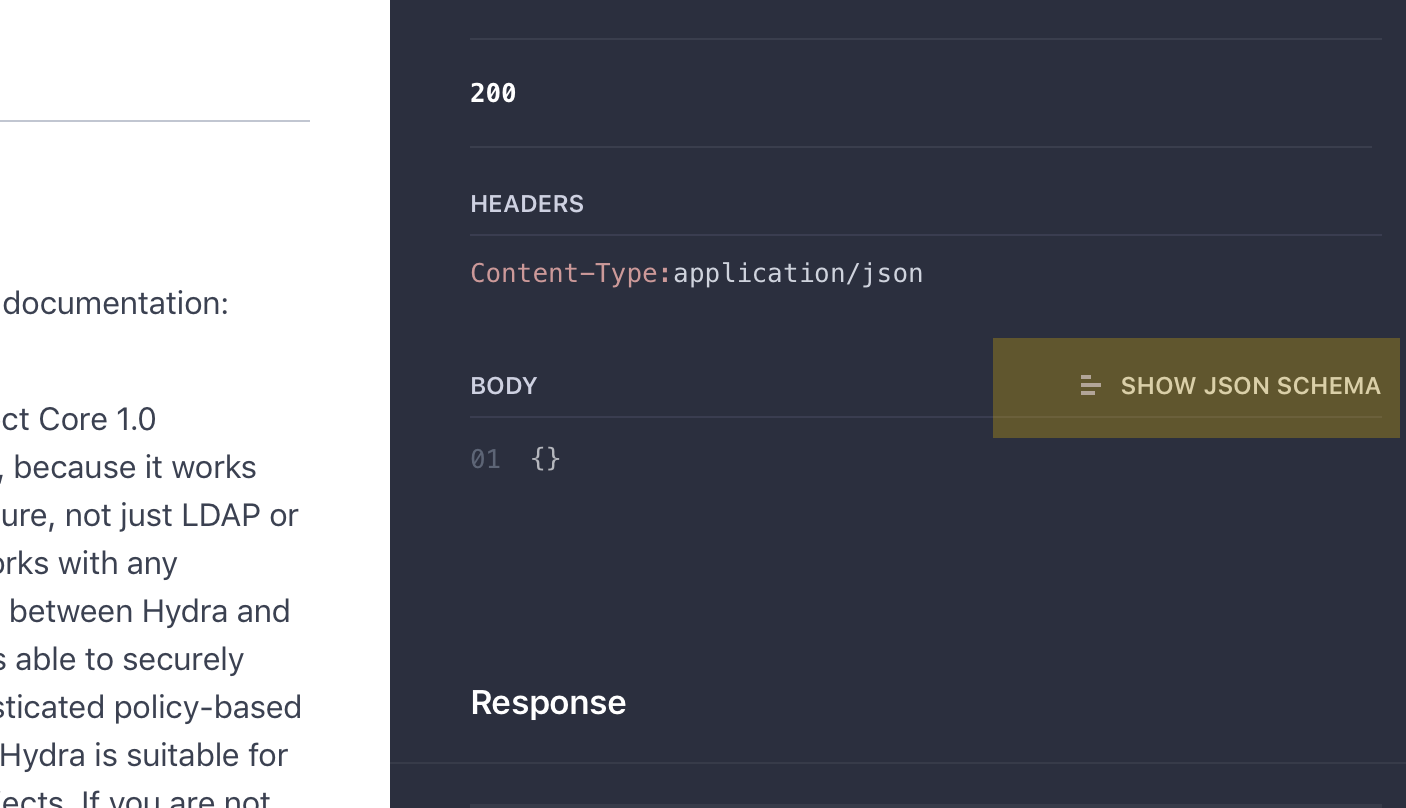 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.ConsentRequestRejection = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The ConsentRequestRejection model module.
+ * @module model/ConsentRequestRejection
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new ConsentRequestRejection
.
+ * @alias module:model/ConsentRequestRejection
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a ConsentRequestRejection
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/ConsentRequestRejection} obj Optional instance to populate.
+ * @return {module:model/ConsentRequestRejection} The populated ConsentRequestRejection
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('reason')) {
+ obj['reason'] = ApiClient.convertToType(data['reason'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Reason represents the reason why the user rejected the consent request.
+ * @member {String} reason
+ */
+ exports.prototype['reason'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/Context.js b/sdk/js/swagger/src/model/Context.js
new file mode 100644
index 00000000000..a166a35d1dc
--- /dev/null
+++ b/sdk/js/swagger/src/model/Context.js
@@ -0,0 +1,120 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 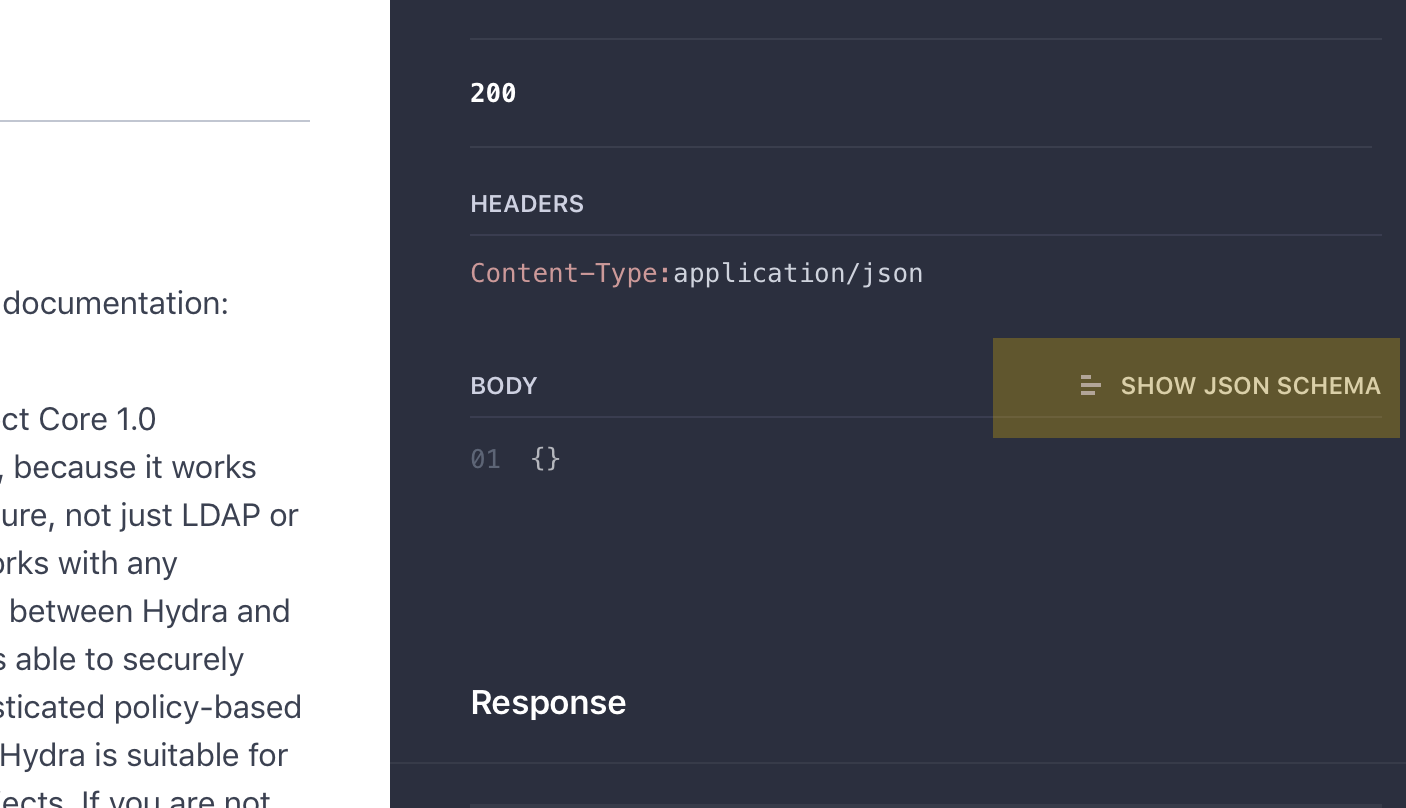 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.Context = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The Context model module.
+ * @module model/Context
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new Context
.
+ * Context contains an access token's session data
+ * @alias module:model/Context
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a Context
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/Context} obj Optional instance to populate.
+ * @return {module:model/Context} The populated Context
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('aud')) {
+ obj['aud'] = ApiClient.convertToType(data['aud'], 'String');
+ }
+ if (data.hasOwnProperty('ext')) {
+ obj['ext'] = ApiClient.convertToType(data['ext'], {'String': Object});
+ }
+ if (data.hasOwnProperty('iss')) {
+ obj['iss'] = ApiClient.convertToType(data['iss'], 'String');
+ }
+ if (data.hasOwnProperty('scopes')) {
+ obj['scopes'] = ApiClient.convertToType(data['scopes'], ['String']);
+ }
+ if (data.hasOwnProperty('sub')) {
+ obj['sub'] = ApiClient.convertToType(data['sub'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Audience is who the token was issued for. This is an OAuth2 app usually.
+ * @member {String} aud
+ */
+ exports.prototype['aud'] = undefined;
+ /**
+ * Extra represents arbitrary session data.
+ * @member {Object.} ext
+ */
+ exports.prototype['ext'] = undefined;
+ /**
+ * Issuer is the id of the issuer, typically an hydra instance.
+ * @member {String} iss
+ */
+ exports.prototype['iss'] = undefined;
+ /**
+ * GrantedScopes is a list of scopes that the subject authorized when asked for consent.
+ * @member {Array.} scopes
+ */
+ exports.prototype['scopes'] = undefined;
+ /**
+ * Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app. This is usually a uuid but you can choose a urn or some other id too.
+ * @member {String} sub
+ */
+ exports.prototype['sub'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/Firewall.js b/sdk/js/swagger/src/model/Firewall.js
new file mode 100644
index 00000000000..03fa9e5b81d
--- /dev/null
+++ b/sdk/js/swagger/src/model/Firewall.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 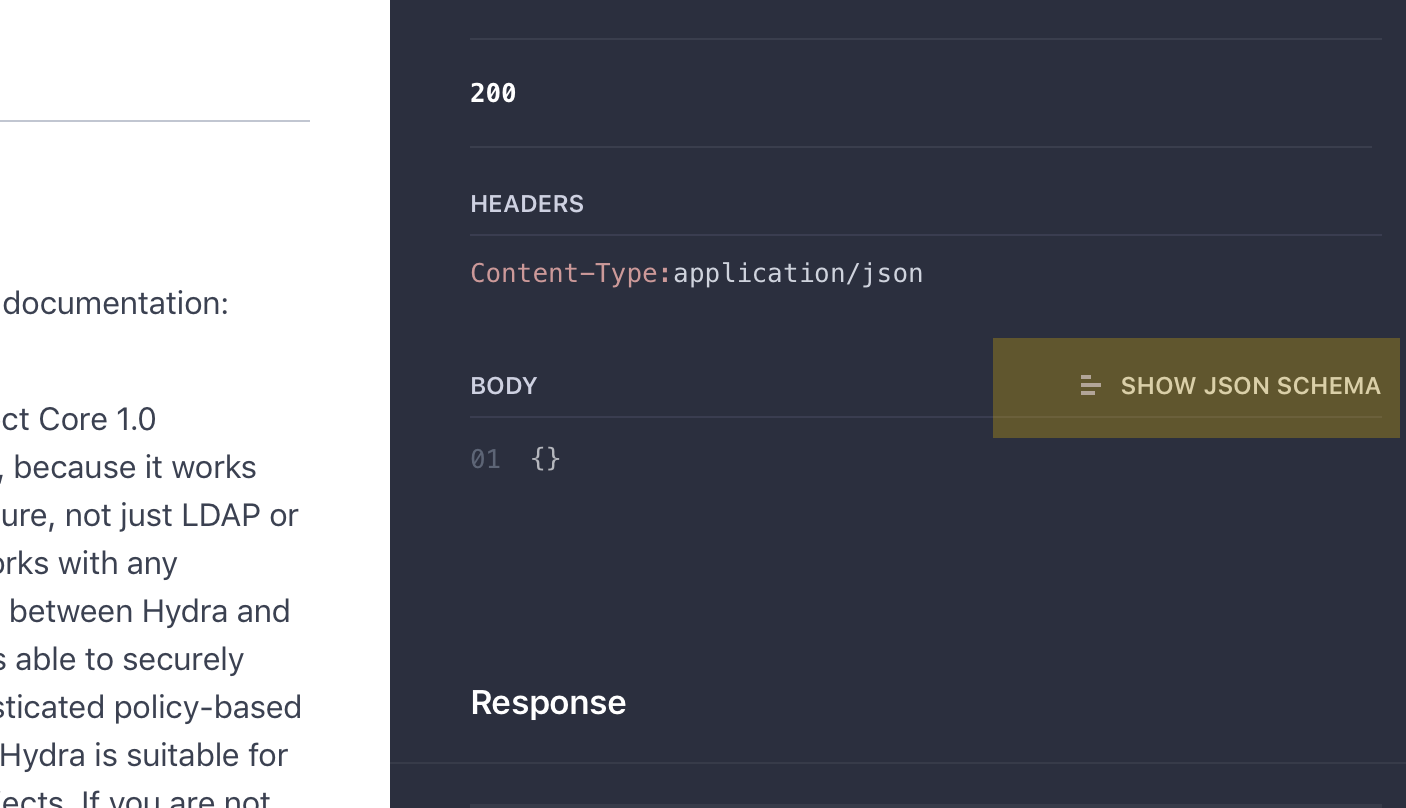 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.Firewall = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The Firewall model module.
+ * @module model/Firewall
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new Firewall
.
+ * @alias module:model/Firewall
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+ };
+
+ /**
+ * Constructs a Firewall
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/Firewall} obj Optional instance to populate.
+ * @return {module:model/Firewall} The populated Firewall
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ }
+ return obj;
+ }
+
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/Group.js b/sdk/js/swagger/src/model/Group.js
new file mode 100644
index 00000000000..beda1066336
--- /dev/null
+++ b/sdk/js/swagger/src/model/Group.js
@@ -0,0 +1,93 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 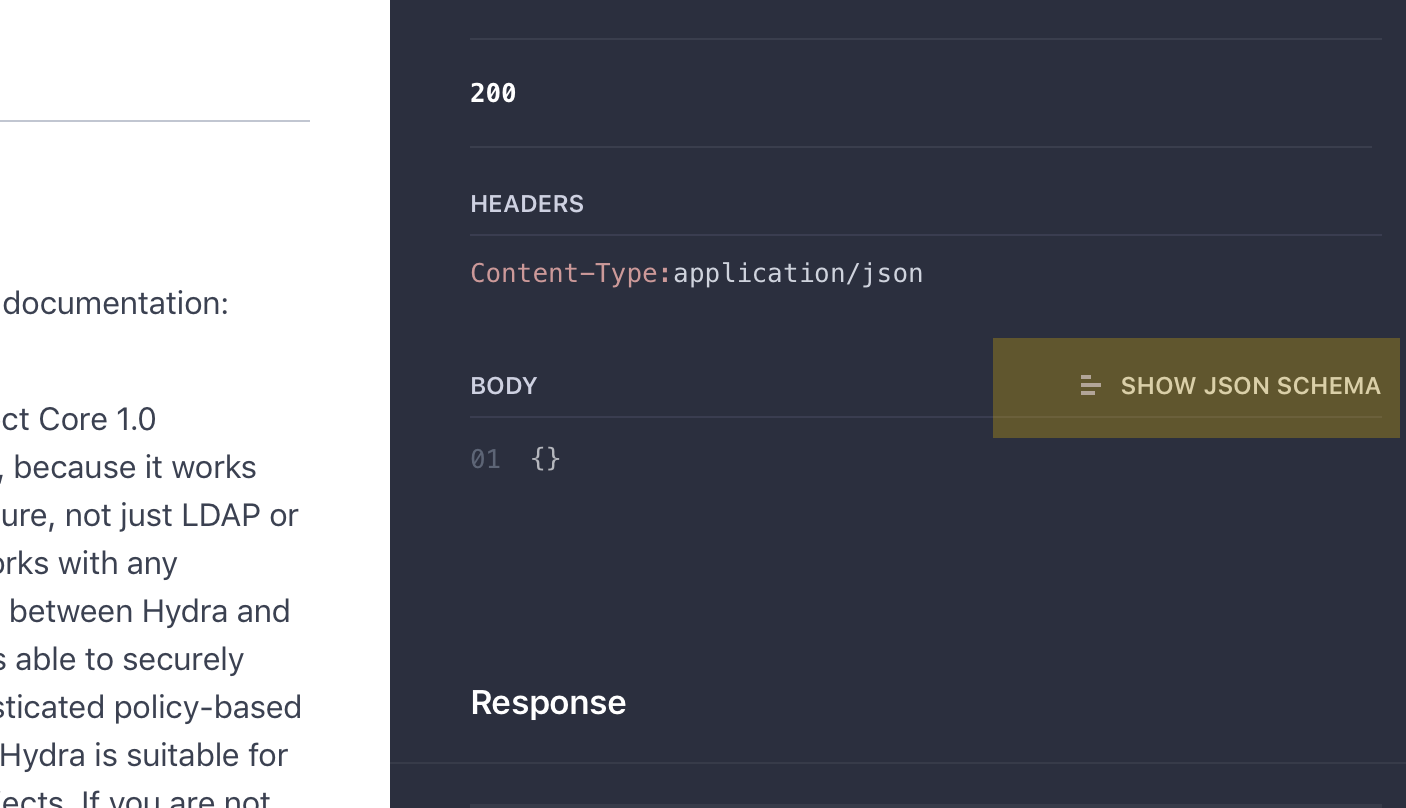 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.Group = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The Group model module.
+ * @module model/Group
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new Group
.
+ * Group represents a warden group
+ * @alias module:model/Group
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+ };
+
+ /**
+ * Constructs a Group
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/Group} obj Optional instance to populate.
+ * @return {module:model/Group} The populated Group
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ if (data.hasOwnProperty('members')) {
+ obj['members'] = ApiClient.convertToType(data['members'], ['String']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * ID is the groups id.
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+ /**
+ * Members is who belongs to the group.
+ * @member {Array.} members
+ */
+ exports.prototype['members'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/GroupMembers.js b/sdk/js/swagger/src/model/GroupMembers.js
new file mode 100644
index 00000000000..64c6657b1e2
--- /dev/null
+++ b/sdk/js/swagger/src/model/GroupMembers.js
@@ -0,0 +1,82 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 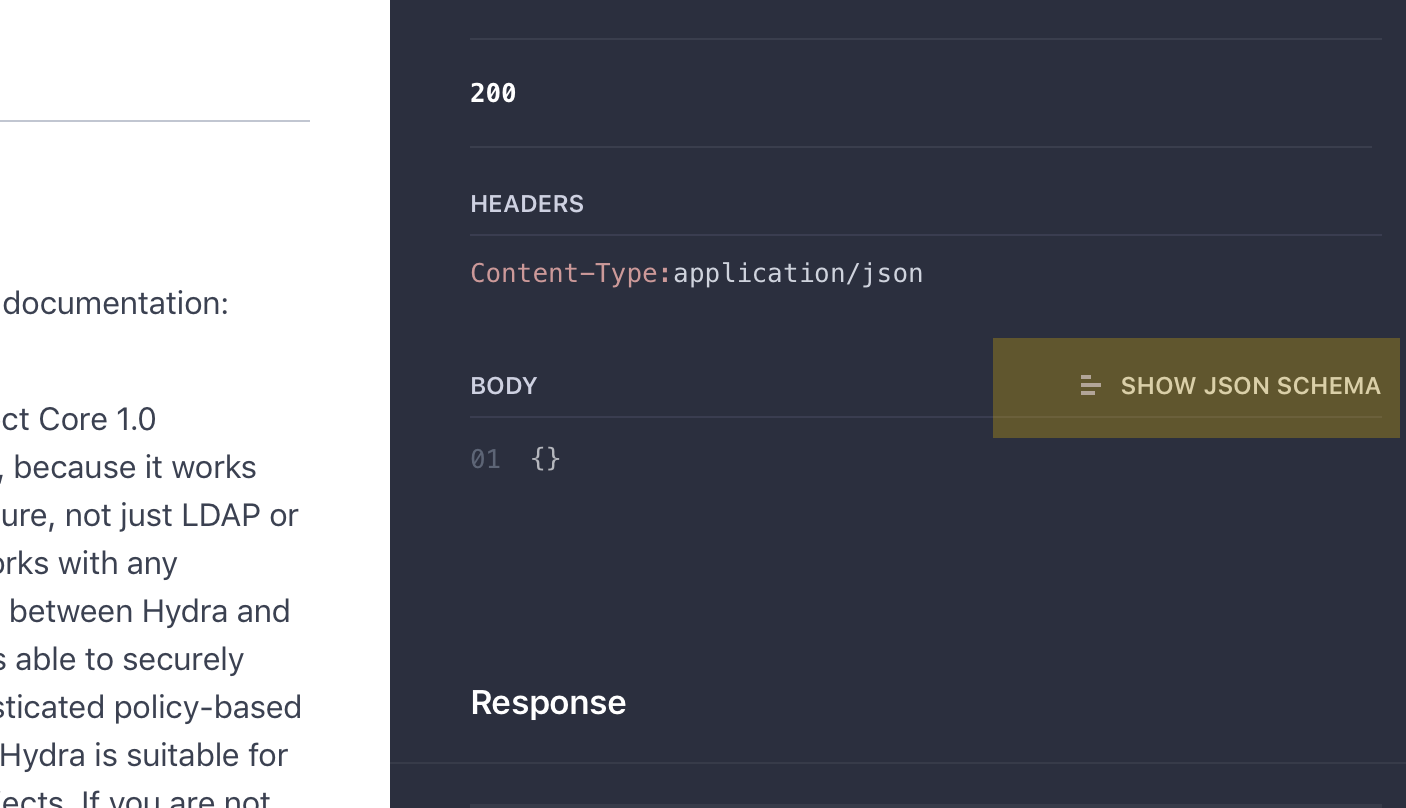 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.GroupMembers = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The GroupMembers model module.
+ * @module model/GroupMembers
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new GroupMembers
.
+ * @alias module:model/GroupMembers
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a GroupMembers
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/GroupMembers} obj Optional instance to populate.
+ * @return {module:model/GroupMembers} The populated GroupMembers
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('members')) {
+ obj['members'] = ApiClient.convertToType(data['members'], ['String']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {Array.} members
+ */
+ exports.prototype['members'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/Handler.js b/sdk/js/swagger/src/model/Handler.js
new file mode 100644
index 00000000000..d3605d49cfe
--- /dev/null
+++ b/sdk/js/swagger/src/model/Handler.js
@@ -0,0 +1,106 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 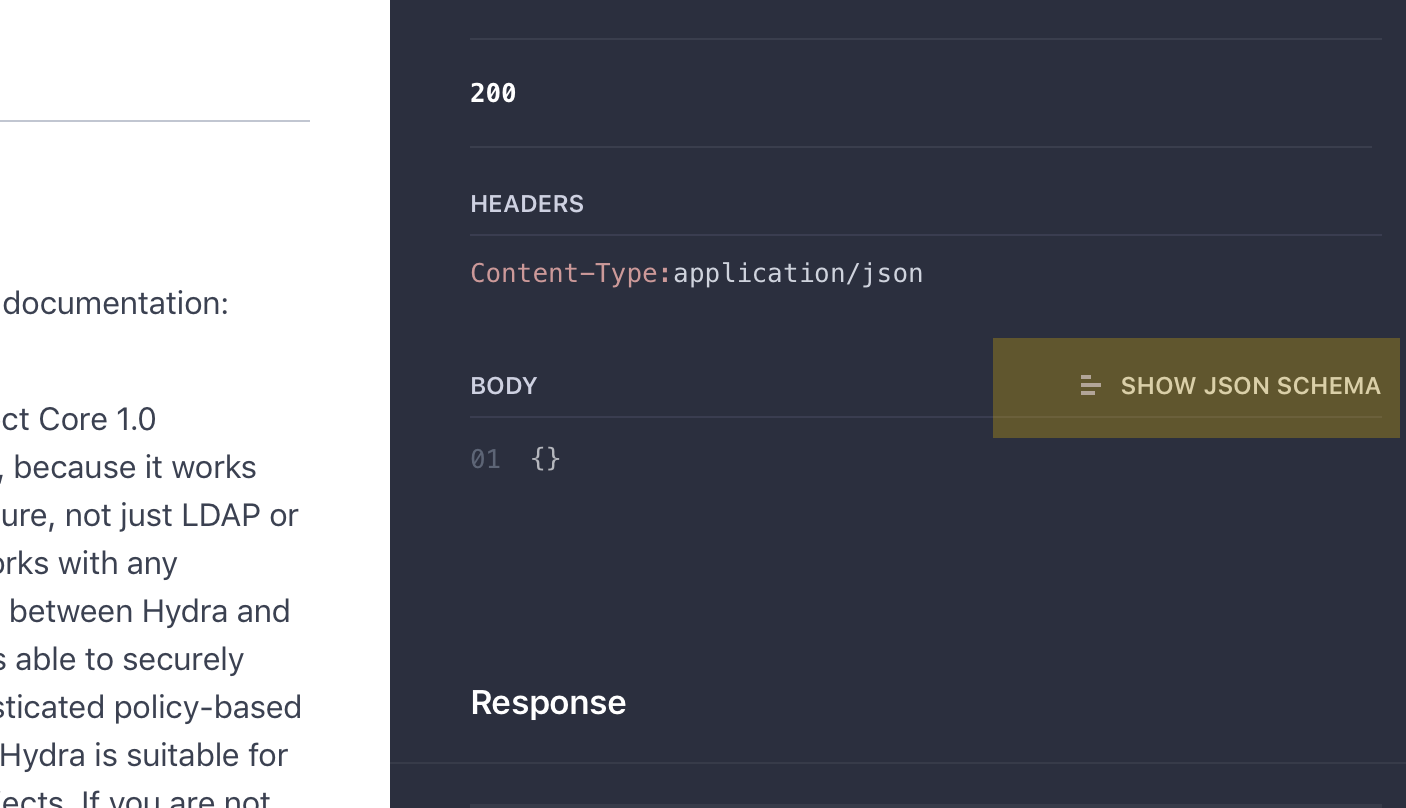 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/Firewall', 'model/KeyGenerator', 'model/Manager', 'model/Writer'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./Firewall'), require('./KeyGenerator'), require('./Manager'), require('./Writer'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.Handler = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.Firewall, root.HydraOAuth2OpenIdConnectServer.KeyGenerator, root.HydraOAuth2OpenIdConnectServer.Manager, root.HydraOAuth2OpenIdConnectServer.Writer);
+ }
+}(this, function(ApiClient, Firewall, KeyGenerator, Manager, Writer) {
+ 'use strict';
+
+
+
+
+ /**
+ * The Handler model module.
+ * @module model/Handler
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new Handler
.
+ * @alias module:model/Handler
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a Handler
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/Handler} obj Optional instance to populate.
+ * @return {module:model/Handler} The populated Handler
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Generators')) {
+ obj['Generators'] = ApiClient.convertToType(data['Generators'], {'String': KeyGenerator});
+ }
+ if (data.hasOwnProperty('H')) {
+ obj['H'] = Writer.constructFromObject(data['H']);
+ }
+ if (data.hasOwnProperty('Manager')) {
+ obj['Manager'] = Manager.constructFromObject(data['Manager']);
+ }
+ if (data.hasOwnProperty('W')) {
+ obj['W'] = Firewall.constructFromObject(data['W']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {Object.} Generators
+ */
+ exports.prototype['Generators'] = undefined;
+ /**
+ * @member {module:model/Writer} H
+ */
+ exports.prototype['H'] = undefined;
+ /**
+ * @member {module:model/Manager} Manager
+ */
+ exports.prototype['Manager'] = undefined;
+ /**
+ * @member {module:model/Firewall} W
+ */
+ exports.prototype['W'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/InlineResponse200.js b/sdk/js/swagger/src/model/InlineResponse200.js
new file mode 100644
index 00000000000..9caf85af25e
--- /dev/null
+++ b/sdk/js/swagger/src/model/InlineResponse200.js
@@ -0,0 +1,83 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 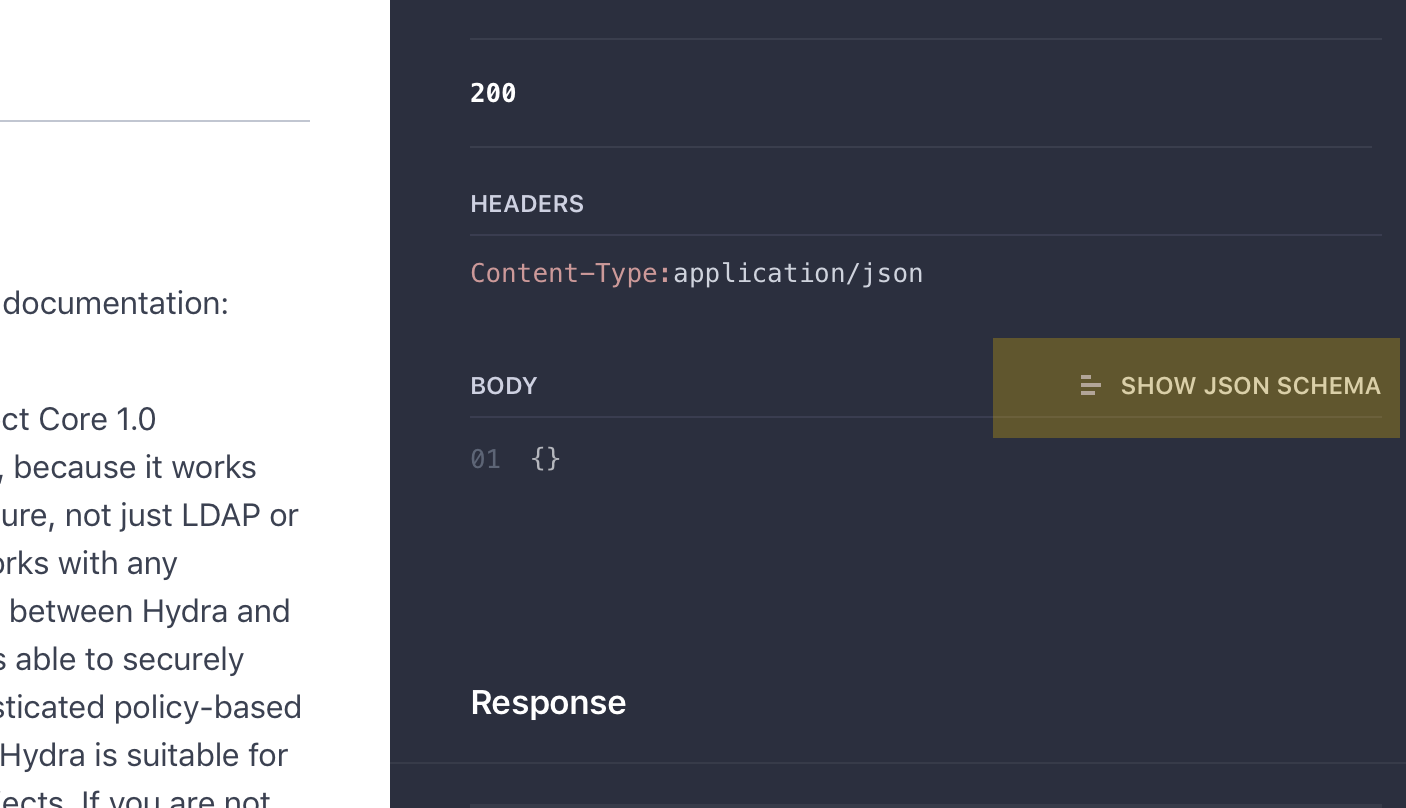 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.InlineResponse200 = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The InlineResponse200 model module.
+ * @module model/InlineResponse200
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new InlineResponse200
.
+ * @alias module:model/InlineResponse200
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a InlineResponse200
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/InlineResponse200} obj Optional instance to populate.
+ * @return {module:model/InlineResponse200} The populated InlineResponse200
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('status')) {
+ obj['status'] = ApiClient.convertToType(data['status'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Status always contains \"ok\"
+ * @member {String} status
+ */
+ exports.prototype['status'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/InlineResponse2001.js b/sdk/js/swagger/src/model/InlineResponse2001.js
new file mode 100644
index 00000000000..6f69f93deff
--- /dev/null
+++ b/sdk/js/swagger/src/model/InlineResponse2001.js
@@ -0,0 +1,128 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 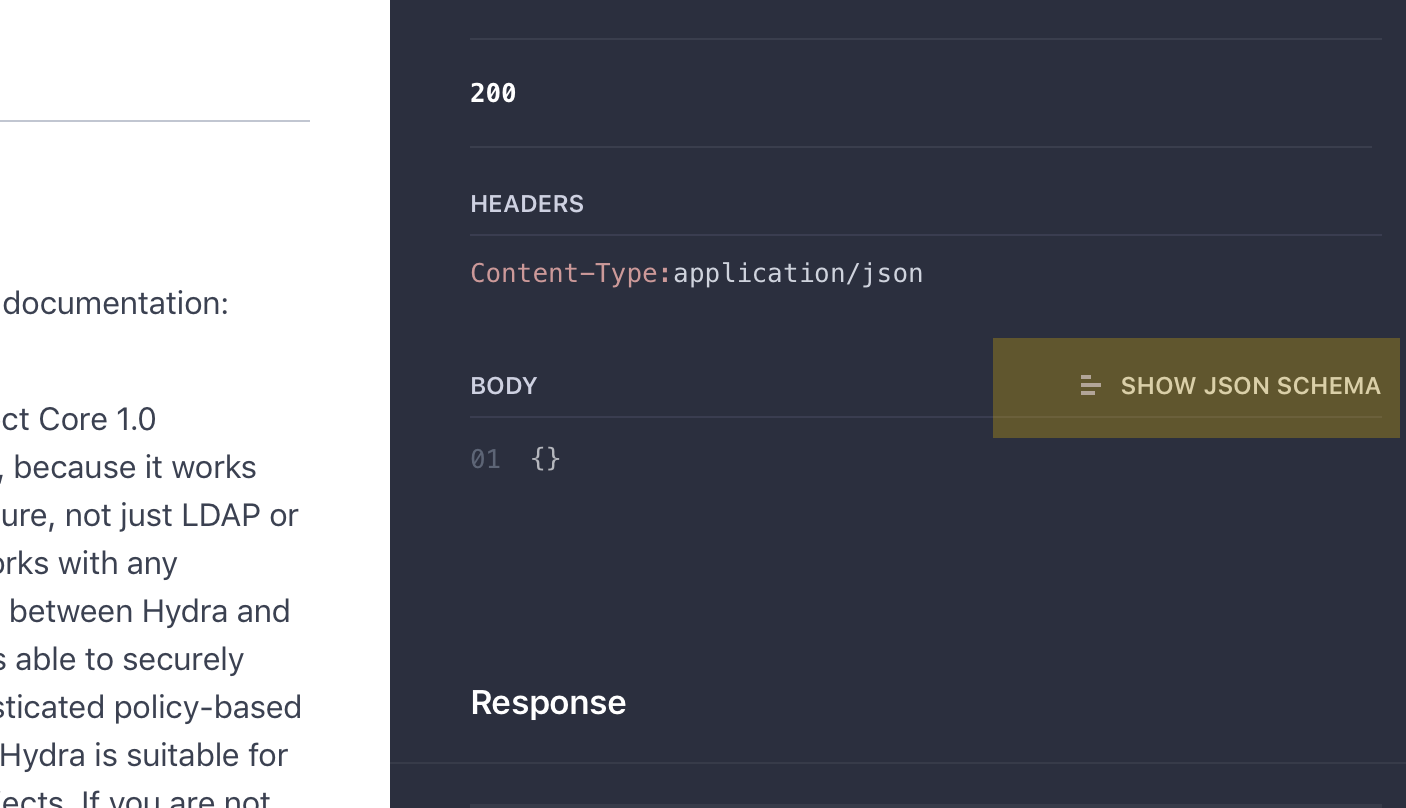 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.InlineResponse2001 = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The InlineResponse2001 model module.
+ * @module model/InlineResponse2001
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new InlineResponse2001
.
+ * @alias module:model/InlineResponse2001
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a InlineResponse2001
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/InlineResponse2001} obj Optional instance to populate.
+ * @return {module:model/InlineResponse2001} The populated InlineResponse2001
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('access_token')) {
+ obj['access_token'] = ApiClient.convertToType(data['access_token'], 'String');
+ }
+ if (data.hasOwnProperty('expires_in')) {
+ obj['expires_in'] = ApiClient.convertToType(data['expires_in'], 'Number');
+ }
+ if (data.hasOwnProperty('id_token')) {
+ obj['id_token'] = ApiClient.convertToType(data['id_token'], 'Number');
+ }
+ if (data.hasOwnProperty('refresh_token')) {
+ obj['refresh_token'] = ApiClient.convertToType(data['refresh_token'], 'String');
+ }
+ if (data.hasOwnProperty('scope')) {
+ obj['scope'] = ApiClient.convertToType(data['scope'], 'Number');
+ }
+ if (data.hasOwnProperty('token_type')) {
+ obj['token_type'] = ApiClient.convertToType(data['token_type'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The access token issued by the authorization server.
+ * @member {String} access_token
+ */
+ exports.prototype['access_token'] = undefined;
+ /**
+ * The lifetime in seconds of the access token. For example, the value \"3600\" denotes that the access token will expire in one hour from the time the response was generated.
+ * @member {Number} expires_in
+ */
+ exports.prototype['expires_in'] = undefined;
+ /**
+ * To retrieve a refresh token request the id_token scope.
+ * @member {Number} id_token
+ */
+ exports.prototype['id_token'] = undefined;
+ /**
+ * The refresh token, which can be used to obtain new access tokens. To retrieve it add the scope \"offline\" to your access token request.
+ * @member {String} refresh_token
+ */
+ exports.prototype['refresh_token'] = undefined;
+ /**
+ * The scope of the access token
+ * @member {Number} scope
+ */
+ exports.prototype['scope'] = undefined;
+ /**
+ * The type of the token issued
+ * @member {String} token_type
+ */
+ exports.prototype['token_type'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/InlineResponse401.js b/sdk/js/swagger/src/model/InlineResponse401.js
new file mode 100644
index 00000000000..c5d4da2809f
--- /dev/null
+++ b/sdk/js/swagger/src/model/InlineResponse401.js
@@ -0,0 +1,122 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 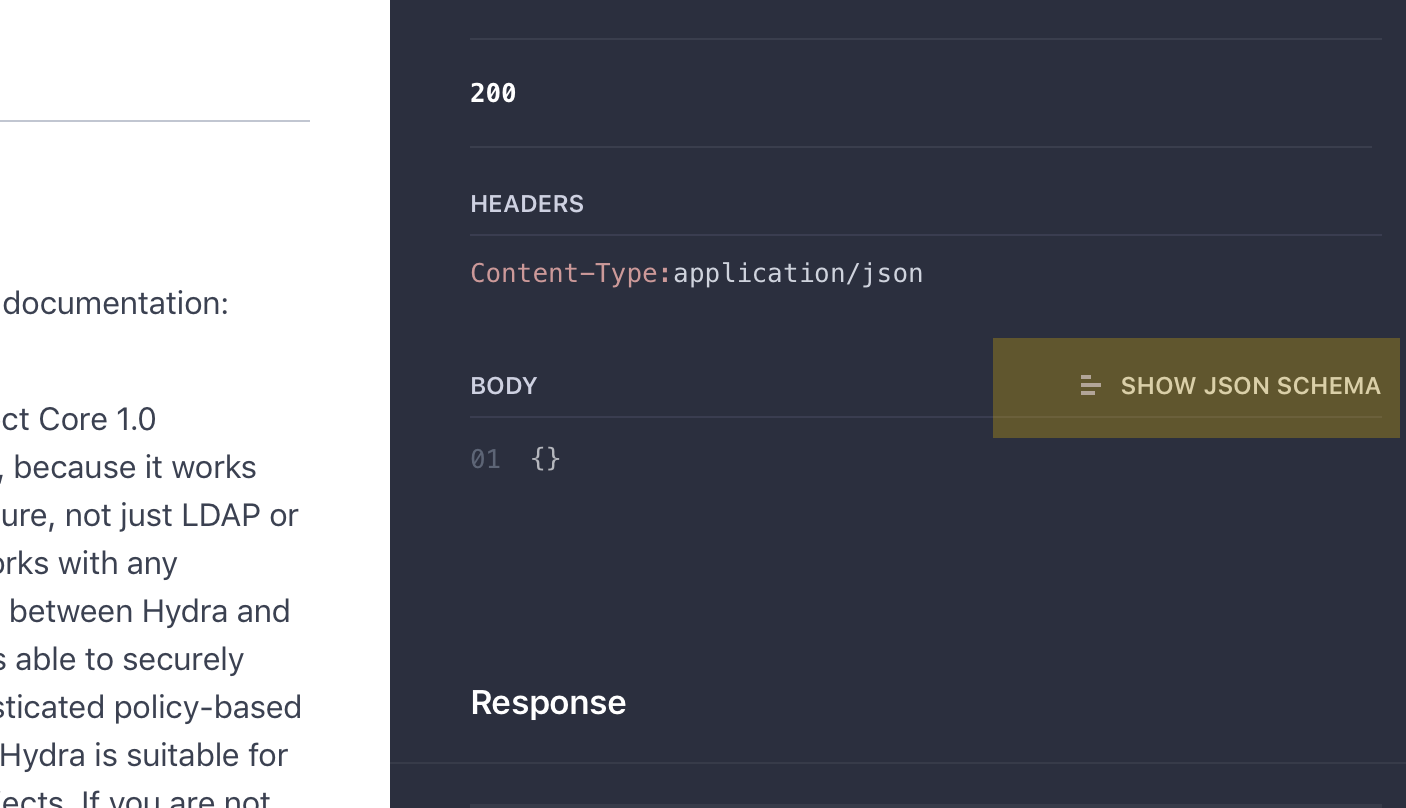 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.InlineResponse401 = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The InlineResponse401 model module.
+ * @module model/InlineResponse401
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new InlineResponse401
.
+ * @alias module:model/InlineResponse401
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a InlineResponse401
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/InlineResponse401} obj Optional instance to populate.
+ * @return {module:model/InlineResponse401} The populated InlineResponse401
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('code')) {
+ obj['code'] = ApiClient.convertToType(data['code'], 'Number');
+ }
+ if (data.hasOwnProperty('details')) {
+ obj['details'] = ApiClient.convertToType(data['details'], [{'String': Object}]);
+ }
+ if (data.hasOwnProperty('message')) {
+ obj['message'] = ApiClient.convertToType(data['message'], 'String');
+ }
+ if (data.hasOwnProperty('reason')) {
+ obj['reason'] = ApiClient.convertToType(data['reason'], 'String');
+ }
+ if (data.hasOwnProperty('request')) {
+ obj['request'] = ApiClient.convertToType(data['request'], 'String');
+ }
+ if (data.hasOwnProperty('status')) {
+ obj['status'] = ApiClient.convertToType(data['status'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {Number} code
+ */
+ exports.prototype['code'] = undefined;
+ /**
+ * @member {Array.>} details
+ */
+ exports.prototype['details'] = undefined;
+ /**
+ * @member {String} message
+ */
+ exports.prototype['message'] = undefined;
+ /**
+ * @member {String} reason
+ */
+ exports.prototype['reason'] = undefined;
+ /**
+ * @member {String} request
+ */
+ exports.prototype['request'] = undefined;
+ /**
+ * @member {String} status
+ */
+ exports.prototype['status'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/JoseWebKeySetRequest.js b/sdk/js/swagger/src/model/JoseWebKeySetRequest.js
new file mode 100644
index 00000000000..7ceb625efba
--- /dev/null
+++ b/sdk/js/swagger/src/model/JoseWebKeySetRequest.js
@@ -0,0 +1,82 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 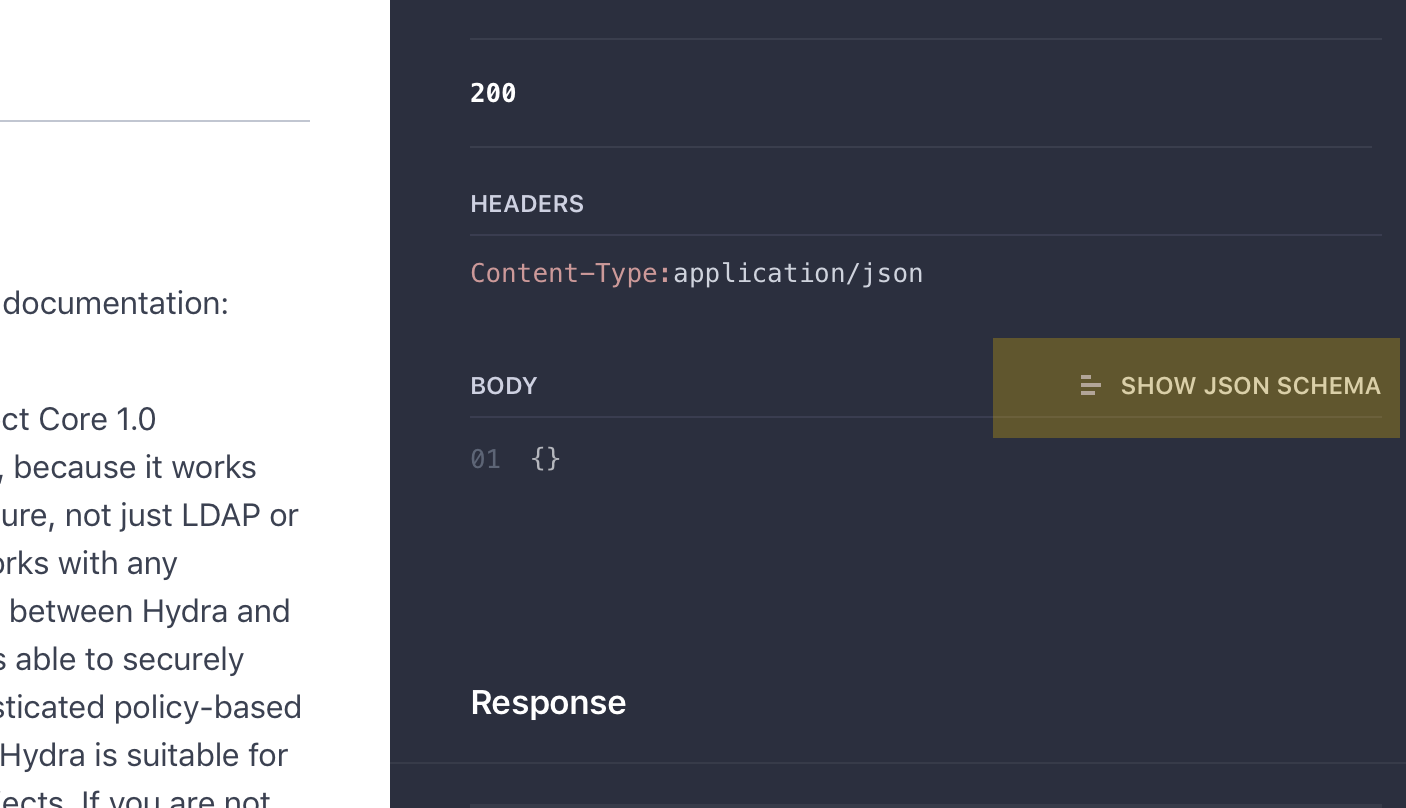 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/RawMessage'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./RawMessage'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.JoseWebKeySetRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.RawMessage);
+ }
+}(this, function(ApiClient, RawMessage) {
+ 'use strict';
+
+
+
+
+ /**
+ * The JoseWebKeySetRequest model module.
+ * @module model/JoseWebKeySetRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new JoseWebKeySetRequest
.
+ * @alias module:model/JoseWebKeySetRequest
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a JoseWebKeySetRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/JoseWebKeySetRequest} obj Optional instance to populate.
+ * @return {module:model/JoseWebKeySetRequest} The populated JoseWebKeySetRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('keys')) {
+ obj['keys'] = ApiClient.convertToType(data['keys'], [RawMessage]);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {Array.} keys
+ */
+ exports.prototype['keys'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/JsonWebKey.js b/sdk/js/swagger/src/model/JsonWebKey.js
new file mode 100644
index 00000000000..d34e05ea435
--- /dev/null
+++ b/sdk/js/swagger/src/model/JsonWebKey.js
@@ -0,0 +1,215 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 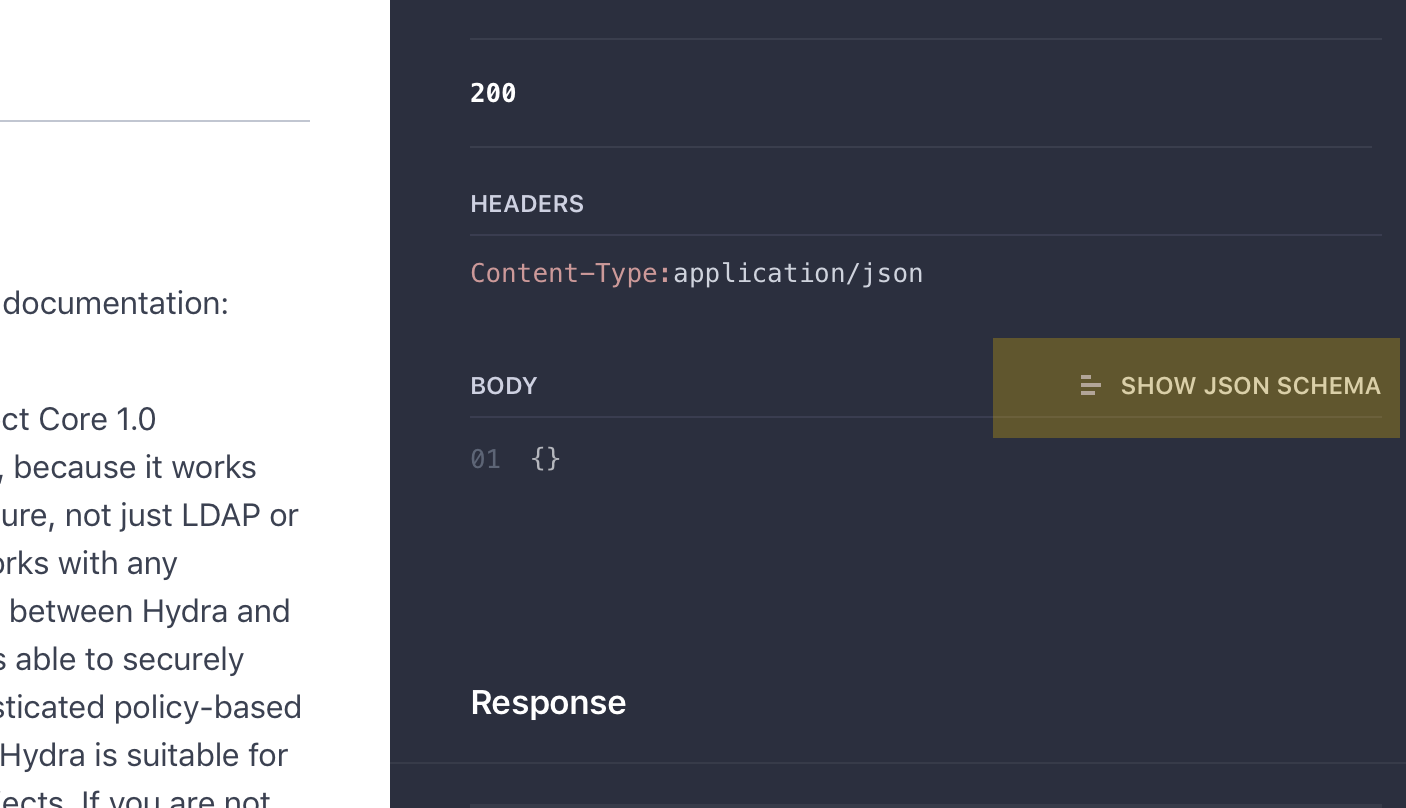 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.JsonWebKey = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The JsonWebKey model module.
+ * @module model/JsonWebKey
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new JsonWebKey
.
+ * @alias module:model/JsonWebKey
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a JsonWebKey
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/JsonWebKey} obj Optional instance to populate.
+ * @return {module:model/JsonWebKey} The populated JsonWebKey
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('alg')) {
+ obj['alg'] = ApiClient.convertToType(data['alg'], 'String');
+ }
+ if (data.hasOwnProperty('crv')) {
+ obj['crv'] = ApiClient.convertToType(data['crv'], 'String');
+ }
+ if (data.hasOwnProperty('d')) {
+ obj['d'] = ApiClient.convertToType(data['d'], 'String');
+ }
+ if (data.hasOwnProperty('dp')) {
+ obj['dp'] = ApiClient.convertToType(data['dp'], 'String');
+ }
+ if (data.hasOwnProperty('dq')) {
+ obj['dq'] = ApiClient.convertToType(data['dq'], 'String');
+ }
+ if (data.hasOwnProperty('e')) {
+ obj['e'] = ApiClient.convertToType(data['e'], 'String');
+ }
+ if (data.hasOwnProperty('k')) {
+ obj['k'] = ApiClient.convertToType(data['k'], 'String');
+ }
+ if (data.hasOwnProperty('kid')) {
+ obj['kid'] = ApiClient.convertToType(data['kid'], 'String');
+ }
+ if (data.hasOwnProperty('kty')) {
+ obj['kty'] = ApiClient.convertToType(data['kty'], 'String');
+ }
+ if (data.hasOwnProperty('n')) {
+ obj['n'] = ApiClient.convertToType(data['n'], 'String');
+ }
+ if (data.hasOwnProperty('p')) {
+ obj['p'] = ApiClient.convertToType(data['p'], 'String');
+ }
+ if (data.hasOwnProperty('q')) {
+ obj['q'] = ApiClient.convertToType(data['q'], 'String');
+ }
+ if (data.hasOwnProperty('qi')) {
+ obj['qi'] = ApiClient.convertToType(data['qi'], 'String');
+ }
+ if (data.hasOwnProperty('use')) {
+ obj['use'] = ApiClient.convertToType(data['use'], 'String');
+ }
+ if (data.hasOwnProperty('x')) {
+ obj['x'] = ApiClient.convertToType(data['x'], 'String');
+ }
+ if (data.hasOwnProperty('x5c')) {
+ obj['x5c'] = ApiClient.convertToType(data['x5c'], ['String']);
+ }
+ if (data.hasOwnProperty('y')) {
+ obj['y'] = ApiClient.convertToType(data['y'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The \"alg\" (algorithm) parameter identifies the algorithm intended for use with the key. The values used should either be registered in the IANA \"JSON Web Signature and Encryption Algorithms\" registry established by [JWA] or be a value that contains a Collision- Resistant Name.
+ * @member {String} alg
+ */
+ exports.prototype['alg'] = undefined;
+ /**
+ * @member {String} crv
+ */
+ exports.prototype['crv'] = undefined;
+ /**
+ * @member {String} d
+ */
+ exports.prototype['d'] = undefined;
+ /**
+ * @member {String} dp
+ */
+ exports.prototype['dp'] = undefined;
+ /**
+ * @member {String} dq
+ */
+ exports.prototype['dq'] = undefined;
+ /**
+ * @member {String} e
+ */
+ exports.prototype['e'] = undefined;
+ /**
+ * @member {String} k
+ */
+ exports.prototype['k'] = undefined;
+ /**
+ * The \"kid\" (key ID) parameter is used to match a specific key. This is used, for instance, to choose among a set of keys within a JWK Set during key rollover. The structure of the \"kid\" value is unspecified. When \"kid\" values are used within a JWK Set, different keys within the JWK Set SHOULD use distinct \"kid\" values. (One example in which different keys might use the same \"kid\" value is if they have different \"kty\" (key type) values but are considered to be equivalent alternatives by the application using them.) The \"kid\" value is a case-sensitive string.
+ * @member {String} kid
+ */
+ exports.prototype['kid'] = undefined;
+ /**
+ * The \"kty\" (key type) parameter identifies the cryptographic algorithm family used with the key, such as \"RSA\" or \"EC\". \"kty\" values should either be registered in the IANA \"JSON Web Key Types\" registry established by [JWA] or be a value that contains a Collision- Resistant Name. The \"kty\" value is a case-sensitive string.
+ * @member {String} kty
+ */
+ exports.prototype['kty'] = undefined;
+ /**
+ * @member {String} n
+ */
+ exports.prototype['n'] = undefined;
+ /**
+ * @member {String} p
+ */
+ exports.prototype['p'] = undefined;
+ /**
+ * @member {String} q
+ */
+ exports.prototype['q'] = undefined;
+ /**
+ * @member {String} qi
+ */
+ exports.prototype['qi'] = undefined;
+ /**
+ * The \"use\" (public key use) parameter identifies the intended use of the public key. The \"use\" parameter is employed to indicate whether a public key is used for encrypting data or verifying the signature on data. Values are commonly \"sig\" (signature) or \"enc\" (encryption).
+ * @member {String} use
+ */
+ exports.prototype['use'] = undefined;
+ /**
+ * @member {String} x
+ */
+ exports.prototype['x'] = undefined;
+ /**
+ * The \"x5c\" (X.509 certificate chain) parameter contains a chain of one or more PKIX certificates [RFC5280]. The certificate chain is represented as a JSON array of certificate value strings. Each string in the array is a base64-encoded (Section 4 of [RFC4648] -- not base64url-encoded) DER [ITU.X690.1994] PKIX certificate value. The PKIX certificate containing the key value MUST be the first certificate.
+ * @member {Array.} x5c
+ */
+ exports.prototype['x5c'] = undefined;
+ /**
+ * @member {String} y
+ */
+ exports.prototype['y'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/JsonWebKeySet.js b/sdk/js/swagger/src/model/JsonWebKeySet.js
new file mode 100644
index 00000000000..ac1ee583a62
--- /dev/null
+++ b/sdk/js/swagger/src/model/JsonWebKeySet.js
@@ -0,0 +1,83 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 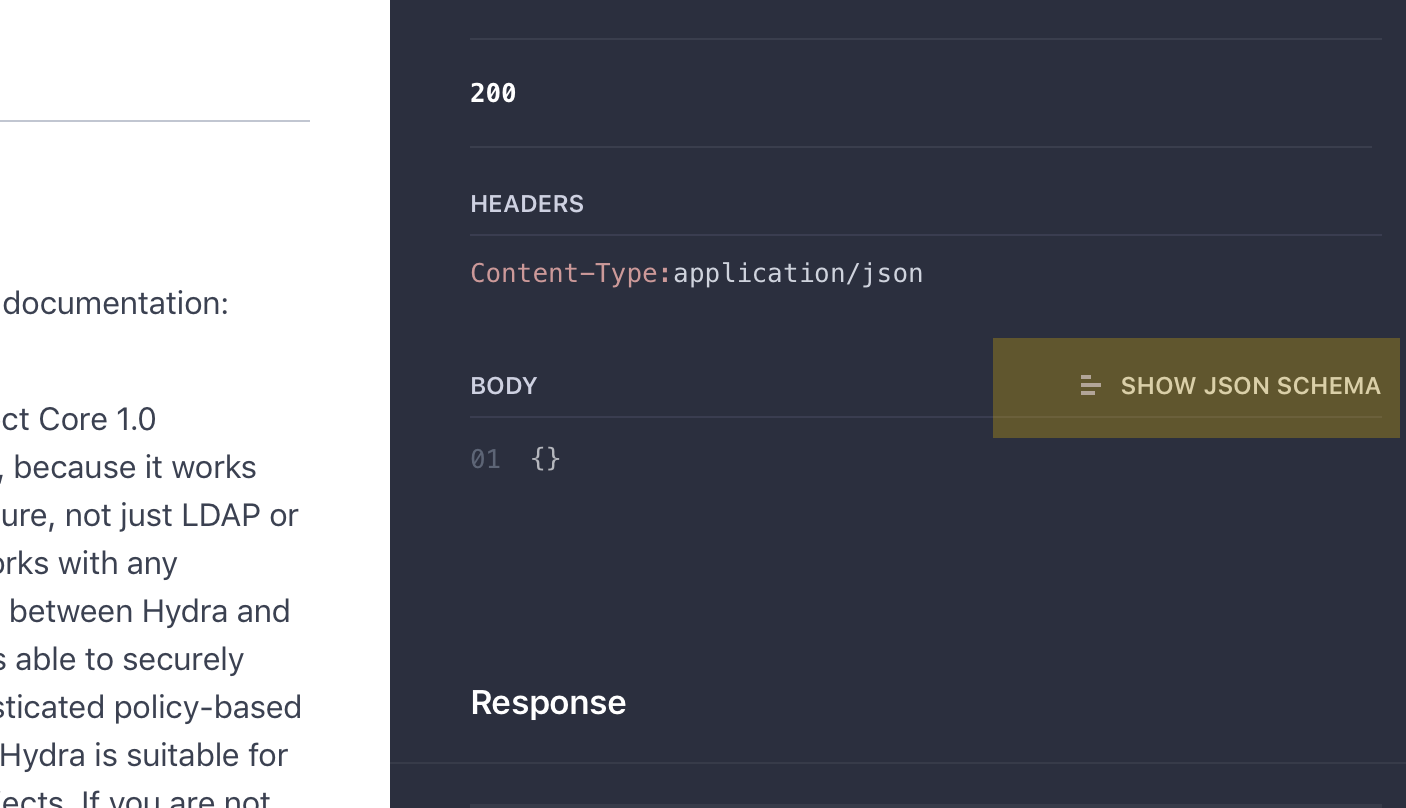 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/JsonWebKey'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./JsonWebKey'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.JsonWebKeySet = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.JsonWebKey);
+ }
+}(this, function(ApiClient, JsonWebKey) {
+ 'use strict';
+
+
+
+
+ /**
+ * The JsonWebKeySet model module.
+ * @module model/JsonWebKeySet
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new JsonWebKeySet
.
+ * @alias module:model/JsonWebKeySet
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a JsonWebKeySet
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/JsonWebKeySet} obj Optional instance to populate.
+ * @return {module:model/JsonWebKeySet} The populated JsonWebKeySet
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('keys')) {
+ obj['keys'] = ApiClient.convertToType(data['keys'], [JsonWebKey]);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The value of the \"keys\" parameter is an array of JWK values. By default, the order of the JWK values within the array does not imply an order of preference among them, although applications of JWK Sets can choose to assign a meaning to the order for their purposes, if desired.
+ * @member {Array.} keys
+ */
+ exports.prototype['keys'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/JsonWebKeySetGeneratorRequest.js b/sdk/js/swagger/src/model/JsonWebKeySetGeneratorRequest.js
new file mode 100644
index 00000000000..807b8be43f9
--- /dev/null
+++ b/sdk/js/swagger/src/model/JsonWebKeySetGeneratorRequest.js
@@ -0,0 +1,94 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 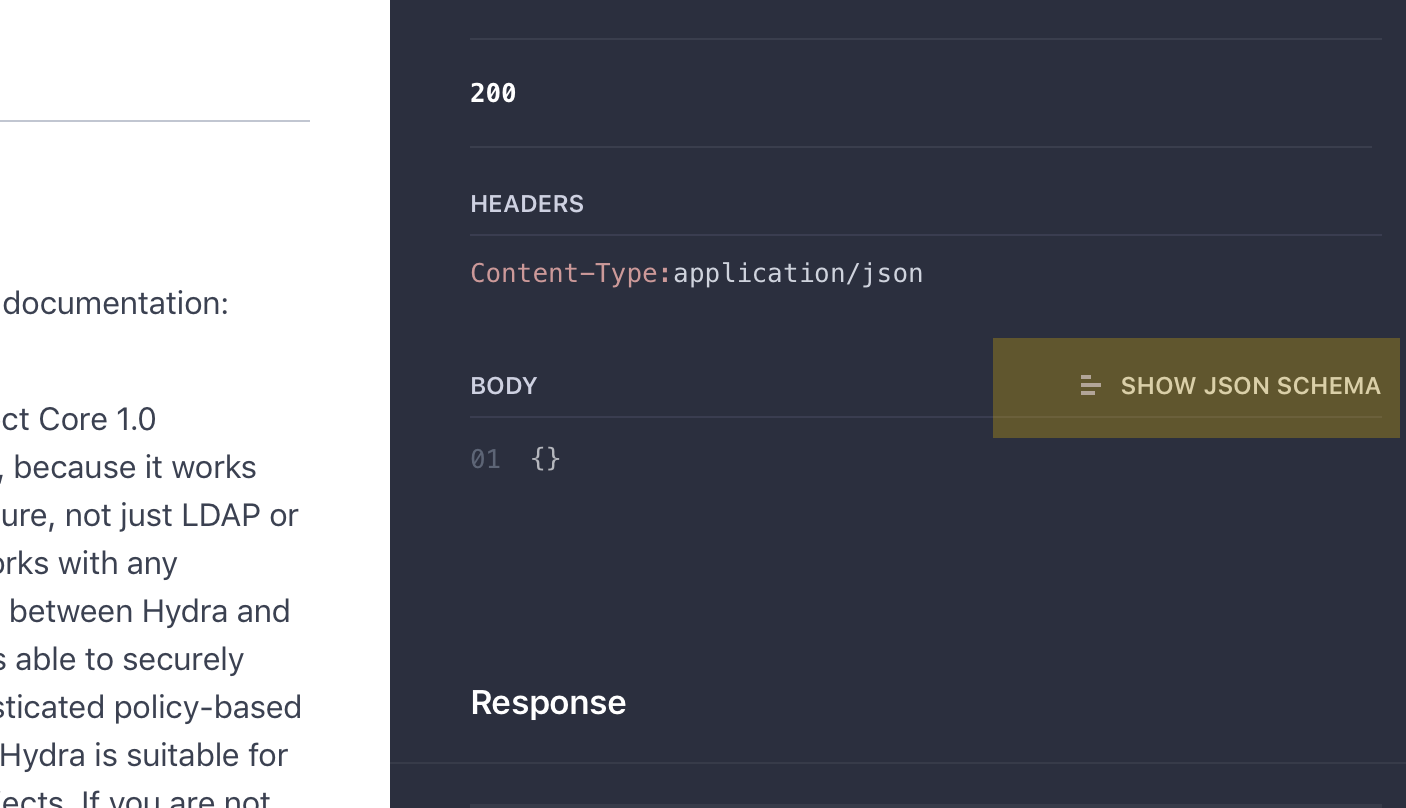 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The JsonWebKeySetGeneratorRequest model module.
+ * @module model/JsonWebKeySetGeneratorRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new JsonWebKeySetGeneratorRequest
.
+ * @alias module:model/JsonWebKeySetGeneratorRequest
+ * @class
+ * @param alg {String} The algorithm to be used for creating the key. Supports \"RS256\", \"ES521\" and \"HS256\"
+ * @param kid {String} The kid of the key to be created
+ */
+ var exports = function(alg, kid) {
+ var _this = this;
+
+ _this['alg'] = alg;
+ _this['kid'] = kid;
+ };
+
+ /**
+ * Constructs a JsonWebKeySetGeneratorRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/JsonWebKeySetGeneratorRequest} obj Optional instance to populate.
+ * @return {module:model/JsonWebKeySetGeneratorRequest} The populated JsonWebKeySetGeneratorRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('alg')) {
+ obj['alg'] = ApiClient.convertToType(data['alg'], 'String');
+ }
+ if (data.hasOwnProperty('kid')) {
+ obj['kid'] = ApiClient.convertToType(data['kid'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The algorithm to be used for creating the key. Supports \"RS256\", \"ES521\" and \"HS256\"
+ * @member {String} alg
+ */
+ exports.prototype['alg'] = undefined;
+ /**
+ * The kid of the key to be created
+ * @member {String} kid
+ */
+ exports.prototype['kid'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/KeyGenerator.js b/sdk/js/swagger/src/model/KeyGenerator.js
new file mode 100644
index 00000000000..310d0d038ea
--- /dev/null
+++ b/sdk/js/swagger/src/model/KeyGenerator.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 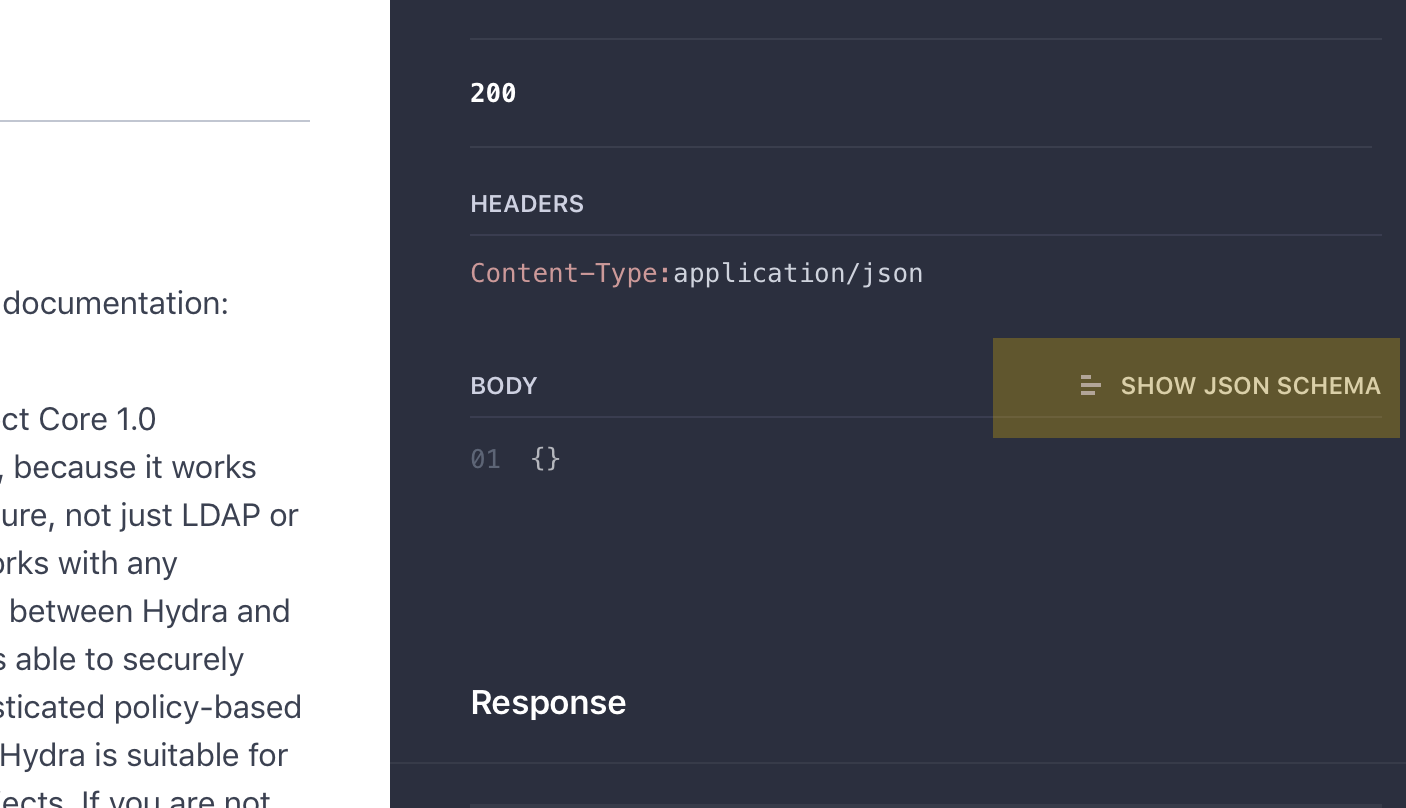 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.KeyGenerator = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The KeyGenerator model module.
+ * @module model/KeyGenerator
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new KeyGenerator
.
+ * @alias module:model/KeyGenerator
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+ };
+
+ /**
+ * Constructs a KeyGenerator
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/KeyGenerator} obj Optional instance to populate.
