-
Notifications
You must be signed in to change notification settings - Fork 126
/
Copy pathL0257_BinaryTreePaths.java
89 lines (76 loc) · 2.58 KB
/
L0257_BinaryTreePaths.java
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
import java.util.ArrayList;
import java.util.List;
/**
* https://leetcode.cn/problems/binary-tree-paths/
*
* 给你一个二叉树的根节点 root ,按 任意顺序 ,返回所有从根节点到叶子节点的路径。
*
* 叶子节点 是指没有子节点的节点。
*
* 示例 1:
* 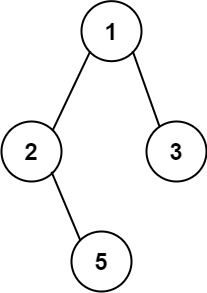
* 输入:root = [1,2,3,null,5]
* 输出:["1->2->5","1->3"]
*
* 示例 2:
* 输入:root = [1]
* 输出:["1"]
*
* 提示:
* - 树中节点的数目在范围 [1, 100] 内
* - -100 <= Node.val <= 100
*/
public class L0257_BinaryTreePaths {
public static class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode() {}
TreeNode(int val) {
this.val = val;
}
TreeNode(int val, TreeNode left, TreeNode right) {
this.val = val;
this.left = left;
this.right = right;
}
}
public List<String> binaryTreePaths(TreeNode root) {
List<String> paths = new ArrayList<>();
if (root == null) {
return paths;
}
// 如果是叶子节点,直接将节点值转换为字符串并返回
if (root.left == null && root.right == null) {
paths.add(String.valueOf(root.val));
return paths;
}
// 递归获取左子树的所有路径
List<String> leftPaths = binaryTreePaths(root.left);
for (String path : leftPaths) {
paths.add(root.val + "->" + path);
}
// 递归获取右子树的所有路径
List<String> rightPaths = binaryTreePaths(root.right);
for (String path : rightPaths) {
paths.add(root.val + "->" + path);
}
return paths;
}
public static void main(String[] args) {
L0257_BinaryTreePaths solution = new L0257_BinaryTreePaths();
// 测试用例 1
TreeNode root1 = new TreeNode(1);
root1.left = new TreeNode(2);
root1.right = new TreeNode(3);
root1.left.right = new TreeNode(5);
System.out.println("测试用例 1:");
System.out.println("输入:[1,2,3,null,5]");
System.out.println("输出:" + solution.binaryTreePaths(root1));
// 测试用例 2
TreeNode root2 = new TreeNode(1);
System.out.println("\n测试用例 2:");
System.out.println("输入:[1]");
System.out.println("输出:" + solution.binaryTreePaths(root2));
}
}