+ * @return {module:model/KeyGenerator} The populated KeyGenerator
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ }
+ return obj;
+ }
+
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/Manager.js b/sdk/js/swagger/src/model/Manager.js
new file mode 100644
index 00000000000..1bcb2d2c87e
--- /dev/null
+++ b/sdk/js/swagger/src/model/Manager.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 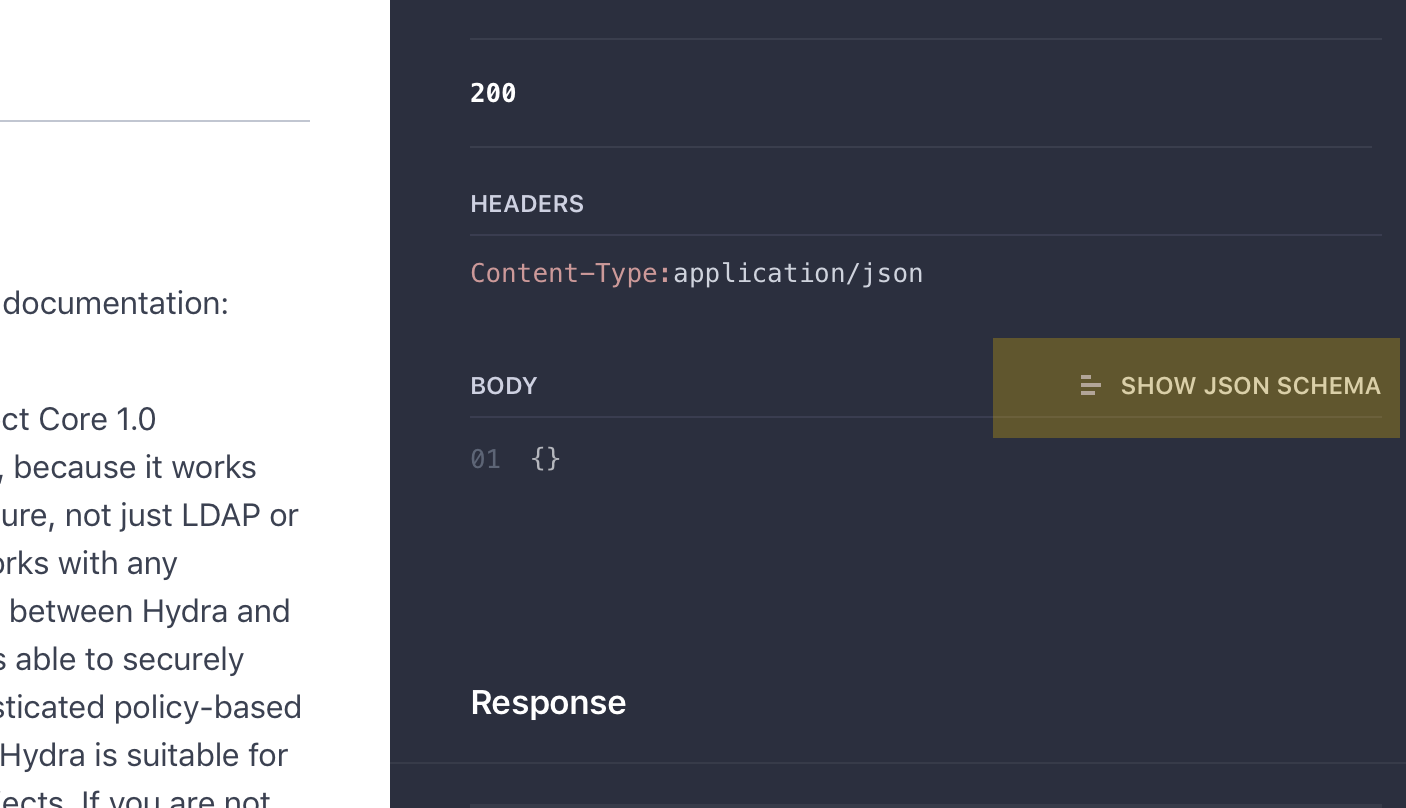 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.Manager = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The Manager model module.
+ * @module model/Manager
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new Manager
.
+ * @alias module:model/Manager
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+ };
+
+ /**
+ * Constructs a Manager
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/Manager} obj Optional instance to populate.
+ * @return {module:model/Manager} The populated Manager
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ }
+ return obj;
+ }
+
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/OAuth2Client.js b/sdk/js/swagger/src/model/OAuth2Client.js
new file mode 100644
index 00000000000..81f2234912f
--- /dev/null
+++ b/sdk/js/swagger/src/model/OAuth2Client.js
@@ -0,0 +1,200 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 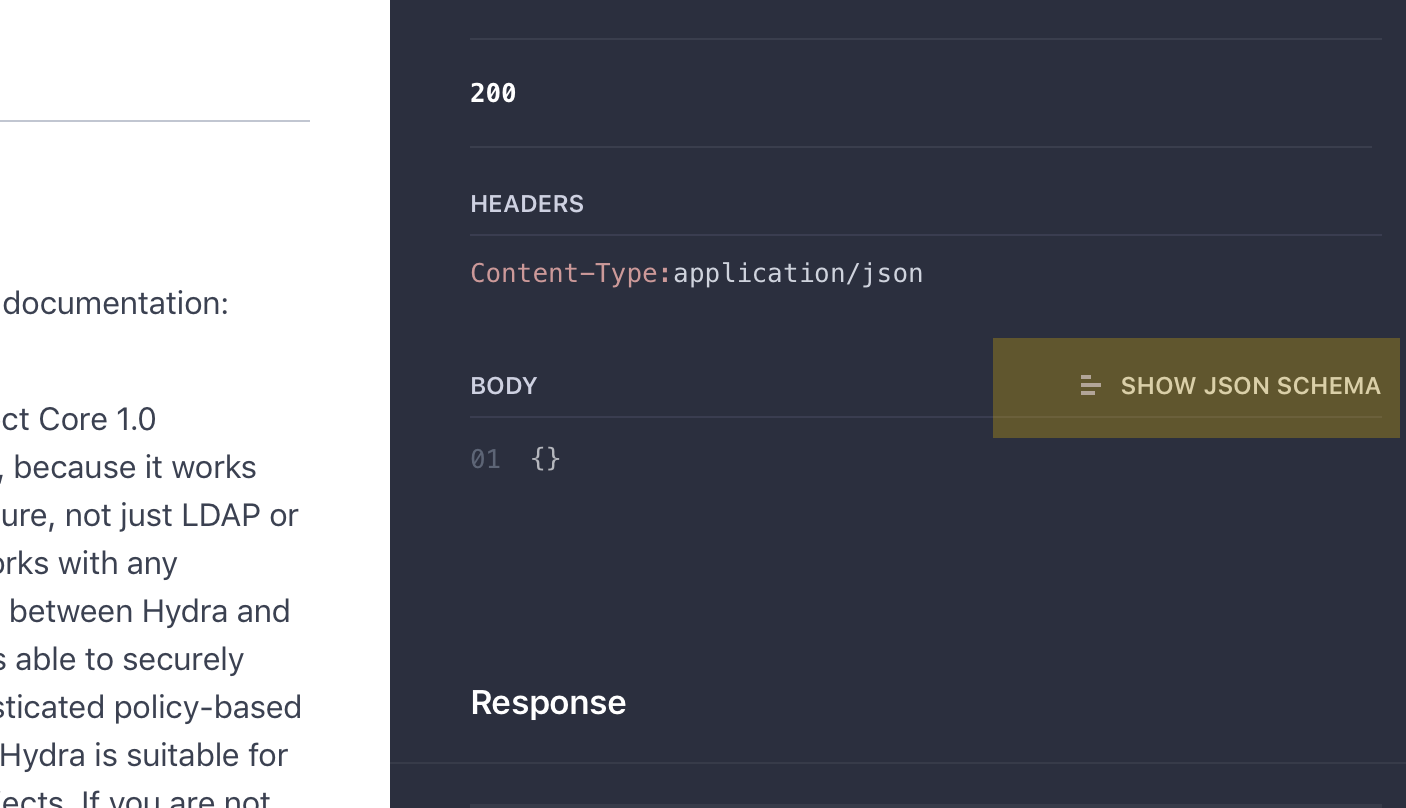 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.OAuth2Client = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The OAuth2Client model module.
+ * @module model/OAuth2Client
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new OAuth2Client
.
+ * @alias module:model/OAuth2Client
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a OAuth2Client
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/OAuth2Client} obj Optional instance to populate.
+ * @return {module:model/OAuth2Client} The populated OAuth2Client
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('client_name')) {
+ obj['client_name'] = ApiClient.convertToType(data['client_name'], 'String');
+ }
+ if (data.hasOwnProperty('client_secret')) {
+ obj['client_secret'] = ApiClient.convertToType(data['client_secret'], 'String');
+ }
+ if (data.hasOwnProperty('client_uri')) {
+ obj['client_uri'] = ApiClient.convertToType(data['client_uri'], 'String');
+ }
+ if (data.hasOwnProperty('contacts')) {
+ obj['contacts'] = ApiClient.convertToType(data['contacts'], ['String']);
+ }
+ if (data.hasOwnProperty('grant_types')) {
+ obj['grant_types'] = ApiClient.convertToType(data['grant_types'], ['String']);
+ }
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ if (data.hasOwnProperty('logo_uri')) {
+ obj['logo_uri'] = ApiClient.convertToType(data['logo_uri'], 'String');
+ }
+ if (data.hasOwnProperty('owner')) {
+ obj['owner'] = ApiClient.convertToType(data['owner'], 'String');
+ }
+ if (data.hasOwnProperty('policy_uri')) {
+ obj['policy_uri'] = ApiClient.convertToType(data['policy_uri'], 'String');
+ }
+ if (data.hasOwnProperty('public')) {
+ obj['public'] = ApiClient.convertToType(data['public'], 'Boolean');
+ }
+ if (data.hasOwnProperty('redirect_uris')) {
+ obj['redirect_uris'] = ApiClient.convertToType(data['redirect_uris'], ['String']);
+ }
+ if (data.hasOwnProperty('response_types')) {
+ obj['response_types'] = ApiClient.convertToType(data['response_types'], ['String']);
+ }
+ if (data.hasOwnProperty('scope')) {
+ obj['scope'] = ApiClient.convertToType(data['scope'], 'String');
+ }
+ if (data.hasOwnProperty('tos_uri')) {
+ obj['tos_uri'] = ApiClient.convertToType(data['tos_uri'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Name is the human-readable string name of the client to be presented to the end-user during authorization.
+ * @member {String} client_name
+ */
+ exports.prototype['client_name'] = undefined;
+ /**
+ * Secret is the client's secret. The secret will be included in the create request as cleartext, and then never again. The secret is stored using BCrypt so it is impossible to recover it. Tell your users that they need to write the secret down as it will not be made available again.
+ * @member {String} client_secret
+ */
+ exports.prototype['client_secret'] = undefined;
+ /**
+ * ClientURI is an URL string of a web page providing information about the client. If present, the server SHOULD display this URL to the end-user in a clickable fashion.
+ * @member {String} client_uri
+ */
+ exports.prototype['client_uri'] = undefined;
+ /**
+ * Contacts is a array of strings representing ways to contact people responsible for this client, typically email addresses.
+ * @member {Array.} contacts
+ */
+ exports.prototype['contacts'] = undefined;
+ /**
+ * GrantTypes is an array of grant types the client is allowed to use.
+ * @member {Array.} grant_types
+ */
+ exports.prototype['grant_types'] = undefined;
+ /**
+ * ID is the id for this client.
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+ /**
+ * LogoURI is an URL string that references a logo for the client.
+ * @member {String} logo_uri
+ */
+ exports.prototype['logo_uri'] = undefined;
+ /**
+ * Owner is a string identifying the owner of the OAuth 2.0 Client.
+ * @member {String} owner
+ */
+ exports.prototype['owner'] = undefined;
+ /**
+ * PolicyURI is a URL string that points to a human-readable privacy policy document that describes how the deployment organization collects, uses, retains, and discloses personal data.
+ * @member {String} policy_uri
+ */
+ exports.prototype['policy_uri'] = undefined;
+ /**
+ * Public is a boolean that identifies this client as public, meaning that it does not have a secret. It will disable the client_credentials grant type for this client if set.
+ * @member {Boolean} public
+ */
+ exports.prototype['public'] = undefined;
+ /**
+ * RedirectURIs is an array of allowed redirect urls for the client, for example: http://mydomain/oauth/callback .
+ * @member {Array.} redirect_uris
+ */
+ exports.prototype['redirect_uris'] = undefined;
+ /**
+ * ResponseTypes is an array of the OAuth 2.0 response type strings that the client can use at the authorization endpoint.
+ * @member {Array.} response_types
+ */
+ exports.prototype['response_types'] = undefined;
+ /**
+ * Scope is a string containing a space-separated list of scope values (as described in Section 3.3 of OAuth 2.0 [RFC6749]) that the client can use when requesting access tokens.
+ * @member {String} scope
+ */
+ exports.prototype['scope'] = undefined;
+ /**
+ * TermsOfServiceURI is a URL string that points to a human-readable terms of service document for the client that describes a contractual relationship between the end-user and the client that the end-user accepts when authorizing the client.
+ * @member {String} tos_uri
+ */
+ exports.prototype['tos_uri'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/OAuth2TokenIntrospection.js b/sdk/js/swagger/src/model/OAuth2TokenIntrospection.js
new file mode 100644
index 00000000000..5b42144ed63
--- /dev/null
+++ b/sdk/js/swagger/src/model/OAuth2TokenIntrospection.js
@@ -0,0 +1,173 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 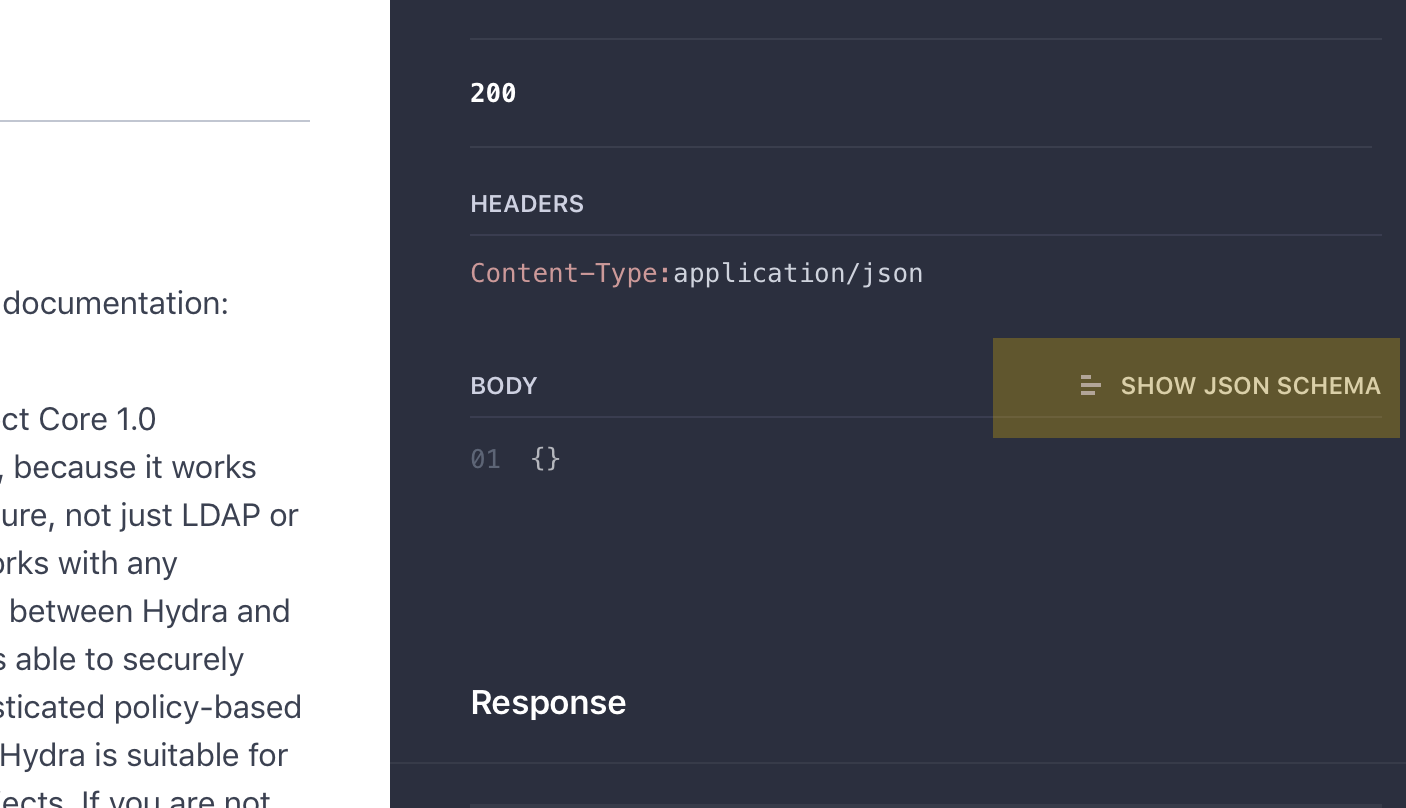 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The OAuth2TokenIntrospection model module.
+ * @module model/OAuth2TokenIntrospection
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new OAuth2TokenIntrospection
.
+ * @alias module:model/OAuth2TokenIntrospection
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a OAuth2TokenIntrospection
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/OAuth2TokenIntrospection} obj Optional instance to populate.
+ * @return {module:model/OAuth2TokenIntrospection} The populated OAuth2TokenIntrospection
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('active')) {
+ obj['active'] = ApiClient.convertToType(data['active'], 'Boolean');
+ }
+ if (data.hasOwnProperty('aud')) {
+ obj['aud'] = ApiClient.convertToType(data['aud'], 'String');
+ }
+ if (data.hasOwnProperty('client_id')) {
+ obj['client_id'] = ApiClient.convertToType(data['client_id'], 'String');
+ }
+ if (data.hasOwnProperty('exp')) {
+ obj['exp'] = ApiClient.convertToType(data['exp'], 'Number');
+ }
+ if (data.hasOwnProperty('ext')) {
+ obj['ext'] = ApiClient.convertToType(data['ext'], {'String': Object});
+ }
+ if (data.hasOwnProperty('iat')) {
+ obj['iat'] = ApiClient.convertToType(data['iat'], 'Number');
+ }
+ if (data.hasOwnProperty('iss')) {
+ obj['iss'] = ApiClient.convertToType(data['iss'], 'String');
+ }
+ if (data.hasOwnProperty('nbf')) {
+ obj['nbf'] = ApiClient.convertToType(data['nbf'], 'Number');
+ }
+ if (data.hasOwnProperty('scope')) {
+ obj['scope'] = ApiClient.convertToType(data['scope'], 'String');
+ }
+ if (data.hasOwnProperty('sub')) {
+ obj['sub'] = ApiClient.convertToType(data['sub'], 'String');
+ }
+ if (data.hasOwnProperty('username')) {
+ obj['username'] = ApiClient.convertToType(data['username'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Active is a boolean indicator of whether or not the presented token is currently active. The specifics of a token's \"active\" state will vary depending on the implementation of the authorization server and the information it keeps about its tokens, but a \"true\" value return for the \"active\" property will generally indicate that a given token has been issued by this authorization server, has not been revoked by the resource owner, and is within its given time window of validity (e.g., after its issuance time and before its expiration time).
+ * @member {Boolean} active
+ */
+ exports.prototype['active'] = undefined;
+ /**
+ * Audience is a service-specific string identifier or list of string identifiers representing the intended audience for this token.
+ * @member {String} aud
+ */
+ exports.prototype['aud'] = undefined;
+ /**
+ * ClientID is aclient identifier for the OAuth 2.0 client that requested this token.
+ * @member {String} client_id
+ */
+ exports.prototype['client_id'] = undefined;
+ /**
+ * Expires at is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token will expire.
+ * @member {Number} exp
+ */
+ exports.prototype['exp'] = undefined;
+ /**
+ * Extra is arbitrary data set by the session.
+ * @member {Object.} ext
+ */
+ exports.prototype['ext'] = undefined;
+ /**
+ * Issued at is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token was originally issued.
+ * @member {Number} iat
+ */
+ exports.prototype['iat'] = undefined;
+ /**
+ * Issuer is a string representing the issuer of this token
+ * @member {String} iss
+ */
+ exports.prototype['iss'] = undefined;
+ /**
+ * NotBefore is an integer timestamp, measured in the number of seconds since January 1 1970 UTC, indicating when this token is not to be used before.
+ * @member {Number} nbf
+ */
+ exports.prototype['nbf'] = undefined;
+ /**
+ * Scope is a JSON string containing a space-separated list of scopes associated with this token.
+ * @member {String} scope
+ */
+ exports.prototype['scope'] = undefined;
+ /**
+ * Subject of the token, as defined in JWT [RFC7519]. Usually a machine-readable identifier of the resource owner who authorized this token.
+ * @member {String} sub
+ */
+ exports.prototype['sub'] = undefined;
+ /**
+ * Username is a human-readable identifier for the resource owner who authorized this token.
+ * @member {String} username
+ */
+ exports.prototype['username'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/OAuth2consentRequest.js b/sdk/js/swagger/src/model/OAuth2consentRequest.js
new file mode 100644
index 00000000000..1a0f31bad34
--- /dev/null
+++ b/sdk/js/swagger/src/model/OAuth2consentRequest.js
@@ -0,0 +1,110 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 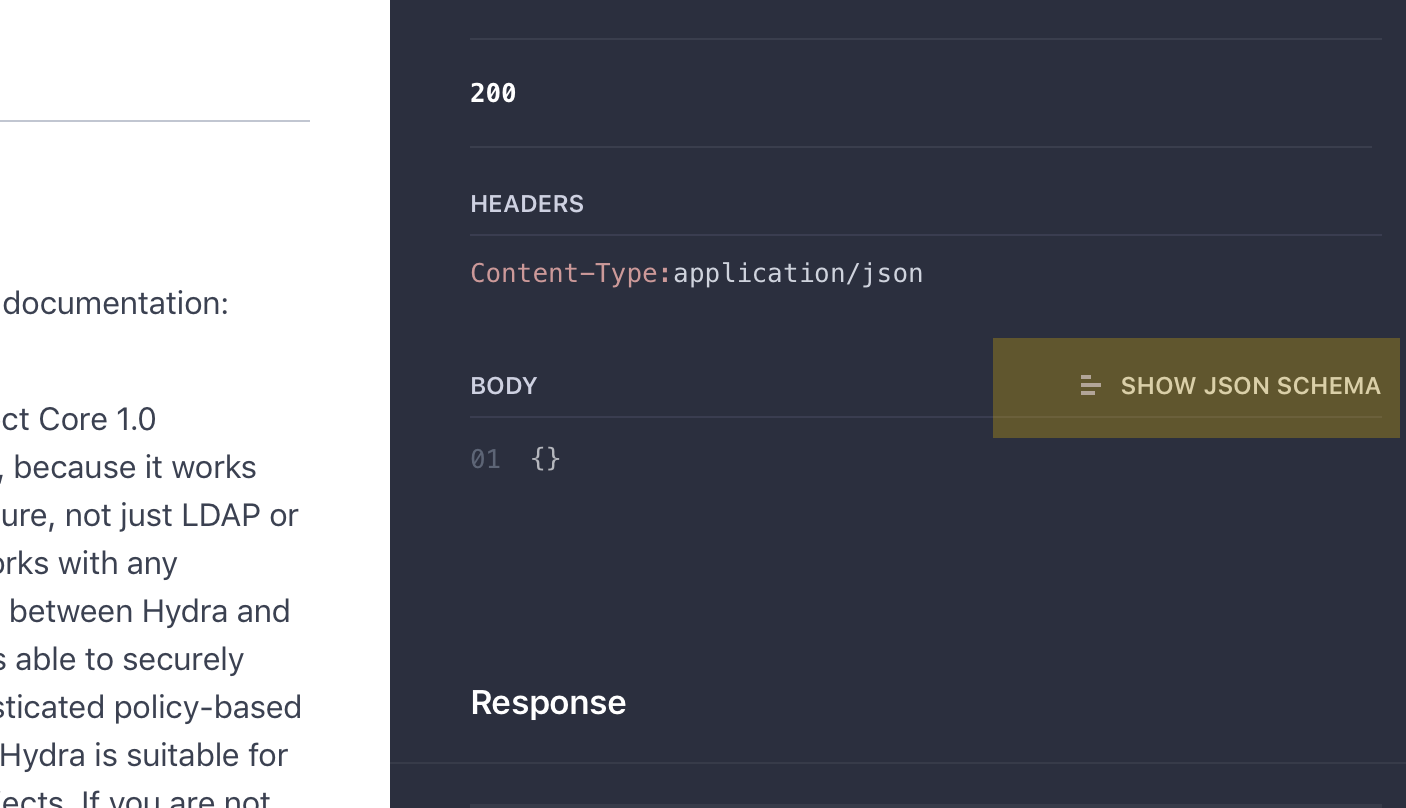 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.OAuth2consentRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The OAuth2consentRequest model module.
+ * @module model/OAuth2consentRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new OAuth2consentRequest
.
+ * @alias module:model/OAuth2consentRequest
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a OAuth2consentRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/OAuth2consentRequest} obj Optional instance to populate.
+ * @return {module:model/OAuth2consentRequest} The populated OAuth2consentRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('audience')) {
+ obj['audience'] = ApiClient.convertToType(data['audience'], 'String');
+ }
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ if (data.hasOwnProperty('redirectUrl')) {
+ obj['redirectUrl'] = ApiClient.convertToType(data['redirectUrl'], 'String');
+ }
+ if (data.hasOwnProperty('requestedScopes')) {
+ obj['requestedScopes'] = ApiClient.convertToType(data['requestedScopes'], ['String']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Audience is the client id that initiated the OAuth2 request.
+ * @member {String} audience
+ */
+ exports.prototype['audience'] = undefined;
+ /**
+ * ID is the id of this consent request.
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+ /**
+ * Redirect URL is the URL where the user agent should be redirected to after the consent has been accepted or rejected.
+ * @member {String} redirectUrl
+ */
+ exports.prototype['redirectUrl'] = undefined;
+ /**
+ * RequestedScopes represents a list of scopes that have been requested by the OAuth2 request initiator.
+ * @member {Array.} requestedScopes
+ */
+ exports.prototype['requestedScopes'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/Policy.js b/sdk/js/swagger/src/model/Policy.js
new file mode 100644
index 00000000000..d44b0392e66
--- /dev/null
+++ b/sdk/js/swagger/src/model/Policy.js
@@ -0,0 +1,137 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 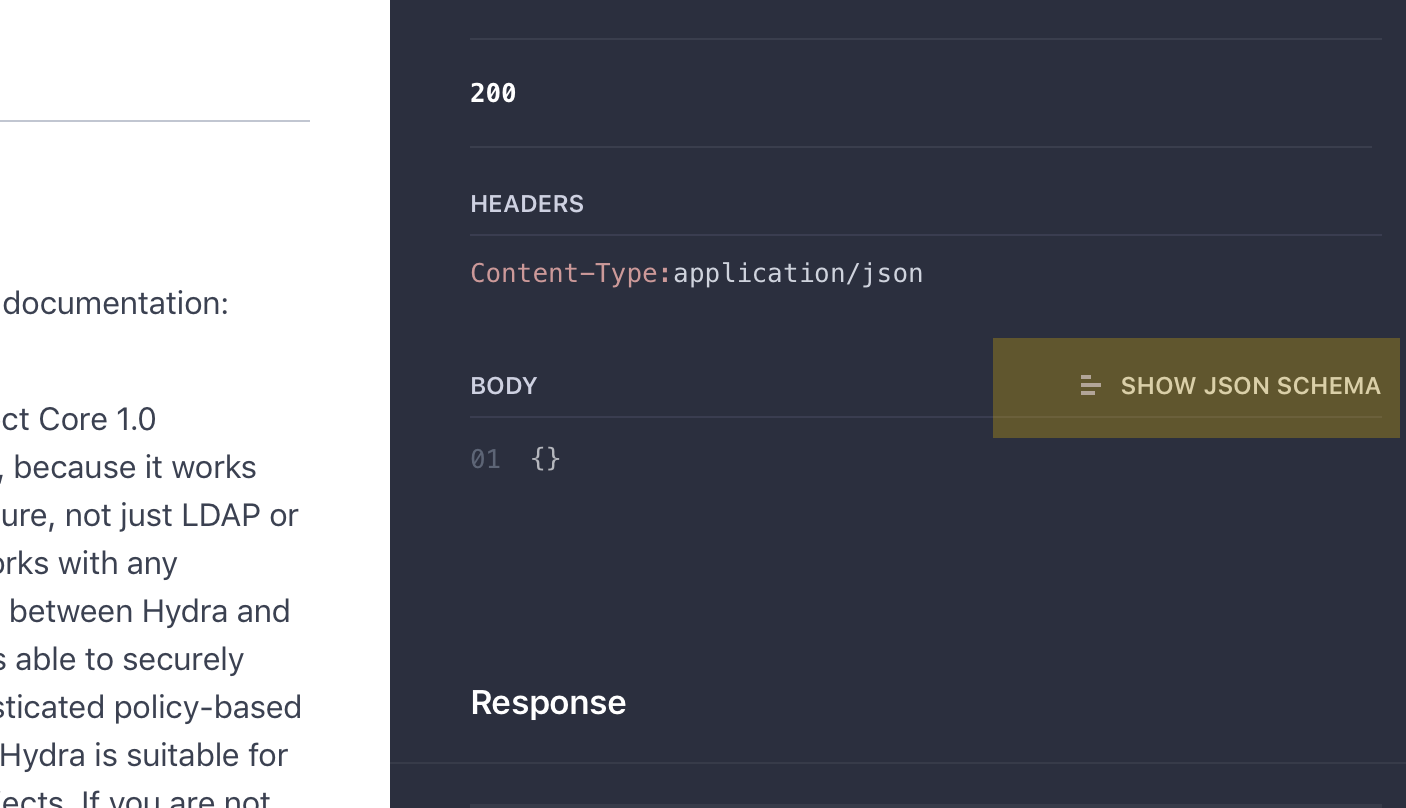 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/PolicyConditions'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./PolicyConditions'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.Policy = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.PolicyConditions);
+ }
+}(this, function(ApiClient, PolicyConditions) {
+ 'use strict';
+
+
+
+
+ /**
+ * The Policy model module.
+ * @module model/Policy
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new Policy
.
+ * @alias module:model/Policy
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a Policy
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/Policy} obj Optional instance to populate.
+ * @return {module:model/Policy} The populated Policy
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('actions')) {
+ obj['actions'] = ApiClient.convertToType(data['actions'], ['String']);
+ }
+ if (data.hasOwnProperty('conditions')) {
+ obj['conditions'] = ApiClient.convertToType(data['conditions'], {'String': PolicyConditions});
+ }
+ if (data.hasOwnProperty('description')) {
+ obj['description'] = ApiClient.convertToType(data['description'], 'String');
+ }
+ if (data.hasOwnProperty('effect')) {
+ obj['effect'] = ApiClient.convertToType(data['effect'], 'String');
+ }
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ if (data.hasOwnProperty('resources')) {
+ obj['resources'] = ApiClient.convertToType(data['resources'], ['String']);
+ }
+ if (data.hasOwnProperty('subjects')) {
+ obj['subjects'] = ApiClient.convertToType(data['subjects'], ['String']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Actions impacted by the policy.
+ * @member {Array.} actions
+ */
+ exports.prototype['actions'] = undefined;
+ /**
+ * Conditions under which the policy is active.
+ * @member {Object.} conditions
+ */
+ exports.prototype['conditions'] = undefined;
+ /**
+ * Description of the policy.
+ * @member {String} description
+ */
+ exports.prototype['description'] = undefined;
+ /**
+ * Effect of the policy
+ * @member {String} effect
+ */
+ exports.prototype['effect'] = undefined;
+ /**
+ * ID of the policy.
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+ /**
+ * Resources impacted by the policy.
+ * @member {Array.} resources
+ */
+ exports.prototype['resources'] = undefined;
+ /**
+ * Subjects impacted by the policy.
+ * @member {Array.} subjects
+ */
+ exports.prototype['subjects'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/PolicyConditions.js b/sdk/js/swagger/src/model/PolicyConditions.js
new file mode 100644
index 00000000000..77bde845c57
--- /dev/null
+++ b/sdk/js/swagger/src/model/PolicyConditions.js
@@ -0,0 +1,90 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 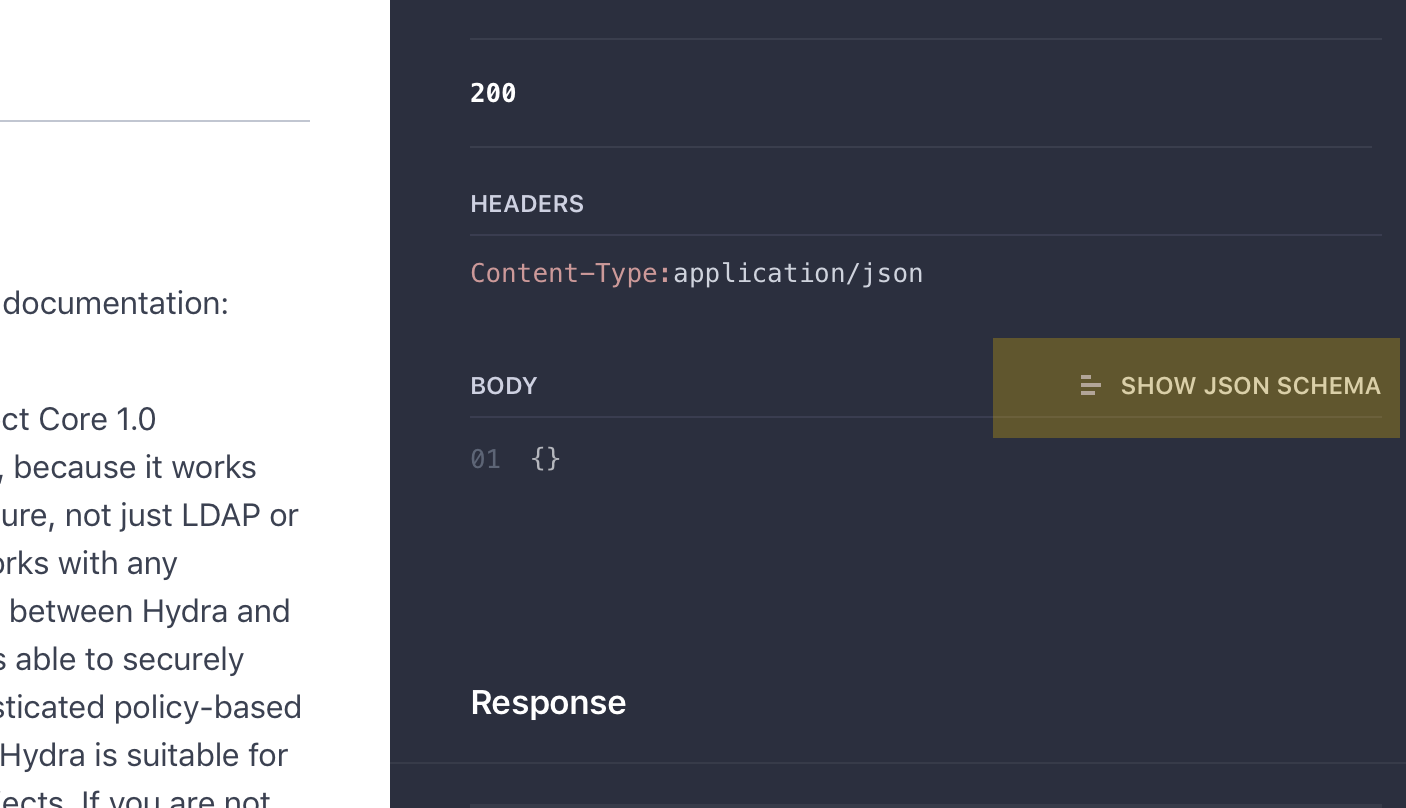 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.PolicyConditions = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The PolicyConditions model module.
+ * @module model/PolicyConditions
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new PolicyConditions
.
+ * @alias module:model/PolicyConditions
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+ };
+
+ /**
+ * Constructs a PolicyConditions
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/PolicyConditions} obj Optional instance to populate.
+ * @return {module:model/PolicyConditions} The populated PolicyConditions
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('options')) {
+ obj['options'] = ApiClient.convertToType(data['options'], {'String': Object});
+ }
+ if (data.hasOwnProperty('type')) {
+ obj['type'] = ApiClient.convertToType(data['type'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {Object.} options
+ */
+ exports.prototype['options'] = undefined;
+ /**
+ * @member {String} type
+ */
+ exports.prototype['type'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/RawMessage.js b/sdk/js/swagger/src/model/RawMessage.js
new file mode 100644
index 00000000000..876b9a09c38
--- /dev/null
+++ b/sdk/js/swagger/src/model/RawMessage.js
@@ -0,0 +1,80 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 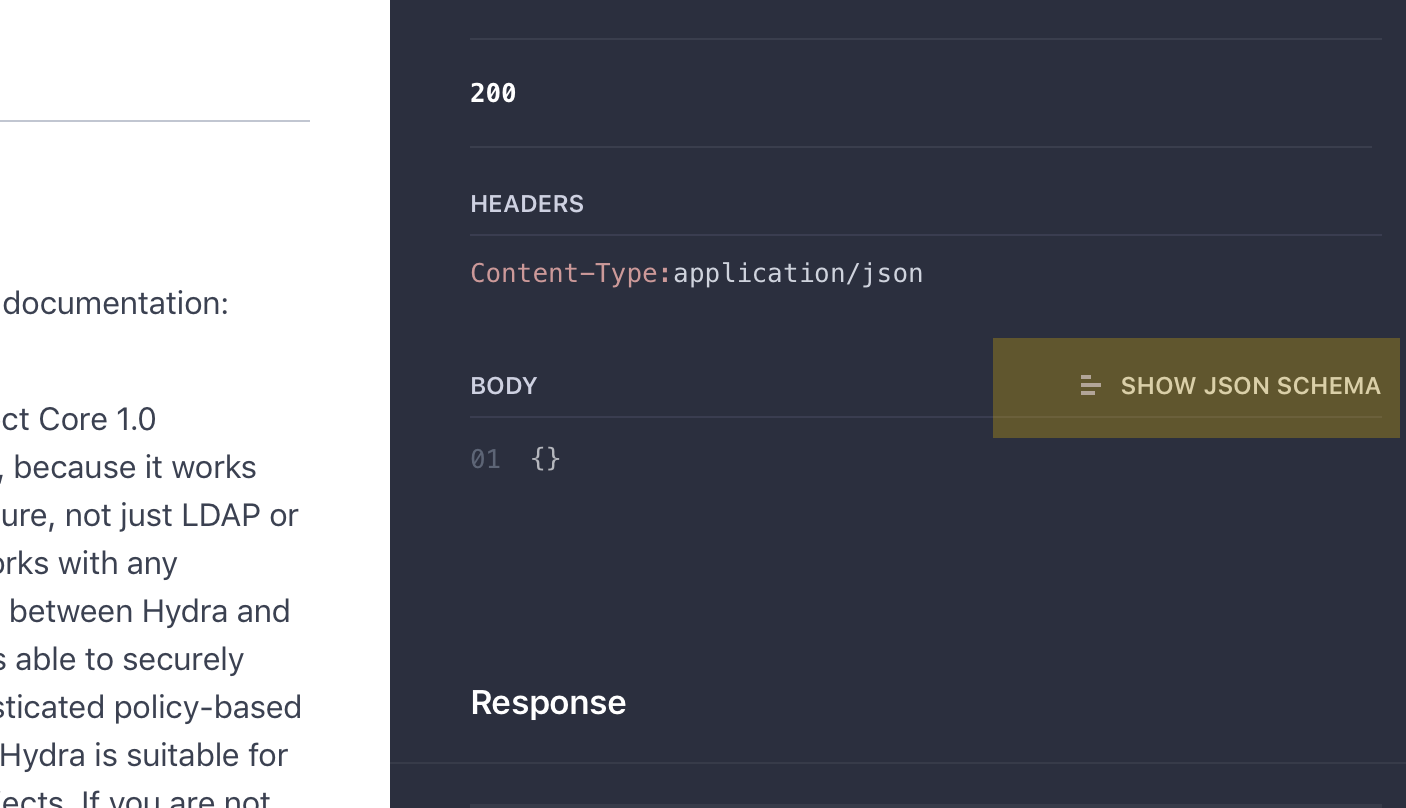 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.RawMessage = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The RawMessage model module.
+ * @module model/RawMessage
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new RawMessage
.
+ * It implements Marshaler and Unmarshaler and can be used to delay JSON decoding or precompute a JSON encoding.
+ * @alias module:model/RawMessage
+ * @class
+ * @extends Array
+ */
+ var exports = function() {
+ var _this = this;
+ _this = new Array();
+ Object.setPrototypeOf(_this, exports);
+
+ return _this;
+ };
+
+ /**
+ * Constructs a RawMessage
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/RawMessage} obj Optional instance to populate.
+ * @return {module:model/RawMessage} The populated RawMessage
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+ ApiClient.constructFromObject(data, obj, 'Number');
+
+ }
+ return obj;
+ }
+
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerAcceptConsentRequest.js b/sdk/js/swagger/src/model/SwaggerAcceptConsentRequest.js
new file mode 100644
index 00000000000..e4ef0278e9e
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerAcceptConsentRequest.js
@@ -0,0 +1,93 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 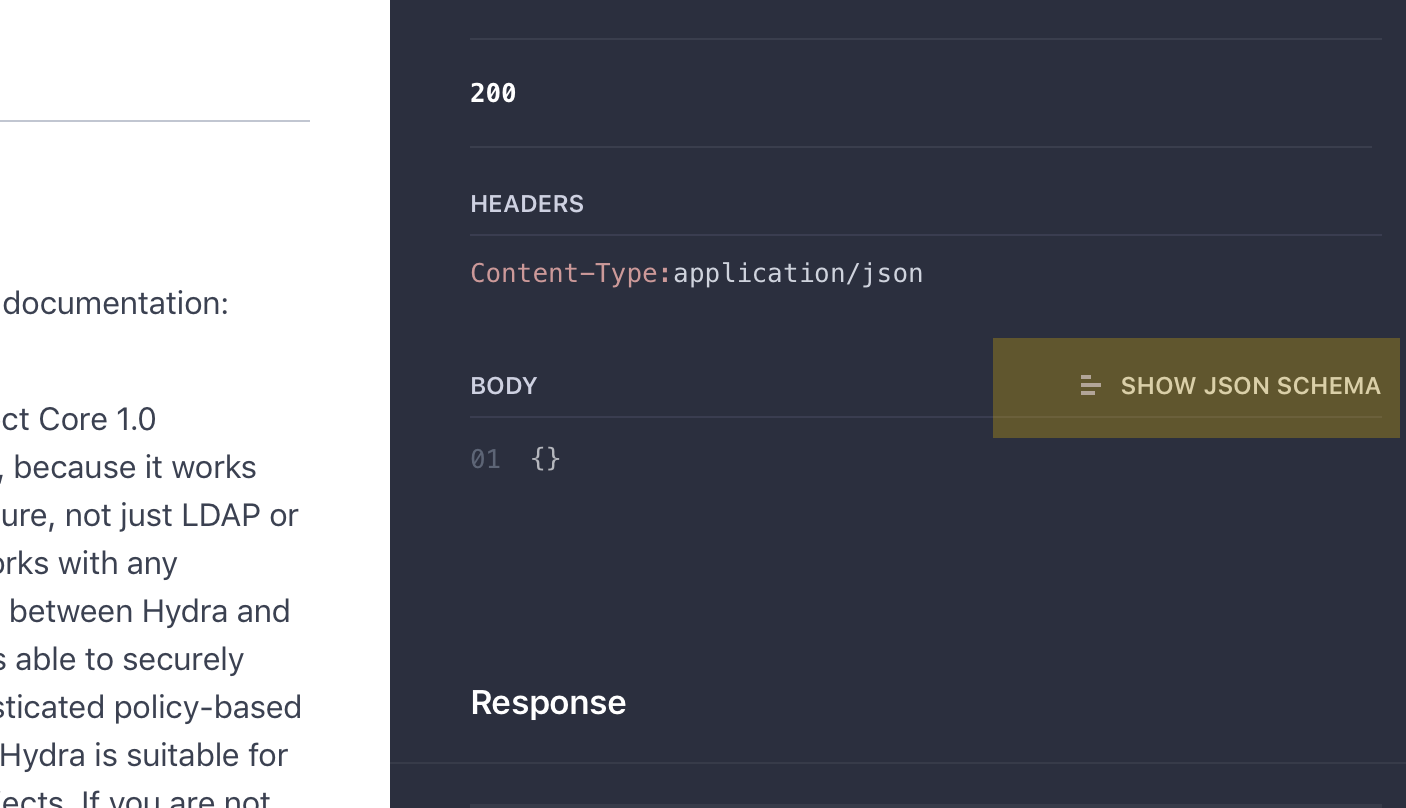 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/ConsentRequestAcceptance'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./ConsentRequestAcceptance'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerAcceptConsentRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance);
+ }
+}(this, function(ApiClient, ConsentRequestAcceptance) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerAcceptConsentRequest model module.
+ * @module model/SwaggerAcceptConsentRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerAcceptConsentRequest
.
+ * @alias module:model/SwaggerAcceptConsentRequest
+ * @class
+ * @param body {module:model/ConsentRequestAcceptance}
+ * @param id {String} in: path
+ */
+ var exports = function(body, id) {
+ var _this = this;
+
+ _this['Body'] = body;
+ _this['id'] = id;
+ };
+
+ /**
+ * Constructs a SwaggerAcceptConsentRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerAcceptConsentRequest} obj Optional instance to populate.
+ * @return {module:model/SwaggerAcceptConsentRequest} The populated SwaggerAcceptConsentRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = ConsentRequestAcceptance.constructFromObject(data['Body']);
+ }
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/ConsentRequestAcceptance} Body
+ */
+ exports.prototype['Body'] = undefined;
+ /**
+ * in: path
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerCreatePolicyParameters.js b/sdk/js/swagger/src/model/SwaggerCreatePolicyParameters.js
new file mode 100644
index 00000000000..2c82d4ef853
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerCreatePolicyParameters.js
@@ -0,0 +1,82 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 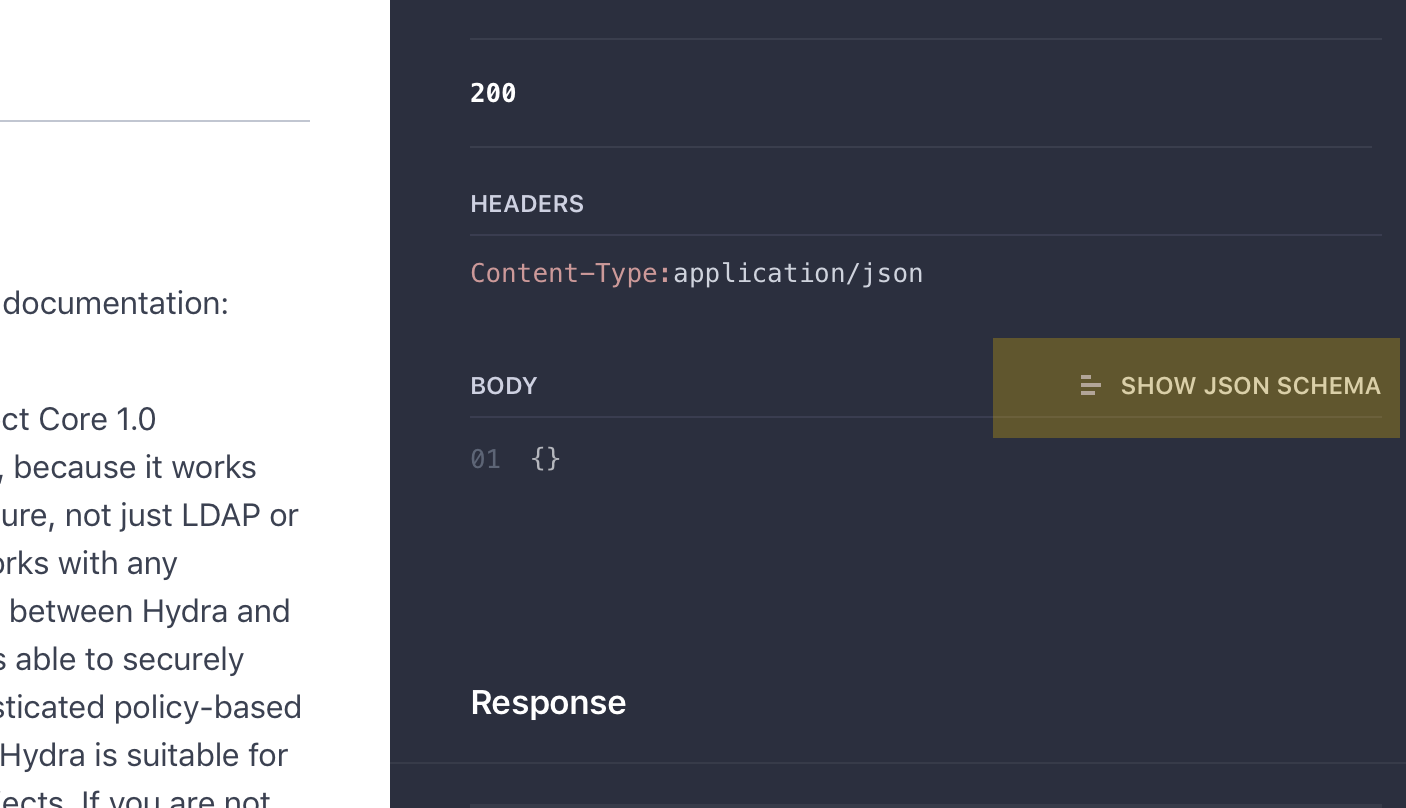 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/Policy'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./Policy'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerCreatePolicyParameters = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.Policy);
+ }
+}(this, function(ApiClient, Policy) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerCreatePolicyParameters model module.
+ * @module model/SwaggerCreatePolicyParameters
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerCreatePolicyParameters
.
+ * @alias module:model/SwaggerCreatePolicyParameters
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerCreatePolicyParameters
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerCreatePolicyParameters} obj Optional instance to populate.
+ * @return {module:model/SwaggerCreatePolicyParameters} The populated SwaggerCreatePolicyParameters
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = Policy.constructFromObject(data['Body']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/Policy} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerDoesWardenAllowAccessRequestParameters.js b/sdk/js/swagger/src/model/SwaggerDoesWardenAllowAccessRequestParameters.js
new file mode 100644
index 00000000000..ac856b8f1a1
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerDoesWardenAllowAccessRequestParameters.js
@@ -0,0 +1,82 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 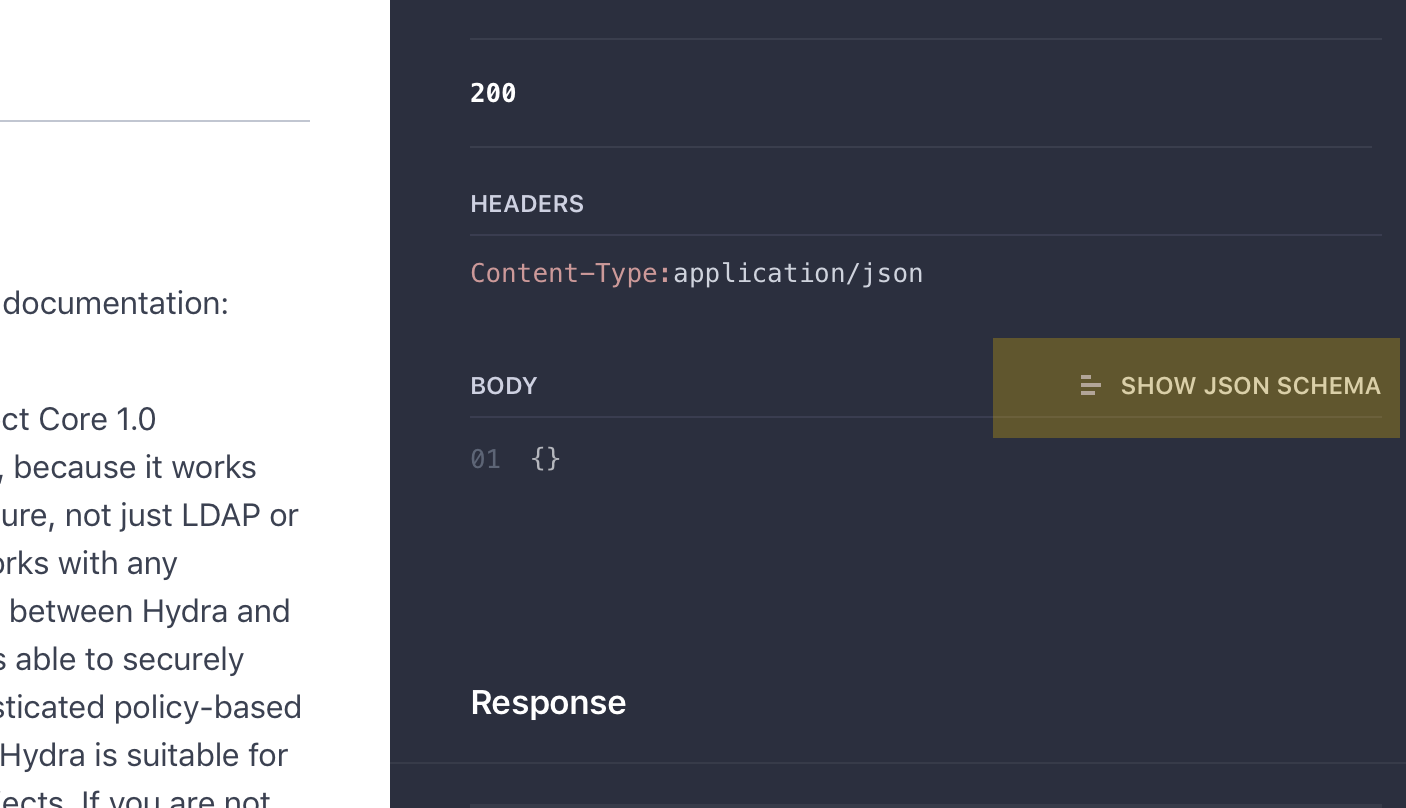 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/WardenAccessRequest'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./WardenAccessRequest'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowAccessRequestParameters = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.WardenAccessRequest);
+ }
+}(this, function(ApiClient, WardenAccessRequest) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerDoesWardenAllowAccessRequestParameters model module.
+ * @module model/SwaggerDoesWardenAllowAccessRequestParameters
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerDoesWardenAllowAccessRequestParameters
.
+ * @alias module:model/SwaggerDoesWardenAllowAccessRequestParameters
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerDoesWardenAllowAccessRequestParameters
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerDoesWardenAllowAccessRequestParameters} obj Optional instance to populate.
+ * @return {module:model/SwaggerDoesWardenAllowAccessRequestParameters} The populated SwaggerDoesWardenAllowAccessRequestParameters
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = WardenAccessRequest.constructFromObject(data['Body']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/WardenAccessRequest} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerDoesWardenAllowTokenAccessRequestParameters.js b/sdk/js/swagger/src/model/SwaggerDoesWardenAllowTokenAccessRequestParameters.js
new file mode 100644
index 00000000000..4c456a16a40
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerDoesWardenAllowTokenAccessRequestParameters.js
@@ -0,0 +1,82 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 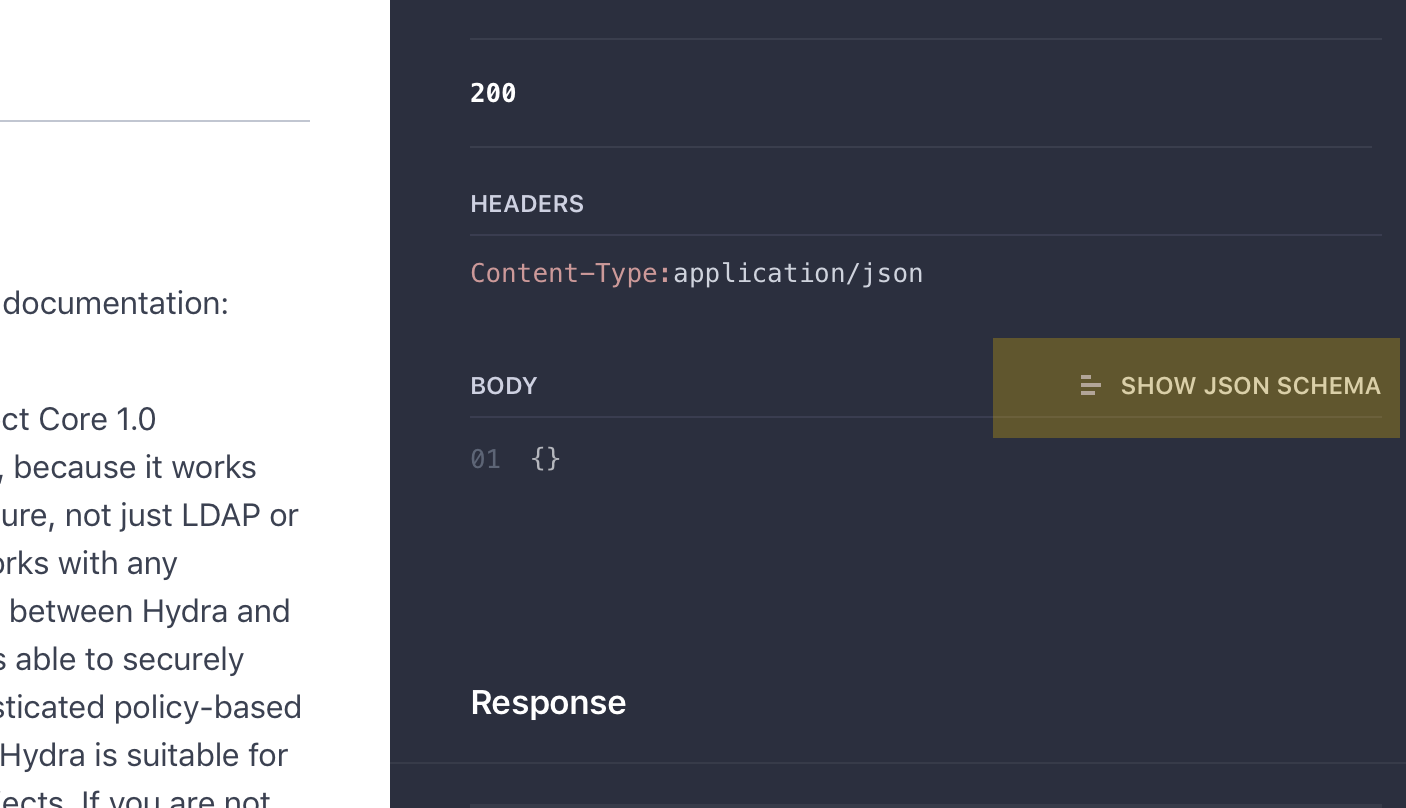 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/WardenTokenAccessRequest'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./WardenTokenAccessRequest'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowTokenAccessRequestParameters = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest);
+ }
+}(this, function(ApiClient, WardenTokenAccessRequest) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerDoesWardenAllowTokenAccessRequestParameters model module.
+ * @module model/SwaggerDoesWardenAllowTokenAccessRequestParameters
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerDoesWardenAllowTokenAccessRequestParameters
.
+ * @alias module:model/SwaggerDoesWardenAllowTokenAccessRequestParameters
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerDoesWardenAllowTokenAccessRequestParameters
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerDoesWardenAllowTokenAccessRequestParameters} obj Optional instance to populate.
+ * @return {module:model/SwaggerDoesWardenAllowTokenAccessRequestParameters} The populated SwaggerDoesWardenAllowTokenAccessRequestParameters
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = WardenTokenAccessRequest.constructFromObject(data['Body']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/WardenTokenAccessRequest} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerGetPolicyParameters.js b/sdk/js/swagger/src/model/SwaggerGetPolicyParameters.js
new file mode 100644
index 00000000000..e03a6c0d555
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerGetPolicyParameters.js
@@ -0,0 +1,83 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 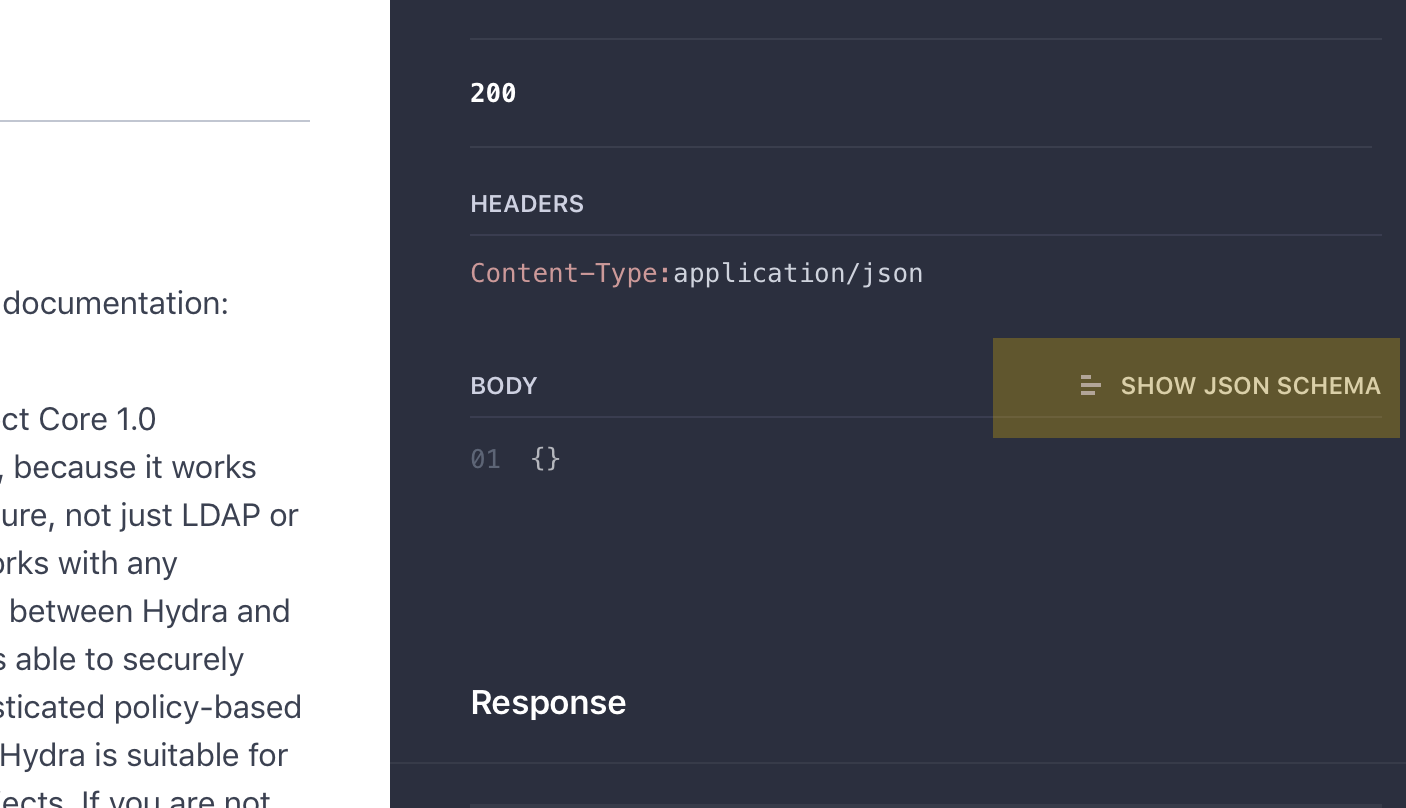 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerGetPolicyParameters = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerGetPolicyParameters model module.
+ * @module model/SwaggerGetPolicyParameters
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerGetPolicyParameters
.
+ * @alias module:model/SwaggerGetPolicyParameters
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerGetPolicyParameters
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerGetPolicyParameters} obj Optional instance to populate.
+ * @return {module:model/SwaggerGetPolicyParameters} The populated SwaggerGetPolicyParameters
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The id of the policy. in: path
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerJsonWebKeyQuery.js b/sdk/js/swagger/src/model/SwaggerJsonWebKeyQuery.js
new file mode 100644
index 00000000000..6e88976df1e
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerJsonWebKeyQuery.js
@@ -0,0 +1,94 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 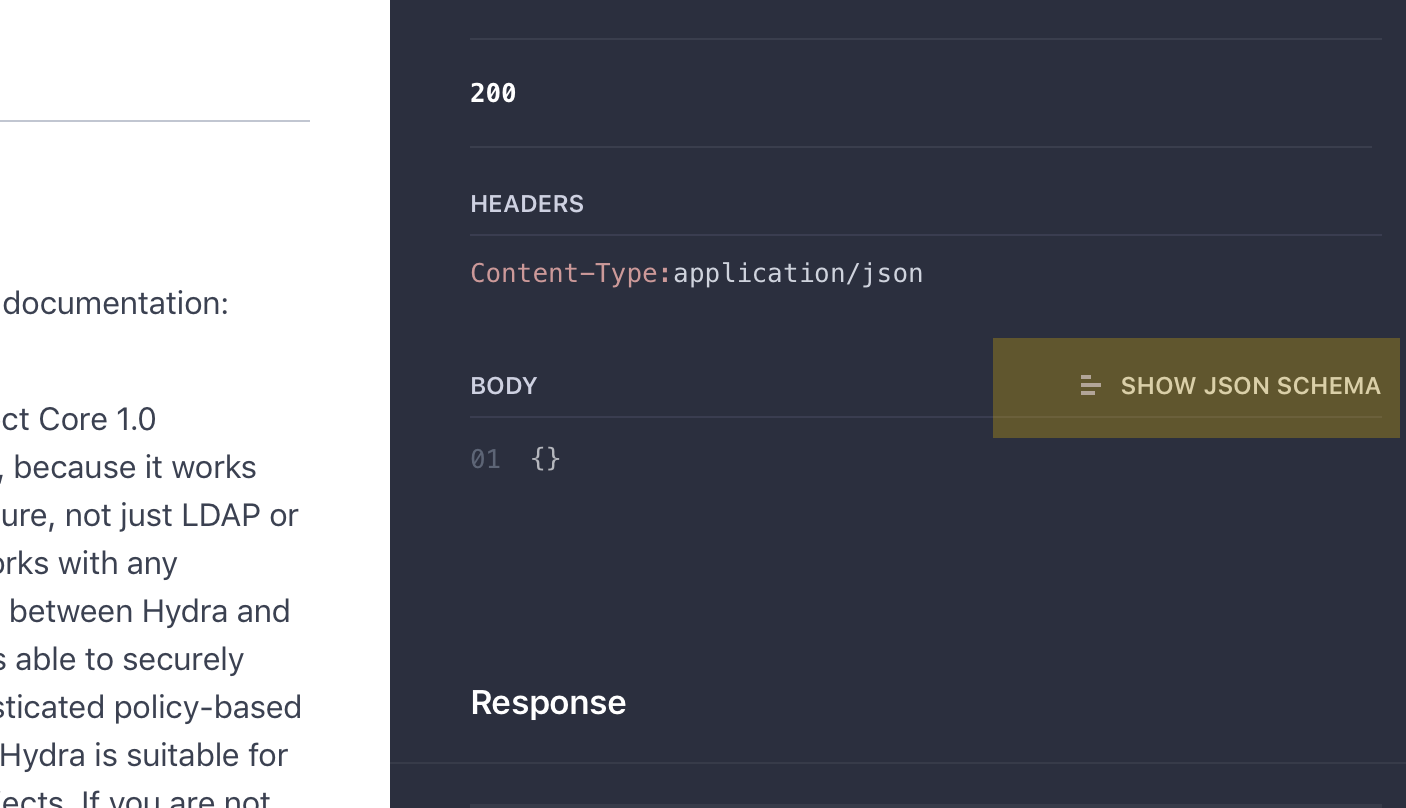 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerJsonWebKeyQuery = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerJsonWebKeyQuery model module.
+ * @module model/SwaggerJsonWebKeyQuery
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerJsonWebKeyQuery
.
+ * @alias module:model/SwaggerJsonWebKeyQuery
+ * @class
+ * @param kid {String} The kid of the desired key in: path
+ * @param set {String} The set in: path
+ */
+ var exports = function(kid, set) {
+ var _this = this;
+
+ _this['kid'] = kid;
+ _this['set'] = set;
+ };
+
+ /**
+ * Constructs a SwaggerJsonWebKeyQuery
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerJsonWebKeyQuery} obj Optional instance to populate.
+ * @return {module:model/SwaggerJsonWebKeyQuery} The populated SwaggerJsonWebKeyQuery
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('kid')) {
+ obj['kid'] = ApiClient.convertToType(data['kid'], 'String');
+ }
+ if (data.hasOwnProperty('set')) {
+ obj['set'] = ApiClient.convertToType(data['set'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The kid of the desired key in: path
+ * @member {String} kid
+ */
+ exports.prototype['kid'] = undefined;
+ /**
+ * The set in: path
+ * @member {String} set
+ */
+ exports.prototype['set'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerJwkCreateSet.js b/sdk/js/swagger/src/model/SwaggerJwkCreateSet.js
new file mode 100644
index 00000000000..612f7833432
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerJwkCreateSet.js
@@ -0,0 +1,92 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 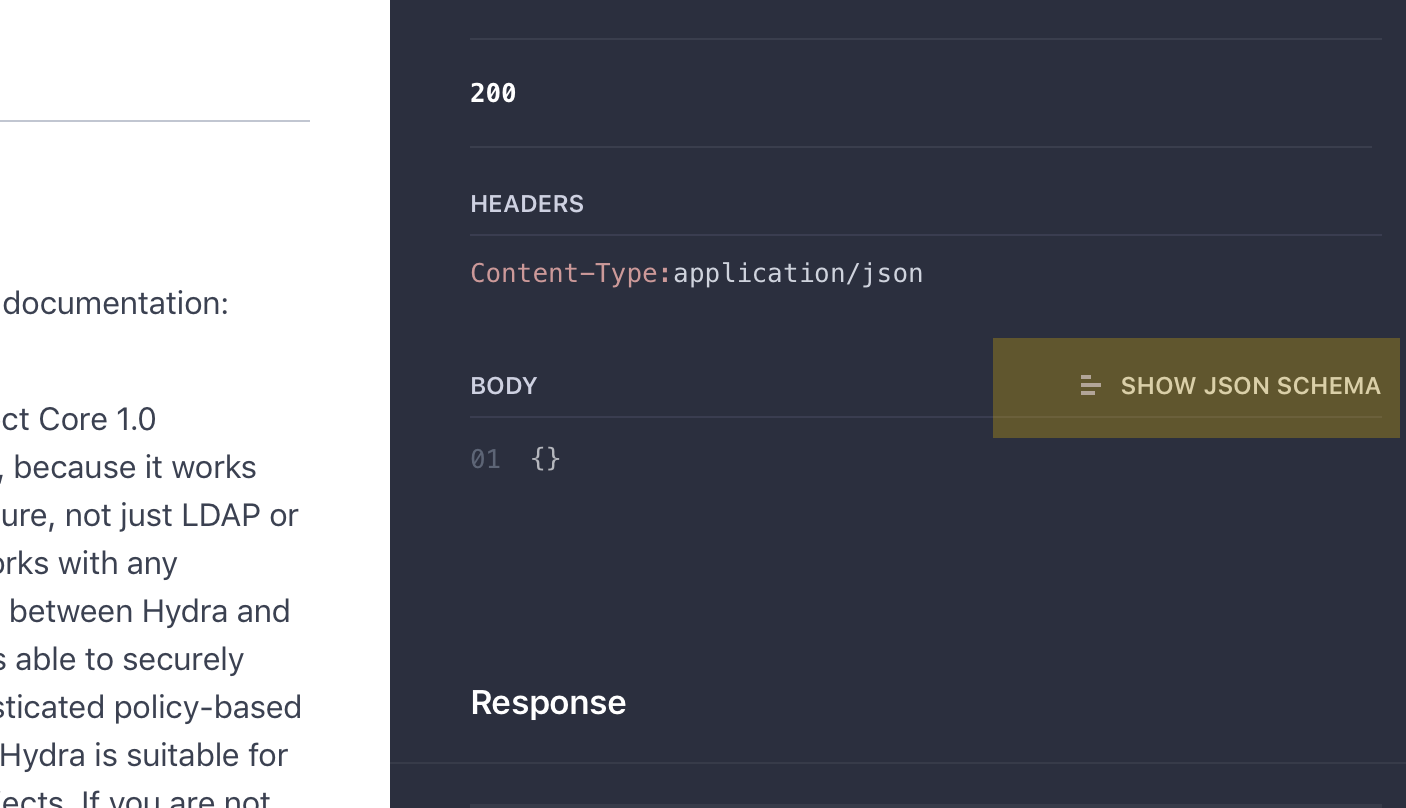 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/JsonWebKeySetGeneratorRequest'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./JsonWebKeySetGeneratorRequest'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerJwkCreateSet = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest);
+ }
+}(this, function(ApiClient, JsonWebKeySetGeneratorRequest) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerJwkCreateSet model module.
+ * @module model/SwaggerJwkCreateSet
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerJwkCreateSet
.
+ * @alias module:model/SwaggerJwkCreateSet
+ * @class
+ * @param set {String} The set in: path
+ */
+ var exports = function(set) {
+ var _this = this;
+
+
+ _this['set'] = set;
+ };
+
+ /**
+ * Constructs a SwaggerJwkCreateSet
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerJwkCreateSet} obj Optional instance to populate.
+ * @return {module:model/SwaggerJwkCreateSet} The populated SwaggerJwkCreateSet
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = JsonWebKeySetGeneratorRequest.constructFromObject(data['Body']);
+ }
+ if (data.hasOwnProperty('set')) {
+ obj['set'] = ApiClient.convertToType(data['set'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/JsonWebKeySetGeneratorRequest} Body
+ */
+ exports.prototype['Body'] = undefined;
+ /**
+ * The set in: path
+ * @member {String} set
+ */
+ exports.prototype['set'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerJwkSetQuery.js b/sdk/js/swagger/src/model/SwaggerJwkSetQuery.js
new file mode 100644
index 00000000000..ed7992e663c
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerJwkSetQuery.js
@@ -0,0 +1,84 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 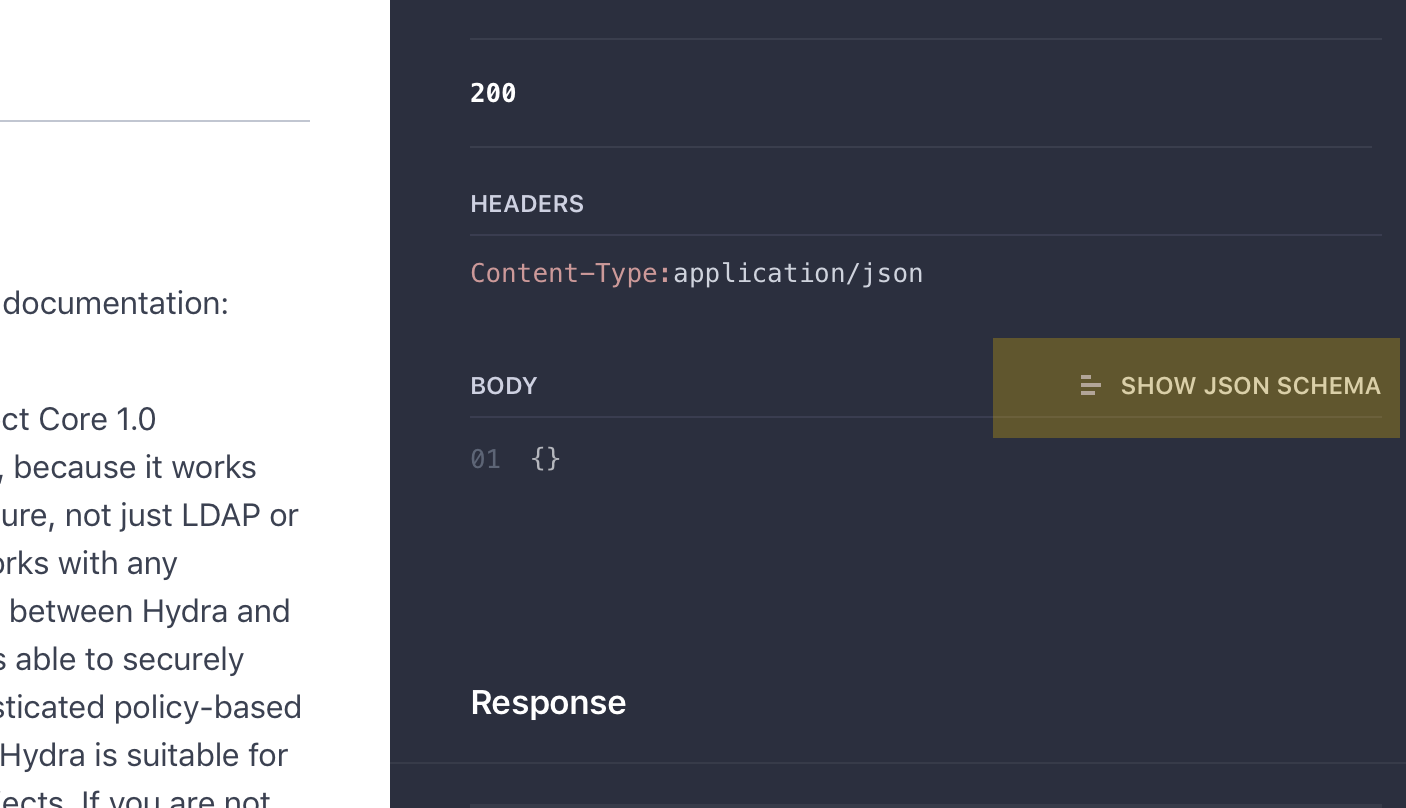 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerJwkSetQuery = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerJwkSetQuery model module.
+ * @module model/SwaggerJwkSetQuery
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerJwkSetQuery
.
+ * @alias module:model/SwaggerJwkSetQuery
+ * @class
+ * @param set {String} The set in: path
+ */
+ var exports = function(set) {
+ var _this = this;
+
+ _this['set'] = set;
+ };
+
+ /**
+ * Constructs a SwaggerJwkSetQuery
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerJwkSetQuery} obj Optional instance to populate.
+ * @return {module:model/SwaggerJwkSetQuery} The populated SwaggerJwkSetQuery
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('set')) {
+ obj['set'] = ApiClient.convertToType(data['set'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The set in: path
+ * @member {String} set
+ */
+ exports.prototype['set'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerJwkUpdateSet.js b/sdk/js/swagger/src/model/SwaggerJwkUpdateSet.js
new file mode 100644
index 00000000000..c0003816070
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerJwkUpdateSet.js
@@ -0,0 +1,92 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 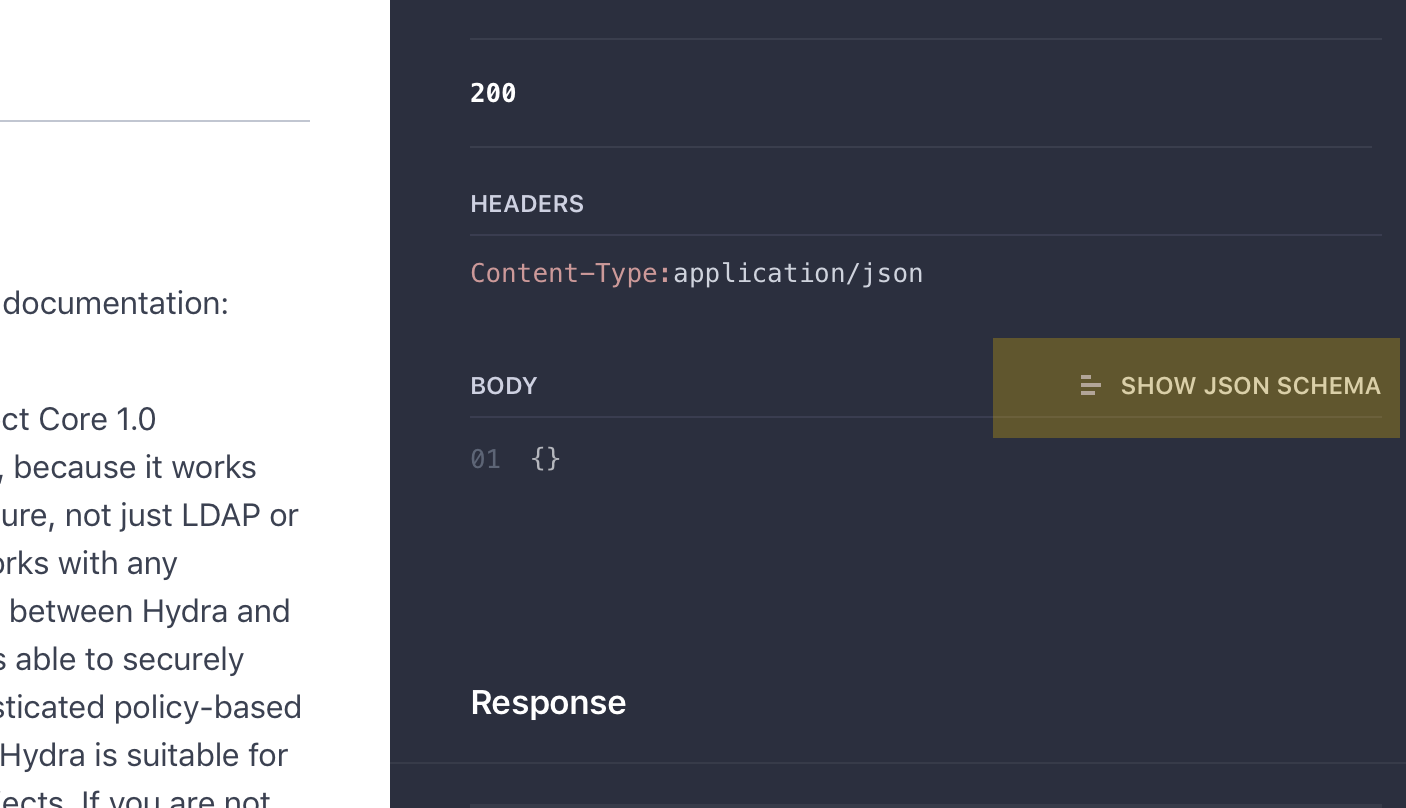 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/JsonWebKeySet'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./JsonWebKeySet'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSet = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.JsonWebKeySet);
+ }
+}(this, function(ApiClient, JsonWebKeySet) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerJwkUpdateSet model module.
+ * @module model/SwaggerJwkUpdateSet
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerJwkUpdateSet
.
+ * @alias module:model/SwaggerJwkUpdateSet
+ * @class
+ * @param set {String} The set in: path
+ */
+ var exports = function(set) {
+ var _this = this;
+
+
+ _this['set'] = set;
+ };
+
+ /**
+ * Constructs a SwaggerJwkUpdateSet
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerJwkUpdateSet} obj Optional instance to populate.
+ * @return {module:model/SwaggerJwkUpdateSet} The populated SwaggerJwkUpdateSet
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = JsonWebKeySet.constructFromObject(data['Body']);
+ }
+ if (data.hasOwnProperty('set')) {
+ obj['set'] = ApiClient.convertToType(data['set'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/JsonWebKeySet} Body
+ */
+ exports.prototype['Body'] = undefined;
+ /**
+ * The set in: path
+ * @member {String} set
+ */
+ exports.prototype['set'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerJwkUpdateSetKey.js b/sdk/js/swagger/src/model/SwaggerJwkUpdateSetKey.js
new file mode 100644
index 00000000000..986b2c93909
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerJwkUpdateSetKey.js
@@ -0,0 +1,102 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 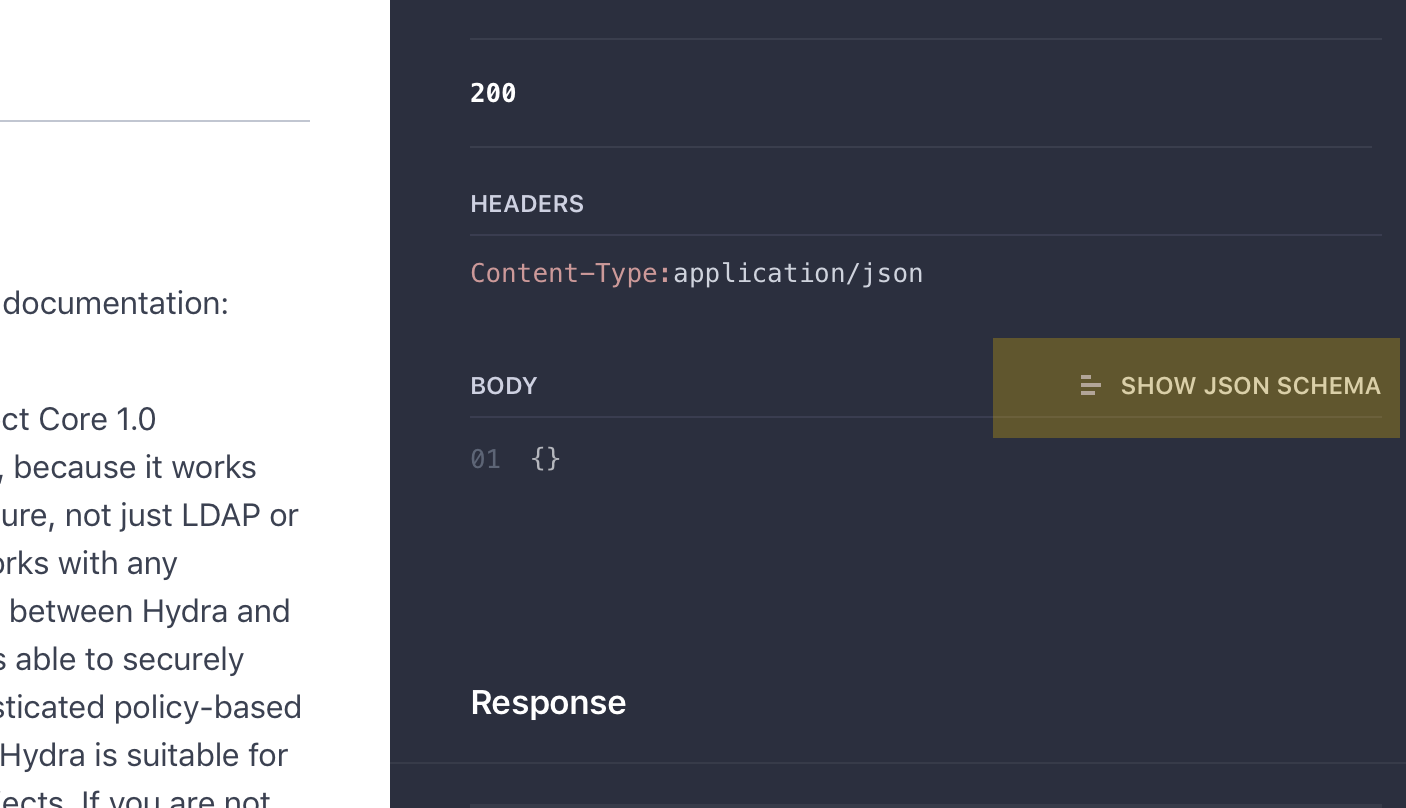 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/JsonWebKey'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./JsonWebKey'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.JsonWebKey);
+ }
+}(this, function(ApiClient, JsonWebKey) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerJwkUpdateSetKey model module.
+ * @module model/SwaggerJwkUpdateSetKey
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerJwkUpdateSetKey
.
+ * @alias module:model/SwaggerJwkUpdateSetKey
+ * @class
+ * @param kid {String} The kid of the desired key in: path
+ * @param set {String} The set in: path
+ */
+ var exports = function(kid, set) {
+ var _this = this;
+
+
+ _this['kid'] = kid;
+ _this['set'] = set;
+ };
+
+ /**
+ * Constructs a SwaggerJwkUpdateSetKey
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerJwkUpdateSetKey} obj Optional instance to populate.
+ * @return {module:model/SwaggerJwkUpdateSetKey} The populated SwaggerJwkUpdateSetKey
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = JsonWebKey.constructFromObject(data['Body']);
+ }
+ if (data.hasOwnProperty('kid')) {
+ obj['kid'] = ApiClient.convertToType(data['kid'], 'String');
+ }
+ if (data.hasOwnProperty('set')) {
+ obj['set'] = ApiClient.convertToType(data['set'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/JsonWebKey} Body
+ */
+ exports.prototype['Body'] = undefined;
+ /**
+ * The kid of the desired key in: path
+ * @member {String} kid
+ */
+ exports.prototype['kid'] = undefined;
+ /**
+ * The set in: path
+ * @member {String} set
+ */
+ exports.prototype['set'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerListPolicyParameters.js b/sdk/js/swagger/src/model/SwaggerListPolicyParameters.js
new file mode 100644
index 00000000000..14896102869
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerListPolicyParameters.js
@@ -0,0 +1,92 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 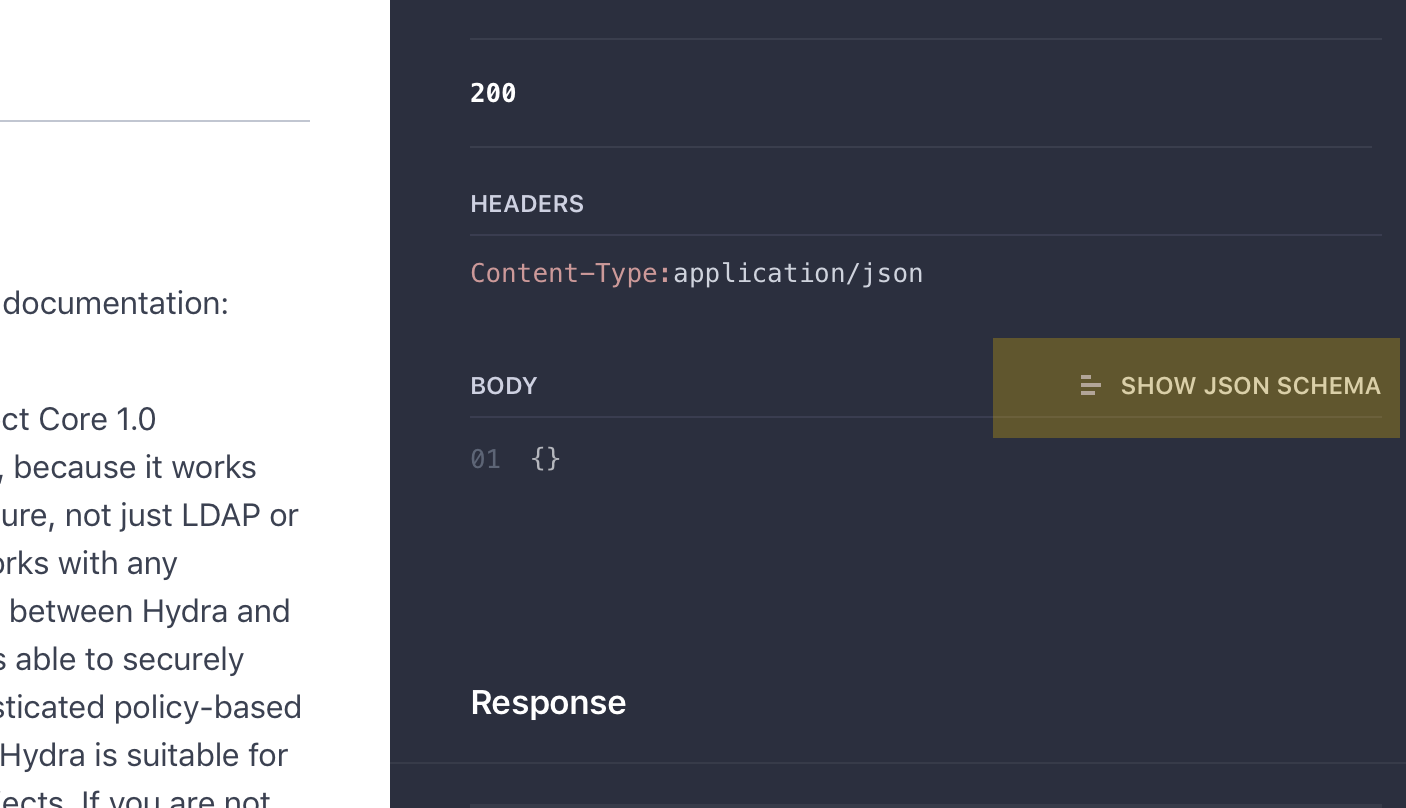 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerListPolicyParameters = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerListPolicyParameters model module.
+ * @module model/SwaggerListPolicyParameters
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerListPolicyParameters
.
+ * @alias module:model/SwaggerListPolicyParameters
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+ };
+
+ /**
+ * Constructs a SwaggerListPolicyParameters
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerListPolicyParameters} obj Optional instance to populate.
+ * @return {module:model/SwaggerListPolicyParameters} The populated SwaggerListPolicyParameters
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('limit')) {
+ obj['limit'] = ApiClient.convertToType(data['limit'], 'Number');
+ }
+ if (data.hasOwnProperty('offset')) {
+ obj['offset'] = ApiClient.convertToType(data['offset'], 'Number');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The maximum amount of policies returned. in: query
+ * @member {Number} limit
+ */
+ exports.prototype['limit'] = undefined;
+ /**
+ * The offset from where to start looking. in: query
+ * @member {Number} offset
+ */
+ exports.prototype['offset'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerListPolicyResponse.js b/sdk/js/swagger/src/model/SwaggerListPolicyResponse.js
new file mode 100644
index 00000000000..708aa5b57ba
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerListPolicyResponse.js
@@ -0,0 +1,84 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 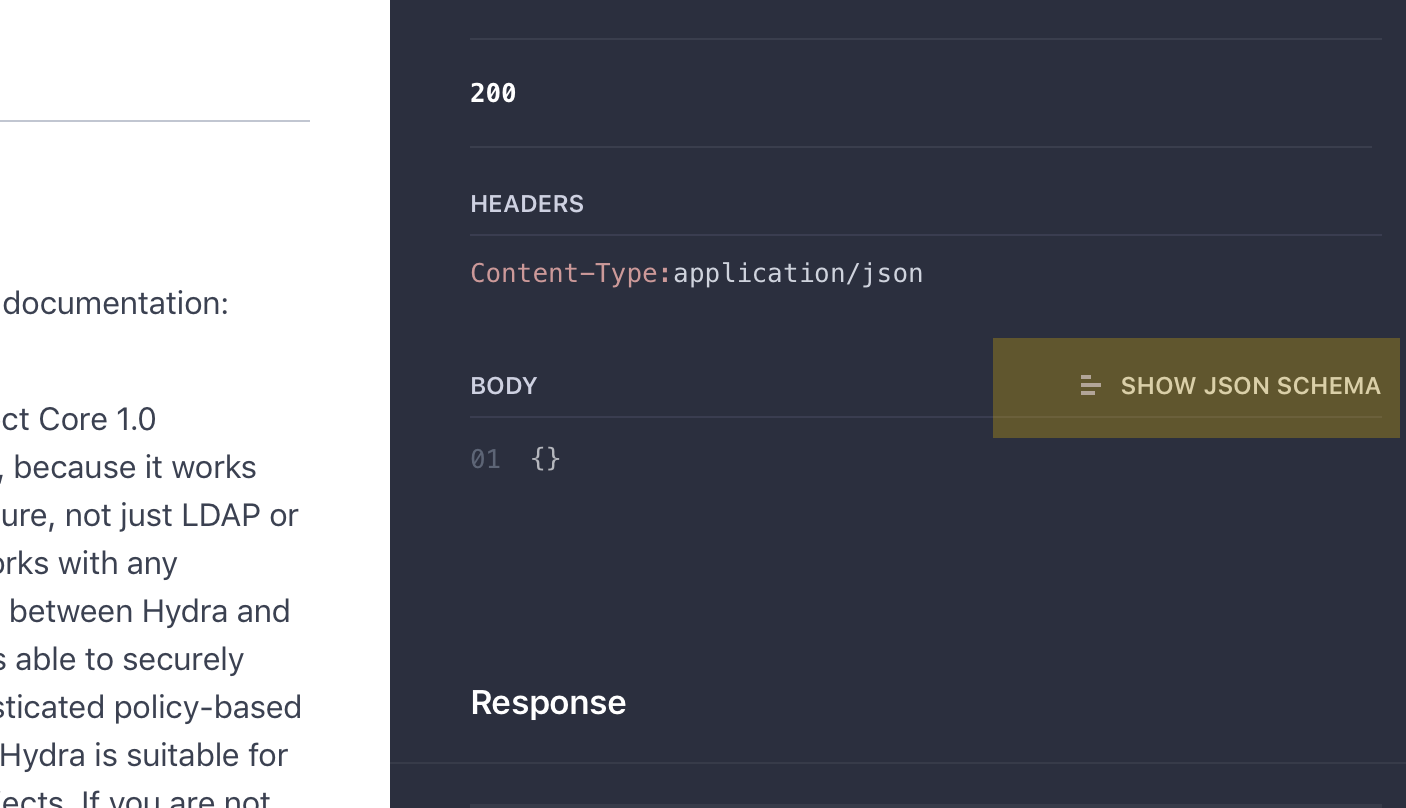 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/Policy'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./Policy'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerListPolicyResponse = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.Policy);
+ }
+}(this, function(ApiClient, Policy) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerListPolicyResponse model module.
+ * @module model/SwaggerListPolicyResponse
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerListPolicyResponse
.
+ * A policy
+ * @alias module:model/SwaggerListPolicyResponse
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerListPolicyResponse
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerListPolicyResponse} obj Optional instance to populate.
+ * @return {module:model/SwaggerListPolicyResponse} The populated SwaggerListPolicyResponse
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = ApiClient.convertToType(data['Body'], [Policy]);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * in: body type: array
+ * @member {Array.} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerOAuthConsentRequest.js b/sdk/js/swagger/src/model/SwaggerOAuthConsentRequest.js
new file mode 100644
index 00000000000..4adad988afb
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerOAuthConsentRequest.js
@@ -0,0 +1,83 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 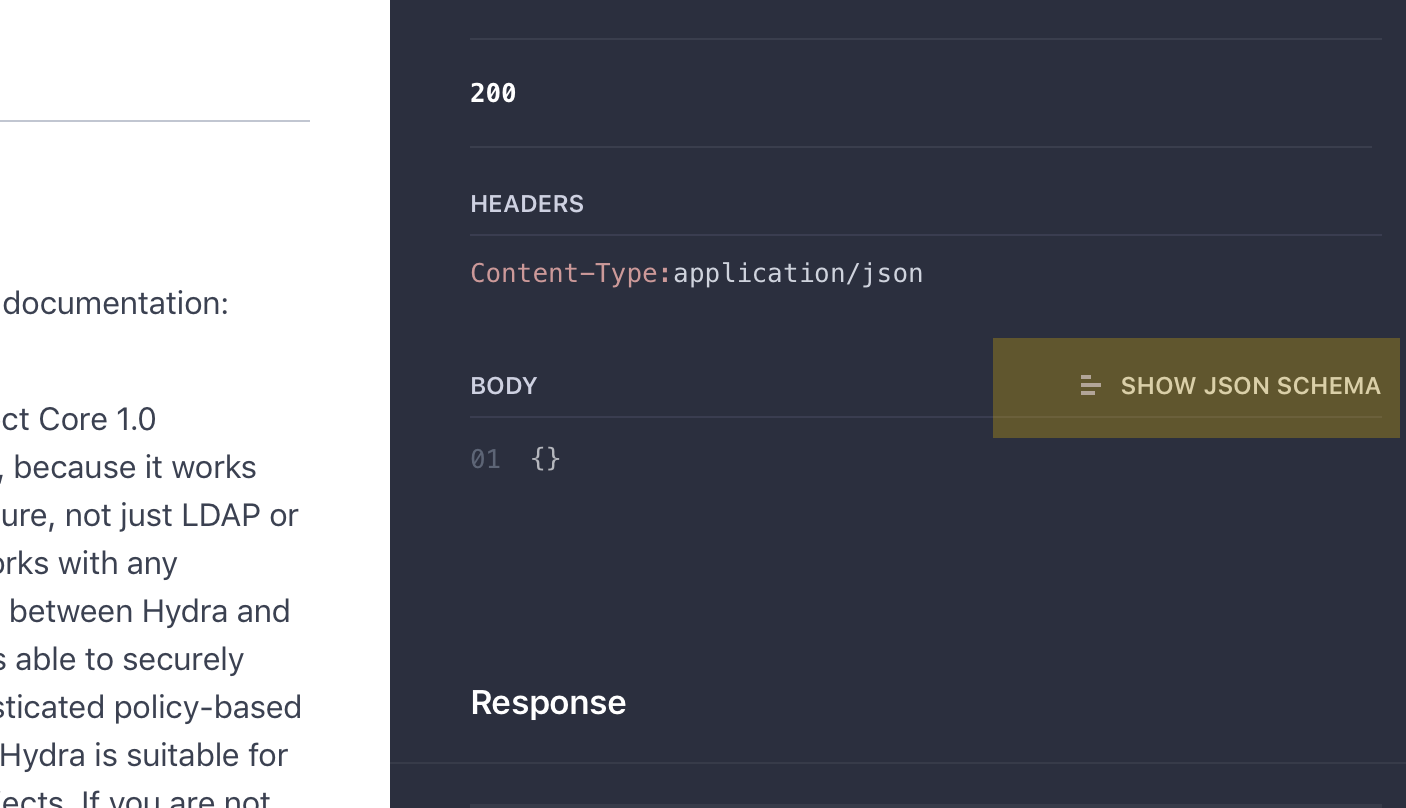 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/OAuth2consentRequest'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./OAuth2consentRequest'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.OAuth2consentRequest);
+ }
+}(this, function(ApiClient, OAuth2consentRequest) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerOAuthConsentRequest model module.
+ * @module model/SwaggerOAuthConsentRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerOAuthConsentRequest
.
+ * The consent request response
+ * @alias module:model/SwaggerOAuthConsentRequest
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerOAuthConsentRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerOAuthConsentRequest} obj Optional instance to populate.
+ * @return {module:model/SwaggerOAuthConsentRequest} The populated SwaggerOAuthConsentRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = OAuth2consentRequest.constructFromObject(data['Body']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/OAuth2consentRequest} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerOAuthConsentRequestPayload.js b/sdk/js/swagger/src/model/SwaggerOAuthConsentRequestPayload.js
new file mode 100644
index 00000000000..1fc83404e2d
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerOAuthConsentRequestPayload.js
@@ -0,0 +1,84 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 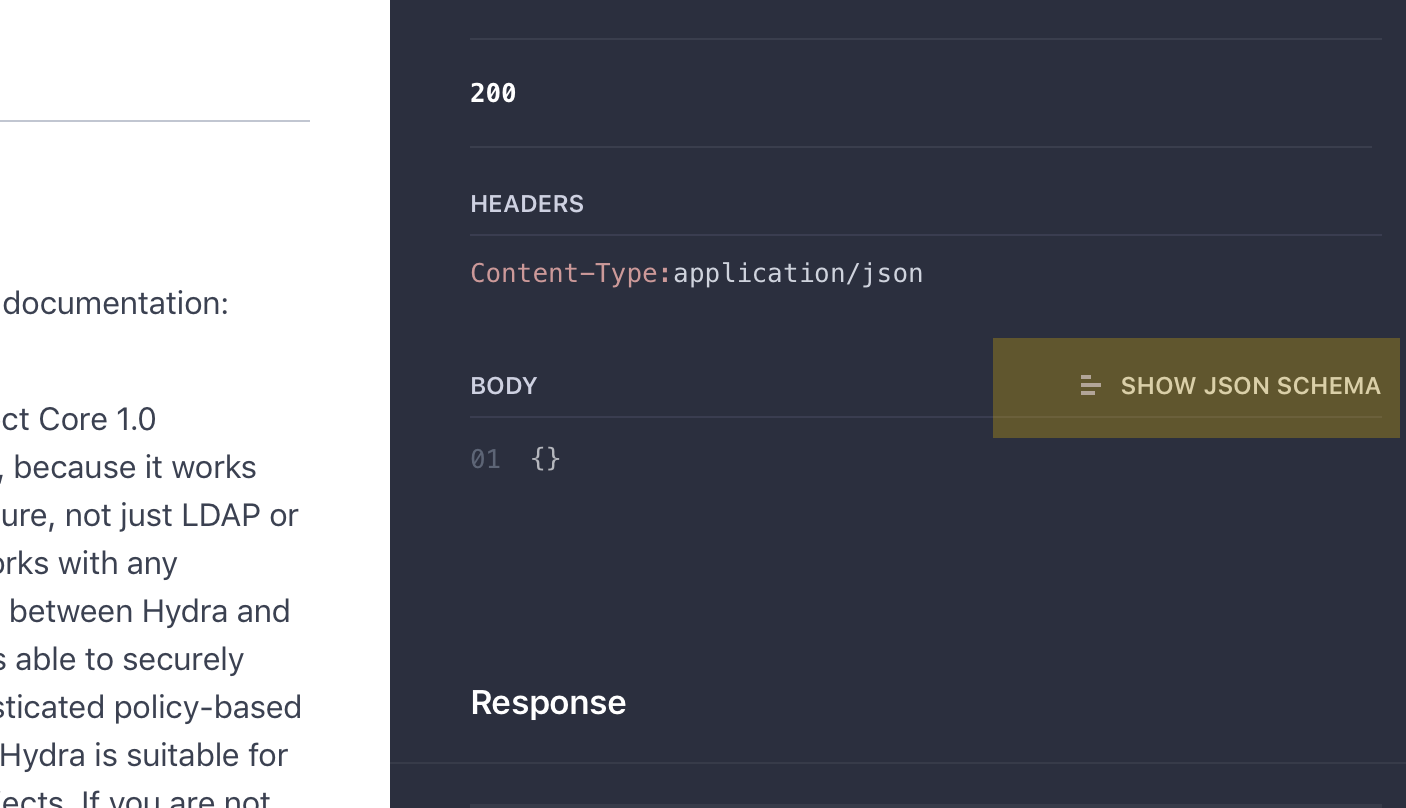 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequestPayload = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerOAuthConsentRequestPayload model module.
+ * @module model/SwaggerOAuthConsentRequestPayload
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerOAuthConsentRequestPayload
.
+ * @alias module:model/SwaggerOAuthConsentRequestPayload
+ * @class
+ * @param id {String} The id of the OAuth 2.0 Consent Request.
+ */
+ var exports = function(id) {
+ var _this = this;
+
+ _this['id'] = id;
+ };
+
+ /**
+ * Constructs a SwaggerOAuthConsentRequestPayload
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerOAuthConsentRequestPayload} obj Optional instance to populate.
+ * @return {module:model/SwaggerOAuthConsentRequestPayload} The populated SwaggerOAuthConsentRequestPayload
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The id of the OAuth 2.0 Consent Request.
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerOAuthIntrospectionRequest.js b/sdk/js/swagger/src/model/SwaggerOAuthIntrospectionRequest.js
new file mode 100644
index 00000000000..e66b79f9e9d
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerOAuthIntrospectionRequest.js
@@ -0,0 +1,93 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 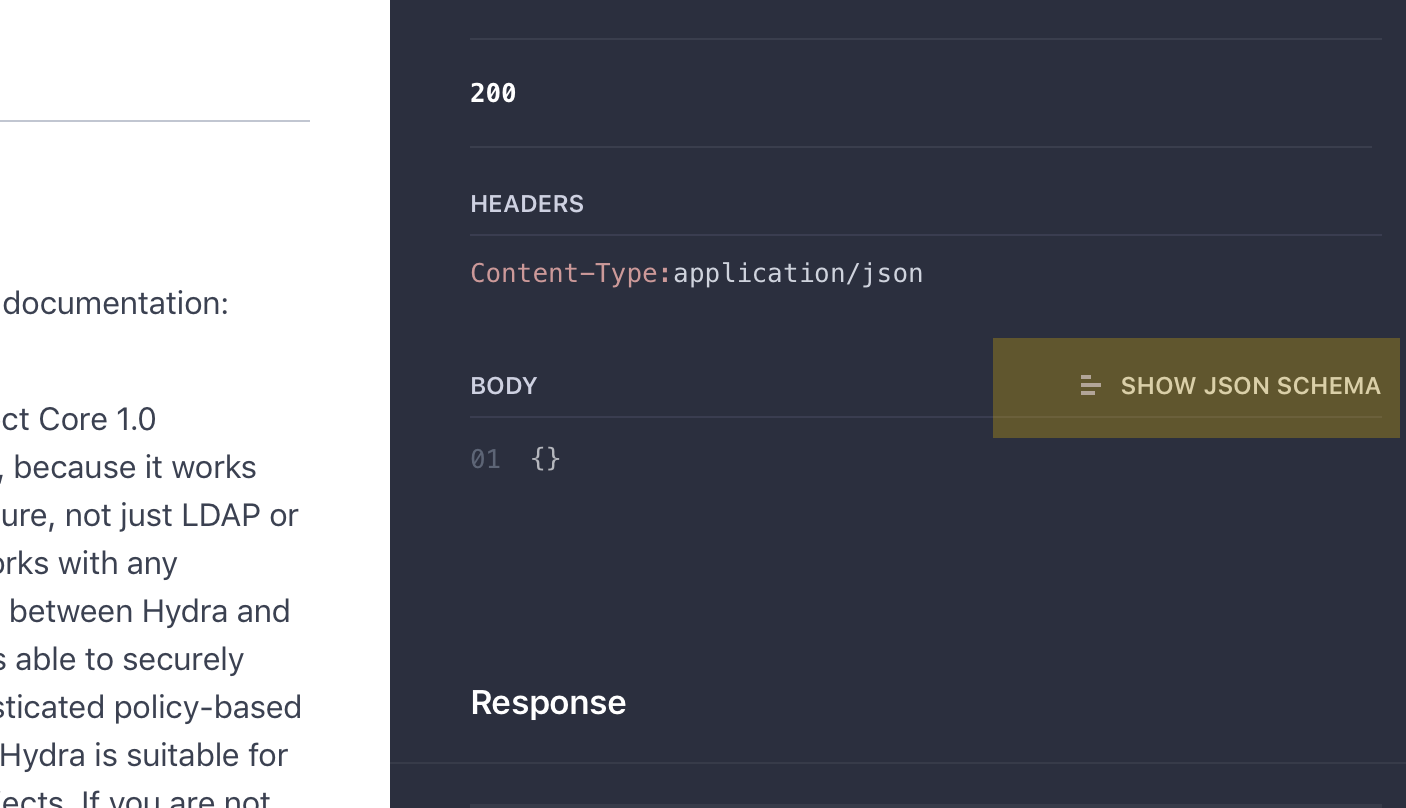 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerOAuthIntrospectionRequest model module.
+ * @module model/SwaggerOAuthIntrospectionRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerOAuthIntrospectionRequest
.
+ * @alias module:model/SwaggerOAuthIntrospectionRequest
+ * @class
+ * @param token {String} The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation.
+ */
+ var exports = function(token) {
+ var _this = this;
+
+
+ _this['token'] = token;
+ };
+
+ /**
+ * Constructs a SwaggerOAuthIntrospectionRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerOAuthIntrospectionRequest} obj Optional instance to populate.
+ * @return {module:model/SwaggerOAuthIntrospectionRequest} The populated SwaggerOAuthIntrospectionRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('scope')) {
+ obj['scope'] = ApiClient.convertToType(data['scope'], 'String');
+ }
+ if (data.hasOwnProperty('token')) {
+ obj['token'] = ApiClient.convertToType(data['token'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * An optional, space separated list of required scopes. If the access token was not granted one of the scopes, the result of active will be false. in: formData
+ * @member {String} scope
+ */
+ exports.prototype['scope'] = undefined;
+ /**
+ * The string value of the token. For access tokens, this is the \"access_token\" value returned from the token endpoint defined in OAuth 2.0 [RFC6749], Section 5.1. This endpoint DOES NOT accept refresh tokens for validation.
+ * @member {String} token
+ */
+ exports.prototype['token'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerOAuthIntrospectionResponse.js b/sdk/js/swagger/src/model/SwaggerOAuthIntrospectionResponse.js
new file mode 100644
index 00000000000..5b741f8c564
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerOAuthIntrospectionResponse.js
@@ -0,0 +1,83 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 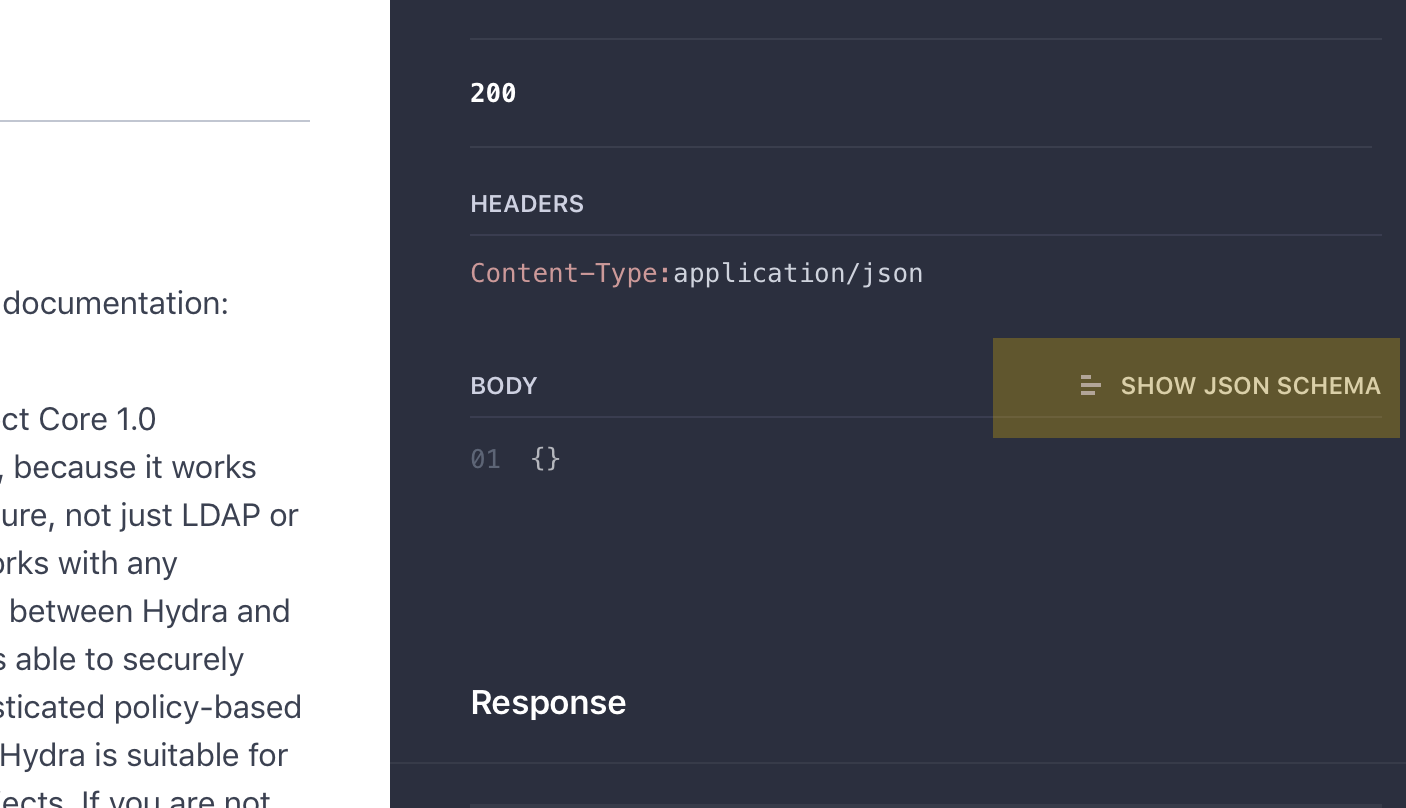 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/OAuth2TokenIntrospection'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./OAuth2TokenIntrospection'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionResponse = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection);
+ }
+}(this, function(ApiClient, OAuth2TokenIntrospection) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerOAuthIntrospectionResponse model module.
+ * @module model/SwaggerOAuthIntrospectionResponse
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerOAuthIntrospectionResponse
.
+ * The token introspection response
+ * @alias module:model/SwaggerOAuthIntrospectionResponse
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerOAuthIntrospectionResponse
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerOAuthIntrospectionResponse} obj Optional instance to populate.
+ * @return {module:model/SwaggerOAuthIntrospectionResponse} The populated SwaggerOAuthIntrospectionResponse
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = OAuth2TokenIntrospection.constructFromObject(data['Body']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/OAuth2TokenIntrospection} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerOAuthTokenResponse.js b/sdk/js/swagger/src/model/SwaggerOAuthTokenResponse.js
new file mode 100644
index 00000000000..1bc1510ff82
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerOAuthTokenResponse.js
@@ -0,0 +1,83 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 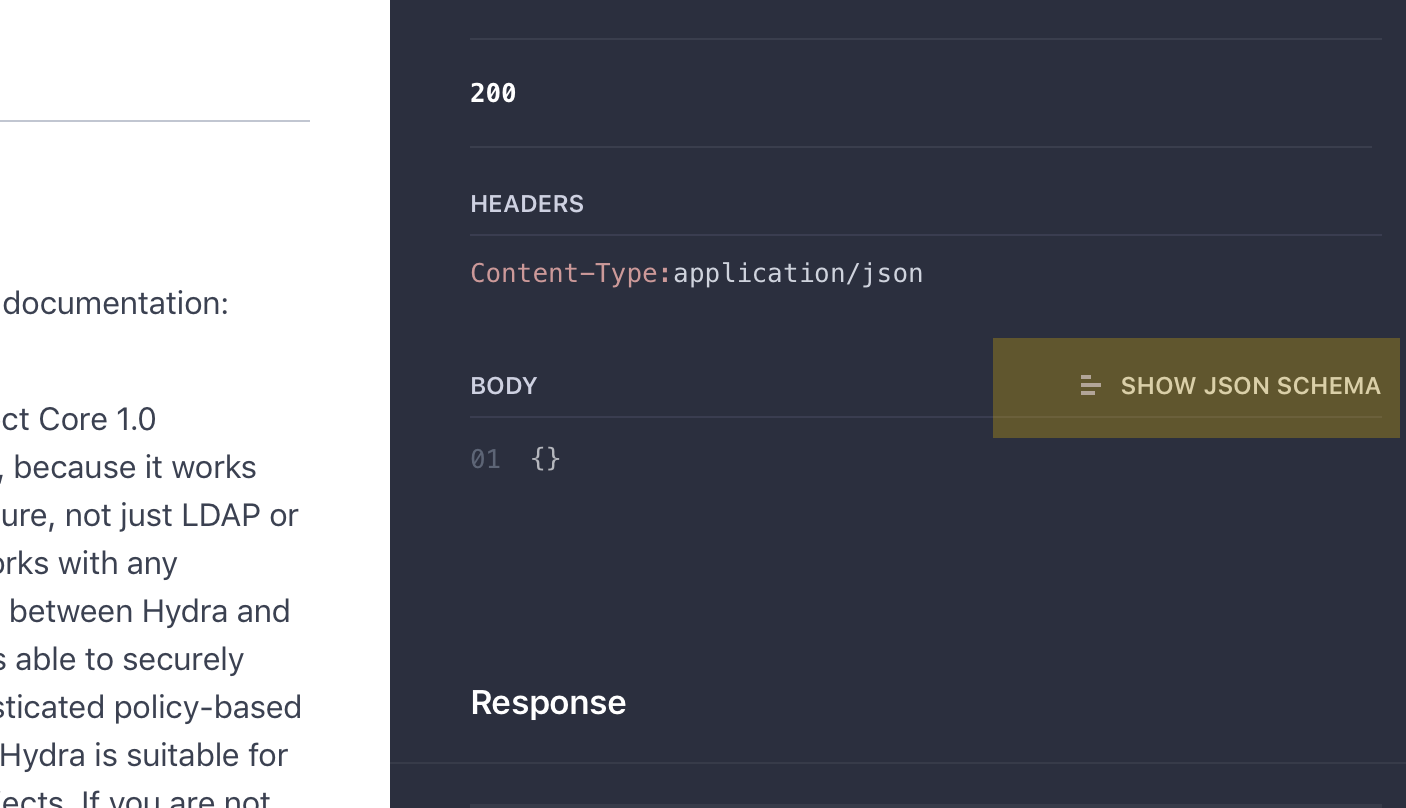 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/SwaggerOAuthTokenResponseBody'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./SwaggerOAuthTokenResponseBody'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponse = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody);
+ }
+}(this, function(ApiClient, SwaggerOAuthTokenResponseBody) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerOAuthTokenResponse model module.
+ * @module model/SwaggerOAuthTokenResponse
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerOAuthTokenResponse
.
+ * The token response
+ * @alias module:model/SwaggerOAuthTokenResponse
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerOAuthTokenResponse
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerOAuthTokenResponse} obj Optional instance to populate.
+ * @return {module:model/SwaggerOAuthTokenResponse} The populated SwaggerOAuthTokenResponse
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = SwaggerOAuthTokenResponseBody.constructFromObject(data['Body']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/SwaggerOAuthTokenResponseBody} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerOAuthTokenResponseBody.js b/sdk/js/swagger/src/model/SwaggerOAuthTokenResponseBody.js
new file mode 100644
index 00000000000..5b322d2f4d2
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerOAuthTokenResponseBody.js
@@ -0,0 +1,129 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 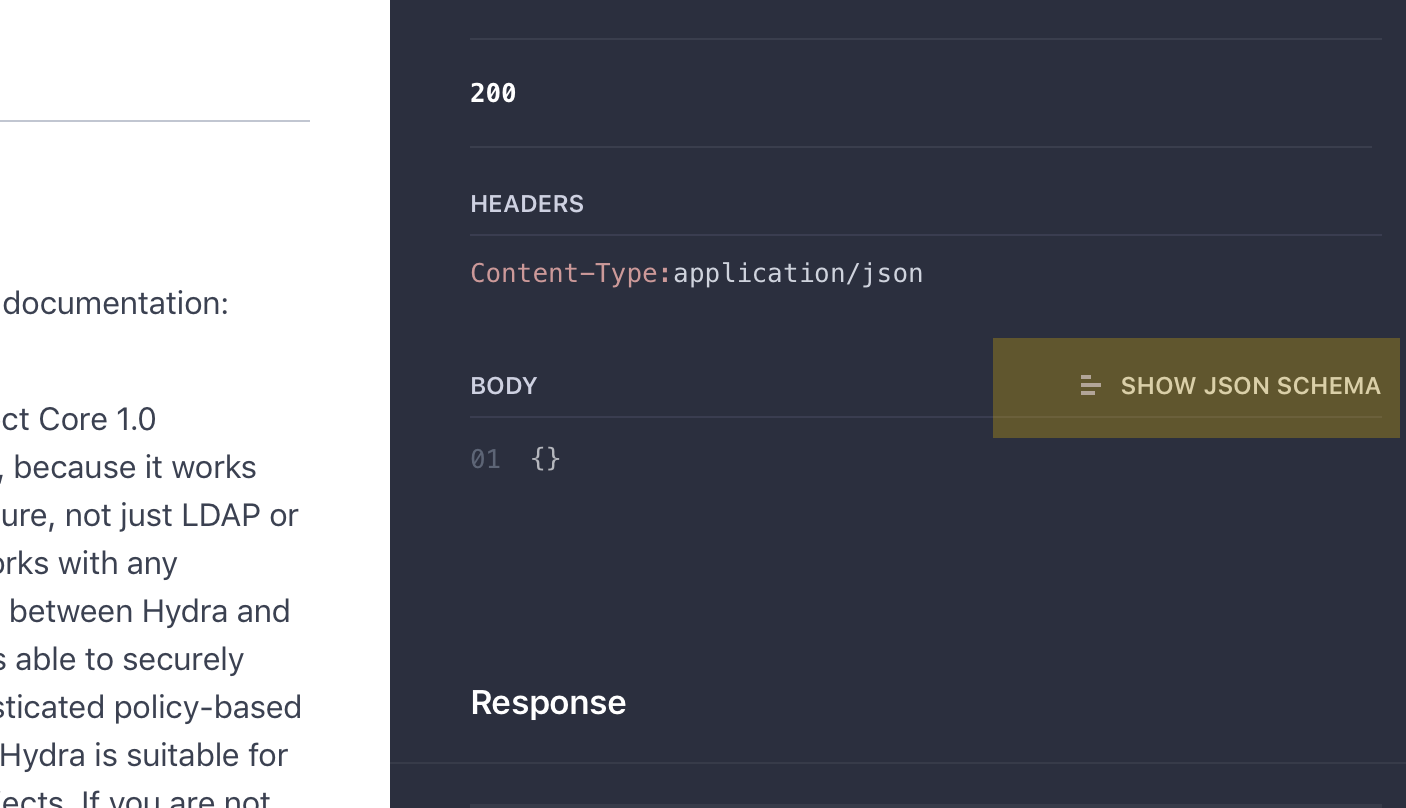 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerOAuthTokenResponseBody model module.
+ * @module model/SwaggerOAuthTokenResponseBody
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerOAuthTokenResponseBody
.
+ * in: body
+ * @alias module:model/SwaggerOAuthTokenResponseBody
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a SwaggerOAuthTokenResponseBody
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerOAuthTokenResponseBody} obj Optional instance to populate.
+ * @return {module:model/SwaggerOAuthTokenResponseBody} The populated SwaggerOAuthTokenResponseBody
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('access_token')) {
+ obj['access_token'] = ApiClient.convertToType(data['access_token'], 'String');
+ }
+ if (data.hasOwnProperty('expires_in')) {
+ obj['expires_in'] = ApiClient.convertToType(data['expires_in'], 'Number');
+ }
+ if (data.hasOwnProperty('id_token')) {
+ obj['id_token'] = ApiClient.convertToType(data['id_token'], 'Number');
+ }
+ if (data.hasOwnProperty('refresh_token')) {
+ obj['refresh_token'] = ApiClient.convertToType(data['refresh_token'], 'String');
+ }
+ if (data.hasOwnProperty('scope')) {
+ obj['scope'] = ApiClient.convertToType(data['scope'], 'Number');
+ }
+ if (data.hasOwnProperty('token_type')) {
+ obj['token_type'] = ApiClient.convertToType(data['token_type'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * The access token issued by the authorization server.
+ * @member {String} access_token
+ */
+ exports.prototype['access_token'] = undefined;
+ /**
+ * The lifetime in seconds of the access token. For example, the value \"3600\" denotes that the access token will expire in one hour from the time the response was generated.
+ * @member {Number} expires_in
+ */
+ exports.prototype['expires_in'] = undefined;
+ /**
+ * To retrieve a refresh token request the id_token scope.
+ * @member {Number} id_token
+ */
+ exports.prototype['id_token'] = undefined;
+ /**
+ * The refresh token, which can be used to obtain new access tokens. To retrieve it add the scope \"offline\" to your access token request.
+ * @member {String} refresh_token
+ */
+ exports.prototype['refresh_token'] = undefined;
+ /**
+ * The scope of the access token
+ * @member {Number} scope
+ */
+ exports.prototype['scope'] = undefined;
+ /**
+ * The type of the token issued
+ * @member {String} token_type
+ */
+ exports.prototype['token_type'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerRejectConsentRequest.js b/sdk/js/swagger/src/model/SwaggerRejectConsentRequest.js
new file mode 100644
index 00000000000..0a6da0188bf
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerRejectConsentRequest.js
@@ -0,0 +1,93 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 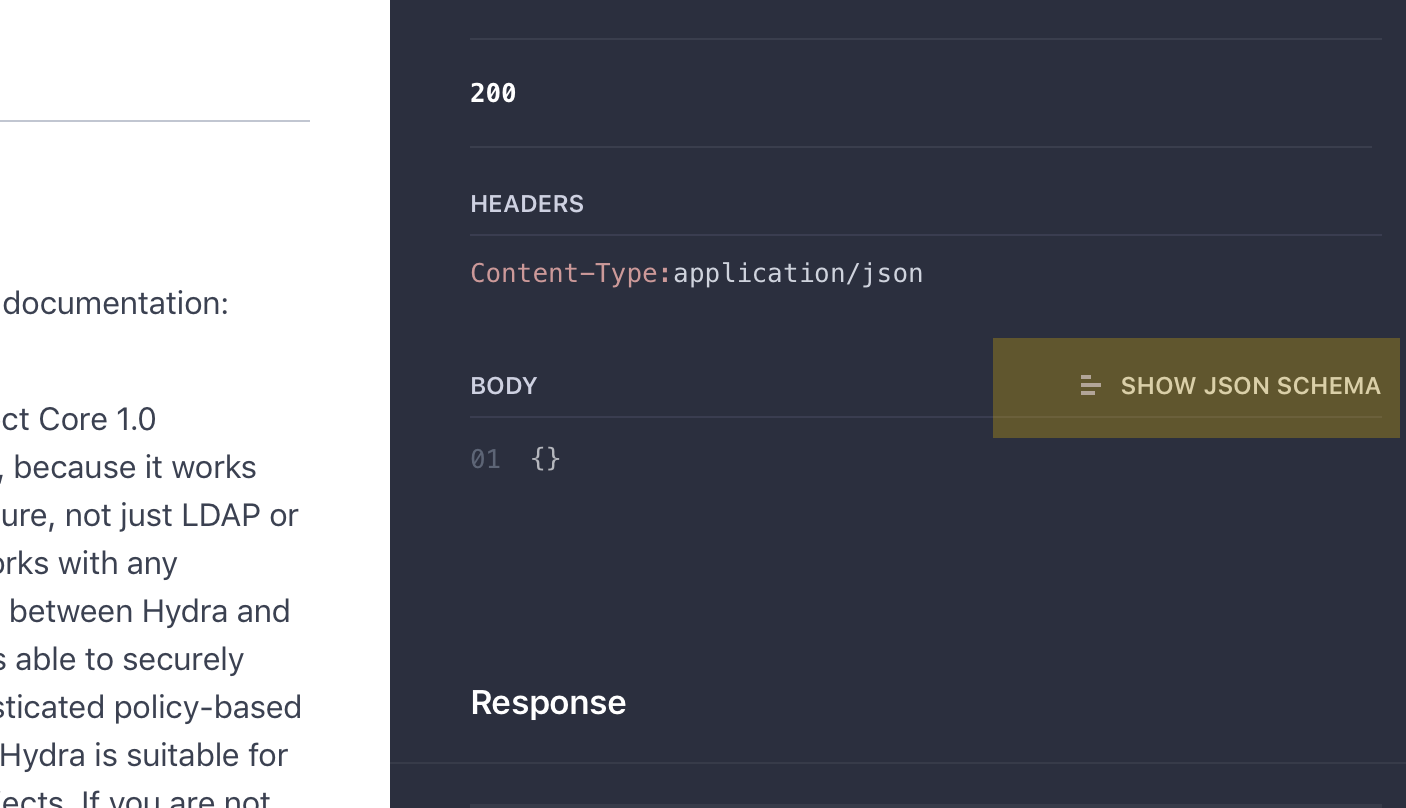 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/ConsentRequestRejection'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./ConsentRequestRejection'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerRejectConsentRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.ConsentRequestRejection);
+ }
+}(this, function(ApiClient, ConsentRequestRejection) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerRejectConsentRequest model module.
+ * @module model/SwaggerRejectConsentRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerRejectConsentRequest
.
+ * @alias module:model/SwaggerRejectConsentRequest
+ * @class
+ * @param body {module:model/ConsentRequestRejection}
+ * @param id {String} in: path
+ */
+ var exports = function(body, id) {
+ var _this = this;
+
+ _this['Body'] = body;
+ _this['id'] = id;
+ };
+
+ /**
+ * Constructs a SwaggerRejectConsentRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerRejectConsentRequest} obj Optional instance to populate.
+ * @return {module:model/SwaggerRejectConsentRequest} The populated SwaggerRejectConsentRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = ConsentRequestRejection.constructFromObject(data['Body']);
+ }
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/ConsentRequestRejection} Body
+ */
+ exports.prototype['Body'] = undefined;
+ /**
+ * in: path
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerRevokeOAuth2TokenParameters.js b/sdk/js/swagger/src/model/SwaggerRevokeOAuth2TokenParameters.js
new file mode 100644
index 00000000000..ba67b757d2c
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerRevokeOAuth2TokenParameters.js
@@ -0,0 +1,84 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 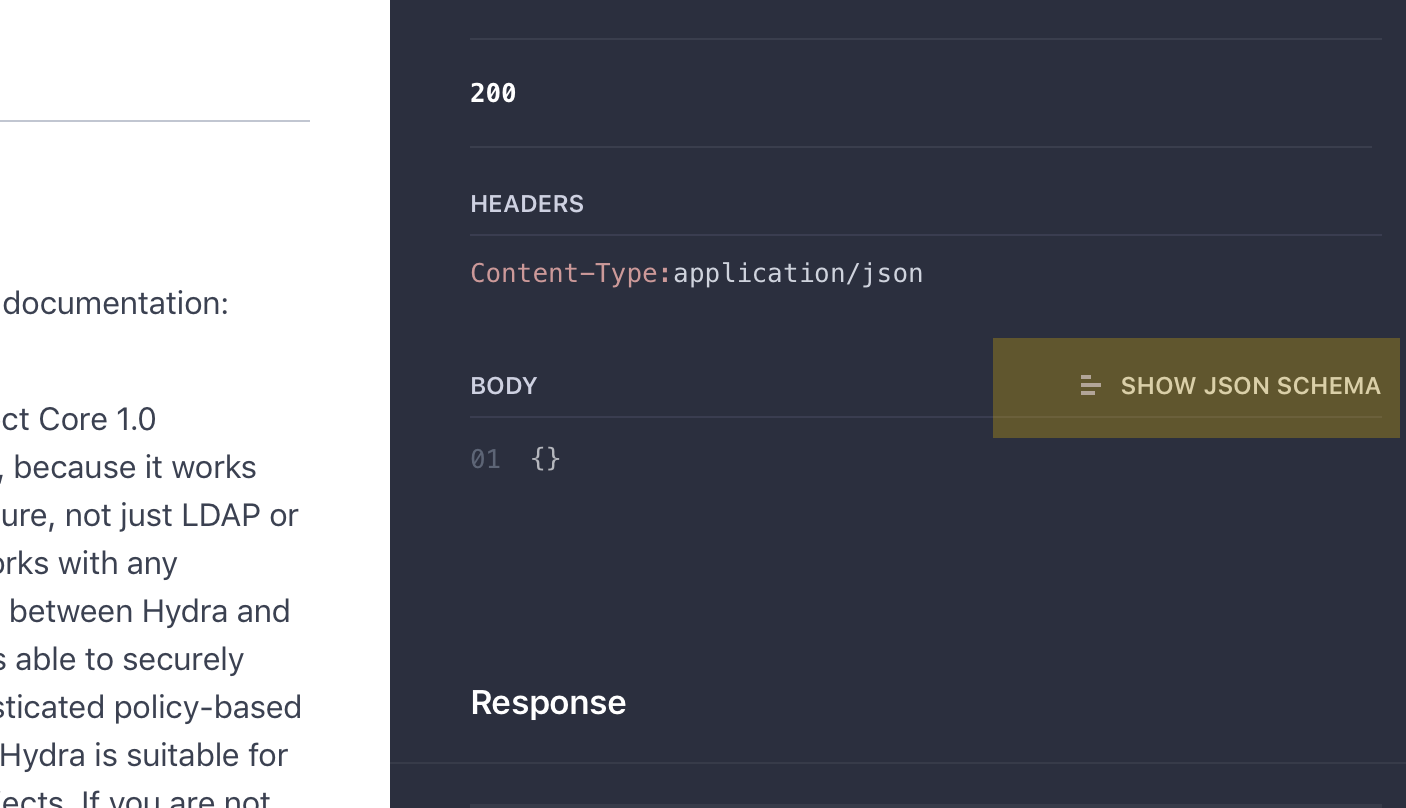 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerRevokeOAuth2TokenParameters = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerRevokeOAuth2TokenParameters model module.
+ * @module model/SwaggerRevokeOAuth2TokenParameters
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerRevokeOAuth2TokenParameters
.
+ * @alias module:model/SwaggerRevokeOAuth2TokenParameters
+ * @class
+ * @param token {String} in: formData
+ */
+ var exports = function(token) {
+ var _this = this;
+
+ _this['token'] = token;
+ };
+
+ /**
+ * Constructs a SwaggerRevokeOAuth2TokenParameters
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerRevokeOAuth2TokenParameters} obj Optional instance to populate.
+ * @return {module:model/SwaggerRevokeOAuth2TokenParameters} The populated SwaggerRevokeOAuth2TokenParameters
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('token')) {
+ obj['token'] = ApiClient.convertToType(data['token'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * in: formData
+ * @member {String} token
+ */
+ exports.prototype['token'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerUpdatePolicyParameters.js b/sdk/js/swagger/src/model/SwaggerUpdatePolicyParameters.js
new file mode 100644
index 00000000000..6160030a70c
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerUpdatePolicyParameters.js
@@ -0,0 +1,91 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 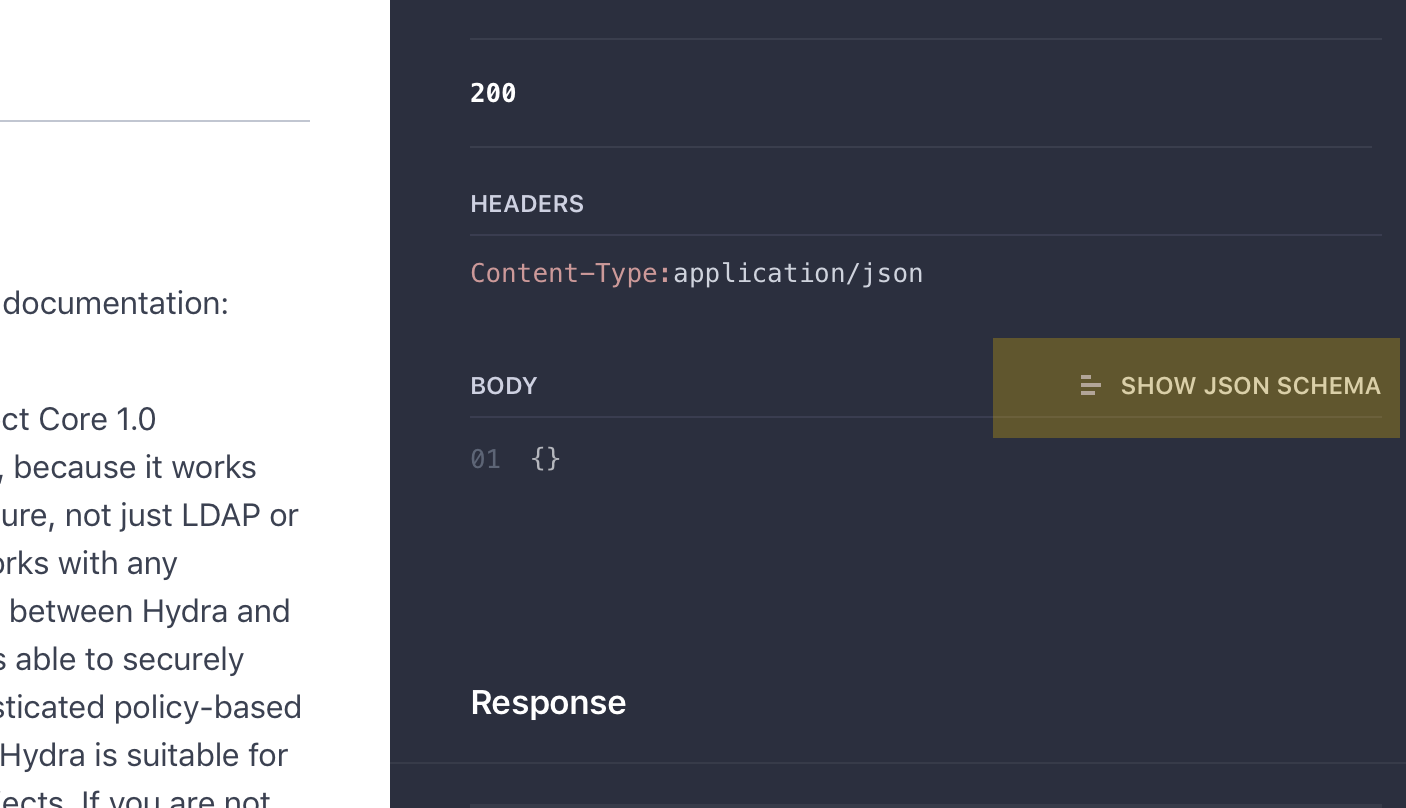 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/Policy'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./Policy'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerUpdatePolicyParameters = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.Policy);
+ }
+}(this, function(ApiClient, Policy) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerUpdatePolicyParameters model module.
+ * @module model/SwaggerUpdatePolicyParameters
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerUpdatePolicyParameters
.
+ * @alias module:model/SwaggerUpdatePolicyParameters
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+ };
+
+ /**
+ * Constructs a SwaggerUpdatePolicyParameters
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerUpdatePolicyParameters} obj Optional instance to populate.
+ * @return {module:model/SwaggerUpdatePolicyParameters} The populated SwaggerUpdatePolicyParameters
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = Policy.constructFromObject(data['Body']);
+ }
+ if (data.hasOwnProperty('id')) {
+ obj['id'] = ApiClient.convertToType(data['id'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/Policy} Body
+ */
+ exports.prototype['Body'] = undefined;
+ /**
+ * The id of the policy. in: path
+ * @member {String} id
+ */
+ exports.prototype['id'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerWardenAccessRequestResponseParameters.js b/sdk/js/swagger/src/model/SwaggerWardenAccessRequestResponseParameters.js
new file mode 100644
index 00000000000..714efeb8654
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerWardenAccessRequestResponseParameters.js
@@ -0,0 +1,82 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 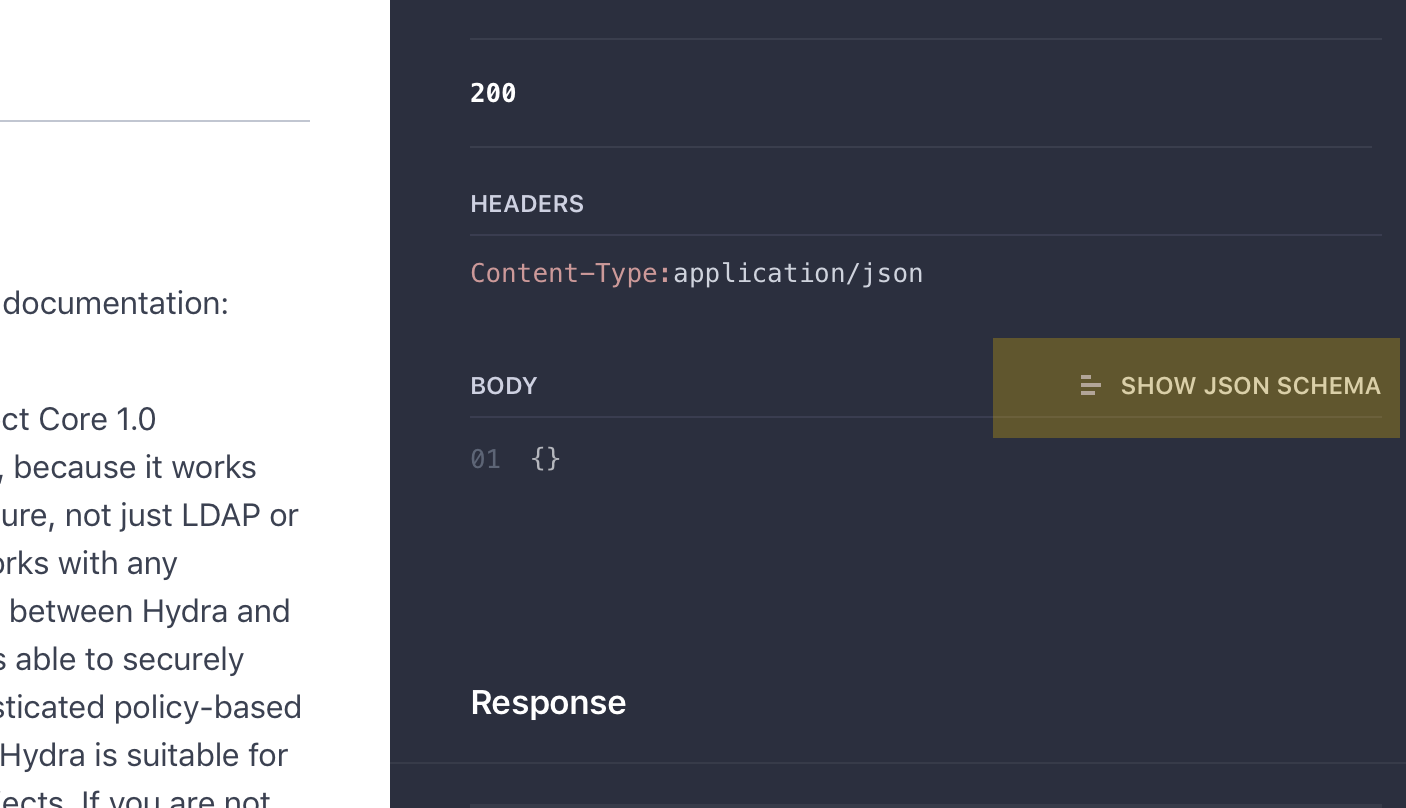 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/WardenAccessRequestResponse'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./WardenAccessRequestResponse'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerWardenAccessRequestResponseParameters = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse);
+ }
+}(this, function(ApiClient, WardenAccessRequestResponse) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerWardenAccessRequestResponseParameters model module.
+ * @module model/SwaggerWardenAccessRequestResponseParameters
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerWardenAccessRequestResponseParameters
.
+ * @alias module:model/SwaggerWardenAccessRequestResponseParameters
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerWardenAccessRequestResponseParameters
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerWardenAccessRequestResponseParameters} obj Optional instance to populate.
+ * @return {module:model/SwaggerWardenAccessRequestResponseParameters} The populated SwaggerWardenAccessRequestResponseParameters
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = WardenAccessRequestResponse.constructFromObject(data['Body']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/WardenAccessRequestResponse} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/SwaggerWardenTokenAccessRequestResponse.js b/sdk/js/swagger/src/model/SwaggerWardenTokenAccessRequestResponse.js
new file mode 100644
index 00000000000..e935b05ce7d
--- /dev/null
+++ b/sdk/js/swagger/src/model/SwaggerWardenTokenAccessRequestResponse.js
@@ -0,0 +1,82 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 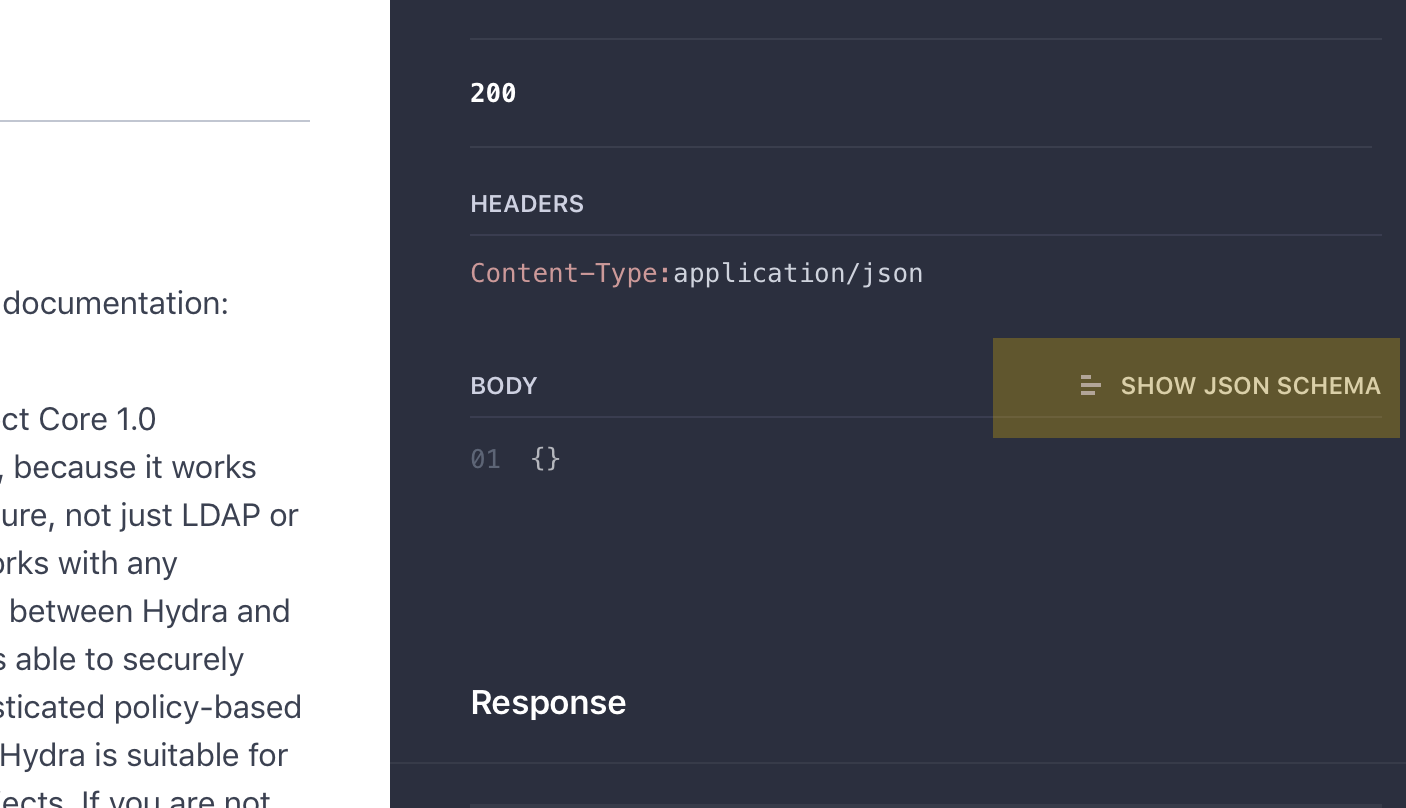 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient', 'model/WardenTokenAccessRequestResponsePayload'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'), require('./WardenTokenAccessRequestResponsePayload'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.SwaggerWardenTokenAccessRequestResponse = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient, root.HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload);
+ }
+}(this, function(ApiClient, WardenTokenAccessRequestResponsePayload) {
+ 'use strict';
+
+
+
+
+ /**
+ * The SwaggerWardenTokenAccessRequestResponse model module.
+ * @module model/SwaggerWardenTokenAccessRequestResponse
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new SwaggerWardenTokenAccessRequestResponse
.
+ * @alias module:model/SwaggerWardenTokenAccessRequestResponse
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a SwaggerWardenTokenAccessRequestResponse
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/SwaggerWardenTokenAccessRequestResponse} obj Optional instance to populate.
+ * @return {module:model/SwaggerWardenTokenAccessRequestResponse} The populated SwaggerWardenTokenAccessRequestResponse
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('Body')) {
+ obj['Body'] = WardenTokenAccessRequestResponsePayload.constructFromObject(data['Body']);
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * @member {module:model/WardenTokenAccessRequestResponsePayload} Body
+ */
+ exports.prototype['Body'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/TokenAllowedRequest.js b/sdk/js/swagger/src/model/TokenAllowedRequest.js
new file mode 100644
index 00000000000..86d0d9d4aa2
--- /dev/null
+++ b/sdk/js/swagger/src/model/TokenAllowedRequest.js
@@ -0,0 +1,101 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 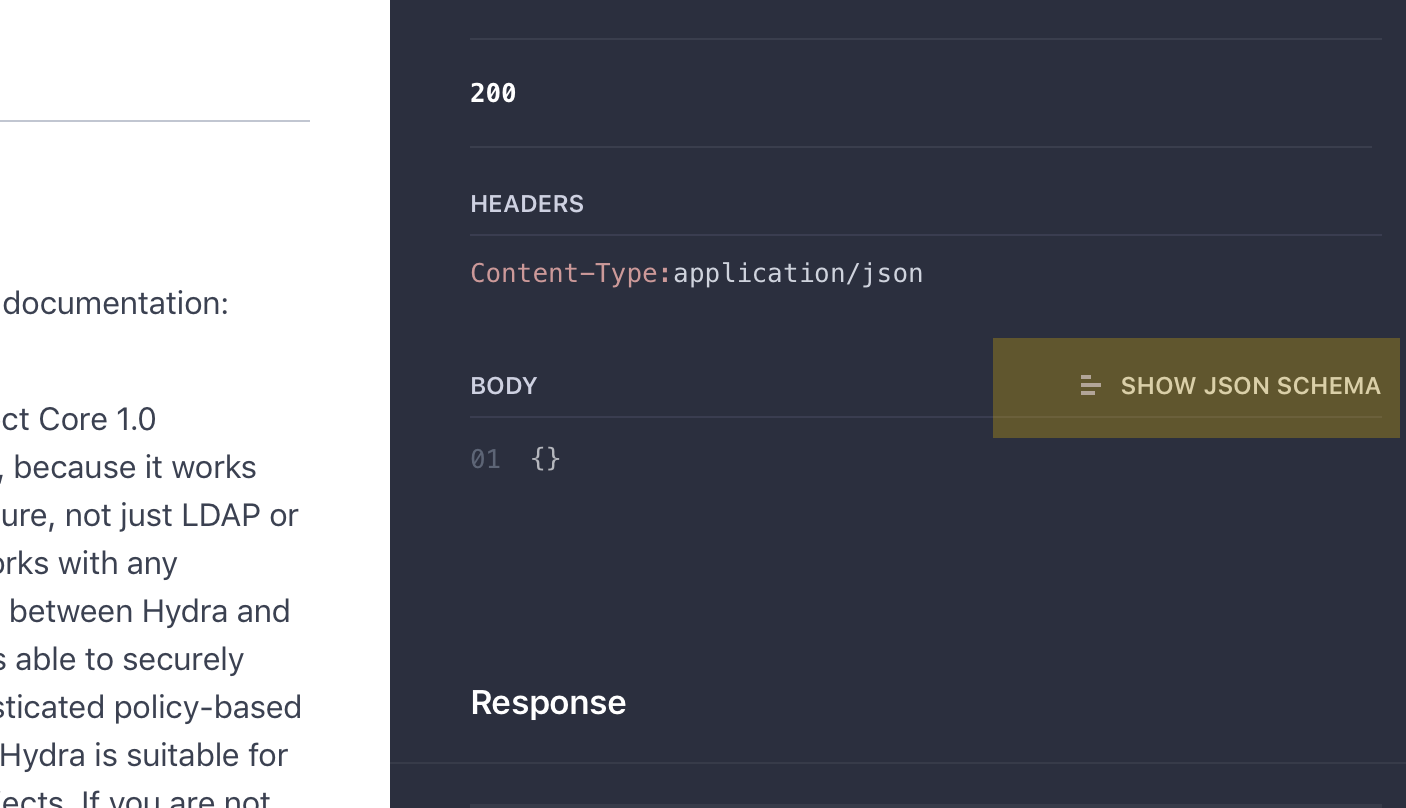 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.TokenAllowedRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The TokenAllowedRequest model module.
+ * @module model/TokenAllowedRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new TokenAllowedRequest
.
+ * @alias module:model/TokenAllowedRequest
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+ };
+
+ /**
+ * Constructs a TokenAllowedRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/TokenAllowedRequest} obj Optional instance to populate.
+ * @return {module:model/TokenAllowedRequest} The populated TokenAllowedRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('action')) {
+ obj['action'] = ApiClient.convertToType(data['action'], 'String');
+ }
+ if (data.hasOwnProperty('context')) {
+ obj['context'] = ApiClient.convertToType(data['context'], {'String': Object});
+ }
+ if (data.hasOwnProperty('resource')) {
+ obj['resource'] = ApiClient.convertToType(data['resource'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Action is the action that is requested on the resource.
+ * @member {String} action
+ */
+ exports.prototype['action'] = undefined;
+ /**
+ * Context is the request's environmental context.
+ * @member {Object.} context
+ */
+ exports.prototype['context'] = undefined;
+ /**
+ * Resource is the resource that access is requested to.
+ * @member {String} resource
+ */
+ exports.prototype['resource'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/WardenAccessRequest.js b/sdk/js/swagger/src/model/WardenAccessRequest.js
new file mode 100644
index 00000000000..37c73cc2098
--- /dev/null
+++ b/sdk/js/swagger/src/model/WardenAccessRequest.js
@@ -0,0 +1,110 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 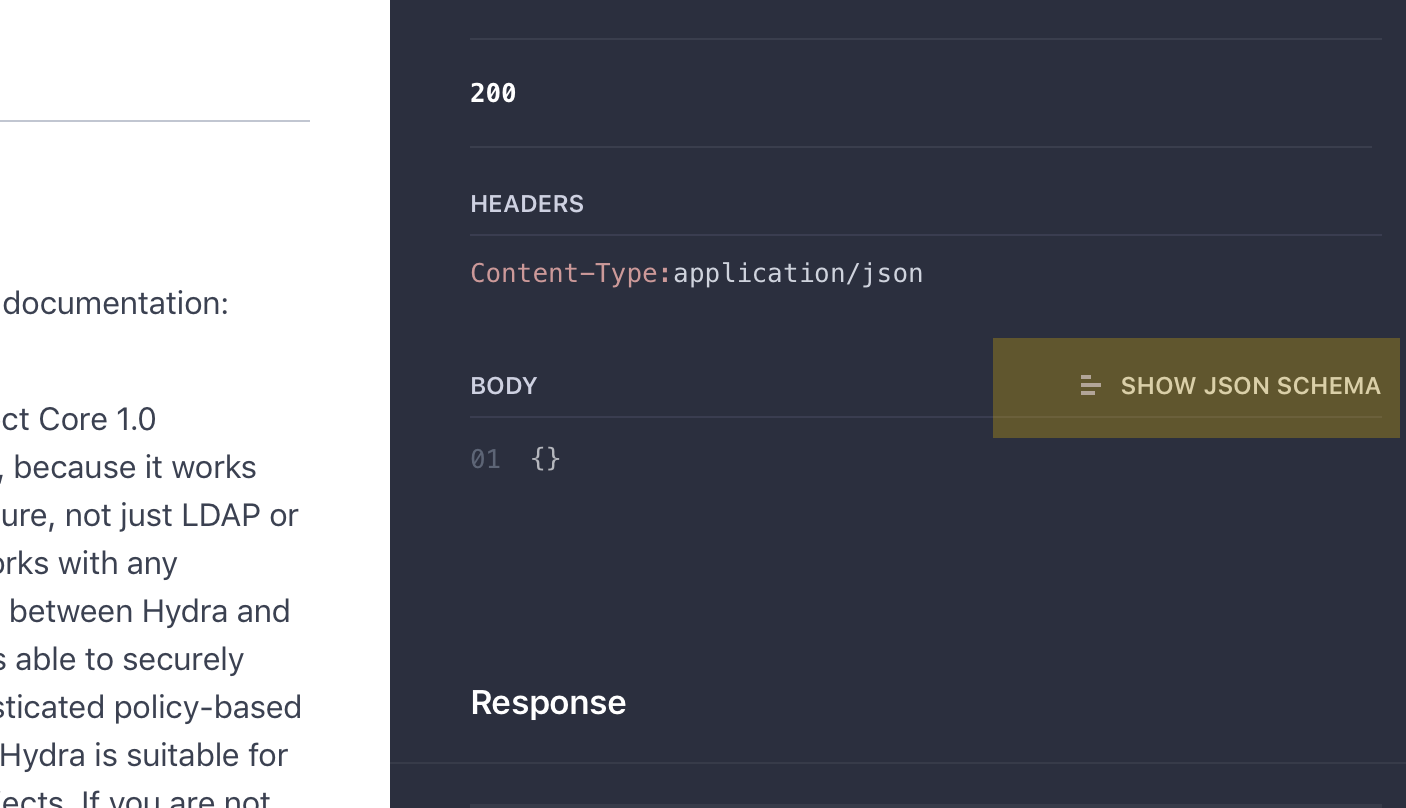 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.WardenAccessRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The WardenAccessRequest model module.
+ * @module model/WardenAccessRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new WardenAccessRequest
.
+ * @alias module:model/WardenAccessRequest
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a WardenAccessRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/WardenAccessRequest} obj Optional instance to populate.
+ * @return {module:model/WardenAccessRequest} The populated WardenAccessRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('action')) {
+ obj['action'] = ApiClient.convertToType(data['action'], 'String');
+ }
+ if (data.hasOwnProperty('context')) {
+ obj['context'] = ApiClient.convertToType(data['context'], {'String': Object});
+ }
+ if (data.hasOwnProperty('resource')) {
+ obj['resource'] = ApiClient.convertToType(data['resource'], 'String');
+ }
+ if (data.hasOwnProperty('subject')) {
+ obj['subject'] = ApiClient.convertToType(data['subject'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Action is the action that is requested on the resource.
+ * @member {String} action
+ */
+ exports.prototype['action'] = undefined;
+ /**
+ * Context is the request's environmental context.
+ * @member {Object.} context
+ */
+ exports.prototype['context'] = undefined;
+ /**
+ * Resource is the resource that access is requested to.
+ * @member {String} resource
+ */
+ exports.prototype['resource'] = undefined;
+ /**
+ * Subejct is the subject that is requesting access.
+ * @member {String} subject
+ */
+ exports.prototype['subject'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/WardenAccessRequestResponse.js b/sdk/js/swagger/src/model/WardenAccessRequestResponse.js
new file mode 100644
index 00000000000..78b9385ad75
--- /dev/null
+++ b/sdk/js/swagger/src/model/WardenAccessRequestResponse.js
@@ -0,0 +1,83 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 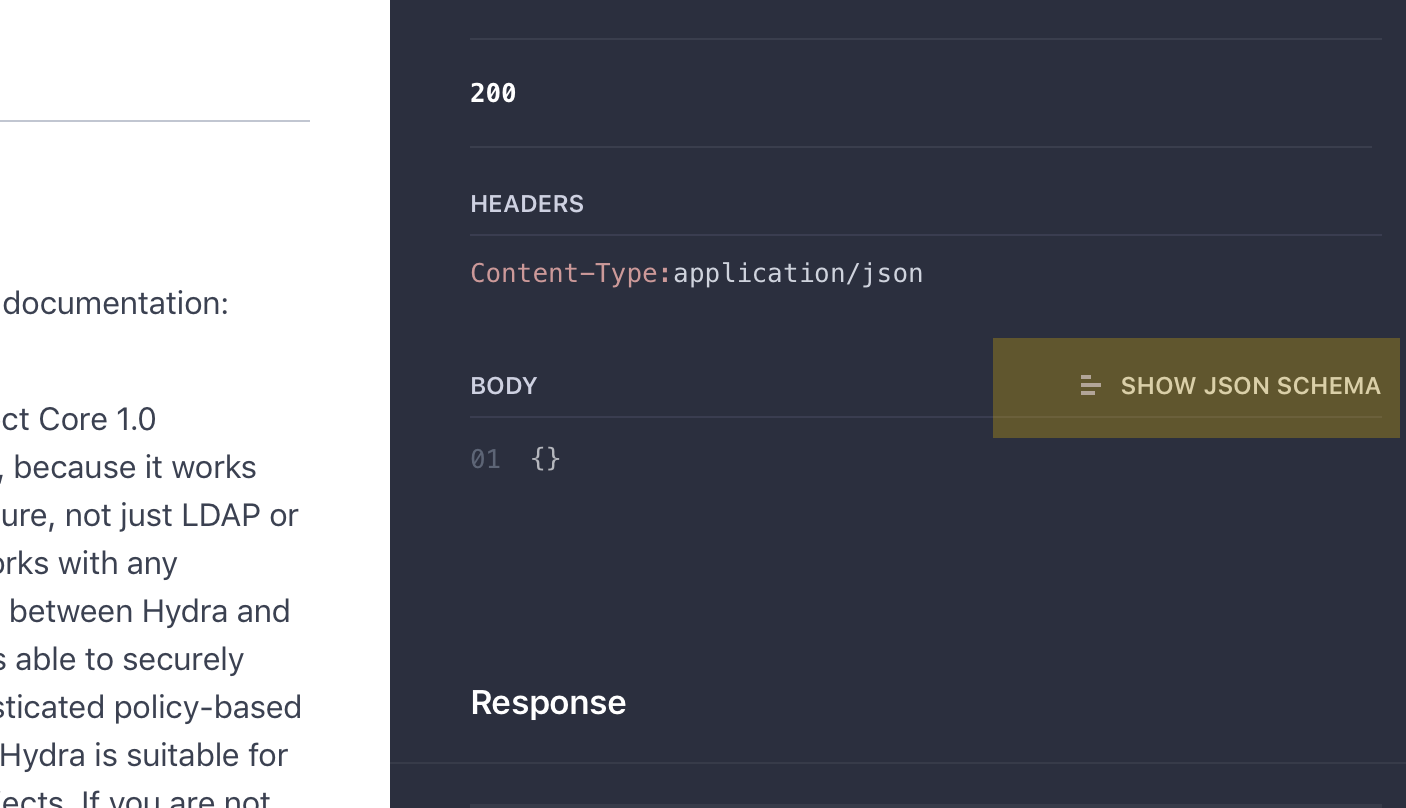 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The WardenAccessRequestResponse model module.
+ * @module model/WardenAccessRequestResponse
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new WardenAccessRequestResponse
.
+ * @alias module:model/WardenAccessRequestResponse
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+ };
+
+ /**
+ * Constructs a WardenAccessRequestResponse
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/WardenAccessRequestResponse} obj Optional instance to populate.
+ * @return {module:model/WardenAccessRequestResponse} The populated WardenAccessRequestResponse
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('allowed')) {
+ obj['allowed'] = ApiClient.convertToType(data['allowed'], 'Boolean');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Allowed is true if the request is allowed and false otherwise.
+ * @member {Boolean} allowed
+ */
+ exports.prototype['allowed'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/WardenTokenAccessRequest.js b/sdk/js/swagger/src/model/WardenTokenAccessRequest.js
new file mode 100644
index 00000000000..09ea4ca4164
--- /dev/null
+++ b/sdk/js/swagger/src/model/WardenTokenAccessRequest.js
@@ -0,0 +1,119 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 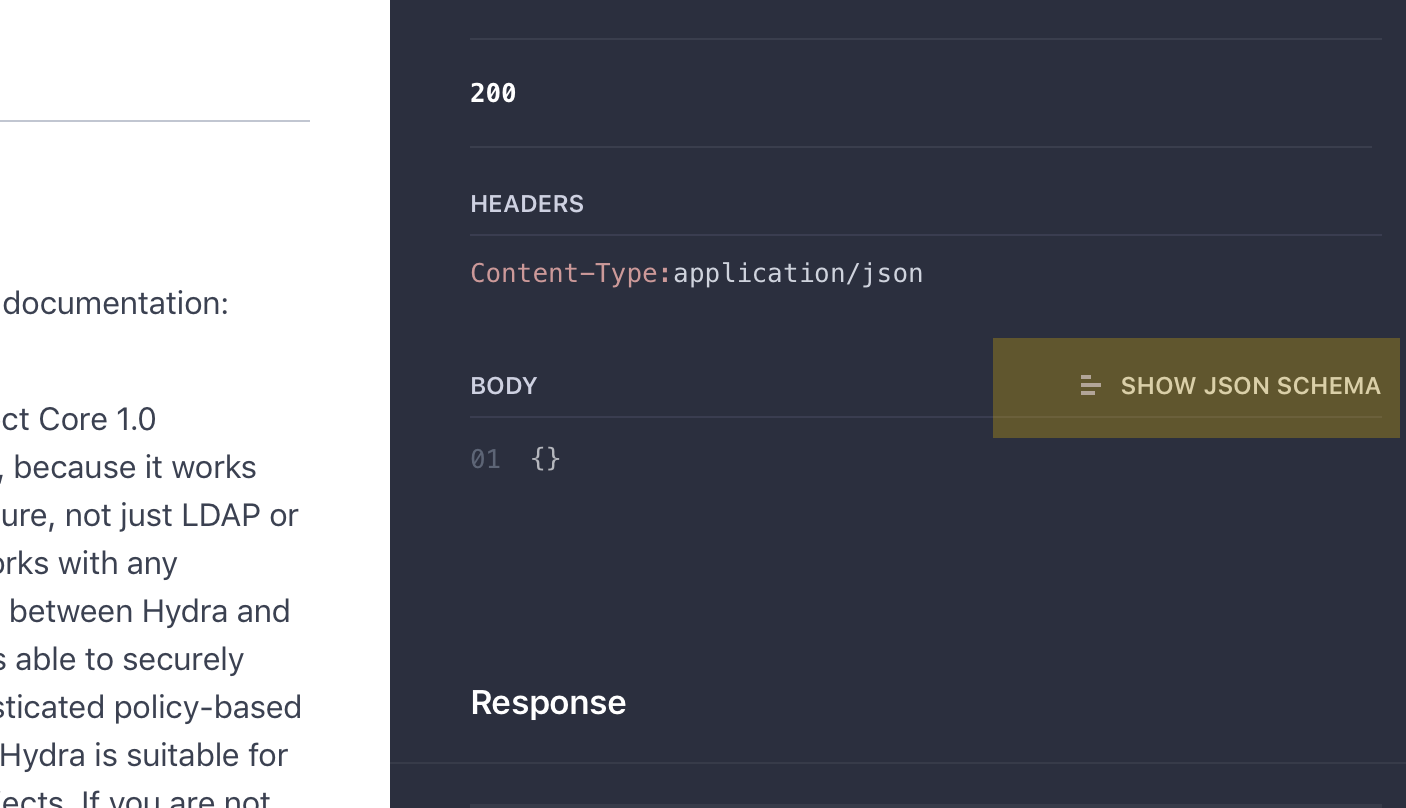 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The WardenTokenAccessRequest model module.
+ * @module model/WardenTokenAccessRequest
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new WardenTokenAccessRequest
.
+ * @alias module:model/WardenTokenAccessRequest
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a WardenTokenAccessRequest
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/WardenTokenAccessRequest} obj Optional instance to populate.
+ * @return {module:model/WardenTokenAccessRequest} The populated WardenTokenAccessRequest
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('action')) {
+ obj['action'] = ApiClient.convertToType(data['action'], 'String');
+ }
+ if (data.hasOwnProperty('context')) {
+ obj['context'] = ApiClient.convertToType(data['context'], {'String': Object});
+ }
+ if (data.hasOwnProperty('resource')) {
+ obj['resource'] = ApiClient.convertToType(data['resource'], 'String');
+ }
+ if (data.hasOwnProperty('scopes')) {
+ obj['scopes'] = ApiClient.convertToType(data['scopes'], ['String']);
+ }
+ if (data.hasOwnProperty('token')) {
+ obj['token'] = ApiClient.convertToType(data['token'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Action is the action that is requested on the resource.
+ * @member {String} action
+ */
+ exports.prototype['action'] = undefined;
+ /**
+ * Context is the request's environmental context.
+ * @member {Object.} context
+ */
+ exports.prototype['context'] = undefined;
+ /**
+ * Resource is the resource that access is requested to.
+ * @member {String} resource
+ */
+ exports.prototype['resource'] = undefined;
+ /**
+ * Scopes is an array of scopes that are requried.
+ * @member {Array.} scopes
+ */
+ exports.prototype['scopes'] = undefined;
+ /**
+ * Token is the token to introspect.
+ * @member {String} token
+ */
+ exports.prototype['token'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/WardenTokenAccessRequestResponsePayload.js b/sdk/js/swagger/src/model/WardenTokenAccessRequestResponsePayload.js
new file mode 100644
index 00000000000..808f5e9ade5
--- /dev/null
+++ b/sdk/js/swagger/src/model/WardenTokenAccessRequestResponsePayload.js
@@ -0,0 +1,146 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 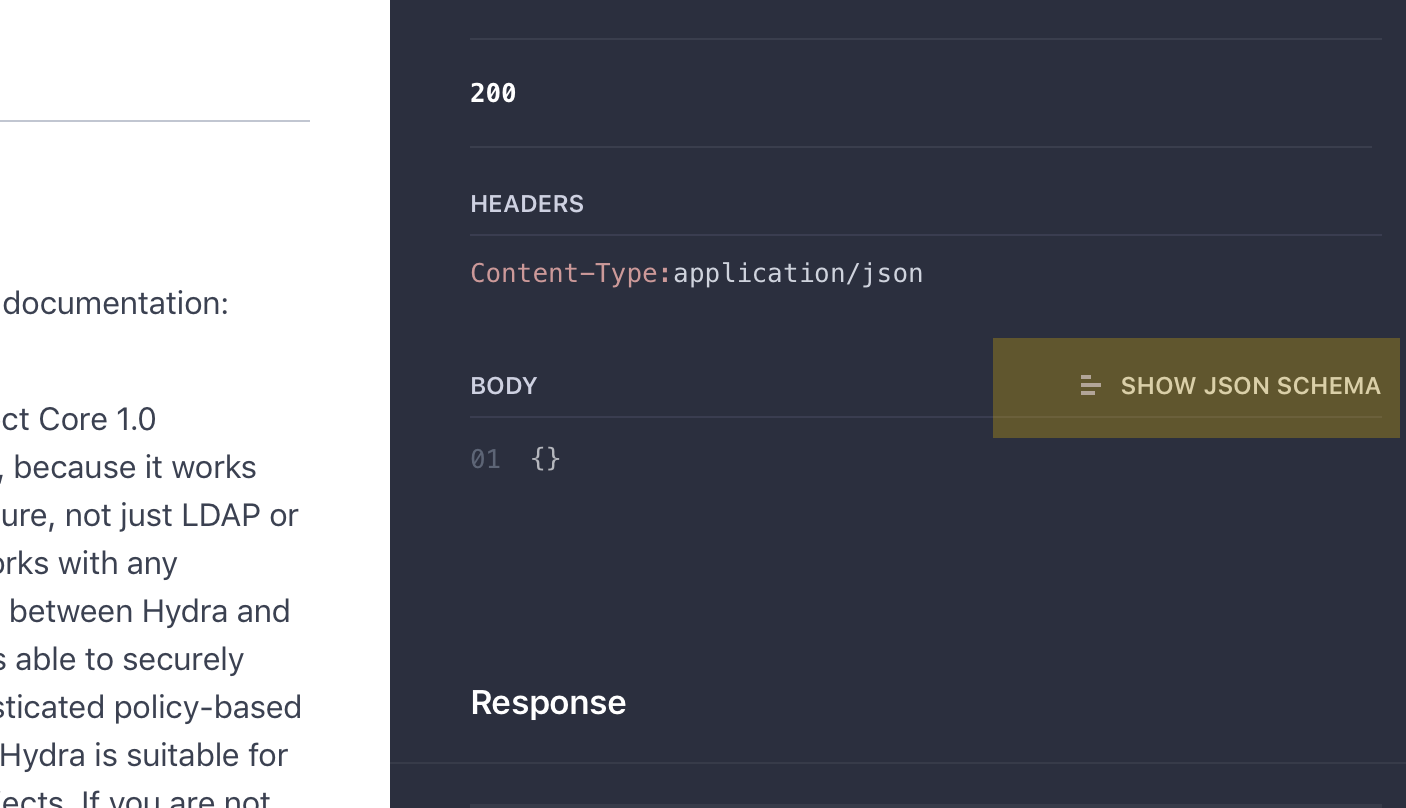 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The WardenTokenAccessRequestResponsePayload model module.
+ * @module model/WardenTokenAccessRequestResponsePayload
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new WardenTokenAccessRequestResponsePayload
.
+ * @alias module:model/WardenTokenAccessRequestResponsePayload
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+
+
+
+
+
+
+
+
+ };
+
+ /**
+ * Constructs a WardenTokenAccessRequestResponsePayload
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/WardenTokenAccessRequestResponsePayload} obj Optional instance to populate.
+ * @return {module:model/WardenTokenAccessRequestResponsePayload} The populated WardenTokenAccessRequestResponsePayload
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('allowed')) {
+ obj['allowed'] = ApiClient.convertToType(data['allowed'], 'Boolean');
+ }
+ if (data.hasOwnProperty('aud')) {
+ obj['aud'] = ApiClient.convertToType(data['aud'], 'String');
+ }
+ if (data.hasOwnProperty('exp')) {
+ obj['exp'] = ApiClient.convertToType(data['exp'], 'String');
+ }
+ if (data.hasOwnProperty('ext')) {
+ obj['ext'] = ApiClient.convertToType(data['ext'], {'String': Object});
+ }
+ if (data.hasOwnProperty('iat')) {
+ obj['iat'] = ApiClient.convertToType(data['iat'], 'String');
+ }
+ if (data.hasOwnProperty('iss')) {
+ obj['iss'] = ApiClient.convertToType(data['iss'], 'String');
+ }
+ if (data.hasOwnProperty('scopes')) {
+ obj['scopes'] = ApiClient.convertToType(data['scopes'], ['String']);
+ }
+ if (data.hasOwnProperty('sub')) {
+ obj['sub'] = ApiClient.convertToType(data['sub'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * Allowed is true if the request is allowed and false otherwise.
+ * @member {Boolean} allowed
+ */
+ exports.prototype['allowed'] = undefined;
+ /**
+ * Audience is who the token was issued for. This is an OAuth2 app usually.
+ * @member {String} aud
+ */
+ exports.prototype['aud'] = undefined;
+ /**
+ * ExpiresAt is the expiry timestamp.
+ * @member {String} exp
+ */
+ exports.prototype['exp'] = undefined;
+ /**
+ * Extra represents arbitrary session data.
+ * @member {Object.} ext
+ */
+ exports.prototype['ext'] = undefined;
+ /**
+ * IssuedAt is the token creation time stamp.
+ * @member {String} iat
+ */
+ exports.prototype['iat'] = undefined;
+ /**
+ * Issuer is the id of the issuer, typically an hydra instance.
+ * @member {String} iss
+ */
+ exports.prototype['iss'] = undefined;
+ /**
+ * GrantedScopes is a list of scopes that the subject authorized when asked for consent.
+ * @member {Array.} scopes
+ */
+ exports.prototype['scopes'] = undefined;
+ /**
+ * Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app. This is usually a uuid but you can choose a urn or some other id too.
+ * @member {String} sub
+ */
+ exports.prototype['sub'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/WellKnown.js b/sdk/js/swagger/src/model/WellKnown.js
new file mode 100644
index 00000000000..1280207d860
--- /dev/null
+++ b/sdk/js/swagger/src/model/WellKnown.js
@@ -0,0 +1,144 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 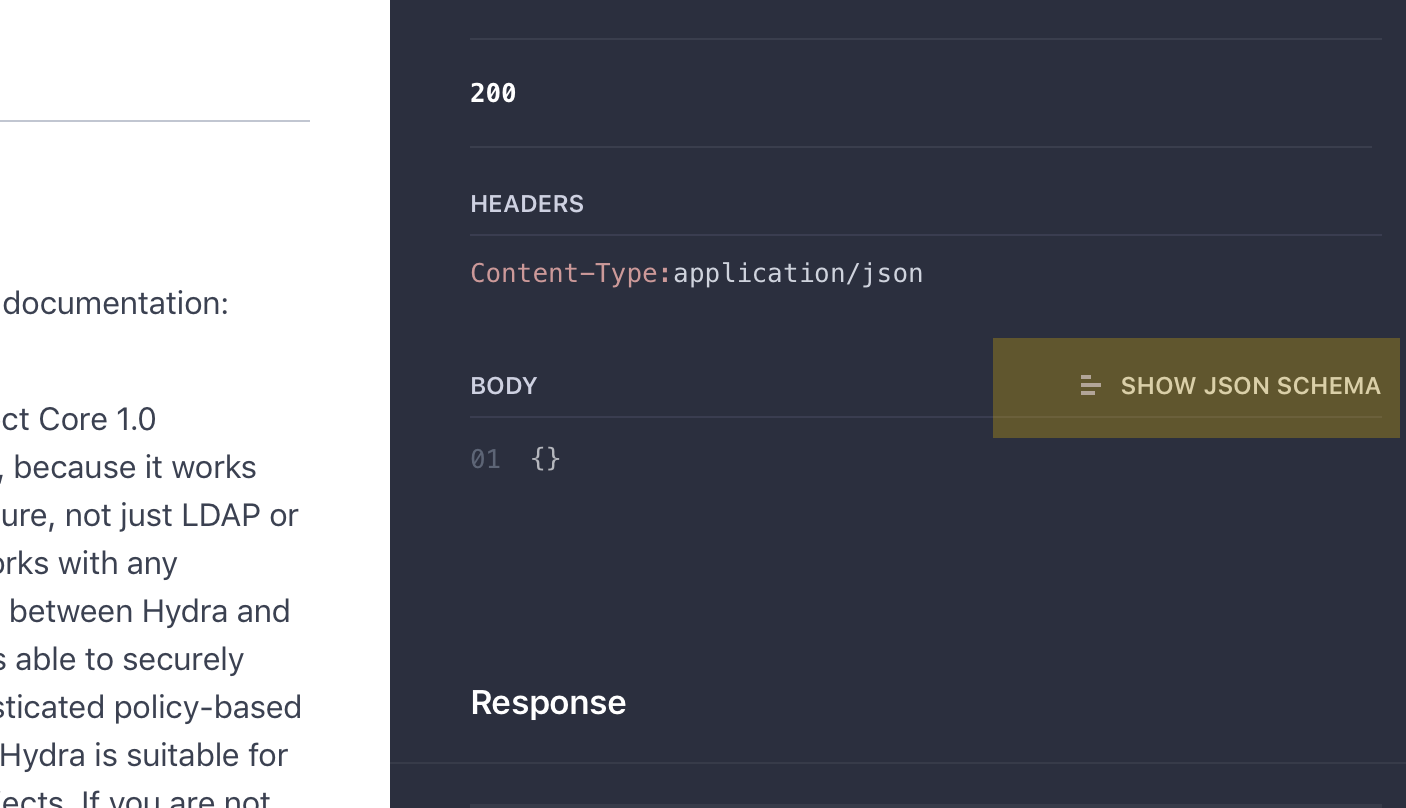 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.WellKnown = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The WellKnown model module.
+ * @module model/WellKnown
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new WellKnown
.
+ * @alias module:model/WellKnown
+ * @class
+ * @param authorizationEndpoint {String} URL of the OP's OAuth 2.0 Authorization Endpoint
+ * @param idTokenSigningAlgValuesSupported {Array.} JSON array containing a list of the JWS signing algorithms (alg values) supported by the OP for the ID Token to encode the Claims in a JWT [JWT]. The algorithm RS256 MUST be included. The value none MAY be supported, but MUST NOT be used unless the Response Type used returns no ID Token from the Authorization Endpoint (such as when using the Authorization Code Flow).
+ * @param issuer {String} URL using the https scheme with no query or fragment component that the OP asserts as its Issuer Identifier. If Issuer discovery is supported , this value MUST be identical to the issuer value returned by WebFinger. This also MUST be identical to the iss Claim value in ID Tokens issued from this Issuer.
+ * @param jwksUri {String} URL of the OP's JSON Web Key Set [JWK] document. This contains the signing key(s) the RP uses to validate signatures from the OP. The JWK Set MAY also contain the Server's encryption key(s), which are used by RPs to encrypt requests to the Server. When both signing and encryption keys are made available, a use (Key Use) parameter value is REQUIRED for all keys in the referenced JWK Set to indicate each key's intended usage. Although some algorithms allow the same key to be used for both signatures and encryption, doing so is NOT RECOMMENDED, as it is less secure. The JWK x5c parameter MAY be used to provide X.509 representations of keys provided. When used, the bare key values MUST still be present and MUST match those in the certificate.
+ * @param responseTypesSupported {Array.} JSON array containing a list of the OAuth 2.0 response_type values that this OP supports. Dynamic OpenID Providers MUST support the code, id_token, and the token id_token Response Type values.
+ * @param subjectTypesSupported {Array.} JSON array containing a list of the Subject Identifier types that this OP supports. Valid types include pairwise and public.
+ * @param tokenEndpoint {String} URL of the OP's OAuth 2.0 Token Endpoint
+ */
+ var exports = function(authorizationEndpoint, idTokenSigningAlgValuesSupported, issuer, jwksUri, responseTypesSupported, subjectTypesSupported, tokenEndpoint) {
+ var _this = this;
+
+ _this['authorization_endpoint'] = authorizationEndpoint;
+ _this['id_token_signing_alg_values_supported'] = idTokenSigningAlgValuesSupported;
+ _this['issuer'] = issuer;
+ _this['jwks_uri'] = jwksUri;
+ _this['response_types_supported'] = responseTypesSupported;
+ _this['subject_types_supported'] = subjectTypesSupported;
+ _this['token_endpoint'] = tokenEndpoint;
+ };
+
+ /**
+ * Constructs a WellKnown
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/WellKnown} obj Optional instance to populate.
+ * @return {module:model/WellKnown} The populated WellKnown
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ if (data.hasOwnProperty('authorization_endpoint')) {
+ obj['authorization_endpoint'] = ApiClient.convertToType(data['authorization_endpoint'], 'String');
+ }
+ if (data.hasOwnProperty('id_token_signing_alg_values_supported')) {
+ obj['id_token_signing_alg_values_supported'] = ApiClient.convertToType(data['id_token_signing_alg_values_supported'], ['String']);
+ }
+ if (data.hasOwnProperty('issuer')) {
+ obj['issuer'] = ApiClient.convertToType(data['issuer'], 'String');
+ }
+ if (data.hasOwnProperty('jwks_uri')) {
+ obj['jwks_uri'] = ApiClient.convertToType(data['jwks_uri'], 'String');
+ }
+ if (data.hasOwnProperty('response_types_supported')) {
+ obj['response_types_supported'] = ApiClient.convertToType(data['response_types_supported'], ['String']);
+ }
+ if (data.hasOwnProperty('subject_types_supported')) {
+ obj['subject_types_supported'] = ApiClient.convertToType(data['subject_types_supported'], ['String']);
+ }
+ if (data.hasOwnProperty('token_endpoint')) {
+ obj['token_endpoint'] = ApiClient.convertToType(data['token_endpoint'], 'String');
+ }
+ }
+ return obj;
+ }
+
+ /**
+ * URL of the OP's OAuth 2.0 Authorization Endpoint
+ * @member {String} authorization_endpoint
+ */
+ exports.prototype['authorization_endpoint'] = undefined;
+ /**
+ * JSON array containing a list of the JWS signing algorithms (alg values) supported by the OP for the ID Token to encode the Claims in a JWT [JWT]. The algorithm RS256 MUST be included. The value none MAY be supported, but MUST NOT be used unless the Response Type used returns no ID Token from the Authorization Endpoint (such as when using the Authorization Code Flow).
+ * @member {Array.} id_token_signing_alg_values_supported
+ */
+ exports.prototype['id_token_signing_alg_values_supported'] = undefined;
+ /**
+ * URL using the https scheme with no query or fragment component that the OP asserts as its Issuer Identifier. If Issuer discovery is supported , this value MUST be identical to the issuer value returned by WebFinger. This also MUST be identical to the iss Claim value in ID Tokens issued from this Issuer.
+ * @member {String} issuer
+ */
+ exports.prototype['issuer'] = undefined;
+ /**
+ * URL of the OP's JSON Web Key Set [JWK] document. This contains the signing key(s) the RP uses to validate signatures from the OP. The JWK Set MAY also contain the Server's encryption key(s), which are used by RPs to encrypt requests to the Server. When both signing and encryption keys are made available, a use (Key Use) parameter value is REQUIRED for all keys in the referenced JWK Set to indicate each key's intended usage. Although some algorithms allow the same key to be used for both signatures and encryption, doing so is NOT RECOMMENDED, as it is less secure. The JWK x5c parameter MAY be used to provide X.509 representations of keys provided. When used, the bare key values MUST still be present and MUST match those in the certificate.
+ * @member {String} jwks_uri
+ */
+ exports.prototype['jwks_uri'] = undefined;
+ /**
+ * JSON array containing a list of the OAuth 2.0 response_type values that this OP supports. Dynamic OpenID Providers MUST support the code, id_token, and the token id_token Response Type values.
+ * @member {Array.} response_types_supported
+ */
+ exports.prototype['response_types_supported'] = undefined;
+ /**
+ * JSON array containing a list of the Subject Identifier types that this OP supports. Valid types include pairwise and public.
+ * @member {Array.} subject_types_supported
+ */
+ exports.prototype['subject_types_supported'] = undefined;
+ /**
+ * URL of the OP's OAuth 2.0 Token Endpoint
+ * @member {String} token_endpoint
+ */
+ exports.prototype['token_endpoint'] = undefined;
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/src/model/Writer.js b/sdk/js/swagger/src/model/Writer.js
new file mode 100644
index 00000000000..cdedaddca3b
--- /dev/null
+++ b/sdk/js/swagger/src/model/Writer.js
@@ -0,0 +1,75 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 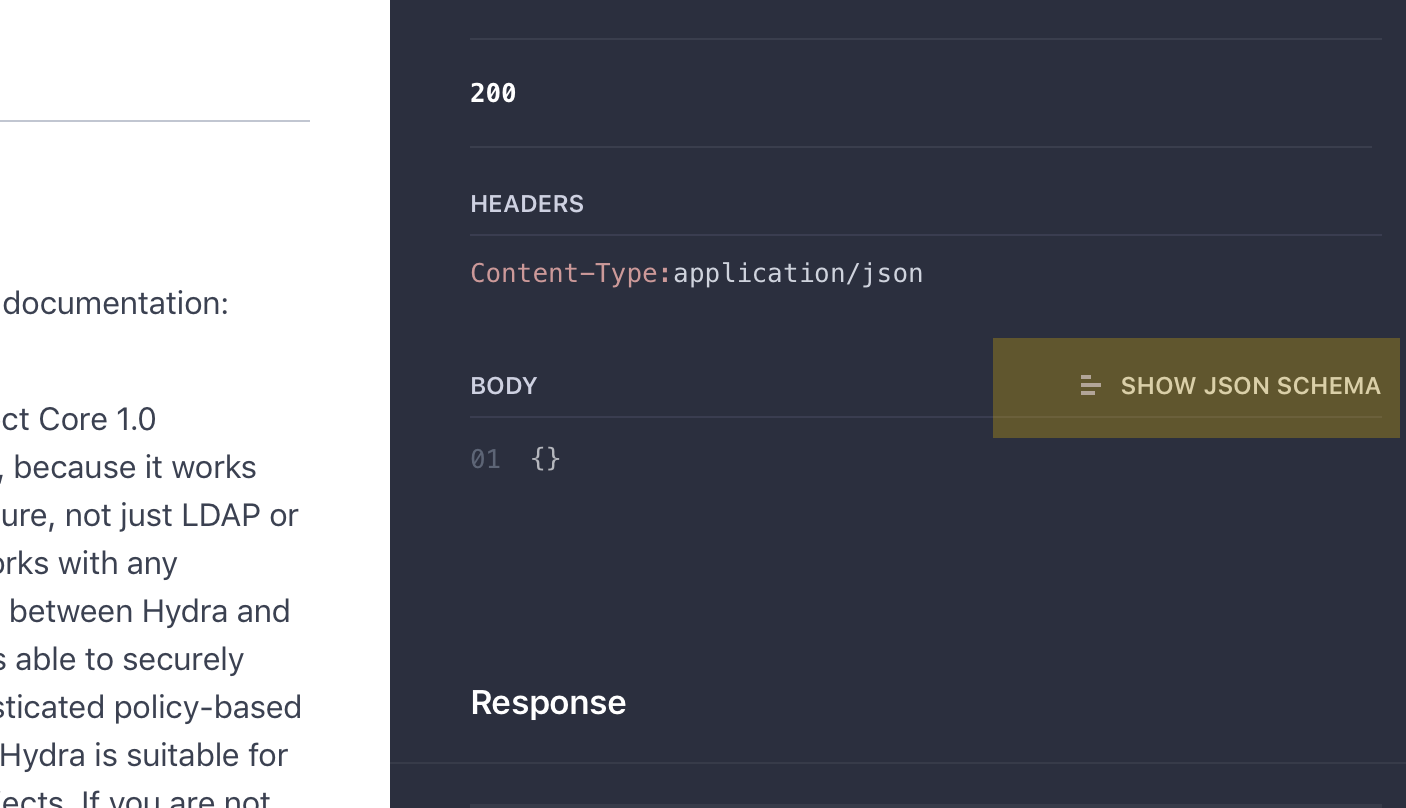 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD. Register as an anonymous module.
+ define(['ApiClient'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ module.exports = factory(require('../ApiClient'));
+ } else {
+ // Browser globals (root is window)
+ if (!root.HydraOAuth2OpenIdConnectServer) {
+ root.HydraOAuth2OpenIdConnectServer = {};
+ }
+ root.HydraOAuth2OpenIdConnectServer.Writer = factory(root.HydraOAuth2OpenIdConnectServer.ApiClient);
+ }
+}(this, function(ApiClient) {
+ 'use strict';
+
+
+
+
+ /**
+ * The Writer model module.
+ * @module model/Writer
+ * @version Latest
+ */
+
+ /**
+ * Constructs a new Writer
.
+ * Writer is a helper to write arbitrary data to a ResponseWriter
+ * @alias module:model/Writer
+ * @class
+ */
+ var exports = function() {
+ var _this = this;
+
+ };
+
+ /**
+ * Constructs a Writer
from a plain JavaScript object, optionally creating a new instance.
+ * Copies all relevant properties from data
to obj
if supplied or a new instance if not.
+ * @param {Object} data The plain JavaScript object bearing properties of interest.
+ * @param {module:model/Writer} obj Optional instance to populate.
+ * @return {module:model/Writer} The populated Writer
instance.
+ */
+ exports.constructFromObject = function(data, obj) {
+ if (data) {
+ obj = obj || new exports();
+
+ }
+ return obj;
+ }
+
+
+
+
+ return exports;
+}));
+
+
diff --git a/sdk/js/swagger/test/api/HealthApi.spec.js b/sdk/js/swagger/test/api/HealthApi.spec.js
new file mode 100644
index 00000000000..808944773c5
--- /dev/null
+++ b/sdk/js/swagger/test/api/HealthApi.spec.js
@@ -0,0 +1,76 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 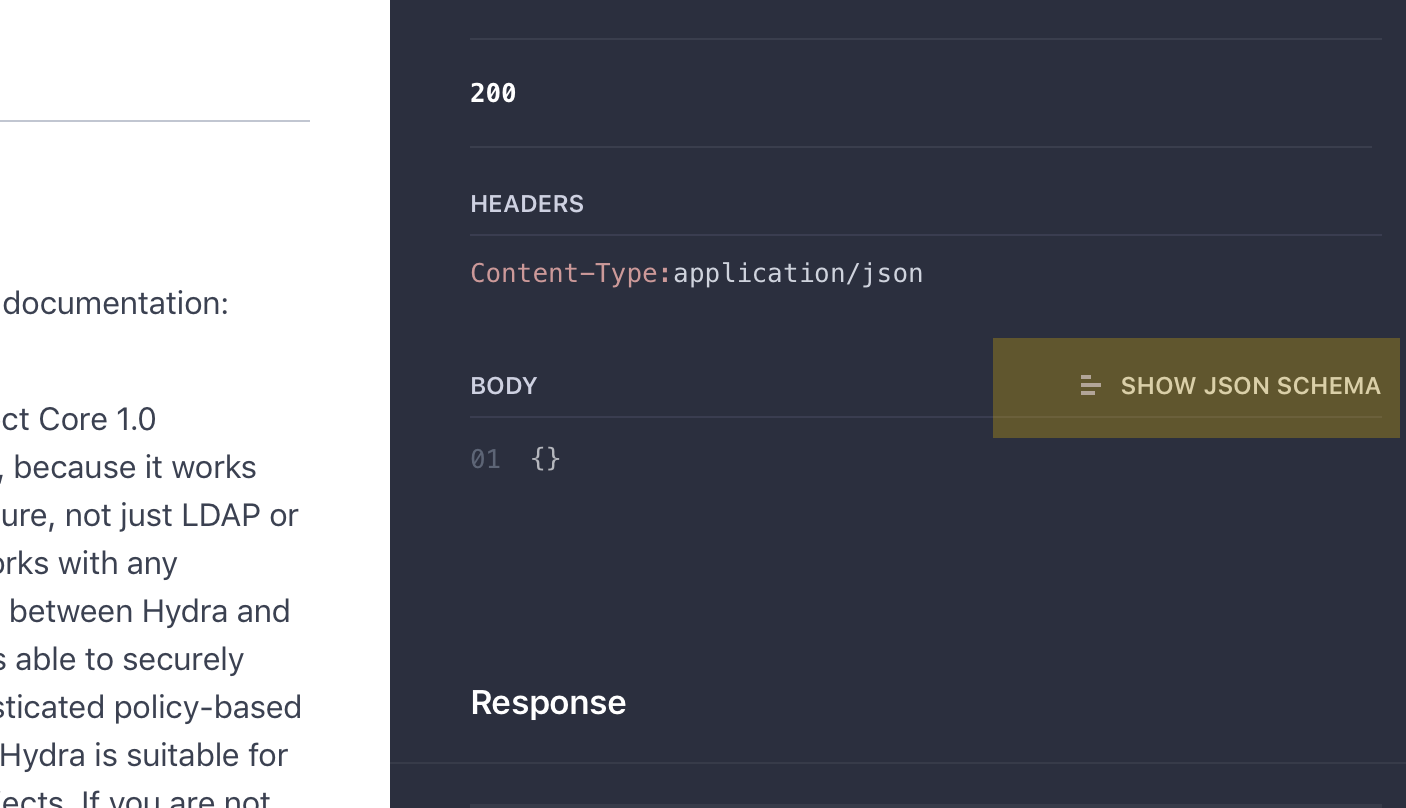 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.HealthApi();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('HealthApi', function() {
+ describe('getInstanceMetrics', function() {
+ it('should call getInstanceMetrics successfully', function(done) {
+ //uncomment below and update the code to test getInstanceMetrics
+ //instance.getInstanceMetrics(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('getInstanceStatus', function() {
+ it('should call getInstanceStatus successfully', function(done) {
+ //uncomment below and update the code to test getInstanceStatus
+ //instance.getInstanceStatus(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ });
+
+}));
diff --git a/sdk/js/swagger/test/api/JsonWebKeyApi.spec.js b/sdk/js/swagger/test/api/JsonWebKeyApi.spec.js
new file mode 100644
index 00000000000..93b470be9b0
--- /dev/null
+++ b/sdk/js/swagger/test/api/JsonWebKeyApi.spec.js
@@ -0,0 +1,126 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 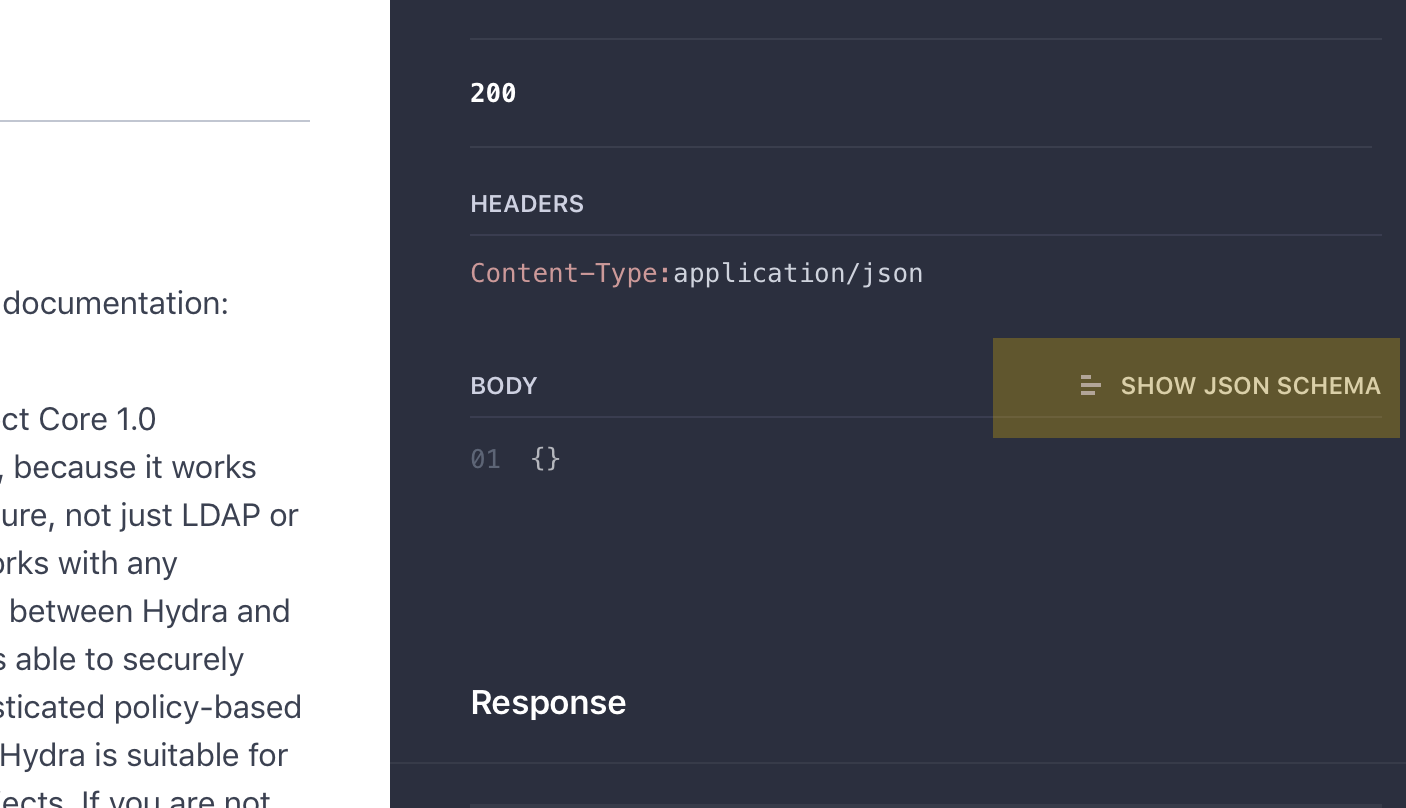 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.JsonWebKeyApi();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('JsonWebKeyApi', function() {
+ describe('createJsonWebKeySet', function() {
+ it('should call createJsonWebKeySet successfully', function(done) {
+ //uncomment below and update the code to test createJsonWebKeySet
+ //instance.createJsonWebKeySet(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('deleteJsonWebKey', function() {
+ it('should call deleteJsonWebKey successfully', function(done) {
+ //uncomment below and update the code to test deleteJsonWebKey
+ //instance.deleteJsonWebKey(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('deleteJsonWebKeySet', function() {
+ it('should call deleteJsonWebKeySet successfully', function(done) {
+ //uncomment below and update the code to test deleteJsonWebKeySet
+ //instance.deleteJsonWebKeySet(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('getJsonWebKey', function() {
+ it('should call getJsonWebKey successfully', function(done) {
+ //uncomment below and update the code to test getJsonWebKey
+ //instance.getJsonWebKey(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('getJsonWebKeySet', function() {
+ it('should call getJsonWebKeySet successfully', function(done) {
+ //uncomment below and update the code to test getJsonWebKeySet
+ //instance.getJsonWebKeySet(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('updateJsonWebKey', function() {
+ it('should call updateJsonWebKey successfully', function(done) {
+ //uncomment below and update the code to test updateJsonWebKey
+ //instance.updateJsonWebKey(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('updateJsonWebKeySet', function() {
+ it('should call updateJsonWebKeySet successfully', function(done) {
+ //uncomment below and update the code to test updateJsonWebKeySet
+ //instance.updateJsonWebKeySet(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ });
+
+}));
diff --git a/sdk/js/swagger/test/api/OAuth2Api.spec.js b/sdk/js/swagger/test/api/OAuth2Api.spec.js
new file mode 100644
index 00000000000..a779b1c2b6f
--- /dev/null
+++ b/sdk/js/swagger/test/api/OAuth2Api.spec.js
@@ -0,0 +1,196 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 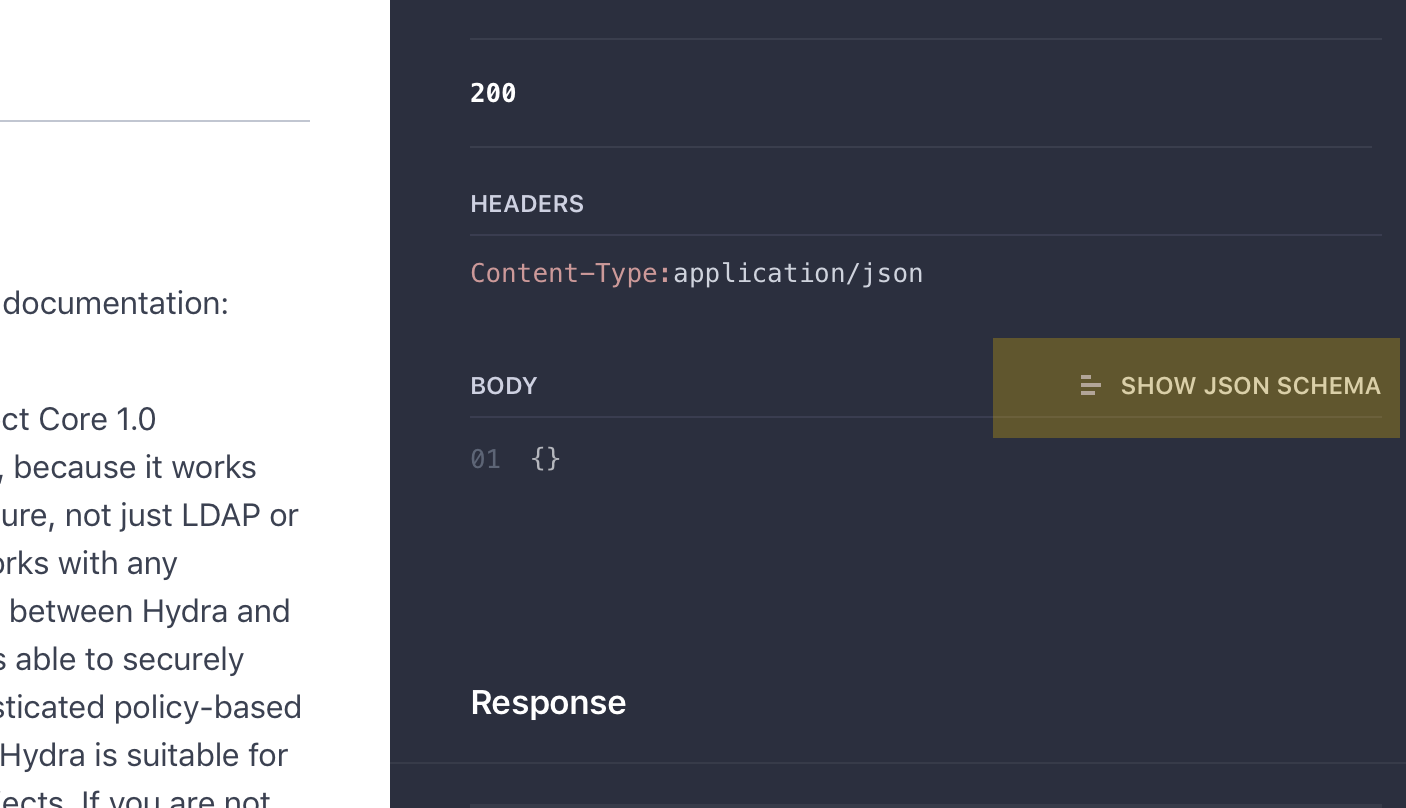 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.OAuth2Api();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('OAuth2Api', function() {
+ describe('acceptOAuth2ConsentRequest', function() {
+ it('should call acceptOAuth2ConsentRequest successfully', function(done) {
+ //uncomment below and update the code to test acceptOAuth2ConsentRequest
+ //instance.acceptOAuth2ConsentRequest(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('createOAuth2Client', function() {
+ it('should call createOAuth2Client successfully', function(done) {
+ //uncomment below and update the code to test createOAuth2Client
+ //instance.createOAuth2Client(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('deleteOAuth2Client', function() {
+ it('should call deleteOAuth2Client successfully', function(done) {
+ //uncomment below and update the code to test deleteOAuth2Client
+ //instance.deleteOAuth2Client(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('getOAuth2Client', function() {
+ it('should call getOAuth2Client successfully', function(done) {
+ //uncomment below and update the code to test getOAuth2Client
+ //instance.getOAuth2Client(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('getOAuth2ConsentRequest', function() {
+ it('should call getOAuth2ConsentRequest successfully', function(done) {
+ //uncomment below and update the code to test getOAuth2ConsentRequest
+ //instance.getOAuth2ConsentRequest(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('getWellKnown', function() {
+ it('should call getWellKnown successfully', function(done) {
+ //uncomment below and update the code to test getWellKnown
+ //instance.getWellKnown(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('introspectOAuth2Token', function() {
+ it('should call introspectOAuth2Token successfully', function(done) {
+ //uncomment below and update the code to test introspectOAuth2Token
+ //instance.introspectOAuth2Token(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('listOAuth2Clients', function() {
+ it('should call listOAuth2Clients successfully', function(done) {
+ //uncomment below and update the code to test listOAuth2Clients
+ //instance.listOAuth2Clients(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('oauthAuth', function() {
+ it('should call oauthAuth successfully', function(done) {
+ //uncomment below and update the code to test oauthAuth
+ //instance.oauthAuth(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('oauthToken', function() {
+ it('should call oauthToken successfully', function(done) {
+ //uncomment below and update the code to test oauthToken
+ //instance.oauthToken(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('rejectOAuth2ConsentRequest', function() {
+ it('should call rejectOAuth2ConsentRequest successfully', function(done) {
+ //uncomment below and update the code to test rejectOAuth2ConsentRequest
+ //instance.rejectOAuth2ConsentRequest(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('revokeOAuth2Token', function() {
+ it('should call revokeOAuth2Token successfully', function(done) {
+ //uncomment below and update the code to test revokeOAuth2Token
+ //instance.revokeOAuth2Token(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('updateOAuth2Client', function() {
+ it('should call updateOAuth2Client successfully', function(done) {
+ //uncomment below and update the code to test updateOAuth2Client
+ //instance.updateOAuth2Client(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('wellKnown', function() {
+ it('should call wellKnown successfully', function(done) {
+ //uncomment below and update the code to test wellKnown
+ //instance.wellKnown(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ });
+
+}));
diff --git a/sdk/js/swagger/test/api/PolicyApi.spec.js b/sdk/js/swagger/test/api/PolicyApi.spec.js
new file mode 100644
index 00000000000..23fc1ba6d1b
--- /dev/null
+++ b/sdk/js/swagger/test/api/PolicyApi.spec.js
@@ -0,0 +1,106 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 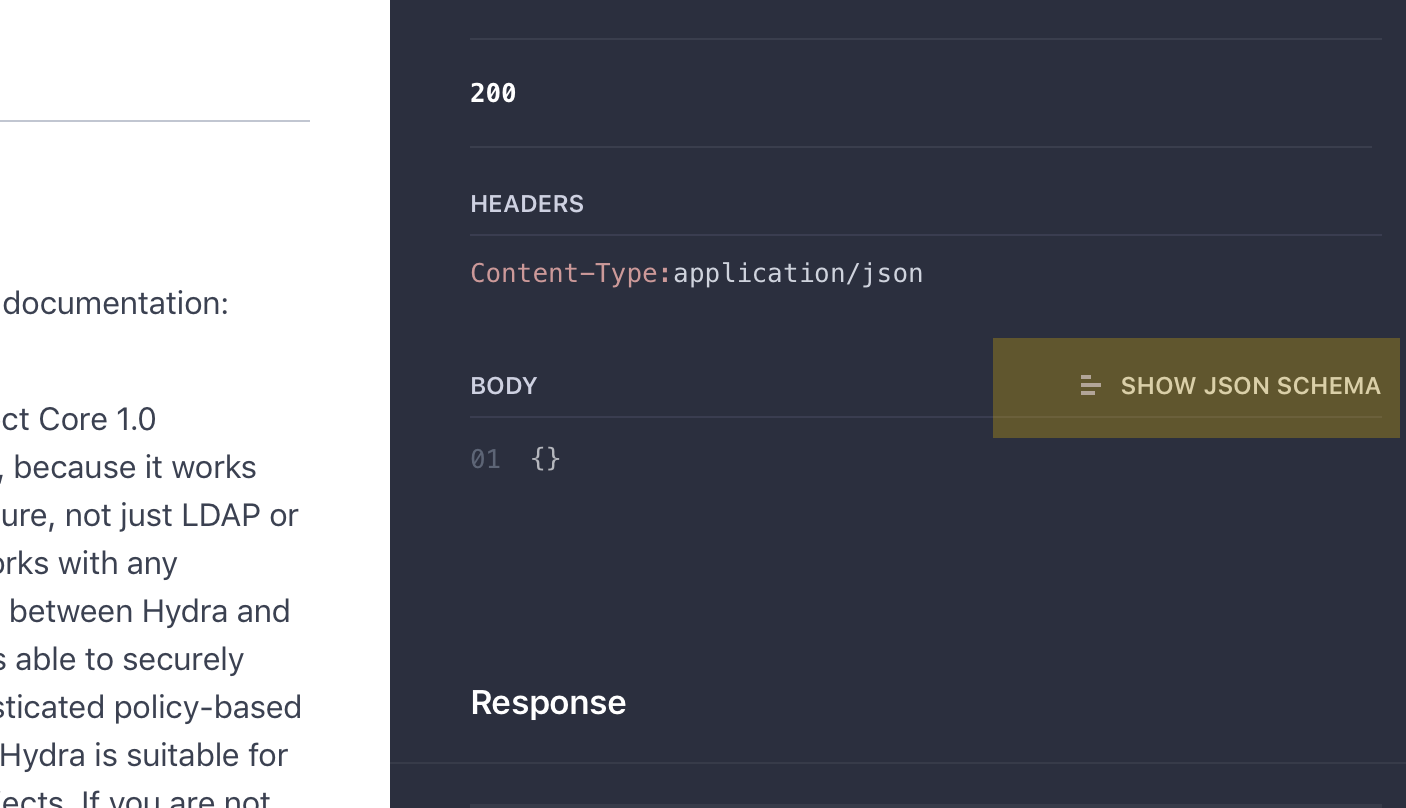 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.PolicyApi();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('PolicyApi', function() {
+ describe('createPolicy', function() {
+ it('should call createPolicy successfully', function(done) {
+ //uncomment below and update the code to test createPolicy
+ //instance.createPolicy(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('deletePolicy', function() {
+ it('should call deletePolicy successfully', function(done) {
+ //uncomment below and update the code to test deletePolicy
+ //instance.deletePolicy(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('getPolicy', function() {
+ it('should call getPolicy successfully', function(done) {
+ //uncomment below and update the code to test getPolicy
+ //instance.getPolicy(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('listPolicies', function() {
+ it('should call listPolicies successfully', function(done) {
+ //uncomment below and update the code to test listPolicies
+ //instance.listPolicies(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('updatePolicy', function() {
+ it('should call updatePolicy successfully', function(done) {
+ //uncomment below and update the code to test updatePolicy
+ //instance.updatePolicy(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ });
+
+}));
diff --git a/sdk/js/swagger/test/api/WardenApi.spec.js b/sdk/js/swagger/test/api/WardenApi.spec.js
new file mode 100644
index 00000000000..a460ea5e4ed
--- /dev/null
+++ b/sdk/js/swagger/test/api/WardenApi.spec.js
@@ -0,0 +1,136 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 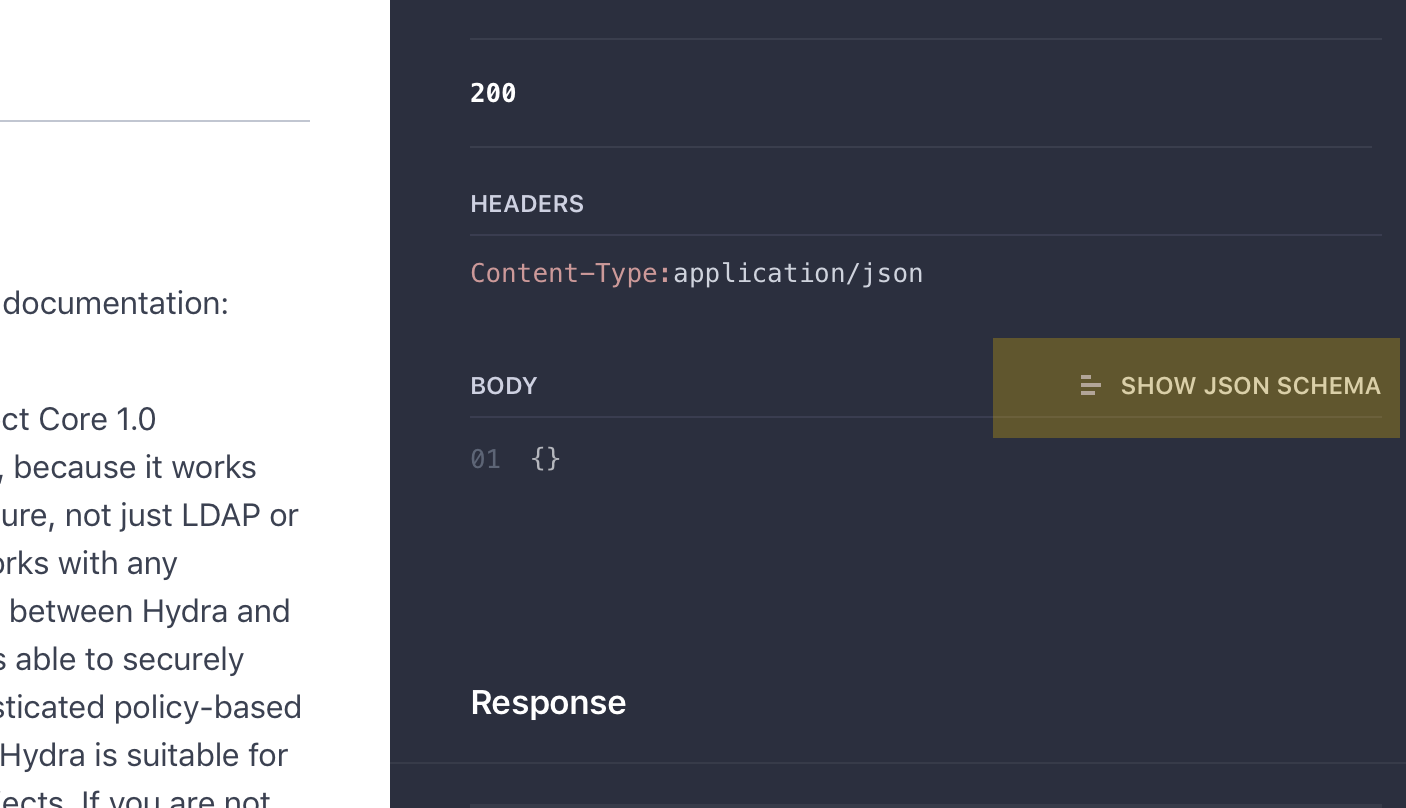 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.WardenApi();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('WardenApi', function() {
+ describe('addMembersToGroup', function() {
+ it('should call addMembersToGroup successfully', function(done) {
+ //uncomment below and update the code to test addMembersToGroup
+ //instance.addMembersToGroup(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('createGroup', function() {
+ it('should call createGroup successfully', function(done) {
+ //uncomment below and update the code to test createGroup
+ //instance.createGroup(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('deleteGroup', function() {
+ it('should call deleteGroup successfully', function(done) {
+ //uncomment below and update the code to test deleteGroup
+ //instance.deleteGroup(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('doesWardenAllowAccessRequest', function() {
+ it('should call doesWardenAllowAccessRequest successfully', function(done) {
+ //uncomment below and update the code to test doesWardenAllowAccessRequest
+ //instance.doesWardenAllowAccessRequest(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('doesWardenAllowTokenAccessRequest', function() {
+ it('should call doesWardenAllowTokenAccessRequest successfully', function(done) {
+ //uncomment below and update the code to test doesWardenAllowTokenAccessRequest
+ //instance.doesWardenAllowTokenAccessRequest(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('findGroupsByMember', function() {
+ it('should call findGroupsByMember successfully', function(done) {
+ //uncomment below and update the code to test findGroupsByMember
+ //instance.findGroupsByMember(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('getGroup', function() {
+ it('should call getGroup successfully', function(done) {
+ //uncomment below and update the code to test getGroup
+ //instance.getGroup(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ describe('removeMembersFromGroup', function() {
+ it('should call removeMembersFromGroup successfully', function(done) {
+ //uncomment below and update the code to test removeMembersFromGroup
+ //instance.removeMembersFromGroup(function(error) {
+ // if (error) throw error;
+ //expect().to.be();
+ //});
+ done();
+ });
+ });
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/ConsentRequestAcceptance.spec.js b/sdk/js/swagger/test/model/ConsentRequestAcceptance.spec.js
new file mode 100644
index 00000000000..5d68538482b
--- /dev/null
+++ b/sdk/js/swagger/test/model/ConsentRequestAcceptance.spec.js
@@ -0,0 +1,86 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 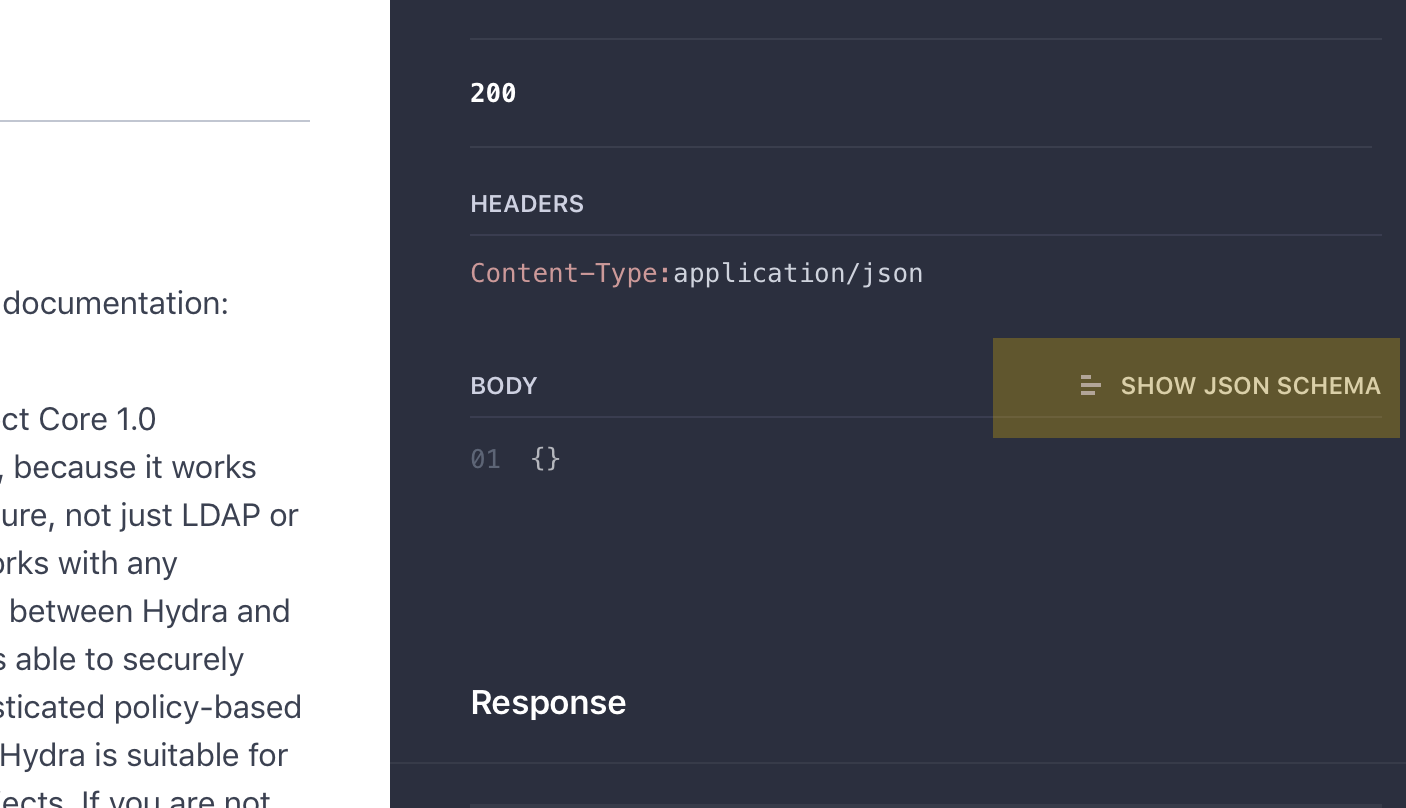 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('ConsentRequestAcceptance', function() {
+ it('should create an instance of ConsentRequestAcceptance', function() {
+ // uncomment below and update the code to test ConsentRequestAcceptance
+ //var instane = new HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance);
+ });
+
+ it('should have the property accessTokenExtra (base name: "accessTokenExtra")', function() {
+ // uncomment below and update the code to test the property accessTokenExtra
+ //var instane = new HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property grantScopes (base name: "grantScopes")', function() {
+ // uncomment below and update the code to test the property grantScopes
+ //var instane = new HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property idTokenExtra (base name: "idTokenExtra")', function() {
+ // uncomment below and update the code to test the property idTokenExtra
+ //var instane = new HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property subject (base name: "subject")', function() {
+ // uncomment below and update the code to test the property subject
+ //var instane = new HydraOAuth2OpenIdConnectServer.ConsentRequestAcceptance();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/ConsentRequestManager.spec.js b/sdk/js/swagger/test/model/ConsentRequestManager.spec.js
new file mode 100644
index 00000000000..b33ffff86bd
--- /dev/null
+++ b/sdk/js/swagger/test/model/ConsentRequestManager.spec.js
@@ -0,0 +1,62 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 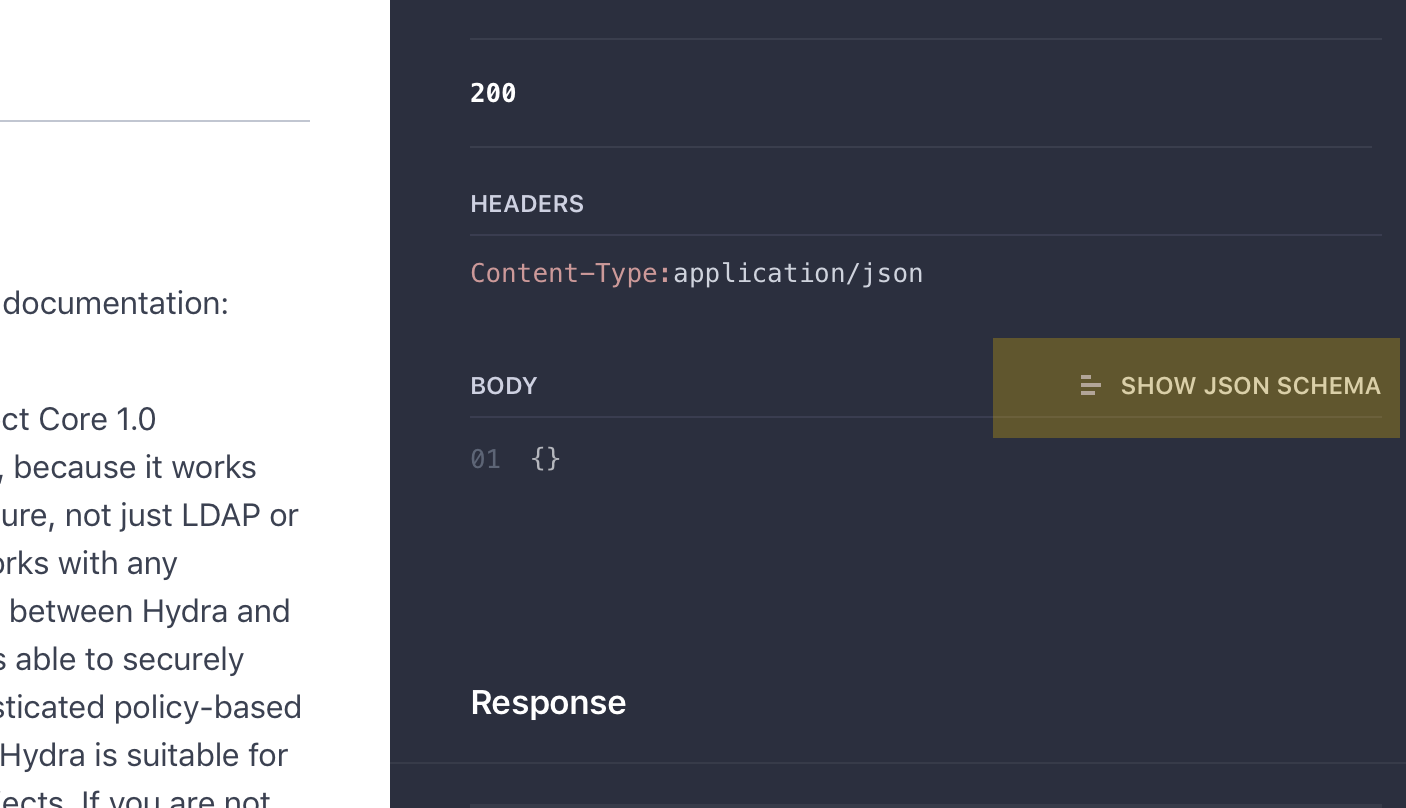 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.ConsentRequestManager();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('ConsentRequestManager', function() {
+ it('should create an instance of ConsentRequestManager', function() {
+ // uncomment below and update the code to test ConsentRequestManager
+ //var instane = new HydraOAuth2OpenIdConnectServer.ConsentRequestManager();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.ConsentRequestManager);
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/ConsentRequestRejection.spec.js b/sdk/js/swagger/test/model/ConsentRequestRejection.spec.js
new file mode 100644
index 00000000000..ae8d13ac0dd
--- /dev/null
+++ b/sdk/js/swagger/test/model/ConsentRequestRejection.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 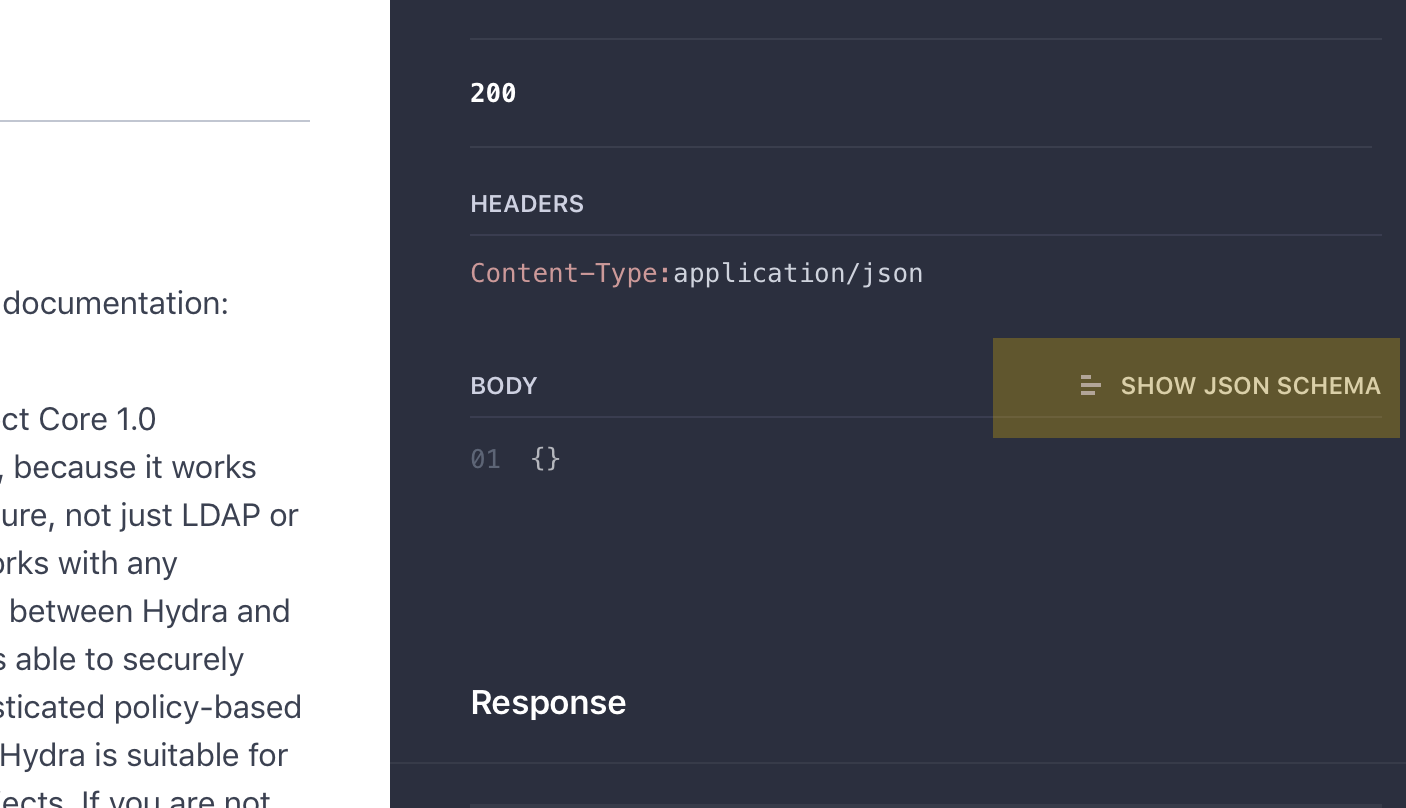 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.ConsentRequestRejection();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('ConsentRequestRejection', function() {
+ it('should create an instance of ConsentRequestRejection', function() {
+ // uncomment below and update the code to test ConsentRequestRejection
+ //var instane = new HydraOAuth2OpenIdConnectServer.ConsentRequestRejection();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.ConsentRequestRejection);
+ });
+
+ it('should have the property reason (base name: "reason")', function() {
+ // uncomment below and update the code to test the property reason
+ //var instane = new HydraOAuth2OpenIdConnectServer.ConsentRequestRejection();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/Context.spec.js b/sdk/js/swagger/test/model/Context.spec.js
new file mode 100644
index 00000000000..5bfeade1790
--- /dev/null
+++ b/sdk/js/swagger/test/model/Context.spec.js
@@ -0,0 +1,92 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 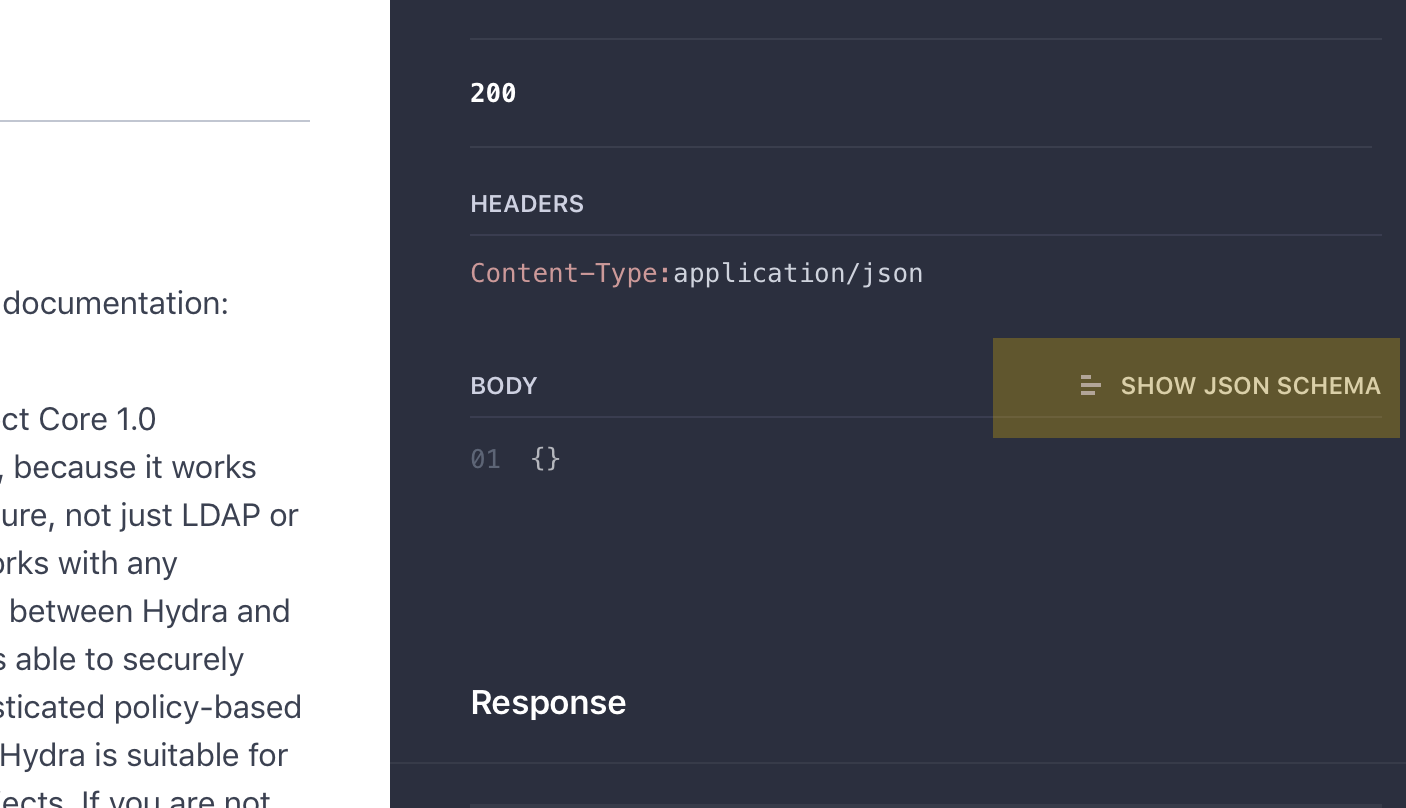 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.Context();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('Context', function() {
+ it('should create an instance of Context', function() {
+ // uncomment below and update the code to test Context
+ //var instane = new HydraOAuth2OpenIdConnectServer.Context();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.Context);
+ });
+
+ it('should have the property aud (base name: "aud")', function() {
+ // uncomment below and update the code to test the property aud
+ //var instane = new HydraOAuth2OpenIdConnectServer.Context();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property ext (base name: "ext")', function() {
+ // uncomment below and update the code to test the property ext
+ //var instane = new HydraOAuth2OpenIdConnectServer.Context();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property iss (base name: "iss")', function() {
+ // uncomment below and update the code to test the property iss
+ //var instane = new HydraOAuth2OpenIdConnectServer.Context();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property scopes (base name: "scopes")', function() {
+ // uncomment below and update the code to test the property scopes
+ //var instane = new HydraOAuth2OpenIdConnectServer.Context();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property sub (base name: "sub")', function() {
+ // uncomment below and update the code to test the property sub
+ //var instane = new HydraOAuth2OpenIdConnectServer.Context();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/Firewall.spec.js b/sdk/js/swagger/test/model/Firewall.spec.js
new file mode 100644
index 00000000000..54158ba9103
--- /dev/null
+++ b/sdk/js/swagger/test/model/Firewall.spec.js
@@ -0,0 +1,62 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 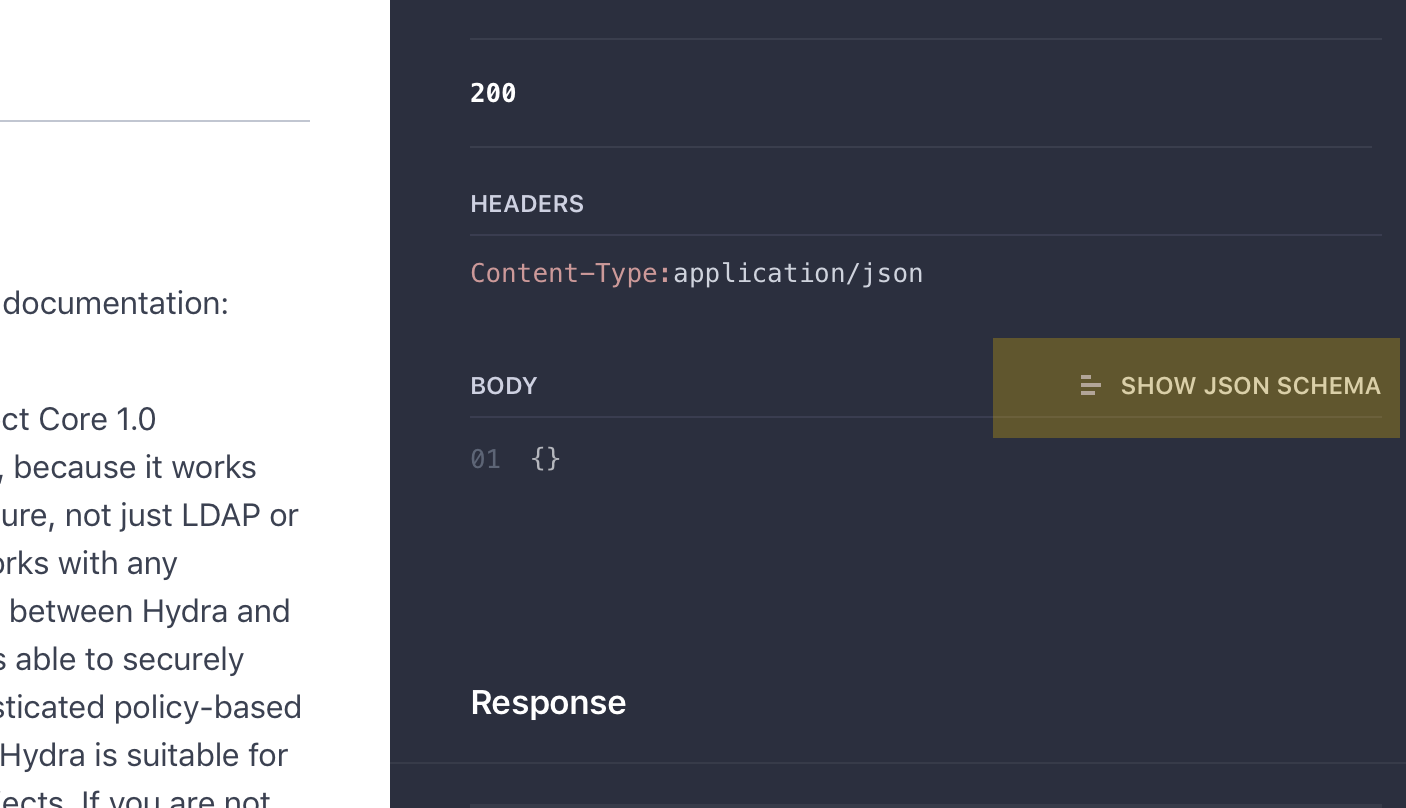 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.Firewall();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('Firewall', function() {
+ it('should create an instance of Firewall', function() {
+ // uncomment below and update the code to test Firewall
+ //var instane = new HydraOAuth2OpenIdConnectServer.Firewall();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.Firewall);
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/Group.spec.js b/sdk/js/swagger/test/model/Group.spec.js
new file mode 100644
index 00000000000..647b77023df
--- /dev/null
+++ b/sdk/js/swagger/test/model/Group.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 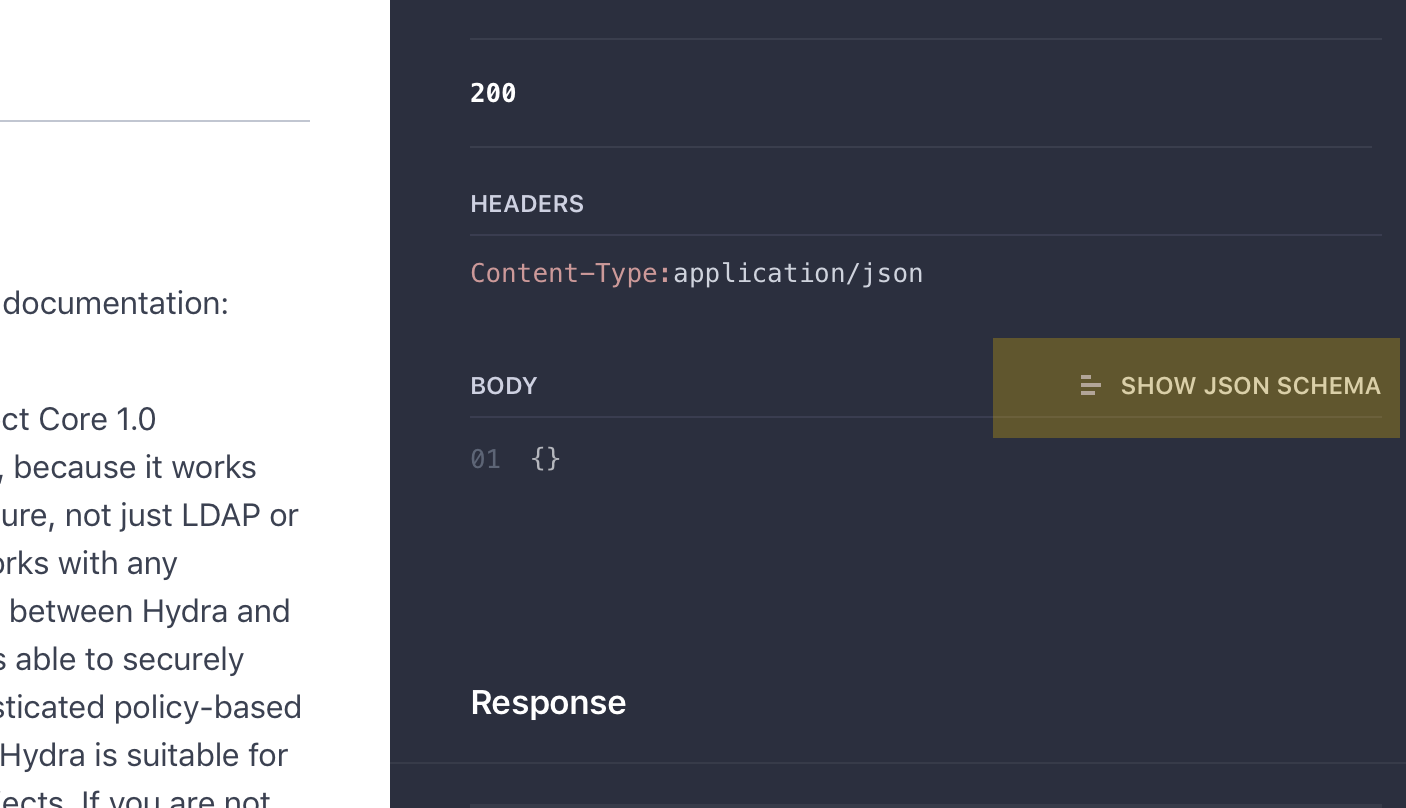 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.Group();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('Group', function() {
+ it('should create an instance of Group', function() {
+ // uncomment below and update the code to test Group
+ //var instane = new HydraOAuth2OpenIdConnectServer.Group();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.Group);
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.Group();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property members (base name: "members")', function() {
+ // uncomment below and update the code to test the property members
+ //var instane = new HydraOAuth2OpenIdConnectServer.Group();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/GroupMembers.spec.js b/sdk/js/swagger/test/model/GroupMembers.spec.js
new file mode 100644
index 00000000000..497b6e421fb
--- /dev/null
+++ b/sdk/js/swagger/test/model/GroupMembers.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 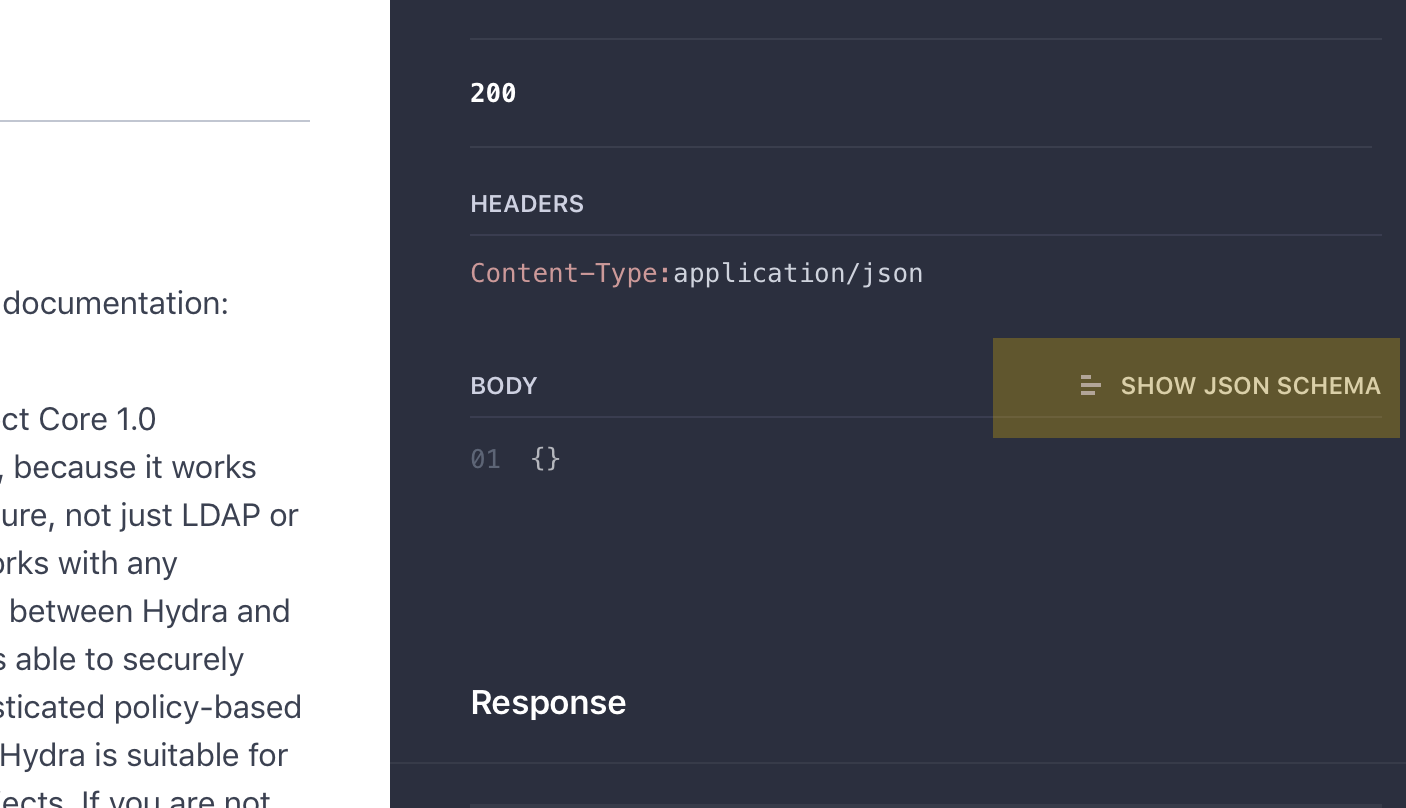 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.GroupMembers();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('GroupMembers', function() {
+ it('should create an instance of GroupMembers', function() {
+ // uncomment below and update the code to test GroupMembers
+ //var instane = new HydraOAuth2OpenIdConnectServer.GroupMembers();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.GroupMembers);
+ });
+
+ it('should have the property members (base name: "members")', function() {
+ // uncomment below and update the code to test the property members
+ //var instane = new HydraOAuth2OpenIdConnectServer.GroupMembers();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/Handler.spec.js b/sdk/js/swagger/test/model/Handler.spec.js
new file mode 100644
index 00000000000..c5890c3f753
--- /dev/null
+++ b/sdk/js/swagger/test/model/Handler.spec.js
@@ -0,0 +1,86 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 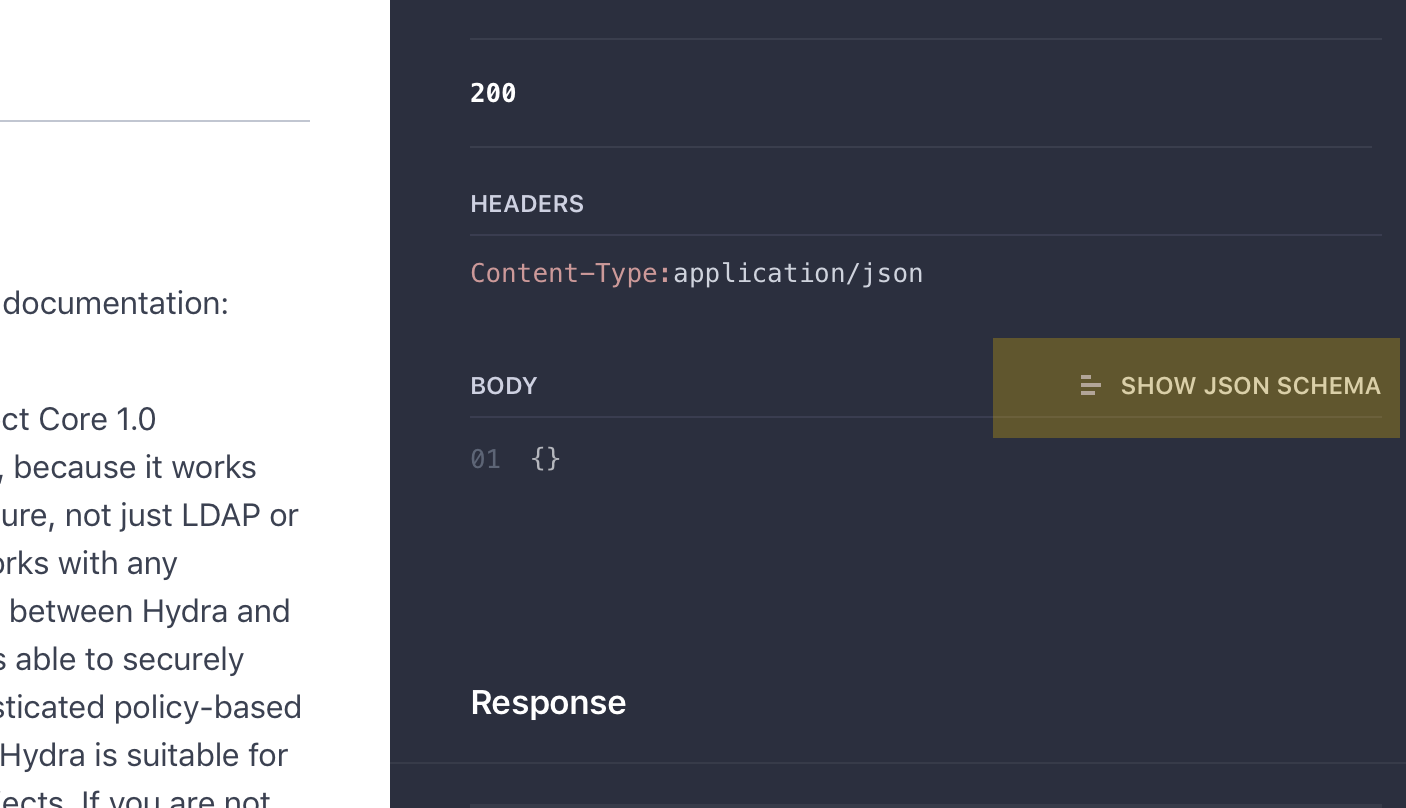 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.Handler();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('Handler', function() {
+ it('should create an instance of Handler', function() {
+ // uncomment below and update the code to test Handler
+ //var instane = new HydraOAuth2OpenIdConnectServer.Handler();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.Handler);
+ });
+
+ it('should have the property generators (base name: "Generators")', function() {
+ // uncomment below and update the code to test the property generators
+ //var instane = new HydraOAuth2OpenIdConnectServer.Handler();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property H (base name: "H")', function() {
+ // uncomment below and update the code to test the property H
+ //var instane = new HydraOAuth2OpenIdConnectServer.Handler();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property manager (base name: "Manager")', function() {
+ // uncomment below and update the code to test the property manager
+ //var instane = new HydraOAuth2OpenIdConnectServer.Handler();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property W (base name: "W")', function() {
+ // uncomment below and update the code to test the property W
+ //var instane = new HydraOAuth2OpenIdConnectServer.Handler();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/InlineResponse200.spec.js b/sdk/js/swagger/test/model/InlineResponse200.spec.js
new file mode 100644
index 00000000000..2a18f958489
--- /dev/null
+++ b/sdk/js/swagger/test/model/InlineResponse200.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 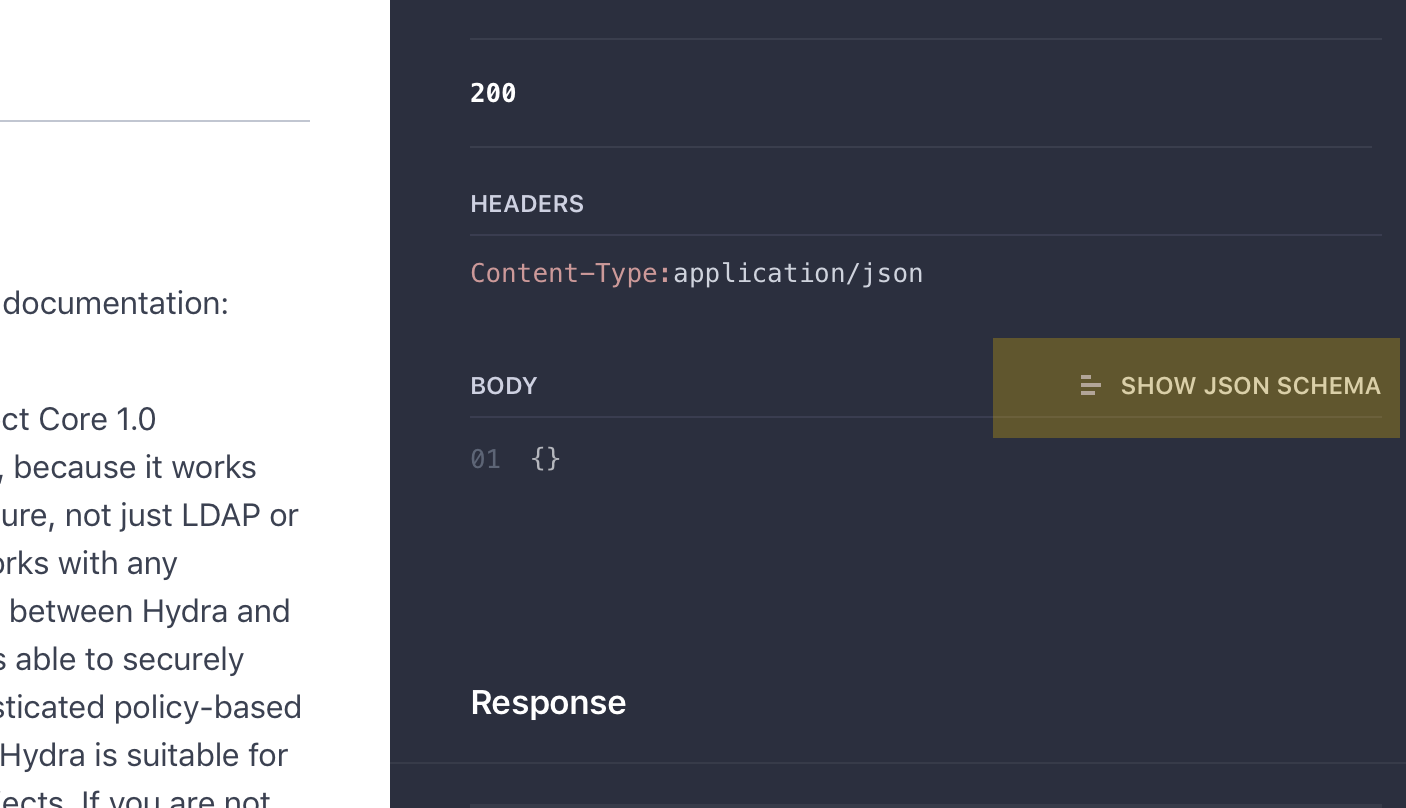 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.InlineResponse200();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('InlineResponse200', function() {
+ it('should create an instance of InlineResponse200', function() {
+ // uncomment below and update the code to test InlineResponse200
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse200();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.InlineResponse200);
+ });
+
+ it('should have the property status (base name: "status")', function() {
+ // uncomment below and update the code to test the property status
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse200();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/InlineResponse2001.spec.js b/sdk/js/swagger/test/model/InlineResponse2001.spec.js
new file mode 100644
index 00000000000..5daadd3321e
--- /dev/null
+++ b/sdk/js/swagger/test/model/InlineResponse2001.spec.js
@@ -0,0 +1,98 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 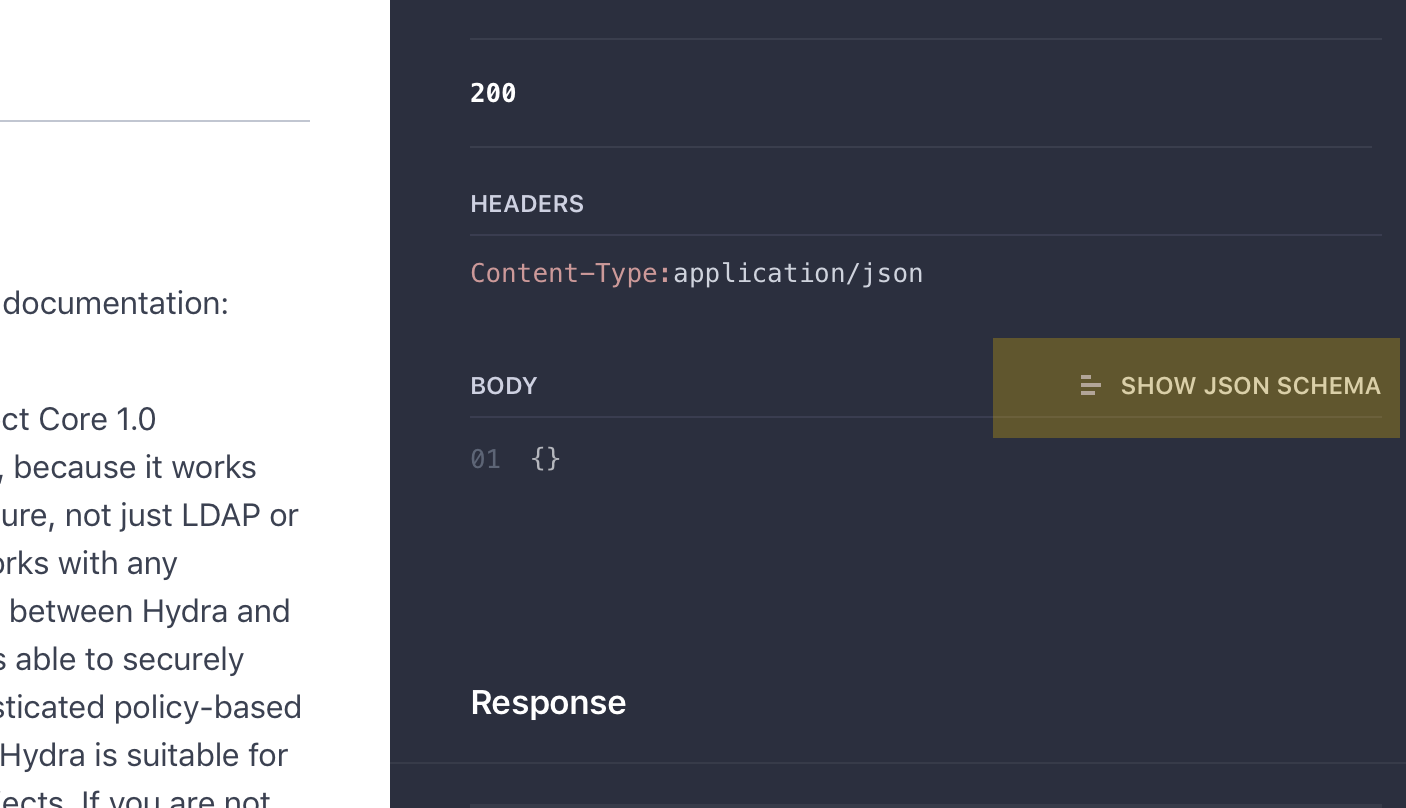 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.InlineResponse2001();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('InlineResponse2001', function() {
+ it('should create an instance of InlineResponse2001', function() {
+ // uncomment below and update the code to test InlineResponse2001
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse2001();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.InlineResponse2001);
+ });
+
+ it('should have the property accessToken (base name: "access_token")', function() {
+ // uncomment below and update the code to test the property accessToken
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse2001();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property expiresIn (base name: "expires_in")', function() {
+ // uncomment below and update the code to test the property expiresIn
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse2001();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property idToken (base name: "id_token")', function() {
+ // uncomment below and update the code to test the property idToken
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse2001();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property refreshToken (base name: "refresh_token")', function() {
+ // uncomment below and update the code to test the property refreshToken
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse2001();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property scope (base name: "scope")', function() {
+ // uncomment below and update the code to test the property scope
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse2001();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property tokenType (base name: "token_type")', function() {
+ // uncomment below and update the code to test the property tokenType
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse2001();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/InlineResponse401.spec.js b/sdk/js/swagger/test/model/InlineResponse401.spec.js
new file mode 100644
index 00000000000..f9d00fea10a
--- /dev/null
+++ b/sdk/js/swagger/test/model/InlineResponse401.spec.js
@@ -0,0 +1,98 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 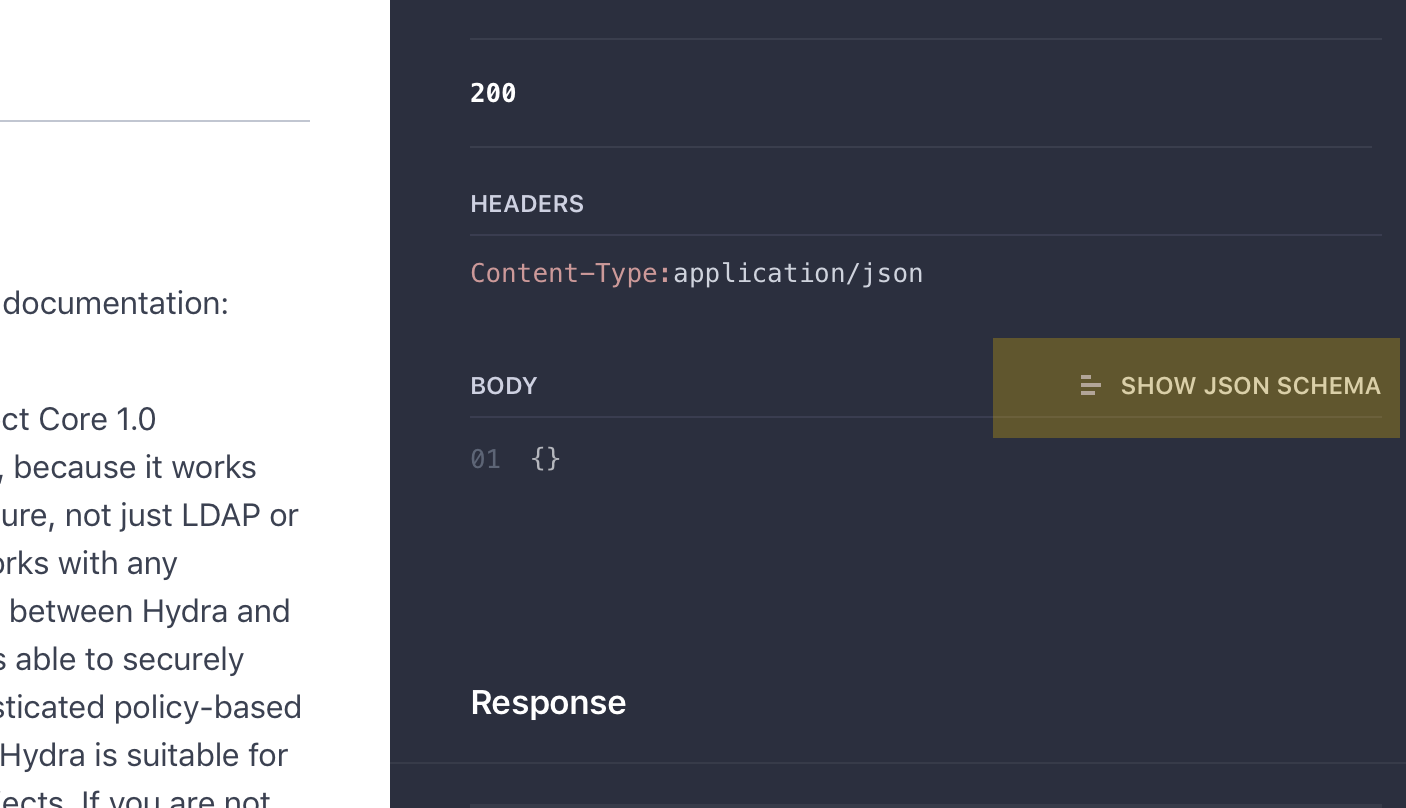 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.InlineResponse401();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('InlineResponse401', function() {
+ it('should create an instance of InlineResponse401', function() {
+ // uncomment below and update the code to test InlineResponse401
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse401();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.InlineResponse401);
+ });
+
+ it('should have the property code (base name: "code")', function() {
+ // uncomment below and update the code to test the property code
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse401();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property details (base name: "details")', function() {
+ // uncomment below and update the code to test the property details
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse401();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property message (base name: "message")', function() {
+ // uncomment below and update the code to test the property message
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse401();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property reason (base name: "reason")', function() {
+ // uncomment below and update the code to test the property reason
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse401();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property request (base name: "request")', function() {
+ // uncomment below and update the code to test the property request
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse401();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property status (base name: "status")', function() {
+ // uncomment below and update the code to test the property status
+ //var instane = new HydraOAuth2OpenIdConnectServer.InlineResponse401();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/JoseWebKeySetRequest.spec.js b/sdk/js/swagger/test/model/JoseWebKeySetRequest.spec.js
new file mode 100644
index 00000000000..01107845e40
--- /dev/null
+++ b/sdk/js/swagger/test/model/JoseWebKeySetRequest.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 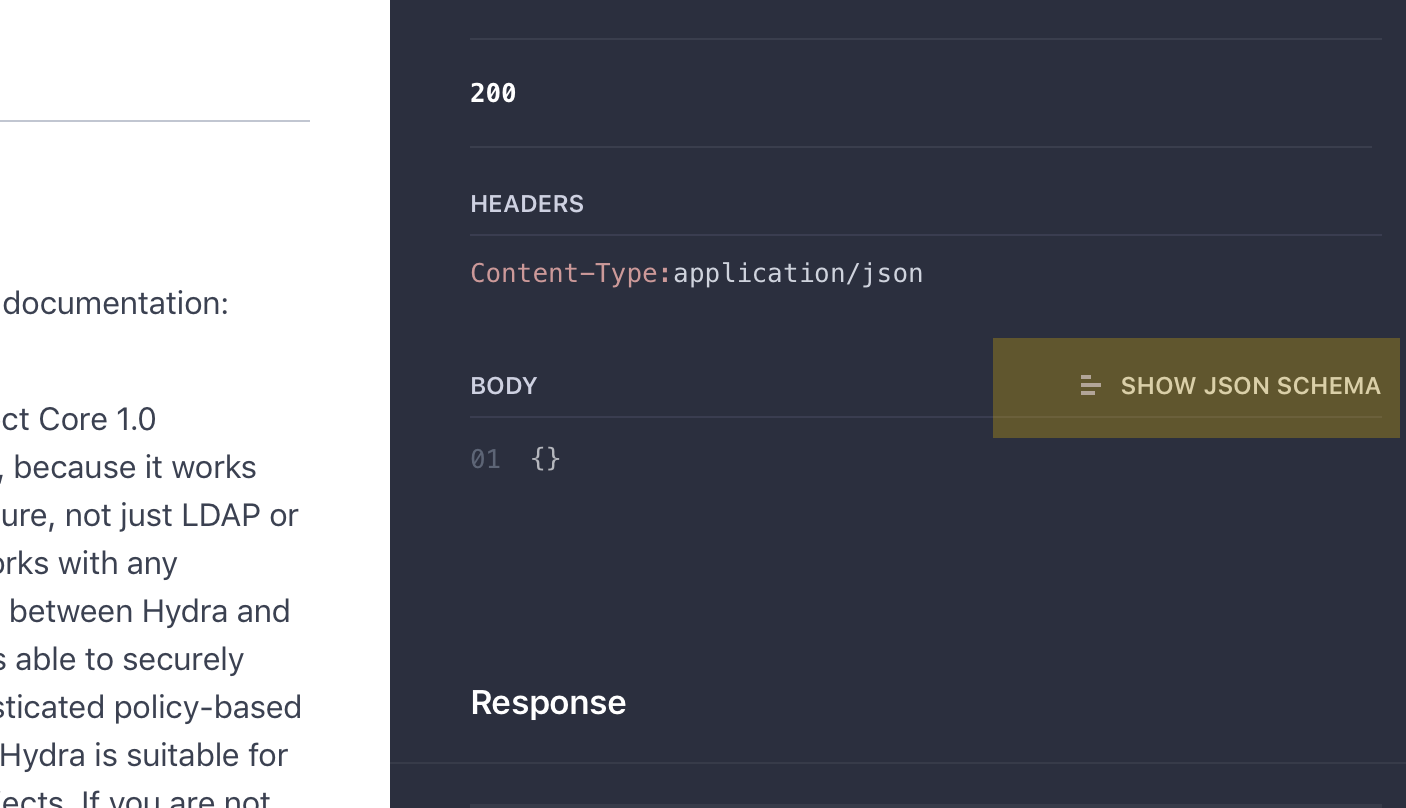 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.JoseWebKeySetRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('JoseWebKeySetRequest', function() {
+ it('should create an instance of JoseWebKeySetRequest', function() {
+ // uncomment below and update the code to test JoseWebKeySetRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.JoseWebKeySetRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.JoseWebKeySetRequest);
+ });
+
+ it('should have the property keys (base name: "keys")', function() {
+ // uncomment below and update the code to test the property keys
+ //var instane = new HydraOAuth2OpenIdConnectServer.JoseWebKeySetRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/JsonWebKey.spec.js b/sdk/js/swagger/test/model/JsonWebKey.spec.js
new file mode 100644
index 00000000000..8de500210bf
--- /dev/null
+++ b/sdk/js/swagger/test/model/JsonWebKey.spec.js
@@ -0,0 +1,164 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 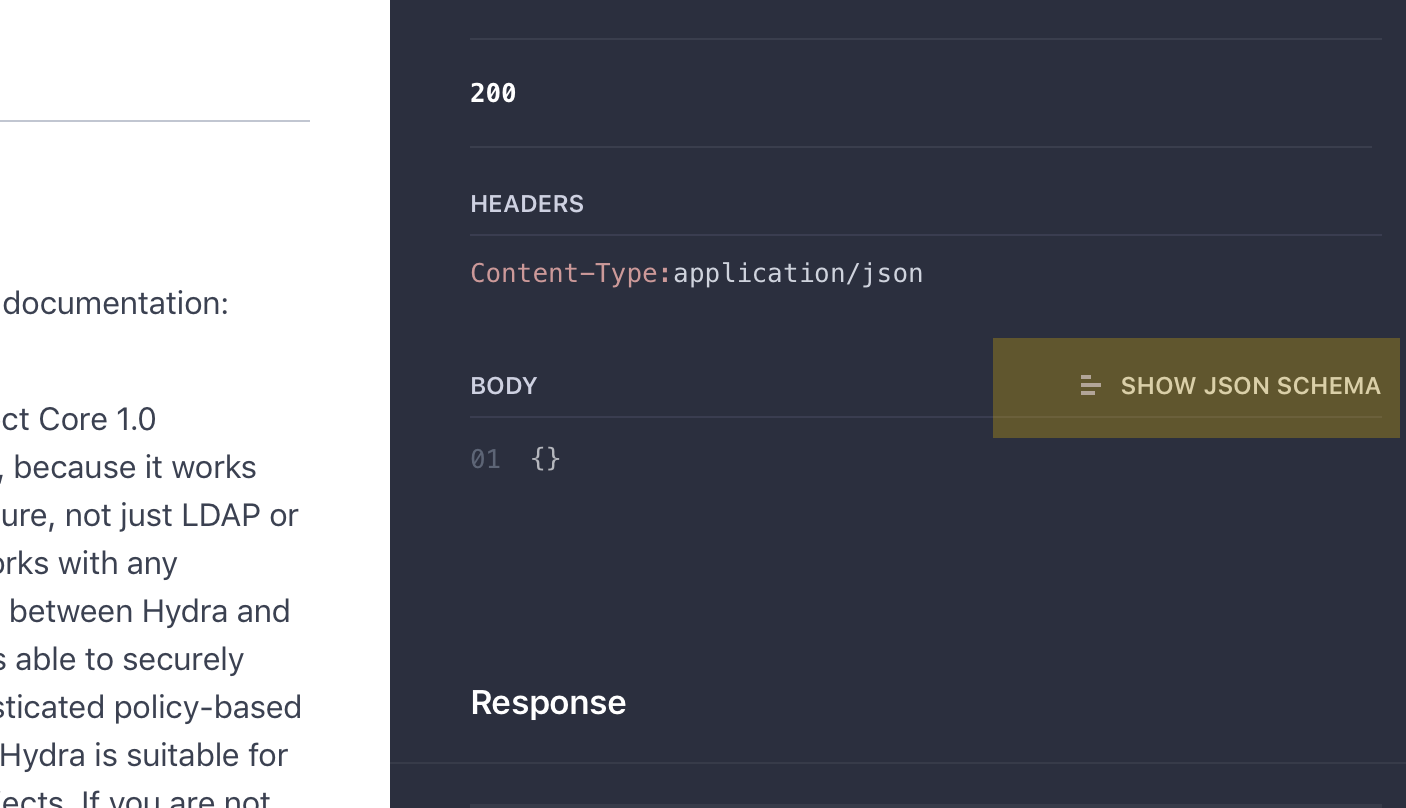 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('JsonWebKey', function() {
+ it('should create an instance of JsonWebKey', function() {
+ // uncomment below and update the code to test JsonWebKey
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.JsonWebKey);
+ });
+
+ it('should have the property alg (base name: "alg")', function() {
+ // uncomment below and update the code to test the property alg
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property crv (base name: "crv")', function() {
+ // uncomment below and update the code to test the property crv
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property d (base name: "d")', function() {
+ // uncomment below and update the code to test the property d
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property dp (base name: "dp")', function() {
+ // uncomment below and update the code to test the property dp
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property dq (base name: "dq")', function() {
+ // uncomment below and update the code to test the property dq
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property e (base name: "e")', function() {
+ // uncomment below and update the code to test the property e
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property k (base name: "k")', function() {
+ // uncomment below and update the code to test the property k
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property kid (base name: "kid")', function() {
+ // uncomment below and update the code to test the property kid
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property kty (base name: "kty")', function() {
+ // uncomment below and update the code to test the property kty
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property n (base name: "n")', function() {
+ // uncomment below and update the code to test the property n
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property p (base name: "p")', function() {
+ // uncomment below and update the code to test the property p
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property q (base name: "q")', function() {
+ // uncomment below and update the code to test the property q
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property qi (base name: "qi")', function() {
+ // uncomment below and update the code to test the property qi
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property use (base name: "use")', function() {
+ // uncomment below and update the code to test the property use
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property x (base name: "x")', function() {
+ // uncomment below and update the code to test the property x
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property x5c (base name: "x5c")', function() {
+ // uncomment below and update the code to test the property x5c
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property y (base name: "y")', function() {
+ // uncomment below and update the code to test the property y
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKey();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/JsonWebKeySet.spec.js b/sdk/js/swagger/test/model/JsonWebKeySet.spec.js
new file mode 100644
index 00000000000..a32fb62db59
--- /dev/null
+++ b/sdk/js/swagger/test/model/JsonWebKeySet.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 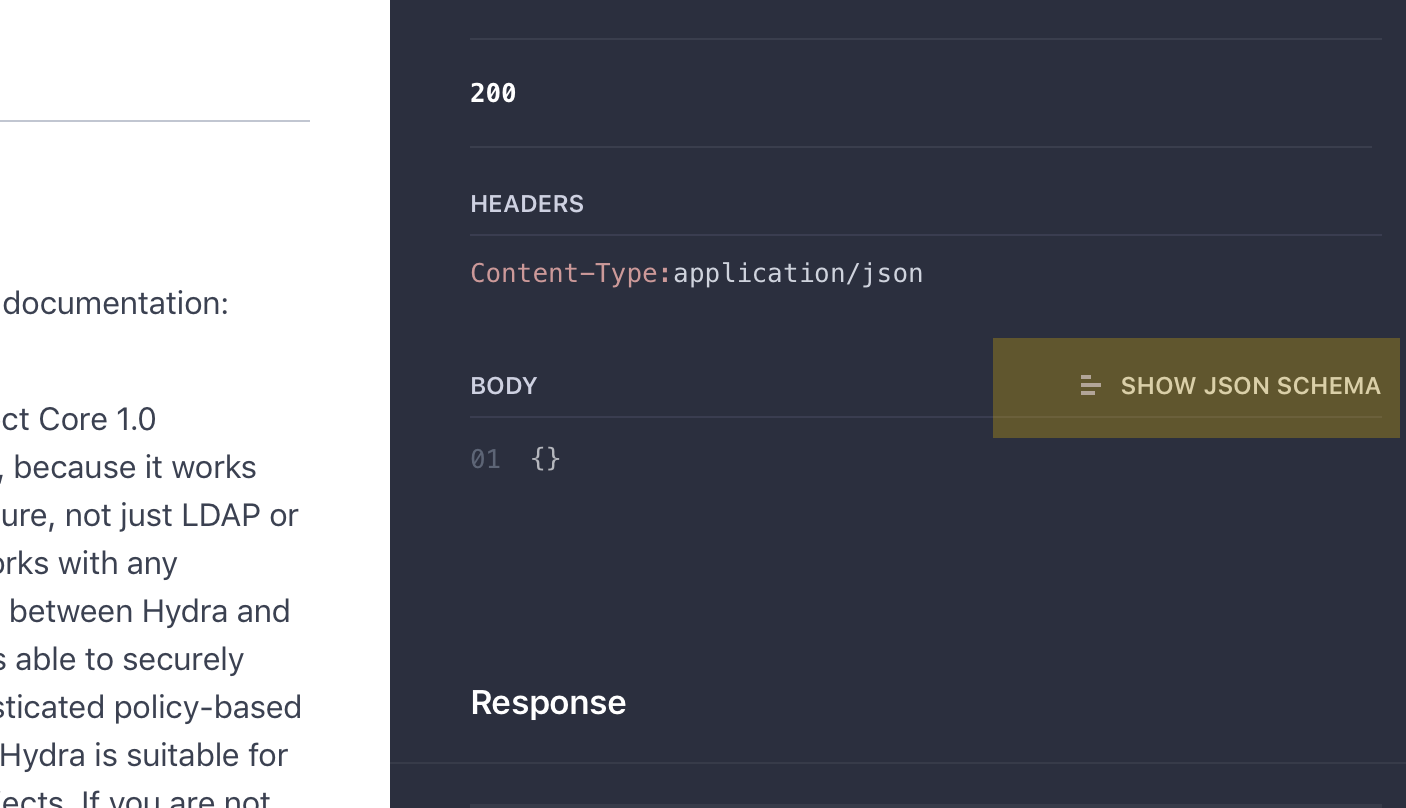 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.JsonWebKeySet();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('JsonWebKeySet', function() {
+ it('should create an instance of JsonWebKeySet', function() {
+ // uncomment below and update the code to test JsonWebKeySet
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKeySet();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.JsonWebKeySet);
+ });
+
+ it('should have the property keys (base name: "keys")', function() {
+ // uncomment below and update the code to test the property keys
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKeySet();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/JsonWebKeySetGeneratorRequest.spec.js b/sdk/js/swagger/test/model/JsonWebKeySetGeneratorRequest.spec.js
new file mode 100644
index 00000000000..7be64492e3b
--- /dev/null
+++ b/sdk/js/swagger/test/model/JsonWebKeySetGeneratorRequest.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 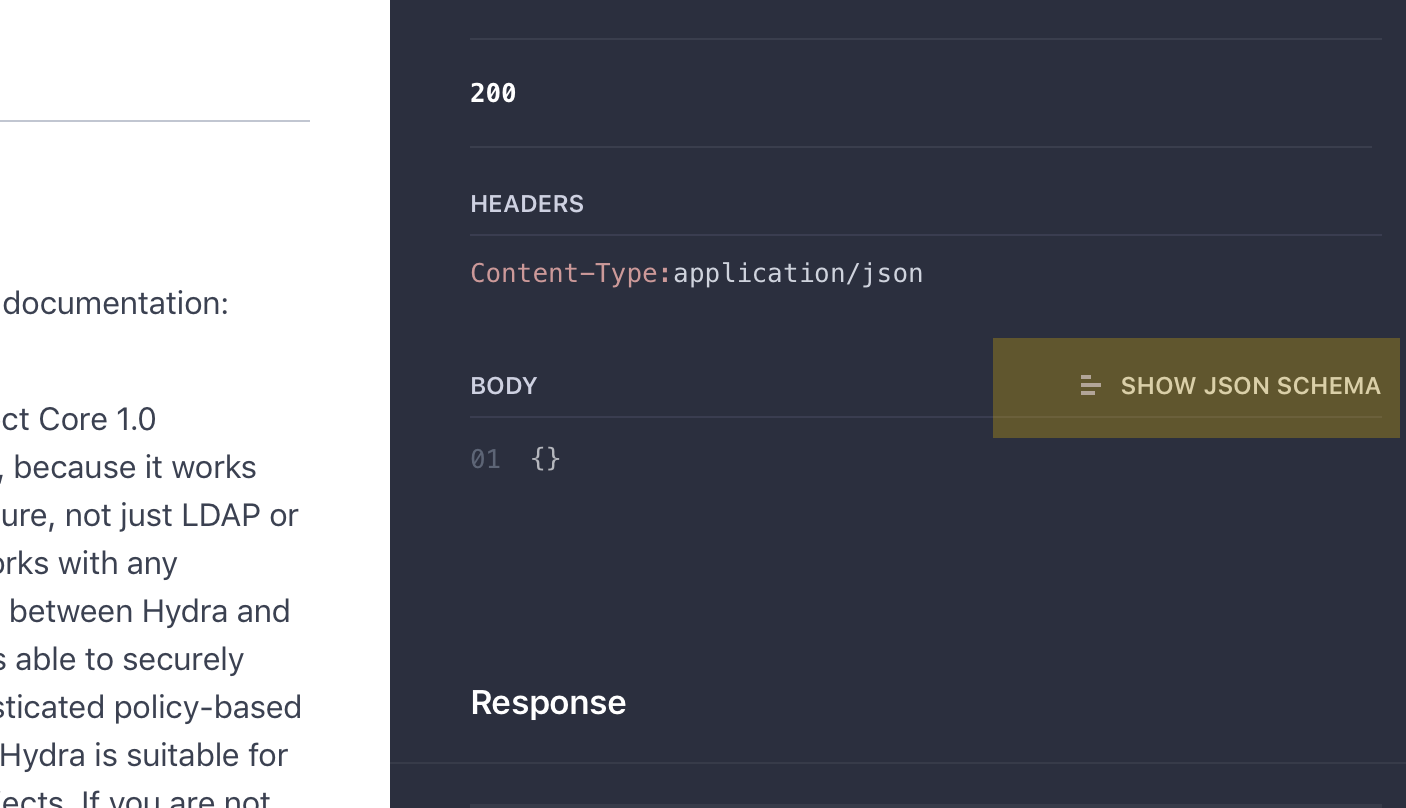 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('JsonWebKeySetGeneratorRequest', function() {
+ it('should create an instance of JsonWebKeySetGeneratorRequest', function() {
+ // uncomment below and update the code to test JsonWebKeySetGeneratorRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest);
+ });
+
+ it('should have the property alg (base name: "alg")', function() {
+ // uncomment below and update the code to test the property alg
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property kid (base name: "kid")', function() {
+ // uncomment below and update the code to test the property kid
+ //var instane = new HydraOAuth2OpenIdConnectServer.JsonWebKeySetGeneratorRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/KeyGenerator.spec.js b/sdk/js/swagger/test/model/KeyGenerator.spec.js
new file mode 100644
index 00000000000..048d6c6be63
--- /dev/null
+++ b/sdk/js/swagger/test/model/KeyGenerator.spec.js
@@ -0,0 +1,62 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 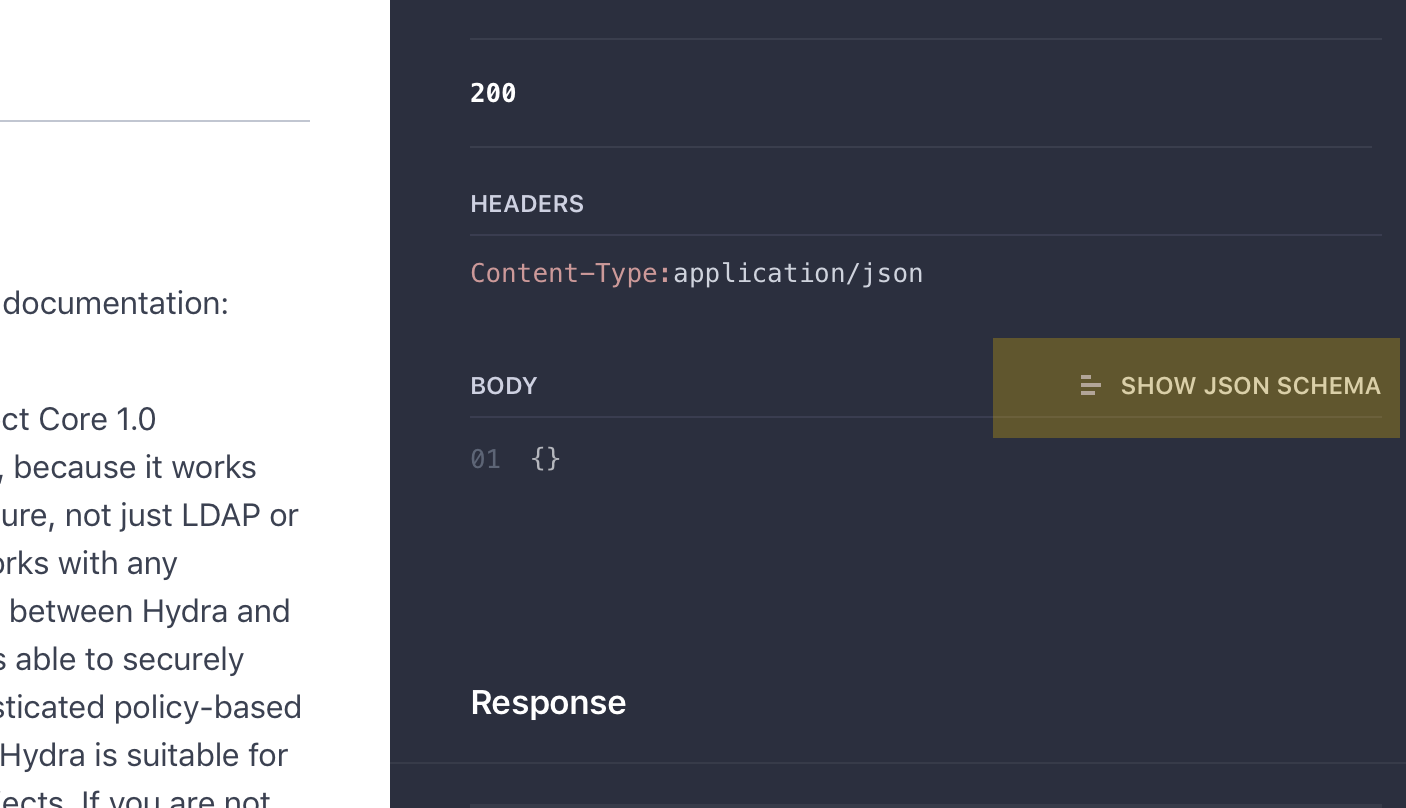 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.KeyGenerator();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('KeyGenerator', function() {
+ it('should create an instance of KeyGenerator', function() {
+ // uncomment below and update the code to test KeyGenerator
+ //var instane = new HydraOAuth2OpenIdConnectServer.KeyGenerator();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.KeyGenerator);
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/Manager.spec.js b/sdk/js/swagger/test/model/Manager.spec.js
new file mode 100644
index 00000000000..5550416a962
--- /dev/null
+++ b/sdk/js/swagger/test/model/Manager.spec.js
@@ -0,0 +1,62 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 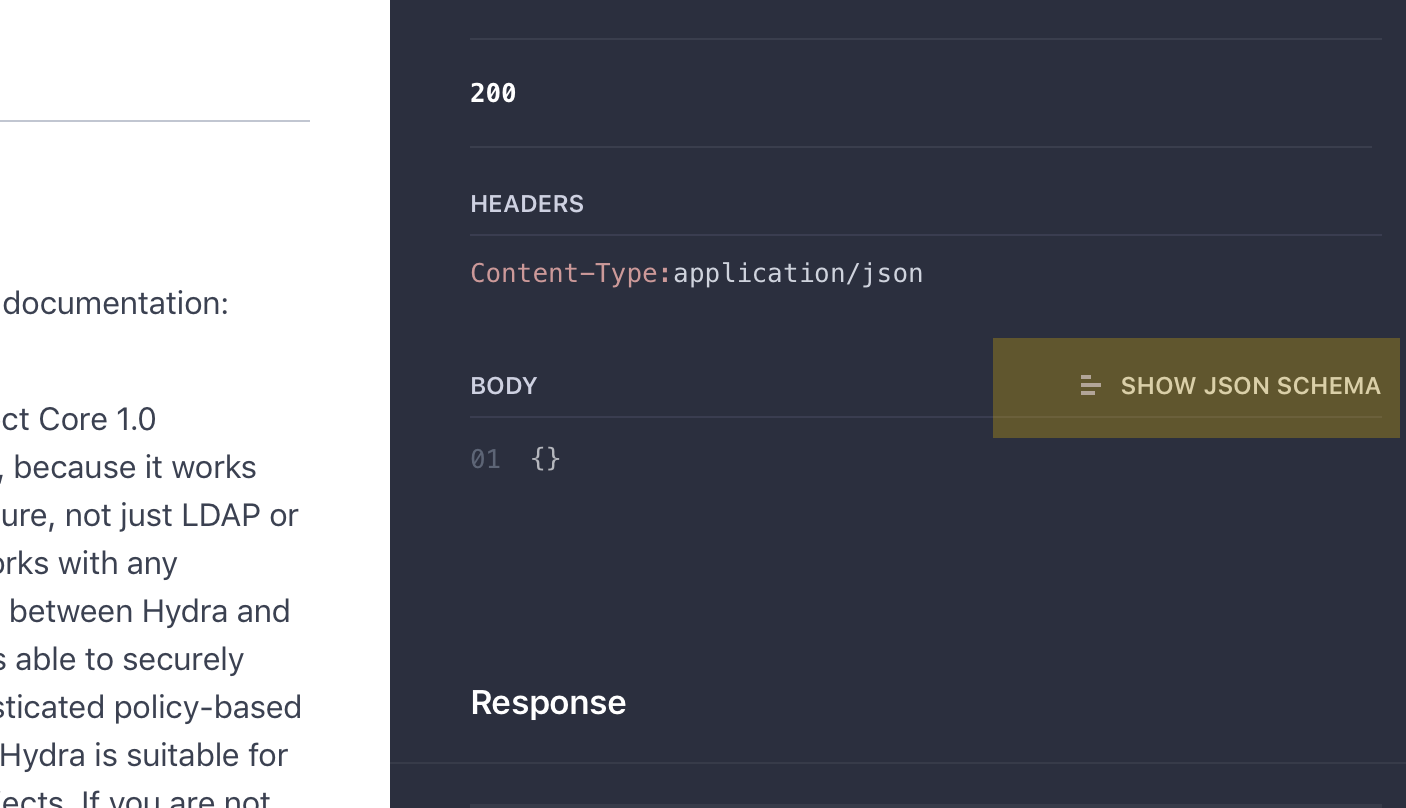 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.Manager();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('Manager', function() {
+ it('should create an instance of Manager', function() {
+ // uncomment below and update the code to test Manager
+ //var instane = new HydraOAuth2OpenIdConnectServer.Manager();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.Manager);
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/OAuth2Client.spec.js b/sdk/js/swagger/test/model/OAuth2Client.spec.js
new file mode 100644
index 00000000000..4f29614f37b
--- /dev/null
+++ b/sdk/js/swagger/test/model/OAuth2Client.spec.js
@@ -0,0 +1,146 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 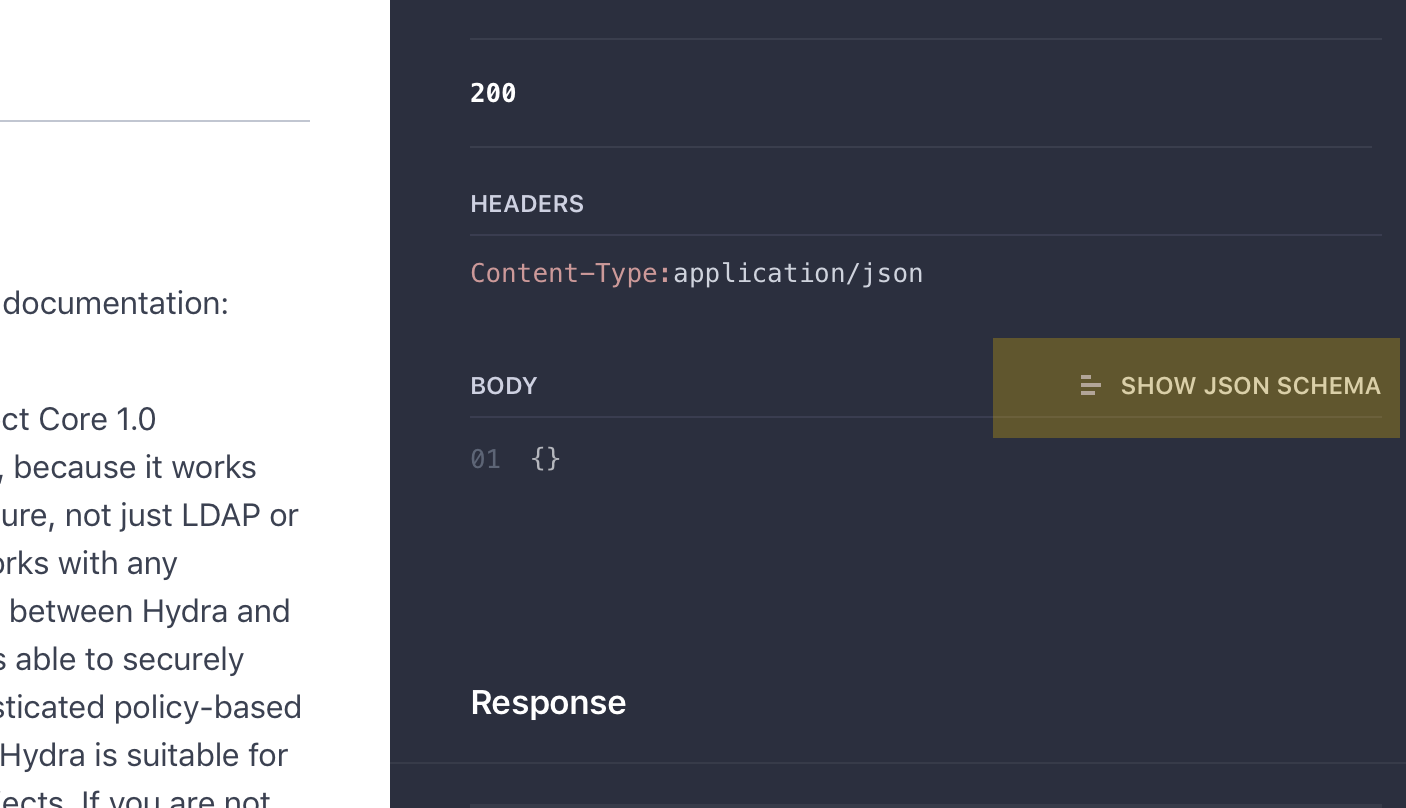 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('OAuth2Client', function() {
+ it('should create an instance of OAuth2Client', function() {
+ // uncomment below and update the code to test OAuth2Client
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.OAuth2Client);
+ });
+
+ it('should have the property clientName (base name: "client_name")', function() {
+ // uncomment below and update the code to test the property clientName
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property clientSecret (base name: "client_secret")', function() {
+ // uncomment below and update the code to test the property clientSecret
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property clientUri (base name: "client_uri")', function() {
+ // uncomment below and update the code to test the property clientUri
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property contacts (base name: "contacts")', function() {
+ // uncomment below and update the code to test the property contacts
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property grantTypes (base name: "grant_types")', function() {
+ // uncomment below and update the code to test the property grantTypes
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property logoUri (base name: "logo_uri")', function() {
+ // uncomment below and update the code to test the property logoUri
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property owner (base name: "owner")', function() {
+ // uncomment below and update the code to test the property owner
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property policyUri (base name: "policy_uri")', function() {
+ // uncomment below and update the code to test the property policyUri
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property _public (base name: "public")', function() {
+ // uncomment below and update the code to test the property _public
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property redirectUris (base name: "redirect_uris")', function() {
+ // uncomment below and update the code to test the property redirectUris
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property responseTypes (base name: "response_types")', function() {
+ // uncomment below and update the code to test the property responseTypes
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property scope (base name: "scope")', function() {
+ // uncomment below and update the code to test the property scope
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property tosUri (base name: "tos_uri")', function() {
+ // uncomment below and update the code to test the property tosUri
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2Client();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/OAuth2TokenIntrospection.spec.js b/sdk/js/swagger/test/model/OAuth2TokenIntrospection.spec.js
new file mode 100644
index 00000000000..12d45305b41
--- /dev/null
+++ b/sdk/js/swagger/test/model/OAuth2TokenIntrospection.spec.js
@@ -0,0 +1,128 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 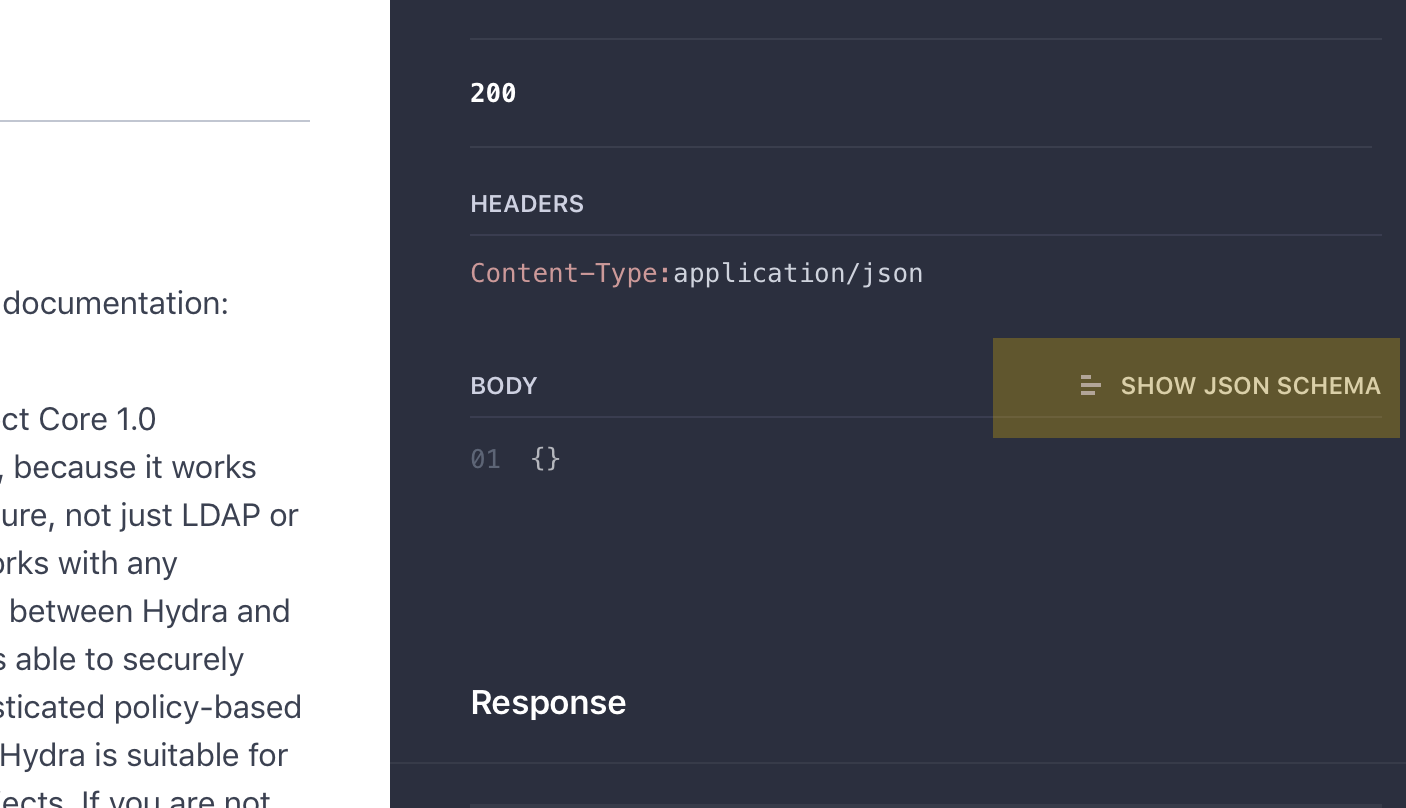 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('OAuth2TokenIntrospection', function() {
+ it('should create an instance of OAuth2TokenIntrospection', function() {
+ // uncomment below and update the code to test OAuth2TokenIntrospection
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection);
+ });
+
+ it('should have the property active (base name: "active")', function() {
+ // uncomment below and update the code to test the property active
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property aud (base name: "aud")', function() {
+ // uncomment below and update the code to test the property aud
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property clientId (base name: "client_id")', function() {
+ // uncomment below and update the code to test the property clientId
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property exp (base name: "exp")', function() {
+ // uncomment below and update the code to test the property exp
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property ext (base name: "ext")', function() {
+ // uncomment below and update the code to test the property ext
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property iat (base name: "iat")', function() {
+ // uncomment below and update the code to test the property iat
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property iss (base name: "iss")', function() {
+ // uncomment below and update the code to test the property iss
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property nbf (base name: "nbf")', function() {
+ // uncomment below and update the code to test the property nbf
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property scope (base name: "scope")', function() {
+ // uncomment below and update the code to test the property scope
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property sub (base name: "sub")', function() {
+ // uncomment below and update the code to test the property sub
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property username (base name: "username")', function() {
+ // uncomment below and update the code to test the property username
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2TokenIntrospection();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/OAuth2consentRequest.spec.js b/sdk/js/swagger/test/model/OAuth2consentRequest.spec.js
new file mode 100644
index 00000000000..8b2df48ec04
--- /dev/null
+++ b/sdk/js/swagger/test/model/OAuth2consentRequest.spec.js
@@ -0,0 +1,86 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 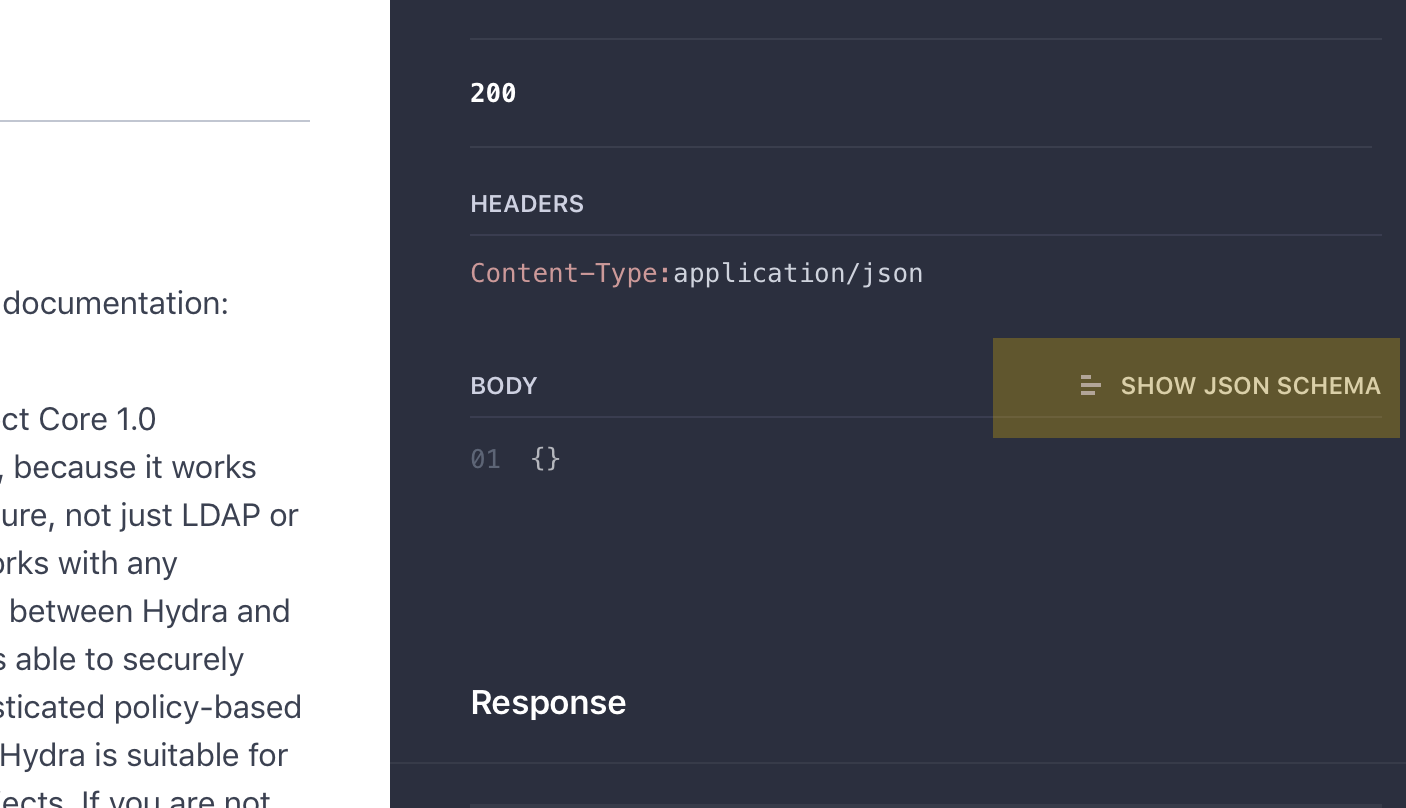 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.OAuth2consentRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('OAuth2consentRequest', function() {
+ it('should create an instance of OAuth2consentRequest', function() {
+ // uncomment below and update the code to test OAuth2consentRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2consentRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.OAuth2consentRequest);
+ });
+
+ it('should have the property audience (base name: "audience")', function() {
+ // uncomment below and update the code to test the property audience
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2consentRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2consentRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property redirectUrl (base name: "redirectUrl")', function() {
+ // uncomment below and update the code to test the property redirectUrl
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2consentRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property requestedScopes (base name: "requestedScopes")', function() {
+ // uncomment below and update the code to test the property requestedScopes
+ //var instane = new HydraOAuth2OpenIdConnectServer.OAuth2consentRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/Policy.spec.js b/sdk/js/swagger/test/model/Policy.spec.js
new file mode 100644
index 00000000000..588af35d4ee
--- /dev/null
+++ b/sdk/js/swagger/test/model/Policy.spec.js
@@ -0,0 +1,104 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 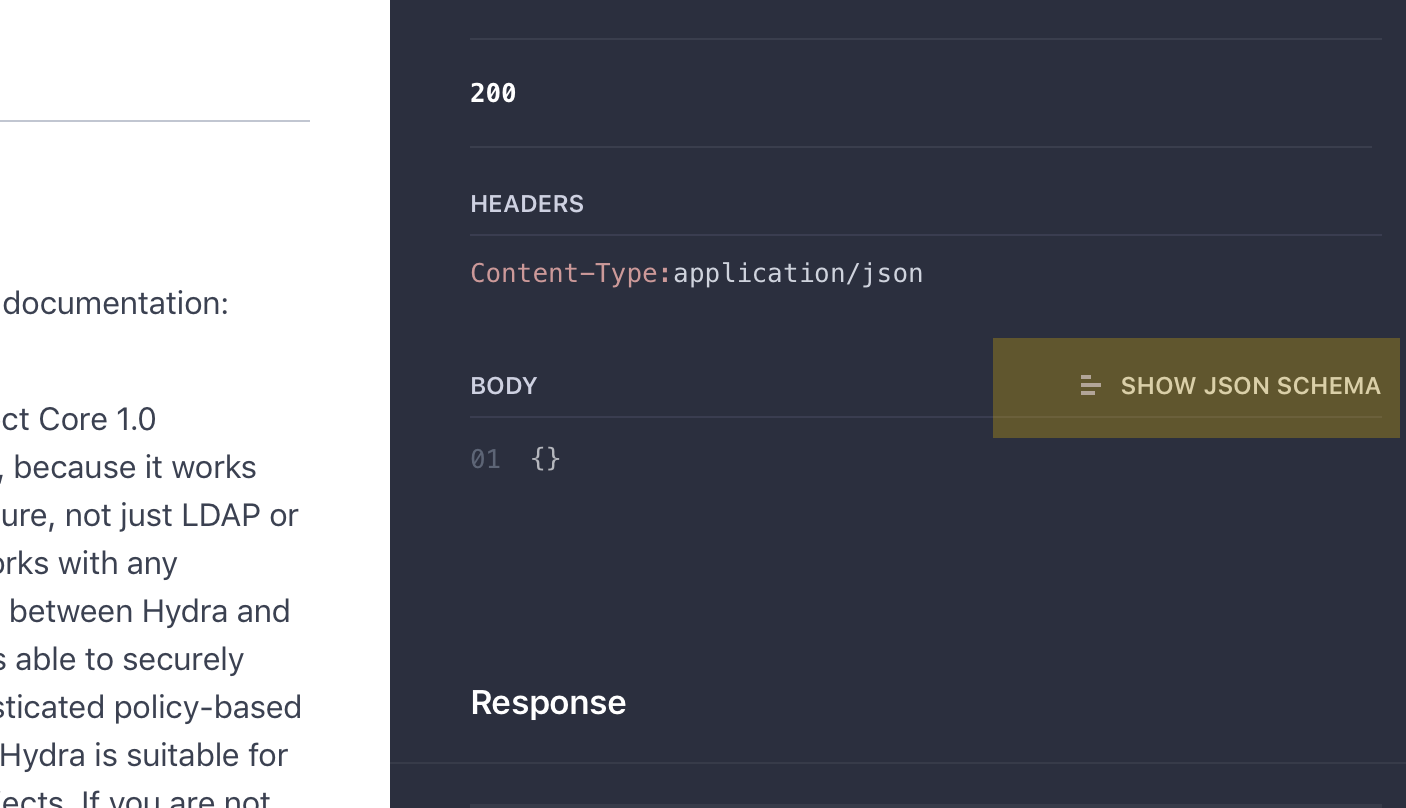 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.Policy();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('Policy', function() {
+ it('should create an instance of Policy', function() {
+ // uncomment below and update the code to test Policy
+ //var instane = new HydraOAuth2OpenIdConnectServer.Policy();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.Policy);
+ });
+
+ it('should have the property actions (base name: "actions")', function() {
+ // uncomment below and update the code to test the property actions
+ //var instane = new HydraOAuth2OpenIdConnectServer.Policy();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property conditions (base name: "conditions")', function() {
+ // uncomment below and update the code to test the property conditions
+ //var instane = new HydraOAuth2OpenIdConnectServer.Policy();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property description (base name: "description")', function() {
+ // uncomment below and update the code to test the property description
+ //var instane = new HydraOAuth2OpenIdConnectServer.Policy();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property effect (base name: "effect")', function() {
+ // uncomment below and update the code to test the property effect
+ //var instane = new HydraOAuth2OpenIdConnectServer.Policy();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.Policy();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property resources (base name: "resources")', function() {
+ // uncomment below and update the code to test the property resources
+ //var instane = new HydraOAuth2OpenIdConnectServer.Policy();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property subjects (base name: "subjects")', function() {
+ // uncomment below and update the code to test the property subjects
+ //var instane = new HydraOAuth2OpenIdConnectServer.Policy();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/PolicyConditions.spec.js b/sdk/js/swagger/test/model/PolicyConditions.spec.js
new file mode 100644
index 00000000000..9a0d2ab5463
--- /dev/null
+++ b/sdk/js/swagger/test/model/PolicyConditions.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 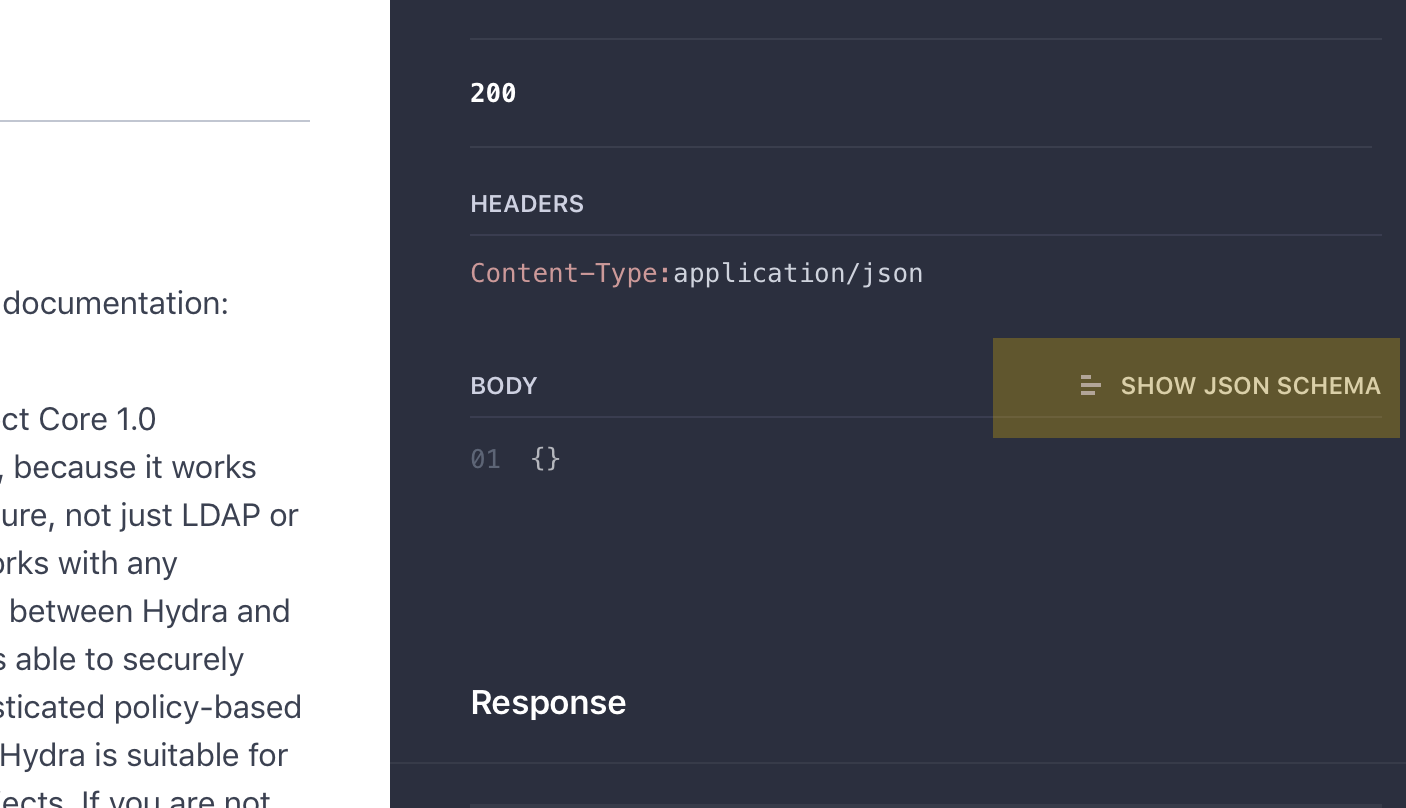 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.PolicyConditions();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('PolicyConditions', function() {
+ it('should create an instance of PolicyConditions', function() {
+ // uncomment below and update the code to test PolicyConditions
+ //var instane = new HydraOAuth2OpenIdConnectServer.PolicyConditions();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.PolicyConditions);
+ });
+
+ it('should have the property options (base name: "options")', function() {
+ // uncomment below and update the code to test the property options
+ //var instane = new HydraOAuth2OpenIdConnectServer.PolicyConditions();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property type (base name: "type")', function() {
+ // uncomment below and update the code to test the property type
+ //var instane = new HydraOAuth2OpenIdConnectServer.PolicyConditions();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/RawMessage.spec.js b/sdk/js/swagger/test/model/RawMessage.spec.js
new file mode 100644
index 00000000000..e89613cb20c
--- /dev/null
+++ b/sdk/js/swagger/test/model/RawMessage.spec.js
@@ -0,0 +1,62 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 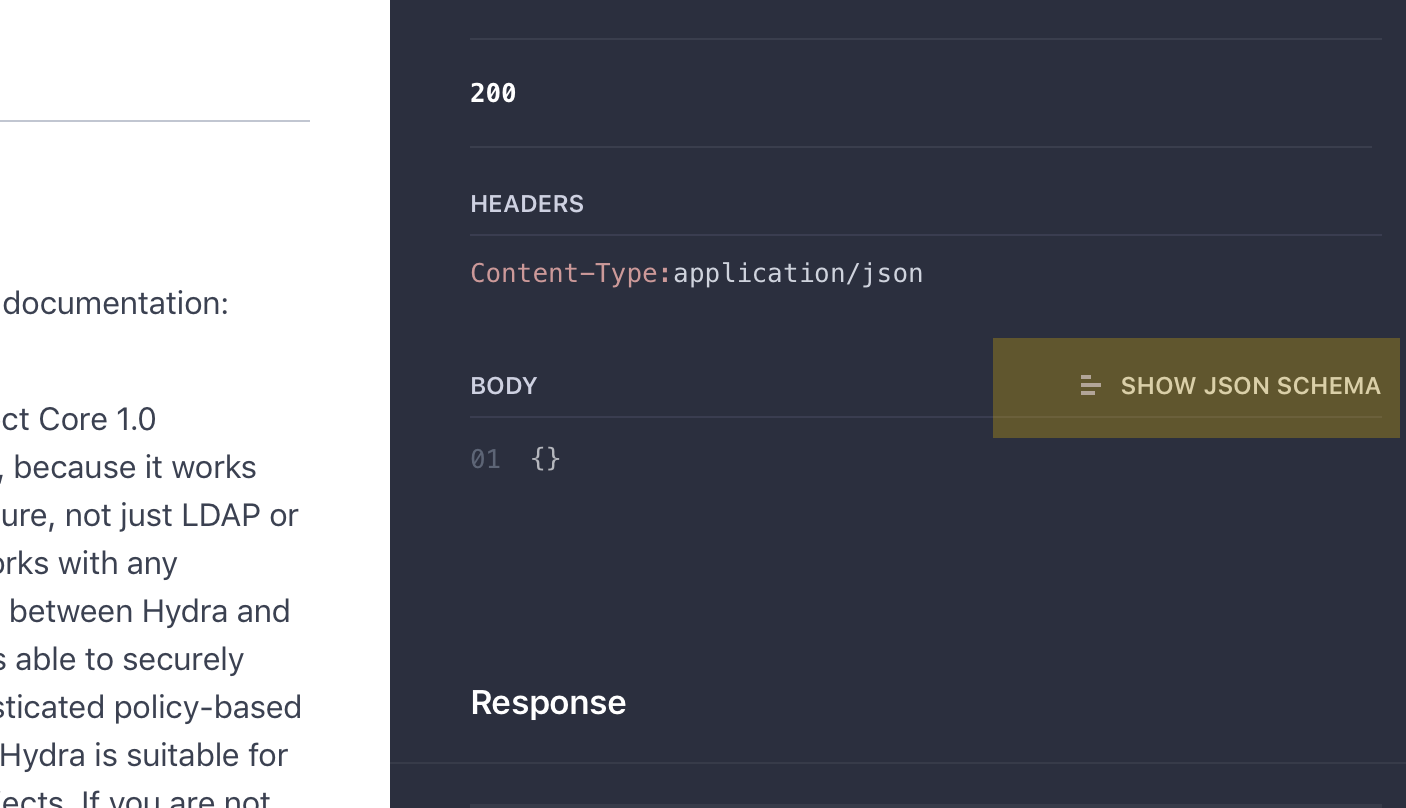 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.RawMessage();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('RawMessage', function() {
+ it('should create an instance of RawMessage', function() {
+ // uncomment below and update the code to test RawMessage
+ //var instane = new HydraOAuth2OpenIdConnectServer.RawMessage();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.RawMessage);
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerAcceptConsentRequest.spec.js b/sdk/js/swagger/test/model/SwaggerAcceptConsentRequest.spec.js
new file mode 100644
index 00000000000..10586306028
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerAcceptConsentRequest.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 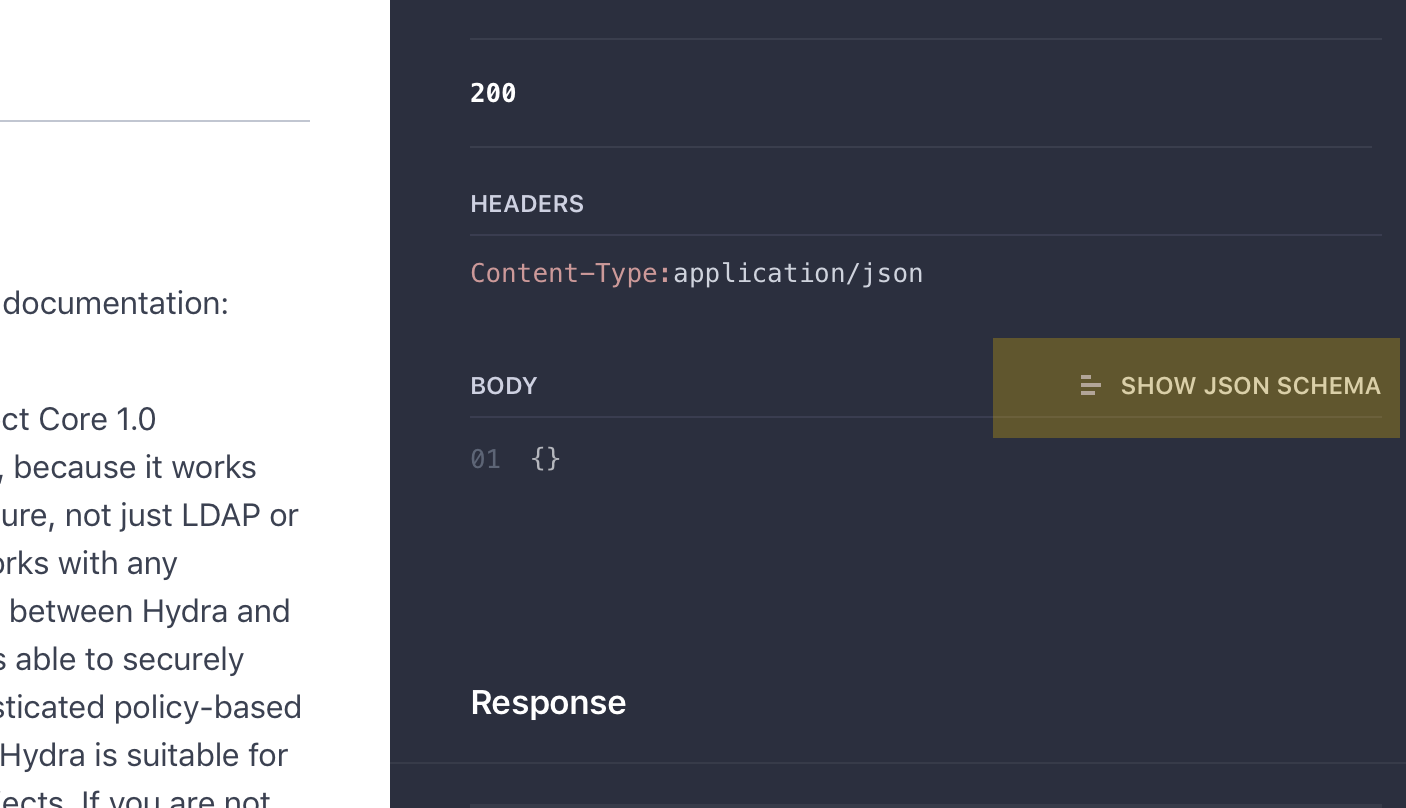 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerAcceptConsentRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerAcceptConsentRequest', function() {
+ it('should create an instance of SwaggerAcceptConsentRequest', function() {
+ // uncomment below and update the code to test SwaggerAcceptConsentRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerAcceptConsentRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerAcceptConsentRequest);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerAcceptConsentRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerAcceptConsentRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerCreatePolicyParameters.spec.js b/sdk/js/swagger/test/model/SwaggerCreatePolicyParameters.spec.js
new file mode 100644
index 00000000000..7ba01e6088e
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerCreatePolicyParameters.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 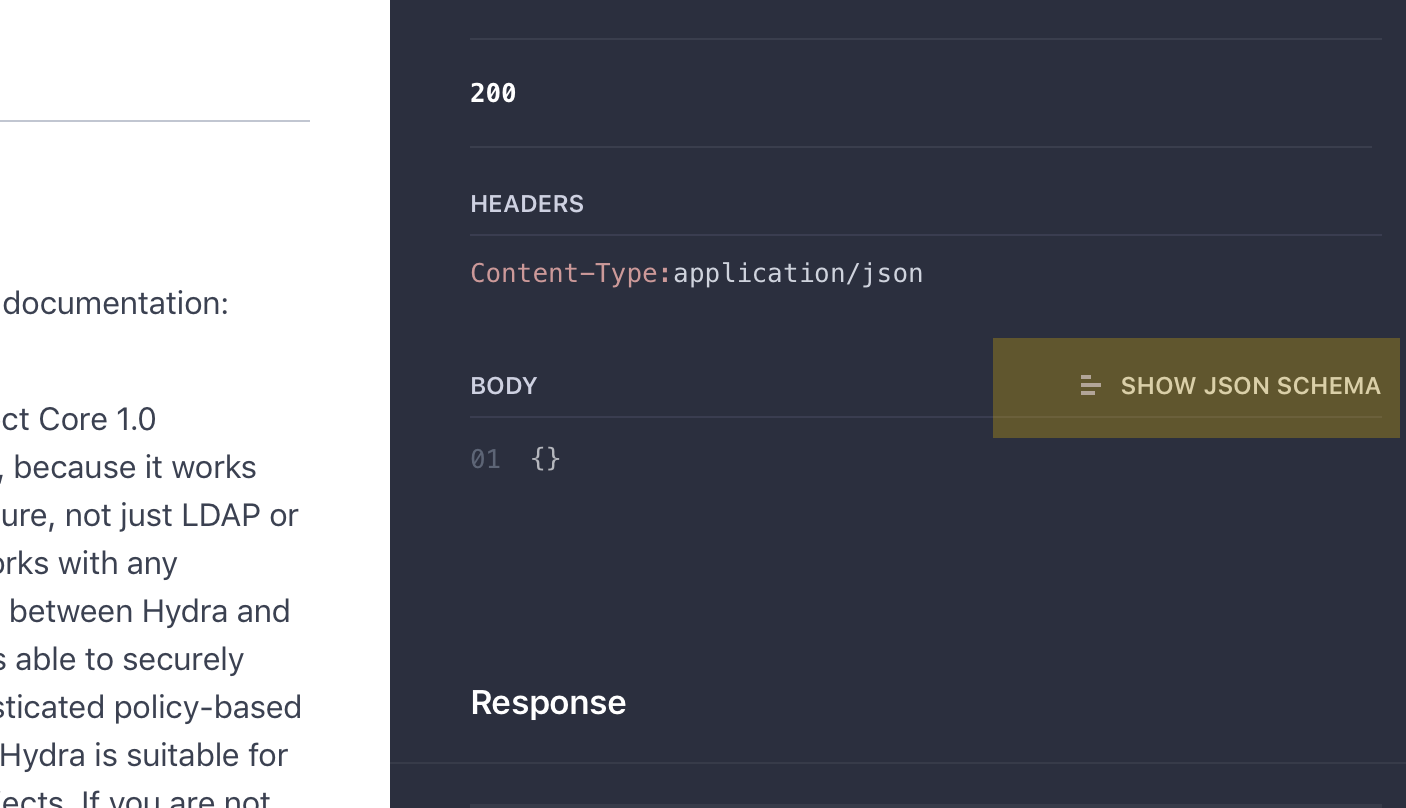 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerCreatePolicyParameters();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerCreatePolicyParameters', function() {
+ it('should create an instance of SwaggerCreatePolicyParameters', function() {
+ // uncomment below and update the code to test SwaggerCreatePolicyParameters
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerCreatePolicyParameters();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerCreatePolicyParameters);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerCreatePolicyParameters();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerDoesWardenAllowAccessRequestParameters.spec.js b/sdk/js/swagger/test/model/SwaggerDoesWardenAllowAccessRequestParameters.spec.js
new file mode 100644
index 00000000000..15da6fc2303
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerDoesWardenAllowAccessRequestParameters.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 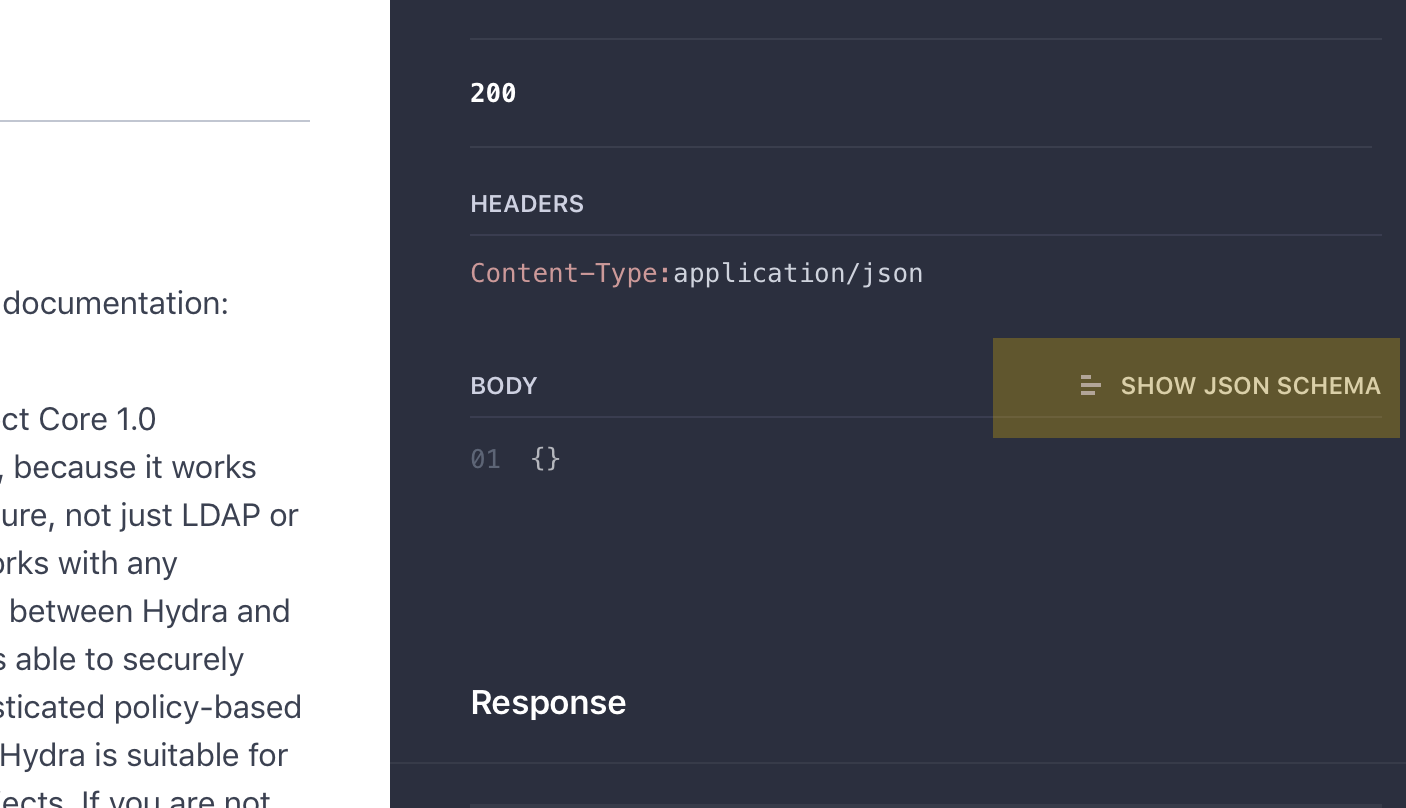 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowAccessRequestParameters();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerDoesWardenAllowAccessRequestParameters', function() {
+ it('should create an instance of SwaggerDoesWardenAllowAccessRequestParameters', function() {
+ // uncomment below and update the code to test SwaggerDoesWardenAllowAccessRequestParameters
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowAccessRequestParameters();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowAccessRequestParameters);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowAccessRequestParameters();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerDoesWardenAllowTokenAccessRequestParameters.spec.js b/sdk/js/swagger/test/model/SwaggerDoesWardenAllowTokenAccessRequestParameters.spec.js
new file mode 100644
index 00000000000..4dcbe6a88a9
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerDoesWardenAllowTokenAccessRequestParameters.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 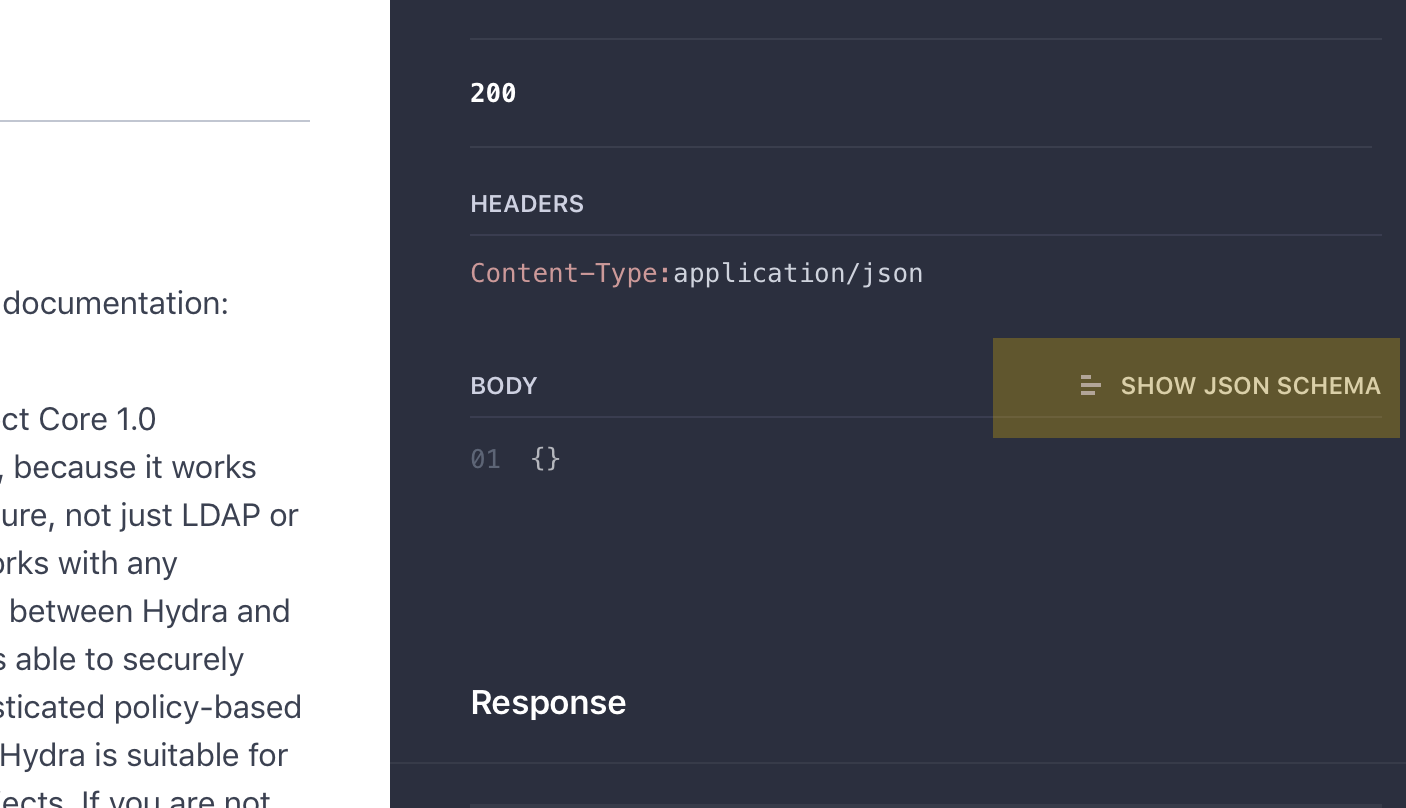 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowTokenAccessRequestParameters();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerDoesWardenAllowTokenAccessRequestParameters', function() {
+ it('should create an instance of SwaggerDoesWardenAllowTokenAccessRequestParameters', function() {
+ // uncomment below and update the code to test SwaggerDoesWardenAllowTokenAccessRequestParameters
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowTokenAccessRequestParameters();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowTokenAccessRequestParameters);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerDoesWardenAllowTokenAccessRequestParameters();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerGetPolicyParameters.spec.js b/sdk/js/swagger/test/model/SwaggerGetPolicyParameters.spec.js
new file mode 100644
index 00000000000..870778b7617
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerGetPolicyParameters.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 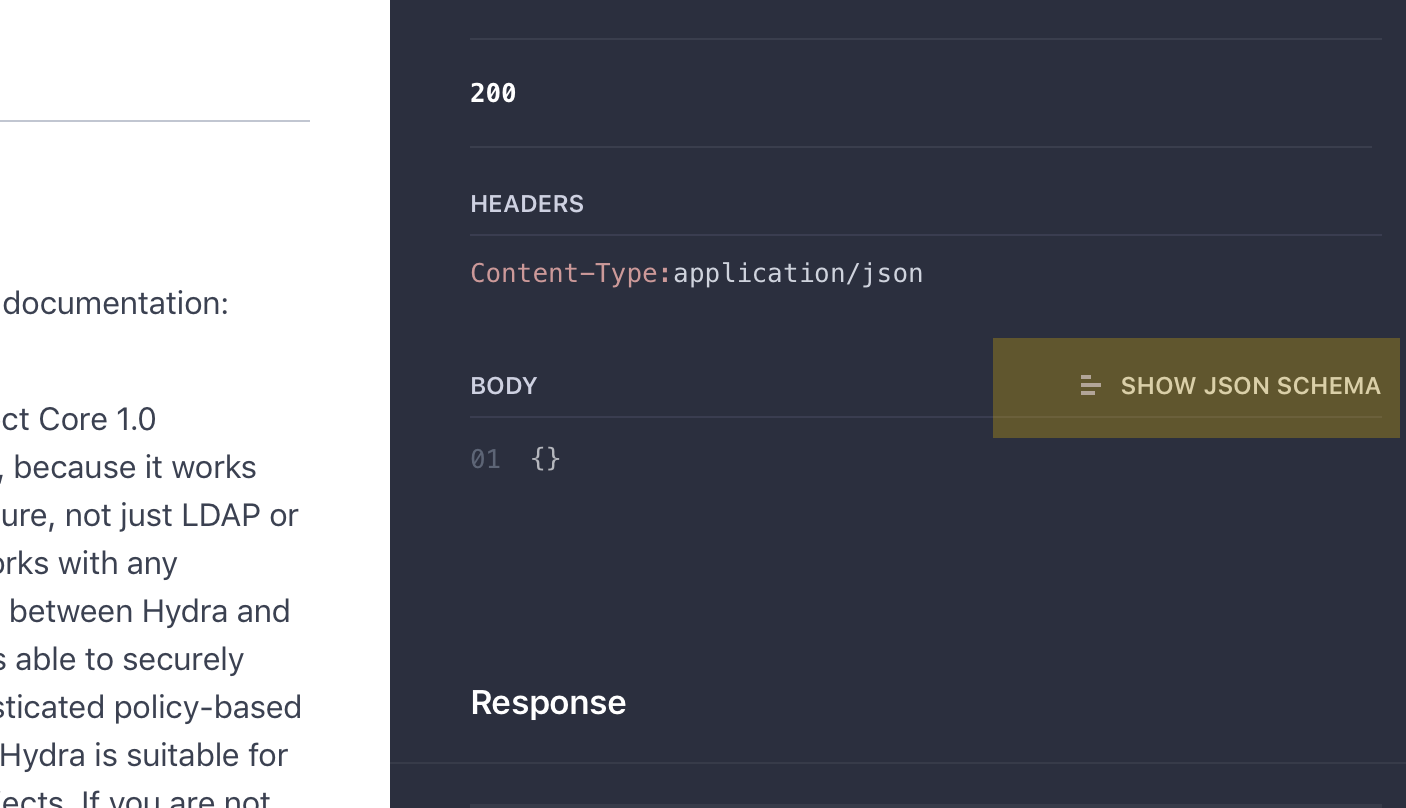 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerGetPolicyParameters();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerGetPolicyParameters', function() {
+ it('should create an instance of SwaggerGetPolicyParameters', function() {
+ // uncomment below and update the code to test SwaggerGetPolicyParameters
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerGetPolicyParameters();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerGetPolicyParameters);
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerGetPolicyParameters();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerJsonWebKeyQuery.spec.js b/sdk/js/swagger/test/model/SwaggerJsonWebKeyQuery.spec.js
new file mode 100644
index 00000000000..7e0f2a2d9f5
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerJsonWebKeyQuery.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 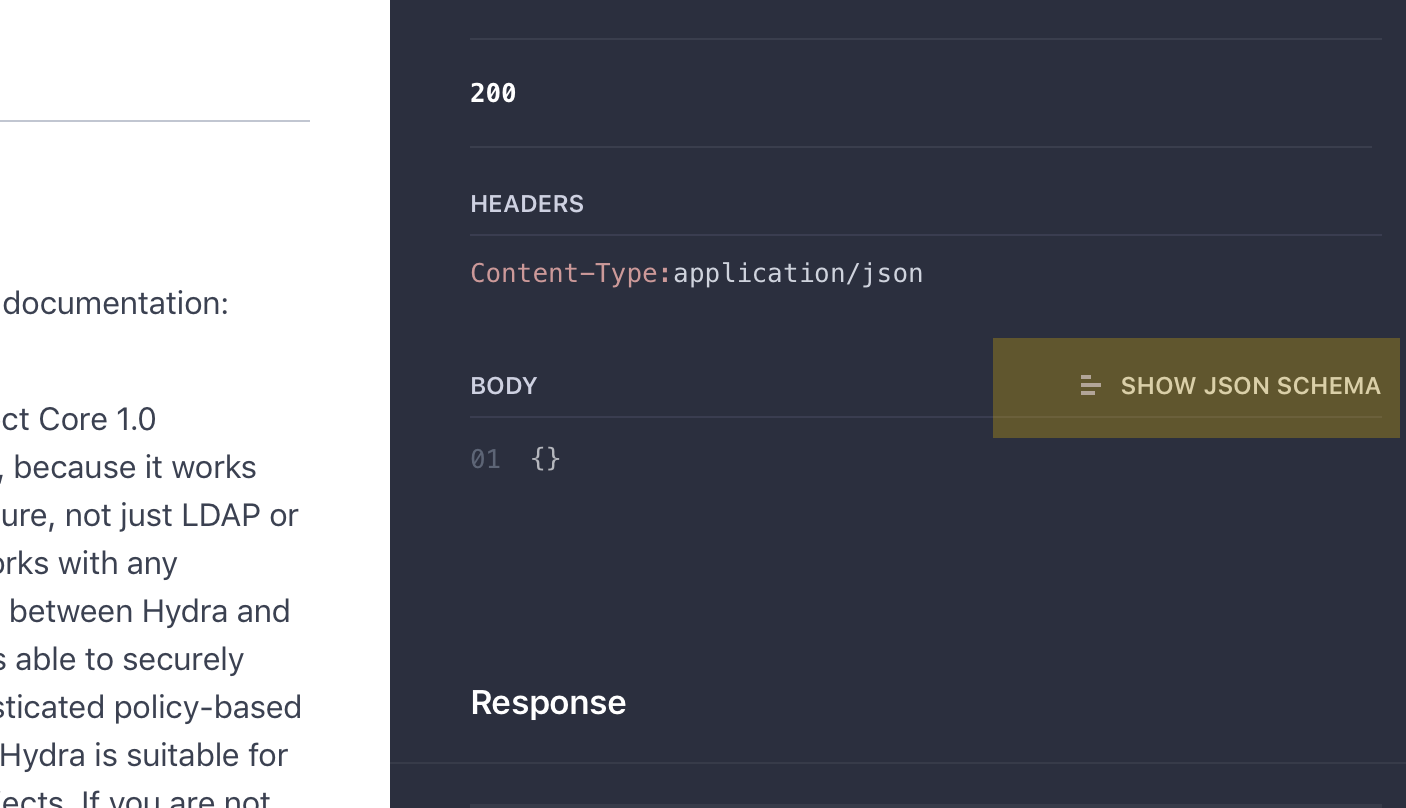 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerJsonWebKeyQuery();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerJsonWebKeyQuery', function() {
+ it('should create an instance of SwaggerJsonWebKeyQuery', function() {
+ // uncomment below and update the code to test SwaggerJsonWebKeyQuery
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJsonWebKeyQuery();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerJsonWebKeyQuery);
+ });
+
+ it('should have the property kid (base name: "kid")', function() {
+ // uncomment below and update the code to test the property kid
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJsonWebKeyQuery();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property set (base name: "set")', function() {
+ // uncomment below and update the code to test the property set
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJsonWebKeyQuery();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerJwkCreateSet.spec.js b/sdk/js/swagger/test/model/SwaggerJwkCreateSet.spec.js
new file mode 100644
index 00000000000..5f3254418a4
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerJwkCreateSet.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 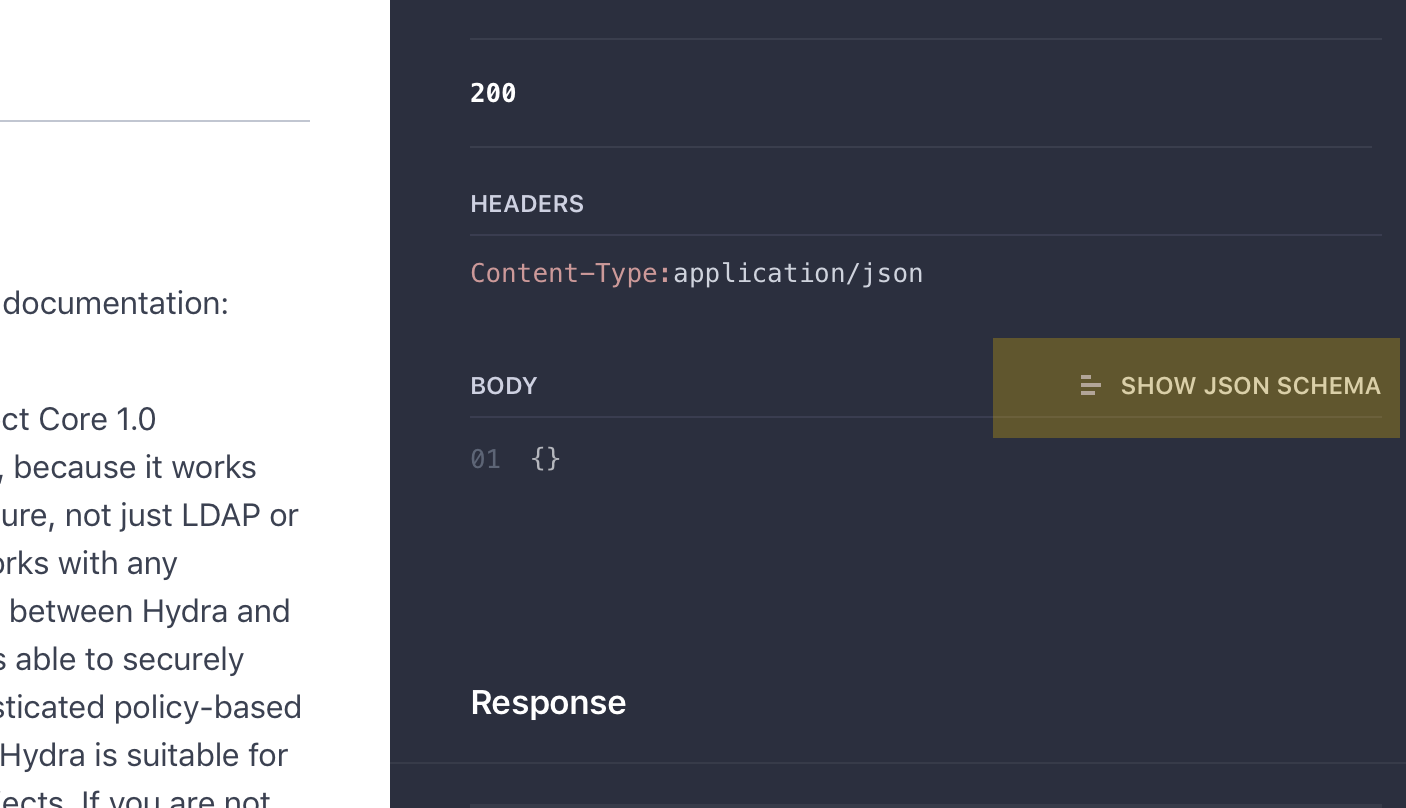 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerJwkCreateSet();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerJwkCreateSet', function() {
+ it('should create an instance of SwaggerJwkCreateSet', function() {
+ // uncomment below and update the code to test SwaggerJwkCreateSet
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkCreateSet();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerJwkCreateSet);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkCreateSet();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property set (base name: "set")', function() {
+ // uncomment below and update the code to test the property set
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkCreateSet();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerJwkSetQuery.spec.js b/sdk/js/swagger/test/model/SwaggerJwkSetQuery.spec.js
new file mode 100644
index 00000000000..33c11c0abf5
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerJwkSetQuery.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 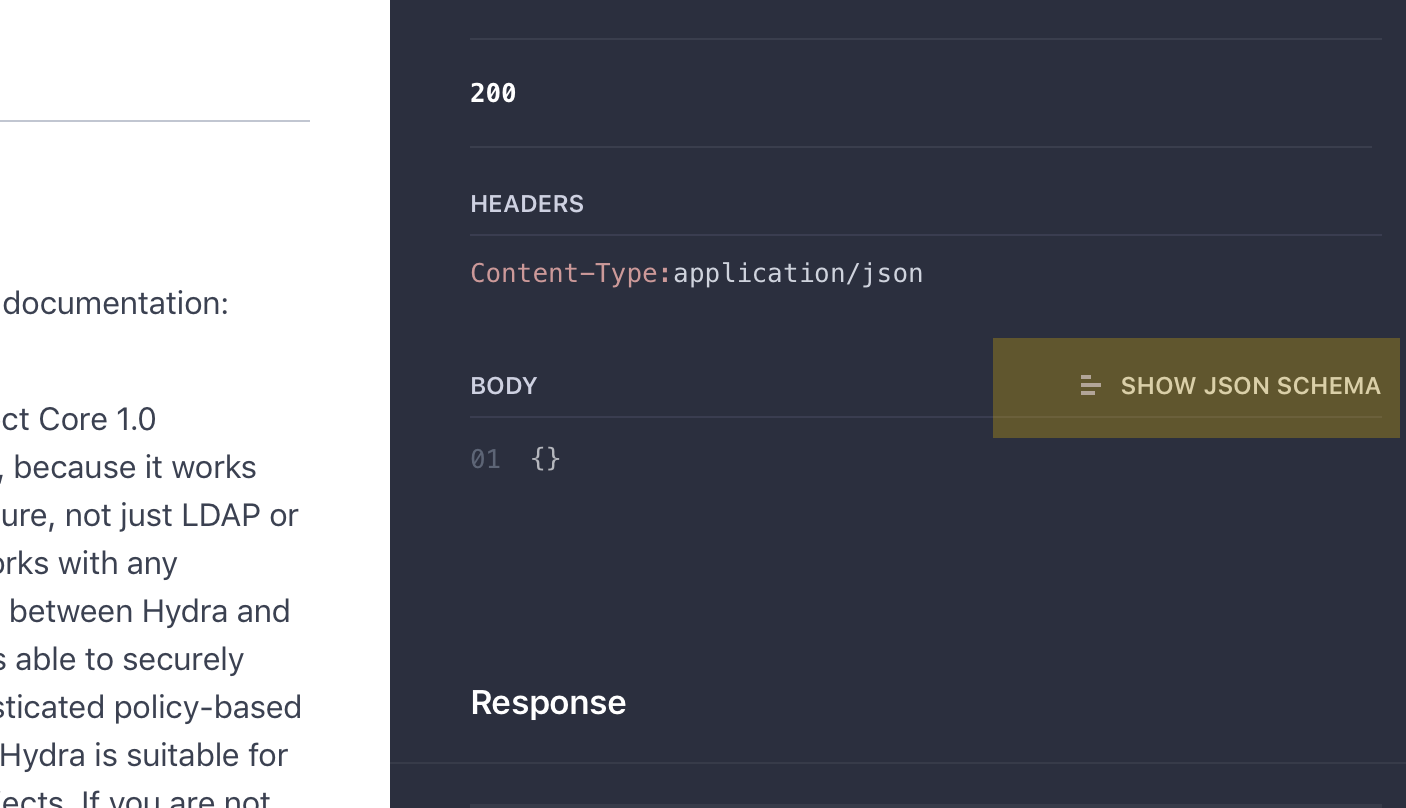 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerJwkSetQuery();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerJwkSetQuery', function() {
+ it('should create an instance of SwaggerJwkSetQuery', function() {
+ // uncomment below and update the code to test SwaggerJwkSetQuery
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkSetQuery();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerJwkSetQuery);
+ });
+
+ it('should have the property set (base name: "set")', function() {
+ // uncomment below and update the code to test the property set
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkSetQuery();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerJwkUpdateSet.spec.js b/sdk/js/swagger/test/model/SwaggerJwkUpdateSet.spec.js
new file mode 100644
index 00000000000..c8adab13232
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerJwkUpdateSet.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 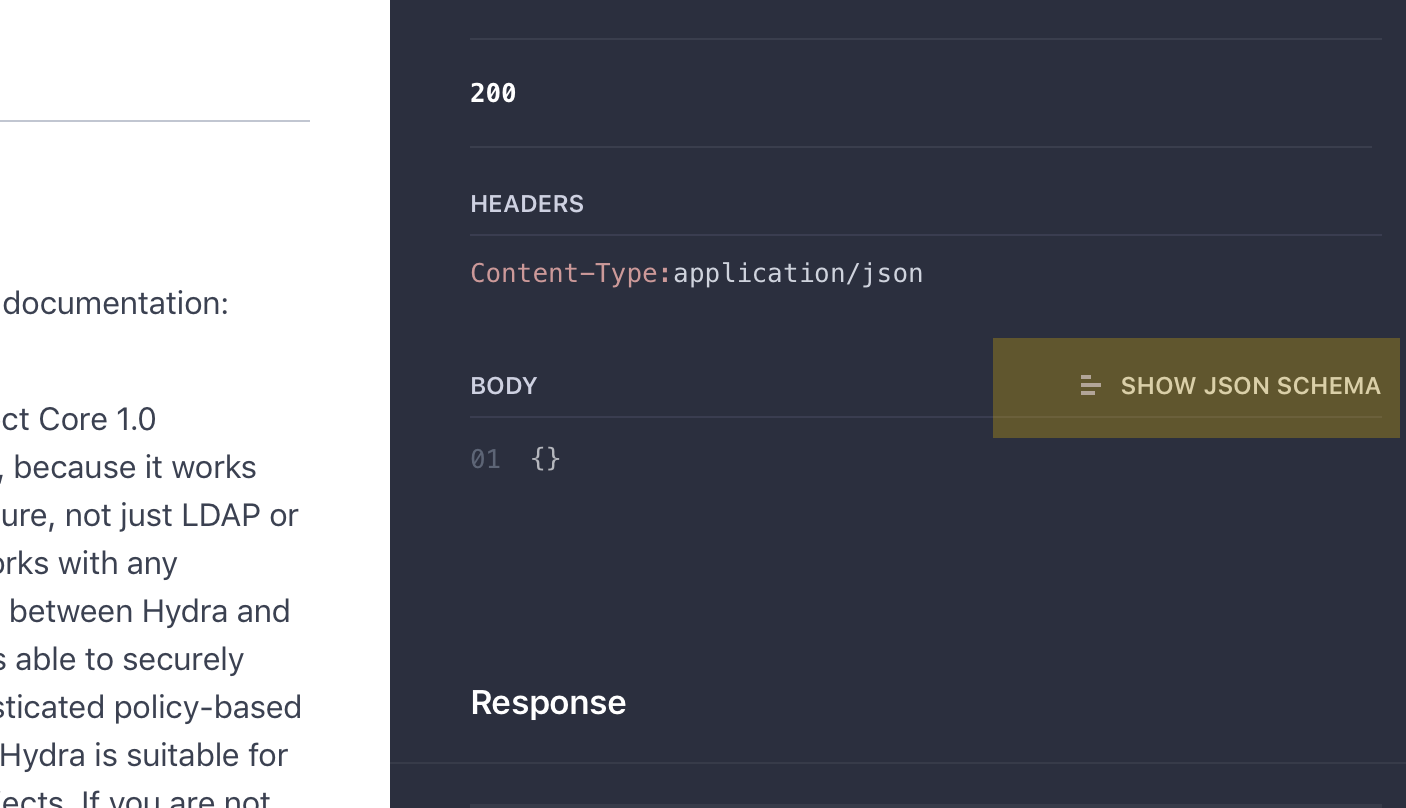 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSet();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerJwkUpdateSet', function() {
+ it('should create an instance of SwaggerJwkUpdateSet', function() {
+ // uncomment below and update the code to test SwaggerJwkUpdateSet
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSet();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSet);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSet();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property set (base name: "set")', function() {
+ // uncomment below and update the code to test the property set
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSet();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerJwkUpdateSetKey.spec.js b/sdk/js/swagger/test/model/SwaggerJwkUpdateSetKey.spec.js
new file mode 100644
index 00000000000..e6bf790108b
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerJwkUpdateSetKey.spec.js
@@ -0,0 +1,80 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 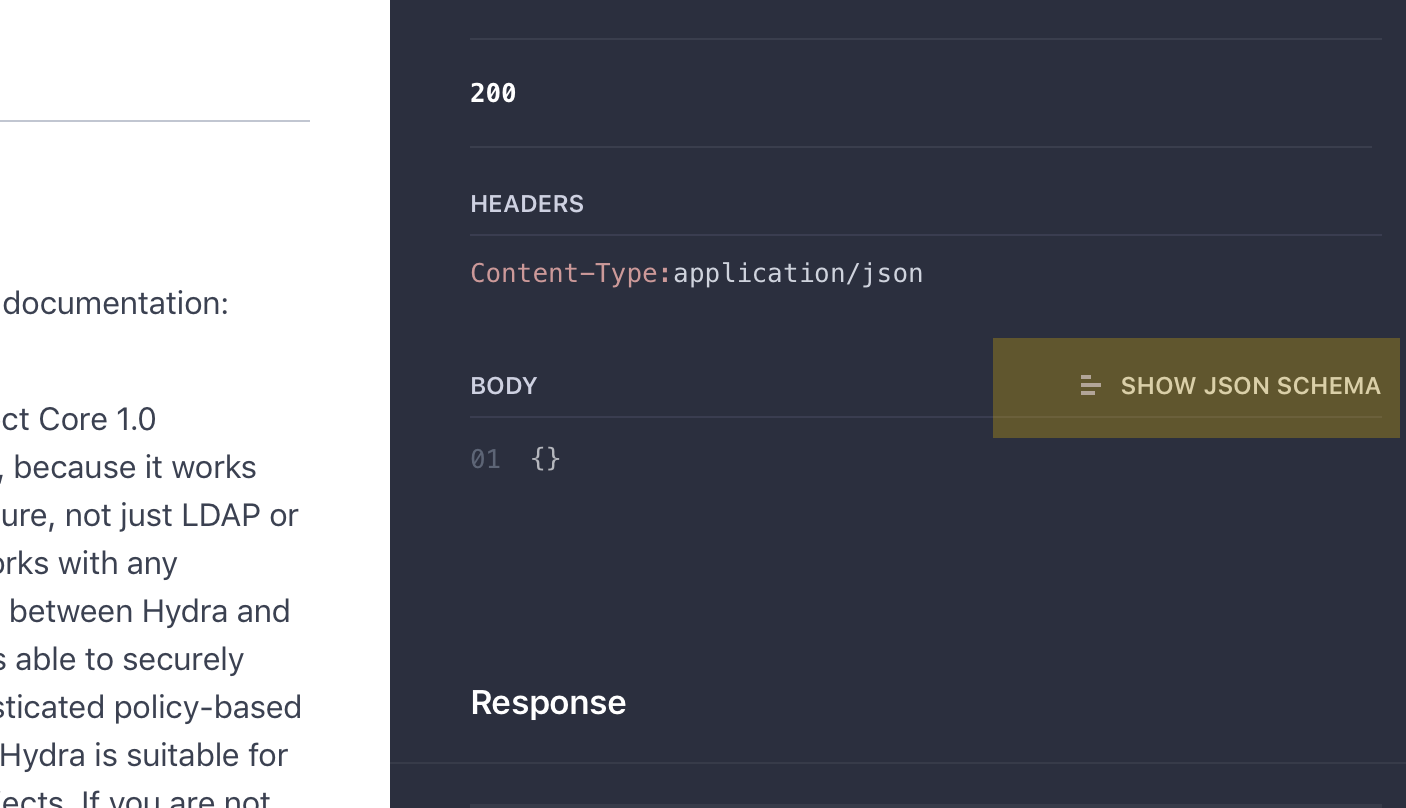 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerJwkUpdateSetKey', function() {
+ it('should create an instance of SwaggerJwkUpdateSetKey', function() {
+ // uncomment below and update the code to test SwaggerJwkUpdateSetKey
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property kid (base name: "kid")', function() {
+ // uncomment below and update the code to test the property kid
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property set (base name: "set")', function() {
+ // uncomment below and update the code to test the property set
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerJwkUpdateSetKey();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerListPolicyParameters.spec.js b/sdk/js/swagger/test/model/SwaggerListPolicyParameters.spec.js
new file mode 100644
index 00000000000..d5ccb3d8f64
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerListPolicyParameters.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 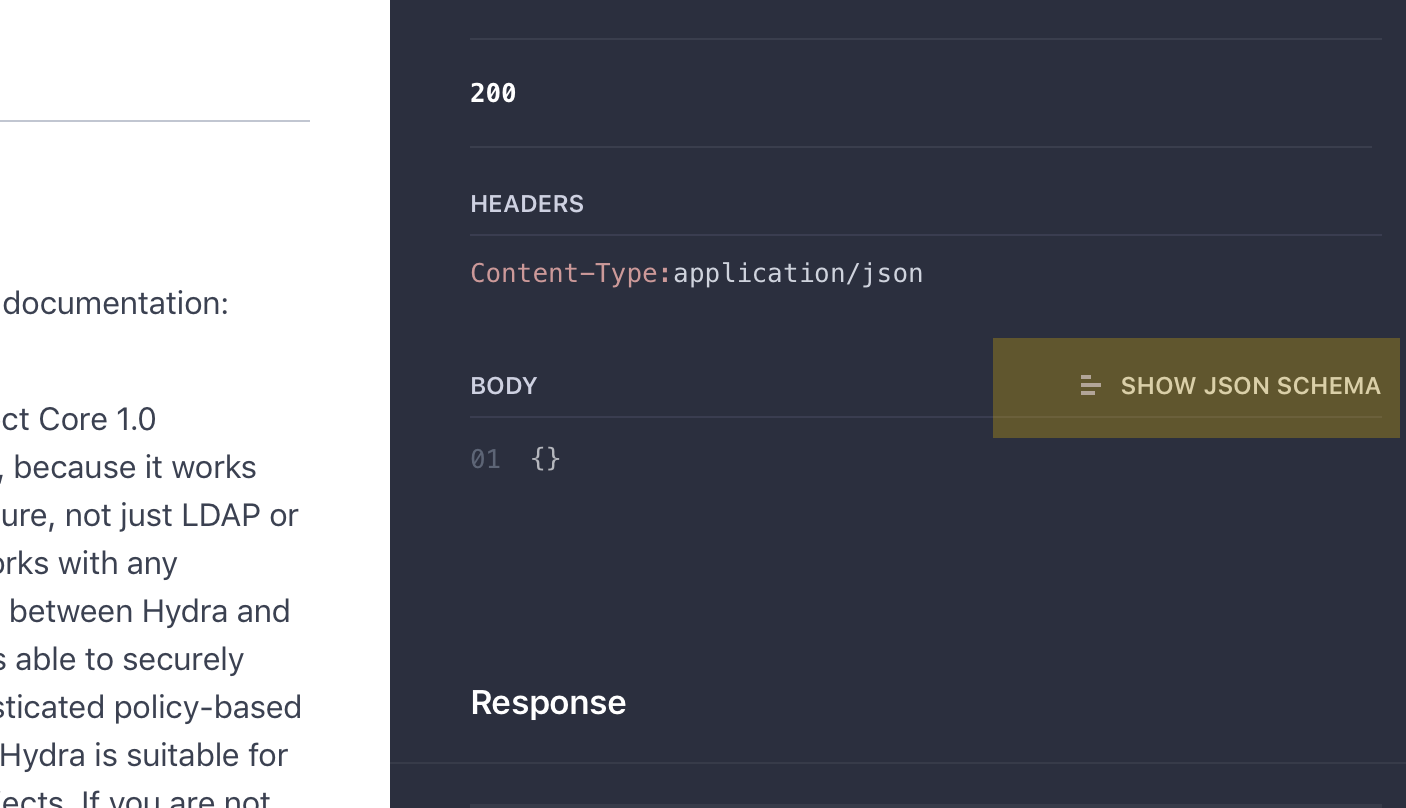 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerListPolicyParameters();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerListPolicyParameters', function() {
+ it('should create an instance of SwaggerListPolicyParameters', function() {
+ // uncomment below and update the code to test SwaggerListPolicyParameters
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerListPolicyParameters();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerListPolicyParameters);
+ });
+
+ it('should have the property limit (base name: "limit")', function() {
+ // uncomment below and update the code to test the property limit
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerListPolicyParameters();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property offset (base name: "offset")', function() {
+ // uncomment below and update the code to test the property offset
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerListPolicyParameters();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerListPolicyResponse.spec.js b/sdk/js/swagger/test/model/SwaggerListPolicyResponse.spec.js
new file mode 100644
index 00000000000..dd78e8be172
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerListPolicyResponse.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 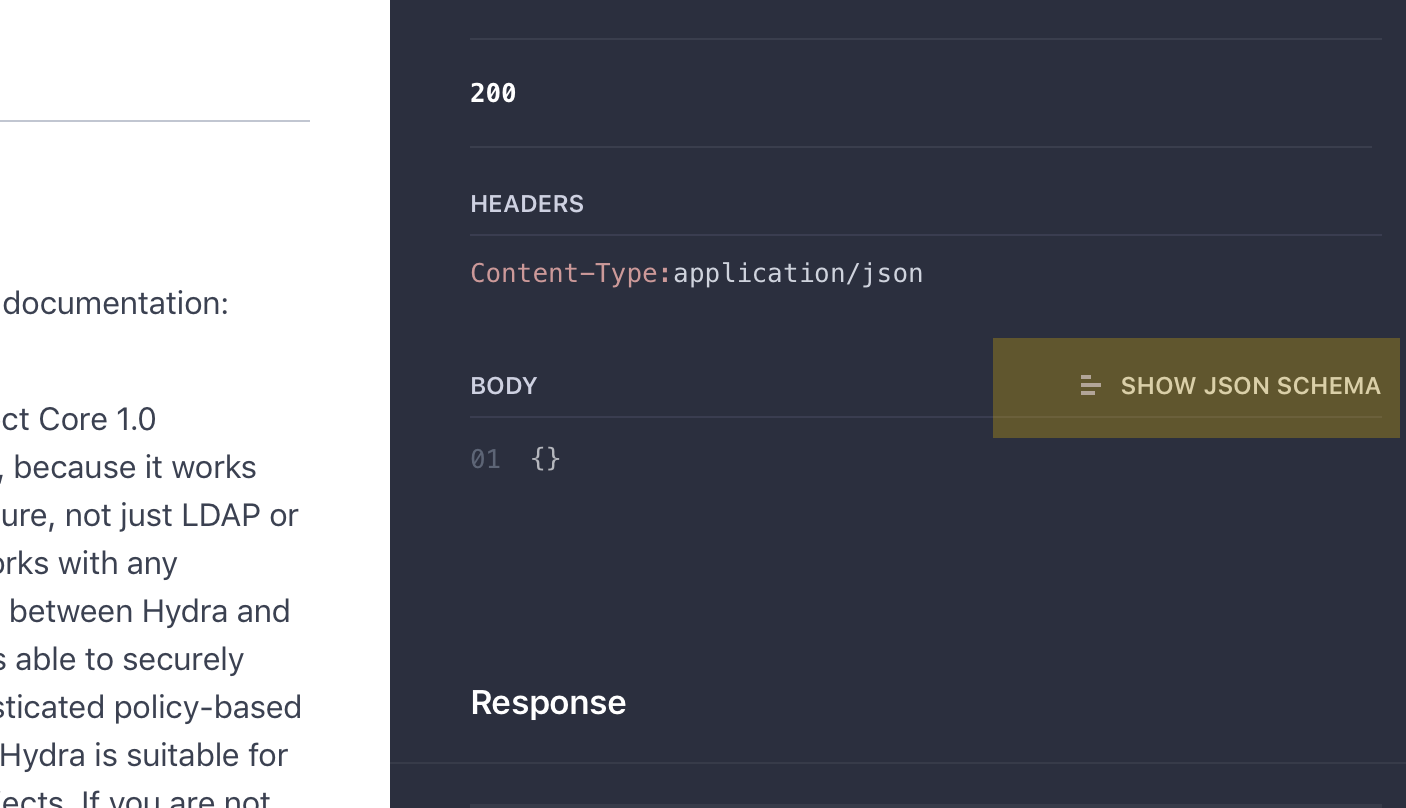 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerListPolicyResponse();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerListPolicyResponse', function() {
+ it('should create an instance of SwaggerListPolicyResponse', function() {
+ // uncomment below and update the code to test SwaggerListPolicyResponse
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerListPolicyResponse();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerListPolicyResponse);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerListPolicyResponse();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerOAuthConsentRequest.spec.js b/sdk/js/swagger/test/model/SwaggerOAuthConsentRequest.spec.js
new file mode 100644
index 00000000000..46b2105dc52
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerOAuthConsentRequest.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 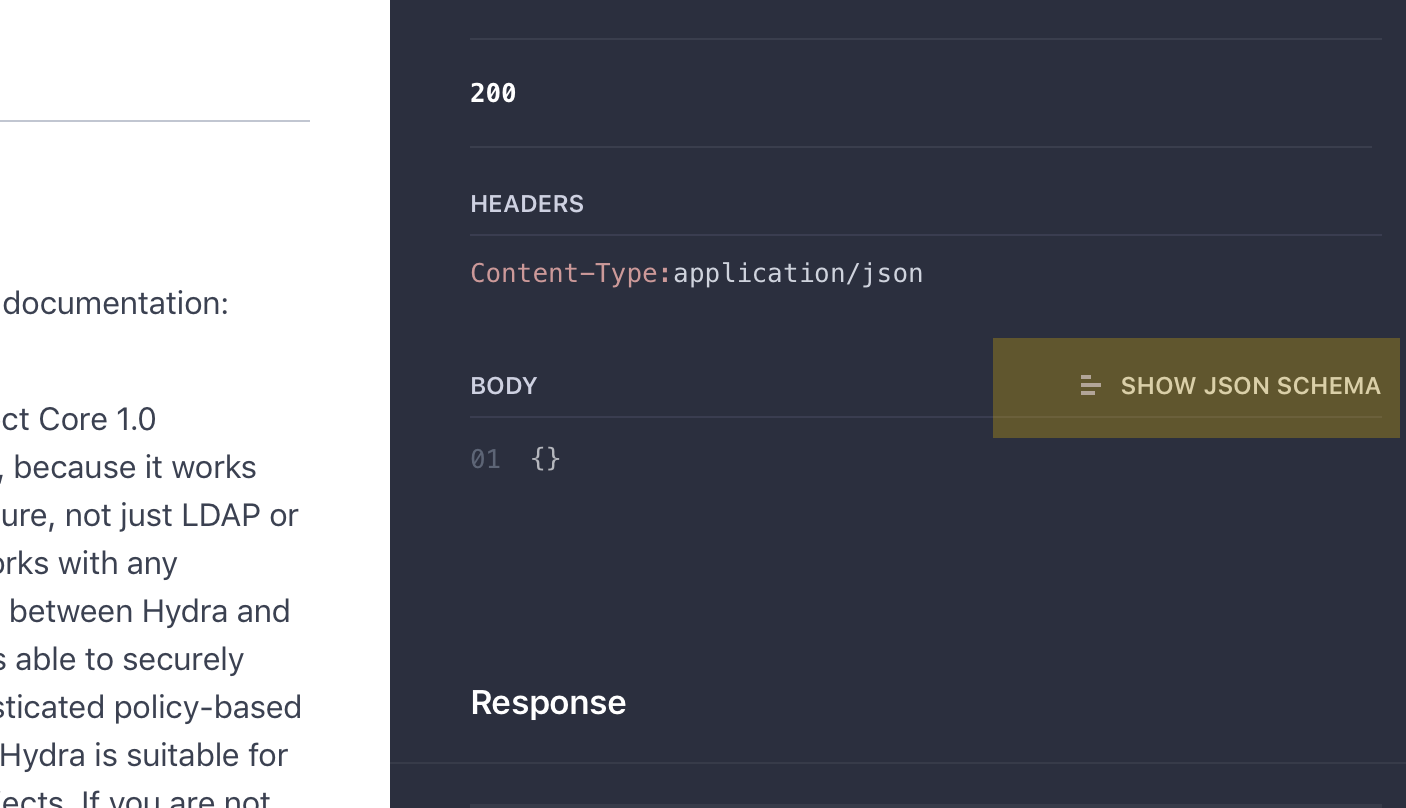 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerOAuthConsentRequest', function() {
+ it('should create an instance of SwaggerOAuthConsentRequest', function() {
+ // uncomment below and update the code to test SwaggerOAuthConsentRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequest);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerOAuthConsentRequestPayload.spec.js b/sdk/js/swagger/test/model/SwaggerOAuthConsentRequestPayload.spec.js
new file mode 100644
index 00000000000..cb8a206ba5e
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerOAuthConsentRequestPayload.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 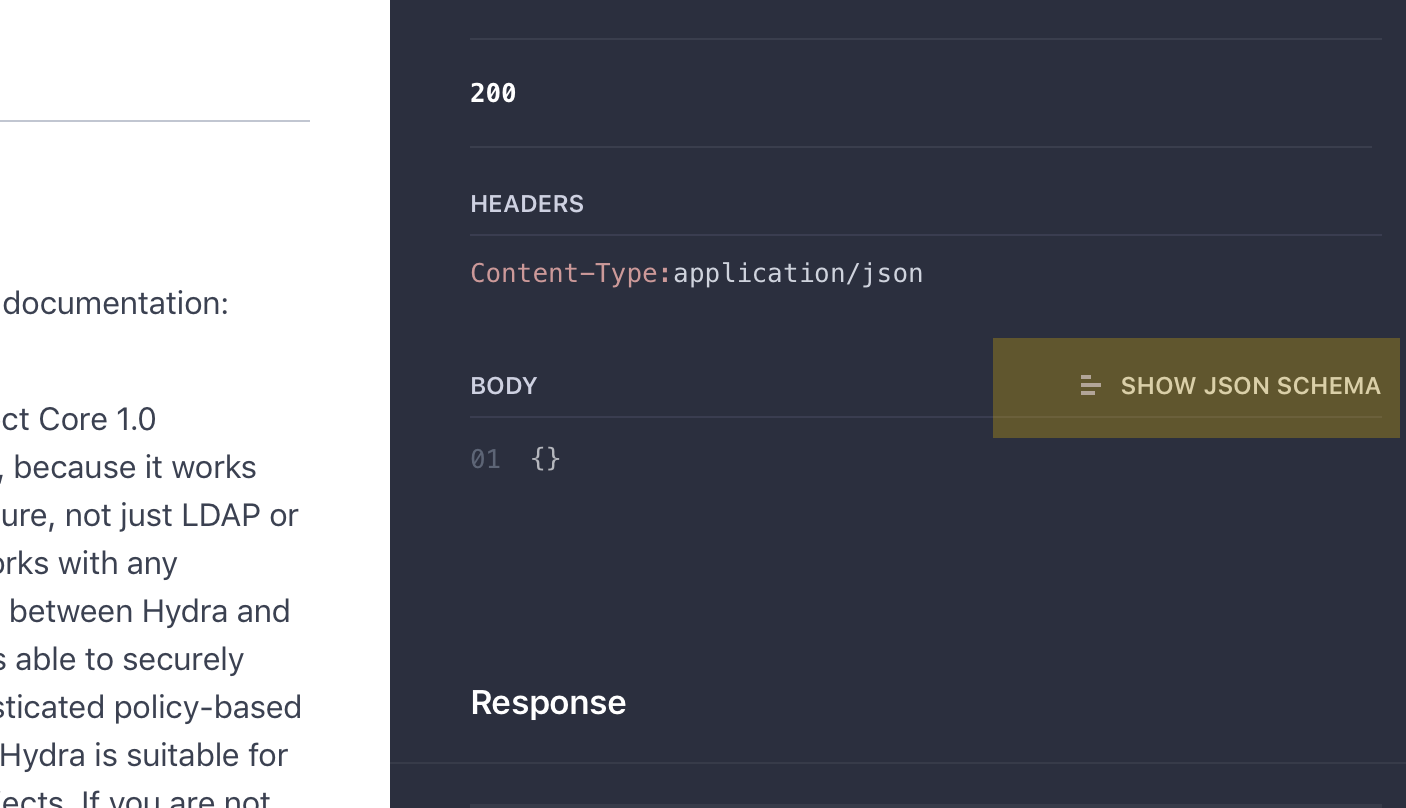 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequestPayload();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerOAuthConsentRequestPayload', function() {
+ it('should create an instance of SwaggerOAuthConsentRequestPayload', function() {
+ // uncomment below and update the code to test SwaggerOAuthConsentRequestPayload
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequestPayload();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequestPayload);
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthConsentRequestPayload();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerOAuthIntrospectionRequest.spec.js b/sdk/js/swagger/test/model/SwaggerOAuthIntrospectionRequest.spec.js
new file mode 100644
index 00000000000..c52aabe49ad
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerOAuthIntrospectionRequest.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 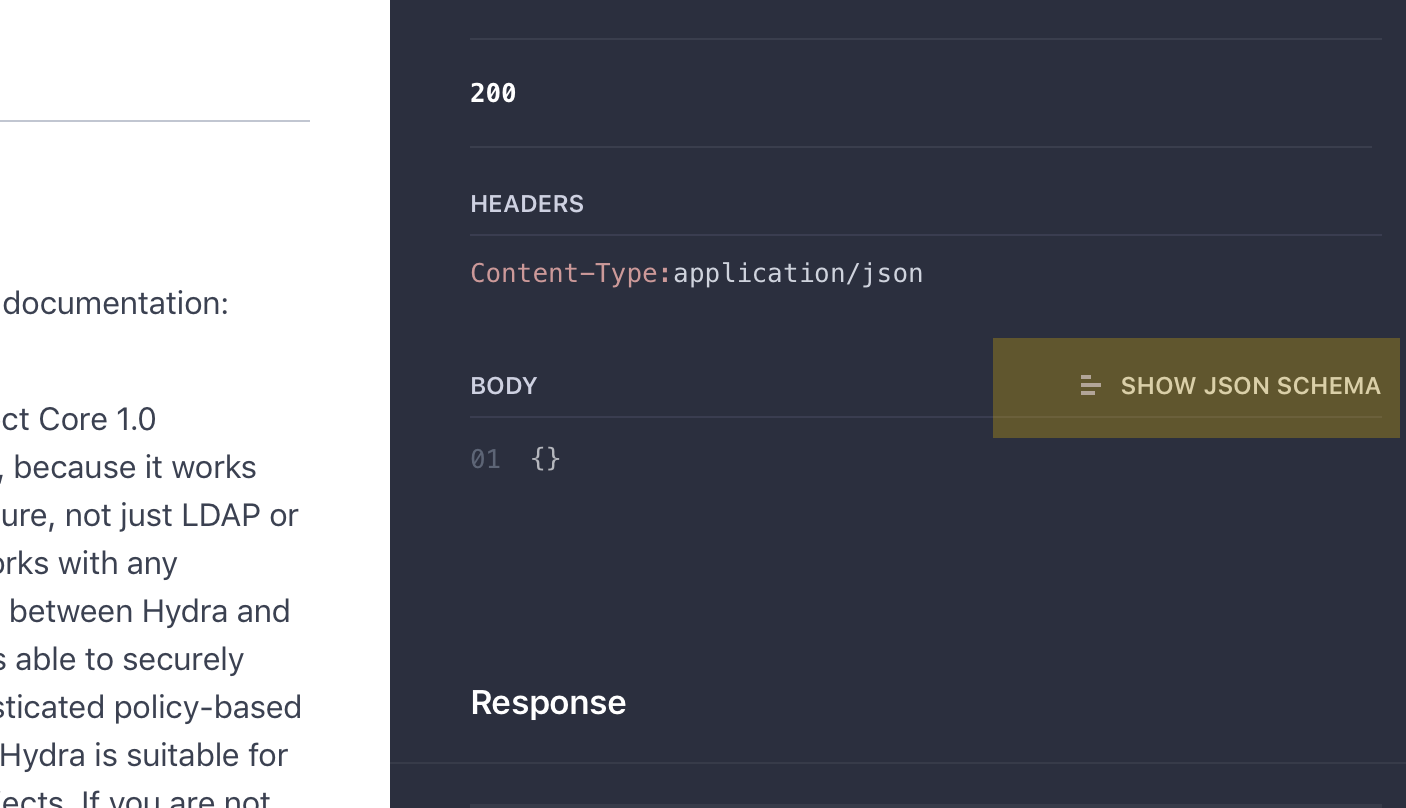 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerOAuthIntrospectionRequest', function() {
+ it('should create an instance of SwaggerOAuthIntrospectionRequest', function() {
+ // uncomment below and update the code to test SwaggerOAuthIntrospectionRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionRequest);
+ });
+
+ it('should have the property scope (base name: "scope")', function() {
+ // uncomment below and update the code to test the property scope
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property token (base name: "token")', function() {
+ // uncomment below and update the code to test the property token
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerOAuthIntrospectionResponse.spec.js b/sdk/js/swagger/test/model/SwaggerOAuthIntrospectionResponse.spec.js
new file mode 100644
index 00000000000..d6d9d3ad1a9
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerOAuthIntrospectionResponse.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 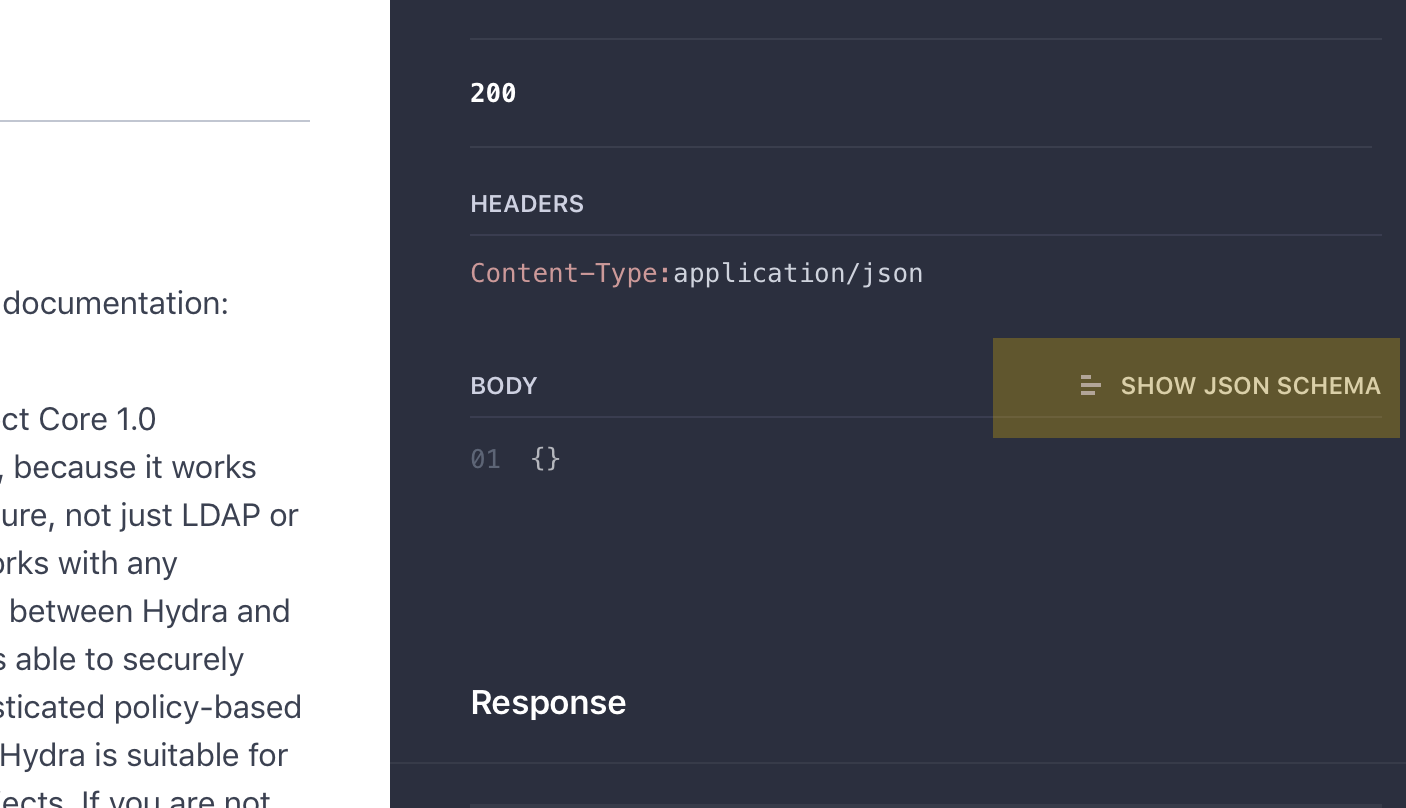 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionResponse();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerOAuthIntrospectionResponse', function() {
+ it('should create an instance of SwaggerOAuthIntrospectionResponse', function() {
+ // uncomment below and update the code to test SwaggerOAuthIntrospectionResponse
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionResponse();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionResponse);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthIntrospectionResponse();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerOAuthTokenResponse.spec.js b/sdk/js/swagger/test/model/SwaggerOAuthTokenResponse.spec.js
new file mode 100644
index 00000000000..8986f3fa9bd
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerOAuthTokenResponse.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 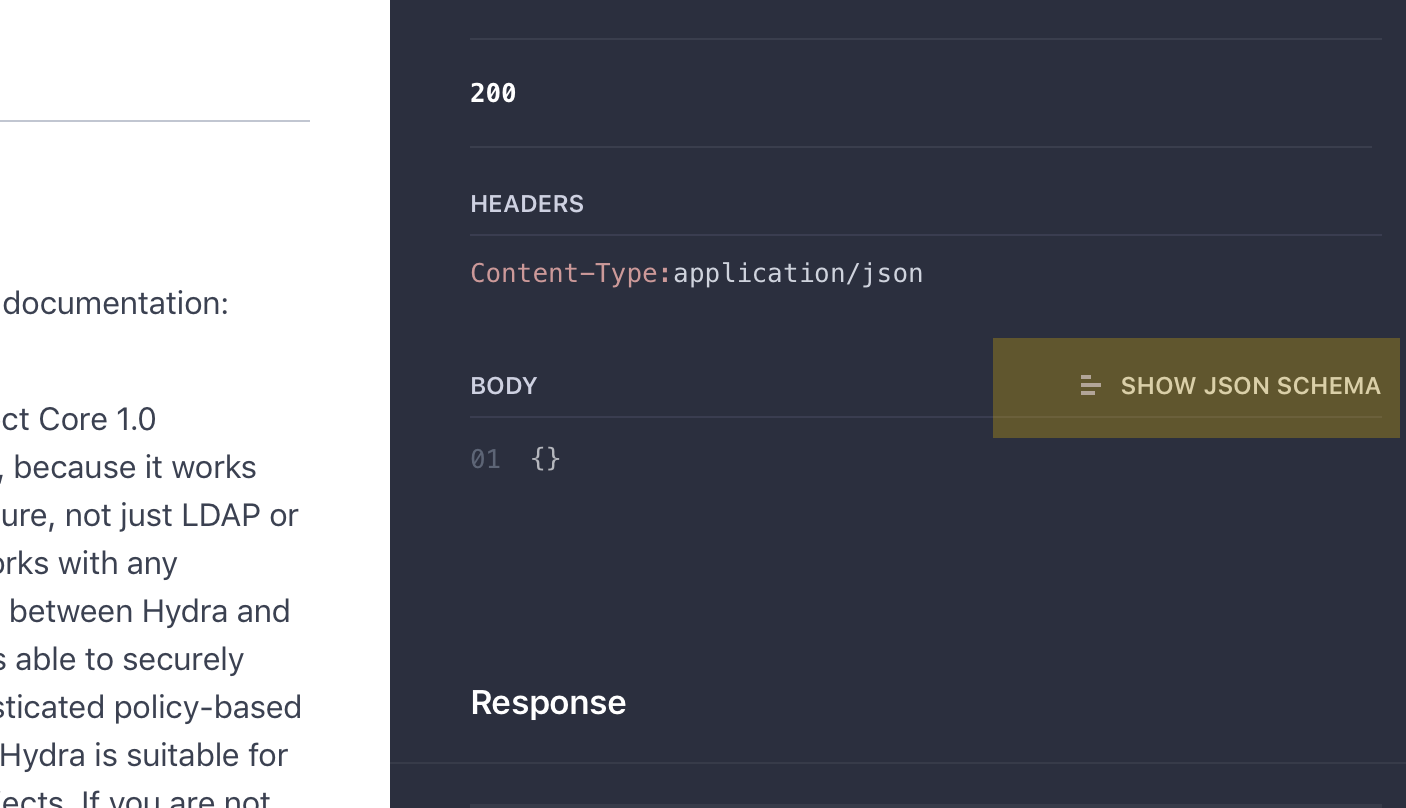 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponse();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerOAuthTokenResponse', function() {
+ it('should create an instance of SwaggerOAuthTokenResponse', function() {
+ // uncomment below and update the code to test SwaggerOAuthTokenResponse
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponse();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponse);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponse();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerOAuthTokenResponseBody.spec.js b/sdk/js/swagger/test/model/SwaggerOAuthTokenResponseBody.spec.js
new file mode 100644
index 00000000000..7955cf44402
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerOAuthTokenResponseBody.spec.js
@@ -0,0 +1,98 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 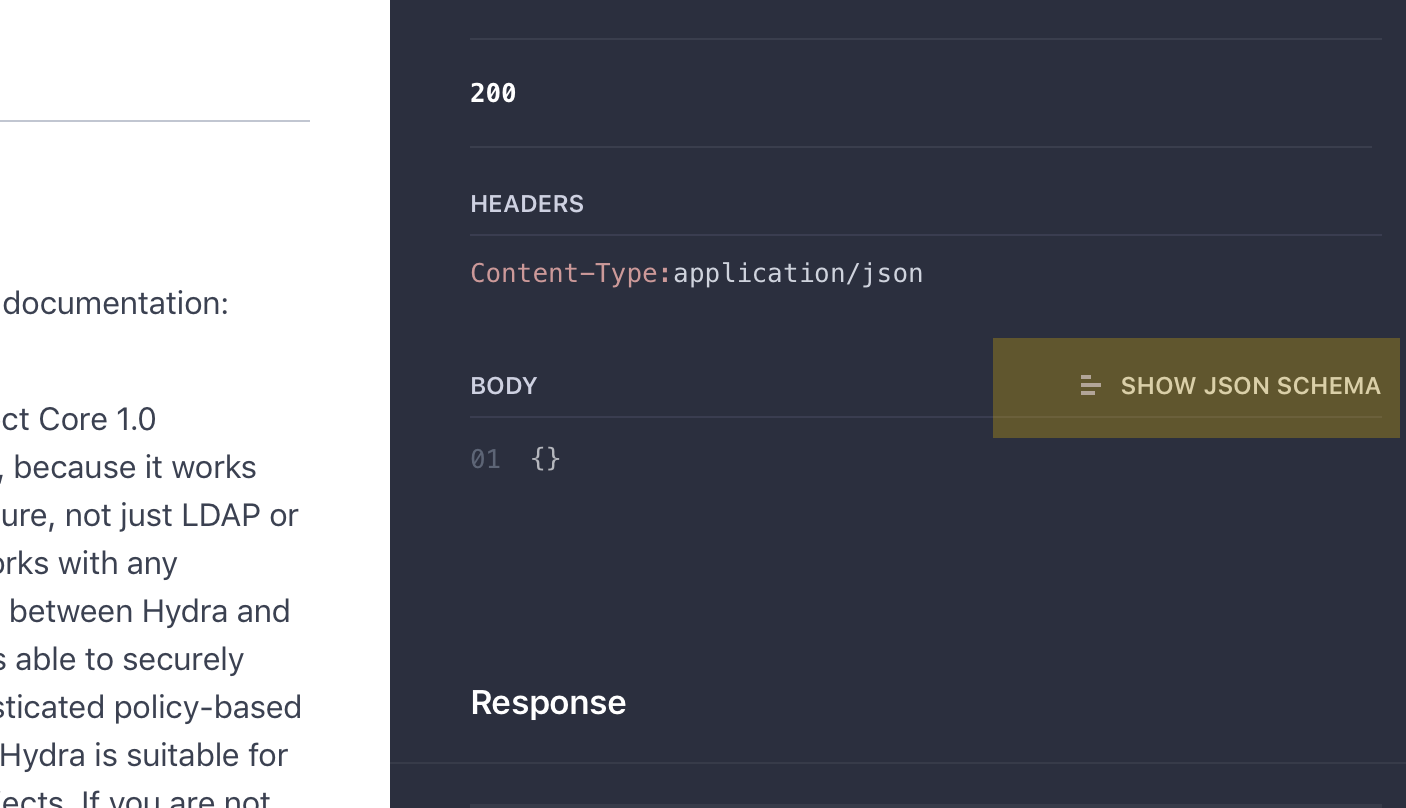 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerOAuthTokenResponseBody', function() {
+ it('should create an instance of SwaggerOAuthTokenResponseBody', function() {
+ // uncomment below and update the code to test SwaggerOAuthTokenResponseBody
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody);
+ });
+
+ it('should have the property accessToken (base name: "access_token")', function() {
+ // uncomment below and update the code to test the property accessToken
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property expiresIn (base name: "expires_in")', function() {
+ // uncomment below and update the code to test the property expiresIn
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property idToken (base name: "id_token")', function() {
+ // uncomment below and update the code to test the property idToken
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property refreshToken (base name: "refresh_token")', function() {
+ // uncomment below and update the code to test the property refreshToken
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property scope (base name: "scope")', function() {
+ // uncomment below and update the code to test the property scope
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property tokenType (base name: "token_type")', function() {
+ // uncomment below and update the code to test the property tokenType
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerOAuthTokenResponseBody();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerRejectConsentRequest.spec.js b/sdk/js/swagger/test/model/SwaggerRejectConsentRequest.spec.js
new file mode 100644
index 00000000000..f91483ee665
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerRejectConsentRequest.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 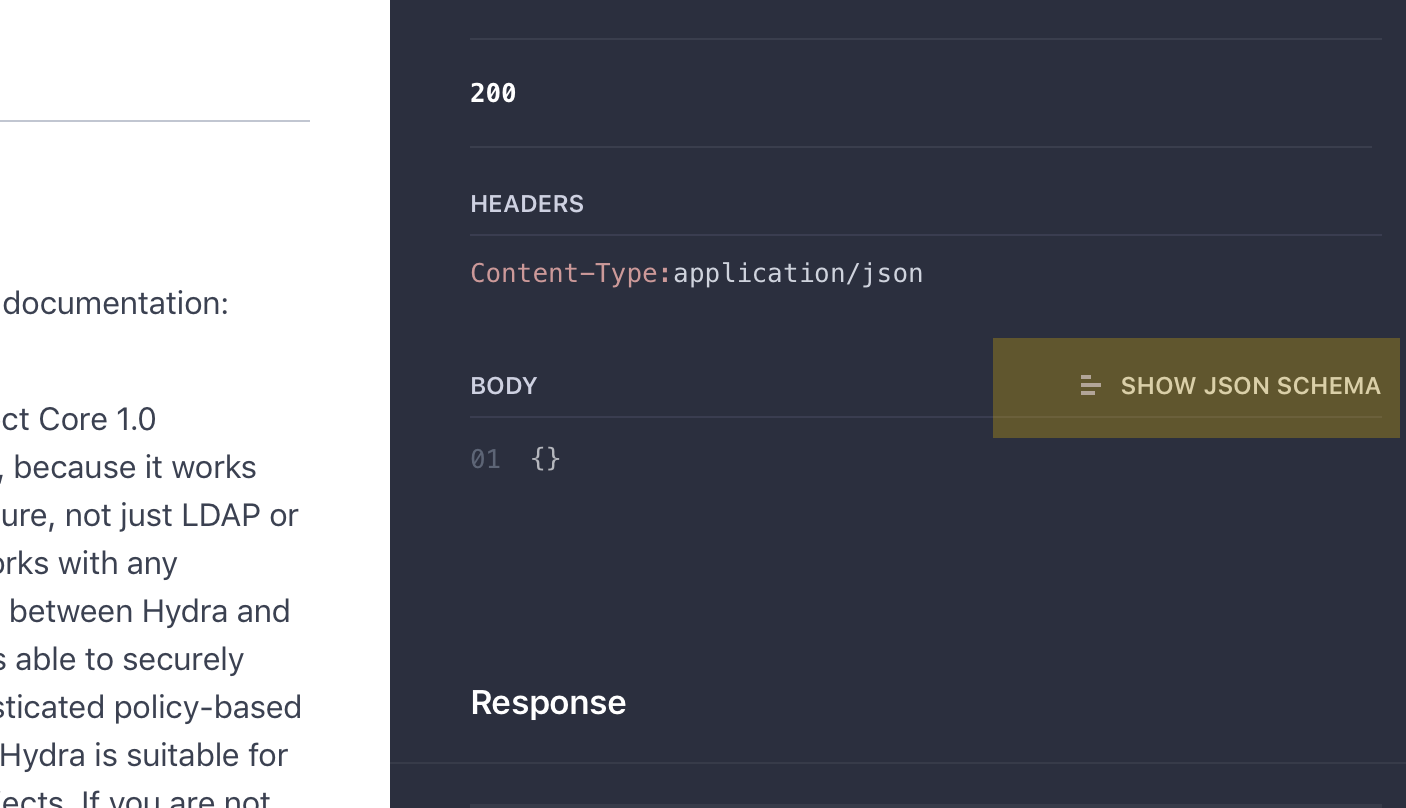 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerRejectConsentRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerRejectConsentRequest', function() {
+ it('should create an instance of SwaggerRejectConsentRequest', function() {
+ // uncomment below and update the code to test SwaggerRejectConsentRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerRejectConsentRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerRejectConsentRequest);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerRejectConsentRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerRejectConsentRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerRevokeOAuth2TokenParameters.spec.js b/sdk/js/swagger/test/model/SwaggerRevokeOAuth2TokenParameters.spec.js
new file mode 100644
index 00000000000..836ac6d8401
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerRevokeOAuth2TokenParameters.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 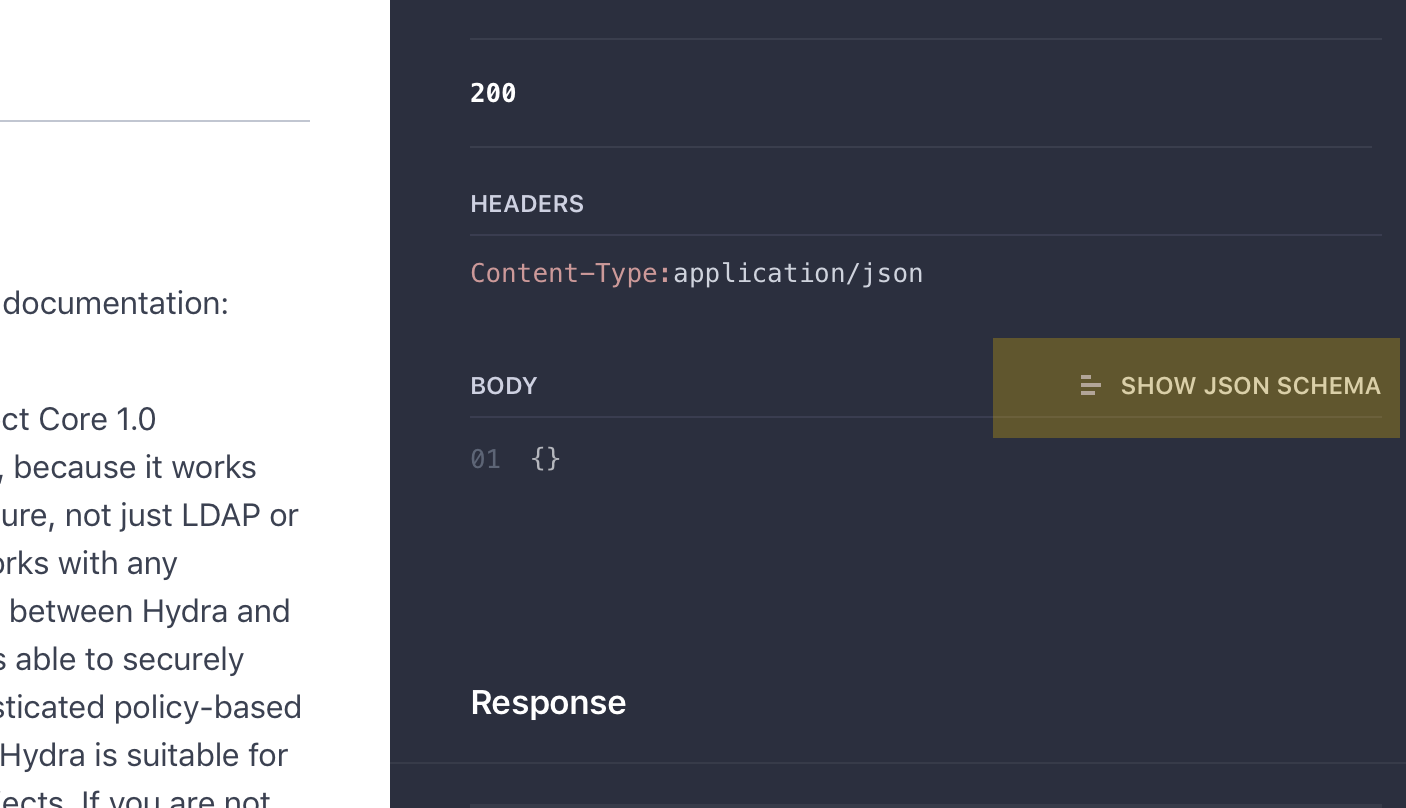 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerRevokeOAuth2TokenParameters();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerRevokeOAuth2TokenParameters', function() {
+ it('should create an instance of SwaggerRevokeOAuth2TokenParameters', function() {
+ // uncomment below and update the code to test SwaggerRevokeOAuth2TokenParameters
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerRevokeOAuth2TokenParameters();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerRevokeOAuth2TokenParameters);
+ });
+
+ it('should have the property token (base name: "token")', function() {
+ // uncomment below and update the code to test the property token
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerRevokeOAuth2TokenParameters();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerUpdatePolicyParameters.spec.js b/sdk/js/swagger/test/model/SwaggerUpdatePolicyParameters.spec.js
new file mode 100644
index 00000000000..8a58e13838e
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerUpdatePolicyParameters.spec.js
@@ -0,0 +1,74 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 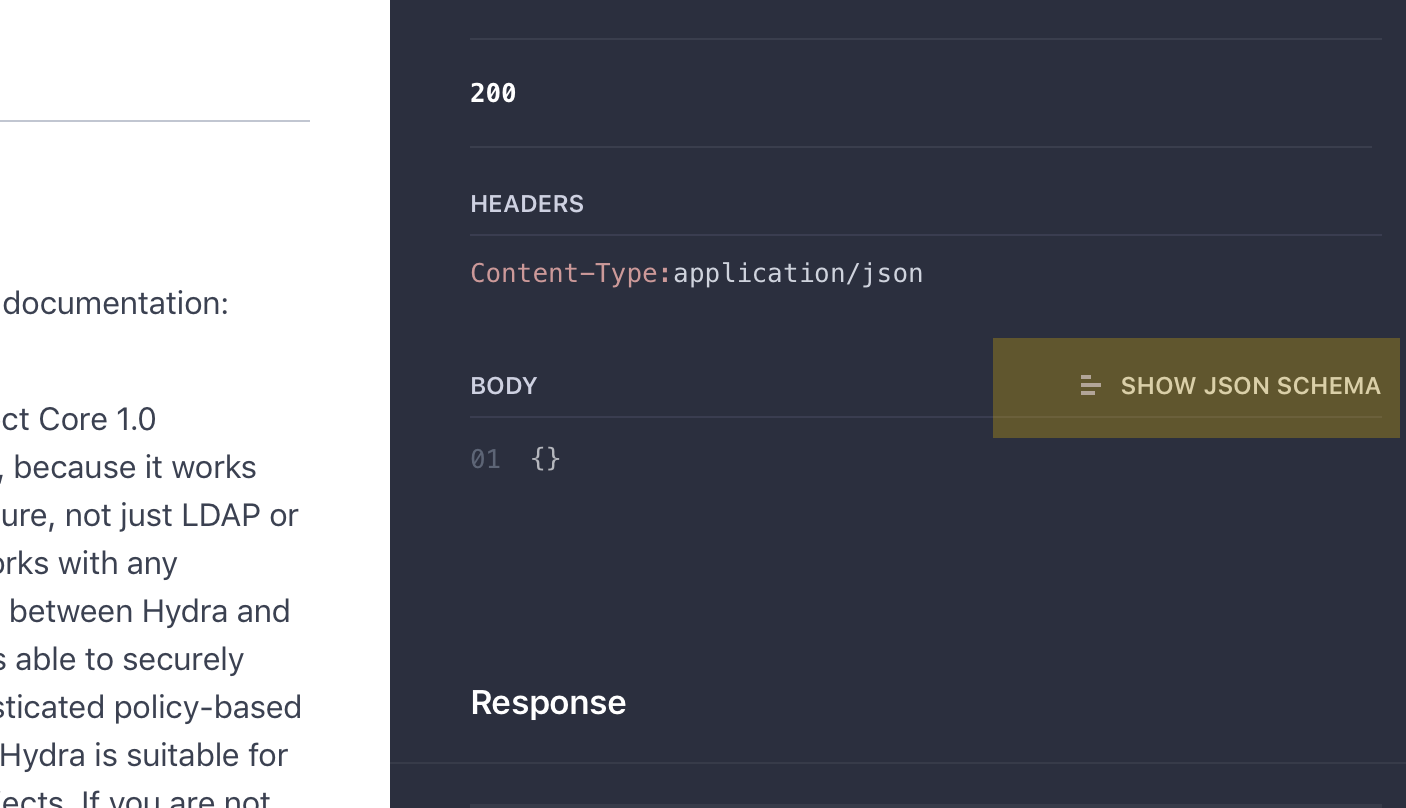 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerUpdatePolicyParameters();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerUpdatePolicyParameters', function() {
+ it('should create an instance of SwaggerUpdatePolicyParameters', function() {
+ // uncomment below and update the code to test SwaggerUpdatePolicyParameters
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerUpdatePolicyParameters();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerUpdatePolicyParameters);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerUpdatePolicyParameters();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property id (base name: "id")', function() {
+ // uncomment below and update the code to test the property id
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerUpdatePolicyParameters();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerWardenAccessRequestResponseParameters.spec.js b/sdk/js/swagger/test/model/SwaggerWardenAccessRequestResponseParameters.spec.js
new file mode 100644
index 00000000000..5717f6ec06f
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerWardenAccessRequestResponseParameters.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 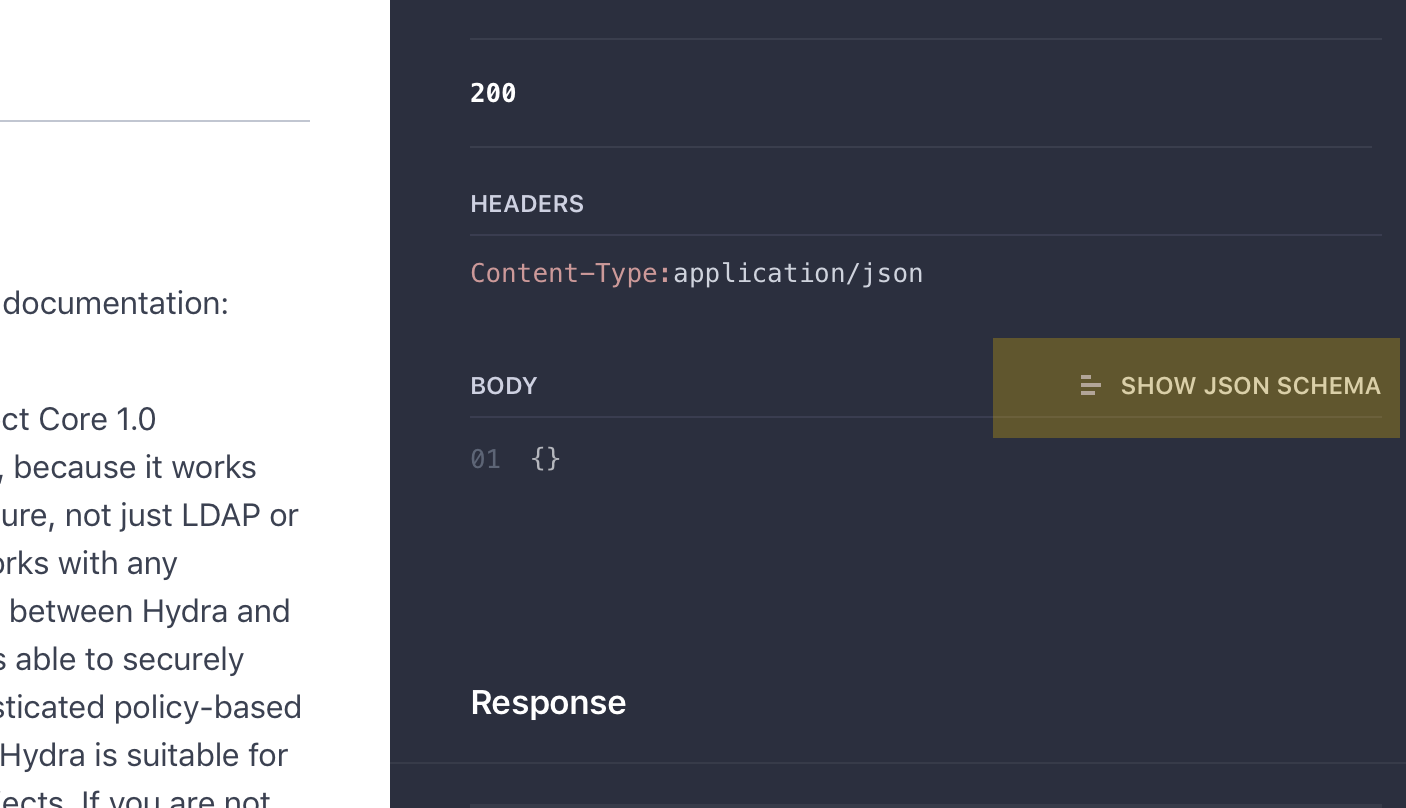 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerWardenAccessRequestResponseParameters();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerWardenAccessRequestResponseParameters', function() {
+ it('should create an instance of SwaggerWardenAccessRequestResponseParameters', function() {
+ // uncomment below and update the code to test SwaggerWardenAccessRequestResponseParameters
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerWardenAccessRequestResponseParameters();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerWardenAccessRequestResponseParameters);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerWardenAccessRequestResponseParameters();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/SwaggerWardenTokenAccessRequestResponse.spec.js b/sdk/js/swagger/test/model/SwaggerWardenTokenAccessRequestResponse.spec.js
new file mode 100644
index 00000000000..bdbc4087652
--- /dev/null
+++ b/sdk/js/swagger/test/model/SwaggerWardenTokenAccessRequestResponse.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 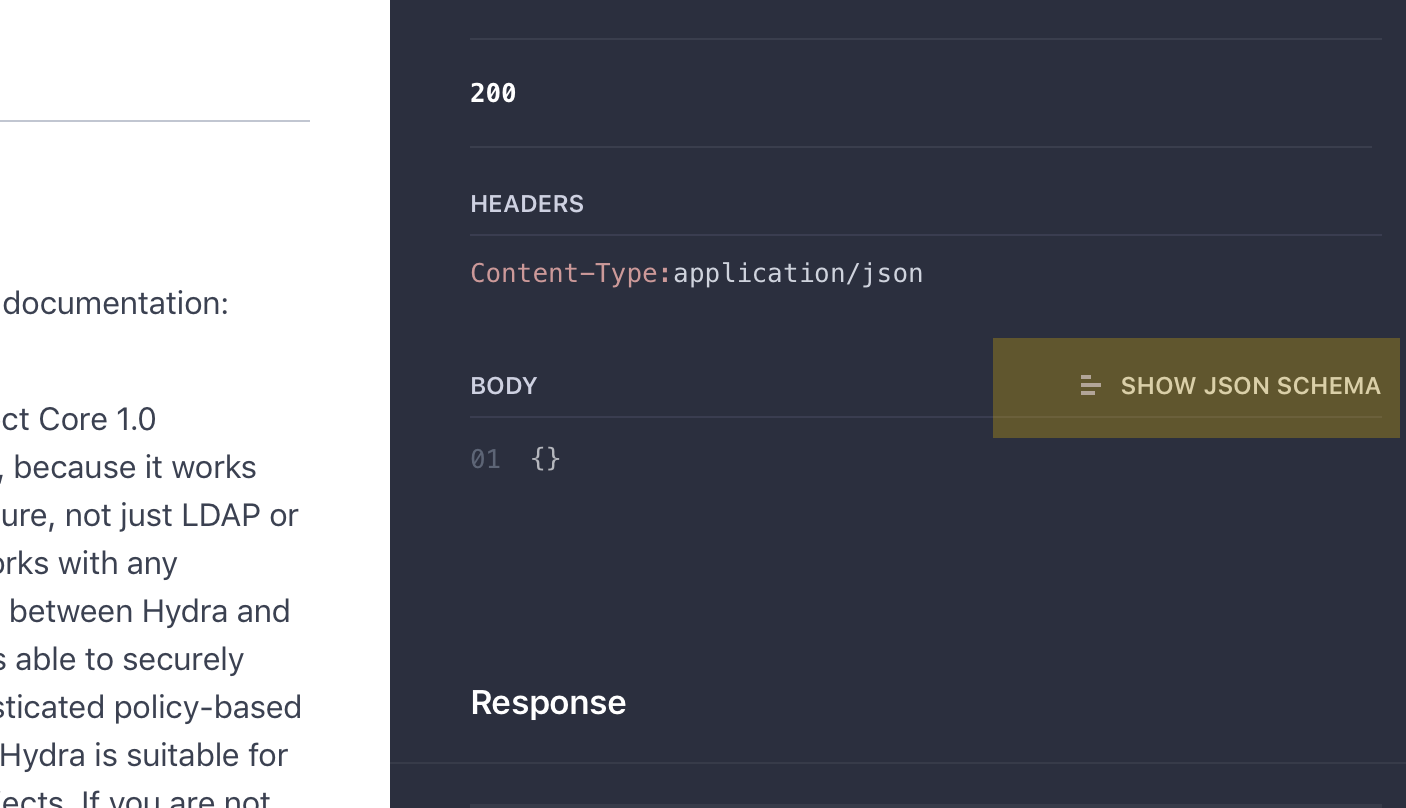 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.SwaggerWardenTokenAccessRequestResponse();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('SwaggerWardenTokenAccessRequestResponse', function() {
+ it('should create an instance of SwaggerWardenTokenAccessRequestResponse', function() {
+ // uncomment below and update the code to test SwaggerWardenTokenAccessRequestResponse
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerWardenTokenAccessRequestResponse();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.SwaggerWardenTokenAccessRequestResponse);
+ });
+
+ it('should have the property body (base name: "Body")', function() {
+ // uncomment below and update the code to test the property body
+ //var instane = new HydraOAuth2OpenIdConnectServer.SwaggerWardenTokenAccessRequestResponse();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/TokenAllowedRequest.spec.js b/sdk/js/swagger/test/model/TokenAllowedRequest.spec.js
new file mode 100644
index 00000000000..0fb3e14fa79
--- /dev/null
+++ b/sdk/js/swagger/test/model/TokenAllowedRequest.spec.js
@@ -0,0 +1,80 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 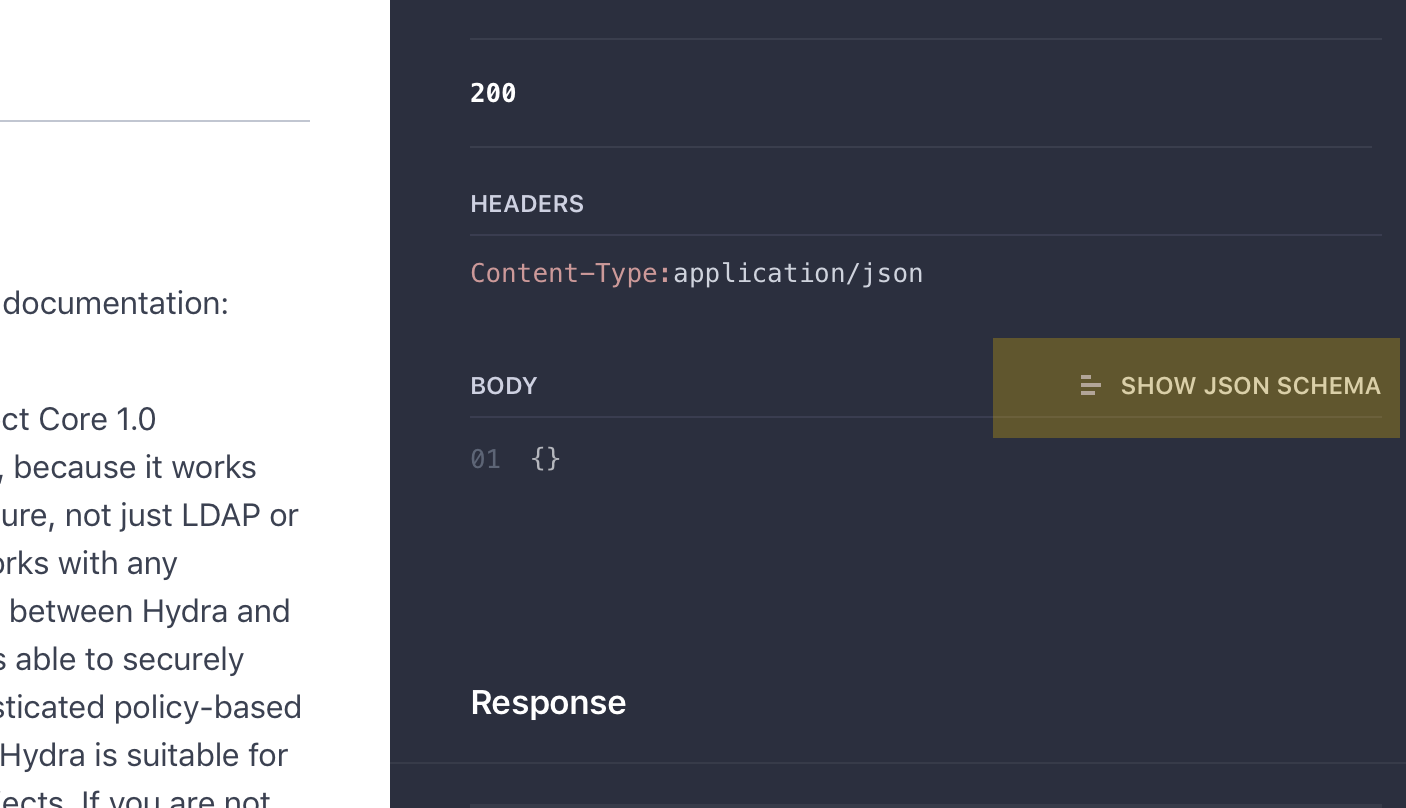 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.TokenAllowedRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('TokenAllowedRequest', function() {
+ it('should create an instance of TokenAllowedRequest', function() {
+ // uncomment below and update the code to test TokenAllowedRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.TokenAllowedRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.TokenAllowedRequest);
+ });
+
+ it('should have the property action (base name: "action")', function() {
+ // uncomment below and update the code to test the property action
+ //var instane = new HydraOAuth2OpenIdConnectServer.TokenAllowedRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property context (base name: "context")', function() {
+ // uncomment below and update the code to test the property context
+ //var instane = new HydraOAuth2OpenIdConnectServer.TokenAllowedRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property resource (base name: "resource")', function() {
+ // uncomment below and update the code to test the property resource
+ //var instane = new HydraOAuth2OpenIdConnectServer.TokenAllowedRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/WardenAccessRequest.spec.js b/sdk/js/swagger/test/model/WardenAccessRequest.spec.js
new file mode 100644
index 00000000000..705b2cc44c2
--- /dev/null
+++ b/sdk/js/swagger/test/model/WardenAccessRequest.spec.js
@@ -0,0 +1,86 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 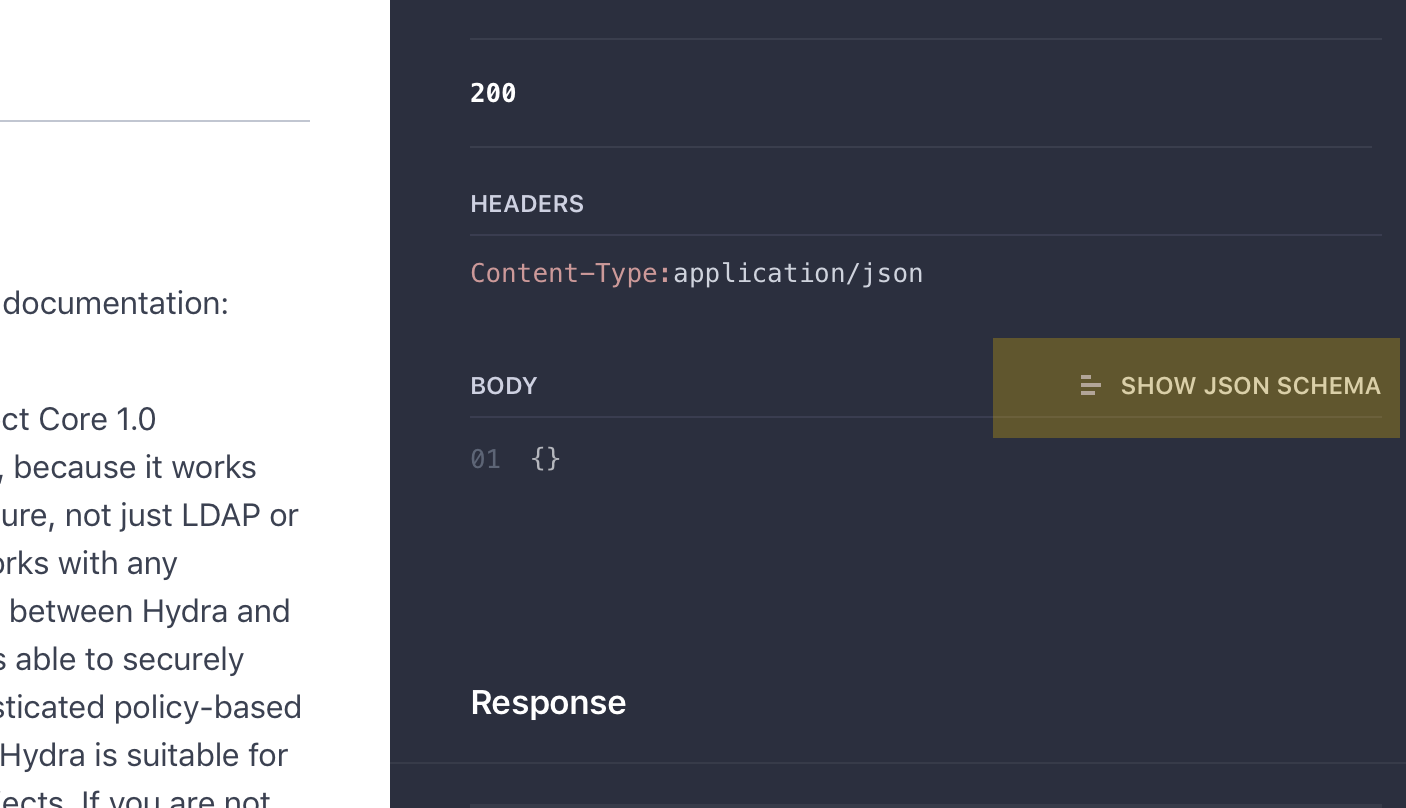 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.WardenAccessRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('WardenAccessRequest', function() {
+ it('should create an instance of WardenAccessRequest', function() {
+ // uncomment below and update the code to test WardenAccessRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenAccessRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.WardenAccessRequest);
+ });
+
+ it('should have the property action (base name: "action")', function() {
+ // uncomment below and update the code to test the property action
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property context (base name: "context")', function() {
+ // uncomment below and update the code to test the property context
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property resource (base name: "resource")', function() {
+ // uncomment below and update the code to test the property resource
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property subject (base name: "subject")', function() {
+ // uncomment below and update the code to test the property subject
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/WardenAccessRequestResponse.spec.js b/sdk/js/swagger/test/model/WardenAccessRequestResponse.spec.js
new file mode 100644
index 00000000000..46d5a66dca0
--- /dev/null
+++ b/sdk/js/swagger/test/model/WardenAccessRequestResponse.spec.js
@@ -0,0 +1,68 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 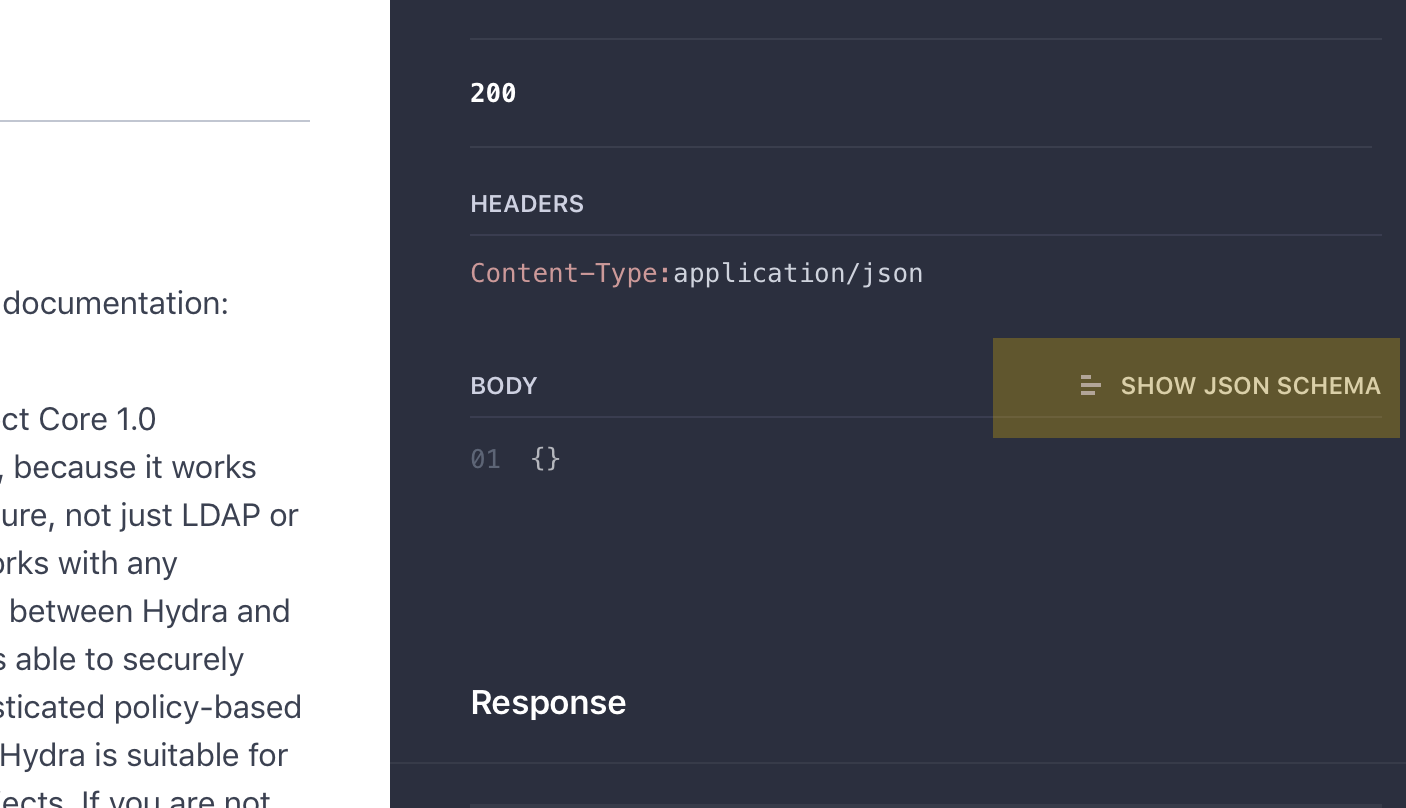 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('WardenAccessRequestResponse', function() {
+ it('should create an instance of WardenAccessRequestResponse', function() {
+ // uncomment below and update the code to test WardenAccessRequestResponse
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse);
+ });
+
+ it('should have the property allowed (base name: "allowed")', function() {
+ // uncomment below and update the code to test the property allowed
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenAccessRequestResponse();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/WardenTokenAccessRequest.spec.js b/sdk/js/swagger/test/model/WardenTokenAccessRequest.spec.js
new file mode 100644
index 00000000000..5d28f12845c
--- /dev/null
+++ b/sdk/js/swagger/test/model/WardenTokenAccessRequest.spec.js
@@ -0,0 +1,92 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 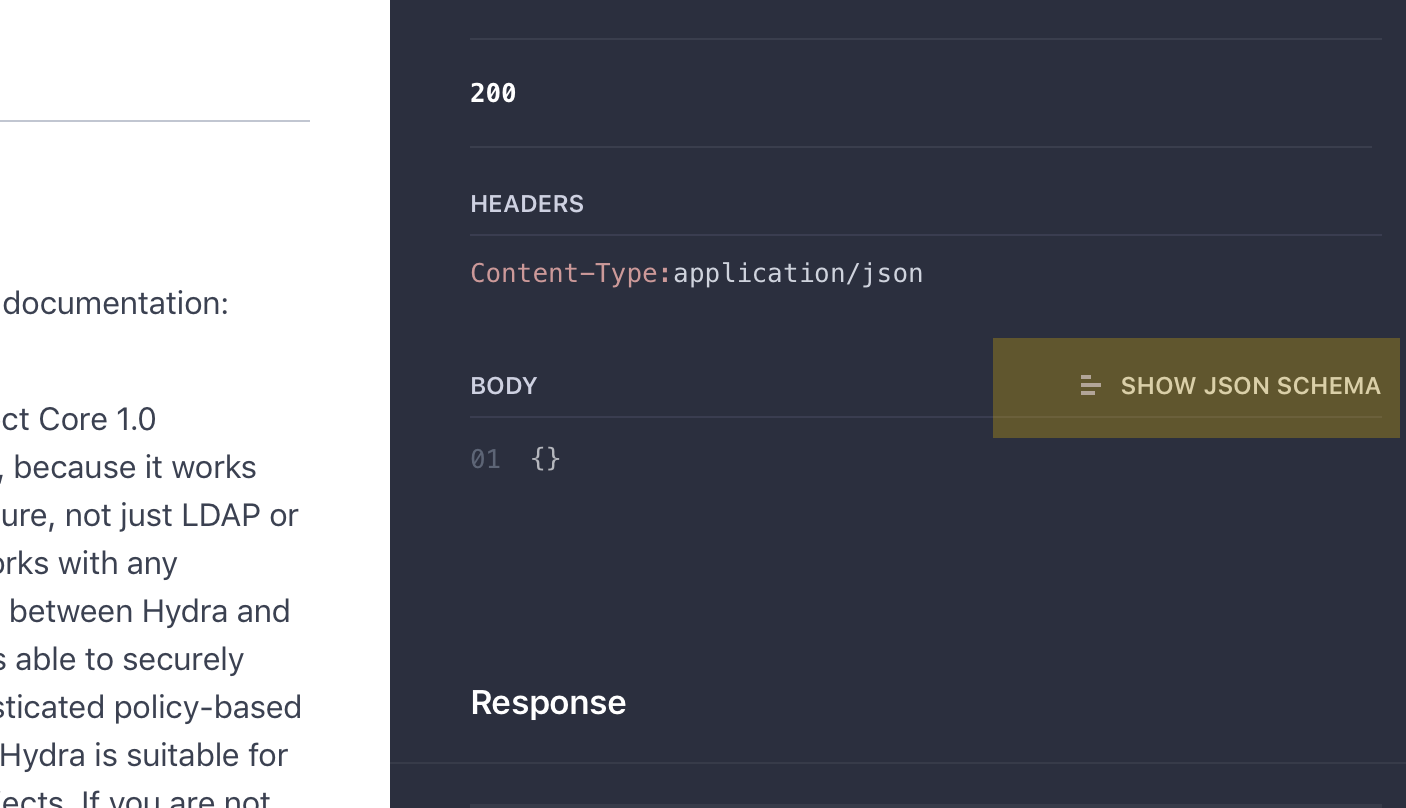 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('WardenTokenAccessRequest', function() {
+ it('should create an instance of WardenTokenAccessRequest', function() {
+ // uncomment below and update the code to test WardenTokenAccessRequest
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest);
+ });
+
+ it('should have the property action (base name: "action")', function() {
+ // uncomment below and update the code to test the property action
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property context (base name: "context")', function() {
+ // uncomment below and update the code to test the property context
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property resource (base name: "resource")', function() {
+ // uncomment below and update the code to test the property resource
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property scopes (base name: "scopes")', function() {
+ // uncomment below and update the code to test the property scopes
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property token (base name: "token")', function() {
+ // uncomment below and update the code to test the property token
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequest();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/WardenTokenAccessRequestResponsePayload.spec.js b/sdk/js/swagger/test/model/WardenTokenAccessRequestResponsePayload.spec.js
new file mode 100644
index 00000000000..e1686c67910
--- /dev/null
+++ b/sdk/js/swagger/test/model/WardenTokenAccessRequestResponsePayload.spec.js
@@ -0,0 +1,110 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 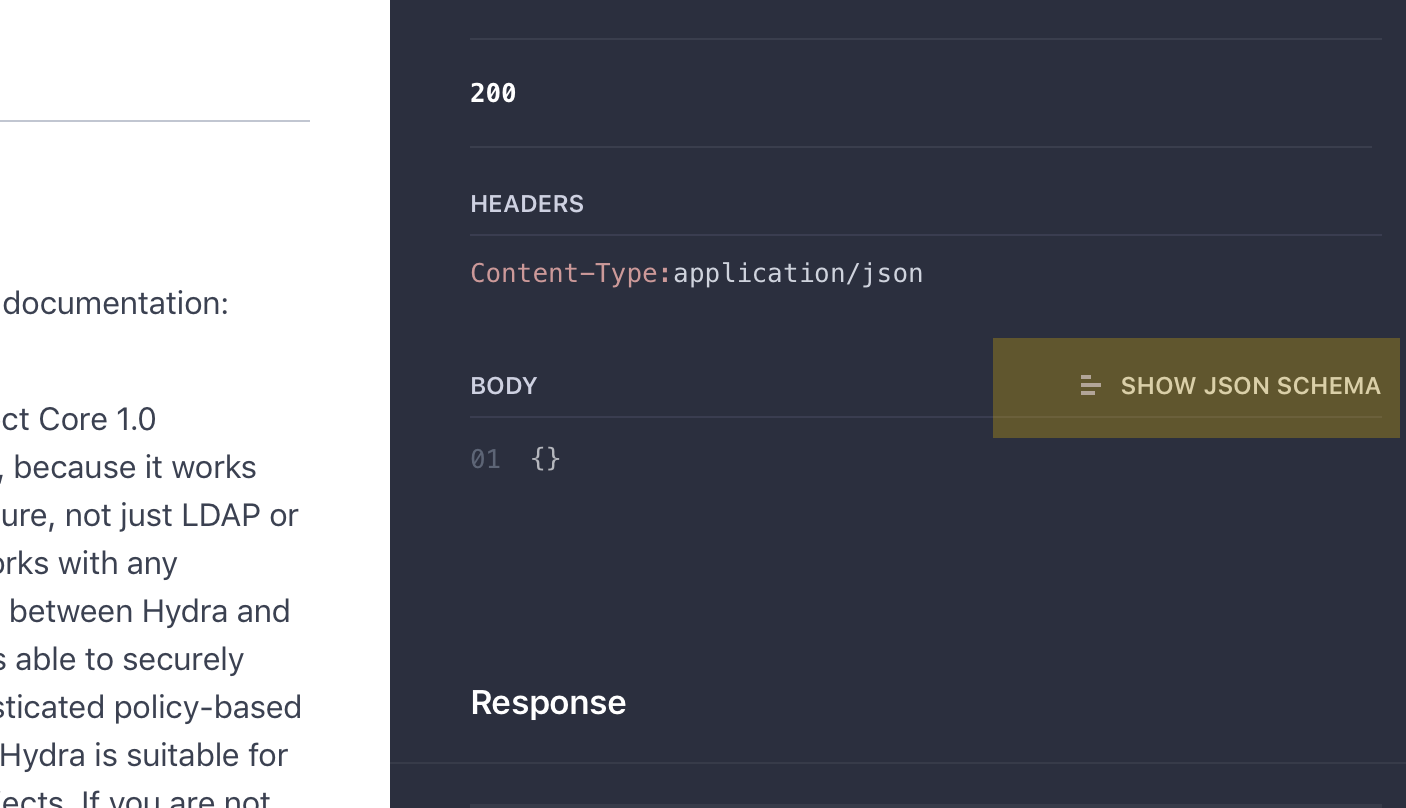 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('WardenTokenAccessRequestResponsePayload', function() {
+ it('should create an instance of WardenTokenAccessRequestResponsePayload', function() {
+ // uncomment below and update the code to test WardenTokenAccessRequestResponsePayload
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload);
+ });
+
+ it('should have the property allowed (base name: "allowed")', function() {
+ // uncomment below and update the code to test the property allowed
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property aud (base name: "aud")', function() {
+ // uncomment below and update the code to test the property aud
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property exp (base name: "exp")', function() {
+ // uncomment below and update the code to test the property exp
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property ext (base name: "ext")', function() {
+ // uncomment below and update the code to test the property ext
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property iat (base name: "iat")', function() {
+ // uncomment below and update the code to test the property iat
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property iss (base name: "iss")', function() {
+ // uncomment below and update the code to test the property iss
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property scopes (base name: "scopes")', function() {
+ // uncomment below and update the code to test the property scopes
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property sub (base name: "sub")', function() {
+ // uncomment below and update the code to test the property sub
+ //var instane = new HydraOAuth2OpenIdConnectServer.WardenTokenAccessRequestResponsePayload();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/WellKnown.spec.js b/sdk/js/swagger/test/model/WellKnown.spec.js
new file mode 100644
index 00000000000..0a5528a50a2
--- /dev/null
+++ b/sdk/js/swagger/test/model/WellKnown.spec.js
@@ -0,0 +1,104 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 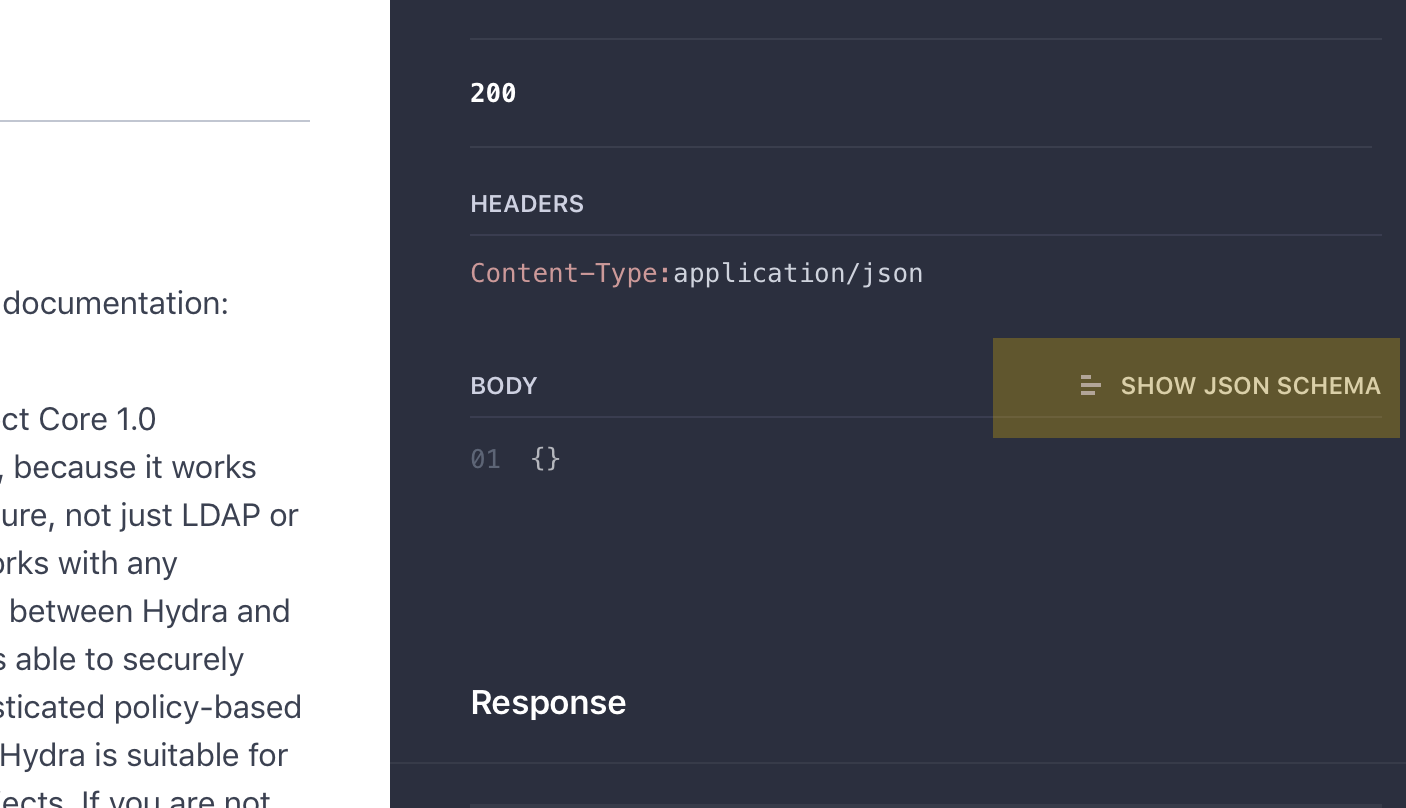 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('WellKnown', function() {
+ it('should create an instance of WellKnown', function() {
+ // uncomment below and update the code to test WellKnown
+ //var instane = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.WellKnown);
+ });
+
+ it('should have the property authorizationEndpoint (base name: "authorization_endpoint")', function() {
+ // uncomment below and update the code to test the property authorizationEndpoint
+ //var instane = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property idTokenSigningAlgValuesSupported (base name: "id_token_signing_alg_values_supported")', function() {
+ // uncomment below and update the code to test the property idTokenSigningAlgValuesSupported
+ //var instane = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property issuer (base name: "issuer")', function() {
+ // uncomment below and update the code to test the property issuer
+ //var instane = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property jwksUri (base name: "jwks_uri")', function() {
+ // uncomment below and update the code to test the property jwksUri
+ //var instane = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property responseTypesSupported (base name: "response_types_supported")', function() {
+ // uncomment below and update the code to test the property responseTypesSupported
+ //var instane = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property subjectTypesSupported (base name: "subject_types_supported")', function() {
+ // uncomment below and update the code to test the property subjectTypesSupported
+ //var instane = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ //expect(instance).to.be();
+ });
+
+ it('should have the property tokenEndpoint (base name: "token_endpoint")', function() {
+ // uncomment below and update the code to test the property tokenEndpoint
+ //var instane = new HydraOAuth2OpenIdConnectServer.WellKnown();
+ //expect(instance).to.be();
+ });
+
+ });
+
+}));
diff --git a/sdk/js/swagger/test/model/Writer.spec.js b/sdk/js/swagger/test/model/Writer.spec.js
new file mode 100644
index 00000000000..d4744cb0dfc
--- /dev/null
+++ b/sdk/js/swagger/test/model/Writer.spec.js
@@ -0,0 +1,62 @@
+/**
+ * Hydra OAuth2 & OpenID Connect Server
+ * Please refer to the user guide for in-depth documentation: https://ory.gitbooks.io/hydra/content/ Hydra offers OAuth 2.0 and OpenID Connect Core 1.0 capabilities as a service. Hydra is different, because it works with any existing authentication infrastructure, not just LDAP or SAML. By implementing a consent app (works with any programming language) you build a bridge between Hydra and your authentication infrastructure. Hydra is able to securely manage JSON Web Keys, and has a sophisticated policy-based access control you can use if you want to. Hydra is suitable for green- (new) and brownfield (existing) projects. If you are not familiar with OAuth 2.0 and are working on a greenfield project, we recommend evaluating if OAuth 2.0 really serves your purpose. Knowledge of OAuth 2.0 is imperative in understanding what Hydra does and how it works. The official repository is located at https://github.com/ory/hydra ### Important REST API Documentation Notes The swagger generator used to create this documentation does currently not support example responses. To see request and response payloads click on **\"Show JSON schema\"**: 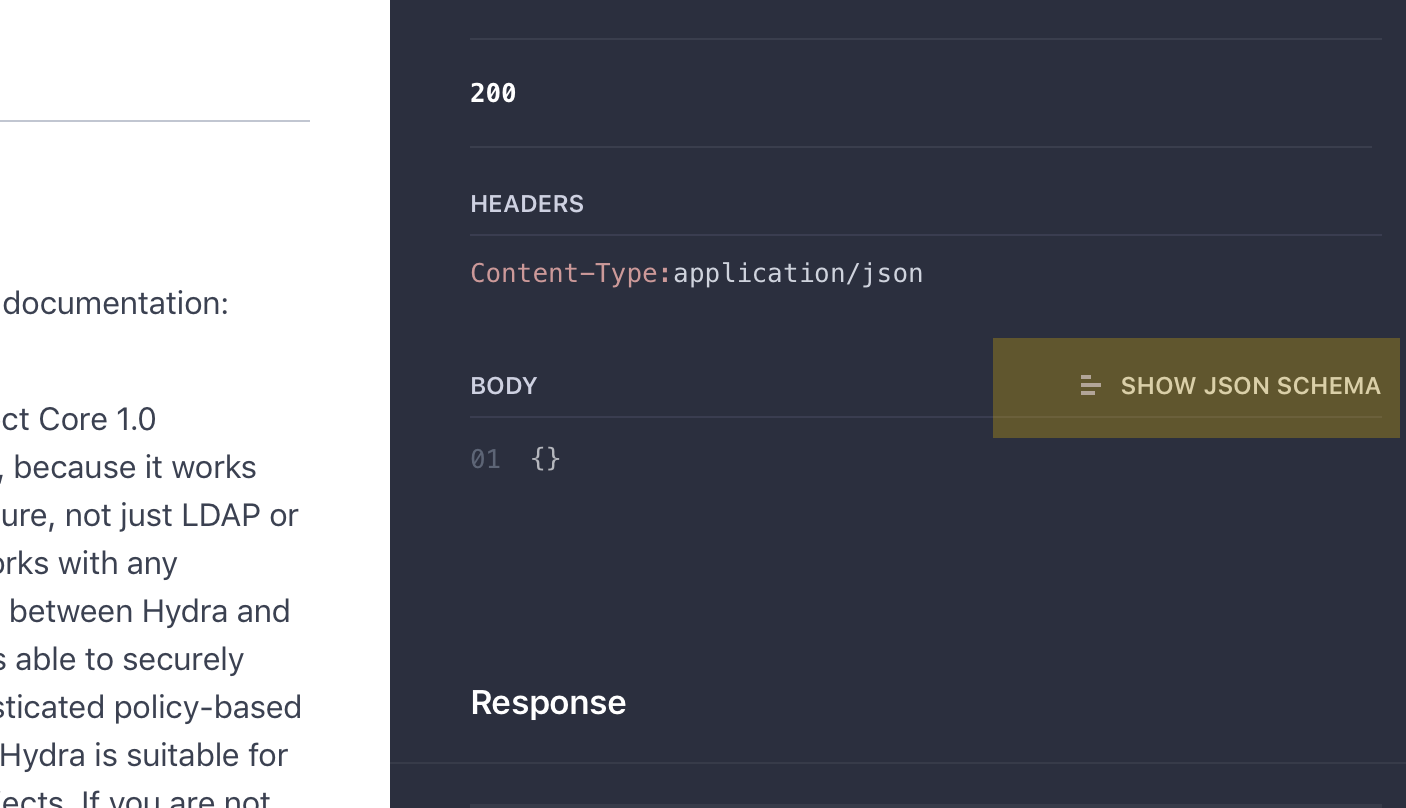 The API documentation always refers to the latest tagged version of ORY Hydra. For previous API documentations, please refer to https://github.com/ory/hydra/blob//docs/api.swagger.yaml - for example: 0.9.13: https://github.com/ory/hydra/blob/v0.9.13/docs/api.swagger.yaml 0.8.1: https://github.com/ory/hydra/blob/v0.8.1/docs/api.swagger.yaml
+ *
+ * OpenAPI spec version: Latest
+ * Contact: hi@ory.am
+ *
+ * NOTE: This class is auto generated by the swagger code generator program.
+ * https://github.com/swagger-api/swagger-codegen.git
+ *
+ * Swagger Codegen version: 2.2.3
+ *
+ * Do not edit the class manually.
+ *
+ */
+
+(function(root, factory) {
+ if (typeof define === 'function' && define.amd) {
+ // AMD.
+ define(['expect.js', '../../src/index'], factory);
+ } else if (typeof module === 'object' && module.exports) {
+ // CommonJS-like environments that support module.exports, like Node.
+ factory(require('expect.js'), require('../../src/index'));
+ } else {
+ // Browser globals (root is window)
+ factory(root.expect, root.HydraOAuth2OpenIdConnectServer);
+ }
+}(this, function(expect, HydraOAuth2OpenIdConnectServer) {
+ 'use strict';
+
+ var instance;
+
+ beforeEach(function() {
+ instance = new HydraOAuth2OpenIdConnectServer.Writer();
+ });
+
+ var getProperty = function(object, getter, property) {
+ // Use getter method if present; otherwise, get the property directly.
+ if (typeof object[getter] === 'function')
+ return object[getter]();
+ else
+ return object[property];
+ }
+
+ var setProperty = function(object, setter, property, value) {
+ // Use setter method if present; otherwise, set the property directly.
+ if (typeof object[setter] === 'function')
+ object[setter](value);
+ else
+ object[property] = value;
+ }
+
+ describe('Writer', function() {
+ it('should create an instance of Writer', function() {
+ // uncomment below and update the code to test Writer
+ //var instane = new HydraOAuth2OpenIdConnectServer.Writer();
+ //expect(instance).to.be.a(HydraOAuth2OpenIdConnectServer.Writer);
+ });
+
+ });
+
+}));
diff --git a/warden/doc.go b/warden/doc.go
index 0e765f001b8..e08db9b4be9 100644
--- a/warden/doc.go
+++ b/warden/doc.go
@@ -1,42 +1,36 @@
-// Package warden decides if access requests should be allowed or denied. In a scientific taxonomy, the warden
-// is classified as a Policy Decision Point. THe warden's primary goal is to implement `github.com/ory-am/hydra/firewall.Firewall`.
-// To read up on the warden, go to:
-//
-// - https://ory-am.gitbooks.io/hydra/content/policy.html
-//
-// - http://docs.hydra13.apiary.io/#reference/warden:-access-control-for-resource-providers
-//
-// Contains source files:
-//
-// - handler.go: A HTTP handler capable of validating access tokens.
-//
-// - warden_http.go: A Go API using HTTP to validate access tokens.
-//
-// - warden_local.go: A Go API using storage managers to validate access tokens.
-//
-// - warden_test.go: Functional tests all of the above.
+// Package warden implements endpoints capable of making access control decisions based on Access Control Policies
package warden
-import "github.com/ory/hydra/firewall"
+import (
+ "github.com/ory/hydra/firewall"
+)
-// The allowed response
-// swagger:response wardenAllowedResponse
-type swaggerWardenAllowedResponse struct {
+// swagger:response wardenAccessRequestResponse
+type swaggerWardenAccessRequestResponseParameters struct {
// in: body
- Body struct {
- // Allowed is true if the request is allowed or false otherwise
- Allowed bool `json:"allowed"`
- }
+ Body swaggerWardenAccessRequestResponse
}
-// swagger:parameters wardenAllowed
-type swaggerWardenAllowedParameters struct {
+// swagger:model wardenAccessRequestResponse
+type swaggerWardenAccessRequestResponse struct {
+ // Allowed is true if the request is allowed and false otherwise.
+ Allowed bool `json:"allowed"`
+}
+
+// swagger:parameters doesWardenAllowAccessRequest
+type swaggerDoesWardenAllowAccessRequestParameters struct {
// in: body
Body firewall.AccessRequest
}
-// swagger:model wardenTokenAllowedBody
-type swaggerWardenTokenAllowedBody struct {
+// swagger:parameters doesWardenAllowTokenAccessRequest
+type swaggerDoesWardenAllowTokenAccessRequestParameters struct {
+ // in: body
+ Body swaggerWardenTokenAccessRequest
+}
+
+// swagger:model wardenTokenAccessRequest
+type swaggerWardenTokenAccessRequest struct {
// Scopes is an array of scopes that are requried.
Scopes []string `json:"scopes"`
@@ -53,20 +47,36 @@ type swaggerWardenTokenAllowedBody struct {
Context map[string]interface{} `json:"context"`
}
-// swagger:parameters wardenTokenAllowed
-type swaggerWardenTokenAllowedParameters struct {
+// swagger:response wardenTokenAccessRequestResponse
+type swaggerWardenTokenAccessRequestResponse struct {
// in: body
- Body swaggerWardenTokenAllowedBody
+ Body swaggerWardenTokenAccessRequestResponsePayload
}
-// The token allowed response
-// swagger:response wardenTokenAllowedResponse
-type swaggerWardenTokenAllowedResponse struct {
- // in: body
- Body struct {
- *firewall.Context
+// swagger:model wardenTokenAccessRequestResponsePayload
+type swaggerWardenTokenAccessRequestResponsePayload struct {
+ // Subject is the identity that authorized issuing the token, for example a user or an OAuth2 app.
+ // This is usually a uuid but you can choose a urn or some other id too.
+ Subject string `json:"sub"`
+
+ // GrantedScopes is a list of scopes that the subject authorized when asked for consent.
+ GrantedScopes []string `json:"scopes"`
+
+ // Issuer is the id of the issuer, typically an hydra instance.
+ Issuer string `json:"iss"`
+
+ // Audience is who the token was issued for. This is an OAuth2 app usually.
+ Audience string `json:"aud"`
+
+ // IssuedAt is the token creation time stamp.
+ IssuedAt string `json:"iat"`
+
+ // ExpiresAt is the expiry timestamp.
+ ExpiresAt string `json:"exp"`
+
+ // Extra represents arbitrary session data.
+ Extra map[string]interface{} `json:"ext"`
- // Allowed is true if the request is allowed or false otherwise
- Allowed bool `json:"allowed"`
- }
+ // Allowed is true if the request is allowed and false otherwise.
+ Allowed bool `json:"allowed"`
}
diff --git a/warden/group/doc.go b/warden/group/doc.go
index 930c154e169..6758ed73a66 100644
--- a/warden/group/doc.go
+++ b/warden/group/doc.go
@@ -1,32 +1,42 @@
// Package group offers capabilities for grouping subjects together, making policy management easier.
+//
+// This endpoint is **experimental**, use it at your own risk.
package group
// A list of groups the member is belonging to
// swagger:response findGroupsByMemberResponse
type swaggerFindGroupsByMemberResponse struct {
// in: body
- Body []string
+ // type: array
+ Body []Group
}
// swagger:parameters findGroupsByMember
type swaggerFindGroupsByMemberParameters struct {
// The id of the member to look up.
// in: query
- Member int `json:"member"`
+ // required: true
+ Member string `json:"member"`
+}
+
+// swagger:parameters createGroup
+type swaggerCreateGroupParameters struct {
+ // in: body
+ Body Group
}
// swagger:parameters getGroup deleteGroup
type swaggerGetGroupParameters struct {
// The id of the group to look up.
// in: path
- ID int `json:"id"`
+ ID string `json:"id"`
}
// swagger:parameters removeMembersFromGroup addMembersToGroup
type swaggerModifyMembersParameters struct {
// The id of the group to modify.
// in: path
- ID int `json:"id"`
+ ID string `json:"id"`
// in: body
Body membersRequest
diff --git a/warden/group/handler.go b/warden/group/handler.go
index cb28cb7f8be..d2f2e6d7d92 100644
--- a/warden/group/handler.go
+++ b/warden/group/handler.go
@@ -11,6 +11,7 @@ import (
"github.com/pkg/errors"
)
+// swagger:model groupMembers
type membersRequest struct {
Members []string `json:"members"`
}
@@ -40,16 +41,16 @@ func (h *Handler) SetRoutes(r *httprouter.Router) {
r.DELETE(GroupsHandlerPath+"/:id/members", h.RemoveGroupMembers)
}
-// swagger:route GET /warden/groups warden groups findGroupsByMember
+// swagger:route GET /warden/groups warden findGroupsByMember
//
-// Find group IDs by member
+// Find groups by member
//
// The subject making the request needs to be assigned to a policy containing:
//
// ```
// {
-// "resources": ["rn:hydra:warden:groups:"],
-// "actions": ["get"],
+// "resources": ["rn:hydra:warden:groups"],
+// "actions": ["list"],
// "effect": "allow"
// }
// ```
@@ -74,24 +75,24 @@ func (h *Handler) FindGroupNames(w http.ResponseWriter, r *http.Request, _ httpr
var ctx = r.Context()
var member = r.URL.Query().Get("member")
- g, err := h.Manager.FindGroupNames(member)
- if err != nil {
+ if _, err := h.W.TokenAllowed(ctx, h.W.TokenFromRequest(r), &firewall.TokenAccessRequest{
+ Resource: GroupsResource,
+ Action: "list",
+ }, Scope); err != nil {
h.H.WriteError(w, r, err)
return
}
- if _, err := h.W.TokenAllowed(ctx, h.W.TokenFromRequest(r), &firewall.TokenAccessRequest{
- Resource: fmt.Sprintf(GroupResource, member),
- Action: "get",
- }, Scope); err != nil {
+ groups, err := h.Manager.FindGroupsByMember(member)
+ if err != nil {
h.H.WriteError(w, r, err)
return
}
- h.H.Write(w, r, g)
+ h.H.Write(w, r, groups)
}
-// swagger:route POST /warden/groups warden groups createGroup
+// swagger:route POST /warden/groups warden createGroup
//
// Create a group
//
@@ -146,7 +147,7 @@ func (h *Handler) CreateGroup(w http.ResponseWriter, r *http.Request, _ httprout
h.H.WriteCreated(w, r, GroupsHandlerPath+"/"+g.ID, &g)
}
-// swagger:route GET /warden/groups/{id} warden groups getGroup
+// swagger:route GET /warden/groups/{id} warden getGroup
//
// Get a group by id
//
@@ -197,7 +198,7 @@ func (h *Handler) GetGroup(w http.ResponseWriter, r *http.Request, ps httprouter
h.H.Write(w, r, g)
}
-// swagger:route DELETE /warden/groups/{id} warden groups deleteGroup
+// swagger:route DELETE /warden/groups/{id} warden deleteGroup
//
// Delete a group by id
//
@@ -247,7 +248,7 @@ func (h *Handler) DeleteGroup(w http.ResponseWriter, r *http.Request, ps httprou
w.WriteHeader(http.StatusNoContent)
}
-// swagger:route POST /warden/groups/{id}/members warden groups addMembersToGroup
+// swagger:route POST /warden/groups/{id}/members warden addMembersToGroup
//
// Add members to a group
//
@@ -303,7 +304,7 @@ func (h *Handler) AddGroupMembers(w http.ResponseWriter, r *http.Request, ps htt
w.WriteHeader(http.StatusNoContent)
}
-// swagger:route DELETE /warden/groups/{id}/members warden groups removeMembersFromGroup
+// swagger:route DELETE /warden/groups/{id}/members warden removeMembersFromGroup
//
// Remove members from a group
//
diff --git a/warden/group/manager.go b/warden/group/manager.go
index 6f20a50f6d6..593dfd042f6 100644
--- a/warden/group/manager.go
+++ b/warden/group/manager.go
@@ -19,5 +19,5 @@ type Manager interface {
AddGroupMembers(group string, members []string) error
RemoveGroupMembers(group string, members []string) error
- FindGroupNames(subject string) ([]string, error)
+ FindGroupsByMember(subject string) ([]Group, error)
}
diff --git a/warden/group/manager_http.go b/warden/group/manager_http.go
deleted file mode 100644
index 64f8d68ac4b..00000000000
--- a/warden/group/manager_http.go
+++ /dev/null
@@ -1,105 +0,0 @@
-package group
-
-import (
- "net/http"
- "net/url"
-
- "bytes"
- "encoding/json"
- "io/ioutil"
-
- "github.com/ory/hydra/pkg"
- "github.com/pkg/errors"
-)
-
-type HTTPManager struct {
- Client *http.Client
- Endpoint *url.URL
- FakeTLSTermination bool
- Dry bool
-}
-
-func (m *HTTPManager) CreateGroup(g *Group) error {
- var r = pkg.NewSuperAgent(m.Endpoint.String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Create(g)
-}
-
-func (m *HTTPManager) GetGroup(id string) (*Group, error) {
- var g Group
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- if err := r.Get(&g); err != nil {
- return nil, err
- }
-
- return &g, nil
-}
-
-func (m *HTTPManager) DeleteGroup(id string) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, id).String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Delete()
-}
-
-func (m *HTTPManager) AddGroupMembers(group string, members []string) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, group, "members").String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- return r.Create(&membersRequest{
- Members: members,
- })
-}
-
-func (m *HTTPManager) RemoveGroupMembers(group string, members []string) error {
- var r = pkg.NewSuperAgent(pkg.JoinURL(m.Endpoint, group, "members").String())
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- send, err := json.Marshal(&membersRequest{Members: members})
- if err != nil {
- return errors.WithStack(err)
- }
-
- req, err := http.NewRequest("DELETE", r.URL, bytes.NewReader(send))
- if err != nil {
- return errors.WithStack(err)
- }
-
- if err := r.DoDry(req); err != nil {
- return err
- }
-
- resp, err := r.Client.Do(req)
- if err != nil {
- return errors.WithStack(err)
- }
- defer resp.Body.Close()
-
- if resp.StatusCode != http.StatusNoContent {
- body, _ := ioutil.ReadAll(resp.Body)
- return errors.Errorf("Expected status code %d, got %d.\n%s\n", http.StatusNoContent, resp.StatusCode, body)
- }
-
- return nil
-}
-
-func (m *HTTPManager) FindGroupNames(subject string) ([]string, error) {
- var g []string
- var r = pkg.NewSuperAgent(m.Endpoint.String() + "?member=" + subject)
- r.Client = m.Client
- r.Dry = m.Dry
- r.FakeTLSTermination = m.FakeTLSTermination
- if err := r.Get(&g); err != nil {
- return nil, err
- }
-
- return g, nil
-}
diff --git a/warden/group/manager_memory.go b/warden/group/manager_memory.go
index 3320732fd58..d5bae4b2c83 100644
--- a/warden/group/manager_memory.go
+++ b/warden/group/manager_memory.go
@@ -3,6 +3,7 @@ package group
import (
"sync"
+ "github.com/ory/hydra/pkg"
"github.com/pborman/uuid"
"github.com/pkg/errors"
)
@@ -32,7 +33,7 @@ func (m *MemoryManager) CreateGroup(g *Group) error {
func (m *MemoryManager) GetGroup(id string) (*Group, error) {
if g, ok := m.Groups[id]; !ok {
- return nil, errors.New("not found")
+ return nil, errors.WithStack(pkg.ErrNotFound)
} else {
return &g, nil
}
@@ -76,16 +77,16 @@ func (m *MemoryManager) RemoveGroupMembers(group string, subjects []string) erro
return m.CreateGroup(g)
}
-func (m *MemoryManager) FindGroupNames(subject string) ([]string, error) {
+func (m *MemoryManager) FindGroupsByMember(subject string) ([]Group, error) {
if m.Groups == nil {
m.Groups = map[string]Group{}
}
- var res []string
+ var res = []Group{}
for _, g := range m.Groups {
for _, s := range g.Members {
if s == subject {
- res = append(res, g.ID)
+ res = append(res, g)
break
}
}
diff --git a/warden/group/manager_sql.go b/warden/group/manager_sql.go
index 76fc04d5be7..86907ddf3ad 100644
--- a/warden/group/manager_sql.go
+++ b/warden/group/manager_sql.go
@@ -1,7 +1,10 @@
package group
import (
+ "database/sql"
+
"github.com/jmoiron/sqlx"
+ "github.com/ory/hydra/pkg"
"github.com/pborman/uuid"
"github.com/pkg/errors"
"github.com/rubenv/sql-migrate"
@@ -31,9 +34,9 @@ type SQLManager struct {
DB *sqlx.DB
}
-func (s *SQLManager) CreateSchemas() (int, error) {
+func (m *SQLManager) CreateSchemas() (int, error) {
migrate.SetTable("hydra_groups_migration")
- n, err := migrate.Exec(s.DB.DB, s.DB.DriverName(), migrations, migrate.Up)
+ n, err := migrate.Exec(m.DB.DB, m.DB.DriverName(), migrations, migrate.Up)
if err != nil {
return 0, errors.Wrapf(err, "Could not migrate sql schema, applied %d migrations", n)
}
@@ -59,7 +62,9 @@ func (m *SQLManager) GetGroup(id string) (*Group, error) {
}
var q []string
- if err := m.DB.Select(&q, m.DB.Rebind("SELECT member from hydra_warden_group_member WHERE group_id = ?"), found); err != nil {
+ if err := m.DB.Select(&q, m.DB.Rebind("SELECT member from hydra_warden_group_member WHERE group_id = ?"), found); err == sql.ErrNoRows {
+ return nil, errors.WithStack(pkg.ErrNotFound)
+ } else if err != nil {
return nil, errors.WithStack(err)
}
@@ -123,11 +128,23 @@ func (m *SQLManager) RemoveGroupMembers(group string, subjects []string) error {
return nil
}
-func (m *SQLManager) FindGroupNames(subject string) ([]string, error) {
- var q []string
- if err := m.DB.Select(&q, m.DB.Rebind("SELECT group_id from hydra_warden_group_member WHERE member = ? GROUP BY group_id"), subject); err != nil {
+func (m *SQLManager) FindGroupsByMember(subject string) ([]Group, error) {
+ var ids []string
+ if err := m.DB.Select(&ids, m.DB.Rebind("SELECT group_id from hydra_warden_group_member WHERE member = ? GROUP BY group_id"), subject); err == sql.ErrNoRows {
+ return nil, errors.WithStack(pkg.ErrNotFound)
+ } else if err != nil {
return nil, errors.WithStack(err)
}
- return q, nil
+ var groups = make([]Group, len(ids))
+ for k, id := range ids {
+ group, err := m.GetGroup(id)
+ if err != nil {
+ return nil, errors.WithStack(err)
+ }
+
+ groups[k] = *group
+ }
+
+ return groups, nil
}
diff --git a/warden/group/manager_test.go b/warden/group/manager_test.go
index 25513a8714a..01af2da2d99 100644
--- a/warden/group/manager_test.go
+++ b/warden/group/manager_test.go
@@ -4,54 +4,18 @@ import (
"flag"
"fmt"
"log"
- "net/http/httptest"
- "net/url"
"os"
"testing"
- "github.com/julienschmidt/httprouter"
_ "github.com/lib/pq"
- "github.com/ory/fosite"
- "github.com/ory/herodot"
- "github.com/ory/hydra/compose"
"github.com/ory/hydra/integration"
. "github.com/ory/hydra/warden/group"
- "github.com/ory/ladon"
)
-var clientManagers = map[string]Manager{}
-var ts *httptest.Server
-
-func init() {
- clientManagers["memory"] = &MemoryManager{
+var clientManagers = map[string]Manager{
+ "memory": &MemoryManager{
Groups: map[string]Group{},
- }
-
- localWarden, httpClient := compose.NewMockFirewall("foo", "alice", fosite.Arguments{Scope}, &ladon.DefaultPolicy{
- ID: "1",
- Subjects: []string{"alice"},
- Resources: []string{"rn:hydra:warden<.*>"},
- Actions: []string{"create", "get", "delete", "update", "members.add", "members.remove"},
- Effect: ladon.AllowAccess,
- })
-
- s := &Handler{
- Manager: &MemoryManager{
- Groups: map[string]Group{},
- },
- H: herodot.NewJSONWriter(nil),
- W: localWarden,
- }
-
- routing := httprouter.New()
- s.SetRoutes(routing)
- ts = httptest.NewServer(routing)
-
- u, _ := url.Parse(ts.URL + GroupsHandlerPath)
- clientManagers["http"] = &HTTPManager{
- Client: httpClient,
- Endpoint: u,
- }
+ },
}
func TestMain(m *testing.M) {
diff --git a/warden/group/manager_test_helper.go b/warden/group/manager_test_helper.go
index 99a0cee4369..98d116876cc 100644
--- a/warden/group/manager_test_helper.go
+++ b/warden/group/manager_test_helper.go
@@ -27,18 +27,18 @@ func TestHelperManagers(m Manager) func(t *testing.T) {
assert.EqualValues(t, c.Members, d.Members)
assert.EqualValues(t, c.ID, d.ID)
- ds, err := m.FindGroupNames("foo")
+ ds, err := m.FindGroupsByMember("foo")
require.NoError(t, err)
assert.Len(t, ds, 2)
assert.NoError(t, m.AddGroupMembers("1", []string{"baz"}))
- ds, err = m.FindGroupNames("baz")
+ ds, err = m.FindGroupsByMember("baz")
require.NoError(t, err)
assert.Len(t, ds, 1)
assert.NoError(t, m.RemoveGroupMembers("1", []string{"baz"}))
- ds, err = m.FindGroupNames("baz")
+ ds, err = m.FindGroupsByMember("baz")
require.NoError(t, err)
assert.Len(t, ds, 0)
diff --git a/warden/group/sdk_test.go b/warden/group/sdk_test.go
new file mode 100644
index 00000000000..dca663071a5
--- /dev/null
+++ b/warden/group/sdk_test.go
@@ -0,0 +1,100 @@
+package group_test
+
+import (
+ "net/http/httptest"
+ "testing"
+
+ "net/http"
+
+ "github.com/julienschmidt/httprouter"
+ _ "github.com/lib/pq"
+ "github.com/ory/fosite"
+ "github.com/ory/herodot"
+ "github.com/ory/hydra/compose"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
+ . "github.com/ory/hydra/warden/group"
+ "github.com/ory/ladon"
+ "github.com/stretchr/testify/assert"
+ "github.com/stretchr/testify/require"
+)
+
+func TestGroupSDK(t *testing.T) {
+ clientManagers["memory"] = &MemoryManager{
+ Groups: map[string]Group{},
+ }
+
+ localWarden, httpClient := compose.NewMockFirewall("foo", "alice", fosite.Arguments{Scope}, &ladon.DefaultPolicy{
+ ID: "1",
+ Subjects: []string{"alice"},
+ Resources: []string{"rn:hydra:warden<.*>"},
+ Actions: []string{"list", "create", "get", "delete", "update", "members.add", "members.remove"},
+ Effect: ladon.AllowAccess,
+ })
+
+ handler := &Handler{
+ Manager: &MemoryManager{
+ Groups: map[string]Group{},
+ },
+ H: herodot.NewJSONWriter(nil),
+ W: localWarden,
+ }
+
+ router := httprouter.New()
+ handler.SetRoutes(router)
+ server := httptest.NewServer(router)
+
+ client := hydra.NewWardenApiWithBasePath(server.URL)
+ client.Configuration.Transport = httpClient.Transport
+
+ t.Run("flows", func(*testing.T) {
+ _, response, err := client.GetGroup("4321")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNotFound, response.StatusCode)
+
+ firstGroup := hydra.Group{Id: "1", Members: []string{"bar", "foo"}}
+ result, response, err := client.CreateGroup(firstGroup)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusCreated, response.StatusCode)
+ assert.EqualValues(t, firstGroup, *result)
+
+ secondGroup := hydra.Group{Id: "2", Members: []string{"foo"}}
+ result, response, err = client.CreateGroup(secondGroup)
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusCreated, response.StatusCode)
+ assert.EqualValues(t, secondGroup, *result)
+
+ result, response, err = client.GetGroup("1")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
+ assert.EqualValues(t, firstGroup, *result)
+
+ results, response, err := client.FindGroupsByMember("foo")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
+ assert.Len(t, results, 2)
+
+ client.AddMembersToGroup("1", hydra.GroupMembers{Members: []string{"baz"}})
+
+ results, response, err = client.FindGroupsByMember("baz")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
+ assert.Len(t, results, 1)
+
+ response, err = client.RemoveMembersFromGroup("1", hydra.GroupMembers{Members: []string{"baz"}})
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNoContent, response.StatusCode)
+
+ results, response, err = client.FindGroupsByMember("baz")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusOK, response.StatusCode)
+ assert.Len(t, results, 0)
+
+ response, err = client.DeleteGroup("1")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNoContent, response.StatusCode)
+
+ _, response, err = client.GetGroup("4321")
+ require.NoError(t, err)
+ assert.Equal(t, http.StatusNotFound, response.StatusCode)
+ })
+}
diff --git a/warden/handler.go b/warden/handler.go
index 34ef18f3d0e..cd121fa3da2 100644
--- a/warden/handler.go
+++ b/warden/handler.go
@@ -60,13 +60,14 @@ func (h *WardenHandler) SetRoutes(r *httprouter.Router) {
r.POST(AllowedHandlerPath, h.Allowed)
}
-// swagger:route POST /warden/allowed warden wardenAllowed
+// swagger:route POST /warden/allowed warden doesWardenAllowAccessRequest
//
-// Check if a subject is allowed to do something
+// Check if an access request is valid (without providing an access token)
+//
+// Checks if a subject (typically a user or a service) is allowed to perform an action on a resource. This endpoint requires a subject,
+// a resource name, an action name and a context. If the subject is not allowed to perform the action on the resource,
+// this endpoint returns a 200 response with `{ "allowed": false}`, otherwise `{ "allowed": true }` is returned.
//
-// Checks if an arbitrary subject is allowed to perform an action on a resource. This endpoint requires a subject,
-// a resource name, an action name and a context.If the subject is not allowed to perform the action on the resource,
-// this endpoint returns a 200 response with `{ "allowed": false} }`.
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -90,7 +91,7 @@ func (h *WardenHandler) SetRoutes(r *httprouter.Router) {
// oauth2: hydra.warden
//
// Responses:
-// 200: wardenAllowedResponse
+// 200: wardenAccessRequestResponse
// 401: genericError
// 403: genericError
// 500: genericError
@@ -121,18 +122,20 @@ func (h *WardenHandler) Allowed(w http.ResponseWriter, r *http.Request, _ httpro
h.H.Write(w, r, &res)
}
-// swagger:route POST /warden/token/allowed warden wardenTokenAllowed
+// swagger:route POST /warden/token/allowed warden doesWardenAllowTokenAccessRequest
//
-// Check if the subject of a token is allowed to do something
+// Check if an access request is valid (providing an access token)
//
-// Checks if a token is valid and if the token owner is allowed to perform an action on a resource.
+// Checks if a token is valid and if the token subject is allowed to perform an action on a resource.
// This endpoint requires a token, a scope, a resource name, an action name and a context.
//
+//
// If a token is expired/invalid, has not been granted the requested scope or the subject is not allowed to
-// perform the action on the resource, this endpoint returns a 200 response with `{ "allowed": false} }`.
+// perform the action on the resource, this endpoint returns a 200 response with `{ "allowed": false}`.
+//
+//
+// Extra data set through the `accessTokenExtra` field in the consent flow will be included in the response.
//
-// Extra data set through the `at_ext` claim in the consent response will be included in the response.
-// The `id_ext` claim will never be returned by this endpoint.
//
// The subject making the request needs to be assigned to a policy containing:
//
@@ -156,7 +159,7 @@ func (h *WardenHandler) Allowed(w http.ResponseWriter, r *http.Request, _ httpro
// oauth2: hydra.warden
//
// Responses:
-// 200: wardenTokenAllowedResponse
+// 200: wardenTokenAccessRequestResponse
// 401: genericError
// 403: genericError
// 500: genericError
diff --git a/warden/helper_test.go b/warden/helper_test.go
new file mode 100644
index 00000000000..fda0389c7ce
--- /dev/null
+++ b/warden/helper_test.go
@@ -0,0 +1,230 @@
+package warden_test
+
+import (
+ "testing"
+ "time"
+
+ "os"
+
+ "github.com/ory/fosite"
+ "github.com/ory/fosite/storage"
+ "github.com/ory/hydra/firewall"
+ "github.com/ory/hydra/oauth2"
+ "github.com/ory/hydra/pkg"
+ "github.com/ory/hydra/warden"
+ "github.com/ory/hydra/warden/group"
+ "github.com/ory/ladon"
+ "github.com/sirupsen/logrus"
+ "github.com/stretchr/testify/assert"
+)
+
+var (
+ accessRequestTestCases = []struct {
+ req *firewall.AccessRequest
+ expectErr bool
+ assert func(*firewall.Context)
+ }{
+ {
+ req: &firewall.AccessRequest{
+ Subject: "alice",
+ Resource: "other-thing",
+ Action: "create",
+ Context: ladon.Context{},
+ },
+ expectErr: true,
+ },
+ {
+ req: &firewall.AccessRequest{
+ Subject: "alice",
+ Resource: "matrix",
+ Action: "delete",
+ Context: ladon.Context{},
+ },
+ expectErr: true,
+ },
+ {
+ req: &firewall.AccessRequest{
+ Subject: "alice",
+ Resource: "matrix",
+ Action: "create",
+ Context: ladon.Context{},
+ },
+ expectErr: false,
+ },
+ }
+ wardens = map[string]firewall.Firewall{}
+ ladonWarden = pkg.LadonWarden(map[string]ladon.Policy{
+ "1": &ladon.DefaultPolicy{
+ ID: "1",
+ Subjects: []string{"alice", "group1"},
+ Resources: []string{"matrix", "forbidden_matrix", "rn:hydra:token<.*>"},
+ Actions: []string{"create", "decide"},
+ Effect: ladon.AllowAccess,
+ },
+ "2": &ladon.DefaultPolicy{
+ ID: "2",
+ Subjects: []string{"siri"},
+ Resources: []string{"<.*>"},
+ Actions: []string{"decide"},
+ Effect: ladon.AllowAccess,
+ },
+ "3": &ladon.DefaultPolicy{
+ ID: "3",
+ Subjects: []string{"group1"},
+ Resources: []string{"forbidden_matrix", "rn:hydra:token<.*>"},
+ Actions: []string{"create", "decide"},
+ Effect: ladon.DenyAccess,
+ },
+ })
+ fositeStore = pkg.FositeStore()
+ now = time.Now().Round(time.Second)
+ tokens = pkg.Tokens(4)
+ accessRequestTokenTestCases = []struct {
+ token string
+ req *firewall.TokenAccessRequest
+ scopes []string
+ expectErr bool
+ assert func(*testing.T, *firewall.Context)
+ }{
+ {
+ token: "invalid",
+ req: &firewall.TokenAccessRequest{},
+ scopes: []string{},
+ expectErr: true,
+ },
+ {
+ token: tokens[0][1],
+ req: &firewall.TokenAccessRequest{},
+ scopes: []string{"core"},
+ expectErr: true,
+ },
+ {
+ token: tokens[0][1],
+ req: &firewall.TokenAccessRequest{},
+ scopes: []string{"foo"},
+ expectErr: true,
+ },
+ {
+ token: tokens[0][1],
+ req: &firewall.TokenAccessRequest{
+ Resource: "matrix",
+ Action: "create",
+ Context: ladon.Context{},
+ },
+ scopes: []string{"foo"},
+ expectErr: true,
+ },
+ {
+ token: tokens[0][1],
+ req: &firewall.TokenAccessRequest{
+ Resource: "matrix",
+ Action: "delete",
+ Context: ladon.Context{},
+ },
+ scopes: []string{"core"},
+ expectErr: true,
+ },
+ {
+ token: tokens[0][1],
+ req: &firewall.TokenAccessRequest{
+ Resource: "matrix",
+ Action: "create",
+ Context: ladon.Context{},
+ },
+ scopes: []string{"illegal"},
+ expectErr: true,
+ },
+ {
+ token: tokens[0][1],
+ req: &firewall.TokenAccessRequest{
+ Resource: "matrix",
+ Action: "create",
+ Context: ladon.Context{},
+ },
+ scopes: []string{"core"},
+ expectErr: false,
+ assert: func(t *testing.T, c *firewall.Context) {
+ assert.Equal(t, "siri", c.Audience)
+ assert.Equal(t, "alice", c.Subject)
+ assert.Equal(t, "tests", c.Issuer)
+ assert.Equal(t, now.Add(time.Hour).Unix(), c.ExpiresAt.Unix())
+ assert.Equal(t, now.Unix(), c.IssuedAt.Unix())
+ },
+ },
+ {
+ token: tokens[3][1],
+ req: &firewall.TokenAccessRequest{
+ Resource: "forbidden_matrix",
+ Action: "create",
+ Context: ladon.Context{},
+ },
+ scopes: []string{"core"},
+ expectErr: true,
+ },
+ {
+ token: tokens[3][1],
+ req: &firewall.TokenAccessRequest{
+ Resource: "matrix",
+ Action: "create",
+ Context: ladon.Context{},
+ },
+ scopes: []string{"core"},
+ expectErr: false,
+ assert: func(t *testing.T, c *firewall.Context) {
+ assert.Equal(t, "siri", c.Audience)
+ assert.Equal(t, "ken", c.Subject)
+ assert.Equal(t, "tests", c.Issuer)
+ assert.Equal(t, now.Add(time.Hour).Unix(), c.ExpiresAt.Unix())
+ assert.Equal(t, now.Unix(), c.IssuedAt.Unix())
+ },
+ },
+ }
+)
+
+func createAccessTokenSession(subject, client string, token string, expiresAt time.Time, fs *storage.MemoryStore, scopes fosite.Arguments) {
+ ar := fosite.NewAccessRequest(oauth2.NewSession(subject))
+ ar.GrantedScopes = fosite.Arguments{"core"}
+ if scopes != nil {
+ ar.GrantedScopes = scopes
+ }
+ ar.RequestedAt = time.Now().Round(time.Second)
+ ar.Client = &fosite.DefaultClient{ID: client}
+ ar.Session.SetExpiresAt(fosite.AccessToken, expiresAt)
+ ar.Session.(*oauth2.Session).Extra = map[string]interface{}{"foo": "bar"}
+ fs.CreateAccessTokenSession(nil, token, ar)
+}
+
+func TestMain(m *testing.M) {
+ wardens["local"] = &warden.LocalWarden{
+ Warden: ladonWarden,
+ L: logrus.New(),
+ OAuth2: &fosite.Fosite{
+ Store: fositeStore,
+ TokenIntrospectionHandlers: fosite.TokenIntrospectionHandlers{
+ &warden.TokenValidator{
+ CoreStrategy: pkg.HMACStrategy,
+ CoreStorage: fositeStore,
+ ScopeStrategy: fosite.HierarchicScopeStrategy,
+ },
+ },
+ ScopeStrategy: fosite.HierarchicScopeStrategy,
+ },
+ Groups: &group.MemoryManager{
+ Groups: map[string]group.Group{
+ "group1": {
+ ID: "group1",
+ Members: []string{"ken"},
+ },
+ },
+ },
+ Issuer: "tests",
+ AccessTokenLifespan: time.Hour,
+ }
+
+ createAccessTokenSession("alice", "siri", tokens[0][0], now.Add(time.Hour), fositeStore, fosite.Arguments{"core", "hydra.warden"})
+ createAccessTokenSession("siri", "bob", tokens[1][0], now.Add(time.Hour), fositeStore, fosite.Arguments{"core", "hydra.warden"})
+ createAccessTokenSession("siri", "doesnt-exist", tokens[2][0], now.Add(-time.Hour), fositeStore, fosite.Arguments{"core", "hydra.warden"})
+ createAccessTokenSession("ken", "siri", tokens[3][0], now.Add(time.Hour), fositeStore, fosite.Arguments{"core", "hydra.warden"})
+
+ os.Exit(m.Run())
+}
diff --git a/warden/sdk_test.go b/warden/sdk_test.go
new file mode 100644
index 00000000000..aeca92d9d92
--- /dev/null
+++ b/warden/sdk_test.go
@@ -0,0 +1,98 @@
+package warden_test
+
+import (
+ "fmt"
+ "net/http"
+ "net/http/httptest"
+ "testing"
+ "time"
+
+ "log"
+
+ "github.com/julienschmidt/httprouter"
+ "github.com/ory/herodot"
+ "github.com/ory/hydra/firewall"
+ hydra "github.com/ory/hydra/sdk/go/hydra/swagger"
+ "github.com/ory/hydra/warden"
+ "github.com/stretchr/testify/assert"
+ "github.com/stretchr/testify/require"
+ coauth2 "golang.org/x/oauth2"
+)
+
+func TestWardenSDK(t *testing.T) {
+ router := httprouter.New()
+ handler := &warden.WardenHandler{
+ H: herodot.NewJSONWriter(nil),
+ Warden: wardens["local"],
+ }
+ handler.SetRoutes(router)
+ server := httptest.NewServer(router)
+
+ conf := &coauth2.Config{
+ Scopes: []string{},
+ Endpoint: coauth2.Endpoint{},
+ }
+
+ client := hydra.NewWardenApiWithBasePath(server.URL)
+ client.Configuration.Transport = conf.Client(coauth2.NoContext, &coauth2.Token{
+ AccessToken: tokens[1][1],
+ Expiry: time.Now().Add(time.Hour),
+ TokenType: "bearer",
+ }).Transport
+
+ t.Run("DoesWardenAllowAccessRequest", func(t *testing.T) {
+ for k, c := range accessRequestTestCases {
+ t.Run(fmt.Sprintf("case=%d", k), func(t *testing.T) {
+ result, response, err := client.DoesWardenAllowAccessRequest(hydra.WardenAccessRequest{
+ Action: c.req.Action,
+ Resource: c.req.Resource,
+ Subject: c.req.Subject,
+ Context: c.req.Context,
+ })
+
+ require.NoError(t, err)
+ require.Equal(t, http.StatusOK, response.StatusCode)
+ assert.Equal(t, !c.expectErr, result.Allowed)
+ })
+ }
+ })
+
+ t.Run("DoesWardenAllowAccessRequest", func(t *testing.T) {
+ for k, c := range accessRequestTokenTestCases {
+ t.Run(fmt.Sprintf("case=%d", k), func(t *testing.T) {
+ result, response, err := client.DoesWardenAllowTokenAccessRequest(hydra.WardenTokenAccessRequest{
+ Action: c.req.Action,
+ Resource: c.req.Resource,
+ Token: c.token,
+ Scopes: c.scopes,
+ Context: c.req.Context,
+ })
+
+ require.NoError(t, err)
+ require.Equal(t, http.StatusOK, response.StatusCode)
+ assert.Equal(t, !c.expectErr, result.Allowed)
+
+ if err == nil && c.assert != nil {
+ c.assert(t, &firewall.Context{
+ Subject: result.Sub,
+ GrantedScopes: result.Scopes,
+ Issuer: result.Iss,
+ Audience: result.Aud,
+ Extra: result.Ext,
+ ExpiresAt: mustParseTime(result.Exp),
+ IssuedAt: mustParseTime(result.Iat),
+ })
+ }
+ })
+ }
+ })
+}
+
+func mustParseTime(t string) time.Time {
+ result, err := time.Parse(time.RFC3339Nano, t)
+ if err != nil {
+ log.Fatalf("Could not parse date time %s because %s", t, err)
+ return time.Time{}
+ }
+ return result
+}
diff --git a/warden/warden_local.go b/warden/warden_local.go
index 985027013b7..b5c569a638b 100644
--- a/warden/warden_local.go
+++ b/warden/warden_local.go
@@ -91,7 +91,7 @@ func (w *LocalWarden) TokenAllowed(ctx context.Context, token string, a *firewal
}
func (w *LocalWarden) isAllowed(ctx context.Context, a *ladon.Request) error {
- groups, err := w.Groups.FindGroupNames(a.Subject)
+ groups, err := w.Groups.FindGroupsByMember(a.Subject)
if err != nil {
return err
}
@@ -108,7 +108,7 @@ func (w *LocalWarden) isAllowed(ctx context.Context, a *ladon.Request) error {
errs[k+1] = w.Warden.IsAllowed(&ladon.Request{
Resource: a.Resource,
Action: a.Action,
- Subject: g,
+ Subject: g.ID,
Context: a.Context,
})
}
diff --git a/warden/warden_test.go b/warden/warden_test.go
index afbc7f2bb3f..522887fd0b4 100644
--- a/warden/warden_test.go
+++ b/warden/warden_test.go
@@ -1,250 +1,17 @@
package warden_test
import (
- "log"
- "net/http/httptest"
- "net/url"
- "testing"
- "time"
-
"context"
-
"fmt"
+ "testing"
- "github.com/julienschmidt/httprouter"
- "github.com/ory/fosite"
- "github.com/ory/herodot"
- "github.com/ory/hydra/firewall"
- "github.com/ory/hydra/oauth2"
- "github.com/ory/hydra/pkg"
- "github.com/ory/hydra/warden"
- "github.com/ory/hydra/warden/group"
- "github.com/ory/ladon"
- "github.com/sirupsen/logrus"
- "github.com/stretchr/testify/assert"
"github.com/stretchr/testify/require"
- coauth2 "golang.org/x/oauth2"
)
-var ts *httptest.Server
-
-var wardens = map[string]firewall.Firewall{}
-
-var ladonWarden = pkg.LadonWarden(map[string]ladon.Policy{
- "1": &ladon.DefaultPolicy{
- ID: "1",
- Subjects: []string{"alice", "group1"},
- Resources: []string{"matrix", "forbidden_matrix", "rn:hydra:token<.*>"},
- Actions: []string{"create", "decide"},
- Effect: ladon.AllowAccess,
- },
- "2": &ladon.DefaultPolicy{
- ID: "2",
- Subjects: []string{"siri"},
- Resources: []string{"<.*>"},
- Actions: []string{"decide"},
- Effect: ladon.AllowAccess,
- },
- "3": &ladon.DefaultPolicy{
- ID: "3",
- Subjects: []string{"group1"},
- Resources: []string{"forbidden_matrix", "rn:hydra:token<.*>"},
- Actions: []string{"create", "decide"},
- Effect: ladon.DenyAccess,
- },
-})
-
-var fositeStore = pkg.FositeStore()
-
-var now = time.Now().Round(time.Second)
-
-var tokens = pkg.Tokens(4)
-
-func init() {
- wardens["local"] = &warden.LocalWarden{
- Warden: ladonWarden,
- L: logrus.New(),
- OAuth2: &fosite.Fosite{
- Store: fositeStore,
- TokenIntrospectionHandlers: fosite.TokenIntrospectionHandlers{
- &warden.TokenValidator{
- CoreStrategy: pkg.HMACStrategy,
- CoreStorage: fositeStore,
- ScopeStrategy: fosite.HierarchicScopeStrategy,
- },
- },
- ScopeStrategy: fosite.HierarchicScopeStrategy,
- },
- Groups: &group.MemoryManager{
- Groups: map[string]group.Group{
- "group1": {
- ID: "group1",
- Members: []string{"ken"},
- },
- },
- },
- Issuer: "tests",
- AccessTokenLifespan: time.Hour,
- }
-
- r := httprouter.New()
- serv := &warden.WardenHandler{
- H: herodot.NewJSONWriter(nil),
- Warden: wardens["local"],
- }
- serv.SetRoutes(r)
- ts = httptest.NewServer(r)
-
- url, err := url.Parse(ts.URL + warden.TokenAllowedHandlerPath)
- if err != nil {
- log.Fatalf("%s", err)
- }
-
- ar := fosite.NewAccessRequest(oauth2.NewSession("alice"))
- ar.GrantedScopes = fosite.Arguments{"core", "hydra.warden"}
- ar.RequestedAt = now
- ar.Client = &fosite.DefaultClient{ID: "siri"}
- ar.Session.SetExpiresAt(fosite.AccessToken, time.Now().Add(time.Hour).Round(time.Second))
- fositeStore.CreateAccessTokenSession(nil, tokens[0][0], ar)
-
- ar2 := fosite.NewAccessRequest(oauth2.NewSession("siri"))
- ar2.GrantedScopes = fosite.Arguments{"core", "hydra.warden"}
- ar2.RequestedAt = now
- ar2.Client = &fosite.DefaultClient{ID: "bob"}
- ar2.Session.SetExpiresAt(fosite.AccessToken, time.Now().Add(time.Hour).Round(time.Second))
- fositeStore.CreateAccessTokenSession(nil, tokens[1][0], ar2)
-
- ar3 := fosite.NewAccessRequest(oauth2.NewSession("siri"))
- ar3.GrantedScopes = fosite.Arguments{"core", "hydra.warden"}
- ar3.RequestedAt = now
- ar3.Client = &fosite.DefaultClient{ID: "doesnt-exist"}
- ar3.Session.SetExpiresAt(fosite.AccessToken, time.Now().Add(-time.Hour).Round(time.Second))
- fositeStore.CreateAccessTokenSession(nil, tokens[2][0], ar3)
-
- ar4 := fosite.NewAccessRequest(oauth2.NewSession("ken"))
- ar4.GrantedScopes = fosite.Arguments{"core", "hydra.warden"}
- ar4.RequestedAt = now
- ar4.Client = &fosite.DefaultClient{ID: "siri"}
- ar4.Session.SetExpiresAt(fosite.AccessToken, time.Now().Add(time.Hour).Round(time.Second))
- fositeStore.CreateAccessTokenSession(nil, tokens[3][0], ar4)
-
- conf := &coauth2.Config{
- Scopes: []string{},
- Endpoint: coauth2.Endpoint{},
- }
- wardens["http"] = &warden.HTTPWarden{
- Endpoint: url,
- Client: conf.Client(coauth2.NoContext, &coauth2.Token{
- AccessToken: tokens[1][1],
- Expiry: time.Now().Add(time.Hour),
- TokenType: "bearer",
- }),
- }
-}
-
func TestActionAllowed(t *testing.T) {
for n, w := range wardens {
t.Run("warden="+n, func(t *testing.T) {
- for k, c := range []struct {
- token string
- req *firewall.TokenAccessRequest
- scopes []string
- expectErr bool
- assert func(*firewall.Context)
- }{
- {
- token: "invalid",
- req: &firewall.TokenAccessRequest{},
- scopes: []string{},
- expectErr: true,
- },
- {
- token: tokens[0][1],
- req: &firewall.TokenAccessRequest{},
- scopes: []string{"core"},
- expectErr: true,
- },
- {
- token: tokens[0][1],
- req: &firewall.TokenAccessRequest{},
- scopes: []string{"foo"},
- expectErr: true,
- },
- {
- token: tokens[0][1],
- req: &firewall.TokenAccessRequest{
- Resource: "matrix",
- Action: "create",
- Context: ladon.Context{},
- },
- scopes: []string{"foo"},
- expectErr: true,
- },
- {
- token: tokens[0][1],
- req: &firewall.TokenAccessRequest{
- Resource: "matrix",
- Action: "delete",
- Context: ladon.Context{},
- },
- scopes: []string{"core"},
- expectErr: true,
- },
- {
- token: tokens[0][1],
- req: &firewall.TokenAccessRequest{
- Resource: "matrix",
- Action: "create",
- Context: ladon.Context{},
- },
- scopes: []string{"illegal"},
- expectErr: true,
- },
- {
- token: tokens[0][1],
- req: &firewall.TokenAccessRequest{
- Resource: "matrix",
- Action: "create",
- Context: ladon.Context{},
- },
- scopes: []string{"core"},
- expectErr: false,
- assert: func(c *firewall.Context) {
- assert.Equal(t, "siri", c.Audience)
- assert.Equal(t, "alice", c.Subject)
- assert.Equal(t, "tests", c.Issuer)
- assert.Equal(t, now.Add(time.Hour).Unix(), c.ExpiresAt.Unix())
- assert.Equal(t, now.Unix(), c.IssuedAt.Unix())
- },
- },
- {
- token: tokens[3][1],
- req: &firewall.TokenAccessRequest{
- Resource: "forbidden_matrix",
- Action: "create",
- Context: ladon.Context{},
- },
- scopes: []string{"core"},
- expectErr: true,
- },
- {
- token: tokens[3][1],
- req: &firewall.TokenAccessRequest{
- Resource: "matrix",
- Action: "create",
- Context: ladon.Context{},
- },
- scopes: []string{"core"},
- expectErr: false,
- assert: func(c *firewall.Context) {
- assert.Equal(t, "siri", c.Audience)
- assert.Equal(t, "ken", c.Subject)
- assert.Equal(t, "tests", c.Issuer)
- assert.Equal(t, now.Add(time.Hour).Unix(), c.ExpiresAt.Unix())
- assert.Equal(t, now.Unix(), c.IssuedAt.Unix())
- },
- },
- } {
+ for k, c := range accessRequestTokenTestCases {
t.Run(fmt.Sprintf("case=%d", k), func(t *testing.T) {
ctx, err := w.TokenAllowed(context.Background(), c.token, c.req, c.scopes...)
if c.expectErr {
@@ -254,7 +21,7 @@ func TestActionAllowed(t *testing.T) {
}
if err == nil && c.assert != nil {
- c.assert(ctx)
+ c.assert(t, ctx)
}
})
}
@@ -265,39 +32,7 @@ func TestActionAllowed(t *testing.T) {
func TestAllowed(t *testing.T) {
for n, w := range wardens {
t.Run("warden="+n, func(t *testing.T) {
- for k, c := range []struct {
- req *firewall.AccessRequest
- expectErr bool
- assert func(*firewall.Context)
- }{
- {
- req: &firewall.AccessRequest{
- Subject: "alice",
- Resource: "other-thing",
- Action: "create",
- Context: ladon.Context{},
- },
- expectErr: true,
- },
- {
- req: &firewall.AccessRequest{
- Subject: "alice",
- Resource: "matrix",
- Action: "delete",
- Context: ladon.Context{},
- },
- expectErr: true,
- },
- {
- req: &firewall.AccessRequest{
- Subject: "alice",
- Resource: "matrix",
- Action: "create",
- Context: ladon.Context{},
- },
- expectErr: false,
- },
- } {
+ for k, c := range accessRequestTestCases {
t.Run(fmt.Sprintf("case=%d", k), func(t *testing.T) {
err := w.IsAllowed(context.Background(), c.req)
if c.expectErr {